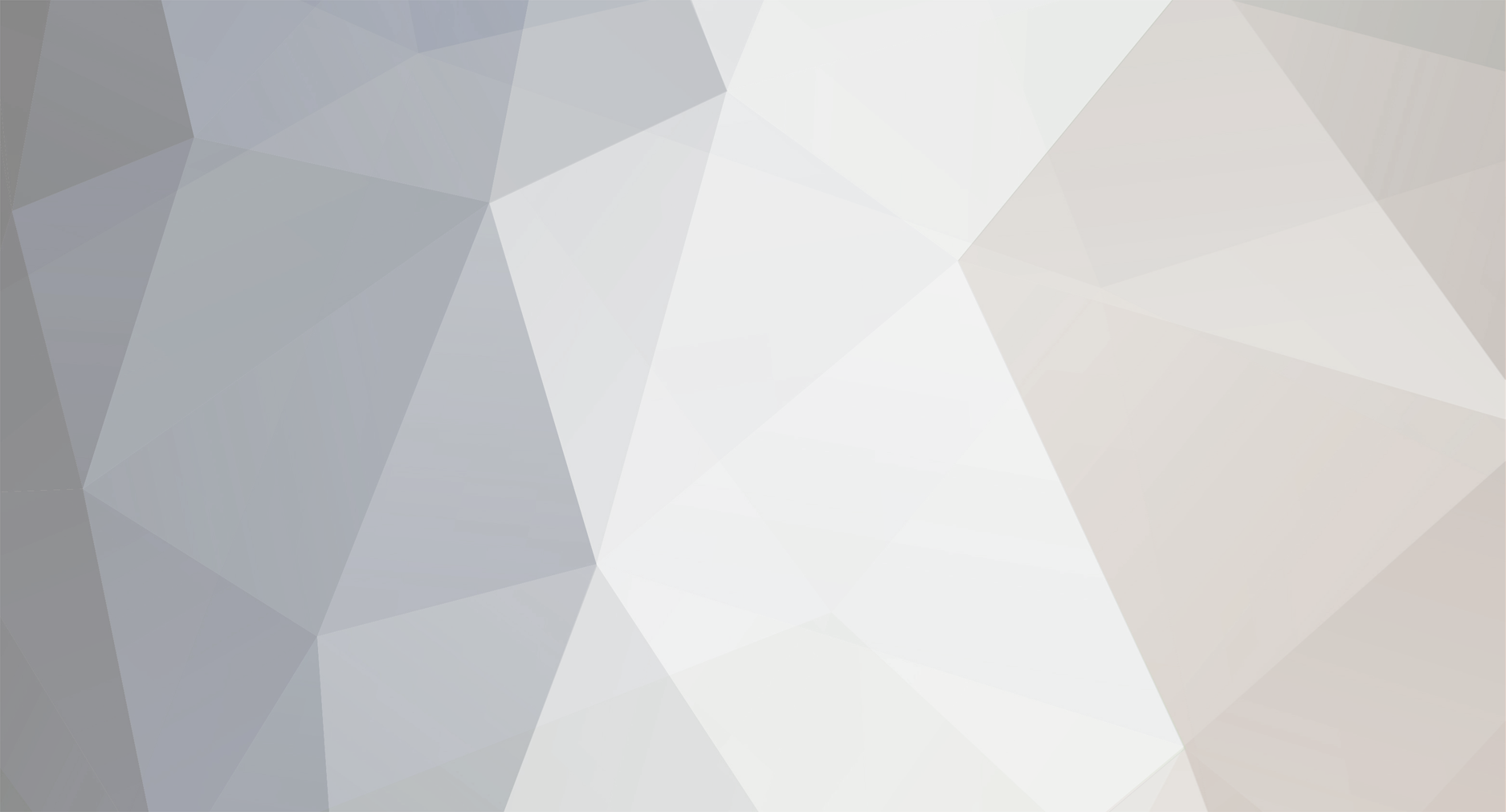
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
You'll need to set the width for all your table cells (td) in the header section. This way you force FF to use what you want.
-
you can always use a $_REQUEST instead of $_POST or $_GET. $REQUEST will work for both.
Or you can always alter the links to submit a form using javascript to fill in the start, dis and the search term. -
if you have php5, you'll be missing a ) in this like
$num=count(scandir($dir);
change it to
$num=count(scandir($dir));
if you dont, then you'll need to go through the directory and count the files. You can go to [a href=\"http://us3.php.net/manual/en/function.opendir.php\" target=\"_blank\"]http://us3.php.net/manual/en/function.opendir.php[/a] for more details regarding the open directory function, and here is a small example to count the files
[code]
<?php
$dir = "/etc/php5/";
$num_of_files = 0;
// Open a known directory, and proceed to read its contents
if (is_dir($dir)) {
if ($dh = opendir($dir)) {
while (($file = readdir($dh)) !== false) {
if (is_file($dir . $file)) $num_of_files++;
}
closedir($dh);
}
}
echo "There are $num_of_files file(s)";
?>
[/code]
make sure the dir is the correct path. -
make sure the $cards is declared as an array somewhere in your script. I am not sure if you have it in the 'cards.php' file or not. But if you don't have it there, and it's not a global variable that is set as an array, you'll need to add
$cards = array();
before you use it in the array_search() function
-
My space won't allow you to include a javascript in your file. For a counter, you'll need to use a php image file like <img src="http://www.domain/my_count_script.php" />
Also, if you want to count the unique, return, and number of pages, you'll need to generate a PP3 (I think that what's called) privacy policy that is sent with the header in your image. This way, the browser can except your cookies.
If you want, you can click on my signture and check out the "Free Tracking" service. -
You can use a check box instead of the radio button like this
Example check box
[code]
<input type="checkbox" name="Department_name" value="Yes" /> Department Name
[/code]
in you php, you just need to add the extra email address to the "To"
[code]
$to = "your_email@domain.com";
if ($_POST['Department_name'] == "Yes"){
$to .= ", department_email@domain.com";
}
[/code]
Let me know if you need radio buttons and an example of your form if you need more help. -
I agree with poirot that the script is a resource hog and if there are a lot of people running at the same time, it may crash your server.
But you always can flush the output by using [a href=\"http://us2.php.net/manual/en/function.flush.php\" target=\"_blank\"]flush()[/a] This way the user will start seeing data before the script is done processing. -
you need to add this to the top of your page (under $category = $_GET['category'];)
[code]
$page = $_GET['page'];
[/code]
-
set the style "overflow:hidden" so the extra text won't show up.
-
too complicated for just opening a search page.
Just use the "target=_blank" in the form and you don't need any javascript
[code]
<form method="get" action="http://www.google.com/search" target="_blank" name="form1">
<input type="hidden" name="hl" value="en" />
[/code]
If you still want to use javascript
you'll need to use (document.getElementById('put your field box id here').value) instead of the (document.form1.search.value) and value shouldn't have () after it. -
you can trying something like this. Let's say you are passing ID as a variable to page.php?ID=3
[code]
<?PHP
$iframe_link = "iframe_page.php?ID=".$_GET['ID']
?>
<iframe src="<?= $iframe_link ?>"></iframe>
<button onclick="window.close();">Close</button>
[/code]
this should pass the ID variable to the iframe page. -
I don't see any 1s but try this
"link"=>"<A HREF=\"profile.php?id=".$row['id']."\"><IMG SRC=\"pictures/".$row['gallery1']."\" WIDTH=\"132\" HEIGHT=\"175\" BORDER=\"0\"></A>", -
I found a few websites that offers alternative service to cron a while back, but I never tried them. you can search Cron Service on google to find more details. Here are two quick links
[a href=\"http://www.fastcron.com/\" target=\"_blank\"]http://www.fastcron.com/[/a]
[a href=\"http://www.webbasedcron.com/\" target=\"_blank\"]http://www.webbasedcron.com/[/a] -
I don't think so, but I am not 100% sure.
Maybe if you tried to load the page content in an iframe and the button on the page itself. -
You'll need to use the attachEvent for IE and addEventListener for FireFox. Check this page out for more details
[a href=\"http://www.captain.at/howto-addeventlistener-attachevent-parameters.php\" target=\"_blank\"]http://www.captain.at/howto-addeventlisten...-parameters.php[/a]
You still need ids for your divs -
You only can close a window if it was open by a script. Once the page is reloaded (or the URL is changed) the page is not considered to be opened by a script anymore, so the self.close() won't work.
-
your shop script uses relative paths, so it'll always look for the files in the same folder (not the shop folder) if you include it in the index.
You can try to use file_get_contents('../shop/index.php') function which will run the '../shop/index.php' and return the HTML output.
Otherwise, you'll need to change you shop files to use absolute paths -
you can also call the php from your cron job "php /path/to/your/php/script.php"
and you need the path to the php compiler (I think that what it's called) in the tob of you php file "#!/usr/local/bin/php -q" -
check [a href=\"http://us2.php.net/manual/en/function.time.php\" target=\"_blank\"]time()[/a] and [a href=\"http://us2.php.net/manual/en/function.mktime.php\" target=\"_blank\"]mktime()[/a]
You can use the [a href=\"http://us2.php.net/manual/en/function.date.php\" target=\"_blank\"]date()[/a] function to format the date or time -
Height is a mystery for all browsers and CSS (none of them treat it the same way). If you want to use height:100% you have to set all the parent elements height (including the body and html)
What I usually do when I have a lot of column is to set the height using javascript. when the page load, I get the window height and the three columns, than set the height to the highest amount. -
I never done it personally, but you can use the .htpasswd to create a password protected directories. You can search for it online for more details.
Here are a couple of links I found.
[a href=\"http://koivi.com/php-http-auth/\" target=\"_blank\"]http://koivi.com/php-http-auth/[/a]
[a href=\"http://elonen.iki.fi/code/misc-notes/htpasswd-php/index.html\" target=\"_blank\"]http://elonen.iki.fi/code/misc-notes/htpasswd-php/index.html[/a]
-
try this
list($width, $height, $type, $attr) = getimagesize($pic_directory.$picture);
you'll get the $width and $height as variables. Also make sure all you paths are correct and there are actuall images. -
Well, I am not really sure if this is the best solution, but since you have php, html, javascript, and css all stored togather I don't think you can do anything else.
Try to write the $body content to a file, and than include that file in an include("new_file.php");
If the content of the body will change for different users and over time. You can delete the file when the page is done processing.
Good luck -
instead of print ($body); use eval($body);
if php stored as a string, you need to use the eval function
[a href=\"http://us2.php.net/manual/en/function.eval.php\" target=\"_blank\"]http://us2.php.net/manual/en/function.eval.php[/a]
PHP pages not updating?
in PHP Coding Help
Posted