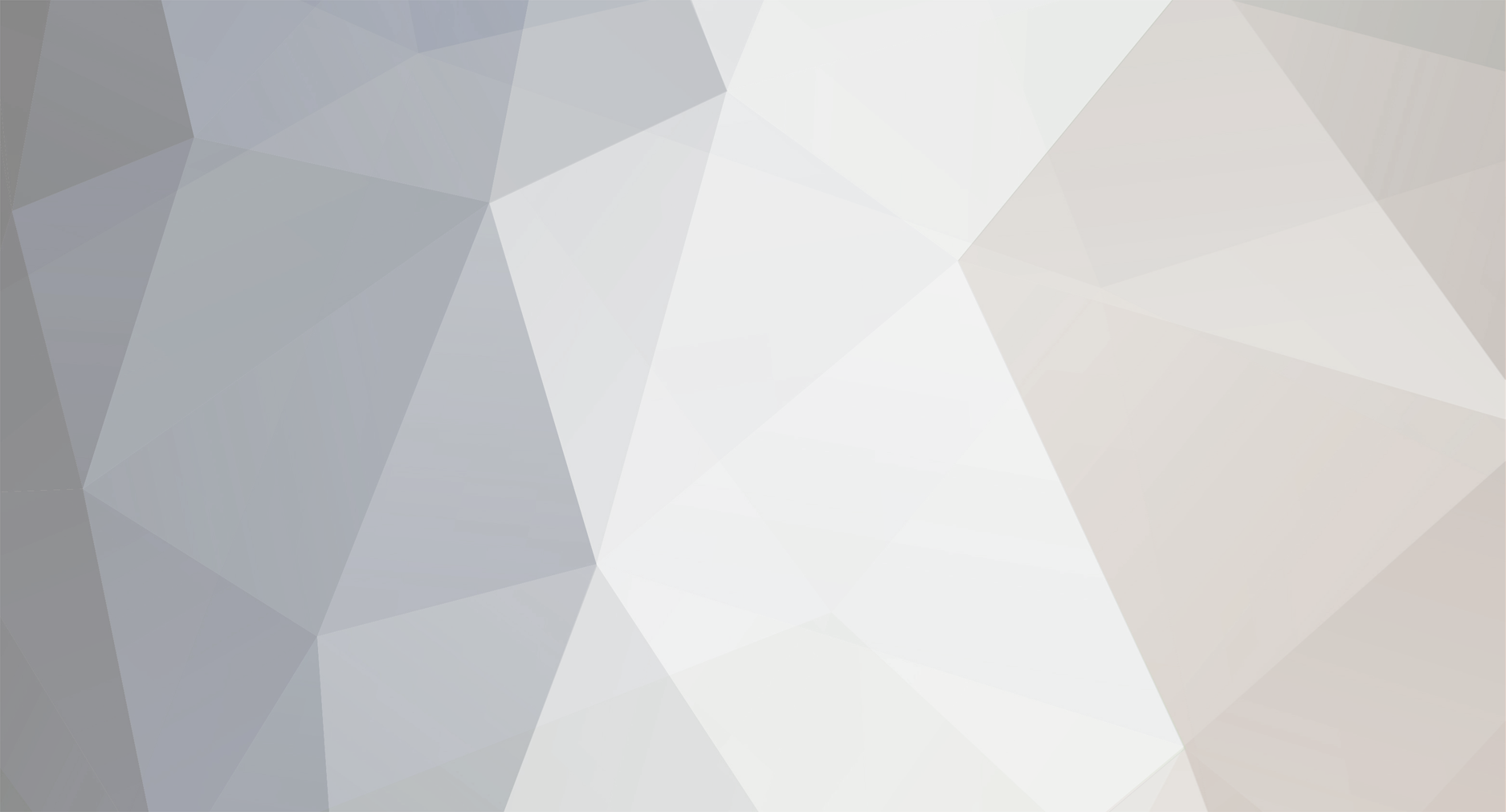
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
You have to write lots and lots of code to have a chance at being a good programmer. Programming is always about efficiency. How can you do the same tasks better and faster. The only way you can make a process more efficient is by understanding it thoroughly. Basically write 10 small programs that do similar things. As you write each one you'll realize you are repeating certain steps and that is where you take a step back and go, "Now how can I do this so I'm not always repeating myself?" Basically if you want to be a better programmer you have to thoroughly understand the process of writing code. And you won't have that until you write lots of code.
-
I suppose you could search for the string <img within your string. // Not tested var aString = "this contains an image <img src='myimg' /> in it"; if( aString.search( "<img" ) != -1 ){ alert("has image"); } I have a feeling that's not going to give you what you want. Are you trying to find a specific image within the page?
-
Someone in IRC asked about dynamically choosing which table to JOIN on. I did a small amount of research and came up with this: http://www.paste2.org/p/19928 /* #1 */ SELECT 1 AS `foo`, t.`id` FROM `wv_user` INNER JOIN `byr_surveys` t ON t.`id`=1 WHERE 1 LIMIT 1 ; /* #2 */ SELECT 1 AS `foo`, t.`id` FROM `wv_user` INNER JOIN `byr_surveys_response` t ON t.`id`=1 WHERE 1 LIMIT 1 ; /* THE MAGIC FOLLOWS */ /* now determine which table to use dynamically */ SET @myStmt := CONCAT( 'SELECT 1 AS `foo`, t.`id` FROM `wv_user` ', 'INNER JOIN ', IF( NULL IS NULL, '`byr_surveys`', '`byr_surveys_response`' ), ' t ON t.`id`=1 WHERE 1 LIMIT 1' ); /* prepare the statement */ PREPARE tmpStmt FROM @myStmt; /* execute the statement */ EXECUTE tmpStmt; DEALLOCATE PREPARE tmpStmt; #1 and #2 are just examples. When I run the significant statements in phpMyAdmin on the EXECUTE portion it says it found records but it won't return them; this is with or without the DEALLOCATE statement. I'm hoping one of the better DB guys could offer something up for accessing that record set?
-
They will only be known if the URL has ?bedrijfsnaam=someValue&categorien=otherValue You can't force the URL to have either of those values so the best you can do is check for them and use defaults if they're not found. <?php // Top of your script $Defaults = array(); $vars = array( 'bedrijfsnaam', 'categorien' ); foreach($vars as $var){ $Defaults[$var] = isset($_GET[$var]) ? $_GET[$var] : ''; } // Later when you need to use them, instead of $_GET use $Defaults... echo $Defaults['bedrijfsnaam']; ?>
-
Can you be more specific?
-
Not as much as you think. DirectX is the multimedia platform for Windows, even OpenGL is emulated by DirectX at this point AFAIK. Just like the monkey is on Microsoft's back to get their browser up to speed in term of standards, the monkey is on the backs of the major supporters of Linux to get it up to speed in the gaming arena. I've been out of the 3D graphics loop for a while, but it used to be the case that OpenGL outperformed DirectX in every department. I don't think that is the case any longer. (Edit) Not to mention that OpenGL is purely for rendering. DirectX provides access to all functions including sound, networking, input, etc.
-
Just don't make it public and you're fine! This IMO is what MS really needs to focus on. In the beginning of computing hardware was expensive so the typical setup was large servers surrounded by dumb terminals. As hardware went down software on the "dumb" terminals began to improve but now we have a maintenance nightmare for IT guys. With the current progression of web services and web applications large corporations are moving back to the "dumb" terminal system and virtualization. From the stand-point of big business it's better if I can run a handful of minimal and fine-tuned installations off a single piece of hardware rather than having one set of hardware per installation or the current situation. As far as the end-user goes, if Linux could just jump over the gaming hurdle millions of low budget individuals would drop windows in a heart beat. IMO this is what the large companies involved in Linux should be after if they really want it to be the most popular desktop OS.
-
apostrophe's and slashes, but not stripping
roopurt18 replied to mikefrederick's topic in PHP Coding Help
You can write a function that removes them at the beginning of the script before you do anything with the values. For one such function (which happens to be the top-most comment in the Magic Quotes documentation): http://talks.php.net/show/php-best-practices/26 It helps to view that page with CSS disabled. -
apostrophe's and slashes, but not stripping
roopurt18 replied to mikefrederick's topic in PHP Coding Help
Read the manual about Magic Quotes. -
The only thing VB really has against it IMO is that it is unnecessarily verbose.
-
Exactly. But they have to make their content publicly accessible or the search engine bots wouldn't be able to index it.
-
Oh wow. So after doing some googling on experts exchange, I learned that the answers are visible at the bottom of the page if you just scroll down. Looks like they're banking on our collective ADD to not notice this and it's working.
-
And so can I. Depending on the question I can find the answer between 30 seconds or 30 minutes. Over time this extra time spent searching for answers can add up; this is why I'm curious about the quality of their service and the answer there. Even if their answer is just a post-back to an obscure blog that you would have found eventually anyways, it's the potential time saved that matters. If they save me just 15 minutes per month then it more than pays for the price. The thing is there's no indication on the quality of the responses and I'm not about to sign up for a trial and give them my billing info at the same time. That's why I was hoping someone here might actually have used them.
-
I'd probably be willing to try the 7 day trial if they didn't want my billing information so they could roll it directly into a monthly fee. Also if they'd increase the trial to 14 days I think that would be more helpful.
-
More and more I'm finding experts exchange in the first or second page of my Google searches. I was curious if anyone around these parts actually uses the site and feels it's worth the fee?
-
If SA or anyone else wants to get a taste of the MS way of doing things: MS Visual C# 2008 Express edition can be downloaded and used for free: http://www.microsoft.com/express/vcsharp/ C# Tutorials and introductions (I'd start under the section General Tutorials): http://www.functionx.com/vcsharp/index.htm It's worth looking at if you have a spare weekend or two. I know that we're all busy and often times don't look at things beyond our immediate horizon, but I often times draw on concepts from completely unrelated fields (such as game programming) in accomplishing tasks in web programming. I.E. the more exposure you have to different fields the more tools you can carry in your toolbox, rather than just a hammer.
-
Sure, but mind you I'm speaking out my butt here. When it comes to Win32 programming most of it is performed in MS IDEs; in fact you'd be crazy not to, their IDEs are so good. Anyways, all windows applications (or 99% of them) are just subclasses of the existing windows framework. Program initialization, execution, event handling, display, etc. are all the same. Also, the UIs are all designed and manipulated the same way. It is all very, very standard because they've been developing these tools for decades and always applying previous experience to make it better. The only structure or framework inherent in programming PHP applications is that imposed by the language. If I toss 3 different PHP applications your way you have no idea how any of them are structured. The one written by a novice is going to be a mess and a headache. The one written by an intermediate guy might use a framework; but if he's using one like Zend which is modular, you have no idea how he has used it (which pieces, what did he customize, etc.), and the one written by the guy doing it for 10 years could be entirely custom but very organized. In any case, you won't know until you look at it. Going back to the windows world, most of the applications will follow the same structure no matter who writes them. Sure the larger apps and the apps written by more experienced guys are going to be even more organized. But they still all rely on the same fundamental groundwork which is more than just that imposed by the language (c++, vb, c#). Remember MS does more than just supply compilers and IDEs, they create the necessary libraries for interacting with their operating system. If you know how to use MS tools, then you really do know what rapid application development is. Now I'm not saying this doesn't exist to some degree in the php (or open source) world. It does, it's just not as wide spread. There's more methodologies, frameworks, libraries, etc. that are available. Here's another way to look at it. People are really excited about Ruby on Rails and Zend Framework, which are relatively new (the past two years maybe?). Either of them is probably as sophisticated as Microsoft's development frameworks were a decade ago. A lot of windows programmers also take Microsoft certifications or training provided by Microsoft, which means that they're all trained to develop the same way. You don't have nearly as much of that in the open source world. The biggest problem I see with MS products is they become bloated. There's really not much they can do about this because they support business; they can't just willy-nilly decide to stop supporting something of theirs because their customers still rely on it. Going slightly off topic, linux and mac users like to take digs at windows and how bloated or inefficient it is. Believe me, if linux and mac every occupy significant market share for non-technical users those OSes will likely become just as bad in some respects. I use linux at home and like it a lot, but it's not without its flaws. Again, this is all opinion.
-
You use sessions to store user-specific data that is critical to your application and can't (or shouldn't) be stored in the URL. For instance, you could design your application such that the URLs look like this: http://www.mysite.com/shopping/<product_id>-<quantity>/<product_id>-<quantity> Basically after the shopping portion of the URL you can store product_id-quantity combinations. You could also use a get array: http://www.mysite.com/shopping?product[id1]=quantity&product[id2]=quantity&product[id3]=quantity In that example, id1, id2, id3, etc. are product IDs from your database. To make your life easier, you should use mod_rewrite to funnel all http requests through a single index.php and you should start the session at the top of this script. Sessions will always be active on your site, even when they're not being used, but it's very little overhead. As for when you should use sessions, the best example is when a user logs in to a site. You would typically store a flag in the session variable named 'User' that is null if the user is not logged in and their user_id if they are logged in. That is usually all I store in session with the exception a small message that can be displayed after page redirects. Other than that I use the URL for all of my parameters.
-
They are both good and can be used for the same purpose. This is like asking, "Which is better for pulling a small trailer, a truck or a hummer?" ASP / ASP.NET / whatever are Microsoft technologies. This means if you use them you pay for them. It also means there are standardized methods for accomplishing goals. The idea is that a programmer can move from one project to another because they're all using the same standard technology. This is also why .NET programmers tend to, on average, earn more money. .NET also has advantages for the business types in that they know a company supports it if they have problems (although the support is not really any better than having a smart developer on your team in the first place). PHP is free and not backed by any one company. This means it doesn't have the same assurances for the business folk. It also means there isn't a single standard method for building sites or using the language. If you pick up a PHP project maintained by another programmer it might be custom or it might use one of a dozen frameworks. You will likely hit a learning curve. Like I said it's free, but it can be harder to find the answers you are looking for in certain situations. In the end they are the same - tools that can accomplish the same tasks.
-
This question is related to Zend Framework. My sites often have a standard navigation for major site components such as news, forums, etc. I also tend to create sub-navigation that depends on the module. The catch is that there might be standard items I want to appear on the main and / or sub nav on every single page. So what I'm looking for is a convenient way of defining site-wide navigation but also allowing the controllers and actions to modify the menus. I'm not familiar enough with the framework to know if there's an already established way of doing this. I'm curious how any of you handle this task.
-
I found a significant error in my post. Where I said, I meant "inside" where I said "outside." If you need clarification I can provide that.
-
/* Your existing user table */ `users` : `id`, <your other fields> /* Each user can have multiple image galleries */ `galleries` : `id`, `user_id`, `name`, `description`, `created`, `modified` /* Each gallery can have multiple images */ `gallery_images` : `id`, `gallery_id`, `description`, `file_extension`, `created`, `modified` The best way to handle uploaded files is to save them on the file system and save only their path and other information in the database. If the files are only available to users that are logged in, then you must take care not to store them inside of public_html or www on your server. One thing I like to do is name uploaded files after their auto_incrementing `id` in the database. Since it's guaranteed to be unique you don't have to worry about the file already existing when you try and save it. You also don't have to worry about invalid or pesky characters in the filename provided by the user, such as quotes, slashes, spaces, etc. Once you decided where to save them, you have to decide how you will organize them. Here are a couple of different structures: /public_html/web_root/user_data/galleries/<gallery_id>/ ~or~ /public_html/web_root/galleries/<user_id>/<gallery_id>/ Wherever you save them, you will have a bunch of image files for the gallery: <img_id>.jpg <img_id>.jpg <img_id>.gif <img_id>.png etc. You will need to store the file extension as well so you can create a name or mime-type a browser will know what to do with. I'll warn you now, do not use the file extension provided in the $_FILES array or by parsing the original file name. Those values can be faked and are not to be trusted. Look into a library like finfo (or fileinfo) or try and manipulate the uploaded file with PHP's GD library to determine for a fact that it is indeed an image and nothing disguised as one. Now you have a catch. PHP provides functions is_uploaded_file() and move_uploaded_file() to determine if a file is actually one uploaded from a browser. You should be using them before you enter anything into the database, but you won't know the parameters to pass to move_uploaded_file() until you get the mysql_insert_id() from the database. Here's a skeleton that you can fill in: <?php // Determine that the file is uploaded if( is_uploaded_file() ){ // You should know the user_id and gallery_id, so you know which directory // to place the file in. What you're missing is the image_id. That's OK. // When PHP accepted the upload, it gave the file a temp name, just use that // temp name for now to move it into the proper place if( move_uploaded_file() ){ $file_ext = ... // write code to determine the extension $image_id = ... // write code that inserts into DB and returns false // or mysql_insert_id() if( $image_id === false ){ // failed to insert into db // delete file from file system }else{ // rename temp file after $image_id . $file_ext } } } ?>
-
Are you trying to combat spam on your site?
-
I use PHP as my template engine. I don't see any reason to add another layer on what can already become a bogged down process (i.e. generating a page).