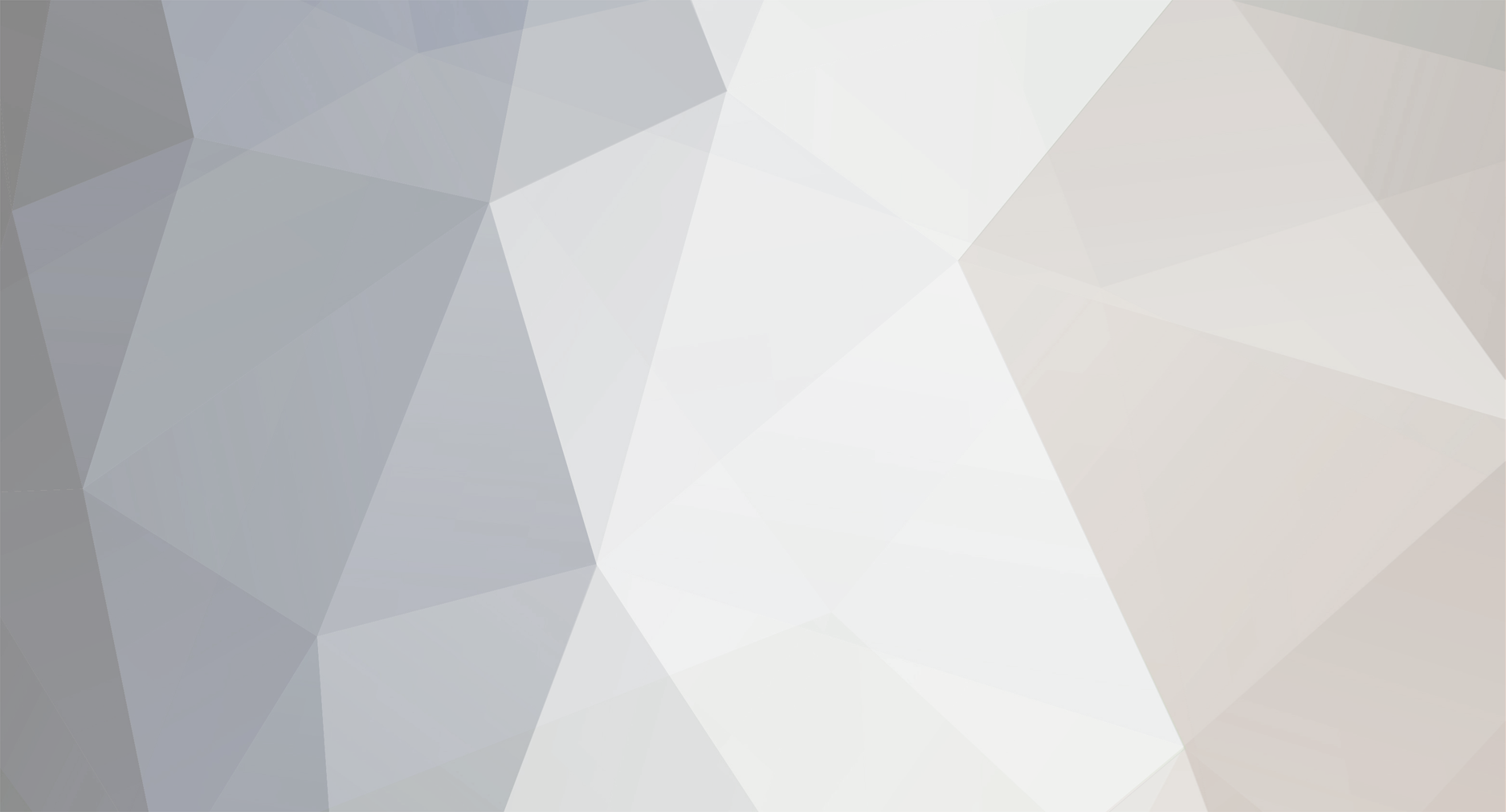
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Aside from the fact that the 2nd method you've shown won't work due to syntax errors, I assume you meant the use of mysql_real_escape_string() concatenated within the query string itself. No, there is nothing 'wrong' with it. I personally hate the second approach as it makes it difficult to read the query in an easy manner. But, then again I think both of those approaches are poor. I always advise against ever creating the query inside the mysql_query() function. I always create my queries as string variables then use those in the mysql_query() function. Then if there are any problems I can echo the query to the page for debugging purposes. By the way, this would be the correct syntax $data = mysql_query("SELECT * FROM table WHERE upper(email) = '" . mysql_real_escape_string($find) . "' LIMIT 1"); And, there is another option as well - sprintf(). I don't usually use this myself, but it does make the use of mysql_real_escape_string() to create your query - without the need to create temp variables - very easy. $query = sprintf("SELECT * FROM table WHERE upper(email) = '%s' LIMIT 1", mysql_real_escape_string($find)); $data = mysql_query($query);
-
There's no need to run individual queries to delete each record and including many OR's is inefficient as well.. A better option is to use an IN clause. Plus you need some logic to prevent SQL injection. if (isset($_POST["submit2"]) && $_POST["submit2"] == "DELETE SELECTED") { //Force POST values to be INTs $deleteIDs_ary = array_map('intval', $_POST['chkColor']); //Remove any 'false' value $deleteIDs_ary = array_filter($delete_ids); //Check that there was at least one valid value if(count($deleteIDs_ary)) { //Create comma separated string of the IDs $deleteIDs_str = implode(',', $deleteIDs_ary); //Create and run one query to perform all the deletes $query = "DELETE FROM crew_messages WHERE id IN ()"; if(mysql_query($query)) { echo "Records deleted successfully"; } else { echo "There was a problem running the query.<br>" . mysql_error(); } } else { echo "Invalid ID data passed."; } }
-
From what I see the login functionality, validation is done by running a query to get the values for "user_id", "first_name", and "user_level" where the username and password match what the user entered. If a record is returned then authentication "passes" and the result of the query are stores in a session variable: // Query the database: $q = "SELECT user_id, first_name, user_level FROM users WHERE (email='$e' AND pass=SHA1('$p')) AND active IS NULL"; $r = mysqli_query ($dbc, $q) or trigger_error("Query: $q\n<br />MySQL Error: " . mysqli_error($dbc)); if (@mysqli_num_rows($r) == 1) { // A match was made. // Register the values & redirect: $_SESSION = mysqli_fetch_array ($r, MYSQLI_ASSOC); You will notice on the second script you posted that there is a check for "first_name" as a session value to determine if the user is logged in or not. if (!isset($_SESSION['first_name'])) { Well, the user_id and user_level are both stored in the session as well. So, as long as you initiate the session [i.e. sessio_start() ] on each page load you can access those variables for any "logged in" user using: $_SESSION['first_name'] $_SESSION['user_id'] $_SESSION['user_level']
-
I don't "see" any problems with the query (although you went overboard on the parens). Have you validated that $country is getting set? Per the sprintf() function country should be an int - is that correct? Where is $user['county'] set? Assuming the query is not failing it is probably finding zero results due to a problem with the country value not being set correctly or not correct for what is in the database. Also, you are not sanitizing the user input which leaves you open to SQL Injection attacks. Try the following code to help debug the problem: $result = "-1"; if (isset($_POST['searchField'])) { $search_term = mysql_real_escape_string(trim($_POST['searchField'])); } $County= "-1"; if (isset($user['county'])) { $County = $user['county']; } $result = sprintf("SELECT Clubs.clubID, Clubs.name, Clubs.county, Clubs.logo, Clubs.postcode, Clubs.intro, Clubs.thumbsup, Clubs.cat, Category.*, County.* FROM Clubs INNER JOIN Category ON Clubs.cat = Category.catID INNER JOIN County ON Clubs.county = County.countyID WHERE ( Clubs.name Like %s OR Clubs.cat LIKE %s OR County.county LIKE %s OR Clubs.area LIKE %s OR Clubs.postcode LIKE %s OR Category.categorys LIKE %s ) AND County.countyID = %s", String($search_term . "%", "text"), String($search_term . "%", "text"), String($search_term . "%", "text"), String($search_term . "%", "text"), String($search_term . "%", "text"), String($search_term . "%", "text") String($County. "%", "int") ); echo "DEBUG:<br>"; echo " - 'result' is set as {$result}<br>\n"; echo " - 'County' is set as {$County}<br>\n"; echo " - Query:<br>{$result}<br>\n"; What is output from the debug code?
-
There is nothing to be gained from responding to this. I already provided a general approach. I'm not going to try and explain an entire methodology in a forum post. The easiest approach, IMO, for what you already have is to set specific variables in your main pages that will then be utilized by your header script. In addition to defining the area being displayed (so you can highlight the appropriate tab) you can set the page title so it is appropriate based upon the content being displayed. Example: In the main script for displaying articles: $content_area = "articles"; $page_title = "Article: " . $article_name; In the header script you could do something like this. (note: this is just an example, I'm not saying you follow this exact process.) //Define the tab class for each tab if(!isset($content_area)) { $content_area=='home'; } $homeClass = ($content_area=='home') ? 'highlight' : ''; $articlesClass = ($content_area=='articles') ? 'highlight' : ''; //Display the tabs echo "<a href='index.php' class='{$homeClass}'>Home</a><br>\n"; echo "<a href='articles.php.php' class='{$articlesClass}'>Articles</a><br>\n";
-
First, can you please explain what you were getting all frustrated about? Did I not do what you recommend with my original issue?? I'm not frustrated. I simply stated you needed to fix your logic for the tabs. Your current logic keys off the file names. That's a poor implementation in my opinion (many sites have several index.php files). I almost always use "controller" files which handle all the logic for my pages which allows me a great deal of flexibility. I set any number of variables on the "called" page which then includes the controller script which then does all the heavy lifting. You can solve this new problem in any manner you choose. I provided a solution for the problem you started this thread for - which caused a separate, unrelated problem. I gave you a suggestion on how to fix that. You can either take my suggestion or you can reject it.
-
Then you need to "fix" your logic for highlighting the tabs. I would not use the file names to determine that. I would suggest you set a variable in your controller pages before including the header file
-
What? Then why have you been complaining that the "else" condition was not executing - which would redirect the user to your home page? }else{ // Slug not found in URL. header("Location: " . BASE_URL . "index.php"); }//End of ATTEMPT TO RETRIEVE ARTICLE If you want the user to see the article directory then change the code in the else condition to load the article directory. I would still do exactly as I had proposed before, but just change the else condition to show the article index.
-
Looking at the sample data you need an array where the indexes are the option values and the values will be the displayed option text. So, all you need to do is iterate through the result set from the query and use the data to populate an array - which you would then feed to the form class. Here is an example: //sql code here * $serverName = "serverName\sqlexpress"; $connectionInfo = array( "Database"=>"dbName", "UID"=>"username", "PWD"=>"password" ); $conn = sqlsrv_connect( $serverName, $connectionInfo); if( $conn === false ) { die( print_r( sqlsrv_errors(), true)); } //Create and run query to get id & name $sql = "SELECT ticketID, ticketName FROM tickets ORDER BY price DESC"; $stmt = sqlsrv_query( $conn, $sql); //Use results to populate array $optionsArray = array(); while( $row = sqlsrv_fetch_array( $stmt, SQLSRV_FETCH_ASSOC) ) { $optionsArray[$row['ticketID']] = $row['ticketName']; } // single-option select box $obj = &$form->add('select', 'my_select2'); //Pass options array for the select creation $obj->add_options($optionsArray);
-
So if I have if (isset($_GET['slug']) && $_GET['slug']) { then my code should catch the missing slug value and my ELSE will kick in and I will re-drect the user to my Home Page, right? If you change the rewrite rule to work even when there is nothing following articles/ AND you change the if() condition to check that $_GET['slug'] is set AND has a value, then the else condition of that check should be executed Is this a fairly common technique? Don't know and don't care. It will work and does not require any special handling. Simplicity is best IMHO. Right, and there is no need for the additional check of the GET variable to see if it is empty (that was never the source of the problem anyway). However, it's not a bad idea to check for an empty value anyway. And, I 'assume' you have appropriate error handling of the slug in cases where the user submits a value for which there is no article. There are no "empty" folders. You would put the file that handles the article display into that folder with the name index.php or something that will be treated as a default. Then, the modrewrite rule would be changed to redirect to the new location. The elegant part of this solution is that if the user does not put an article name at the end the rewrite rule is not triggered, but the server will automatically load the right file and the slug will not be set. So: 1. Rename 'article.php' to 'index.php' and put into a sub-folder called 'articles' 2. Change rewrite rule to RewriteRule articles/([a-zA-Z0-9_-]+)$ articles/index.php?slug=$1 The only other changes you may need to make are to any relative paths to include files used in that script.
-
What we have here is a failure to communicate. Let me repeat what I stated previously in a different way: User enters: http://local.debbie/articles/ Your rewrite rule RewriteRule articles/([a-zA-Z0-9_-]+)$ article.php?slug=$1 will not do anything because there was NO MATCH! So, the server will try to load the URL as the user entered it - which does not exist and is causing the error you have. So you CAN'T use that "branch" in your code because it will never load that page. You have multiple options that will do what you want: 1. Change the rewrite rule to use an asterisk so the user will be redirected to article.php even if nothing was added at the end of the URL. If you do that then the slug will have an empty value and you would want to use thorpe's suggestion as well. 2. You can add an additional rewrite rule to handle this one specific scenario to redirect the user to article.php without a slug. Pretty stupid IMHO 3. You can create an 'articles' folder with a default file that can do whatever you want. It can redirect the user to another page, it can provide a message, whatever. Again, this woulod exists just for this one specific scenario which seems a waste to me. 4. (I like this the best). Change your article.php file to index.php and put it in a folder called 'articles'. Then change your rewrite rule to RewriteRule articles/([a-zA-Z0-9_-]+)$ articles/index.php?slug=$1. You would still use the same links that you are using now. All this would do is move the file that is loaded. But, now, if the user enters in articles/ with nothing on the end, then the same file will be loaded without a slug parameter. This is one of those types of solutions that "just works" I've given you four options that can all do what you need if implemented correctly.
-
OK, there's an even easier solution. If there is no data at the end of articles/ then the server will look for a default file in the "articles" subfolder (which may not currently exist). You could create that folder and add an index.php file which simply includes the articles.php file (assuming that is where you want it to go). Then no changes to the modrewrite would be needed. Also, the solution that thorpe suggested wouldn't be needed either.
-
That's what I was thinking as well. But, looking at the rewrite rule: RewriteRule articles/([a-zA-Z0-9_-]+)$ article.php?slug=$1 The plus symbol after the matching character classes stands for "one or more matches". Since there was no match, the server tried to load the URL as is articles/ - which is looking for a default file in that directory and it apparently doesn't exist. So, in addition to the change you suggested we would also want the mod rewrite rule to send the user to the correct page (even if the slug will be empty). I believe this can be achieved by changing the quantifier to an asterisk RewriteRule articles/([a-zA-Z0-9_-]*)$ article.php?slug=$1 Now, if the user has "articles/" as the end of the URL, the user will always be directed to "article.php" with the 'slug' set to the content after ""articles/" - which could be an empty value (which your suggestion will take care of). C'mon DoubleDee, you've been around long enough to know the difference between isset() and a check for an empty value
-
OK, I just looked at your login script and it really needs some work: case "Login": include("dbstuff.inc.php"); $cxn = mysqli_connect($host,$user,$password,$database) or die ("Query died: connect"); $sql = "SELECT user_name FROM Customer WHERE user_name = '$_POST[fusername]'"; $result = mysqli_query($cxn,$sql) or die ("Query died: fuser_name"); $num = mysqli_num_rows($result); if ( $num > 0 ) { $sql = "SELECT user_name FROM Customer WHERE user_name = '$_POST[fusername]' AND password = md5 ('$_POST[fpassword]')"; $result2 = mysqli_query($cxn,$sql) or die ("Query died: fpassword"); $num2 = mysqli_num_rows($result2); if ( $num2 > 0) //password matches { $_SESSION['auth'] = "yes"; $_SESSION['logname'] = $_POST ['fusername']; header (" Location: SecretPage.php"); } else //password does not match { $message_1="The Login Name, '$_POST[fusername]' exists, but you have not entered the correct passowrd! Please try again."; $fusername = strip_tags(trim($_POST[fusername])); include ("form_login_reg.inc") ; } // end if $num > 0 elseif ($num == 0) //login name not found { $message_1 = "The User Name that you entered does not exist! Please try agian."; include("form_login_reg.inc"); } break; 1. You do NOT need to be doing two queries. You have one query to get records matching the username and then another for those matching the username and password. That's not necessary. If you wanted to do the two different checks then just do one query to get the password of those record with the selected username. If there are 0 records returned you know the username doesn't exist. Then you could use the result of that query to check the password. But, that is a poor implementation. You should just tell the user that you were unable to validate their information. You should not give any information about "why" the validation failed (username vs. password). That give malicious users more information to try and infiltrate your application. 2. You are doing absolutely NO sanitizing of the user input and are wide open to SQL Injection! 3. Do not use yes/no for variables. For Booleans you should be using the logical True/False or 1/0 Give the code below a try for your login section. If login fails you should get the query on the page (for debugging only). Then check what values are in the database to see if the results are correct or not. However, I think I see your problem. In your code to INSERT the user record you are using the variable $password [with an MD5() hash]. However, that is the same password variable that you are using to connect to the database. It looks like you are converting the POST data into variables using the same name as the index foreach ($_POST as $field => $value) { $$field = strip_tags(trim($value)); } That is a BAD idea as this problem illustrates. User could potentially submit additional fields which could have the same names of other variables in your script to cause any number of problems. Looking at this specific example, if you did have a field called 'password' in the form, then the database connection line would fail because you are defining those variables before you connect to the database. If the db connection succeeds then you don't have a 'password' field in your form (at least not with that exact name). Sample, improved script for login process case "Login": include("dbstuff.inc.php"); $cxn = mysqli_connect($host,$user,$password,$database) or die ("Query died: connect"); $uname = mysql_real_escape_string(trim($_POST['fusername'])); $pword =md5($_POST['fpassword']); $sql = "SELECT user_name FROM Customer WHERE user_name = '$uname' AND password = '$pword'"; $result = mysqli_query($cxn,$sql) or die ("Query died: fuser_name"); $num = mysqli_num_rows($result); if (!mysqli_num_rows($result)) { $message_1 = "Unable to authenticate username/password! Please try again."; ##THIS LINE FOR DEBUGGING ONLY $message_1 .= "<br>Query: {$sql}"; $fusername = strip_tags(trim($_POST[fusername])); include ("form_login_reg.inc") ; } else { $_SESSION['auth'] = "yes"; $_SESSION['logname'] = $_POST ['fusername']; header (" Location: SecretPage.php"); } break;
-
I think you are not going to find anyone to read through all of your code to try and find errors. If you wrote this code you should be able to narrow the problem down to a specific section of code. You need to provide what input you are providing, the expected output and the actual output (along with any error messages). If you are getting a message that the username/password are incorrect when attempting to log in then you simply need to look at the conditions you are using. I would assume you are doing a query to find a matching record based upon the username and the hashed password. Therefor I would start by echoing the query to the page to make sure it looks valid (it could be as simple as a variable typo). Then try to run it through PHPMyAdmin. If that all checks out then look at the logic that follows that DB query.
-
When doing these types of queries it can be very difficult to create the entire query to start with. The best approach, in my opinion, is to create the query in steps. We can go ahead and jump to step 2 in your requirements above: 1. Create the query to get all of the products that the target user has scored SELECT product_id, score FROM prod_scores WHERE ps1.user_id = 1 Ok, so we have all the products that the user has scored (and the scores), but we also need all the scoring records by other users for the same products. So, we need to JOIN the record from teh same table where the product ID is the same (but we should exclude those records for the target user). But, sicne we will want some of the same fields we should give the fields dynamic names so we don't get confused with what field is what in the results. NOTE: The ps1 fields are those for the target user and the ps2 fields are those for other users. SELECT ps1.product_id, ps1.score as user_score, ps2.user_id AS other_user, ps2.score AS other_score FROM prod_scores AS ps1 LEFT JOIN prod_scores AS ps2 ON ps1.product_id = ps2.product_id AND ps2.user_id <> 1 WHERE ps1.user_id = 1 That gets us most of the way there. Now, we could calculate the difference while we iterate through the results, but we can do that in the query as well. Also, I assume, you need the product names and the user names - so I have added JOINs for those tables to get that info. NOTE: if you need both the username of the target user and the other users, then you will need to JOIN the user table twice. If you already have the target user's name, then you can remove that one JOIN (and the field fromt eh SELECT). And, we would want to ORDER the results by products to get them in a logic order for processing. SELECT u1.username as user_username, -- target user's username p.productname, -- product names ps1.product_id, ps1.score AS user_score, -- target user's scores u2.username as other_username, -- other user's usernames ps2.user_id AS other_userid, ps2.score AS other_score, -- other user's scores (ps1.score - ps2.score) AS difference -- calculated difference FROM prod_scores AS ps1 -- target user's scores JOIN products AS p ON ps1.product_id = p.id -- product names JOIN users AS u1 ON u1.id = 1 -- target users username LEFT JOIN prod_scores AS ps2 -- other user's scores ON ps1.product_id = ps2.product_id AND ps2.user_id <> 1 JOIN users AS u2 ON ps2.user_id = u2.id -- other user's usernames WHERE ps1.user_id = 1 ORDER BY ps1.product_id This should get you what you need. I have added comments to specific lines to illustrate the purpose of the line. A couple notes: 1. The LEFT JOIN for the self JOIN is needed so that the results will include scores from the target user if no other users have scored the same product. 2. The results include fields that are probably only needed for debugging purposes (i.e. to make it easier to "see" what the results are. For example, you may not need the user's names and the user's IDs. Plus, you if all you need is the difference you don't need the target user's score and the other user's score in the results. So, once you have validated the results are what you need you can remove the fields from the SELECT query that you don't need. Plus, if you don't need the product names or the user's names you can remove the respective JOINs
-
That script is very dangerous. You should never use data from the user without sanitizing it first especially when you are using it to access/read files. Anyway, you just need to check the result of each process that performs the reads/writes and provide an appropriate error message when there is a failure. You can suppress the error messages returned by PHP using the '@' symbol before the function. <?php include '../dbc.php'; page_protect(); $result = ''; if (isset($_POST['submit'])) { $file = $_POST['filename']; $target = $_POST['hosttarget']; if(!($file_contents = @file_get_contents($target))) { $result = "ERROR: Unable to retrieve file!"; } elseif(!($file_write = @file_put_contents($file, $file_contents))) { $result = "ERROR: Uunable to create new file!"; } else { $result = "Successfully uploaded {$file}!"; } } ?> <html> <body> <div id="result"><?php echo $result; ?></div> <div class="wrap"> <form enctype="multipart/form-data" action="" method="post"> <div class="download"> <div class="left">Target:<br>Filename:</div> <div class="right"> <input type="text" name="hosttarget" id="text" value=""><br> <input type="text" name="filename" id="text" value=""><br> <input type="submit" name="submit" id="submit " class='submit_btn' value="Get File"> </div> <div class="clear"></div> </div> </form> </div> </body> </html>
-
Laffin is correct, the WHERE clause was missing. I will say it again These are all simple mistakes and ones that would be easily found/fixed if I was working in a live environment. But, I am providing this code with absolutely no way to test with your script/database. I expect people in this forum to be able to debug simple errors. if ($letter=='#'){ $where = " WHERE INSTR('012345679', LEFT(title, 1)) > 0"; } else{ $where = " WHERE LEFT(UPPER(title), 1) = '$letter'"; }
-
I "fixed" the wrong one if ($letter=='#'){ $where = " INSTR('012345679', LEFT(title, 1)) > 0"; } else{ $where = " WHERE LEFT(UPPER(title), 1) = '$letter'"; }
-
Hmm . . . I think the problem is that when you use the BETWEEN operator MySQL is expecting a numeric comparison. So, it is converting the string value from the title into a numeric value. So, anything not a number is converting to 0. This should work if ($letter=='#'){ $where = " WHERE LEFT(title, 1) BETWEEN 0 AND 9"; } else{ $where = " INSTR('012345679', LEFT(title, 1)) > 0"; }
-
Then the query is failing. I provide code as a guide as to the process you can follow to achieve the desired result. I give no guarantees that it is error free (see my signature). I leave it to the recipient to debug any typos and such since I typically don't have the input data (e.g. your database) in order to easily test the code. I didn't check the syntax of the query that Muddy_Funster provided. The error is due to the format of the LEFT() command. He had three parameters, but LEFT() only takes two. Try replacing the if/else section for the where clause with this if ($letter=='#'){ $where = " WHERE LEFT(title, 1) BETWEEN 0 AND 9"; } else{ $where = " WHERE LEFT(UPPER(title), 1) = '$letter'"; }
-
One correction to Muddy_Funster's solution. The value of $letter will be "#" when searching for numeric titles. But, the code above assumes that the user will be selecting 1-9. A couple other things: The original code is appending a " | " after each link - including the last. I assume that is not what you really want (solved below). Also, the isset($letter) check is worthless since you set $letter on the previous line (even if $_GET['letter'] is not set $letter will be). Instead you should be checking if $_GET['letter'] is set. Plus, there is no validation of the input from sql injection. Lastly, the is no handling in the case where the GET value is not in the approved list of values which would cause the script to fail. <?php $alphabet = array('#', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'); foreach ($alphabet as &$letter) { $letter = "<a href=\"?letter={$letter}\">{$letter}</a>"; } $page_links = implode(' | ', $alphabet); echo $page_links; require("db.php"); $where = false; if(isset($_GET['letter'])) { $letter = mysql_real_escape_string(trim(strtoupper($_GET['letter']))); if ($letter=='#'){ $where = " WHERE LEFT(title, 1, 1) BETWEEN 0 AND 9"; } else{ $where = " WHERE LEFT(UPPER(title), 1, 1) = '$letter'"; } } //Only run page query if 'letter' was valid - i.e. $where is set if($where != false) { $query = "SELECT COUNT(*) as num FROM games $where"; $total_pages = mysql_fetch_array(mysql_query($query)); include("pagination.php"); $sql = "SELECT title FROM games {$where} LIMIT $start, $limit"; $result = mysql_query($sql); echo "<table>\n"; while($row = mysql_fetch_array($result)) { echo "<tr><td>{$row['title']}<br></td></tr>\n"; } echo "</table>\n"; echo $pagination; } ?>
-
So, instead of keeping the records its better to maintain duplicate data? But, if you follow the second suggestion I proposed you could have a table that maintains each user's inbox. The minimum that table needs is two columns - the user_id and the message_id. Then, when a user deletes a message you can delete the record that associates the message with the user's inbox. But, you would still maintain the original record for the purpose of the sending user's outbox. But, I would use the same process for outbound messages as well. You could have a separate tables for users' inboxes and outboxes, but it makes more sense to use one table. That way you can easily add the ability to allow users to create custom folders. So, the table might look something like this: user_id, message_id, folder_id Where folder Id is a foreign key reference to values in a table of folders. You could hard-code the inbox and outbox for all users and allow users to create custom folders. Then when a user "deletes" a message you simply delete the record that associates the message with the user's folder. Then you could do a second query to delete the message IF it is not associated with any other user (i.e. if both the sender and recipient have deleted the message). But, that probably isn't necessary. If you properly index your database, it shouldn't have a problem with tens of thousands (if not hundreds of thousands) of records. And, if it does become a problem then you can archive/delete messages for which there are no users who have references to them.
-
Why are you using $i in that logic? Before the code for creating the ext/Prev links $i was used to create individual page links and it *should* be equal to $total_pages. So, you should use $total_pages. There is a reason we give variables meaningful names. OK, typically the Prev/Next links come before and after the page links so you'll want to implement is accordingly. Plus, the first and last links are usually disabled if you are on the first or last page already. Also, the current page is not displayed as a link. Lastly, you will typically want to implement some restrictions on the number of pages that would be displayed in case there are many. By the way, your code is referencing $page, but you don't have such a variable defined. I think you meant to use $show_page Give this a whirl. Not tested, so there may be some typos //Create First and Previous links if($show_page>1) { $prev_page = $show_page-1; $prev = "<a href='events3.php?page={$prev_page}'>« Previous</a> "; $first = "<a href='events3.php?page=1'>«« first</a> "; } else { //On first page, create as non-links $prev = "« Previous"; $first = "« first"; } //Create last and Next links if($show_page<$total_pages) { $next_page = $show_page+1; $next = "<a href='events3.php?page={$prev_page}'>Next »</a> "; $last = "<a href='events3.php?page={$total_pages}'>last »»</a> "; } else { //On last page, create as non-links $next = "Next »"; $last = "last »»"; } //Create individual page links $page_range = 5; //Max # of individual pages to display before & after current page $pages_start = max($show_page-$page_range, 1); $pages_end = min($show_page+$page_range, $total_pages); $start_ellipse = ($pages_start>1) ? '...' : ''; $end_ellipse = ($pages_end>$total_pages) ? '...' : ''; $pages = ''; for ($pg = $pages_start; $pg <= $pages_end; $pg++) { if($pg != $show_page) { $pages .= "<a href='events3.php?page={$pg}'>{$pg}</a> "; } else { $pages .= "{$pg} "; } } echo "</div><p class='pagination'>"; echo $first . $prev . $start_ellipse . $pages . $end_ellipse . $next . $last; echo "</p>";
-
You are going about this the wrong way. Just because a user "deletes" a message from their inbox does not mean you have to delete the database record. I assume that users that send messages have an outgoing box with teh messages that they can delete as well. I can see two different approaches: 1. In the one table for messages you can create two columns - one for sender_delete and the other for receiver_delete. Set the initial values to 0. Then, when a sender/receiver "deletes" the message in their inbox/outbox set the appropriate value to 0. Then just change your select queries accordingly to only retrieve messages that are not deleted by the user for which you are fetching. 2. This will be a little more work, but would be more "proper" from a DB normalization perspective. Have one table for the messages, then a separate table to define the inbox (and folders) for a user. So, when a message is sent you would add the record to the messages table and add a record for the recipient in the "inbox" table. Then when the user "deletes" the message you would just delete the message from the inbox table - not the actual message.