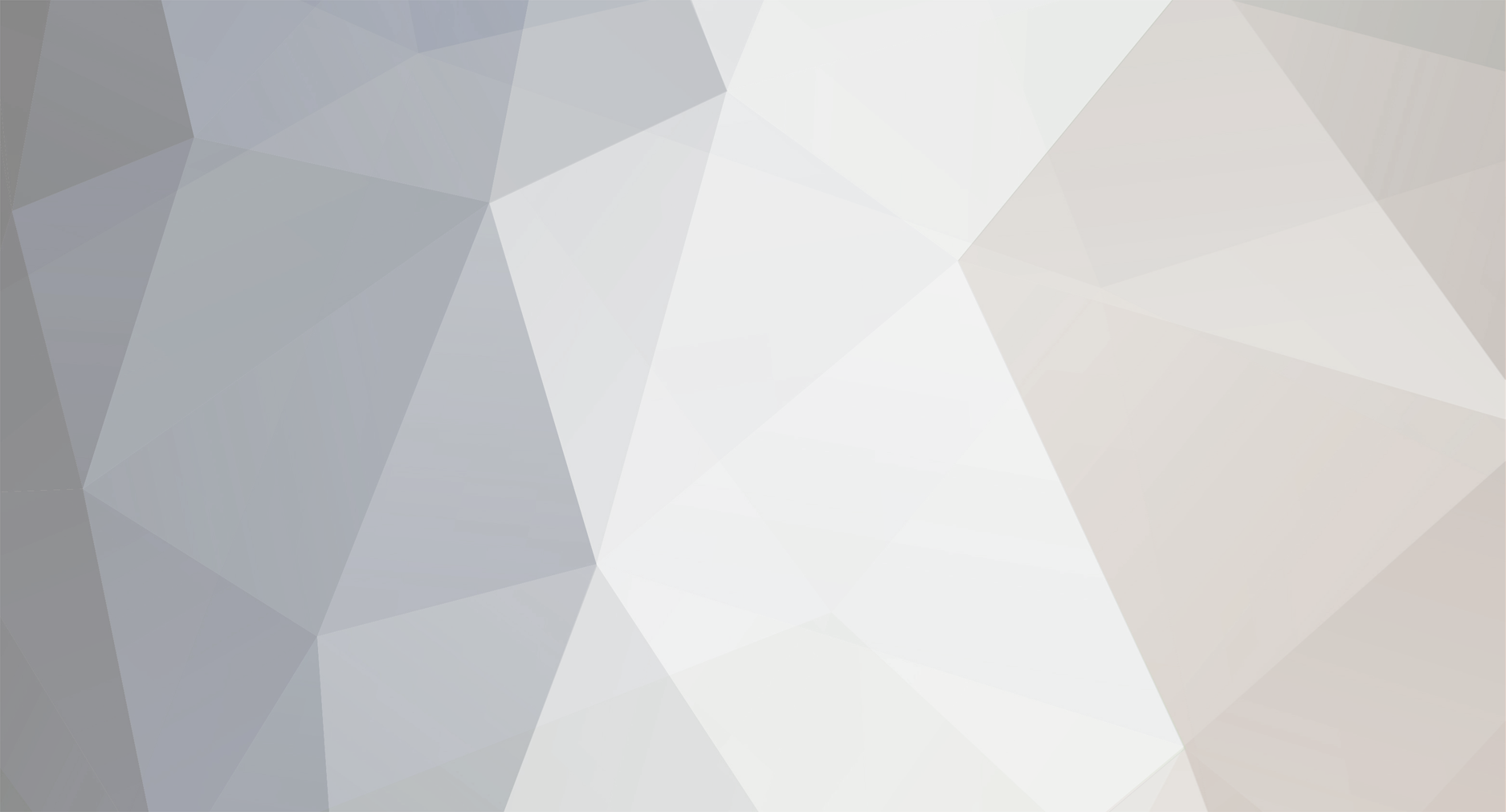
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Upload Security- help with protecting against executable files
roopurt18 replied to clkwebdesign's topic in PHP Coding Help
You should always sanitize data appropriately for whatever database you are using; each database has its own sanitizing function which I'm sure is what you're using. However, sanitizing the data for the database is not the same as sanitizing it for output. If comment posted includes JavaScript, the db-sanitize makes it safe to store in the database. But when you query it and send it to the user's browser they still get JavaScript. If you don't want that sort of thing to happen you need to call htmlentities() or striptags() (or another function) on database data before sending it to the browser. Some people will tell you to "clean" the data in this fashion only upon insertion or updating of your database. For example: <?php $name = $_POST['name']; $stmt = sprintf( "insert into sometable ( name ) values ( %s )", db_sanitize( htmlentities( $name ) ) ); $pdo->query( $stmt ); ?> The logic here is that since htmlentities() was called on the data before insertion into the database, it is safe to display to the browser. I don't have time to go into the details of why but this is a terrible way to do things. 1) Call the appropriate db_sanitize() method for your database before data insertion 2) Call the appropriate escape() method for your output medium before output (i.e. call htmlentities() if displaying to a browser) -
[SOLVED] How to reference HTML tags outside of PHP tags
roopurt18 replied to nothing500's topic in PHP Coding Help
If you want to get a jump start on that, then all you need to know is mod_rewrite for Apache. I design my applications so that the only files that are web accessible are an index.php and served files (JS, CSS, images, etc). All of my PHP files (except the one index.php) reside in a non-web accessible directory. I include mod_rewrite rules that send all requests that don't correspond to an existing file or directory to index.php. Index.php loads my framework, performs some initial set up, and then sets the framework in motion. The framework examines the URL ($_SERVER['REQUEST_URI']) to determine where to send it. -
[SOLVED] How to reference HTML tags outside of PHP tags
roopurt18 replied to nothing500's topic in PHP Coding Help
<?php // Located in: project/app/controllers/ // PostsController.php class PostsController extends Controller { // Displays: http://www.domain.com/Posts/, http://www.domain.com/Posts/Index public function IndexAction() { $this->View->Posts = Posts::GetMostRecent( 10 ); // Most recent 10 posts, just an example of fetching data, this might actually return a PDOStatement } } ?> <?php // Located in: project/app/views/Posts/ // Index.php // The view for the PostsController::IndexAction method ?> <h1>Most Recent Posts</h1> <?php if( $this->Posts->rowCount() ) { ?> <ul> <?php while( $post = Posts::Next( $this->Posts ) ) { ?> <li><?php echo $post->Title; ?></li> <?php } ?> </ul> <?php } else { ?> <p>There are no posts to view.</p> <?php } ?> I have my own framework I use at work that implements nicely the Controller and View. There is a bit of processing I perform that is not shown here that maps URLs to the appropriate Controller, Controller method, and View. -
Upload Security- help with protecting against executable files
roopurt18 replied to clkwebdesign's topic in PHP Coding Help
You need to find out exactly how they hacked you or you may just end up wasting your efforts, especially since you say there is no budget. I don't see how taking away the execute permission from uploaded files is going to protect you. Most of the time (and it depends on your application), when uploaded files are viewed again later they are not execute but just served by Apache or IIS. They only get executed if: + the file itself is a scripting file, such as PHP, + if a PHP script calls exec() or a similar command on the file + or if someone executes it from the shell or command line Turning off the execute bit will only protect you from the last two of those and not the first. Even your extension check should protect against the first one since the extension has to be one that the web server associates with the PHP parser. For example, if you reject all files with a .php extension but I upload PHP code with a .txt extension, when requested by Apache it will not go through the PHP parser because the .txt extension is not associated with PHP by Apache (unless you changed your configuration). Does your site allow for users to post comments? It's more likely that you are not escaping output from the database before passing it to the browser. If this is the case, someone may have posted JavaScript as a comment that modified the DOM on page load. -
If you are indeed using $find as the table name, you can not enclose it in single quotes either. IIRC the proper identifier quote character in MySQL is the backtick: `
-
Change all instances of: $to.= mysql_result($result, 0, 0); to: $to.= ', ' . mysql_result($result, 0, 0); You should only make two changes based on the code you presented, i.e. you don't change the line: $to = mysql_... Also it's really better if you throw just one query at the database rather than three, but you can work on that after you have the initial approach working.
-
Look carefully at what you're telling your program to do: <?php $sql = "SELECT email_address FROM consultant WHERE con_id = '$con_id1'"; $result=mysql_query($sql); $to = mysql_result($result, 0, 0); // $to = 'asdf@asdf.com'; $sql = "SELECT email_address FROM consultant WHERE con_id = '$con_id2'"; $result=mysql_query($sql); $to.= mysql_result($result, 0, 0); // $to = $to . 'foobar@foobar.com' // ==> $to = 'asdf@asdf.com' . 'foobar@foobar.com' // ==> $to = 'asdf@asdf.comfoobar@foobar.com' $sql = "SELECT email_address FROM consultant WHERE con_id = '$con_id2'"; $result=mysql_query($sql); $to.= mysql_result($result, 0, 0); // $to = $to . 'qwerty@qwerty.com' // ==> $to = 'asdf@asdf.comfoobar@foobar.com' . 'qwerty@qwerty.com' // ==> $to = 'asdf@asdf.comfoobar@foobar.comqwerty@qwerty.com' $sender = 'admin@salmonsreach.org'; $headers = "SRC: Administrative Team"; $message = "We would like to notify you that a client has booked an appointment with you. Thanks and Regards, SRC Administrative Team."; $message.= "<br /> Event date: ".$event_start."\n"; $message.= "<br /> Event end date: ".$end_date."\n"; $message.= "<br /> Service: ".$service."\n"; $message.= "<br /> Start time: ".$start_time."\n"; $message.= "<br /> Address: ".$address_first_line."\n"; $message.= "<br /> Post Code: ".$post_code."\n"; // $to contains: 'asdf@asdf.comfoobar@foobar.comqwerty@qwerty.com' // Does $to look like a valid email address to you? mail($to, $sender, $message, $headers); ?>
-
If you want a real answer, then consult a lawyer who specializes in such things. If you were to scrape a page one time just to generate your layout, then probably no one would know or care all that much. If you were constantly scraping it so that you can update your template as they update yours, then yes they would eventually find out. The first clue would be access logs to their site from yours that fit a pattern. If I suspected someone were scraping my page constantly to use my layout as theirs, I would embed something in my source HTML and then see if it shows up in theirs.
-
Need help don't know how to stores images in a database table field
roopurt18 replied to co.ador's topic in MySQL Help
There is no PHP function called db_sanitize(), which is why you receive the error. I included that function as a reminder to you that you have to sanitize user input (stuff from $_POST, $_GET, etc) before you stick it in your database. How you sanitize data will depend on the database back end you are using. image.php is a separate script you have to write, you can not wrap your existing script inside of it. You can not embed images into the HTML page. Images in HTML are created with an img-tag that has a src-attribute that points at a URL. The URL it points at should be image.php, which pulls the image data from the database and dumps it out. -
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
It may also be the case where the designers are unsure exactly how a system will be used. I can't tell you how many times I've began work on a project with the most vague of requirements. In that case you end up going through many iterations of the project until the business end of things says, "It's no longer cost-effective to work on this and we need to move on to other things." Other things might be a different project, or it might be an extension of the existing one, such as adding all sorts of reports or synchronization routines. Eventually you come back to the project as you left it, since the only real constant in software is change, and now you have to support this rubber band ball of a solution. It's rare, although fortunate, when things are perfectly spec'ed out and sufficient budget and time is available to complete a project in full before leaving it. -
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
I didn't want to ninja-edit on you, so this is my would-be edit to my post above: Again, not always the case. If the application is in the early stages then yes, it is better to fix it now. However, this could be a legacy application with all sorts of hooks from outside sources that he is not aware of. In that case it is better to follow the "if it ain't broke don't fix it" as fixing it may break all sorts of things you can't adequately prepare for. Preparing for changes like that take time (which equates to money) that someone may not be willing to pay for. Also, his difficulty in supporting it is not necessarily from the existing bad design (though that doesn't help), but his failure to read the manual for his corresponding database, or his inexperience with databases in general. The next breaking point for his application, however, will be when the field is not wide enough to accommodate the values within. At that point he will be forced to normalize the data at best and at worst change the field definition to something like a text field. A middle ground solution might be something with views and triggers that makes the changes transparent to any other processes or programs that go at this data. Just my thoughts. -
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
But it may not be cost-effective or even worth it. -
Need help don't know how to stores images in a database table field
roopurt18 replied to co.ador's topic in MySQL Help
You create a script that takes a get parameter, such as: image.php?id=47 <?php // image.php $id = db_sanitize( $_GET['id'] ); $stmt = "select img_blob from image_table where id={$id}"; // execute and fetch such that $row is an array of the database fields header( 'Content-Type: image/jpg' ); // or image/gif, image/jpeg, image/png, etc header( 'Content-Size: ' . strlen( $row->img_blob ) ); // maybe set some other headers echo $row->img_blob; // AND MAKE SURE THERE IS NO LEADING OR TRAILING WHITE SPACE IN THIS FILE ?> -
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
<?php $pk = 'the_primary_key'; $add_value = db_escape( 'S04' ); // mysql $u = "update the_table set the_col = concat( the_col, {$add_value} ) where primary_key_col={$pk}"; // postgre $u = "update the_table set the_col = the_col || {$add_value} where primary_key_col={$pk}"; ?> Your next question will then be, "How do I know when to insert the comma?" Depending on the database, you can use some form of a case ... when along with length(). $u = "update the_Table set the_col = case length( the_col )=0 then {$add_value} else concat( the_col, ',', {$add_value} ) end where primary_key_col={$pk}"; -
You should add timestamp columns to your table. I like to call mine created_tm and modified_tm. Each time you insert you set them both equal to now(), or the current time. From then on each time you update one you set modified_tm equal to now(), or the current time. This is best done with a trigger so you don't have to program it in your application code. When you present data to the user, include an: <input type="hidden" name="modified_tm" value="<?php echo $row->modified_tm;?>" /> <input type="hidden" name="primary_key" value="<?php echo $row->primary_key;?>" /> Then in your processing script you can do something like: $moditm = escape_user_input( $_POST['modified_tm'] ); $pk = escape_user_input( $_POST['primary_key'] ); $count = "select count(*) from table where primary_key={$pk} and modified_tm>{$moditm}"; if( $count > 1 ) { // record has been updated since user first saw it, so prompt }else{ // record has NOT been updated since user first saw it, so update } That's pseudo code to outline the basic design so don't copy and paste it and expect it to work. And if you don't want the user to be able to manipulate the hidden fields, then don't send them to the client as hidden fields but instead store them in a session or similar mechanism.
-
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
A) What's in the column before you update it? B) What's in the column after you update it? C) What do you expect to be in the column after you update it? Answer those and maybe we can understand better what you're asking, because right now it doesn't make a whole lot of sense. -
updating row but keeping existing data intact
roopurt18 replied to Walker33's topic in PHP Coding Help
So what's the problem? -
1) Run your markup through a validator. 2) Run your JavaScript through JSLint.
-
From my recollection, it is possible but both domains have to collaborate with each other. I believe I read how to do this in O'Reilly's Complete JavaScript Reference. http://jszen.blogspot.com/2005/03/cross-domain-security-woes.html
-
It's possible that: /sessions actually points at a script file, such as /sessions.php or /process.php or /fooasdfqwertykowijibo.asp. URLs can be virtual, meaning they don't point at an actual resource, but when requested they get directed to an actual resource that does exist.
-
By default browser's don't allow cross-domain JavaScript. I forget how, but there is a way that is supported by the JavaScript language to allow cross-domain scripting. It could also be the case that you install JavaScript on your page that talks to a page on your domain and behind the scenes this page talks to Google or whoever. + You embed ad in your page with JavaScript attached + User clicks ad, JavaScript talks to ad.php on your domain. + ad.php then sends web service request behind the scenes to a third party.
-
[SOLVED] How to reference HTML tags outside of PHP tags
roopurt18 replied to nothing500's topic in PHP Coding Help
This is essentially impossible. In dynamic database driven pages, your template will always be displaying dynamic data. Therefore your template will always need: + a way of inserting dynamic data (i.e. PHP variables and their values) + a way to perform simple logic (i.e. if-[else]-endif ) + a way to iterate (i.e. dumping result sets) Therefore your template always needs, in addition to whatever output syntax you are generating (XHTML, HTML, XML, json, csv) special syntax to accommodate the three needs I presented above. Some people have gone as far as to invent "templating" languages like smarty. I really don't see why they do this though, because PHP is a template language itself. One can make an argument that there are a developer and designer working together and the designer doesn't know PHP. But they'll still have to learn whatever template engine syntax they decide to use, such as smarty. So they might as well learn the basics of PHP anyways. Also, a designer that can't handle simple programming logic in the template is probably not going to create a template sufficient for use in pages powered by lots of JavaScript. Essentially, every designer should know enough of PHP to create templates using the output format (XHTML, HTML, XML, etc) and PHP to drive template decisions. As the developer, if you want to make the amount of PHP the designer has to know and understand minimal, then you can process and simplify the information before it ever gets to them. This can be inefficient though as it leads to things such as iterating over result sets from the database twice. For example the developer fetches the data, iterates over it, cleans some things up, and slams it into an array. Then the code passes over to the template where the designer now iterates over the array and slams it into the template. Now you've just got double processing. Personally I use the controller and view philosophy. My controller selects and prepares data but without iterating over it. I then invoke the view where I perform iterations, some decisions, and maybe other presentation cleanup routines. -
When starting on a brand new project, do you like to create the interface or database first? Or is there something else you start designing? Assume you aren't using any code generation tools as they may skew your decision. For example some tools will build a generic interface when pointed at a database; in this case you would obviously create the database first so you can use the interface generation tool. So again, assume you would be coding everything by hand. Experience has taught me it's usually better to start with the interface. My reasoning is the interface deals directly with what the client or user needs. These needs change constantly and especially while a project is young. Therefore the interface will undergo many iterations before being approved. Since the database is merely persistent storage of what's in the interface, it makes the most sense to me to design it after the interface has become mostly static in design and then connect the two together. Or in other words, the interface usually dictates the database design. I'm curious how others feel.
-
You could encapsulate whatever functionality this is inside a class with properties. Then you don't have to pass anything around and each function has access to them.
-
AFAIK, in PHP 5 and less you can't overload a function in the true OOP sense. You can provide optional parameters or shove a bunch of configuration parameters into a single array parameter though. Perhaps you can in PHP6 but I'm uninformed about that.