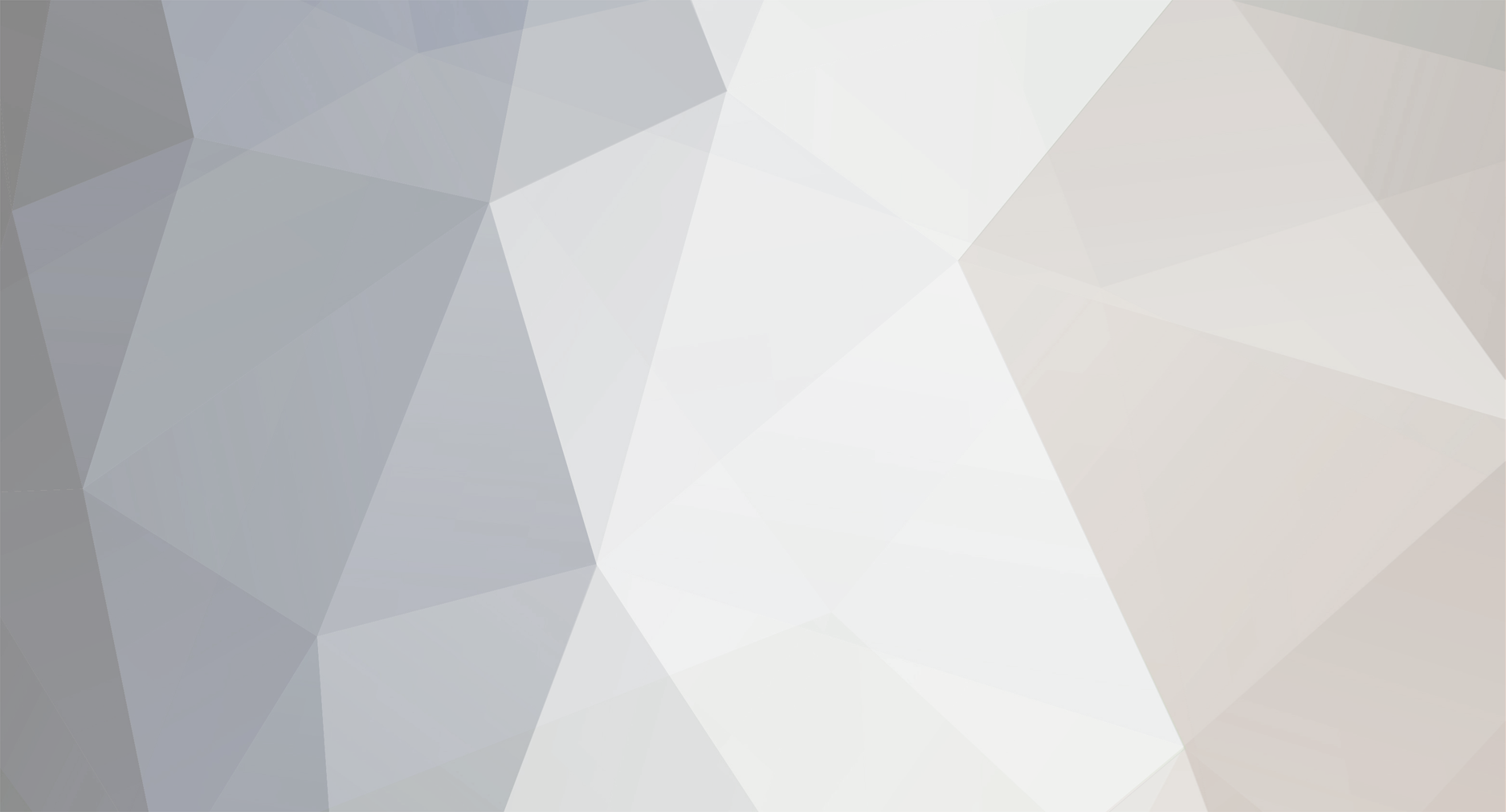
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
OK, yes you *could* build your query to return either the image name or the pending image name based upon the "approved" status (just use an IF condirion in the select statement). But, I would try to keep business logic out of the data abstraction processes. But why would you need to run two queries anyway? Just run one SELECT query to return the image name and the approved value. Then in your business logic (i.e. PHP code) check the approved value to determine if you will display the user submitted image or the pending image. Simple Example function getImage($userID) { $query = "SELECT image_name, approved FROM images WHERE userID = '$userID'"; $result = mysql_query($query); if(!mysql_num_rows($result)) { return false; } $row = mysql_fetch_assoc($result); $imageName = ($row['approved']!=1) ? 'pending.jpg' : $row['image_name']; return $imageName; }
-
@requinix: I have reported your post for the use of vulgar, obscene language which is in violation of the Terms of Service jk, of course
-
Did you try it? Here's a simple test. $input = "This is input with <script type=\"javascript\"> alert('JavaScript'); </script> code."; echo strip_tags($input); And, a simple Google search for "PHP prevent XSS attacks" yielded plenty of good resources.
-
It was an error in the code that was generating the output, wasn't it?
-
It is very difficult to try and debug database issues such as this without first hand understanding of the data. Please show the complete record from the users table and the associated record from the refer table that should be associated with one another. Did you test the query in PHPMyAdmin or some other DB utility before trying to run it and output the results in PHP?
-
Ah, I left off the JOIN condition. Try this $query = "SELECT u.reg_date, u.nick, u.email FROM ". $DBPrefix."users AS u JOIN ". $DBPrefix."refer AS r ON u.email = r.email WHERE r.referrer = '$userName'";
-
Lay out what features you want to create in order of importance then attack one thing at a time. You should remove AJAX from the equation for now since most of what you will need to develop wouldn't require it. I would start by create a process/function to create the map based up coordinates given. Just create a page where you can enter the coordinates and click a button to generate the map - or put the coordinates into the query string. Then determine how you want the navigation to work. If the coordinates are sent through POST values then each array will need to be a form input control and you will need hidden field to provide the current position and the direction change or just the new coordinates you want. If the coordinates are sent through the query string then you just need the nav controls to be links to get the new map. Once all that is working, THEN implement AJAX to call the new map without a page load.
-
This forum has [ code ] tags that you can put around code so it displays in a readable format - please use them. You don't even have line breaks in what you posted. But, from what I see the code is a mess. The problem when you see these errors is that the query failed - therefore any function that tries to use the results of the query will produce errors. You need to implement error handling into your code. Below is a rewrite of your code. I changed a lot and I'm not going to take the time to explain why I changed what I did. But, you can ask specific questions if you want. <?php //Process the input (if exists) $searchTermsStr = isset($_POST['search']) ? trim($_POST['search']) : ''; $searchOutput = ''; //Var to hold the output //Verify there was a valid input if (!empty($searchTermsStr)) { // connect mysql_connect("localhost", "username", "password"); mysql_select_db("databasename"); //Create an array of search terms (filter out empty values) $searchTermsAry = array_filter(explode(" ", $searchTermsStr)); //Process terms array into Query strings foreach($searchTermsAry as $key => $value) { $value = mysql_real_escape_string($value); $searchTermsAry[$key] = "keywords LIKE '%$value%'" } //Create and run query $query = "SELECT url, title, description FROM search WHERE " . implode(' OR ', $searchTermsAry); $result = mysql_query($query); //Verify query results if(!$result) { $searchOutput .= "There was a problem running the query:<br><br>"; $searchOutput .= "Query: $query<br><br>"; $searchOutput .= "Error: " . mysql_error(); } elseif(!mysql_num_rows($result)) { $searchOutput .= "No results found for '<b>{$searchTermsStr}</b>'"; } else { while ($row = mysql_fetch_assoc($query)) { $searchOutput .= "<h2><a href='{$row['url']}'>{$row['title']}</a></h2> {$row['description']}<br /><br />"; } } //disconnect mysql_close(); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Search Engine</title> </head> <body> <center> <h1>Search</h1> <form action='search.php' method='post'> <input type='text' name='search' size='90' value='<?php echo $searchTermsStr; ?>' /> <input type='submit' name='submit' value='Search' > </center> </form> <hr /> <?php echo $searchOutput; ?> </body> </html>
-
exctract entire lines from text file to array
Psycho replied to Inspector Mills's topic in PHP Coding Help
As I stated I would want to analyze the actual files before I set upon a final solution. But, with all due respect, your solution doesn't meet the requirements regarding the required "post" lines. You only check that the line is preceded by " -------". Based upon the actual data that might be sufficient, but I didn't want to make that assumption based upon the requirements the OP posted. -
You would never want to "hide" the content using style properties. You should instead determine of the user is a normal user or admin and then only output the relevant content. $userType = {SOME PROCESS TO DETERMINE USER TYPE }; echo "This is output for all users"; if($userType =="ADMIN") { echo "This is output for all users"; }
-
exctract entire lines from text file to array
Psycho replied to Inspector Mills's topic in PHP Coding Help
If I was able to analyze some test files I might be able to produce a more efficient regular experssion, but based upon your explanation and example, this should work $input = file_get_contents($filePath); preg_match_all("# -*\r\n\s([^\r]*)\r\n\r\n-*#", $input, $matches); print_r($matches[1]); That will work on a windows server, for a unix server I think you need to user just "\r" instead of "\r\n" preg_match_all("# -*\r\s([^\r]*)\r\r-*#", $input, $matches); -
exctract entire lines from text file to array
Psycho replied to Inspector Mills's topic in PHP Coding Help
How big is the file and how often would this be run? The simplest solution, IMHO, is a file_get_contents() and the a preg_match_all() regex to get all those lines. But, if this is a large fine and/or would be run many times such that performance is an issue then using the other file reading functions and string functions may be more efficient. -
Or even $myArray = array(); $res = mysql_query("SELECT server,site,status FROM mytable"); while ($myArray[] = mysql_fetch_row($res)) { } Although I don't really advise that - I think having a loop with nothing in it is just odd. But, anyway, do you even need to do this? You already have the results from the query and all this would do is take those same results and put into an array. There are some scenarios where this would make sense, but most time you can just process the results directly from the query.
-
Is there a way to do this without using a Query Loop?
Psycho replied to Failing_Solutions's topic in PHP Coding Help
I could provide you a solution to what you have now, but . . . . . . you need to change that design. Especially the fields for supplier_model1, supplier_model2, etc. That is unsustainable. I think I know why you did that. I assume the reason you are doing that is that you are getting the same parts from different suppliers who have their own part numbers. If that is the case you need two tables to "describe" the parts. I would have something like this: Table: parts This table would contain fields to describe the common features of the part. It would contain only YOUR part number as well as an auto-increment ID field. Table: parts_suppliers This table would contain a record for every part that each supplier provides. So, if part #1 has 3 suppliers there would be three records. The table would only need the auto-increment ID field from the parts table, the supplier part number and a supplier ID (ideally there would be a separate table to list the suppliers). This table should also use an auto-increment ID field. Now, as for stock. If you need to store the inventory by the supplier then you just need to store the ID from the parts_suppliers table along with a quantity. Using the above structure you could get the total quantity for all parts (per YOUR part number) using a query such as: SELECT parts.part_no, parts.name, SUM(inventory.count) as inventory FROM parts LEFT JOIN parts_suppliers ON parts.part_id = parts_suppliers.part_id LEFT JOIN inventory ON parts_suppliers.part_sup_id = inventory.part_sup_id GROUP BY parts.part_id -
I'll concur with the hidden input suggestion. Using a session *can* work, but it would create problems if users open pages in multiple tabs/browsers.
-
Yes, that is the problem. That line is getting the first record from the database - but it is not used. Then there is a while() loop that starts by getting the second record and displays it. The while loop will then get and output all the subsequent results. In 99% of scenarios you are just going to get the records using the while() loop.
-
You should build your pages without JS and then add JS to enhance the experience. Yes, you can work around the problem of the dynamic text boxes, but it will require having the page load with just the first option along with a submit button. You could do this one of two ways. Have the page load with the all the content but define the style property of the 2nd select and the rest of the form as hidden/disabled. Once the page loads use JS to unhide that content. So, if the user does not have JS enabled they will only see the 1st select and the submit button. If the user has JS enabled they will see all the content. Then you need to modify the processing page to detect when a selection/change is made to the first select as opposed to a compete form submission. Basically, it will get a whole lot more complicated. So, another approach is to do a JS check (typically by trying to set a cookie with JS and checking it with PHP). If the user doesn't have JS enabled give them an appropriate error message and don't allow them to use the site. It all depends how important it is to you that the site work for users w/o JS enabled. With smart phones becoming more common for navigating the net it is starting to become more important where it was almost negligible.
-
And why would you NOT make it a submit button? You can and should use a submit button. If you want specific JS to execute upon submission, the correct way to implement it is with an onsubmit() trigger to call your validation functions. If validations pass the function should return true, else it should return false. Form tag should look something like this. Be sure to use the "return functionName();" format. The use of return allows to you allow/prevent the actual submission based upon the return value of the function. <form name="myForm" action="processingPage.php" method="post" onsubmit="return validateForm(this);"> Then your function will do the validations and return false if there are problems, else it will return true. function validateForm(formObj) { if(formObj.elements['name'] == '') { alert('The name field cannot be empty'); return false; } if(formObj.elements['email'] == '') { alert('The email field cannot be empty'); return false; } return true; } That is just a rough example for explanatory purposes. I would always create my validation logic to check for all possible errors and display then instead of exiting after the first error. Using the above format, if a user has JS enabled, then that functionality will execute. If not, the form will submit normally and the server-side validation will ensure there are no problems.
-
The output doesn't look right even if you had multiple records returned (the first record has an invite date but no reg date?). You should always test and debug your queries in MySQL - not in the PHP code. You may have a bug in the code that produces the output.
-
Well, something doesn't make sense. The query look s fine, but according to the error message there is an errant space between "Auc_" and "refer". Are you sure you built the query correctly?
-
Well, you are doing it wrong. You need to learn how to do JOINS in your queries. that is the whole point of having a relational database. But, before I give you a possible solution I do have one comment. Your current structure relies upon the fact that the person registering is using the same email as the one in which they received the referral. I use many different email addresses for different purposes. Just because someone sends me a referral on one email address doesn't mean I will want to resister on that email. A better approach, IMHO, is to have a referral code sent to the user. Then you could JOIN on that code. Also, depending on the collation you are using for your email fields you may or may not be able to JOIN the email fields. Also, why are you using the use's username in the referrer table instead of an ID? I think you shoudl take some time and do some reading on database schemas. This query should show you all the information relevant to the referrals made by a particular user. SELECT u.reg_date, u.username, u.email FROM users AS u JOIN refer AS r WHERE r.referrer = '$userName'
-
This topic has been moved to Application Design. http://www.phpfreaks.com/forums/index.php?topic=360382.0
-
You should ALWAYS validate and sanitize user input. You can add JavaScript validation to provide a real-time feedback to the user. But, it should only be implemented in addition to the server-side validation logic.
-
How to avoid data duplication after page refresh
Psycho replied to believeinsharing's topic in PHP Coding Help
On the processing page that receives the form submission you simply need to do a header redirect to a confirmation page or even back to the same page. That will "wipe out" the form data. So, if a user refreshes the page they will simply see the confirmation page and no processing takes place. //Process the form data //Processing logic goes here //After processing logic completes redirect to confirmation page header("Location: confirmation_page.php"); -
The checkdate() function is a built-in PHP function. I provided a link to the documentation for that function that explains what it does, what parameters it takes, the possible return values and even provides a couple examples of how to use it. I'm not sure I could do as complete a job of explaining it in this type of venue than the manual already provides. If there is something you don't understand about the documentation, then by all means ask that question and we will attempt to help. But, you have to be an active participant in the process.