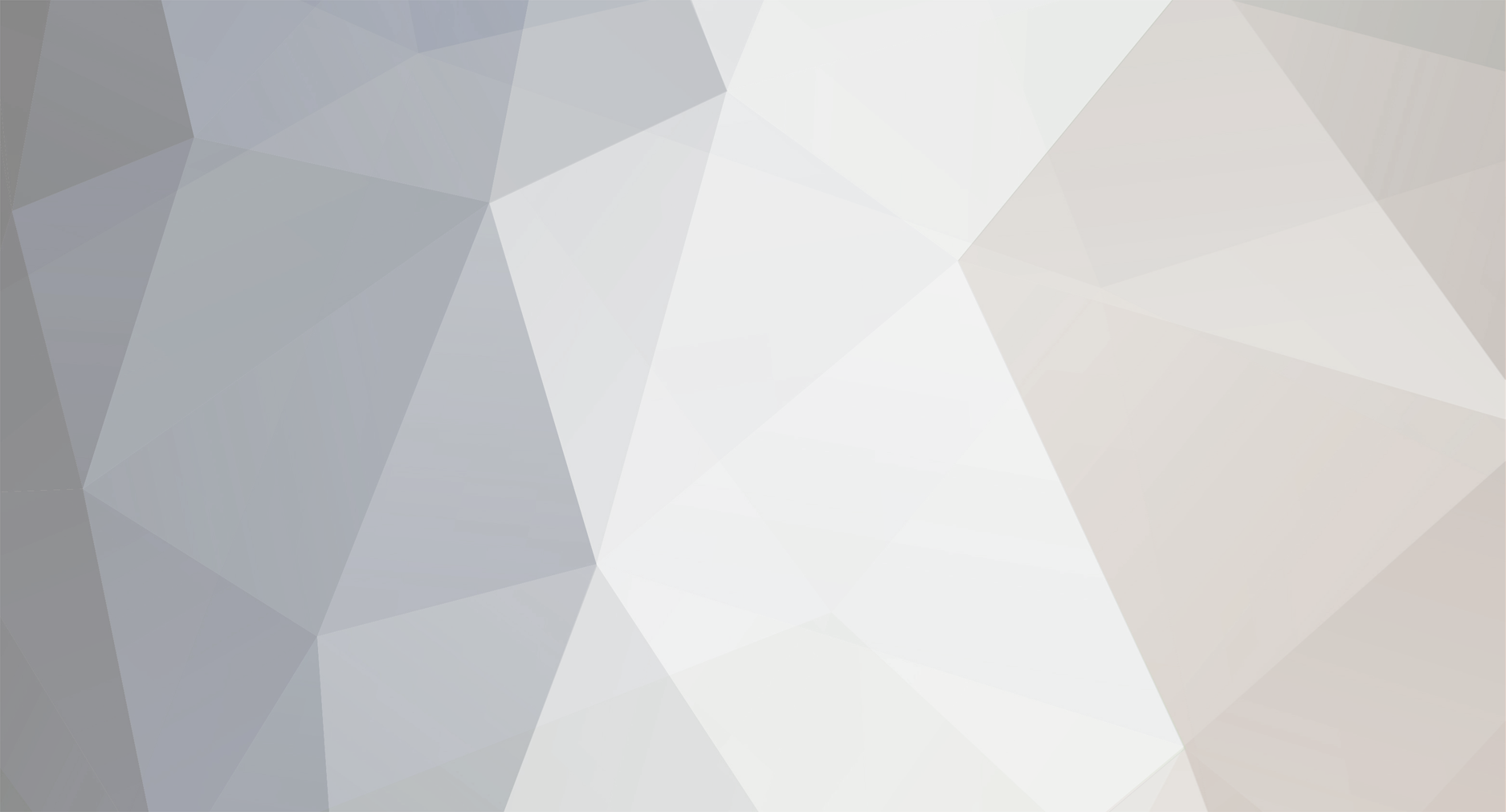
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
(Google Earth - Earth) + Post Secret + Newbie = Help Me!
roopurt18 replied to jsolomon's topic in Application Design
This is one of those ideas that sounds like the dumbest thing ever on paper, but then somehow goes on to become extremely popular out of the sheer novelty factor. Basically you just need to conceptualize your canvas as a box with w x l x h. Then you store each comment with an associated w, l, h, and the comment itself. Then it's a matter of taking what portion of the cube is visible to the viewer, pulling all of those comments from the DB, and then generating an image with the graphic library. A very interesting idea! -
Put this at the top of your file: <?php // Note this is the entire file $url = isset($_GET) && isset($_GET['url']) ? $_GET['url'] : $HTTP_GET_VARS['url']; if(strlen($url)){ header('Location: ' . $url); exit(); }else{ echo 'No URL provided!'; } ?> And if it works correctly just delete the rest of the file.
-
Really strange problem involving Nov. 4, 2007
roopurt18 replied to hoogie's topic in PHP Coding Help
I encountered something similar once. Drove me nuts until the light bulb went off. I had a good chuckle at myself that day. -
You can use mod_rewrite to redirect all requests through a single index.php. You can use the same rewrite rule to append the subdomain as a $_GET variable. RewriteRule ^http://([^.]+).domain.com/ /index.php?sub=$1 Then in index.php: if(isset($_GET['sub'])){ mysql_connect('localhost', 'user', 'pass'); mysql_select_db($_GET['sub']); include('forum.php'); }else{ include('main_site.php'); } Lacking data validation and a slew of other features, but does that help?
-
I'm wondering why you're doing this with meta tags. Anyways, my comments about htmlentities() still stand. You call htmlentities() on user supplied data just before displaying it: $url = htmlentities($url); // <-- INSERT THIS LINE if (ereg("://", $url)) { echo "<meta http-equiv=\"refresh\" content=\"0; URL=" . $url ."\">"; } else { echo "<meta http-equiv=\"refresh\" content=\"0; URL=http://". $url . "\">"; } But I have to ask, why not just do this: // Note this is the entire file $url = isset($_GET) && isset($_GET['url']) ? $_GET['url'] : $HTTP_GET_VARS['url'] if(strlen($url)){ header('Location: ' . $url); exit(); }else{ echo 'No URL provided!'; } Also, your server must be using $_GET because if it wasn't you'd notice you've misspelled $HTTP_GET_VARS.
-
I'm not one to tell people when they can and can't thread stuff, after all it's up to you to decide for yourself. But I will say that generally you can only thread items when there is a parent-child relationship between them. Or in other words, one of the items has to belong to the other. So what you're telling me is that on your site, if I'm viewing a page with 10 comments, you want the comments to indent every other one, even when one of them is not a direct reply to another? Might I suggest just alternating the background color of every other comment, rather than indentation?
-
Can you post the code for dereferrer.php?
-
I don't use PDO in my applications, so I came up with an alternative style. Ditto on your note about XSS. As far as trim() goes, that's a personal preference of mine. There is very little to be gained, IMO, by allowing users to surround their data with whitespace. Despite my comments about htmlentities(), 99% of all data in a MySQL database is going to reappear in a web browser, which just ignores extra white space. Or, if something like nl2br() is used, it just increases the opportunity for users to abuse your system.
-
Because the super_clean_auto_globals() call didn't escape things for the database. Basically, stripslashes() is applied to the auto globals if Magic Quotes is on at the beginning of the script, but the data isn't safe to place into the database. Therefore, just before inserting into the database you must clean it again. I guess I should point out there is a slim chance by the time you are cleaning for database insertion, there is a chance the data cleaned will contain \\ or \', in which case the clean function will revert it to \ or ' respectively. You could get around this with a 4th optional URL parameter, called $skip_stripslashes or something and initializing it to false. Also, my use of a variable named $Clean is a personal preference. The only values I insert int my SQL statements are those that come out of a $Clean array. I do this intentionally so I force myself to clean everything and I usually do it very close to where it's used. If you clean a variable on line 10 and insert it on line 80, how do you know it didn't change to something else in between? Also, you have to admit my structure for SQL statements is very easy to read and maintain.
-
There is a small amount of overhead added by PHP in using double quotes. The reason for this is because you can embed PHP variables in double quotes, like you've done here: echo "<div class='tborder45'><div class='tborder22'>{$row['subject']}</div>"; I also tend to use double quotes for MySQL queries because most queries will contain single quotes somewhere in them. IMO, unless your site is huge in popularity and you can pinpoint major sight slowdown to the extra overhead incurred by using double quotes, then it doesn't really matter. But if your site is so popular that single quotes vs. double quotes is an issue, then you can probably afford a second dedicated server so that Apache can run on one and MySQL can run on the other. Likewise, I've heard about programmers that spent hours modifying code to remove all the double quotes and then the script runs an average of .001ms faster. Big deal. Then you turn around and they're running a query on several hundred thousand rows without an index defined. There are a lot of things you can worry about when optimizing your programs. IMO, single quotes vs. double quotes should not be one of them.
-
This is the only line that isn't PHP: <select name="link" style="font-family : Verdana, Arial, Helvetica, sans-serif; font-size : 11px; width:108px" onchange="if (this.options[selectedIndex].value != '') location.href=this.options[selectedIndex].value"> I don't see what you gain by including that as part of the PHP, so I have to wonder why you're asking to do this?
-
Well, AJAX requests are initiated by JavaScript code that you write. They're not generally seen by the user and search engines don't care about them being friendly. So you could add a query string value named ajax. Then in your scripts: <?php $ajax = isset($_GET['ajax']) && $_GET['ajax'] == 1; if($ajax){ user_interface.php } process_data.php if($ajax){ $user_interface.php } ?> This is cumbersome in two ways though. The first is that you might have to change a lot of scripts. Your life is always much easier when using mod_rewrite to send everything through a single index.php file. That way global site stuff like this only has to be added in a single place. Secondly, the best way to respond back to AJAX requests is with JSON. If you are not familiar with JSON you should read up on it. If you are familiar with JSON, then you will have to come up with a way for your AJAX requests to output pure JSON in place of the normal user_interface.php stuff. For example, I typically respond with JSON that looks like: { ok : false, error : true, error_msg : 'E-mail required.' } Then my JavaScript can do something like: function ajax_handler(xhr){ var obj = eval( xhr.responseText ); if(obj.ok){ alert('success!'); }else if(obj.error){ if(obj.error_msg){ alert(obj.error_msg); }else{ alert('Unknown server error.'); } } } Hope that helps.
-
verdrm, what you are doing is not the best way to store data in a DB unless you are experienced and know what you're doing. Are you familiar with the concept of database normalization? If you're not, then what you want to ask is, "How should I design my tables to do XYZ?"
-
First off, I notice things like this: $_POST[...] = ... $POST_[...] = ... Is that intentional or are you being careless? Because if it's not intentional it's certainly wrong. Then you have this code, which is basically duplicated for $_POST, $_GET, $_COOKIE, etc.: <?php if (isset($_POST)){ $empty = $POST_ = array(); foreach ($_POST as $varname => $varvalue) { if (empty($varvalue)) { $empty[$varname] = $varvalue; } else { $POST_[$varname] = cleanVar($varvalue); $POST_[$varname] = $varvalue; echo "POST var $varname = $POST_[$varname]<br>"; // DEBUG } } } ?> To start with, $_POST is always set so your if() statement is useless as it's always true, at least AFAIK. Further, it's good web practice when your users enter invalid data into forms to repopulate the form with the data they entered. But here you are applying htmlentities() and mysql_real_escape_string() to the user's data. So what happens when your user enters this: In PHP, < is used for less than and > is used for greater than. Let's say your site invalidates the form because the user didn't enter their e-mail address in another field. Your site redisplays the form and populates it with: In PHP, < is used for less than and > is used for greater than. The user doesn't notice their message has changed; when they enter their e-mail address and submit the form, your site will change it again to: In PHP, < is used for less than and > is used for greater than. Later on, someone else views this post (or whatever it is) and sees: In PHP, < is used for less than and > is used for greather than. IMO, not so good! Here is a recursive function that is a one-size fits all: <?php /** * Multi-use function for cleaning user data. Can be used to clean * entire arrays or a single value. * @param array|scalar Data to clean * @param [bool] true to trim * @param [bool] true to mysql_real_escape_string */ function my_super_clean($data, $trim = true, $mysql_escape = true){ // We don't want to call get_magic_quotes_gpc() more than once // so we use a static variable static $gpc_on = null; $gpc_on = $gpc_on === null ? get_magic_quotes_gpc() : $gpc_on; // $data can be an array or a single value if(is_array($data)){ foreach($data as $k => $v){ $data[$k] = my_super_clean($v); } }else{ // Do we stripslashes? $data = $gpc_on ? stripslashes($data) : $data; // Do we trim? $data = $trim ? trim($data) : $data; // Do we prepare for database if($mysql_escape){ // If the value is numeric we do not have to escape it // or enclose it in single quotes. // If the value is not numeric we must enclose in single // quotes and escape $data = is_numeric($data) ? $data : "'" . mysql_real_escape_string($data) . "'"; } } return $data; } ?> Notice how I've completely left off any calls to htmlentities()? In general, you do not want to call htmlentities() on data as it's inserted in the database. For example, pretend I entered << into a field that is stored in a VARCHAR(2) column. If you call htmlentities(), your code is going to try and insert << into a column that can only fit two chars. Wrong. The only thing you store in the database is mysql_real_escape_string()'ed data, optionally trim()'ed. You call htmlentities() after you retrieve the data, just before it is displayed. Also, since you only need to call htmlentities() when displaying to a html-compatible viewer, why bother calling it as you're inserting the data. After all, who says you're always going to display back to a web browser? You can't predict the future functionalities of your application so don't store your data geared for use in a single purpose. Now, the function I've given you above can easily be accompanied by another clean function that will clean $_POST, $_GET, and $_COOKIE, etc. at the beginning of your script: function super_clean_auto_globals(){ $g = Array( '_POST', '_GET', '_COOKIE' ); foreach($g as $v){ // trim() but not mysql_real_escape_string() ${$v} = my_super_clean(${$v}, true, false); } } Now hopefully your site uses mod_rewrite to route all requests through a single index.php file; if so, at the top of it you can place: super_clean_auto_globals(); Which will clean up all of your auto globals for use throughout the entire site. Now, let's say you are inserting data into the database; the recursive function comes in handy again: function insert_db($val1, $val2, $val3){ $Clean = Array(); $Clean['col1'] = my_super_clean($val1); $Clean['col2'] = my_super_clean($val2); $Clean['col3'] = my_super_clean($val3); $sql = " INSERT INTO `table` (`col1`, `col2`, `col3`) VALUES ( {$Clean['col1']}, {$Clean['col2']}, {$Clean['col3']} ) "; $q = mysql_query($sql); // finish up the function } Now this thread might be worthy of a sticky!
-
Your other option is to change the level of error reporting so that PHP doesn't complain about undefined variables.
-
Add this at the top: $op = isset($_GET['op']) ? $_GET['op'] : ''; Then everywhere in your code where you have $_GET['op'], use $op instead. The error is saying the index is not defined, or that $_GET has no index named 'op' associated with it. The line you put at the top of the script will set $op equal to $_GET['op'] if the index is set and the empty string if it is NOT set.
-
You always seem hostile, try a hot bath or jacuzzi sometime? As far as the topic goes, your classmate is basically demonstrating that they don't understand some languages are more applicable for certain tasks than others. Truthfully, C++ is more powerful because anything you can do in PHP you can also do in C++, with the reverse not necessarily being true. I challenge anyone who says otherwise to write a program that controls a space shuttle navigation system in PHP. If it came down to choosing one language as "the best of them all," I'd say it's C, because it's even more widely supported than C++. That said, it doesn't mean PHP is a beginner's language or a "toy." Let's say the average price for creating a web site is $4,000 USD. If you're making your sites in PHP and your friend is making his in C++, there's a good chance you can produce them faster than he can. So he can e-mail you and taunt you that PHP is a "toy" and you can respond when you get back from your vacation. Visual Basic, for almost all intents and purposes, is a horrible programming language. But even it has a niche area in rapid application development where it works very well. Putting it another way, just because I can steer my car with shoes on my hand doesn't mean it's a good idea. Use the proper tool for the job.
-
Well, how you separate them depends on your HTML and CSS. For example, the following will draw dashed lines around the li tags of a ul with a class of reviews: <style type="text/css"> ul.reviews li { border: dashed black 1px; } </style> The HTML you generate would then be: <ul class="reviews"> <li>This product sucks!</li> <li>I love this item!</li> </ul> As far as database goes, let's assume you're attaching reviews to items. You need an items table with a couple of fields: items: id, name Then you need a reviews table with a couple of fields: reviews: id, item_id, review If you wanted to see reviews for a specific item, you'd set up a link that has the item_id as part of the url: reviews.php?item_id=5 On reviews.php, you'd have some sort of code like: $item_id = $_GET['item_id']; $sql = "SELECT * FROM `reviews` WHERE `item_id`={$item_id}"; $q = mysql_query($sql) or die('Error: ' . mysql_error()); echo '<ul class="reviews">'; while($row = mysql_fetch_assoc($q)){ echo '<li>' . $row['review'] . '</li>'; } echo '</ul>'; Those are the steps in the most basic form. There's a lot of stuff left out, such as data validation.
-
I have no idea what you're talking about. I have a feeling you may be attempting to run when you haven't even learned how to walk yet. Are you working out of a book or an online tutorial? As for your error, try changing: SELECT * FROM restaurants WHERE name LIKE %colname% ORDER BY name ASC to $sql = "SELECT * FROM restaurants WHERE name LIKE '%colname%' ORDER BY name ASC"; Also the '%colname%' is likely meant to be a search string, something like: '%Friday%'
-
Ok. So the comments stuff is showing up. It's just not exactly how you'd like it. My first question is, if the pages shown in the iframe are served from the same site, why do you have an iframe to begin with? If you eliminate the iframe the page will grow to fit it's contents. My second question is, how come you have the height attribute on the iframe set to 480? That would seem to be why the page is only partially displaying the comments box. Try a larger setting, like 650, if you are adamant about keeping the iframe.
-
For which platform? Windows? Linux? And if your answer is Linux, why not compile it yourself? I'm not trying to be an ass, but trying to point out the vague nature of your query.
-
Have you ever written a form that inserts data into a database? The reason I ask is because once you write one you've sort of written them all, so it sounds like you've never done it before. In which case the best thing to do would be to look for an online tutorial on how to generate an HTML form and insert the user's submission into a database. After that, find a tutorial that shows you how to pull data from the database and display it. Once you can do both of those things, creating a script where users can click on a 'Reply' or 'Comment' link should be trivial.
-
Read the message: Fatal error: Call to undefined function isLoggedIn() in ..website name.. on line 2 Notice the important parts: Fatal error: Call to undefined function isLoggedIn() in ..website name.. on line 2 Look at your line 2: if (!isLoggedIn()) Now show me where in your code you defined that function. i.e. where in your code do you have this: function isLoggedIn(){ // does something }
-
The same way you'd echo any other link, or any other text for that matter, in PHP: $url = 'http://www.yahoo.com/'; echo '<a href="' . $url . '">Click here!</a>'; The only difference is you don't have a variable named $url. You're pulling the URL from the database so it'll be something like: $sql = "SELECT ..."; $q = mysql_query($sql) or die('Error: ' . mysql_error()); while($row = mysql_fetch_assoc($q)){ echo '<a href="' . $row['url'] . '">Click here!</a>'; }
-
So what's your question?