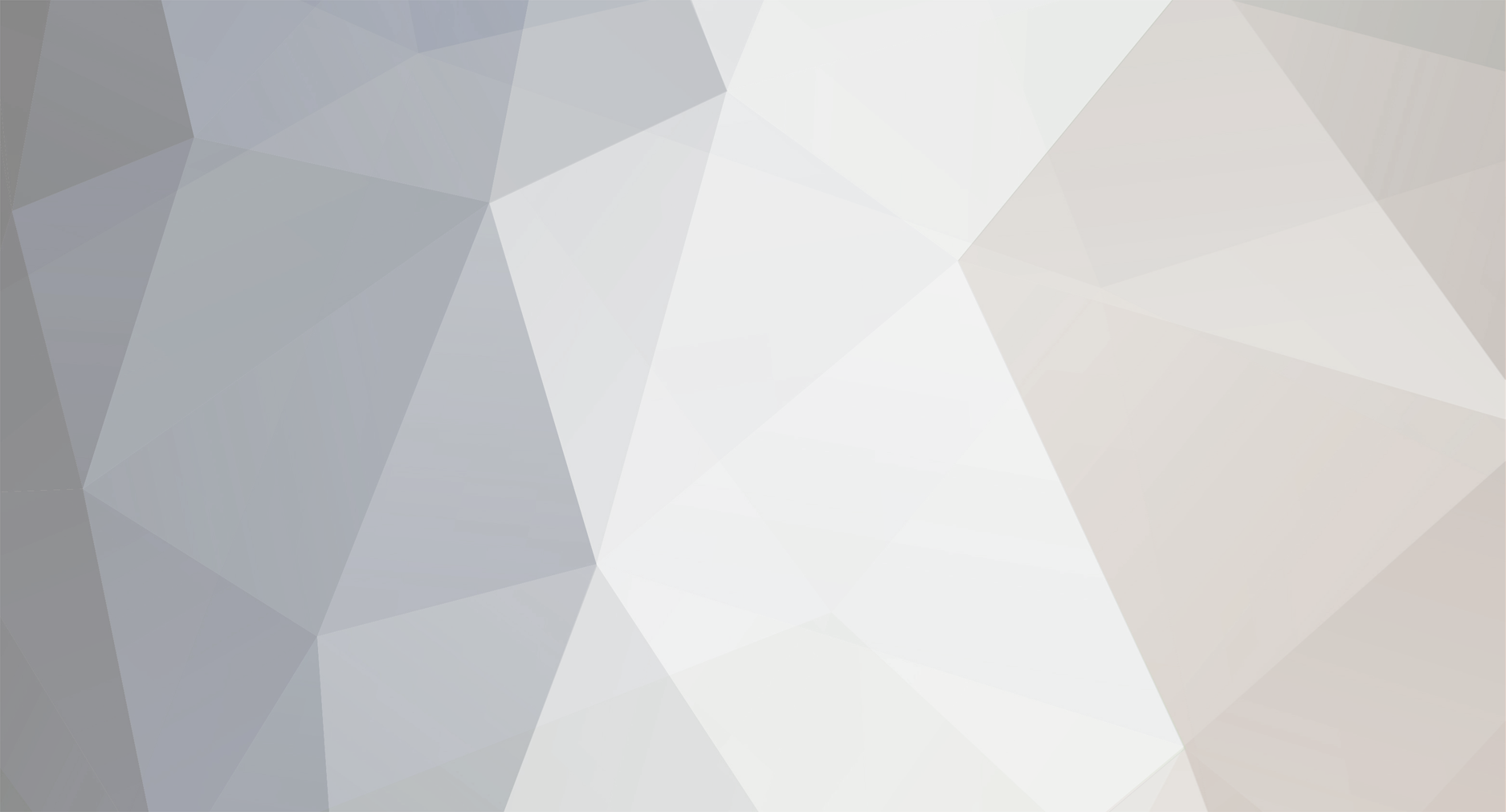
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I've been bit in the butt so many times by MySQL and reserved words that all of my MySQL statements have backticks around all of my identifiers. You especially have to worry about upgrading an existing MySQL installation to a new version where new reserved words have been introduced; in that case all sorts of queries that worked forever may break. I haven't used MySQL in about a year and a half now though; we use PostgreSQL at my current company and it doesn't seem to have this problem.
-
It's possible that read is a reserved word in MySQL, so escape it in backticks. <?php $check_messages = mysql_query("SELECT mid FROM messages WHERE tuid={$_SESSION['uid']} AND `read`=0"); $check_messages1 = mysql_num_rows($check_messages); ?> If it's an int field then the single quotes around the zero should have no effect. It could also be that your $_SESSION['uid'] contains funky characters (like a single quote or semi-colon) that are terminating the statement early.
-
The only user information I keep in sessions is UserId. If it's false, the user is a guest. Otherwise it's their database primary key. Anything else you need can be retrieved from that.
-
Probably. But since he's learning... Consider setting up the MyConfig singleton as I suggested. I also like to use a Database singleton that returns a handle to a PDO object. Then I can just do: <?php $pdo = Database::getPDO(); ?> <?php /* Database.php */ class Database { static private $_pdo = false; static public function getPDO() { if( self::$_pdo === null ) { $cfg = MyConfig::getInstance(); $pdo = new PDO( /* fill this in */ ); if( $pdo instanceof PDO ) { self::$_pdo = $pdo; }else{ throw new Exception( "PDO creation." ); } } return self::$_pdo; } } ?>
-
All this means is your script is trying to use more memory than PHP is configured to allow for. Technically it has nothing to do with PHPMailer. You may want to tell us more about what you're doing and exactly at which point in your script you're running out of memory. Or you can convince your provider to bump up your memory usage or try to do so yourself: http://drupal.org/node/29268
-
Also keep in mind anything sent via get will likely show up in the web server's access logs, which also tend to get archived and shuffled around on disk. The usual rules apply. + Don't trust any input from the user (i.e. sanitize it all) + I'd go as far as to say not to trust data from your own database + Use proper encoding when sending output to a browser + Don't use global variables + Don't allow files uploaded by users to be executed + The only PHP file you need to be web accessible is an index.php, everything else could be safely saved outside of your public_html or www directories (JS, CSS, images, and .htaccess excluded) + Don't rely on stupid security measures + Use encryption / hashing as appropriate + Use SSL for really important data + Don't save sensitive information in permanent storage (remember this can happen accidentally in the case of archived apache access logs and GET variable)
-
[SOLVED] Importing from Windows desktop to localhost
roopurt18 replied to Mr. Green's topic in PostgreSQL
I just use the pgAdmin III interface to perform such tasks. Right click on the db and search through the pop-up menu for the option that allows you to restore. Or you can probably do it from the command prompt. Did you read the postgre manual?!? -
I know you can write triggers in python (and possibly PHP), so if the trigger language can execute scripts I don't see why not. Of course the database user will need the proper execute permissions. An alternative would be in your update trigger you set a modified_tm column or insert into a second table. Then you can write a cron-task, or even a service, to monitor for changes and perform any sync operations you need performed.
-
Perhaps the user's theme is stored in their database profile? You're going to get confused with all those variables floating around the global namespace. You'd be better off creating a singleton class called MyConfig that reads your config.php file and loads members with the config values. Also, you might as well make your config.php a config.ini file and just use PHP's parse_ini_file() function. /* index.php */ require_once( 'classes/MyConfig.php' ); include( 'somefile.php' ); /* somefile.php */ function a_random_func() { $cfg = MyConfig::getInstance(); echo 'dbhost: ' . $cfg->dbhost . "\n"; }
-
It's not a huge concern that sessions use cookies. It's much harder to hijack a session than it is to exploit a poorly designed 'Remember Me' feature. In any case, the only ways I know of to hijack a session are: 1) Compromise the server's security 2) Listen to communication between client and server 3) Have a trojan or spyware installed on the client #1 should be nigh impossible. #2 can be avoided using SSL. #3 should only compromise any users who happen to use that machine, and there's not much you can do about it as the owner of a web site.
-
PHP lives on the server, JavaScript lives on the client. The only way the client sends information to the server is via HTTP requests; an HTTP request always has a URL and can optionally have GET or POST data. Therefore if you want to send data from JavaScript to PHP (i.e. from client to server), then you must make an HTTP request (using XHR). Your request can put the information anywhere you'd like it to be: 1) The URL 2) GET 3) POST Your PHP script should grab the data from wherever your JavaScript placed it; if you'll need it in a future PHP script request, then look at storing it in a SESSION.
-
More specifically: mod_rewrite
-
All you do is cringe? I feel like I got hit in the wobblies with a baseball bat.
-
Almost works. It enters the database as: St. John's Based on that snippet I quoted you are double encoding by the time it gets to the browser. The reason you had to decode only once is to get rid of the second (and erroneous) encoding that is occurring. My guess is your PHP is spitting out encoded values and then your JavaScript is encoding them a second time while building the select-tag.
-
Just to be sure, which database are you using? And are you using PDO?
-
Glad that you got it sorted, but it sounds to me like you are encoding things one too many times. I recommend taking the time to learn when and how to properly decode / encode things to save yourself some headache in the future.
-
I could have sworn I entered it with the proper HTML entity. I've fixed my original post. I intended it to say that by the time it hits the browser it should look like this: <option value='St. John's'>St. John's</option> (edit) SMF did it again! I had to edit this one to get it to appear correctly as well.
-
Easier way to update records / won't update
roopurt18 replied to KeeganWolf's topic in PHP Coding Help
Inventory$store What's in $store? Or, is that $ intentional or an accident? Also, you could just do this: update Inventory$store set case=case - 1 where invnum in ( 11522, 11011, 11121, ..., 11463, 11522 ) -
DarkWater hit it on the head. You're placing invalid characters in your attribute and making your [X]HTML invalid. You said your option looked like this: <option value='St. John\'s'>St. John\'s</option> I don't know where you're generating that markup, from JavaScript or from PHP, but where ever it comes from it needs to look like this when it reaches the browser: <option value='St. John's'>St. John's</option>
-
Not sure what you mean. Your reply sounded like Johnny Cash from his San Quentin album.
-
If it's this line: $cols = array_keys( $_POST['player'][0] ); Change it to: $cols = array_keys( $_POST['player'] ); $cols = array_keys( $_POST['player'][$cols[0]] );
-
Yes, your understanding of how it works is correct. <?php if( !empty( $_POST ) ) { /** * If you print_r( $_POST ) you will find that you have an array named 'players'. * Each index into this array will be POSITIVE and the players database ID if they already exist in the database * The index will be NEGATIVE if the player is new and needs to be inserted. * * And then each player is an array where the associative names should match your column names, * so that you can easily generate your insert / update statements based on what I * showed you earlier. */ $insert = $pdo->prepare( "an insert statement" ); $update = $pdo->prepare( "an update statement" ); foreach( $_POST['players'] as $player_id => $player_info ) { if( $player_id < 0 ) { $insert->execute( $player_info ); }else{ $update->execute( $player_info ); } } exit(); return; // whatever is appropriate to stop processing } $result = mysql_query( $sqlplayers ); $maxrows = 15; $insid = -1; // create a blank player template $cols = array( 'fname' => 'size="10"', 'lname' => 'size="10"', 'address' => 'size="15"', 'city' => 'size="15"', 'state' => 'size="2"', 'zip' => 'size="10"', 'phone' => 'size="10"', 'email' => 'size="20"', 'number' => 'size="2"', 'gradyear' => 'size="4"', 'feet' => 'size="1"', 'inches' => 'size="4"' ); $blankplayer = array(); foreach( $cols as $c => $extra ) { $blankplayer[$c] = ''; } // we now have a blank player template for( $i = 1; $i <= $maxrows; $i++ ) { if( $result ) { $player = mysql_fetch_assoc( $result ); } if( !$player ) { // We've run out of players, so create a blank one to insert $result = null; // stop trying to access result $player = $blankplayer; $player['id'] = $insid--; // first blank player is id -1, second is -2, third is -3, etc. } // dump the fields foreach( $cols as $c => $extra ) { echo "<td>" . tep_draw_input_field( "players[{$player['id']}][{$c}]", $row['player_roster_' . $c], $extra ) ."</td>"; } $player = null; // important! } ?> CURDATE() probably goes along with a modify_tm field or something similar. You don't need to include it in the form at all. When you write your update / insert just make it like: update table set modi_tm=CURDATE() insert table ( modi_tm ) values ( CURDATE() )
-
Also, with your form the way it is now, fill it out and submit it and on the processing page perform a: <?php echo '<pre>' . print_r( $_POST, true ) . '</pre>'; ?> Then try changing your form to this: <td><?php echo tep_draw_input_field("player[$i][fname]", $row['player_roster_fname'], 'size="10"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][lname]", $row['player_roster_lname'], 'size="10"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][address]", $row['player_roster_address'], 'size="15"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][city]", $row['player_roster_city'], 'size="15"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][state]", $row['player_roster_state'], 'size="2"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][zip]", $row['player_roster_zip'], 'size="10"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][phone]", $row['player_roster_phone'], 'size="10"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][email]", $row['player_roster_email'], 'size="20"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][number]", $row['player_roster_number'], 'size="2"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][gradyear]", $row['player_roster_gradyear'], 'size="4"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][feet]", $row['player_roster_height_feet'], 'size="1"'); ?></td> <td><?php echo tep_draw_input_field("player[$i][inches]", $row['player_roster_height_inches'], 'size="4"'); ?></td></tr> Perform the same experiment you did before. Fill out the form and on your processing page do this: <?php echo '<pre>' . print_r( $_POST, true ) . '</pre>'; ?> The second version of the form should be easier to process. <?php /* processing the form */ $cols = array_keys( $_POST['player'][0] ); $stmt = $pdo->prepare( "insert into thetable ( " . implode( ', ', $cols ) . " ) values ( " . implode( ', ', array_fill( 0, count( $cols ), '?' ) ) . " )" ); $success = true; foreach( $_POST['player'] as $idx => $player ) { if( !strlen( trim( implode( "", $player ) ) ) ) { continue; } // empty so do not insert $success = $success && $stmt->execute( $player ); } echo 'Success: ' . ($success ? 'true' : 'false') . "<br />\n"; ?> You could modify that to perform updates as well.
-
You can keep it as is. Before inserting into the database: 1) concatenate all of the fields for a single row 2) trim() result from step 1 3) if strlen( result_from_step_2 ) == 0, then do not insert