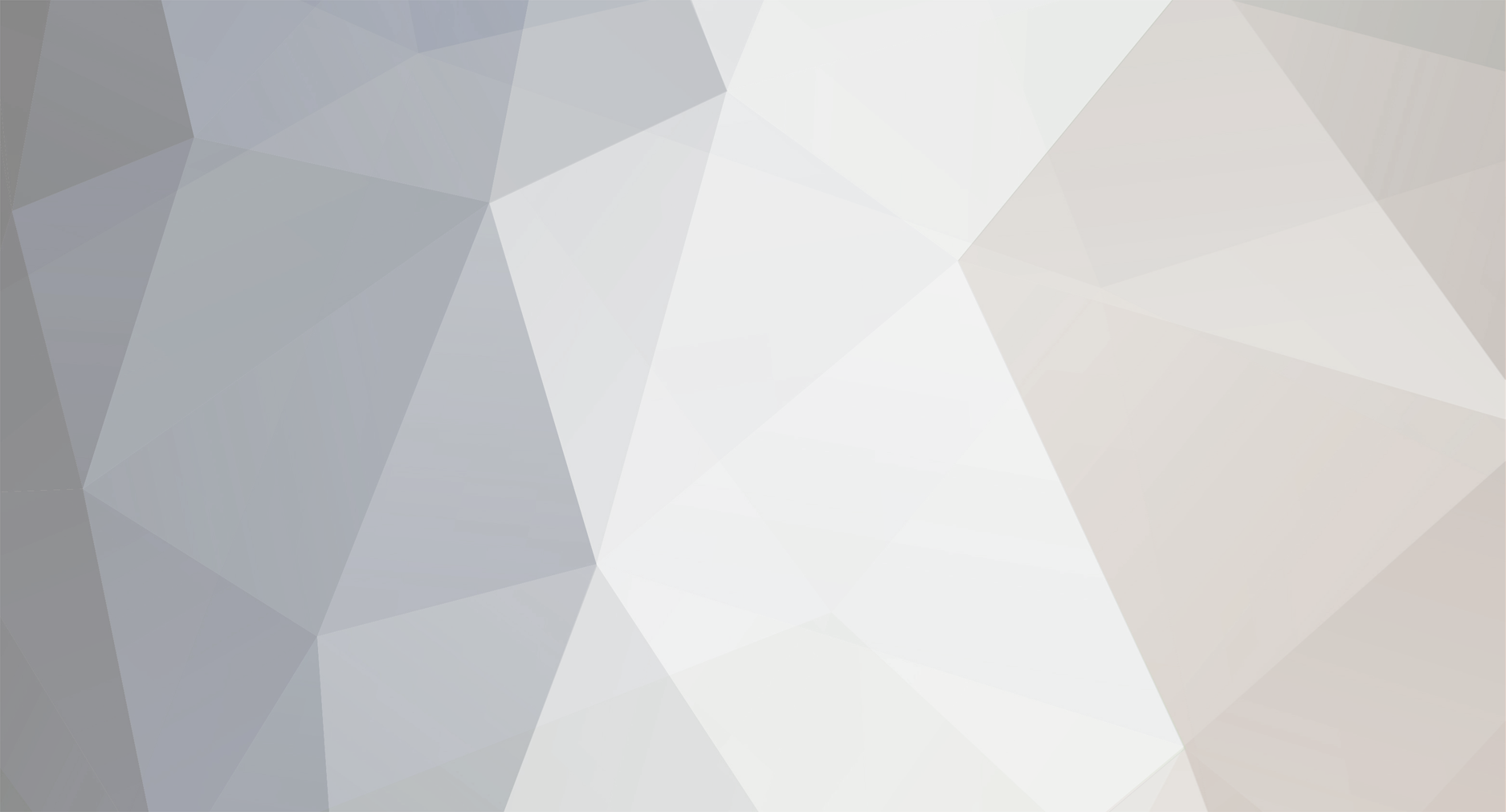
JasonLewis
-
Posts
3,347 -
Joined
-
Last visited
Posts posted by JasonLewis
-
-
This is actually a pretty popular thing to do, Googling will return a few results.
http://www.webmasterworld.com/forum91/5005.htm
http://www.codetoad.com/javascript_get_selected_text.asp
http://www.codingforums.com/archive/index.php/t-44821.html
You'd just need to tweak them a little to replace the text instead of return.
-
(http://www.spinephp.org/phpfreaks.txt)
Okay, so I've been working on this on and off for a little while now. Thought I'd post it here. It's a PHP Framework, similar to that of Cake or CodeIgniter. What I've tried to focus on is simplicity, because I found Cake to do a lot of this auto-generating code crap. Spine leaves a lot of the coding up to you, so it's more flexible.
Spine features a simple but powerful routing engine, an easy to use default templating engine, ability to extend the template engine with Smarty, helpers to make creating a dynamic website easier, sections to eliminate the rewriting of code, an easy to use MySQL database abstraction layer if PDO is unavailable and more.
Currently Spine is still in the beta testing stages, but it is currently quite stable.
You can check SpinePHP out at http://www.spinephp.org
You can get the latest version via SVN or by downloading an package from the website. Docs are still being written so it may be hard to get a hang of, but I'm writing docs when I get the chance too.
If you find any bugs, please report them at http://www.spinephp.org/bugs/
Any comments welcome.
-
-
Replace this:
if ($lower = $lower) { echo $lower; } else { echo $upper; }
With:
if (file_exists('/path/to/image/dir/' . H($mbr->getBarcodeNmbr()) . '.' . $lower)) { echo $lower; } else { echo $upper; }
Place in the correct path the where the image is stored.
-
Use an incrementing value.
Example:
$i = 0; while($row = mysql_fetch_array($get_x)){ echo $i . '<br />'; $i++; }
Just use $i in your div id, like:
echo <<<html <div id="div_unique_{$i}"> ... stuff here ... </div> html;
-
Oooh, gotcha! Clearly wasn't looking carefully enough there! Awesome, thank you both so much for the very clear explanations, sorry for being a bit slow!
Don't worry about, you're learning. I remember when I was afraid of arrays.
-
No problems Billy. I can spot a few things you should fix with your CSS.
In .AdminSuggestionsBox remove all margins, so margin is set to 0 and adjust the left and top appropriately so that it is positioned correctly. Also remove the top border as this is pushing the bottom list option down to far.
I think that was all, so that it positions correctly.
Glad to help Billy and hope its smooth sailing.
Good luck.
-
You can use filter_var for this, with the FILTER_VALIDATE_EMAIL filter. Make sure you check out the types of filters as well though.
If you want a more portable solution though, this article from Linux Journal is a good read.
-
Why not just do it in the loop.
while($row = mysql_fetch_array($get_x)){ echo <<<html <div> <a href="javascript: void(0)" onclick="javascript: jQueryDelete('{$row['id']}')">Delete Record</a> | List other stuff. </div> html; }
-
You should check to see if $_GET['page'] has been set before assigning it to file. That way you can also set a default home page.
<?php // check if it has been set if(!isset($_GET['page'])){ // no page has been set, so lets set a default $page = 'home'; }else{ // page has been set $page = $_GET['page']; } $file = $page . '.php'; // now you can check to see if the file exists, and include it if it does or show the error page if it doesn't. ?>
-
If you don't want the page to change but form data to be posted, you need to look into using Ajax.
-
If you have a tool like Firebug, development is a lot easier. You can change things on the fly and look at how code is generated.
You still haven't updated your textbox.js file to what I have posted above. There are a few things still wrong with it, such as this:
onkeyup=\"suggest(this, inival, 'admin');\"
You need to break out of the string to write inival correctly.
onkeyup=\"suggest(this, '" + inival + "', 'admin');\"
I also surrounded it by single quotes. The same thing applies to the onblur next to it.
As for why you can only click the first name, it's something wrong with your PHP I'm assuming. Only the first <li> tag is being placed inside the <ul> tags, the rest are being placed outside the closing </ul> tag. You can see this by using Firebug.
-
You're not creating your arrays correctly, if you do print_r on your $input4 you'll get an output of:
Array ( [0] => HD, HD, HD, HD, HD, hd )
As you can see, you only have 1 element in your array, this is because you haven't separated them by commas correctly.
However, if you change it too:
$input4 = array("HD", "HD", "HD", "HD", "HD", "hd");
It will output as:
Array ( [0] => HD [1] => HD [2] => HD [3] => HD [4] => HD [5] => hd )
Can you see the difference?
(I'll post this anyway.
)
-
Haven't heard of anything, you need something to do the drawing client side. You could possibly use Flash for this, or even make use of canvas, such as this. Of course you'd need a fairly up to date browser and I don't think IE in all its crapiness supports canvas yet. Try looking into a flash method.
What I can tell you is that the drawing will need to be done using JavaScript, or Flash. From there, you'd need to be able to send the drawing data to PHP and recreate it. I'm not sure exactly how you'd go about it though.
-
can you download the files and check the error as i need to get my site working as quick as possible.
No, I could but I won't. The best we can do here is help you with your coding problems, not code for you.
-
If you're trying to get it from a specific date such as in your example (2010-01-31), and not 3 months from the current date, you can use mktime.
<?php $from = '2010-10-01'; list($year, $month, $day) = explode('-', $from); $date = date('Y-m-d', mktime(0, 0, 0, $month + 3, $day, $year)); echo $date; ?>
-
No what MrAdam was saying was when you have added the item to the cart, redirect your user back to the cart page.
$_SESSION['cart'] = $cart; // Now redirect back to cart page, so they can't refresh it. header("Location: cart.php");
I'd recommend using a form to update your cart though.
-
Is your demo page up to date, I'm not getting any suggestions and it works for me if I add 6 authors then click in the 1st input box and start typing the suggestion box appears underneath the 1st text box, however I don't get any suggestions when typing Peter.
-
Yeah that's how I do it with some applications, have an environment test file. Checks the PHP version, required extensions that may be used etc.
-
Most (I say most) servers should be running PHP5 at least. When coding an application, you should take note of some of the more newer functions you use and the available versions they are on. If you are making an application that you want to run on many different environments and need to make use of a function available on a PHP version that is fairly new, then you should make use of function_exists() so that you can re-create that function.
-
Joel, now I am really confused :
The file is an .html.
This is php code inside the html:
<!-- IF not S_DISPLAY_RECENT --> <!-- INCLUDE portal/block/recent.html --> <!-- ENDIF -->
And that's not PHP code. That is 3 HTML comments.
-
Did you try using the modifier m so it accepts multiple lines.
/<object width=\"([0-9]*)\" height=\"([0-9]*)\"><param name=\"movie\" value=\"(.*)\"><\/param><param name=\"allowFullScreen\" value=\".*\"><\/param><param name=\"allowscriptaccess\" value=\".*\"><\/param><embed src=\".*\" type=\".*\" allowscriptaccess=\".*\" allowfullscreen=\".*\" width=\"[0-9]*\" height=\"[0-9]*\"><\/embed><\/object>/m
Also try the s modifier.
-
You could place this code above the image tag, then set a variable to use in the image tag.
Your if statement still makes no sense. What is stored in the database, the whole filename including the extension or just the filename? Why can't you store the extension in the database, thus removing the need for this?
-
Did you look on line 12?
You haven't escaped your quotes correctly.
<FilesMatch "\.(ico|gif|jpg|JPG|jpeg|png|PNG|swf|css|js|html?|xml|txt)$">
So PHP is thinking you're ending the string $Data.
Questions.
in Regex Help
Posted
You can validate emails with filter_var if it's available to you.