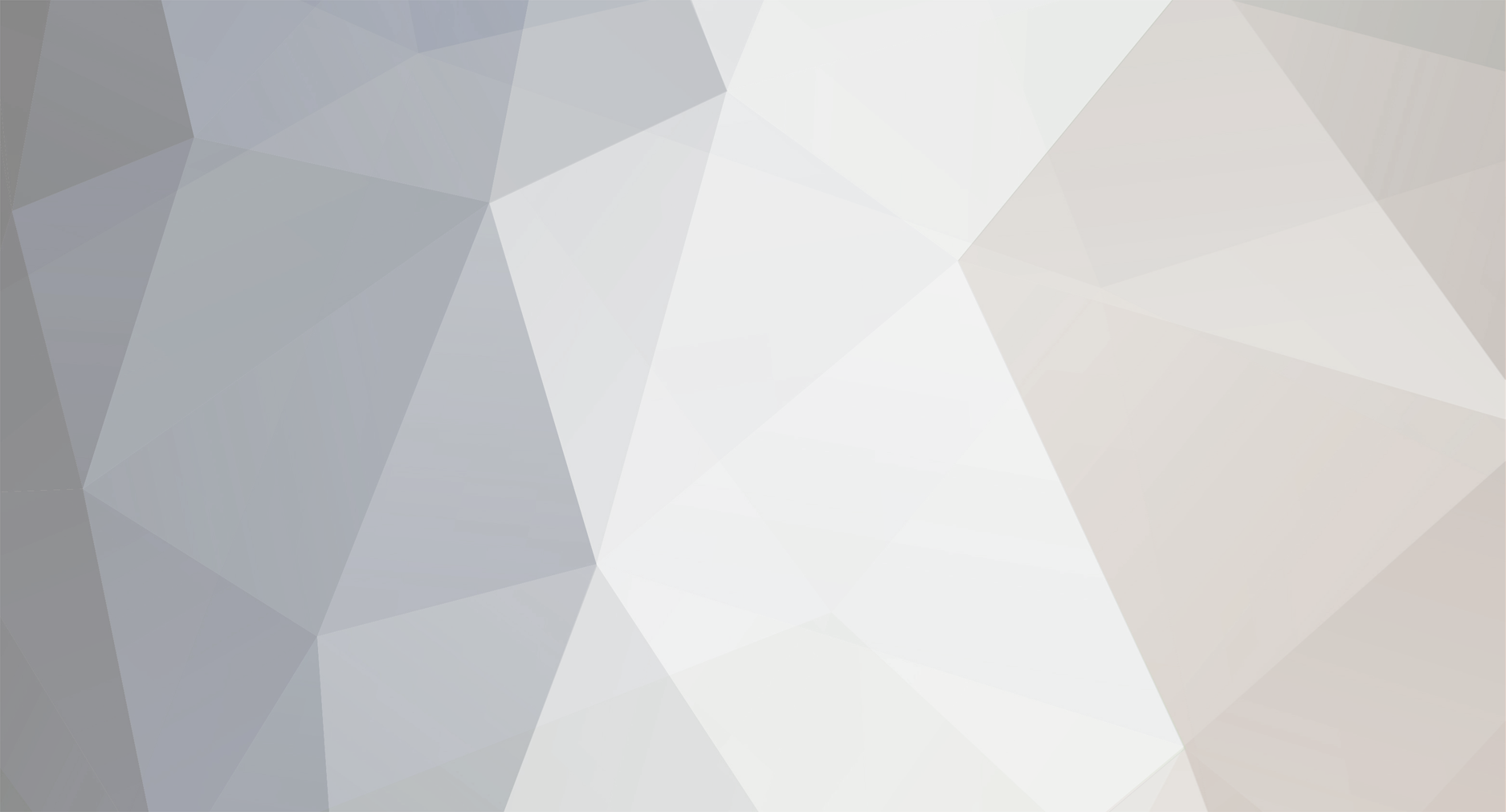
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
select multiple values from mysql between intervals
Psycho replied to charles07's topic in PHP Coding Help
yes it is http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html#function_timediff Yeah, I saw that, but the value returned is in the format '46:58:57.999999' which would still require PHP functionality to convert that into a usable format. So, I'd still stick with doing the calculation in PHP. -
The level of "security" is wholly dependent upon the "needs" of the form. There is no need to eliminate the HTML form - even a form generated from PHP is still going to be an HTML form when it is sent to the user. Here are some rough guidelines from my personal experience. 1. Always ensure you have full coverage on any security implemented on the back end (i.e. PHP). Any, validation you implement on the client-side (i.e. JavaScript) is great for giving the user immediate feedback and a richer user experience but it does not negate the need for the same validation server-side since JavaScript can be disabled 2. Always treat ALL user input as potentially malicious. Just because you have a drop-down field with three specific values does not mean a malicious user cannot actually submit a different value. 3. Always validate/sanitize values as appropriate for their data type. All values in a form are passed as strings, but you should validate based upon the data type that you will store them as: Strings, ints, floats, dates, etc. 4. As a general rule you should always trim() user input. Otherwise a value of a space would pass a required field validation. 5. Also, in my opinion, unless you have a specific reason not to, I would not run functions such as htmlentities(), strip_tags() etc on the input before it is stored. You can safely store the data as the user entered it and then you can use the appropriate functions when outputting the data to make it safe. A function such as strip_tags() would remove content that the user entered. That could cause problems if they are unaware of it (e.g. the username is not the same as they thought it was). And, using such as htmlentities() will increate the string length of the input causing potential problems with max length checks and database field lengths. For your purposes I would think the most important things are ensuring data entered can safely be stored and to reduce the potential for bots. Bots would only be a potential issue if the form can be used by users who are not authenticated (i.e. a login system). Even then, a bots are really only used to enter data on forms - such as forums - so the data will be seen by people. I would concentrate on securing the data. Then worry about preventing bots. Having said all that, I typically like to create my forms such that they post back to themselves for processing. That way, if there are errors, I can redisplay the form with the previously entered data. Example script <?php $errorMsg = ''; //Check if form was posted if($_SERVER['REQUEST_METHOD']=='POST') { //Preprocess input $name = isset($_POST['name']) ? trim($_POST['name']) : false; $phone = isset($_POST['phone']) ? trim($_POST['phone']) : false; $account = isset($_POST['account']) ? trim($_POST['account']) : false; $meter = isset($_POST['meter']) ? trim($_POST['meter']) : false; //Validate user input // - validations will be based on your specific needs, these are examples $errors = array(); if(empty($name)) { $errors[] = "Name is required"; } if(empty($account)) { $errors[] = "Account number is required"; } elseif(!preg_match("#^\d{8}$#", $account)) { $errors[] = "Account number must be an 8 digit number"; } if(empty($meter)) { $errors[] = "Meter number is required"; } elseif(!ctype_digit($meter)) { $errors[] = "Meter reading must be a number"; } //Check if there were any validation errors if(count($errors)) { $errorMsg .= "The following errors occured:<ul>\n"; foreach($errors as $err) { $errorMsg .= "<li>{$err}</li>\n"; } $errorMsg .= "</ul>\n"; } else { //All validations passed, insert record //Ideally the DB connection data should be in a separate fiel and include()d when needed define('DB_NAME', 'example'); define('DB_USER', 'heman'); define('DB_PASSWORD', 'password1'); define('DB_HOST', 'demodatabase'); $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if (!$link) { die('Could not connect: ' . mysql_error()); } $db_selected = mysql_select_db(DB_NAME, $link); if (!$db_selected) { die('Can\'t use ' . DB_NAME . ': ' . mysql_error()); } //Create query, making input safe for query $query = srpintf("INSERT INTO demo (name, phone, account, meter) VALUES ('%s', '%s', '%d', '%s')", mysql_real_escape_string($name), mysql_real_escape_string($phone), intval($account), intval($meter)); $result = mysql_query($query); if(!$result) { echo "There was a problem inserting the record.<br>" . mysql_error(); exit(); } else { //Redirect to confirmation page //This will clear the post data so a page refresh doesn't insert another record header("Location: http://www.example.com/confirm.php"); exit(); } } } ?> <html> <body> <div style="color:red;"><?php echo $errorMsg; ?></div> <form action="demo.php" method="post" /> <p>Name: <br /> <input type="text" name="name" value="<?php echo htmlentities($name); ?>" /></p> <p>Phone Number: <br /> <input type="text" name="phone" value="<?php echo htmlentities($phone); ?>" /></p> <p>Account Number: <br /> <input type="text" name="account" value="<?php echo htmlentities($account); ?>" /></p> <p>Reading: <br /> <input type="text" name="meter value="<?php echo htmlentities($meter); ?>"" /></p> <input type="submit" value="submit" /> </form> </body> </html>
-
select multiple values from mysql between intervals
Psycho replied to charles07's topic in PHP Coding Help
I'm sure there is a MySQL method to get that, but it might be easier to just do that calculation in the PHP code that processes the records $query = "SELECT MIN(`Time`) AS start_time, MAX(`Time`) AS end_time FROM table_name WHERE `Device one` = 'OFF' AND `Device two` = 'OFF' GROUP BY `Remarks` ORDER BY `Remarks`"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { $time_diff_in_sec = strtotime($row['end_time']) - strtotime(['start_time']); //Do something with the results } -
One thing I didn't put in the code was a process to "remove" the image when the user moves the mouse cursor off the image. If you want that, then just set an initial image such as a blank image or a default image. Then add an onmouseout event to call a function that will replace the default image //Function to replace image with default //Call upon mouse out of the images function clearFull() { showFull('default.gif'); } <image src="http://school.discoveryeducation.com/clipart/small/funny4.gif" onmouseover="showFull('funny4.gif');" onmouseout="cearFull();"> </tr>
-
It has to be done with JavaScript. PHP only runs server-side. This is a really easy problem. You simply need to create an onmouseover event for the images in the left column that will change the image in the right column. Here is a rough working example. The URLs for the images are to actual images. <!DOCTYPE html> <html> <head> <script type="text/javascript"> function showFull(imageName) { var imageURL = 'http://school.discoveryeducation.com/clipart/images/' + imageName; document.getElementById('full').src = imageURL; } </script> </head> <body> <table border="1" width="600"> <tr> <td width="100"><image src="http://school.discoveryeducation.com/clipart/small/funny1.gif" onmouseover="showFull('funny1.gif');"></td> <td rowspan="6"><img src="" id="full"></td> </tr> <tr> <td><image src="http://school.discoveryeducation.com/clipart/small/funny2.gif" onmouseover="showFull('funny2.gif');"></td> </tr> <tr> <td><image src="http://school.discoveryeducation.com/clipart/small/funny3.gif" onmouseover="showFull('funny3.gif');"></td> </tr> <tr> <td><image src="http://school.discoveryeducation.com/clipart/small/funny4.gif" onmouseover="showFull('funny4.gif');"></td> </tr> <tr> <td><image src="http://school.discoveryeducation.com/clipart/small/funny5.gif" onmouseover="showFull('funny5.gif');"></td> </tr> <tr> <td><image src="http://school.discoveryeducation.com/clipart/small/funny6.gif" onmouseover="showFull('funny6.gif');"></td> </tr> </html>
-
select multiple values from mysql between intervals
Psycho replied to charles07's topic in PHP Coding Help
I don't think that is exactly what the OP needed. The explanation is pretty poor. What I *think* you need is the MIN() and MAX() of the "Time" for each "remark" value that has one or more records where "Device one" and "Device two" are both off. By the way I would suggest renaming your fields to not have spaces in them. Either remove the space entirely or use an underscore. It will save you problems later. Also, don't use the strings "ON" and "OFF" as you would have to always do string comparisons on them. Instead use 0/1 which can be evaluated as the logical True/False Anyway, I think this will give you what you need: SELECT MIN(`Time`) AS start_time, MAX(`Time`) AS end_time FROM table_name WHERE `Device one` = 'OFF' AND `Device two` = 'OFF' GROUP BY `Remarks` ORDER BY `Remarks` -
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=351491.0
-
No it would go into PHP code that would come before the output for the default page. I'm not going to try and teach you the basics of PHP in a forum post. If you don't even know how to implement PHP code into a page then you might want to ask someone who does to do this for you.
-
The trick is that you do not need to change the link. Instead have the link point to a page that makes the determination as to what page to load. I would probably have the link ALWAYS point to the default page and implement logic at the top of that page to redirect to the radio site at the predetermined hours. $day = date('N'); //1 (for Monday) through 7 (for Sunday) $time = date('Gi'); //HoursMinutes (Hours in 24hour format) //Create condition to check if during radio broadcase //This example looks for Sat or Sun from 2pm - 4pm if( ($day==6 || $day==7) && $time>1400 && $time<1600) { //Redirect to radio broadcase header("Location: http://domain.com/broadcast/radio.php"); exit(); } //Default page content for when broadcast is not avail goes here
-
Not really understanding what you are doing here. If your database is using an MD5() hash why are you wanting to try and authenticate via a different hash? You can only use ONE hashing method for the same value. Since the password is already hashed using MD5 you cannot unhash it and then determine what it's value is using a different hashing method. You would either need to use a consistent hashing method for all authentication processes (correct methodology) or implement multiple hashes for each user record (i.e. and MD5 hash and the SHA1 hash) and use the correct value based upon the authentication method you want to use.
-
If you own/control the site then get the images via the FTP functions built into PHP.
-
Need help getting computer name or machine name.
Psycho replied to monkeybidz's topic in PHP Coding Help
In a word No. There are ways using client-side scripting but it would only work in some browsers and would require the users to lower their security settings to unsafe levels in order for it to work. -
Really? You might want to take a look at an ASCII table (http://www.asciitable.com/). FILTER_FLAG_STRIP_HIGH is supposed to strip everything out with a character code > 127. Those characters are not ones you find on your keyboard. They include characters such as Ç, â, ▓, ¥, ½. The ampersand is character code 38 and would not be covered be either FILTER_FLAG_STRIP_LOW or FILTER_FLAG_STRIP_HIGH. I did some tests and both flags are working as I would expect.
-
Wait, why would you think FILTER_FLAG_STRIP_LOW would strip out the ampersand? Per the manual FILTER_FLAG_STRIP_LOW will strip out characters less than 32. The ampersand is character 38 in the ASCII table. After a quick look at the ASCII table it look slike there are no "printable" characters that are less than 32 only white-space and control characters (line breaks, tabs, etc.). If it worked as you stated it should it would be stripping all the "printable" characters: letters, numbers, etc. http://www.php.net/manual/en/filter.filters.flags.php
-
Well, what are you trying to achieve?
-
Well, if you look at the user notes on the Sanitize filters manual page it looks like there is no consensus over what FILTER_FLAG_STRIP_LOW really does. http://www.php.net/manual/en/filter.filters.sanitize.php You might need to find an alternative function or build your own to do what you need.
-
Why the {1}? You already have the dollar sign to indicate only the end of the string so no repetition criteria is necessary. @John_A: It may not be clear, but with scootstah's replacement solution you do not need to test if there is a -[digit] before trying to remove it. Just run the preg_replace() to remove the -[digit]. If it does not exist it won't be replaced. So, testing for it is a waste of code. For what it's worth, I would use this expression: $str = preg_replace('/-\d$/', '', $str); The \d is a character class for digit.
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=351556.0
-
If there will only be one character that precedes the > characters then this will work: $output = preg_replace("#^[^>]>+#", "", $input); If there can be more than one character, than this should work $output = preg_replace("#^[^>]+>+#", "", $input);
-
When you need to sanitize a value to be entered into the database, you want to use the appropriate method/function based upon the data type and the database you are using. Each database has different functions. Most PHP applications use MySQL, so I'll cover that here: For string/text data you would want to use mysql_real_escape_string(). However that is, as its name implies, for string data. If you have a field that should be an integer or float you could use the functions intval() and floatval(), respectively. For date you want to ensure they are in the right format as well. Basically you need to use the right process for each specific situation. There is no one size fits all. When displaying user content to the HTML page, there are the functions htmlentities() and htmlspecialchars(). Those will transform content that would otherwise be interpreted as HTML code into the character codes/entities that will be displayed harmlessly. So, there is no reason you have to remove the tags from something like "<b>My Value</b>" if you don't want to. So, you don't need to use strip_tags() unless you have a need to actually remove those characters since the other two functions will allow you to display them without risk. Not sure what you are looking for regarding sessions. They are pretty safe since the data is stored on the server - only a session identifier is stored on the user's machine. Cookies are a much bigger risk. you should treat cookies like any other user submitted data (POST/GET) - don't trust them. Make sure you perform any necessary sanitizations, validations before using them. I wouldn't take anything I say as gospel, these are only my opinions. There are whole books written on these subjects. So, go pick one up if you really want to delve into this.
-
Pikachu2000 beat me to it, but I've provided some add'l info, so I'll post anyway. You have two problems in what you are doing. 1) Variables are not parsed inside single quoted strings and 2) You cannot concatenate strings inside the quotes like that. You would need to use double quotes (without the concatenate character - period) or append the string outside the quotes using either single or double quotes. echo $redak["novost_naslov_$home_jezik"]; Or echo $redak['novost_naslov_' . $home_jezik];
-
I don't see anything in that code that would display site titles and such. Not to be rude, but the description of this forum is If you are unable to at least identify the code in question where the problem exists, it is pretty difficult to help you. You might try the freelancing forum to see if someone wants to invest the time (for payment) to help you. However, I will offer another suggestion. Since you are wanting to change the titles and such for the site you probably want to be looking into the header.tpl and footer.tpl files. However, I've never used Smarty templates so I don't know that you'll even find anything useful in those.
-
How can I divide value by x and count how many times it is possible?
Psycho replied to Staggan's topic in PHP Coding Help
Please look at the solution I provided. You don't need a loop. The opposite of a "power" is the "log". The function I provided should get you exactly the result you want with a single line (and no loop). function dividedByCount($number, $base) { return floor(log($number, $base)); // <== SOLUTION } -
How can I divide value by x and count how many times it is possible?
Psycho replied to Staggan's topic in PHP Coding Help
The OP wanted to know how many times he can divide a number by another number - he only gave an example of 2. But, even if it was only 2 square root has no bearing on this. The square root of 36 is 6, but you cant divide that by two 6 times. (1) 36 / 2 = 18, (2) 18 / 2 = 9, (3) 9 / 2 = 4.5, (4) 4 / 2 = 2, (5) 2 / 2 = 1 I only did some rudimentary validation, but I believe my solution will give the exact results. EDIT: Just to be clear, if we are wanting to know how many times a number X can be divided by two we aren't looking for the square root of the number [ X ^ 2 <= Y ], we are solving for [ 2 ^ X <= Y ] -
How can I divide value by x and count how many times it is possible?
Psycho replied to Staggan's topic in PHP Coding Help
No need for loops. I believe you are wanting the log() using a base of 2 (and of course using floor). function dividedByCount($number, $base) { return floor(log($number, $base)); // <== SOLUTION } //Unit test for($i=1; $i<=36; $i++) { $count = dividedByCount($i, 2); echo "$i : $count <br>\n"; } Output of the unit test: 1 : 0 2 : 1 3 : 1 4 : 2 5 : 2 6 : 2 7 : 2 8 : 3 9 : 3 10 : 3 11 : 3 12 : 3 13 : 3 14 : 3 15 : 3 16 : 4 17 : 4 18 : 4 19 : 4 20 : 4 21 : 4 22 : 4 23 : 4 24 : 4 25 : 4 26 : 4 27 : 4 28 : 4 29 : 4 30 : 4 31 : 4 32 : 5 33 : 5 34 : 5 35 : 5 36 : 5