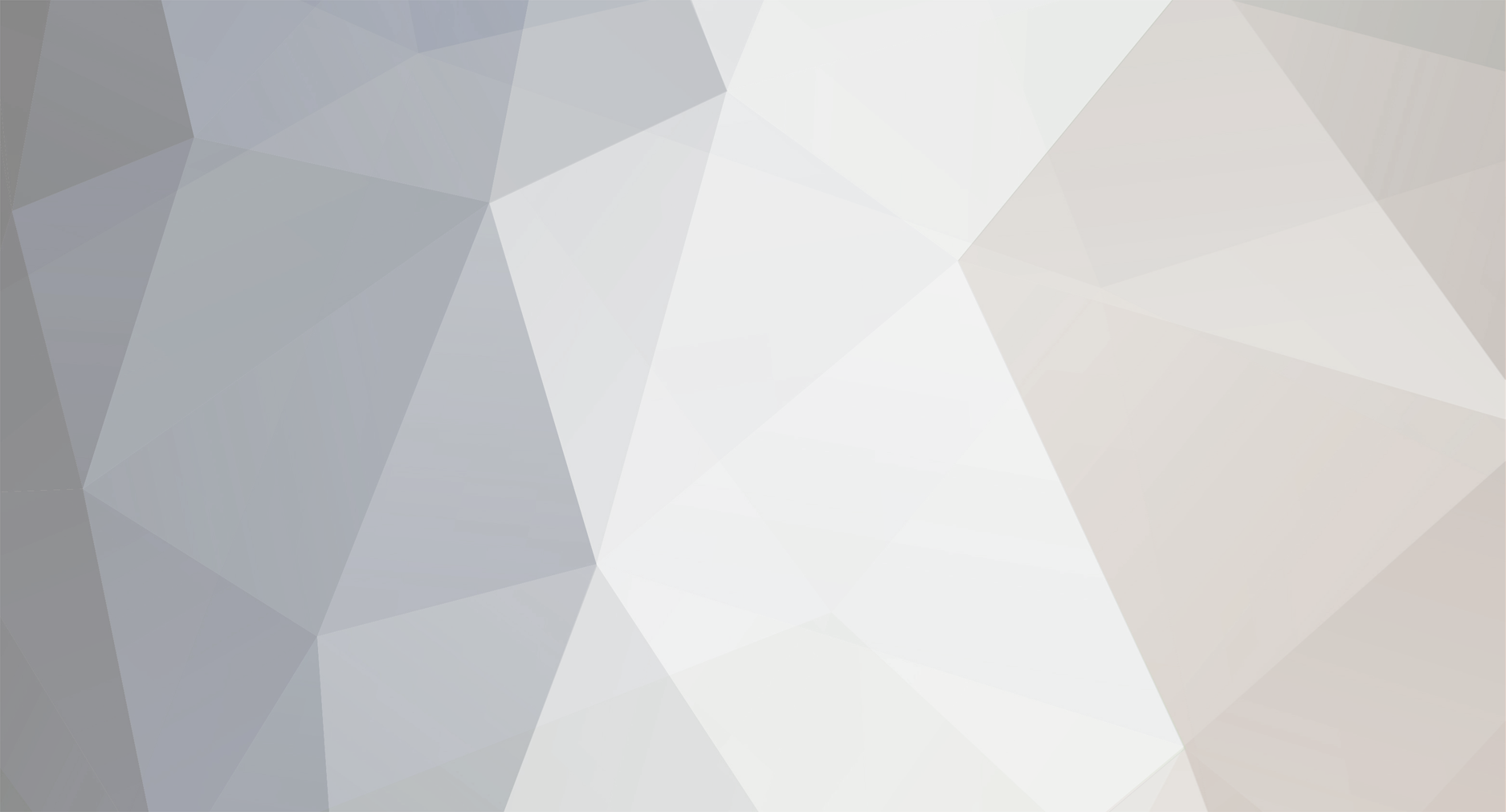
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I don't know if it's the forums doing it but your code indentation is all over the place and it really makes it difficult to follow your program's flow of execution. You're new so you don't have any yet, but you really must give yourself a set of guidelines when you program to make your code more readable. Here are a few I set for myself: 1) No logical block of code should be larger than a single screen height if you can help it. This implies that you should use functions where they make sense. 2) No line of code should be wider than 80 characters. 3) Every time I open a code block, with a curly brace, I indent two spaces. I keep my indentation of two spaces until I close the code block with the matching curly brace. I've developed quite a few more over the years which I won't take the time to type here, but having a systematic approach to programming will greatly reduce the amount of errors you introduce into your code.
-
You can prevent this by doing the following: // // process your post data first // ... // ... // Finished with post data, now immediately redirect header('Location: new/url'); exit(); (edit) What happens is the user posts the data, your script processes it but then redirects the browser to a new page that has no post data submitted to it. When the user presses the back button they are taken to the page that had the form, not the one in the middle that processed the data. If they click forward it returns them to the final page. Basically the processing page is lost to the browser and the alert goes away. (edit x2) Note that you do not need to create 3 files to handle all of this; you can do it in one by being clever.
-
I'm not going to dissect your query in depth, but here are a few steps I take whenever a query is misbehaving. First perform a sanity check on your data. Make sure that the data in the DB is correct, otherwise you might have a perfectly valid query but an application that is inserting bad data. The next step is to build your query in pieces. I usually start with a simple select on the first table and the columns from it. I run it in phpMyAdmin. Looks good? Add the first of my JOINs. Still look good? Add the next JOIN. I repeat this until I'm happy that it is returning the correct data set. Then I add my constraints and grouping if necessary. Finally I take my final query and slap it in my code. This is how I write my queries in code now, I find that it is very easy to read and maintain. <?php $sql = " SELECT col1, col2, col3, DATE_FORMAT(dtCol, 'format') AS `dispDateField', MAYBE_ANOTHER_FUNC() AS `whatever` FROM `table1` t INNER JOIN `table2` t2 ON t.`id`=t2.`owner_id` INNER JOIN `table3` t3 ON t2.`id`=t3.`owner_id` WHERE constraint_one AND constraint_two AND /* To demonstrate how I inserted PHP data */ someCol={$Clean['someCol']} "; ?> The real power to this approach is the query is almost intact in my code. Later when I need to debug or test it I can copy and paste the query as is right from the code, change the areas that say {$Clean['col']} to a real value, and test it quickly in phpMyAdmin. Hopefully that will help you pinpoint your problem.
-
The best way to do this would be to place a UNIQUE constraint on the proper columns in your table. The database will handle the check for you when you attempt to INSERT or UPDATE and the query will fail.
-
So what happens when you only do this when inserting into the database and I bypass your security elsewhere. Then I can insert whatever I want directly into the database and you are not cleaning it when it is displayed.
-
Here's the short of it: If the data came from post or get and Magic Quotes is on, call stripslashes() on it first. Then you call mysql_real_escape_string() on it before inserting it into the database. You do not call functions like striptags() or htmlentities() on data going into the database. Rather you reserve that for when you are about to display that data to a browser. Any time you put data into your database, you want it intact as much as possible. Which means the only things you should do to it is stripslashes() (and only if magic quotes is on) followed by mysql_real_escape_string().
-
I can't comment on bpops reply, but why would you want to reuse primary keys? INTEGER is pretty big. Chances are you'll trash the entire site and rewrite it before you run out of keys.
-
http://www.phpfreaks.com/forums/index.php/topic,37442.0.html
-
*head implodes* Thanks for all the reference points, I'll be sure to check them out.
-
This is the crux of what I'm struggling with. A normalized database establishes a relationship between the data, whereas an object / class establishes a hierarchy. This belongs to that rather than this is related to that. Often the data in the database will be a hierarchy, but it doesn't have to, as exemplified by the portion of your response that I quoted. (edit) I suppose classes represent is related with member variables, but meh.
-
Ok, but if you want the latest orders, which do you choose: Orders::getLatestOrders(); // ~or~ Users::getLatestOrders(); We're dealing with orders, so I would assume we want to use the Orders::getLatestOrders() approach. So let's look at the getLatestOrders() method: // In this implementation, I assume the Order constructor will instantiate a User // object. function getLatestOrders(){ // query the latest orders $sqlOrders = " SELECT x, y, z FROM `orders` WHERE ... ORDER BY ... LIMIT 10 "; $qOrders = mysql_query($sqlOrders); if(!$qOrders){ return Array(); // or null or false } $Models = Array(); while($row = mysql_fetch_assoc($qOrders)){ // Remember, Order constructor creates a User() object of it's own $Models[] = new Order($row); } return $Models; } In that implementation, things are clean, but they are inefficient. There is a single query to pull the 10 latest orders and then 1 query again for each order to pull the user information. So we have 11 DB queries to pull the data we could have gotten from a single query. Additionally, the Order model does not know how it's going to be used (because of good OOP design), so when it creates it's User instance, the user object will likely pull all of the columns for the user from the DB, including the ones we don't want or need. And all of this work is being done just so we can loop over the orders and display them in a table. Seems like overkill to me. Now consider this second approach to the method: // In this implementation, I use a single query to pull ALL of the required data // and the objects will figure it out themselves function getLatestOrders(){ // query the latest orders $sqlOrders = " SELECT x, y, z FROM `orders` INNER JOIN `users` ON ... WHERE ... ORDER BY ... LIMIT 10 "; $qOrders = mysql_query($sqlOrders); if(!$qOrders){ return Array(); // or null or false } $Models = Array(); while($row = mysql_fetch_assoc($qOrders)){ // Remember, Order constructor creates a User() object of it's own // But this time $row contains the user information we want, so it will // pass that information along to the User() constructor so that it // does not re-query the database. $Models[] = new Order($row); } return $Models; } This example is the desired one from the MySQL point of view. A single to-the-point query that grabs just what we need. But now the Orders model knows too much about the User model IMO. Now if we happen to change or add a field in the `users` table, our code changes are not limited to the User model. Now we have to find all the places in our code where we did things the desirable way from a database standpoint.
-
You shouldn't ever store the user's PW in a cookie, encrypted or not. In the case of a 'Remember Me' function that automatically logs a user in, it would be better to store the username and a unique, site-generated key in the cookie. This key is attached to the user's record in the database. When anyone visits the site, you check if the username and this key are present in the cookie. If they are you check if the key matches that in the DB and if it does, log the user in. This way if someone else uses the machine you don't reveal too much about the previous user if they look at the cookie. Optionally you can encrypt the username and key combination in the cookie for additional security.
-
I've been thinking about the model a lot lately and there's just one nagging item in the back of my mind - they seem inefficient. I love good OOP design, as a developer it makes things so much cleaner. I love that the idea that I could create an instance of a User, assign various fields, and then the model will automatically tell me they're invalid or not, etc. 90% of the time I'm dealing with the DB, I'm SELECTing data to display. In this case, which happens to be the majority, objects seem like overkill IMO. If I'm displaying 100 records of data, why create 100 instances of an object when all I'm doing is calling get* methods? It seems to me an array would be more optimal in terms of performance. Additionally, it seems most models I've looked at return just about everything from the DB. I don't always need everything, sometimes I just want 2 or 3 rows out of 10 available. Now I can program the model so that it only requests those 2 or 3 fields, but I'd want to avoid pushing the responsibility of specifying which fields onto the client: <?php // Somewhere in controller or view // The array specifies which fields to pull $user = new User($id, Array( 'username', 'last_login', 'date_registered' ) ); ?> I see this as bad because the view and controller now have seemingly intimate knowledge of the table structure, although I guess you can shove those off as class constants. The other way this is bad is that any other potential fields for the $user model are invalid, so you have to be very careful about how you write your Save() method, i.e. you don't want to replace their e-mail address in the DB with an empty string just because it's not currently present in this limited-field instance. But what happens when I have two different views for a user, showing these fields: username, last_login, date_registered username, signature, e_mail <?php // In one controller $user = new User($id, Array('username', 'last_login', 'date_registered')); // And in another controller $user = new User($id, Array('username', 'signature', 'e_mail')); ?> Again, the controllers have too much knowledge about the User. You could get around this with something like: <?php // In one controller $user = new User(); $user->loadForWhatever($id); // This function knows which fields to load // In another controller $user = new User(); $user->loadForSomethingElse($id); // This function knows which fields to load ?> Now the controller knows less about the inner-workings of the model, but the model knows too much about how it's being used. Ideally all the model does is handle the flow of data back and forth from the DB, it shouldn't know about how it's being used, right? Another item that's bugging me is that relational DB != objects. A lot of the time I'm SELECTing from the DB I'm using JOINs across tables that may not necessarily be related as models. Let's say I want to see the 10 most recent orders and the registered users who purchased them. I imagine a single function that queries the DB for the 10 most recent orders, creates an object for each one, and as each one is created that model creates a $user member that will again query the DB for the user details. I think what I'm trying to get at is I think of all the neat, concise, and efficient MySQL queries I can make and for the life of me can not come up with a way to translate that into models. Is there a way to maintain efficiency of DB querying and still wrap it up neatly as a model for the sake of the developer?
-
(Google Earth - Earth) + Post Secret + Newbie = Help Me!
roopurt18 replied to jsolomon's topic in Application Design
I swear I replied to this earlier today, but I don't see it now. The short version of my reply was, "You need to learn JavaScript and you need to learn it well." Forget the zooming aspect of this project and a lot of the complexity disappears. Most of your issues are then boiled down to rather simple things you can accomplish with JavaScript. If you want to learn from online tutorials, you need to check out anything pertaining to DOM and the Event Models for JavaScript. It also helps to have a solid understanding of JavaScript itself. Since I have a hard time learning from tutorials (my eyes get tired after working all day), when it was time for me to sharpen my JavaScript skills I picked up Javascript: The Definitive Guide (has a Rhinoceros on the cover) from O'Reilly; I consider that book an essential member of my collection. -
[quote author=fooDigi link=topic=101004.msg827634#msg827634 date=1204266678] if i happen to need assembly, then great! i will TRY and learn it.[/quote] So you won't be learning assembly? ;)
-
Form processing - checkboxes & radio buttons
roopurt18 replied to joemamahunt's topic in PHP Coding Help
If that's what is inside of $_POST when you submit the form then something is wrong elsewhere in your code or you did not check any of the boxes on your form. -
Form processing - checkboxes & radio buttons
roopurt18 replied to joemamahunt's topic in PHP Coding Help
joemamahunt I'm going to give you the best advice possible for fixing a broken program: Get more information. 1) Read carefully any error messages 2) If there are no error messages, add code to print error messages (such as echo()'ing mysql_error()) 3) VERIFY your data. Make sure the variables contain what you expect them to contain. This prevents you from using arrays as strings, strings as numbers, etc. As a continuation of #3, the best way to verify your data is to send it as output. Here is a simple little function, that you can expand upon if you wish, to echo your data so that it's easy to read: function debug_echo($val){ $val = print_r($val, true); echo "<pre style='text-align: left;'>{$val}</pre>"; } Add that to your script and at the very top do: debug_echo($_POST); What prints when you submit your form? -
(Google Earth - Earth) + Post Secret + Newbie = Help Me!
roopurt18 replied to jsolomon's topic in Application Design
I agree that overall text will consume much less bandwidth, but assuming a white background and limited colors in the text, all images could be served as gifs to cut down the image bandwidth. The thing that makes this tricky is being able to zoom in and out. It's hard to say ahead of time which will place more or less load on the server. But if you try and do too much on the client with JavaScript you're going to kill those users that have slow machines if you're not careful. I'd say it requires experimentation with both methods to determine which is preferred. -
(Google Earth - Earth) + Post Secret + Newbie = Help Me!
roopurt18 replied to jsolomon's topic in Application Design
Um...You don't need to tile images for infinity. This site would run more like a web application so he could define the width and height. Let's say you want a visible display of 3x3 image tags, or 9. You want an extra row on each side and top and bottom, so that's a total of 5x5 image tags. You load the 9 that are initially visible and then load the non-visible ones. As the user drags images off the screen, you bring in the non-visible ones and then place the ones dragged off on the other side, like a conveyor belt. This is how most internet mapping applications work AFAIK. -
Form processing - checkboxes & radio buttons
roopurt18 replied to joemamahunt's topic in PHP Coding Help
You're also using your labels wrong: <input type="radio" id="radio1" name="radio1" /> <label for="radio1">1</label> -
You will have to use Javascript to disable them on the fly as your users click on them. Essentially you add an onclick event handler to each one and when this handler fires you count how many are checked and disabled the remaining ones. You will still need to verify that only 8 (or however many) have been selected in your PHP script for those users that turn Javascript off or use another means to submit more than the number you want to allow.
-
I saw this comment in the documentation for strptime():
-
Heh, totally wasn't aware of that function. I read some of the comments on that function's documentation page and plenty of other users have written there own functions. I checked out a function on some guy's blog and also stumbled across the GeSHI (Generic Syntax Highlighter) project. Basically it doesn't look like you have to write this yourself. If you want to see how good a syntax highlighter is, give it something tricky: <p>This is HTML! It has reserved words: if, do, while, etc. It also has $variables!</p> <?php echo "<p> This is a tricky PHP string!. It contains reserved words: if, do, while, end, break, foreach, etc. It also contains tricky characters: \", \\, \n. It also contains {$Clean['variables']} and $foobar! </p>"; ?> <p>We're back in HTML!</p> (And just to see how the SMF forums handle it) <p>This is HTML! It has reserved words: if, do, while, etc. It also has $variables!</p> <?php echo "<p> This is a tricky PHP string!. It contains reserved words: if, do, while, end, break, foreach, etc. It also contains tricky characters: \", \\, \n. It also contains {$Clean['variables']} and $foobar! </p>"; ?> <p>We're back in HTML!</p>
-
If you don't know anything about parsers, grammars, tokenizers, lexical analyzers, FSMs, etc. then yes, using some combination of str_replace and / or preg_replace will probably be the easiest solution. Otherwise, what you're wanting to do is very similar to the first couple steps of writing a compiler.
-
No. That's the point. You use mod_rewrite to send every page request through index.php. index.php performs the steps necessary for all of your pages, such as establishing database connections. Then index.php includes the proper file to display, such as search.php, admin.php, forums.php, etc.