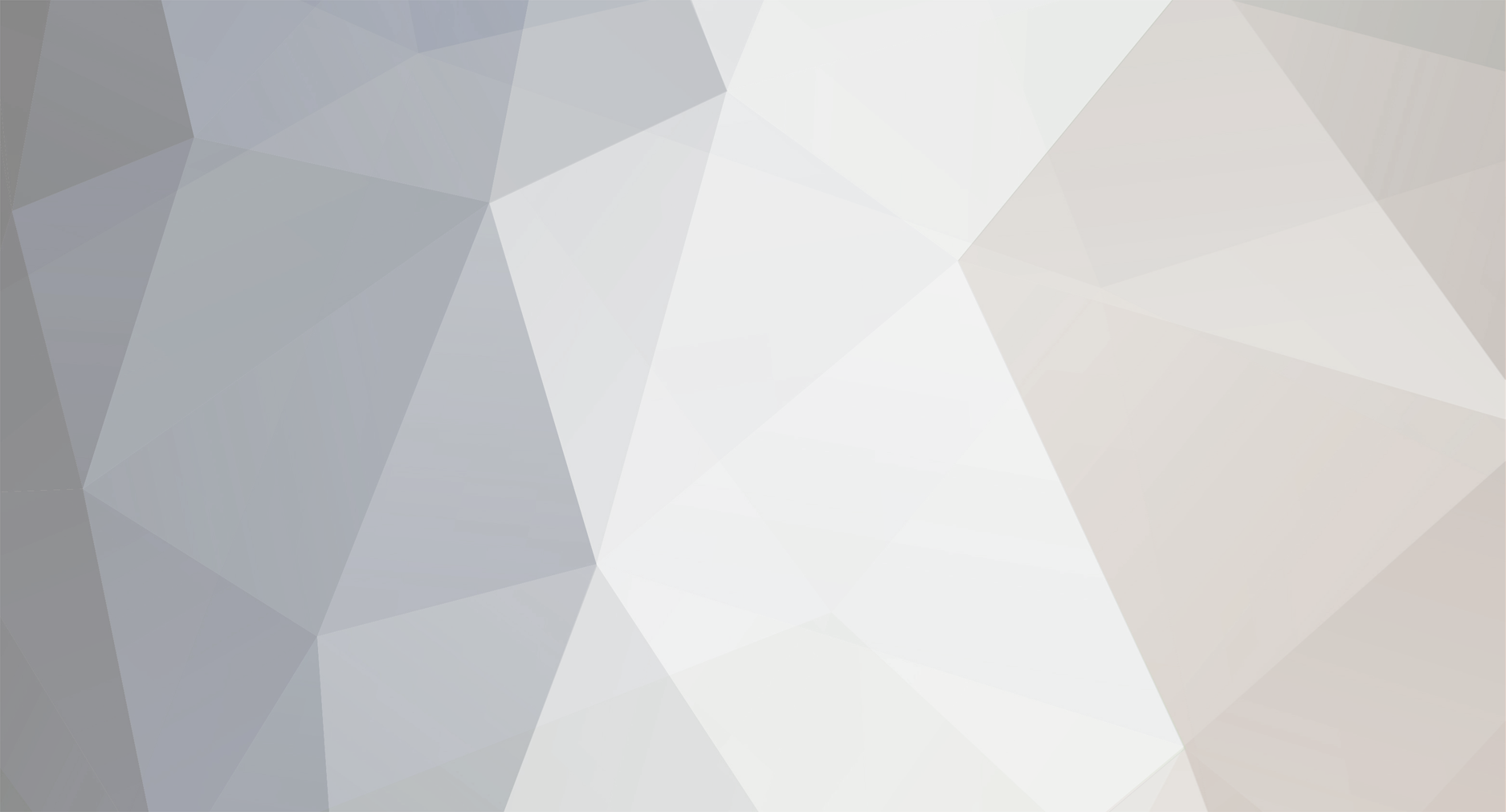
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Setting up subversion for Production and Beta/test site
roopurt18 replied to EvanAgee's topic in Other Web Server Software
Configure your repo so you can access it from home. I would set up the repo directory as: $REPOPATH /project1 /tags /branches /trunk /project2 /tags /branches /trunk /project3 /locationA /tags /branches /trunk /locationB /tags /branches /trunk Let's say you're developing project1. At home you checkout project1 for local development. When you're done, commit your changes to /project1/trunk Then go to beta.domain.com and svn update the beta document_root with trunk. This will move the trunk you committed from local to the beta area. Test the beta site. Make any necessary changes and commit back to trunk. SVN update your local copy and make sure it still works in local. It should work in local and beta. When you're finally satisfied, create a tag of your project to the next version: $REPOPATH /project1 /tags /v0.0.0 /v0.0.1 /v0.0.2 /branches /trunk Now go over to the production and export (not checkout) the latest version into the production doc_root. -
I had to do something similar for work. I have two separate web applications which are standalone. However I needed to "embed" one app into the other. Essentially the user logs into AppA. They then click on a link to AppB. AppB actually opens up in an iframe under AppA's navigation menu. But then AppB presents it's login page. So how did I solve this? Well since I control both apps I control the login mechanism. What I did was when I create the link to AppB I include the user's session_id() in the URL. In AppB I check for the preset session id in $_GET and if it's there I set session_id() before calling session_start(). Essentially I'm allowing my users to hijack their own sessions. I don't know if this will work for you, but it worked in my case.
-
not allowing upload over 100kb - 200kb (estimation)
roopurt18 replied to 9three's topic in PHP Coding Help
Which jamiet757 already mentioned. -
Just to nitpick, how can you know what is "best" at something if you don't know what it is?
-
Write to PDF and place image using PHP
roopurt18 replied to dennismonsewicz's topic in PHP Coding Help
http://php.he.net/manual/en/ref.pdf.php If you look at the functions, you see some named like: pdf_begin_document() pdf_begin_page() I imagine that API segments a document into logical pieces much like an API for a web page would. In other words, in an HTML document you have the head section, the body section, etc. Well in the PDF document you have document scope, page scope, etc. You have to set the library into page scope to call that function. How to do that? I don't know! -
Write to PDF and place image using PHP
roopurt18 replied to dennismonsewicz's topic in PHP Coding Help
Programming languages have data types: integer, string, boolean, float, double, etc. resource is a data type in PHP. Whenever you successfully connect to a database, open a file, a socket connection, etc. the data type returned is a resource. I've never built PDF files with PHP so I can not know for sure (although you can if you read the documentation), but I would guess that the following lines: $pdfdoc = pdf_new(); pdf_open_file($pdfdoc, "test.pdf"); effectively turn your variable $pdfdoc into a resource. The error message can not be any more clear (once you're used to seeing them and understand what they mean): message 'pdf_fit_image() expects parameter 1 to be resource, string given -
not allowing upload over 100kb - 200kb (estimation)
roopurt18 replied to 9three's topic in PHP Coding Help
Don't forget that the form also should have a hidden parameter indicating the max file size as well. -
Write to PDF and place image using PHP
roopurt18 replied to dennismonsewicz's topic in PHP Coding Help
pdf_fit_image('test.pdf', $image, 0, 50, ""); It says argument one must be a resource and you're giving it a string. You probably want to use this instead: pdf_fit_image( $pdfdoc, $image, 0, 50, ""); -
Please review. I have finally got it running. www.newgads.com
roopurt18 replied to ltcchuan's topic in Website Critique
I don't have any time to test anything, but like others my first impression is great design. Good job! -
Replace a string, the code does not go well written but
roopurt18 replied to pibebueno's topic in PHP Coding Help
$ has a special meaning inside double-quoted strings. $s = '1234'; echo "asdf$s"; echo "\n"; echo 'asdf$s' -
No problem. As I said before, tech-forum users are very unlikely to solve your homework for you. But if you present yourself as someone who wants to go through the learning process (as you did yourself), then someone will generally walk you through it. Anyways, I'll throw some more your way. If you've learned about this in your class already, then feel free to implement it in your program. If you've not learned about this already, then you may not want to include it as it will be an indicator to your professor you had outside help and we want them to know that even though you had help, you did do the assignment yourself. (If in doubt, I guess you could give them the URL to this thread.) Notice how you've hard-coded 10 as the upper limit on your array? If you later decide you want it to be 20, 5, or 1000, then you'll need to edit your code in two places. Your program is small and two edits are not a big deal. But imagine your program were much larger. The common example is you are writing a large math program and have coded 3.14 in thousands of places. Then you decide it should be more precise and want to use 3.14159. Well now you have thousands of edits to make! Luckily we have the concept of constants to help with such problems: http://php.net/manual/en/function.define.php
-
http://en.wikipedia.org/wiki/Indent_style Your style doesn't matter and will evolve over time. Just read that wiki article and pick one that works for you and then modify as necessary. If you ever work for a larger company they might dictate a style to you though. Using an if to check that the passed index is within bounds is good. It means you're thinking about error checking. However you should be checking that: if( $index >= 0 && $index <= 10 ) Passing index by reference is unnecessary in this case since your function does not modify it; it uses it as "read only." You only need to pass by reference when your function will change the argument so that the change is seen by the code that called the function. If this doesn't make sense I can give you some resources to help with that. Your my_array_display() doesn't need an $index argument at all since you can use foreach and won't be using the $index argument for anything. As for your my_display(), here's one with a few pointers: <?php $foo = array( 'a', 'b', 'c', array( 'x', 'y', 'z' ), 'l', 'm', 'n' ); echo '<pre>'; my_display( $foo ); echo '</pre>'; function my_display(/*&*/$arr, /*, &$index -- WON'T BE USED, SO NOT NECESSARY*/ $indent = 0 /* AN INDENTATION ARGUMENT */) // WE CAN PASS THE ARRAY "REGULARLY" SINCE WE ARE NOT MODIFYING IT // ALSO, AS IT TURNS OUT, IN PHP5 AND GREATER ARRAYS ARE PASSED BY REFERENCE ANYWAYS { $nl = "\n"; // The function now takes an $indent argument. // Uncomment the following line to see how $indent changes depending on the $arr // contents. How might you combine this increasing value to create your indentation? //echo "Indent is {$indent}{$nl}"; // <-- UNCOMMENT THIS LINE AND PUT ON THE THINKING CAP //if ($index <= 10) // NOT NEEDED SINCE WE'RE USING FOREACH, //{ // IT WILL GET THE VALID INDEXES FOR US if( is_scalar( $arr ) ) { // IS THE ARRAY A SCALAR? IF SO JUST PRINT IT echo $arr . $nl; }else{ foreach ($arr as $key => $value) { if (is_scalar($value)) { // THIS IS A SIMPLE-CASE, NO RECURSION NECESSARY // // WHEN USING VARIABLES IN DOUBLE QUOTED STRING, // I RECOMMEND ENCLOSING THEM IN CURLY BRACKETS // TO AVOID UNEXPECTED RESULTS echo "{$key} => {$value}{$nl}"; } else if( is_array( $value ) ) { echo "$key => Array ({$nl}"; // $value IS AN ARRAY, WHICH IS WHAT my_display() // OPERATES ON. SO FOR RECURSION, HERE IS OUR // SPECIAL CASE. my_display( $value, $indent + 1 ); echo ") {$nl}"; }else{ // THIS IS A SIMPLE-CASE, NO RECURSION NECESSARY // echo "{$key} is not an array or scalar!{$nl}"; } } } // NEXT LINES ARE ATTACHED TO THE COMMENTED-OUT IF ABOVE, SO WILL // BE UNNECESSARY AS WELL //} //else //{ //echo "You must enter a number between 0 and 10."; //} } ?>
-
On average a person with a college degree is going to have more options than one without and make better pay. If you really enjoy programming and problem solving, I suggest (like others) you continue with college and obtain a degree in computer science and possibly even a math minor. Learning a programming language to write programs is the most basic task a programmer will face; any real programmer should be able to learn any language and solve problems with it. In other words, computer science courses are not there to teach you programming languages; you should be able to do that on your own. The courses are there to teach you how to solve complex problems. In addition, core computer science courses will give you a theoretical and scientific background to problem solving, rathr than teaching you how to use the latest technology fads (such as AJAX). However (and this is all on average), if you prefer to make less money, be less desirable in terms of credentials, want to limit your field of expertise, and want to update your certifications every few years, then by all means drop out of school and pursue courses that teach technologies rather than theories.
-
I don't know what these are, but I'm guessing they're for subscribing and reacting to messages. Such a thing could be useful if you were writing a PHP service with child threads.
-
Which PHP Editor do you think is the best? [v2]
roopurt18 replied to Daniel0's topic in Miscellaneous
I got this with quotes in NB 6.8. I can swear to Rasmus it wasn't working this way in 6.7 and below. I have NB 6.8 Beta at home and it works correctly with parens. I haven't noticed one way or the other with quotes; if I remember I'll check tonight. -
That's not true. Object destructors and shutdown functions will still run after a call to die() or exit(). I stand corrected. However, in terms of how the OP asked the question (multiple queries separated by a die()), if the die() executes then none of the "regular" PHP code (such as additional queries) will execute. I guess that's how I should have phrased it.
-
Yup, you sure would! so it really doesn't matter? When you get right down to it, no it doesn't matter if the code is OOP or procedural. There are plenty of great programs written in either or both. Regardless of OOP vs. procedural, you still have to write the code that validates the data. Regardless of OOP vs. procedural, you still have to write the code that saves the data. Regardless of OOP vs. procedural, you can still obtain encapsulation. Regardless of OOP vs. procedural, you can still obtain inheritance (although it will require more procedural code since it's not "built-in") Regardless of OOP vs. procedural, you can still enforce behavior (although it will require a lot more procedural code than OOP code) When you want to design, enforce, and extend behavior, use OOP. You don't use OOP to write behavior because it's impossible to do so in procedural code, because it is possible. You do it with OOP because that's what it was designed to do so it has better facilities for doing so.
-
[quote]My personal favourite is a lack of standards in a script. I see code that is a complete mish-mash of the following: Well, to be fair a programmer's style changes over time. If a project is long-lived, then there is a good chance the programmer may make stylistic changes over the course of it. If the project is financially backed and not just a past-time or pet-project, then there is no budget (time or money) to bother with frivolous modifications such as the case of variables.
-
Which PHP Editor do you think is the best? [v2]
roopurt18 replied to Daniel0's topic in Miscellaneous
Earlier in the thread I mentioned that I use this IDE as well. The only other IDE that compared in terms of speed was Zend 5.5, which was the version before ZendEclipse. There is one thing that PHPED does that drives me insane to no end though. When it auto-completes a function it puts the caret between the parens, which is fine. But when you type the closing paren yourself it adds an extra paren instead of just overwriting the existing one. I tried to find a setting that controls this but couldn't. Example: file_get_contents(|); // # Typed: file_get_cont<space> file_get_contents('foo'|); // # Typed: 'foo' file_get_contents('foo')|); // #typed: ) // and now there's two closing parents -
Yup, you sure would!
-
Functions perform operations. Objects define behavior. See my responses throughout this thread for an explanation of what I mean: http://www.phpfreaks.com/forums/index.php/topic,284048.msg1346916.html#msg1346916 (And more specifically, this response and on: http://www.phpfreaks.com/forums/index.php/topic,284048.msg1347796.html#msg1347796)
-
This is the assignment: This is your function: function array2(&$arr) { You're supposed to accept a second argument and use that to choose which index to set. function array2( &$arr, $index ) { // TODO fill in function body } http://php.net/manual/en/function.is-array.php http://www.php.net/manual/en/function.is-scalar.php http://www.php.net/manual/en/control-structures.foreach.php (Take special note of the $k => $v syntax) Your display() function should be recursive. A recursive function is one that calls itself and stops calling itself when certain conditions are met. For better explanation and examples see: http://en.wikipedia.org/wiki/Recursion_(computer_science) http://www.php.net/manual/en/language.types.string.php Take special note of escape sequences. They start with a backslash, not a forward slash: "\t" is a tab. Based on your output, you are displaying this in a browser. Browsers ignore extra white space. You can have 30 spaces, newlines, and / or tabs in a row and the browser will display one space. You can get around this in at least two ways: 1) Output your code between pre tags: http://www.w3schools.com/TAGS/tag_pre.asp (As an aside, w3schools is an excellent XHTLM reference) 2) Change the content-type of the document to text-plain. To do this, make this the first two lines of your PHP program: <?php header( 'Content-Type: text/plain' ); // program follows... ?> (Strictly speaking it doesnt have to be at the very beginning of your program, but if you (based on your experience) do not put it there, you will encounter mysterious errors.) You also asked about naming conventions for your functions. Your instinct is correct, array1 and array2 are terrible function names. Names should describe what the function does or what the variable represents. Better names would be: array_fill() array_set() However, array_fill() is already a built-in function, so you'll have to use another name: array_populate() array_set Another common practice for assignments or very small programs such as this is to prefix names with something like my_: my_array_populate() my_array_set()
-
If you want to protect your users, then you must perform the following and in the correct order. 1) Whenever inserting or updating database data, you must escape the data properly to prevent injection. 2) Whenever selecting data from the database and displaying in HTML, you must use htmlentities() to prevent malicious markup-based attacks. As far as escaping data when inserting / updating, you can use mysql_real_escape_string() and be safe. Personally, I prefer to use PDO for my database interaction because it allows the user of placeholders and will escape data without my having to do so. You can also configure PDO to throw exceptions on errors, which I prefer as it generally results in less error-handling code. I can not stress enough that you must, absolutely must, call htmlentities() on the data after you've retrieved it from the database. Many programmers insist on calling htmlentities() before inserting data into the database thinking that will save them from calling htmlentities() after retrieving data. The problem with this approach is if the database is attacked directly, the attacker will insert malicious markup that will not pass through htmlentities(). Since the application assumes all data in the database has had htmlentities() applied to it, the application will blindly pass the harmful markup to your users. As far as validating data, it's best to ensure that every piece of user input is in the correct format. Regular expressions are helpful for this in addition to some functions (like http://www.php.net/manual/en/ref.ctype.php). As stated, it doesn't really matter what you accept for input as long as you escape before inserting / updating and escape before displaying to a browser. You will have to be wary of any user input that causes access to the file system. There are plenty of exploits where sites expect something like: showfile.php?filename=foo.txt A malicious user might try: showfile.php?filename=../../../../../etc/passwd If your site doesn't watch out for that type of input, users might gain access to files you don't want them to see. You need to understand the difference between encryption and hashing. Encryption is two-way, meaning you can turn data into junk and then back into the same data. Hashing completely trashes the data in a way you can never, ever, not in a million years know what the original piece of data was again. Password hashing is quite common. md5() is considered a weaker hashing algorithm by today's standards. You should move on to sha1() or sha256(). As mentioned you should salt your hashed data before hashing it; do some research to see why. Never, ever hash a hashed value.
-
There already exist functions to iterate over directories. They might work with URLs the same way that file_get_contents() worked. What was the result from file_get_contents()?
-
You can not name your function array because array is a reserved word for the language. As far as the functions are concerned: http://www.php.net/manual/en/functions.user-defined.php http://www.php.net/manual/en/functions.arguments.php As far as the loop syntax is concerned: http://www.php.net/manual/en/control-structures.while.php http://www.php.net/manual/en/control-structures.do.while.php http://www.php.net/manual/en/control-structures.for.php According to your assignment, you can pick any of the three types of loop for your function. More help with arrays: http://www.php.net/manual/en/language.types.array.php When programming, you should follow a divide and conquer mentality. So let's divide your problem. Step 1) Write a loop that iterates 10 times. Step 2) Write a small program that creates an array and assigns elements to it. Step 3) Now combine #1 and #2 by writing a program that creates an array and uses a loop to assign 10 things to it. Try that and see how far you get.