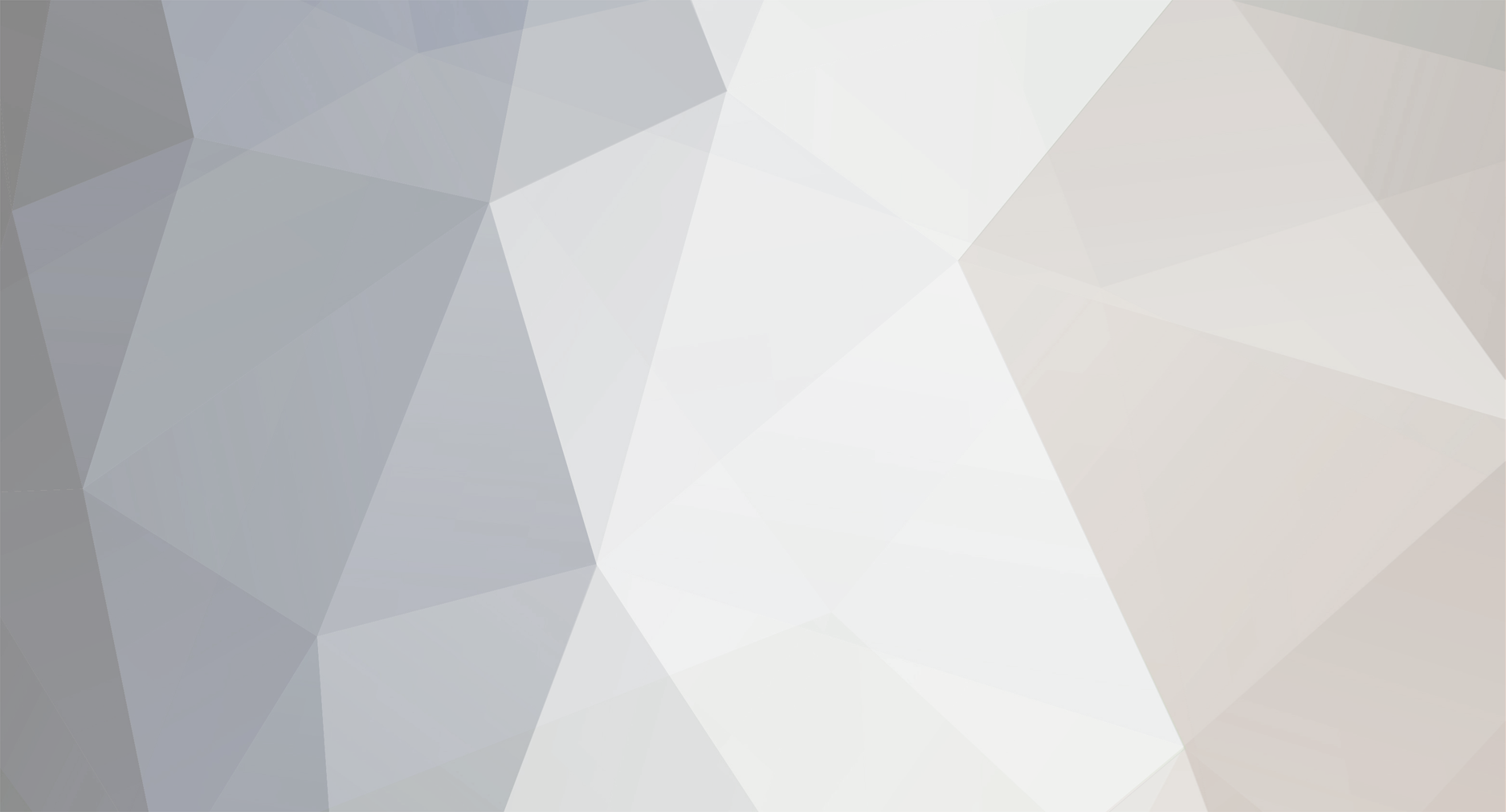
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Yes, you will need regular expressions. [url=http://www.regular-expressions.info/]http://www.regular-expressions.info/[/url]
-
[code] echo '<td> <p align=right><font face=Arial size=2><font color=#006666> Chance of Winning: ' . $row['Rating'] . '</font></font></td>'; [/code] This is the kind of code that will make your life Hell later. I guess it's common practice because PHP allows you to do so, but IMO extending a string onto the next line is bad programming practice. Some editors will treat the third line of that code as if the variable is inside the string, instead of vice versa. In other words, some editors get confused and totally screw up the syntax coloring of your source code.
-
I'm just curious how others separate the data getting dumped by their PHP scripts. I've seen some PHP code with <?php and ?> all over the place, making it difficult to read. A lot of times you'll see something like: [code] $Html = "some_html"; // Do some stuff $Html .= "some_more_html" . $var . "combined_with_a_var"; $Html .= DoMore(); echo $Html; [/code] I use that myself for small segments of HTML. However, for large blocks of HTML it becomes difficult to visualize the structure of the page when you combine a lot of PHP with a lot of HTML. Lately I've been creating .html files that contain large chunks of HTML with slugs surrounded by curly braces. Each chunk of HTML is surrounded by comments that I use in conjunction with a regexp to pull just that chunk from the file. For example: [code] <!--HTML_CHUNK!--> <div> <!-- 10 lines of html !--> {ROWS} <!-- more html !--> {ANOTHER_SLUG} </div> <!--HTML_CHUNK!--> <!--ANOTHER_CHUNK!--> <div> <!-- html for a row !--> {ROW_DATA} </div> <!--ANOTHER_CHUNK!--> [/code] Then in my PHP file I'll use something like: [code] $Html = PullFromFile(HTML_FILE, 'HTML_CHUNK'); $Row = PullFromFile(HTML_FILE, 'ANOTHER_CHUNK'); if(strlen($Html) && strlen($Row)){ $Items = GetData(); if(is_array($Items) && count($Items)){ $Rows = ''; foreach($Items as $Item){ $Rows .= str_replace('{ROW_DATA}', FormatRow($Item), $Row); } $Html = str_replace('{ROWS}', $Rows, $Html); } echo $Html } [/code] IMO, this is much easier to read and maintain. I'm just curious if anyone else does something similar or what solutions you've come up with.
-
How to center pagination links on middle of page [RESOLVED]
roopurt18 replied to AdRock's topic in PHP Coding Help
[code] echo '<div style="text-align: center;">' . implode(', ', $Paginations) . '</div>'; [/code] -
No, the psuedo code I gave will do: [list] [*]Cat 1[list] [*]Sub 1 [*]Sub 2 [/list] [*]Cat 2[list] [*]Sub 1 [*]Sub 2 [/list] [/list] If you want [list] [*]Cat 1 - Sub 1, Sub 2, Sub 3 [*]Cat 2 - Sub 1, Sub 2, Sub 3 [/list] Then: [code] $Cats = GetCategories(); $Html = "<ul>" foreach($Cats as $Cat){ // while loop in your case $CatDets = GetCategoryDetails($Cat); $Html .= "<li>" . PrintCatHeader($Cat, $CatDets); $SubCats = GetSubCats($Cat); if($SubCats){ $SubCatsArr = Array(); foreach($SubCats as $SubCat){ $SubCatDets = GetSubCategoryDetails($SubCat); $SubCatsArr[] = PrintSubCatHeader($SubCat, $SubCatDets); } if(count($SubCatsArr)){ $Html .= ' - ' . implode(', ', $SubCatsArr); } } } $Html .= "</ul>"; echo $Html; [/code]
-
How to retain form values from previous form-with no cookies???
roopurt18 replied to archbeta's topic in PHP Coding Help
This can be done without sessions if the first form is posting to the second form. [code] // Form1.php echo '<form name="form1" method="post" action="Form2.php">' . '<input type="text" name="frm1_txt1" value=""/>' . '<input type="submit name="submit" value="Submit"/>' . '</form>'; [/code] [code] // Form2.php // Check for values from form 1 if(count($_POST)){ $Hiddens = Array(); if(isset($_POST['frm1_txt1'])){ $Hiddens['frm1_txt1'] = $_POST['frm1_txt1']; } } // Create the hiddens HTML if(is_array($Hiddens) && count($Hiddens)){ $HiddensHTML = ''; foreach($Hiddens as $key => $val){ $HiddensHTML .= "<input type=\"hidden\" name=\"{$key}\" value=\"{$val}\"/> } } // Dump form2 echo '<form name="form2" method="post" action="post.php">' . $HiddensHTML . '<input type="text" name="frm2_txt1" value=""/>' . '<input type="submit name="submit" value="Submit"/>' . '</form>'; [/code] But why create a new .php file for each page of the form? They're all the same form after all, so... [code] // Form.php // Call this function to display the form ShowForm(){ if(ValidateForm()){ // Form is valid $Html = ProcessForm(); }else{ // Either form had errors or was never submitted $Html = ShowSpecificForm(); } return $Html; } function ValidateForm(){ if(!count($_POST)){ return false; } $Page = // Get the page of the form to validate, either from $_GET or possibly from a $_POST value switch($Page){ case PAGE_3: $Valid= ValidatePage3(); break; case PAGE_2: $Valid = ValidatePage2(); break; case PAGE_1: $Valid = ValidatePage1(); break; } return $Valid; } function ShowSpecificForm(){ $Page = // Get the page of the form to show, either from $_GET or possibly from a $_POST switch($Page){ case PAGE_3: $Html = ShowPage3(); break; case PAGE_2: $Html = ShowPage2(); break; case PAGE_1: default: $Html = ShowPage1(); break; } return $Html; } function ProcessForm(){ // Follow the same procedure as in validate and showspecific for determining which page // is being processed. } [/code] That puts all the logic for the entire form, even with multiple pages, into a single .php file. If you're good about using functions it will save you a lot of code rewriting and wild goose chases between files. -
if(mysql_insert($sql) == 1){ SendMail(); }
-
Psuedo code: [code] $Cats = GetCategories(); $Html = "<ul>" foreach($Cats as $Cat){ // while loop in your case $CatDets = GetCategoryDetails($Cat); $Html .= "<li>" . PrintCatHeader($Cat, $CatDets); $SubCats = GetSubCats($Cat); if($SubCats){ $Html .= "<ul>"; foreach($SubCats as $SubCat){ $SubCatDets = GetSubCategoryDetails($SubCat); $Html .= "<li>" . PrintSubCatHeader($SubCat, $SubCatDets) . "</li>"; } $Html .= "</ul>"; } $Html .= "</li>"; } $Html .= "</ul>"; echo $Html; [/code] Just make each <li> of the categories also contain a <ul> of the subcategories; this assumes there is nothing wrong with embedding unordered lists in HTML, which AFAIK is OK.
-
I thought when using variable values inside of double quotes you had to enclose them in curly braces. I.E. instead of [code] $qin = "select count(*) from dd_items where ItemCategory = '$ac[CategoryID]' and ItemStatus = 'approved' "; [/code] use [code] $qin = "select count(*) from dd_items where ItemCategory = '{$ac['CategoryID']}' and ItemStatus = 'approved' "; [/code] Or did I miss understand something?
-
I don't see how generating a PDF is going to save me any trouble. My problem at the moment is that not every line in a P.O. is the same height and there's no real method to control it via HTML and CSS. If I generate PDf documents with the information they supply, it's a whole other can of worms. Do I generate the PDF every time they view the P.O. and delete it at the end of the day? Or do I generate it once and keep it around for a while? Then I'd have to go through the trouble of formatting the PDF. Our webhost has PDFLib Lite installed, which means it can only write a single line of text at a time, no text areas. That puts me right back at square one: How much text fits on a single line? Unless you know something about PDF generation I don't, I'd rather not go that route.
-
You can also use the [b]DISTINCT[/b] modifier in your SQL. [code] // Select only unique rows from the table $sql = "SELECT DISTINCT * FROM SomeTable WHERE 1"; // Select only rows with unique row1, row2, ..., rowN combinations $sql = "SELECT DISTINCT row1, row2, ..., rowN FROM SomeTable WHERE 1"; [/code] [url=http://dev.mysql.com/doc/refman/4.1/en/distinct-optimization.html]http://dev.mysql.com/doc/refman/4.1/en/distinct-optimization.html[/url]
-
[quote author=AndyB link=topic=106555.msg426205#msg426205 date=1157144719] Why not ask people to upload their P.O. as a pdf or generate a pdf from the information they supply? [/quote] The P.O. data is coming from server-software. Previously, client's had to generate a P.O., create a pdf, and then upload it. If the P.O. had to be revised, they would have to repeat the entire process. Our client's are home builders, some of which generate hundreds of P.O.s per day. Now we're sending data from the server-software to our site via a dbf file and what used to take our clients an entire afternoon or day now takes about 5 minutes.
-
This is a bit to read but I really appreciate any help in this matter. I'm looking for a solution to a formatting problem. Since I don't know which technology (PHP, CSS, JScript, etc.) will provide the best solution, I'm posting in this forum. Please move this post into the appropriate forum if necessary. The site is driven by PHP, MySQL, CSS, and Javascript so I'm open to any solutions involving those languages / technologies. One feature of the site is to allow our clients to automatically upload purchase orders (POs) for their contractors to view online. The contractors log in, view their POs, and print them on a standard 8 1/2" x 11" sheet of paper. The problem is each PO follows the same basic layout, but specifics need to change according to our clients' data. Each PO has a header and footer which need to be printed on each page of the PO. Then there are a variable number of items to be printed in a table layout. The items are optionally followed by a variable length custom verbage, typically legal information. The factors controlling the number of pages in a PO are the number of items in addition to the length of each item and the length of the custom verbage. [b]Layout of 1 Page in a PO[/b] [img]http://www.ibswebview.com/test/po_imgs/po_format.JPG[/img] [b]Image of a good PO[/b] - We can see that this PO is nicely laid out. [img]http://www.ibswebview.com/test/po_imgs/good_po_01.JPG[/img] [b]Bad PO #1[/b] - Here the verbage is too long and overlapping the footer. [img]http://www.ibswebview.com/test/po_imgs/bad_po_01.jpg[/img] [b]Bad PO #2[/b] - Here the PO has extended to two pages. The cause is some of the PO items are too tall. This is causing a second page to be generated without the header and footer copied. We can also see that the footer is being overwritten by the items that didn't fit on the page. [img]http://www.ibswebview.com/test/po_imgs/bad_po_02.jpg[/img] [b]Current Method for Generating a PO[/b] The header and footer are the same size on every PO. The number of items for a PO are counted and based off of 10 items per page the total number of pages is calculated. As each 10th item is printed, a new div with the [b]style="page-break-before: always;"[/b] is created that includes the header. Then the items are printed from where the previous page left off. On each page, the footer is positioned absolutely with CSS. [b]Probable Solution[/b] I'm thinking I'll have to calculate how tall each line of text is on the page and instead of page-breaking every 10th item, I'll page-break when printing the next item will extend into the footer. However, the height of each line may not be the same for every client. I can try and control it with CSS but there are no absolute garauntees that I know of. So before I go fussing around with that, is there a reliable method, perhaps PHP or JScript standard that will tell me tall a chunk of text will be on the final page? It would be nice to do something like: [code] PrintHeader(); foreach(Item){ if(ItemExtendsIntoFooter()){ PrintFooter(); PageBreak(); PrintHeader(); } PrintItem(Item); } // I will then need to print the custom verbage, which may extend to one or more pages. $Verbage = GetVerbage(); // Return verbage text that will fit on the current page do{ PrintText($Verbage); PrintFooter(); }while($Verbage = GetVerbage()); // Continue until no more verbage [/code] I'm thankful for any responses.
-
Can't see global variable in member function?
roopurt18 replied to roopurt18's topic in PHP Coding Help
It has them. This is a dev site that has always worked until I reinstalled XAMPP. I'm assuming a php setting was changed which is causing the problem. But I'm not sure what it is. [!--quoteo(post=382459:date=Jun 11 2006, 03:54 AM:name=Barand)--][div class=\'quotetop\']QUOTE(Barand @ Jun 11 2006, 03:54 AM) [snapback]382459[/snapback][/div][div class=\'quotemain\'][!--quotec--] included file needs php tags [code]<?php $g_pregs['raidname'] = '/^[a-zA-Z0-9 ()]{1,32}$/'; $g_pregs['charname'] = '/^[A-Z][a-z]{1,28}$/'; $g_pregs['username'] = '/^[a-zA-Z0-9]{2,30}$/'; $g_pregs['password'] = '/^[a-zA-Z0-9]{3,20}$/'; $g_pregs['email'] = '/^[a-zA-Z0-9._%-]+@[a-zA-Z0-9._%-]+(\.[a-zA-Z]{2,4})+$/'; $g_pregs['alpha'] = '/^[a-zA-Z]+$/'; $g_pregs['digit'] = '/^[0-9]+$/'; ?>[/code] [/quote] -
Can't see global variable in member function?
roopurt18 replied to roopurt18's topic in PHP Coding Help
/bump Anyone? I can't develop my site and I have no idea why this is happening. -
I reinstalled XAMPP today on my development machine, set everything up and it works. Except now I have errors about a global error being undefined. I have a global array defined in validate.php [code] $g_pregs['raidname'] = '/^[a-zA-Z0-9 ()]{1,32}$/'; $g_pregs['charname'] = '/^[A-Z][a-z]{1,28}$/'; $g_pregs['username'] = '/^[a-zA-Z0-9]{2,30}$/'; $g_pregs['password'] = '/^[a-zA-Z0-9]{3,20}$/'; $g_pregs['email'] = '/^[a-zA-Z0-9._%-]+@[a-zA-Z0-9._%-]+(\.[a-zA-Z]{2,4})+$/'; $g_pregs['alpha'] = '/^[a-zA-Z]+$/'; $g_pregs['digit'] = '/^[0-9]+$/'; [/code] Then I have a CForm.php that defines a CForm object [code] require_once('validate.php'); class CForm{ // ValidateForm // Do basic form validating, ensuring that all required fields in // $m_required are present, also ensure that all fields in $m_formats // match the required format // RETURN: true on success, false otherwise function ValidateForm(){ global $g_pregs; // Next we check for specific formatting of the fields if( is_array( $this->m_formats ) ){ foreach($this->m_formats as $format){ if( !preg_match($g_pregs[$format[1]], $_POST[$format[0]]) ){ // the format is invalid, add error message $this->AddError($format[2]); $this->AddErrorIndicator($format[0]); } } } /* More function code */ return $value; } } [/code] The problem is now my $g_pregs is empty inside the CForm::ValidateForm method. I'm unsure why.
-
I had a table previously but ran into a problem trying to add fields to it with javascript. I figured if I dropped the table and used <div> tags I could add new rows to the table easily.
-
I have a three column form where I'm trying to set up specific widths for the columns. I have a test page that works fine but when I view it in the context of the actual site none of the widths are being set. The HTML file: [a href=\"http://rdev.ibswebview.com/junk/NewServiceRequest.html\" target=\"_blank\"]http://rdev.ibswebview.com/junk/NewServiceRequest.html[/a] The CSS file: [a href=\"http://rdev.ibswebview.com/junk/webview.css\" target=\"_blank\"]http://rdev.ibswebview.com/junk/webview.css[/a] This is the first time I've really used stylesheets and most of this project I inherited from another individual. I'm sure it's something small that I'm overlooking and I'm hoping someone with more experience can point me in the right direction. The working test HTML file: [a href=\"http://rdev.ibswebview.com/junk/nsr.html\" target=\"_blank\"]http://rdev.ibswebview.com/junk/nsr.html[/a] The working test CSS file: [a href=\"http://rdev.ibswebview.com/junk/stylesheet.css\" target=\"_blank\"]http://rdev.ibswebview.com/junk/stylesheet.css[/a] Thanks!