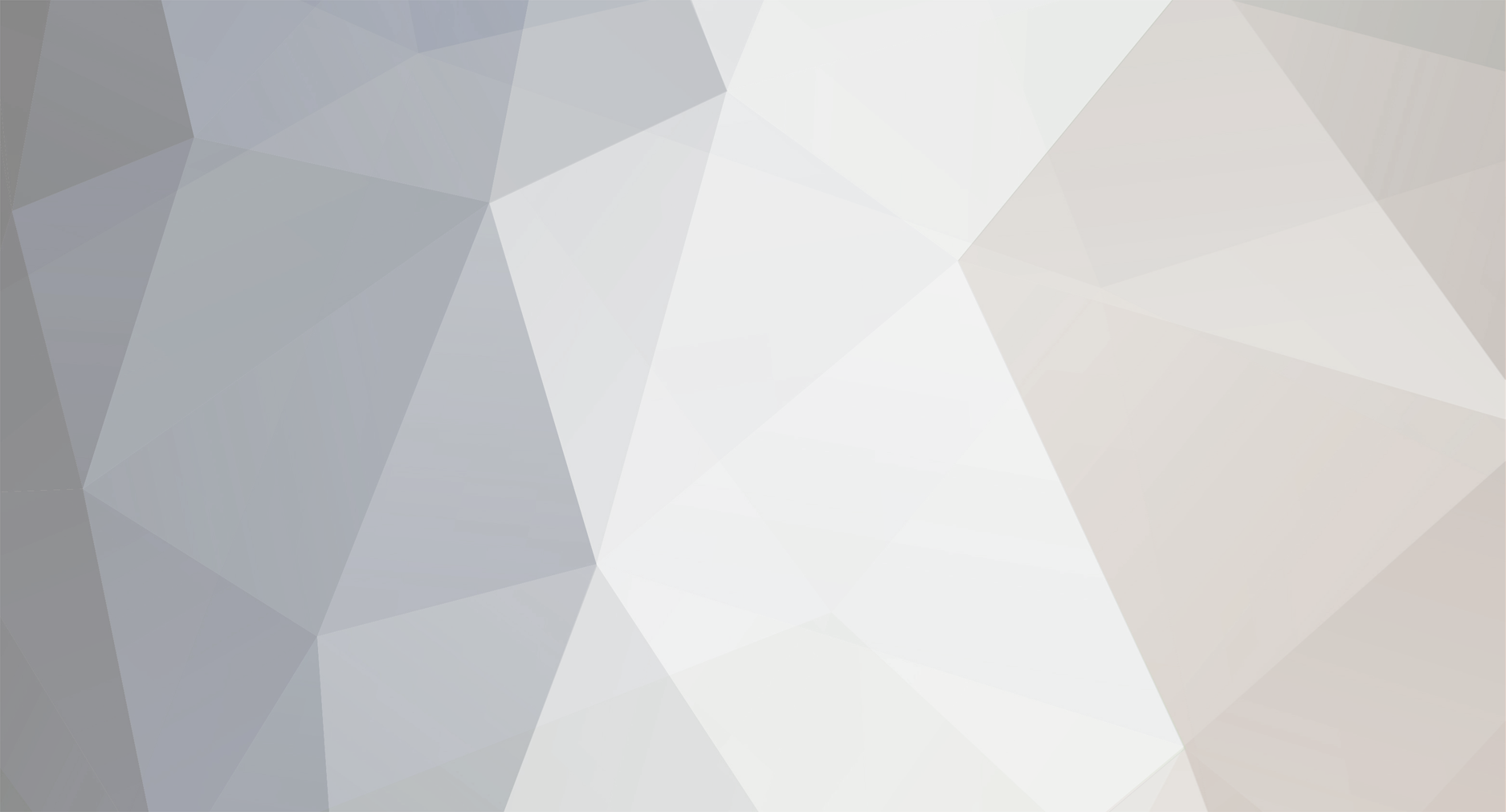
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
In this instance that may not be applicable. Let's say the OP's site is: http://www.op.com/page.php Let's say the images reside publicly in: http://www.somedomain.com/images Some of the directory functions make work already with URL locations. Or the OP might be able to say: $page = file_get_contents( 'http://www.somedomain.com/images' ); print_r( $page ); If file_get_contents() works, the OP could parse the page for the image names. The worst case scenario is opening the URL http://www.somedomain.com/images with either HttpRequest or cURL and parsing those results.
-
You may be new to technical forums in general so you may be unaware that we will not provide solutions to your homework problems. So tread carefully in how you ask for help with this type of stuff. That said, you're not asking for a solution. You're asking for general help with step 4. My question to you is, exactly what part of step 4 is confusing you? Have your written any code for step 4 yet? Can we see it? Step 4 requires that you write a function. Do you know how to write functions? It also requires your function to accept an argument. Do you know how to do that? The argument must be passed by reference. Do you know how to do that? (Kind of odd your teacher would want it by reference since PHP5 will pass arrays by reference anyways; or at least that was my understanding.) You will need to write a loop that executes 10 times. Do you know how to do that? You get the idea.
-
This is actually a PHP question. PHP has several different ways in which you can specify strings: single quotes, double quotes, HEREDOC, and one other I think. When using double-quoted or HEREDOC strings, you can use curly brackets as a shortcut to string concatenation. <?php $somevar = 'Fred'; echo 'Hello, ' . $somevar . "\n"; echo "Hello, " . $somevar . "\n"; echo "Hello, {$somevar}\n"; ?> Those should all produce the same thing.
-
Yes and yes. If you really wanted to do away with this ordering nonsense, you could add the two separate columns to the table and insert and update triggers that explode the string and populates the two new columns for you. Then when you want to order you can just order by those columns. The disadvantage is you'll add extra bytes to each table row. Or you could write a database function and order that way. I haven't used MySQL in ages so I'm assuming it supports triggers and user-defined functions.
-
DROP TABLE IF EXISTS foojunk;# MySQL returned an empty result set (i.e. zero rows). CREATE TABLE foojunk( tcol VARCHAR( 12 ) );# MySQL returned an empty result set (i.e. zero rows). INSERT INTO foojunk( tcol ) VALUES ( 'cr1.se1' ), ( 'cr2.se1' ), ( 'cr1.se2' ), ( 'cr2.se2' ), ( 'cr1.se3' ), ( 'cr2.se3' ), ( 'cr1.bo1' ), ( 'cr2.bo1' ), ( 'cr1.bo2' ), ( 'cr2.bo2' );# Affected rows: 10 SELECT * FROM ( SELECT SUBSTR( tcol, 1, dot_pos -1 ) AS front_part, SUBSTR( tcol, dot_pos +1 ) AS back_part FROM ( SELECT tcol, LOCATE( '.', tcol ) AS `dot_pos` FROM foojunk ) AS t ) AS s ORDER BY back_part ;
-
I don't see any reason to create temporary tables for this. You're using excessive resources for something that can be easily accomplished with a CASE structure. <?php // The ids to search for $ids = array( 595, 585, 159, 112, 140, 629 ); // We want results to be in the same order as the ids, so // we will assign incrementing integers to each id in the results $max = count( $ids ); $cases = array(); for( $i = 0; $i < $max; $i += 1 ) { $cases[] = "WHEN `person_id`={$ids[$i]} THEN {$i}"; } $cases[] = "ELSE {$i}"; $cases = "CASE \n" . implode( "\n", $cases ) . "\nEND CASE"; $sql = " SELECT `last_name`, `person_id`, {$cases} AS `myorder` FROM `our_people` WHERE `person_id` IN (" . implode( ', ', $ids ) . ") ORDER BY `myorder`, `last_name` "; echo $sql; ?> Outputs: SELECT `last_name`, `person_id`, CASE WHEN `person_id`=595 THEN 0 WHEN `person_id`=585 THEN 1 WHEN `person_id`=159 THEN 2 WHEN `person_id`=112 THEN 3 WHEN `person_id`=140 THEN 4 WHEN `person_id`=629 THEN 5 ELSE 6 END CASE AS `myorder` FROM `our_people` WHERE `person_id` IN (595, 585, 159, 112, 140, 629) ORDER BY `myorder`, `last_name`
-
I use regexp all the time when I need to validate formats or extract portions of a string. Although I do agree the regexp engine is probably more "heavy" than basic string operations.
-
I hate to burst your bubble, but there are 86400 seconds in most days and not in all of them.
-
In 2s complement, if the most significant bit is a 1, then the number is negative.
-
Can't you just order by the supervisor in your query? ORDER BY supervisor, user
-
It didn't have to be. I could have just as easily removed the two dots (or concatenation operators) surrounding $town If you fully understand strings and string operators, then you'll see why. http://php.he.net/manual/en/language.types.string.php http://php.he.net/manual/en/language.operators.string.php If, after reading those, you are still unsure, come back.
-
Http responses have two parts: a header and a body. You *must* finish sending all of the header information before the body. Various PHP functions will send header information, including but not limited to: session_start(), header() If you use an echo, as in echo 'hi there' and then do something that modifies header information, you will get this error. I promise you, somewhere you are sending part of the body before you finish with the header info, or you would not get this message. A common source of this problem is trailing white space in a file: <?php // image.php include( 'db.php' ); header( 'Content-Type: image/jpg' ); echo $image; ?> <?php // db.php mysql_connect( /* stuff */ ); // notice there is a trailing newline at the end of this file!!! ?>
-
function getPost($id,$category,$username = 'foo'){ //some code to execute }
-
$query = "SELECT * FROM school WHERE town= '.$town.'"; Did you mean: $query = "SELECT * FROM school WHERE town= '".$town."'";
-
As long as your code isn't doing something fuddy-duddy like losing the database link or connecting to the database multiple times in a single execution, then I would associate this with the server being shared and possibly overloaded. Again, you'd have to make sure that you're not killing the database connection prematurely yourself. And you'd also have to make sure that one HTTP request to the site doesn't make multiple connections to the database. I don't think it would be the number of includes so much as what's in them that would cause these problems. Many people connect to the db with a db.php and use include( 'db.php' ). Are you doing this? Does it connect to the database again even if a connection has been established? Mulitple require_once() on the same file is, IMO, unlikely to cause you severe issues; the performance hit would be more like milliseconds. die() kills the script right then and there; nothing else executes afterward. Not sure if that helps you. Even more reason to set it up locally in a controlled environment. Dedicated servers can run $200 per month. Your time to install it locally might equate to one to three months of dedicated server costs. If the dedicated server turns out to be unnecessary, it would be more cost effective to your client to let you take the time to do this. For your sanity's sake, I suggest in future projects you make it part of the contract that you will develop locally and push releases to a test environment of the client's choosing. If the client will not provide a local environment for you, then you will use your PC as that environment. You'll be able to gauge your application's hardware requirements and your client will be able to test release for stability and acceptable performance. If they won't let you work in this manner, let some other sucker work for them.
-
Apparently 2 1/2 cups of coffee is not enough for me today because I'm making bad suggestions. Instead of: mysite.com/page2.php?track1 Create the URL as: mysite.com/track1/page2.php Then you can use this as a rewrite: RewriteCond %{REQUEST_URI} /track[^/]+/.* RewriteRule /(track[^/]+)/(.*) /$2?__track=$1 [QSA,L] Then in the page, you should be able to use: <?php echo array_key_exists( '__track', $_GET ) ? $_GET['__track'] : 'Tracking not set'; ?>
-
Saving file on remote computer when register?
roopurt18 replied to Deathfire's topic in PHP Coding Help
http://php.he.net/manual/en/class.httprequest.php -
$_SERVER['HTTP_REFERER']
-
ignace has the right idea; with his solution you'll only need to loop over the result-set once. In any case, $result is a resource that represents the results of the query. The resource contains an internal record pointer. If you want to be inefficient and loop over your results multiple times, you can set the internal record pointer's position: http://www.php.net/manual/en/function.mysql-data-seek.php
-
Why use the query string for tracking at all? Why not just store the value in the user's session.
-
In what case can I increment value of i without using loops ?
roopurt18 replied to linux1880's topic in PHP Coding Help
<?php $i = 0; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; $i++; echo $i; ?> -
Hmmm. My car always seems cleaner after a good, hard rain. /shrug
-
It sounds to me like you will have to match the character code to the appropriate mathematical operation.
-
At university I did all of my C coding via 80 char wide telnet session, so I completely understand the hang-up. Even after I started using fancy IDEs I stuck with 80 char wide for a looooong time. Within the past year I've become a bit more lazy and will go to like 120 chars wide for some things. Every once in a while I end up editing a file live on a remote server (to try and figure out WTF is wrong with it) and get stuck with that 80 char limit again.
-
This is the first real rain Southern California has had in I don't know how many years. Take advantage of your free car wash while you can.