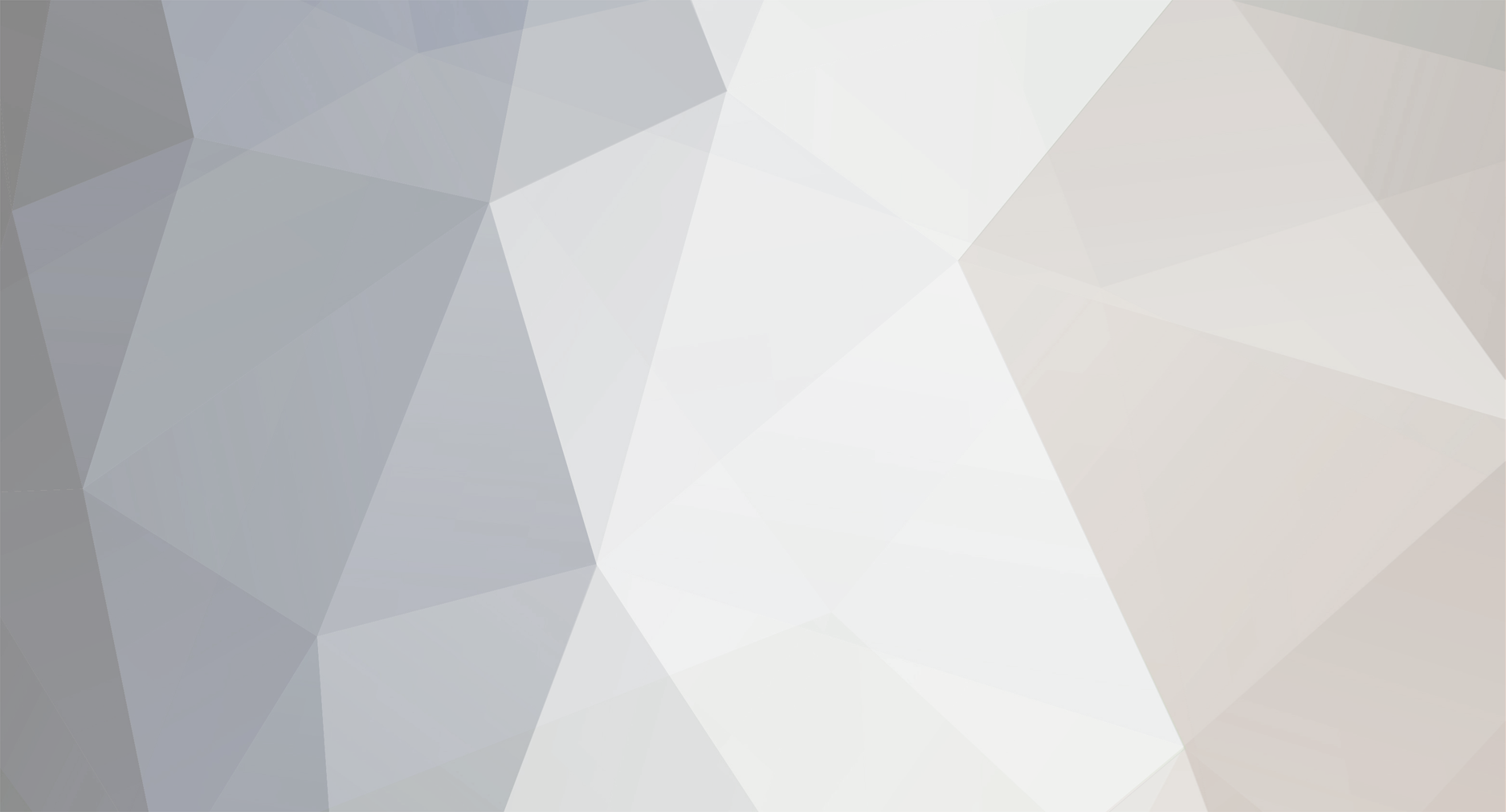
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
Try to add the `` quotes around the table name and the mls like this
[code]
$query = mysql_query("SELECT * FROM `$table` WHERE `mls` = '$MLS'") or die("Error: ". mysql_error(). " with query ". $query);
[/code]
Sometimes this does the trick for me -
If your server uses Apache, use mod_rewrite (search for it on google) in your .htaccess file. Nothing to do with php.
-
use the eval() function to run a string as PHP code, more details in [a href=\"http://us2.php.net/manual/en/function.eval.php\" target=\"_blank\"]http://us2.php.net/manual/en/function.eval.php[/a]
-
or setcookie("Login", $txtLogin), this will delete the cookie when they close the browser.
-
try this [a href=\"http://tincan.co.uk/phplist\" target=\"_blank\"]http://tincan.co.uk/phplist[/a]
-
you can use the PHP time() and date() functions if the dates are not older the 1970.
use time() when you insert the query
to display the time, use date("r", $time) use any format you want instead of the "r" and the $time will be the time you get from the database (the time you recorded).
use mktime() function to update the query with the new time
for details on the functions search for them in [a href=\"http://www.php.net\" target=\"_blank\"]http://www.php.net[/a] -
If you use a form like this
[code]
<form action="process.php" meathod="POST">
Enter your First name: <input type="text" name="first">
Enter your Last name: <input type="text" name="last">
<input type="submit" value="Submit">
</form>
[/code]
in the PHP backend, run through the post variable to output create the message like this
[code]
foreach($_POST as $key=>$value){
$message .= "$key: $value \n";
}
[/code] -
I did something like this long time ago, but I am not sure how flexiable it can be. Excel support .slk files. These files are text so you can write them in php no problem. Usually there is a head with the document format, then the rows with their data (like C;X4;K"test" or C;Y7;K6;ESUM(R[-3]C:R[-1]C) )
The easiest way to make it is to create the form in excel and save it as .slk once you get this, you can play with it to create you file. -
try to include the username in the '' quotes like 'toro'
-
I think the major advantage is that objects can inherit functions from a parent class, so you can have a base class that will do most of the work, and the rest will just extend it. It's like have the function one time or copying 500 times.
-
try to add a ; after the code
[code]
$copy = "<?PHP";
[/code] -
You server may not set up to index.php as the home page. Try to add index.html with a javascript redirection
[code]
<script>
location.href="index.php";
</script>
<a href="index.php">Click Here</a>
[/code] -
You'll need to set a javascript function to disable and enable fields when the select menu is changed.
The select menu will look something like this
[code]<select onChange="disable_fields(this.value);">
<option value="....">....</option>
</select>[/code]
In your javascript add a function -disable_fields()
[code]
function disable_fields(val){
if (val == "...."){
// disabling the field
document.getElementById('field_id').disabled = true;
}
else {
// enabling the field
document.getElementById('field_id').disabled = false;
}
}[/code] -
Just remove the background-color from the class in you style sheet.
If that doesn't work, just make another class with the different background color and use the this.className='whatever' on the mouse event. -
if you want to use the <style> tag in email, you have to put it inside the <body> tag (not in the head) most email programs remove anything above the <body> tag (including the body tag). Second, use classes for everything and don't use universal styles (like a:hover {....})
I know yahoo will put your message inside a div with an id and add #message (or whatever they add) infront of all the style rules in your style sheet. -
If the height might change based on content, you can add a background color to cover up the gab.
It's always a good idea to add a background color of the last pixel of you background image when you repeat-x or y
Something like this
[code].arttop {
background:url(images/index_08.jpg) repeat-x #186b9f;
height:70px;
margin:0;
padding:0;
}[/code] -
I agree with
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]You should really consider redesigning your website. Using absolute position is generally not a good idea and that is what is contributing to the problem.
[/quote]
Your page uses way too many tables, and the main content table is under the </body> tag -
The problem is that the link is the object with the under line (not the image). Because the image is what control the size of the link, the underline goes accrose. To fix this, you would need to make the image float in the page like this
[code]
a img {float:left;}
[/code]
You man have some issues with alignment in the pages though. -
.menu a will only effect the immediate children (in your case the UL under the div) if you want it to effect the a, you can put it directly under the div tag (without the UL and LI) but the easiest solution will be to give the links a class for themselves like .side_links {...}
-
a script can only close a window that was open by a script.
-
If you want to add images, you can use a rich content editor like this one [a href=\"http://www.fckeditor.net/\" target=\"_blank\"]http://www.fckeditor.net/[/a]
-
Have you tried domain.com/page.php?Var1=value&Var2=value2? This will send the variables as a get request. Also, you always can use sessions (in php) to store the data from one page to another.
-
instead on using the image, use a background, than change the background in the javascript.
-
If your radio buttons are dynamic, tracking the ids will be a lot more work. Here is a simple solution that doesn't require ids (only the values 'YES' and 'NO')
[code]
<script language="javascript">
function checkAll(val){
var radioBtns = document.getElementsByTagName('INPUT');
for(i=0; i<radioBtns.length; i++){
if((radioBtns[i].type == "radio") && (radioBtns[i].value == val)){
radioBtns[i].checked = true;
}
}
}
</script>
<TABLE WIDTH="100%" BORDER="1" CELLSPACING="0">
<TR><TD>Yes<BR>
<INPUT TYPE="radio" NAME="rball" VALUE="yesall" onClick="checkAll('YES');">
<BR></TD>
<TD WIDTH="6%">No<BR>
<INPUT TYPE="radio" NAME="rball" VALUE="noall" onClick="checkAll('NO');">
<BR></TD></TR>
<TR><TD><INPUT TYPE="radio" ID="rbrow1Y" NAME="rb1" VALUE="YES" ></TD>
<TD><INPUT TYPE="radio" ID="rbrow1N" NAME="rb1" VALUE="NO"></TD></TR>
<TR><TD><INPUT TYPE="radio" ID="rbrow2Y" NAME="rb2" VALUE="YES" ></TD>
<TD><INPUT TYPE="radio" ID="rbrow2N" NAME="rb2" VALUE="NO"></TD></TR>
</TABLE>[/code]
Cant get the textfield name/id
in Javascript Help
Posted
[code]
function check_date(ob){
var val = ob.value;
}
[/code]
Also you can try to change "fieldname" to something that is not generic (like fldnm) and see if it works.