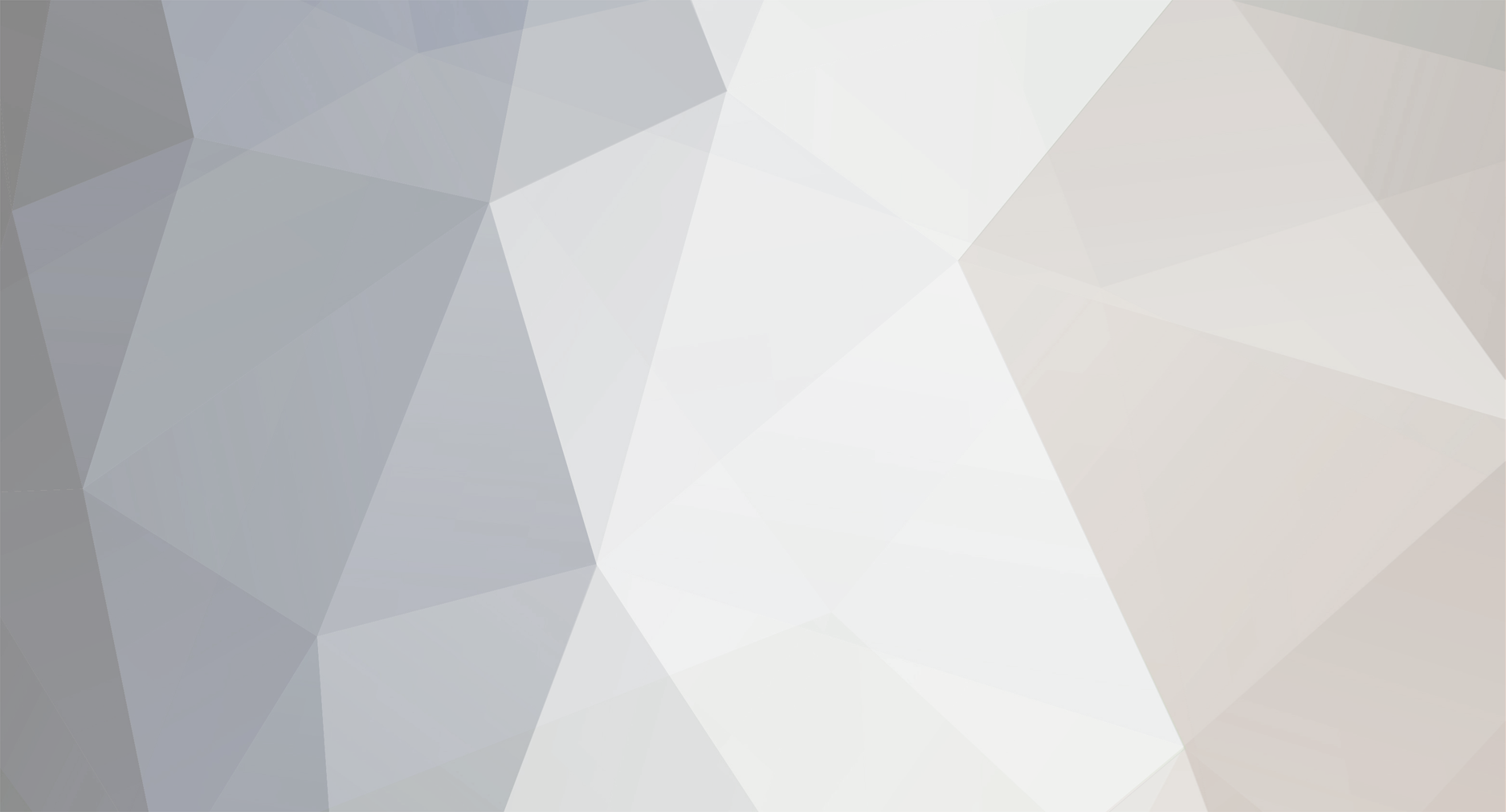
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Someone may have a better idea, but the only solution I can see is to run separate queries for the 5 above and the 5 below. But, you can do them both in a single query using the UNION clause. However, you would have to pre-process the results to get them in the proper order. EDIT: If records can have the same points this probably wont work (you could have the same records show above and below the target). So, can records have the same points? If so, I'll look into a different solution. Otherwise I can provide some sample code.
-
I don't think I could really do an adequate job of explaining how that works without going into detail regarding JOINs. But, suffice it to say that the query is joining the records from the "Shipments" table to the corresponding records in the other four tables. This works for your current need because there is a one-to-one relationship between the "Shipments" table and the other tables. Learning the different types of JOINs and how to use them really unlocks the power of a relational database. I suggest you go and find a tutorial on how to do JOINs. Here is one that seems to have some good examples: http://mysqljoin.com/
-
That is pretty much what I provided to you previously, except: 1. I did not list out the individual fields (since you did not provide that information) 2. Your query does not limit the results based upon the Shipments.Zending_ID as you originally showed. I really prefer to explicitly JOIN my tables (as I showed in the query I provided) rather than to do it in the WHERE caluse as you have much more flexibility on how the JOINs work. However, you should not be using $i in that code to process the DB results. Instead, just use while ($test2 = mysql_fetch_assoc($result)) Also, do you really want a new table for each record (with new column headers)? It seems that one set of column headers would be better. Here is a revise of what you had in a more logical format. Note: I guesses on which fields were coming from which tables. Make sure each field name is prefaced with the appropriate table alias $query = "SELECT z.Naam, b.Bestemming, k.Aantal_Duiven, m.Name FROM Shipments AS s JOIN Zendingen AS z ON s.Zending_ID = z.ID JOIN Klanten AS k ON s.Klant_ID = k.ID JOIN Manden AS m ON s.Mand_ID = m.ID JOIN Bestemmeling AS b ON s.Bestemmeling_ID = b.ID WHERE z.Actief = 1"; $result = mysql_query($query) or die(mysql_error()); echo "<table>\n"; echo "<tr>\n"; echo " <th>Bestemming</th>\n"; echo " <th>Naam</th>\n"; echo " <th>Aantal Duiven</th>\n"; echo " <th>Naam</th>\n"; echo "</tr>\n"; while ($row = mysql_fetch_assoc($result)) { echo "<tr>"; echo " <td>{$row['Bestemming']}</td>\n"; echo " <td>{$row['Naam']}</td>\n"; echo " <td>{$row['Aantal_Duiven']}</td>\n"; echo " <td>{$row['Name']}</td>\n"; echo "</tr>\n"; } echo "</table>\n";
-
I'd also add that trying to do too many things in one line of code can be confusing to the point of any possible efficiencies are outweighed by the inability to read/manage the code. I would probably do somethign such as: <?php $blogInfo = bloginfo('template_directory'); echo htmlentities('<img src="' . $blogInfo . '/images/my-image.png" / rel="nofollow">'); ?> Although, you do realize that the above will not be a hyperlink because of the htmlentities, right?
-
There is no way for us to answer this for you as we know nothing about your current application. Are you storing any information about the uploads now that is separate from the files themselves? If you are storing information in a database, then you would want to add an additional column in the table to store a foreign key reference back to the user who uploaded the file. If you have a user based system then you should have some means of knowing who the user is (SESSION, COOKIE< etc.) - so you should already have the users ID. So, when the user submits a file you would get that value and include it with the other data you are storing. Again, no idea how to tell you actually how to do this since I have no knowledge of your current code. Then when you display the file (or more likely a link to the file) you would query the database for the information you were previously displaying and you would use the foreign key reference for the user ID to join the users table to also get the username.
-
So, the 'Shipments' table is the combinations table? If I am understanding this correctly, each record in the 'shipments' table has a foreign key reference back to primary IDs in the other four tables. If that's correct, then you simply need to JOIN the tables. However, there would potentially be a problem. You say that the ID fields from each table is labeled ID. Because you have those IDs as foreign key fields int eh shipments table with distinctive names you won't have a problem referencing the IDs in the result set. But, if you have other fields with the same names in those tables it is going to be more difficult in referencing the values. I try to always use unique names in may tables. (E.g. If I have a users and a clients table, I would use user_name and client_name rather than use 'name' in both). Having said that, it it werdatabase, I would rename the ID fields in each of the tables to be Zending_ID, Klant_ID, etc. It makes it easier to work with the data and JOINing because more logical. Anyway, with what you have you should be able to get the data using: SELECT * FROM Shipments AS s JOIN Zendingen AS z ON z.id = s.Zending_ID JOIN Klanten AS k ON k.id = s.Klant_ID JOIN Manden AS m ON m.id = s.Mand_ID JOIN Bestemmeling AS b ON b.id = s.Bestemmeling_ID WHERE Zending_ID = 9 If you were to make the ID fields the same as the names in the shipment's table, it becomes even easier SELECT * FROM Shipments JOIN Zendingen USING(Zending_ID) JOIN Klanten USING(Klant_ID) JOIN Manden USING(Mand_ID) JOIN Bestemmeling USING(Bestemmeling_ID) WHERE Zending_ID = 9
-
function timeUntil($endTime, $granularity=3, $format='d:H:i:s') { $difference = $endTime - time(); if($difference < 0) return '0 seconds ago'; elseif($difference < 200630560) { $periods = array('year' => 31570560, 'month' => 2630880, 'week' => 604800,'day' => 86400,'hours' => 3600, 'min' => 60, 'seconds' => 1); $output = ''; foreach($periods as $key => $value) { if($difference >= $value) { $time = round($difference / $value); $difference %= $value; $output .= ($output ? ' ' : '').$time.' '; $output .= (($time > 1 && $key == 'day') ? $key.'s' : $key); $granularity--; } if($granularity == 0) break; } return ($output ? $output : '').''; } else return date($format, $timestamp); } echo timeUntil(time()+9); // 9 seconds echo timeUntil(time()+99); // 2 min 39 seconds echo timeUntil(time()+9999); // 3 hours 47 min 39 seconds echo timeUntil(time()+99999); // 1 day 4 hours 47 min echo timeUntil(time()+999999); // 2 week 5 days 14 hours echo timeUntil(time()+9999999); // 4 month 3 week 3 days echo timeUntil(time()+99999999); // 3 year 2 month 7 hours
-
OK, when a user gets a warning you should update the database to time() + 2 weeks. Then when you want to calculate the time left you should use the database value - time(). You could just update your function to do that calculation for you.
-
Well, you say that it is working corectly when you use $save['removal'] = time() + (300); But I find that hard to believe. because of this line elseif($difference < 31570560) The current timestamp (for me) is 1324312172 which is greater than that hard-coded value in that condition. So ALL results are defaulting the the else statement of else return date($format, $timestamp); What is the purpose of '31570560'? EDIT: Als,o you are passing a timestamp to the function. Then you are using that value as $difference. A difference requires two values to be calculated. It doesn't make sense why the timestamp would be a difference, unless you were calculating the difference from January 1 1970 (which is what the timestamp is)
-
If you could give us some examples and what you are trying to do and some sample data we may be able to help you better. For example, what are you using to consider two records as being the same. You can create uniqueness across rows! For example you can have a first_name column and a last_name column and then set the combination of those two naems as being unique. In that scenario you can have "Tom Smith", "Tom Williams" and "Dave Williams", but you can't ahve two records with the same first_name AND the same last_name. I suspect you probably want to create uniqueness across rows - then you would use ON DUPLICATE.
-
confused, Im gettinbg double entries in text file
Psycho replied to bakhtn's topic in PHP Coding Help
No you do not need to run the query within the loop. The whole point of a relational database is that you can 'relate' the data, for example, using a JOIN. Your initial code was terribly inefficient. 1. Again, no need to run those queries in a loop. Simply do ONE query with appropriate JOINs to get all the data you need. 2. Do not open/close the file within the while loop. Just open it once, write your data, then close it one. I really don't see why your original code would write duplicates, but suspect it might have something to do with opening/closing the file repeatedly. If it isn't hat then you just might have duplicate data. -
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=350117.0
-
<< Moving to MySQL forum >> SELECT SUM(kd.itd_quantity * ip.price) as total FROM item_price AS ip JOIN kill_dropped AS kd ON kd.itd_itm_id = ip.id WHERE kd.itd_kll_id = 7926
-
Display the result of large select_form with many conditions
Psycho replied to Adib's topic in PHP Coding Help
OK, I *think* I understand you. If so, then the current code for creating the options shouldn't change. You should just need to change the code that creates the conditions. But, there's something to consider. What if the user selects a FROM value but no TO value (or vice versa). I would handle that by creating a condition such as "WHERE value > FROM" or "WHERE value < TO". Anyway, I did put all of the POST data into an array to be processed in a loop. If ALL the fields were going to be processed the exact same way that makes sense. If not, then it doesn't. So . . . this is just a rough idea (not tested) <?php function createCondition(&$conditionsAry, $field, $value, $condition) { if(!isset($_POST[$field])) { return; } $value = trim($value); if(empty($value)) { return; } $value = mysql_real_escape_string($value); $conditionsAry[] = "`{$field}` {$condition} '{$value}'" return; } $eigenschappen = $_POST['eigenschappen']; //Process the conditions that were posted and populate temp array $conditionsAry = array(); createCondition($conditionsAry, 'locatie', '='); createCondition($conditionsAry, 'prijsVan', '>='); createCondition($conditionsAry, 'prijsTot', '<='); createCondition($conditionsAry, 'ligging', '='); createCondition($conditionsAry, 'oppervlakte', '='); createCondition($conditionsAry, 'kamers', '='); createCondition($conditionsAry, 'woning', '='); // Error: indien geen keuze gemaakt show deze error if(count($conditionsArray)==0) { echo "<center><font color='#666'> " . postError() . "</font></center>\n"; } else { //Create / Execute query $query .= " AND " . implode(' AND ', processConditions($_POST['conditions'])) $query .= " ORDER BY prijs.ID"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)){ echo $row['image_id'] ; echo $row['locatie'] ; echo $row['prijs']; echo $row['type']; echo $row['kamers']; echo $row['oppervlakte'] . ' m²'; echo $row['ligging']; echo $row['eigenschappen']; } } mysql_close($con); ?> -
confused, Im gettinbg double entries in text file
Psycho replied to bakhtn's topic in PHP Coding Help
//if any items in order table then display all items in order table $order_sql = "SELECT o.size, o.type, o.amount, c.category, m.item_name FROM orders AS o JOIN category AS c USING(category_id) JOIN menu AS m USING(item_id)"; $order_result = $handle->query($order_sql); if($order_result->num_rows) { echo "<table bgcolor='#FFFF66'>\n"; echo "<tr>\n"; echo "<th></th>\n"; echo "<th></th>\n"; echo "<th></th>\n"; echo "<th></th>\n"; echo "<th></th>\n"; echo "<\tr>\n"; while ($order = $order_result -> fetch_assoc()) { echo "<tr>\n"; echo "<td>$order['category']}</td>\n"; echo "<td>{$order['item_name']}</td>\n"; echo "<td>{$order['size']}</td>\n"; echo "<td>{$order['type']}</td>\n"; echo "<td>{$order['amount']}</td>\n"; echo "</tr>\n"; //Append insert data to write into order.txt file $file_insert .="{$order['category']}: {$order['item_name']} {$order['size']} {$order['type']} {$order['amount']}\r\n"; } echo "</table>\n"; @$fp = fopen( 'order.txt', 'ab' ); if($fp) { fwrite($fp, $file_insert, strlen($file_insert) ); fclose($fp); } else { echo 'could not insert data into order.txt file for print, CLICK ON NEW ORDER'; } } -
confused, Im gettinbg double entries in text file
Psycho replied to bakhtn's topic in PHP Coding Help
Well, you should also move the fopen() outside the while() loop as well. Also, you should not run a query inside the while loop. Instead run ONE query to get all the needed info. -
How to format text written to a .txt file ?
Psycho replied to thaidomizil's topic in PHP Coding Help
You should never run queries in loops. How are you defining the $checkbox array? Is it from $_POST['checkbox'] or some other POST data? You should be using the IN condition within your MySQL query with ALL the ids. I don't think chunk_split() is really what you want to use. You would probably want each record to be on a line delimited using commas, tabs or spaces. Anyway, here is an example (not tested). if ($_POST['exporttxt']) { $export_ids = implode(',', $_POST['export_ids']); $query = "SELECT code FROM tickets WHERE id IN ({$export_ids})"; $result = mysql_query($query); $output = ''; while($row = mysql_fetch_assoc($result)) { $output .= implode(' ', str_split($row['code'], 4)) . "\n"; } $filename = "export" . date("Y-m-d") . ".txt"; ob_end_clean(); header("Content-Type: application/octet-stream"); header("Content-disposition: attachment;filename=\"$filename\""); header("Content-Type: application/force-download"); header("Content-Type: application/download"); header("Content-Description: File Transfer"); header("Content-Length: ".strlen($output).";\n"); echo($output); } exit(); -
Display the result of large select_form with many conditions
Psycho replied to Adib's topic in PHP Coding Help
Well, there are several ways: 1. You could change the function to take an array. Then for all the select lists you would run your query and preprocess the data as needed before calling the function. This probably makes the msot sense from the standpoint of keeping the code functionally separated. 2. You could change the function to take an additional parameter (e.g. the euro sign). Then change the logic in the function to use that parameter when generating the output. 3. Simply change the query to put the euro sign into the results. This would only require one simple change $prijsVanOptions = querySelectOptions("SELECT ID, CONCAT('€', prijs) FROM prijs"); This will return the same results but the euro sign will be concatenated to the front of the prijs values -
I think you both missed what I was talking about. I see a lot of people that continually enter/exit the quoted string to concatenate variables where the string they are wanting to create needs to end with a variable. But, I routinely see them concatenating a null string in quotes at the end. Whenever I ask someone why they do that they never have a good answer. Usually something like "I thought it had to be that way". Example: $query = "SELECT * from tbl ORDER BY " . $sort_field . ""; lame
-
The problem is with your condition statement if( ($filetypeCheck != "image/gif") || ($filetypeCheck != "image/jpeg") || ($filetypeCheck != "image/png") ) NOTHING can pass that validation. If the image type was 'image/gif' then is does not equal 'image/jpeg' or 'image/png'. The problem is the OR statements and the negative checks. An image type will always not equal at least two of those consitions and validation fails. There are different solutions, such as if( !($filetypeCheck == "image/gif" || $filetypeCheck == "image/jpeg" || $filetypeCheck == "image/png") ) { //Invalid image type } else { //Valid image type } if( $filetypeCheck == "image/gif" || $filetypeCheck == "image/jpeg" || $filetypeCheck == "image/png") { //Valid image type } else { //Invalid image type } Or my favorite $validImageTypes = array('image/gif', 'image/jpeg', 'image/png'); if( !in_array($filetypeCheck, $validImageTypes)) { //Valid image type } else { //Invalid image type }
-
Well, I find breaking in and out of quotes to concatenate things ugly. So, there. Thankfully, PHP supports both ways of working with strings/variables, your way and the right way Plus, I find it hilarious when I see people do this (note the end of the string) which I see all the time. $query = 'SELECT * FROM table_name WHERE field_id = ' . $fieldID . ''; But, to each his own. EDIT: I suppose that breaking out of quotes to concatenate a variable would make sense if you cannot easily differentiate your variables inside the strings. But, that is an editor dependent issue. The editor I use makes it easy for me to 'see' the variables in the quoted strings. Something to think about if your editor does not do that for you.
-
I think part of his question is also why he couldn't use the POST value directly inside the parens with the forwardslashes such as "/$_POST['withthis']/" As I am sure you know, you can use variables directly inside a double quoted string. But, when you have an array value with quotes around the key, PHP gets confused. The simple solution is to enclose the variables inside curly braces. I try to do this for ALL my variables within double quoted strings out of habit so I don't have to worry about the scenarios where they would fail. This should work for you. $new_text = preg_replace("/{$_POST['replacethis']}/" ,$_POST['withthis'],$_POST['text']);
-
There is only one line in that code that produces the actual output $genTableArray[$rowIndex][] = "<td rowspan='{$rowspan}'>$personIndex - {$familyTreeArray[$personIndex]}</td>\n" Put whatever other content you want in that. In this case, either put an onclick inside the TD tag or change teh content in the TD tags to a hyperlink. At the very least though, you would need to pass a value so the next page knows what dog to create the results on next. I guess you could use the name, but you should be passing an id value. But, your array doesn't contain an id, so . . .
-
OK, here is the code with more comments $generations = 5; //Create temp array to store results $genTableArray = array(); //Run through each column based upon the number of generations. //The column count is generation + 1 for($genCol = 0; $genCol <= $generations; $genCol++) { //Detemine the number of rows/records in the colum (1, 2, 4, 8, 16, ...) $genRowCount = pow(2, $genCol); //Detemine the rowspan for each record/row in this column //This will be the (total # rows / record in this row) //The total # of rows is based upon the number of generations to the power of 2 $rowspan = pow(2, $generations) / $genRowCount; //Run a loop for the number of records in this column for($familyGenCount=0; $familyGenCount<$genRowCount; $familyGenCount++) { //Calculate the person index to use based upon the column / row //First column has index 0, second column has 1/2, third column has 3/4/5/6, etc. //The formula is needed due to the size being dynamic $personIndex = pow(2, $genCol) + $familyGenCount - 1; //Determine the row index for this record. This is dynamic based upon the //total number of columns and the column/record beign displayed. For example, //if there are 3 generation there will be a total of 8 rows. Column 2 will have two records //So record 1 will go in the first row and record 2 will go into the 4th row $rowIndex = $rowspan * $familyGenCount; //Add the recod to the appropriate (row) in the array using the rowspan. The key of the array is the row index $genTableArray[$rowIndex][] = "<td rowspan='{$rowspan}'>$personIndex - {$familyTreeArray[$personIndex]}</td>\n"; } } //Output the array into a table //Since records weren't added to rows in order (0, 1, 2, ...) need to sort //the array by keys to get int he correct order ksort($genTableArray); $familyTreeHTML = ''; //Loop through each row in order and implode the values into an output variable foreach($genTableArray as $rowData) { $familyTreeHTML .= "<tr>\n" . implode("\n", $rowData) . "</tr>\n"; } Also, so you can understand the final process, here is what the temp array looks like before the final processing Array ( [0] => Array ( [0] => <td rowspan='8'>0 - Kegluneq Aaliyah</td> [1] => <td rowspan='4'>1 - Arctictreks Dark As Night</td> [2] => <td rowspan='2'>3 - KEBUCK SCHRIMSHANDER</td> [3] => <td rowspan='1'>7 - Pat Gagnon</td> ) [1] => Array ( [0] => <td rowspan='1'>8 - Coquette La Rouge D'Ericlam</td> ) [2] => Array ( [0] => <td rowspan='2'>4 - ARCTICTREKS CHERISH FOREVER</td> [1] => <td rowspan='1'>9 - Storm Kloud's Chosen to Win</td> ) [3] => Array ( [0] => <td rowspan='1'>10 - Arctictrek's Indiana</td> ) [4] => Array ( [0] => <td rowspan='4'>2 - Arctictrek Silver Spirit</td> [1] => <td rowspan='2'>5 - Shepherdsway Touch of Gold</td> [2] => <td rowspan='1'>11 - Outriggers Red Wolf</td> ) [5] => Array ( [0] => <td rowspan='1'>12 - Eastern Hill Coldfoot Coco</td> ) [6] => Array ( [0] => <td rowspan='2'>6 - Arctictreks Legend of a Lady</td> [1] => <td rowspan='1'>13 - Shepherdsway Phantom Scout</td> ) [7] => Array ( [0] => <td rowspan='1'>14 - Arctictrek's Indiana</td> ) )
-
Good luck. I barely understand it myself (LOL). Actually, I understood each part as I did it, but didn't comment as I went. Some of it is kind of abstract.