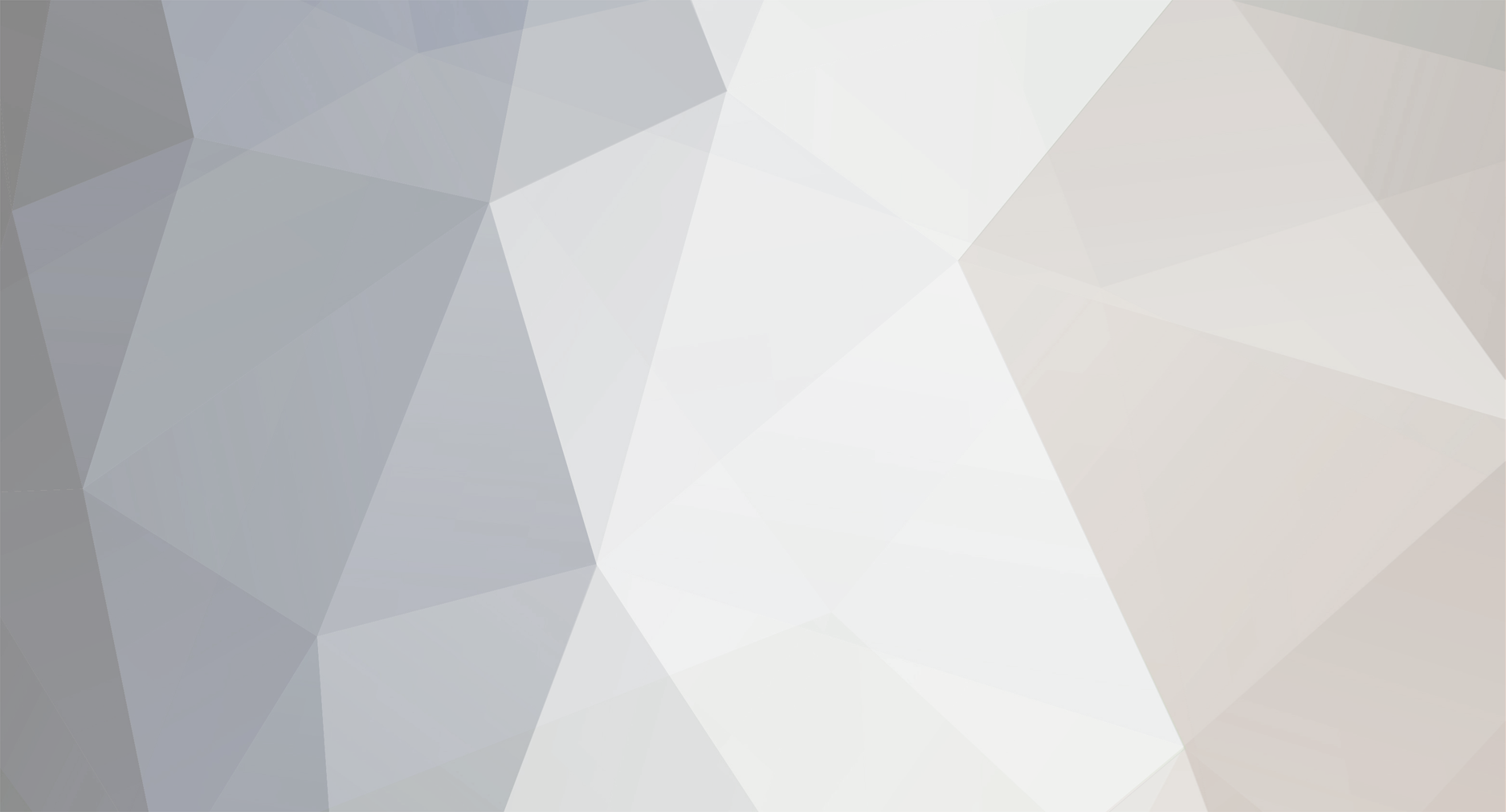
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
What if someone's name is something like... a';DROP TABLE users; SELECT * FROM userinfo WHERE 't' = 't That is why I chose to originally use Regex. Do you understand what it means to escape the values for a query [whether it is with mysql_real_escape_string() or with prepared statements]?. If the input is properly escaped, a name such as that won't do anything - that literal string would simply be inserted into the table. Granted, it's a stupid name, but it won't cause any harm as long as you are handling it correctly. So, your regex is not providing any security it is only creating the possibility that you might reject a valid name.
-
My guess would be that the condition is never true. Without examining the data there's no way to be sure. But, you can add some debugging code to check for yourself foreach ($data[0] as $k => $r) { //Debugging code: echo ($r['feedid'] !== $user_info['uid']) ? "'{$r['feedid']}' !== '{$user_info['uid']}'" : "'{$r['feedid']}' === '{$user_info['uid']}'"; echo " : "; echo ($r['action_id'] == 'newuser') ? "'{$r['action_id']}' == 'newuser'" : "'{$r['action_id']}' != 'newuser'"; echo (($r['feedid'] !== $user_info['uid']) && ($r['action_id'] == 'newuser')) ? "TRUE" : "FALSE"; echo "<br>\n"; if (($r['feedid'] !== $user_info['uid']) && ($r['action_id'] == 'newuser')) { unset($data[0][$k]); } } If the results still don't look right try using var_dump() on the first two values since they must be the same value AND the same type.
-
please help with the logic for this. I cant figure it out :(
Psycho replied to kevinkhan's topic in PHP Coding Help
Yes, you can go with that approach, but as I was trying to find out there is probably a more efficient way to do this with the initial creation of the arrays rather than after the fact. -
Change the function getPictures() to take a parameter for the folder to search. Then change the opendir() call to use that parameter function getPictures($source_folder) { global $max_width, $max_height; if ( $handle = opendir($source_folder) ) { Now just call the function with the path of the imaged in the function call. <?php getPictures('path/to/images/'); ?> However, I think glob() would be a much better choice instead of opendir() but I'm not going to rewrite that script.
-
please help with the logic for this. I cant figure it out :(
Psycho replied to kevinkhan's topic in PHP Coding Help
So, the image names will ALWAYS be a name followed by an underscore and a number? Where are you getting these arrays? Are they generated from a database or a file processing routine? I think there is probably a simpler solution to handle this at the time the arrays are created instead of after the fact. I could provide a solution, but I want to see where the data is coming from first so I'm not providing the wrong solution. -
You will want separate tables for each record "type". For example, you would have a listings table that has one record for each listing. But, each property may have no images or many. So, you would have a separate images table with one record for each image with a field to identify which listing each image belongs to. There are a LOT of different considerations on designing a database - way more to even start going over here. Go google for some beginner articles on database normalization.
-
The code I provided [with the one fix for mysql_num_rows()] would do one of three things: 1) If the query is failing, then you will see an output of the query and the error 2) If the query succeeded, but with no results, there would be output to that effect 3) If the query executed successfully and there was a record returned you would see the output from the two fields in the SELECT query. So, if you get no output, the only thing I can assume is that the matching record has empty values (or only white-space characters) for those two fields or that code is not getting executed. I.e. you have some sort of control structure that is preventing those lines from running such as an if() condition.
-
Well, you aren't outputtting anything in that code. But, assuming you are echoing $name somewhere else, either your query is failing or there were no results. You need to add code to handle those situations. Also, if you are using a LIMIT 1 on your query, there is no reason to use a while() loop. $query = "SELECT firstname, lastname FROM myMembers WHERE id='$logOptions_id' LIMIT 1"; $result = mysql_query($query); if(!$result) { echo "Query: {$query}<br>Error: " . mysql_error(); } elseif(!mysql_num_results($results)) { echo "There were no results."; } else { $row = mysql_fetch_assoc($result); $name = "{$row['firstname']} {$row['lastname']}"; echo $name; }
-
Printing average-value from two tables with PHP/SQL
Psycho replied to ijjed's topic in PHP Coding Help
Then you need to do a LEFT JOIN so you will get ALL records from the first (i.e. Left) table. SELECT class_name, AVG(course_number) as average FROM class LEFT JOIN courses USING (class_id) GROUP BY class_id But, the average results for those without any corresponding records in the "courses" will be an empty value. You can use PHP to convert that to a 0. Or you can have the query do that for you. Not sure on the exact syntax and I don't have time to test SELECT class_name, AVG(IF(course_number<>NULL, course_number, 0)) as average FROM class LEFT JOIN courses USING (class_id) GROUP BY class_id -
Printing average-value from two tables with PHP/SQL
Psycho replied to ijjed's topic in PHP Coding Help
SELECT class_name, AVG(course_number) as average FROM class JOIN courses USING (class_id) GROUP BY class_id -
This should allow you to dynamically determine the odds based upon a max number. $max_num = 4; $card1 = rand(1, $max_num); $card2 = rand(1, $max_num); $odds = pow((1/$max_num), 2); //2 is the number of 'cards'
-
Remove Subarray from Multidimensional Array Based On Value
Psycho replied to jmwhitaker's topic in PHP Coding Help
I think array_filter() would be a better option for this: function removeInactiveSlides($sub) { return (!(isset($sub['slide_active']) && $sub['slide_active']===0)); } $arrSlidesFiltered = array_filter($arrSlides, 'removeInactiveSlides'); -
Not tested, so there may be some typos <?php $monthNames = Array('Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'); $table_head = "<th>Year</th>"; foreach ($monthNames as $month) { $table_head .= "<th>{$month}</th>\n"; } $query = "SELECT count(cus_id) as total_customers, DATE_FORMAT(sign_date, '%b') as month, YEAR(sign_date) as year FROM customers GROUP BY YEAR(sign_date), MONTH(sign_date) ORDER BY sign_date ASC"; $result = @mysqli_query ($dbc, $q); $output = ''; $current_year = false; if (mysqli_affected_rows($dbc) >= 1) { while($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { if($current_year != $row['year']) { $current_year = $row['year']; } $output .= "<tr>\n"; $output .= "<th>{$current_year}</th>\n"; foreach($monthNames as $current_month) { if($current_month == $row['month'] && $current_year == $row['year']) { $count = $row['total_customers']; $row = mysqli_fetch_array($r, MYSQLI_ASSOC); } else { $count = '0'; } $output .= "<td>{$count}</td>\n"; } $output .= "</tr>\n"; } } else { $output = "<tr><td colspan='13'>There were no results.</td></tr>\n" } ?> <div id="dash_chart" class="portlet x9"> <div class="portlet-header"> <h4>Customer Growth</h4> <ul class="portlet-tab-nav"> <li class="portlet-tab-nav-active"><a href="#tab1" rel="tooltip" title="Customers">Customers </a></li> <li class=""><a href="#tab2" rel="tooltip" title="Sales over last 48 hours.">Sales </a></li> </ul> </div> <!-- .portlet-header --> <div class="portlet-content"> <div id="tab1" class="portlet-tab-content portlet-tab-content-active"> <table class="stats" title="area" width="100%" cellpadding="0" cellspacing="0"> <caption>Customer Base Growth</caption> <thead> <tr><?php echo $table_head; ?></tr> <tbody> <?php echo $output; ?> </tbody> </table>
-
There are other validations you are not doing, such as are the entries numbers, are they within the allowed range, etc. This should contain all the validations that you need. you will be left with a final array that contains ALL the picks of the user that are valid. For the error conditions you can completely reject all the picks or just provide an error message that some of the picks were rejected. $numbers = "19|20|19,1|2|3,3|2|1,4|5|6"; $picksAry = array(); foreach(explode(',', $numbers) as $pick) { //Remove duplicate values in pick $pick = array_unique(explode("|", $pick)); //Remove non-numeric values $pick = array_filter($pick, 'ctype_digit'); //Sort the array sort($pick); //Verify if this pick has correct count of unique values if(count($pick) != 3) { //Pick does not contain 3 unique numbers } //Verify if values in pick are within acceptable range else if($pick[0] < 1 || $pick[2] > 56) { //Pick contain values outside acceptable range } else { //Pick is valid add it to result array $picksAry[] = $pick; } } //Check if there are any duplicates between picks if(count($picksAry) != count(array_unique($picksAry))) { //There are duplicate picks. Either reject all or continue with array_unique() values } $validPicks = array_unique($picksAry);
-
Well, first of all that logic doesn't check if item #1 matches item #2. Second of all, that's more complicated than it needs to be. Just use array_unique() on the array to remove duplicate values and then check if the result still has three elements. $numArray = array_unique(explode("|", $numbers)); if(count($numArray)<3) { $std->Error2("You cannot pick 2 numbers with the same ticket"); } I still don't know why you are passing the values in the format "#|#|#" instead of using arrays to being with. You are making your "project" 10x more complicated than it needs to be based on the posts of yours I have seen. As for checking if there are two sets of values with the same numbers that will not be easy with what you have now. From what I have seen you don't have any logic to put the numbers in numerical order. So, a user could have "3|5|8" and "8|3|5". Personally, I would convert ALL the user picks into a mulch-dimensional array. And, when doing so, sort each pick so they are in numerical order. Then in addition to using array_unique() on each pick to ensure there are no duplicate numbers in the pick, you could use array_unique() on the parent array to see if there are any picks with the same numbers.
-
Just add it to where the values are defined $name = mysql_real_escape_string($record[0]); $age = mysql_real_escape_string($record[1]); $addr = mysql_real_escape_string($record[2]); $weight = mysql_real_escape_string($record[3]); $height = mysql_real_escape_string($record[4]);
-
NEVER run queries in loops! Anyway, you state you are trying to populate (i.e. INSERT) records into the second table. BUt, you are using an UPDATE query. An UPDATE query will 'update' existing records. Since, you didn't provide a WHERE clause on the update query it is attempting to update ALL the records in the table. And, apparently, the value you are using is an empty string. So, all the records are being updated with an empty string. Based upon what you are trying to do, however, this should be a ONE-TIME operation. Once you have a table with the unique towns you should use the primary key in that table as a foreign key in the hotels table. Then you would never store the town names in the hotels table. To accomplish the import of the unique town names into the new table you should first set the 'town_name' to be a unique field. Then you need just one query to import the all the new values INSERT INTO town_coords (town_name) SELECT DISTINCT est_town FROM hotels
-
You can't have two records in the same table with the same value in a field that is the primary ID or set as a unique value. Your database structure is flawed. I would suggest the following. 1. Add a new field called something such as parent_topic_id. 2. Let the topicID be auto-assigned for ALL posts - even ones that are in response to another post 3. For new, original, posts set the value of parent_topic_id to 0 4. For posts that are in response to another post set the parent_topic_id to the id of the parent post Then if you want to query a specific parent post and all the responses you could use a query such as SELECT * FROM topics WHERE topicsid = '$tpid' OR parent_topic_id = '$tpid' ORDER BY topic_date
-
Does blank space really matter in performance?
Psycho replied to Ivan Ivković's topic in PHP Coding Help
Such as? If there's smthn wrong in what you see, please gimme a tip. That wasn't an accusation just a statement that anyone will likely gain much more in performance by looking for existing inefficiencies in the logic of their code. Any good developer is constantly learning and implementing new/better processes as they move forward. So, if you were to look at code you wrote 6 months ago you should find things that you would do differently today. The real question is "whether" you should go back and review the code. If it is doing what it is supposed to do and there are no performance problems then the answer is usually no. Plus, if you really need to squeeze out every last bit of efficiency in the code there are always things that we may prefer to write one way but would be more efficient in another. In my case I like using the ternary operator for succinct manner in which I can assign a value to a variable. But, it is less efficient than a longhand if/else statement. But, I will take that infinitesimal hit in performance in order to have code that I feel is easier to analyze/digest. @cyberRobot, doing that to your HTML will them make it extremely difficult to debug any errors produced in your output. Besides just one moderately sized image will normally be larger than all of the HTML code for your HTML file. -
This should already being taken care of in the code I provided. The DB results are sorted such that the correct answer for each question will be listed first. Then in the code that shuffles the questions it FIRST removes one of the incorrect answers using unset($question['answers'][rand(1, 4)]) Since the correct answer will have an index of 0 it would never be removed Good question. You can't simply LIMIT your results since you are getting more results than you have questions. You could always multiply the number of questions you want by 5 since all the questions have 5 answers and use that in your LIMIT. But, that's a poor solution because you may want o add more answers in the future. I would probably implement a subquery. But, then the question is do you want them in order or randomized. As I stated before MySQL does a poor job of randomizing. There are solutions but I don't have them memorized and am not going to take the time to look them up right now. But, if your database of questions would be relatively small you can use ORDER BY RAND() SELECT q.id, q.question, a.answer FROM {$question_table} q JOIN {$answer_table} a ON q.ID = a.QUESTION_ID WHERE q.id IN (SELCT id FROM {$question_table} WHERE quiz_id = 1 ORDER BY RAND() LIMIT $question_count) ORDER BY question_id ASC, correct DESC Huh? Not sure what you are wanting. I believe the shuffle() command resets all the keys in the array. So, question #1 (as given to the user) will have an index of 1, question #2 will be 1 and so forth. So, it already tells you the order that they were given to the user.
-
It looks like the problem is that the 'key' for the question record in the array is not getting set correctly. Looks like it is an empty string. So, each question is determined to be the same based upon that value. The code is supposed to use the question ID as the key. I do see that the question ID is correctly set as one of the sub values in the array, but it is not set as the key for the array element. I thought there might be a typo in the variable/key name in the logic to create the key, but I've reviewed it several times and don't see a problem. Try this revision of the while loop: while($row = mysql_fetch_assoc($result)) { ## DEBUGGING LING ## echo implode(" : ", $row) . "<br>\n"; $qID = $row['question_id']; if(!isset($questions[$qID])) { $questions[$qID] = array( 'question' => $row['question'], 'question_id' => $qID, 'answers' => array() ); } $questions[$qID]['answers'][] = $row['answer']; }
-
Please be specific by what is meant by "Not working". Are you getting ANY output. If so, how does that output differ from your expectations? Did you look at the HTML source code in your browser to see what is in there that isn't directly displayed in the page? If you are not getting any errors it could be: 1. The server does not have PHP installed so the file is sent as a plain HTML file and the PHP code will not be displayed in the browser because the PHP tags are treated like HTML tags. 2. The query is not returning any results
-
adding a add and remove function for a product category.
Psycho replied to omegaultmo2's topic in PHP Coding Help
Yeah, if you are storing the products in an array, presumably in the PHP file, then the ability to add/edit/delete products is kinda weird. Of course you could always store the array in a separate file and create the processes to modify that file. But, that's really a waste of time since you are going to move into a database in the next step. Personally, I would ask the instructor for clarification on what the expectations are. I assume you are storing the cart information in a session variable. You could do the same thing for the product data. So, when a page is loaded you check to see if the complete product list has been saved to a session variable. If not, do that. Then when an "admin" makes changes to the products, those changes are made to the session data. Of course, none of these changes would be seen by "other" users accessing the pages on a different machine/browser and the changes would be wiped out when you closed the browser. But, again, this is just an exercise to get you to the next step using a database. -
OK, then you simply need to check each field before appending the relevant text to the message. You would still want to trim() the values first though. You could create a bunch of inline statements such as $message = ''; if(!empty($name)) { $message .= "Name: {$name}\n"; } if(!empty($company)) { $message .= "Company or Organization:{$company}\n"; } Or you could create a function that you pass the variable and the full line to: function addLine($var, $line) { return (!empty($var)) ? "{$line}\n" : ''; } $message = ''; $message .= addLine($name, "Name: {$name}"); $message .= addLine($company, "Company or Organization:{$company}");
-
First off you should trim() the post data. THEN you need to determine which POST values are required to have data and test those before you generate the message variable $to = trim($_POST['to']); $from = trim($_POST['from']); $name = trim($_POST['name']); $company = trim($_POST['company']; $newcustomer = trim($_POST['newcustomer']); $address1 = trim($_POST['address1']); $address2 = trim($_POST['address2']); $subject = ("Event Rental for " . $name . "")); $description = trim($_POST['description']); $phone = trim($_POST['phone']); //Check required fields if(empty($to) || empty($from) || empty($name) || empty($company)) { //Required fields have no values //Provide error condition and don't generate email } else { //Required fields have values //Generate email }