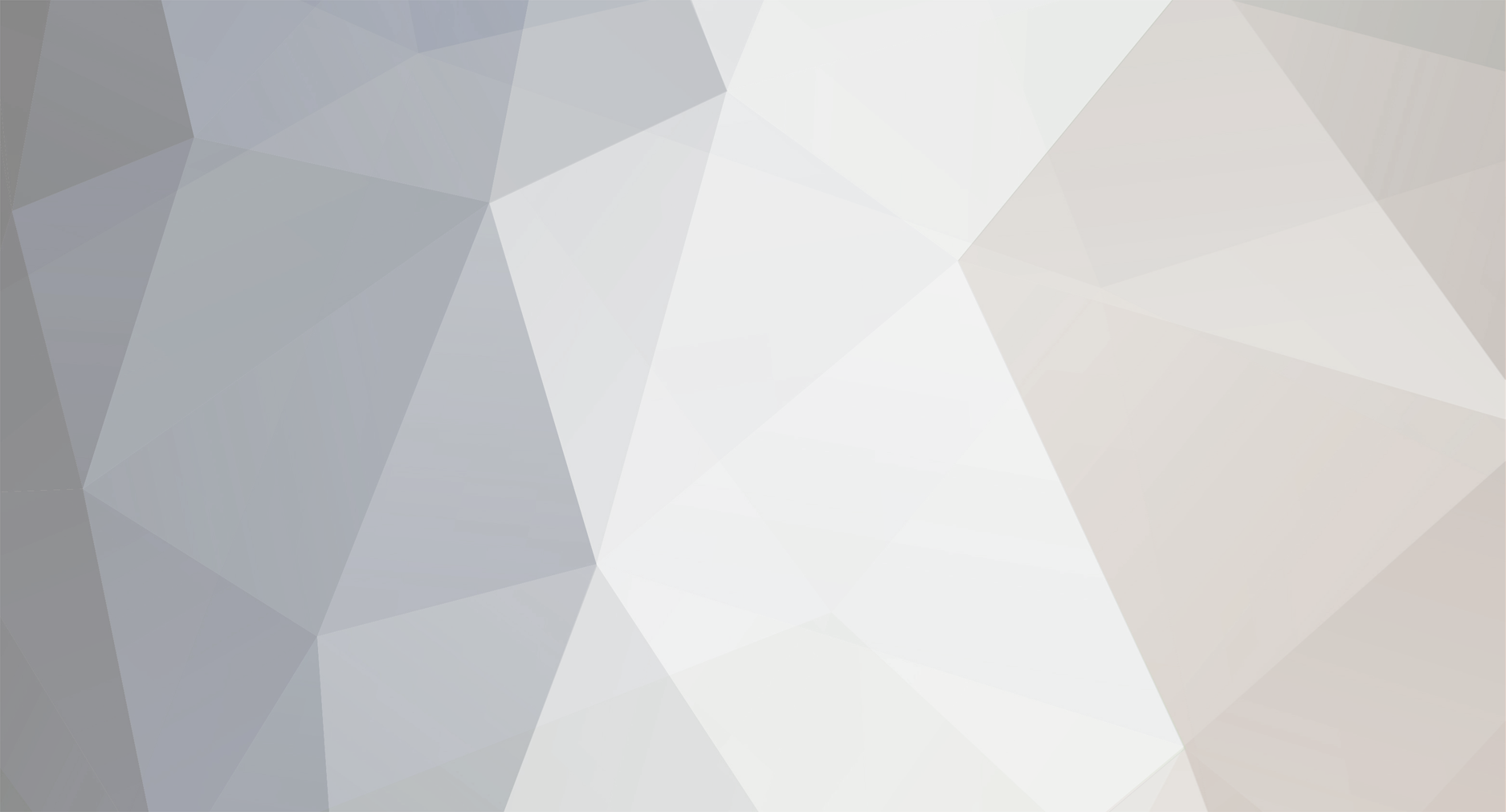
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
try to use the `` around the $division and use mysql_error() to see if there are any errors
[code]
$sql = "SELECT * FROM spareplayers
WHERE `$division` = 'TRUE'
AND `position` = '$position'
ORDER BY `lastName`";
$result = @mysql_query($sql) or die(mysql_error());
[/code]
maybe it'll work -
Now I c why the display=''; doesn't work
display='' will set the display to the defult value defined in the #Loading (which is none), you'll need to take out the display:none from the #Loading defintion and put it in the <div> tag, like this.
[code]
<style>
#Loading {
z-index:12;
color:#fff;
background:#D31858 url(../images/loading.gif) 12px 50% no-repeat;
padding:8px 20px 8px 40px;
position:absolute;
bottom:0px;
right:0px;
cursor:default; }
</style>
<div id="Loading" style="display:none;">
Loading
</div>
<a href="#" onClick="document.getElementById('Loading').style.display = '';">Show</a>
<a href="#" onClick="document.getElementById('Loading').style.display = 'none';">Hide</a>
[/code]
the bottom:0px; right:0px; will place the element at the bottom of the screen (not the page) so, if the page is not scrolled all the way to the bottom, the div won't be in the bottom. This could be a good thing because the user will always see the loading div ;) -
You can float the login button to the right and place it first.
[code]
<form>
<table><tr>
<td>Username:</td><td><input type="text" /></td>
</tr><tr>
<td colspan="2">
<input type="submit" name="login" value="Login" style="float:right;">
<input type="submit" name="lostpass" value="Lost Password">
</td>
</tr>
</table>
</form>
[/code]
Make sure the table is big enough to fit both buttons. -
the display = ''; should work, but sometimes it doesn't.
However, I think once the element (div) is placed in a page, it's location won't change unless you move it using javascript.
You can set a javascript to move the object to the bottom right.
BTW, I am interested in knowing how you style the div to show in the bottom right. -
You don't actually need to float the images, you can just put them one next to the other and add a <br /> at the end of the line.
IE usually put a small margin next to the floated element, if you still want to float them, you can try this
[code]
.floatLeft {
float: left;
_margin-left:-5px;
}
[/code]
I am not sure if it'll work as you want, you may have to change the -5 to something else.
Oh yeah, Line breaks are considered a space in IE, and if there is no <br /> or a closing tag after the image, you'll get a small margin below the image. -
You can orginze your data in folders so sorting and getting the info will be easier (without a database)
for example
for each new car, you make a directory with the car name (for example hona_civic)
When he uploads the images (each car pictures will go into its' own folder) save them with specific names (like pic_1.jpg pic_2.jpg, pic_3.jpg etc)
and save the description as files in the same folders (like desc_1.txt, desc_2.txt, desc_3.txt)
This way each picture will have it's own description.
Hope this helps. -
Even if the javascript opened the window, you cannot get any elements from the new window if it was in a different domain. For example, if you are trying to get the HTML code, or a input value. You'll get "Access Denied" error.
Here are general restriction when opening a window, from MSDN
[a href=\"http://msdn.microsoft.com/library/default.asp?url=/workshop/author/dhtml/overview/window_restric.asp\" target=\"_blank\"]http://msdn.microsoft.com/library/default....dow_restric.asp[/a]
If the application was a desktop application (or a PC) application, it'll be considered as a trusted application so there are less restrictions.
You can try to create an HTA file (normal HTML, just rename it to something.hta) which is a trusted html application. It'll have less restrictions, and you can run it from you PC. I am not sure if you can access remote sites with it or not.
[a href=\"http://msdn.microsoft.com/library/default.asp?url=/workshop/author/hta/overview/htaoverview.asp\" target=\"_blank\"]http://msdn.microsoft.com/library/default....htaoverview.asp[/a] -
you can use an onclick event, like this
[code]
<table class="tablestyle">
<tr class="rowA" onclick="location.href='some_page.php';" style="cursor:pointer;"><td class="cellA">Link</td></tr>
<tr class="rowB" onclick="location.href='some_page.php';" style="cursor:pointer;"><td class="cellB">Link</td></tr>
</table>
[/code]
The style style="cursor:pointer;" will make the mouse change to a finger so it looks clickable. -
If you want to make it scrolls in steps, you gotta make your own scroll bar using javascript.
It'll be a bit complicated and you gotta test it from IE and FF (work differently).
Here is a simple logic that might work
in your list, each item should be an element with and id (like <div id="right_1">Text</div><div id="right_2">Text</div>etc...)
create two images to represent the scroll arrows.
When the top arrow is clicked, you'll need to check how many clicks it's been (keep a counter in javascript) and than scroll into view the corresponding element from that click to the top.
You can use scrollIntoView() function to scroll the element [a href=\"http://msdn.microsoft.com/library/default.asp?url=/workshop/author/dhtml/reference/methods/scrollintoview.asp\" target=\"_blank\"]http://msdn.microsoft.com/library/default....ollintoview.asp[/a]
do the same with the bottom arrow, but you'll need to scroll the previous element into view. -
use the code Crayon Violent suggested and print out any errors, like this
[code]
$query = "INSERT INTO books (isbn,author,title,price) VALUES ('$isbn','$author','$title','$price')";
$result = mysql_query($query) or die(mysql_error());
[/code]
The error message should tell you what's wrong, if you need more help, you can post it here. -
you can replace the ' with & # 3 9 ; using str_replace (without the spaces)
This will print out the ' in any browser. -
[a href=\"http://us3.php.net/manual/en/function.strval.php\" target=\"_blank\"]strval()[/a]
-
you can use something like this
[code]
<input type="radio" name="Email" value="Dep1" /> Dep1
<input type="radio" name="Email" value="Dep2" /> Dep1
<input type="radio" name="Email" value="Dep3" /> Dep1
<input type="radio" name="Email" value="Dep4" /> Dep1
[/code]
and your php
[code]
$to = "your_email@domain.com";
if ($_POST['Email'] == "Dep1"){
$to .= ", dept1_email@domain.com, dept1_email@domain.com, dept1_email@domain.com, dept1_email@domain.com";
}
else if ($_POST['Email'] == "Dep2"){
$to .= ", dept2_email@domain.com, dept2_email@domain.com, dept2_email@domain.com, dept2_email@domain.com";
}
else if ($_POST['Email'] == "Dep3"){
$to .= ", dept3_email@domain.com, dept3_email@domain.com, dept3_email@domain.com, dept3_email@domain.com";
}
else {
$to .= ", dept4_email@domain.com, dept4_email@domain.com, dept4_email@domain.com, dept4_email@domain.com";
}
[/code]
Hope this helps you.
-
Scripts cannot control a page or a window on a different domain (security risk).
-
Pretty interesting problem....
Here is a little trick that works in IE and FF, doesn't work in Opera. I used the dir="rtl" in the first div so the scroll bar will move to the left side of the div. This way it's not between the two lists.
[code]
<style>
.list {width:100px;
height:100px;
overflow:auto;
float:left;
padding:3px;}
</style>
<div class="list" dir="rtl">
List 1<br />
Black<br />
Red<br />
Angry <br />
Happy<br />
Vulgar<br />
</div>
<div class="list">
List 2<br />
Devil<br />
Fish<br />
Nanny<br />
Dog<br />
Jungle<br />
</div>
[/code] -
The line number is not accurate all the time, usually the error is around that line, so check the lines above and under.
if you want ot replace the " " use the str_replace(" ", " ", 'Your text'); -
the a:3:{i:0;s:6:"sb1253";} looks like a serilized array, check these pages out
[a href=\"http://us3.php.net/manual/en/function.serialize.php\" target=\"_blank\"]serialize()[/a]
[a href=\"http://us3.php.net/manual/en/function.unserialize.php\" target=\"_blank\"]unserialize() [/a] -
use [a href=\"http://us3.php.net/manual/en/function.file-get-contents.php\" target=\"_blank\"]file_get_contents()[/a], much easier
-
I think you need a constructor function that will assign the values to your class variables.
The constructor function in php 4 must be the same name as the class "function DB_Connect()"
in that function assign the values of the $server....... -
[a href=\"http://us2.php.net/manual/en/function.microtime.php\" target=\"_blank\"]http://us2.php.net/manual/en/function.microtime.php[/a] have an example of getting the exection time in php 4 and 5
-
When I organize data, I got from the outside to the inside. I am not sure if your doctors can have multiple locations, but that how will I do it.
Location:
ID, fields......
Doctors:
ID, Location, fields...... // Location is the location ID from the location table
Procedures
ID, Location, fields..... // Location is the location ID from the location table
and now to link the doctors to the procedures
Doctors_x_Procedures
ID, Doctor, Procedures // Doctor is the doctor ID from the location table. Procedures is the procedures ID from the procedures table.
-
I didn't got through the whole thing, but if you want to change a class, you can use className
[code]
document.getElementById('id here').className = 'class name here';
[/code] -
try this
[code]
<script language="javascript">
function hide(){
var sub_menus_array = new Array("sub1","sub2","sub3"); // sub menu ids
for(i=0; i<sub_menus_array.length; i++){
document.getElementById(sub_menus_array[i]).style.display = "none";
}
}
function show(sub_menu){
hide();
document.getElementById(sub_menus_array[i]).style.display = "block";
}
<script>
<a href="#" onClick="show('sub1');">MainMenu1</a>
<div id="sub1" style="display:none;">
MM1_SubMenu1
MM1_SubMenu2
</div>
<a href="#" onClick="show('sub2');">MainMenu2</a>
<div id="sub2" style="display:none;">
MM2_SubMenu1
MM2_SubMenu2
</div>
<a href="#" onClick="show('sub3');">MainMenu3</a>
<div id="sub3" style="display:none;">
MM3_SubMenu1
MM3_SubMenu2
</div>
[/code] -
You can always have the the style property to display:none; on the images that you want to show and hide.
[code]
<img src="image source" border="0" alt="text here" style="display:none;" id="Img_ID" />
[/code]
the id is importent.
add a function in your javascript
[code]
function show_image(img_id){
document.getElementById(img_id).style.display = "block";
}
function hide_image(img_id){
document.getElementById(img_id).style.display = "none";
}
[/code]
Then you can show any image by calling the show_image function with the correct id. For example using a onMouseOver event
[code]
<img src="some image" onMouseOver="show_image('Img_ID');" />
[/code]
This will show the image with the id "Img_ID". All images have to have uniqe ids.
for the noscript, it'll work. IE will not turn off javascript on your computer (I haven't found a way to do so), test your page on Firefox if you want to see the <noscript> works on your computer.
variables precendance and descendance
in Javascript Help
Posted