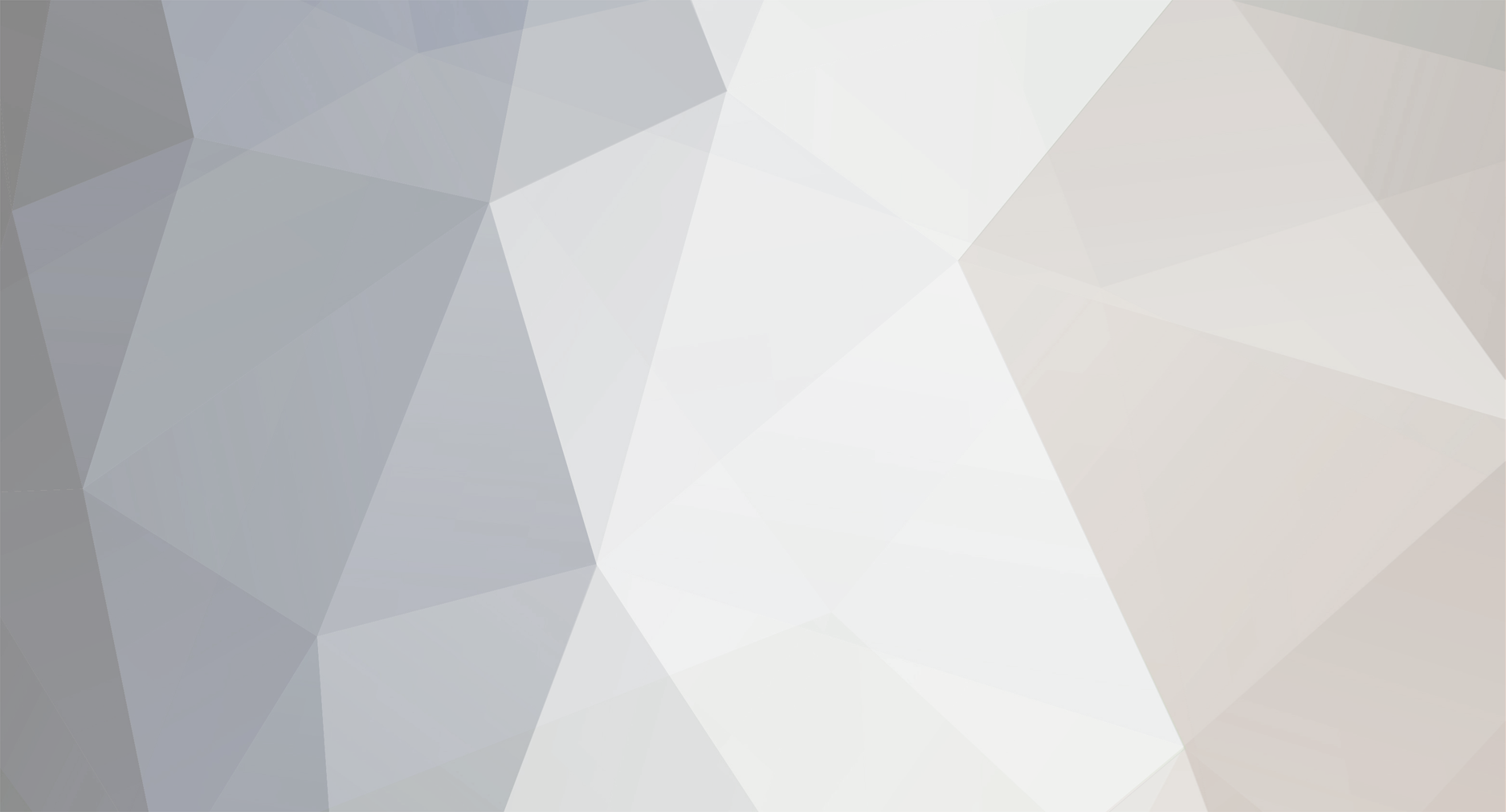
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
XAMPP runs fine on a 64bit Windows machine (at least for me). Are you trying to run XAMPP on 64bit Linux?
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=345840.0
-
What you are asking about is really a JavaScript question and not a PHP question (unless you want to have the user submit the page whenever they select a country). You have several options on how to implement this and the "best" solution for your situation will depend on a few factors. 1. If you have a somewhat limited list of countries and provinces, I would gather all the data and write it out as JavaScript arrays on the page, then handle all the functionality client-side. How many is really personal decision. But, this solution will give the best possible performance. 2. Another option is to utilize AJAX (JavaScript + PHP). The JavaScript code would handle all the same logic as above (detecting a change in country, repopulating the list, etc.) But in this situation the JavaScript would make a "silent" call back to a PHP script to get the list of new provinces to populate whenever the user changes the country. So, there would be a slight performance hit for this. But, with this solution you don't need to download all the Countries/Provinces to the user's PC. 3. As stated originally, you could require the user to submit the page every time they change the country. NOt a very good solution, but you probably need to allow for this with any of the other solutions unless you want your page to be unusable by those without JS enables 4. There is one other solution I can think of. I once built a photo gallery that was completely run by JS. You can use a script tag to include an external JS file. Then dynamically change the source of the file (in this case the list of provinces) on the fly. That was many years ago and I don't know if it would be fully supported in all current browsers. [Moving to JavaScript forum]
-
That's the same process I typically use. However. in the interest of efficiency I would not do the isset() test on each iteration of the loop. Just do it once before the loop and set a variable to test against. $selected_guess = (isset($_POST['guess'])) ? : $_POST['guess'] : false; for ($i = 1; $i < 11; $i++) { $selected = ($selected_guess === $i) ? 'selected="selected"' : ''; echo "<option value='{$i}' {$selected}>{$i}</option>"; }
-
Do not use global in your function. Instead, pass $skus as an additional parameter to the function. I don't see in your code where you are using the ['sizes'] array currently. I see a function call to makesizes(), but you aren't passing anything to that function so I don't know how it would even be working. You show what you want to have happen when there are no sizes, but not what you want to happen when there are sizes. Plus, there are other problems with your code since you didn't follow the advice I gave you previously. You don't need to define all the versions of the band name since you can programmatically create the uppercase and lowercase versions. Besides, you don't pass the band name(s) to your function, so they will not have values in the function. So, you need to pass the band name to the function as a parameter as well. Same goes for the SKU. Based on your prior thread I believe you wanted to make the sizes a drop down list? If so, you can create the makeSizes function accordingly. But, your current code has the beginning/ending SELECT tag hard-coded. That needs to be part of the makeSizes function also. $BANDNAME = "Band Name"; function makeSizes($sizesArray) { if(count($sizesArray)==0) { echo "<input value='{$SKUCAPS}{$skunum}' type='hidden' name='item'>"; } else { echo "<select name='item'>\n"; foreach($sizesArray as $size) { echo "<option value='{$size}'></option\n"; } echo "</select>\n"; } return; } $skus = array( 'shirts' => array( '165' => array( 'name' => 'World Khaos Tour <br> Limited Edition Tour Track Jacket.', 'price' => '70.00', 'sizes' => array('M', 'L', '1X' ) ), '139' => array( 'name' => 'KHAOS LEGIONS ALBUM COVER', 'price' => '15.95', 'sizes' => array('S', 'M', 'L', '1X' ) ), ), //end product type 'misc' => array( '603' => array( 'name' => 'Ring Button', 'price' => '3.00', 'sizes' => array('none') ), '602' => array( 'name' => 'Logo Button', 'price' => '3.00', 'sizes' => array('none') ) ) //end product type ); //end skus function makeProduct($skus, $type, $BANDNAME, $SKU) { $BANDCAPS = strtoupper($BANDNAME); $BANDLOWER = strtolower($BANDNAME); $SKUCAPS = strtoupper($SKU); $SKULOWER = strtolower($SKU); foreach ( $skus[$type] as $skunum => $value) { $price = $skus[$type][$skunum]['price']; $name = $skus[$type][$skunum]['name']; echo "<!-- PRODUCT BEGIN --> <li class='product' > <a href='images/{$SKULOWER}{$skunum}lg.jpg' rel='lightbox'><img src='product_files/{$SKULOWER}{$skunum}.png'></a><br> <strong>{$name}</strong><br>{$SKUCAPS}{$skunum}<br>\${$price}<br> <form name='{$SKUCAPS}{$skunum}' method='GET' target='_blank' action='http://www.jsrdirect.com/cgi-bin/Make-a-Store.cgi'> <input type='hidden' name='band' value='{$BANDCAPS}'> <input type='hidden' name='back' value='http://www.jsrdirect.com/bands/{$BANDLOWER}/index.html'> <strong>Qty:</strong> <input type='text' name='quantity' size='1' value='1' > <strong>Size:</strong> ".makeSizes()." <br><br> <input class='css3button' value='Add To Cart' type='image'> </form> </li> <!-- Product End -->"; } }
-
Extending a class - why can't I access a parent method in a child
Psycho replied to kn0wl3dg3's topic in PHP Coding Help
You need to turn on error reporting. If you did you would see the following error message: Make the property public. -
I think you are still making this more difficult than it needs to be. If I understand you correct you have four different options of which the user will enter an amount, correct? Then just give the user a radio button group to select the offer and one field to enter the amount or have the four radio button options with a text field for each one. Then you only need to check the text field associated with the offer selected.
-
Here is a rough example. Probably not the best implementation for your needs because I don't know enough about your current code or implementation Radio group options Select an offer:<br> <input type="radio" name="offer" value="OFFER_VALUE">Offer Value<br> <input type="radio" name="offer" value="OFFER_PERCENT">Offer Percent<br> <input type="radio" name="offer" value="OFFER_PRICE">Offer Price<br> <input type="radio" name="offer" value="SHIPPING_PRICE">Shipping Price<br> PHP code to validate $valid_offers = array('OFFER_VALUE', 'OFFER_PERCENT', 'OFFER_PRICE', 'SHIPPING_PRICE'); if(isset($_POST['offer']) && in_array($_POST['offer'], $valid_offers)) { $offer = $_POST['offer']; } else { echo "You must select a valid offer."; }
-
As titan21 stated you should be using a Radio Group. This requires the user to select one, and only one, value. No matter which one is selected, the value is passed as the same field name. But, you would still need to validate that the value passed is one of the "approved" values. But, that is a trivial exercise. You can compare against an array of valid values or do a DB query. You are making this much harder than it needs to be.
-
The correct terminology is "not equal to". The correct implementation is if($today!=$projected) { } http://php.net/manual/en/language.operators.comparison.php
-
Ah well, since I already did this work, I'll give you this. Here is your current code which is properly indented to show logical structure. <?php session_start(); if(isset($_SESSION['uname2']) || ($_SESSION['section'])) { $con = mysql_connect("localhost","root",""); if (!$con) { die('Could not connect: ' . mysql_error()); } if(isset($_POST['approve_x'], $_POST['approve_y'])) { mysql_select_db("dbreg", $con); $uname = $_SESSION['uname2']; $sec = $_SESSION['section']; $sql="SELECT * FROM account where sec='$sec'"; $result=mysql_query($sql); if(mysql_num_rows($result)) { while($row = mysql_fetch_assoc($result)) { $fullname = "" .$row['famname']. ", " .$row['gname']. " " .$row['mname']. "."; } foreach ($_POST['list'] as $checkbox) { $sql="SELECT * FROM account WHERE sec='$sec'"; } $result=mysql_query($sql); if(mysql_num_rows($result)) { while($row = mysql_fetch_assoc($result)) { $username = $row['Username']; $password = $row['Password']; $gname = $row['gname']; $mname = $row['mname']; $famname = $row['famname']; $sec = $row['sec']; $studnum = $row['studnum']; $fullname = "" .$row['famname']. ", " .$row['gname']. " " .$row['mname']. "."; $username = stripslashes($username); $password = stripslashes($password); $username = mysql_real_escape_string($username); $password = mysql_real_escape_string($password); $gname = stripslashes($gname); $famname = stripslashes($famname); $gname = mysql_real_escape_string($gname); $famname = mysql_real_escape_string($famname); $mname = stripslashes($mname); $mname = mysql_real_escape_string($mname); foreach ($_POST['list'] as $checkbox) { mysql_query("INSERT INTO dbaccount (Username, Password, gname, famname, mname, sec, studnum, fullname) VALUES ('$username', '$password', '$gname', '$famname', '$mname', '$sec', '$studnum', '$checkbox')") or die(mysql_error); mysql_query("DELETE FROM account WHERE sec='$sec'") or die(mysql_error); } echo '<script type="text/javascript">{alert("Approved!");}</script>'; echo '<meta http-equiv="REFRESH" content="0;url=approvereject2.php">'; mysql_close($con); } } else { echo '<meta http-equiv="REFRESH" content="0;url=approvereject2.php">'; } } elseif(isset($_POST['reject_x'], $_POST['reject'])) { mysql_select_db("dbreg", $con); foreach ($_POST['list'] as $checkbox) { $sql="SELECT * FROM account WHERE sec='$sec'"; } $result=mysql_query($sql); if(mysql_num_rows($result)) { foreach ($_POST['list'] as $checkbox) { mysql_query("DELETE FROM account WHERE sec='$sec'") or die(mysql_error); } echo '<script type="text/javascript">{alert("Rejected!");}</script>'; echo '<meta http-equiv="REFRESH" content="0;url=approvereject2.php">'; } else { echo '<meta http-equiv="REFRESH" content="0;url=approvereject2.php">'; } } else { echo '<meta http-equiv="REFRESH" content="0;url=approvereject2.php">'; } } } else { header("location:failunautho.php");; } ?>
-
You should format your code using indents to show the logical structure for if/else conditions, loops, etc. Otherwise it becomes unreadable. You should also be using comments. It's kinda hard to understand your code when you simply copy/paste large blocks of code where most of it has nothing to do with the problem. The only checkbox I see in your code is this one: echo "<input type='checkbox' name='list[]' value='".$row['Username']."'>".$row['famname'].",".$row['gname']. ",".$row['mname']. ".<br/></td>"; Then in your second script you use the POST data for 'list' in FOUR different places!!!: foreach ($_POST['list'] as $checkbox) { $sql="SELECT * FROM account WHERE sec='$sec'"; } This is worthless. All this does is redefine the string $sql over and over, but doesn't use it. You then run $sql after the loop completes - so the query is only run for one value - the last one. foreach ($_POST['list'] as $checkbox) { mysql_query("INSERT INTO dbaccount (Username, Password, gname, famname, mname, sec, studnum, fullname) VALUES ('$username', '$password', '$gname', '$famname', '$mname', '$sec', '$studnum', '$checkbox')") or die(mysql_error); mysql_query("DELETE FROM account WHERE sec='$sec'") or die(mysql_error); } You should never run queries in loops!!! foreach ($_POST['list'] as $checkbox) { $sql="SELECT * FROM account WHERE sec='$sec'"; } Same as the first one. All this does is redefine the string $sql over and over, but doesn't use it. You then run $sql after the loop completes - so the query is only run for one value - the last one. foreach ($_POST['list'] as $checkbox) { mysql_query("DELETE FROM account WHERE sec='$sec'") or die(mysql_error); } You should never run queries in loops!!! There is just so much wrong with the code that I don't know where to begin. I'll try to give you some help. Do not set the value of the checkboxes as the username. You should be using the unique ID of the records. I was going to try and pick through your code to find what you need to be doing, but I jsut don't have the time today. But, you are doing way more queries than you need to. For example, you don't need to query one table for values to then insert into another table. You can do an INSERT/UPDATE query that automatically inserts data from another table. I think you need to get out a pencil and paper and work out your logic of what you are trying to achieve and go back and take another run at it.
-
I thought I tried to explained that. The mail() function is built to work with the existing email standards. The manual even provides a link to those standards if you are so inclined to read them. The format for the FROM value (and all the other values) is dictated by those standards which have nothing to do with PHP. And, the FROM does NOT have to be in that format (i.e with the <>). That format is only required if you want to include a "friendly name" along with the email address. The reason they are adding the text "From:"? The $headers parameter can take many different values. If you don't specify the name of the header for the value how are the email servers supposed to know what it is? As stated previously you can set the from value in the php.ini file, in which case it isn't required for you to set it in the $headers parameter. So, if you were to set a value of "me@mydomain.com" without naming the header it is for, it could be the FROM address, the Return address, or a CC, or BCC, etc. I guess they didn't make it "Englishy" enough.
-
I assume then that the error is with POST data? I wouldn't do a if(isset(.....)){...} in every place the error occurs - but instead I would define a variable based upon the POST field and use the variable. In fact, I'd use a function so I can also aply trim(0 to the value or anything else I need to do. function getPostVar($varname) { if(isset($_POST[$varname])) { return trim($_POST[$varname]); } return ''; } $name = getPostVar('name'); $email = getPostVar('email'); $phone = getPostVar('phone'); //etc. Just an example.
-
As I already told you in my first response - just append the session variable to the end of the links. I'm really struggling why either you are not understanding what I am saying or why I am not understanding what you are asking. //Query the links in the DB $query = "SELECT link FROM links_table"; $result = mysql_query($query); //Output the links with the session variable while($row = mysql_fetch_assoc($result)) { echo $row['link'] . $_SESSION['login'] . "<br>\n"; }
-
Problem with view next page record in paginated pages
Psycho replied to IreneLing's topic in PHP Coding Help
You are getting the $_GET['id']; from the originating page. So, you *should* just need to add that parameter to the links that you create if($Page!=$Num_Pages) { if($_GET["txtFirstName"] == "" && $_GET["txtLastName"] == "") { echo " <a href ='$_SERVER[sCRIPT_NAME]?Page=$Next_Page&txtCell=$_GET[txtCell]&id=$groupnumber'>Next>></a> "; } else if($_GET["txtFirstName"] == "" ) { echo " <a href ='$_SERVER[sCRIPT_NAME]?Page=$Next_Page&txtLastName=$_GET[txtLastName]&id=$groupnumber'>Next>></a> "; } else { echo " <a href ='$_SERVER[sCRIPT_NAME]?Page=$Next_Page&txtFirstName=$_GET[txtFirstName]&id=$groupnumber'>Next>></a> "; } } -
This topic has been moved to PHP Freelancing. http://www.phpfreaks.com/forums/index.php?topic=345722.0
-
Problem with view next page record in paginated pages
Psycho replied to IreneLing's topic in PHP Coding Help
If you are not passing $groupnumber the the secondary pagination script on the next page loads,then yes, that is the problem. The easiest solutin would be to probably add the groupnumber to the pagination links on the secondary page. -
What makes you think you need to put the code within an invisible css box? You can have code that executes without having an output element. For example, let's say you have a form to add a record that POSTs back to the main page (this is not a working script, just a POC) <?php if(isset($_POST['name']) { $name = mysql_real_escape_string(trim($_POST['name'])); $query = "INSERT INTO users (name) VALUES('$name')"; $result = mysql_query($query); } ?> <html> <body> Welcome to the home page </body> </html>
-
This forum is for people to get help with code they have written. You can either post in the freelance forum to see if anyone wants to do this for you (for $$$) or you can do a simple web search to find a tutorial for using cURL. Give the tutorial a try and if you have problems, post back here with what you have, what problems you encountered, etc. and we will try to help.
-
You don't need AJAX. That's not to say you can't use it, but I think it would be overkill for this. Make it work with a normal page load and you can always add AJAX loading later if you want. Looking at the first script you have the "base" HTML file and nothing is being output inside the content DIV. You simply need to have your links reload the same page and pass a parameter on the query string so you can load the images. Give me a few minutes to review the code. EDIT: Hmm, I would have to see more of the code. But, that would take time to review. Simply have all your URLs point to your default page along with parameters on the URL so you can determine what content to create and display in the content div. E.g. <a href="?action=load_gallery&id=15">Christmas Gallery</a> Then in the default page, or an included() file, add a controller script to get the values from the GET var to determine what to do.
-
http://php.net/manual/en/book.curl.php
-
And, how are you determining 15 days? You shouldn't need any special handling for leap years if you did it right. It would just "work". //Create timestamp 15 days in future $ship_date_ts = str_to_time('+15 days'); //Format the ship date $formatted_ship_date = date('m-d-Y', $ship_date_ts);
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=345714.0
-
Well, how you should approach this will be directly related to "how" these pages are different. I would likely have a "core" page (or pages) for much of the functionality. But, what is included in the core page(s) would be determined by what was similar. For example, if the sign-up pages are only different with respect to style, then the core pages could handle all of the form creation, validation and processing. Or, let's say all the users will be inserted into a main 'users' table, but depending upon the user type there will be additional data inserted into associated tables (admins, clients, etc.). If there is a login system you definitely want ONE table to hold the 'parent' user record. In that case you want some common code to do the validation/insertion of the main user data and then separate, specific processes to do the same for the type specific data. There are countless permutations I can think of, all of which would use a different solution.