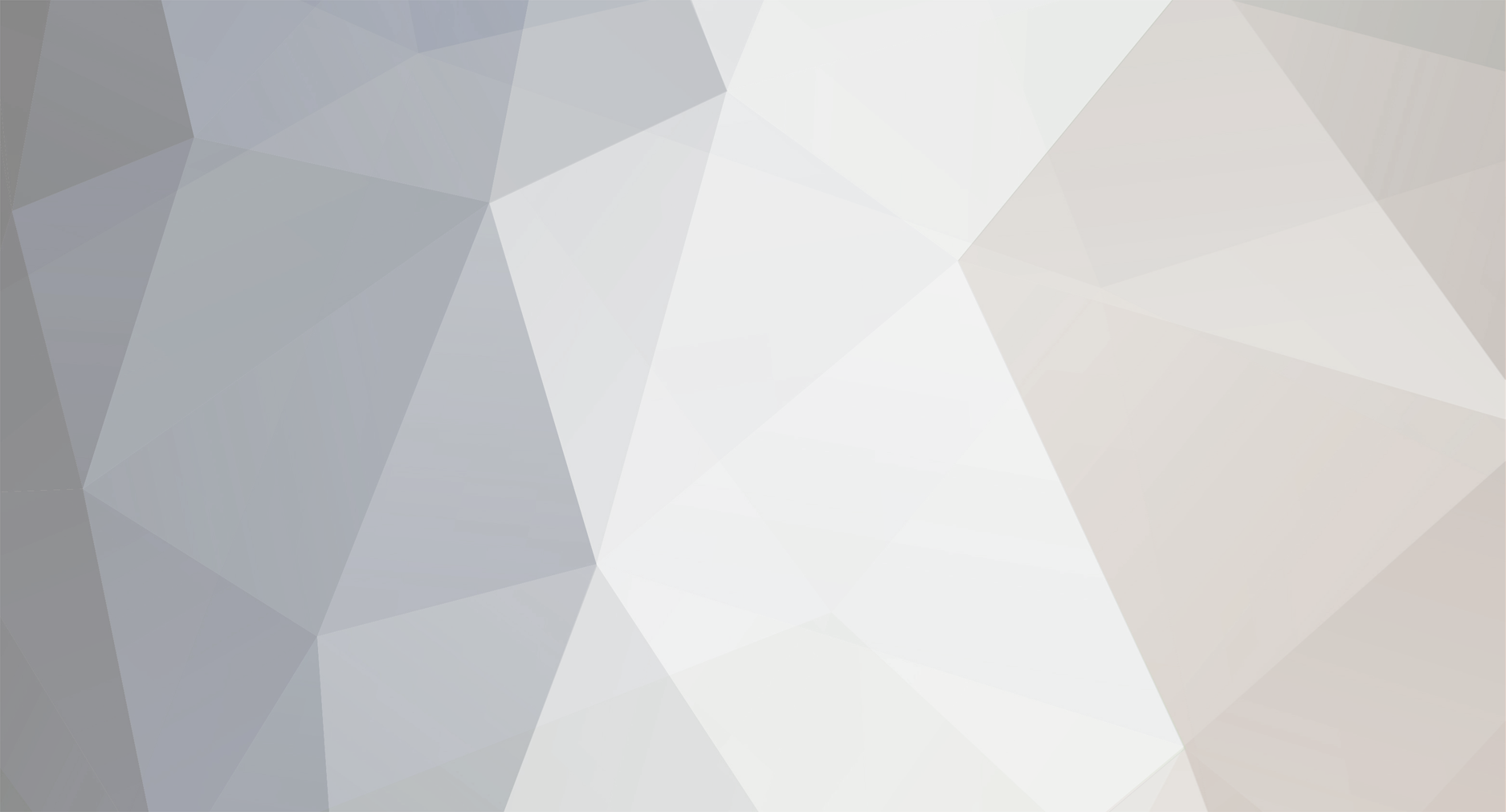
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I think your DB structure is a little out of whack. Not sure why you would need to use DISTINCT on the table that presumably stores the details of the list items. There should only be one record per list item. Also, you are storing the list name in the "list_subscriber" table. You should be storing the ID of the list item - why have an ID for your list items and not use it?. You should really reevaluate your DB design. Also, it will make your life a whole lot easier if you standardize your queries. By this I mean putting SQL functions in ALL CAPS, adding line breaks and spacing to give contextual meaning to your query. It is almost impossible for me to understand your first query without copying it into a text editor and doing that. But, give this query a try with what you have. SELECT distinct(ld.listname) as listname, IF(ld.listname=ul.listname) AS checked FROM list_details AS ld LEFT JOIN (SELECT ls.username as email, ls.id, ls.listname FROM users AS u JOIN list_subscriber AS ls ON ls.username = u.username WHERE u.id = $eid ) AS ul ORDER BY ld.listname
-
If you were to simply echo out the values you *think* you have you would see the problem. I added these lines before the first if statements and the results are indicated in the comments. echo "Firstday: $firstday<br>\n"; // Firstday: 1969-12-28 echo "Sunday: $sunday<br>\n"; // Sunday: 2011-10-02 echo "Lastday: $lastday<br>\n"; // Lastday: 1969-12-27 Your logic for determining the days seems overly complicated to me. If you were to explain what days you are trying to define, there might be a simpler method than all the nested strtotime() functions.
-
Unfortunately, computers suck at interpretation. A human can easily see that the period in "3.5" is not the end of the sentence. Now, that's not to say you can't make a better pattern. For example, to determine the end of a sentence you should be looking for a period followed by a space or the end of the text. That's the best pattern I can think of. There could be plenty of valid scenarios where that logic would fail. You either have to make more complex patterns or live with the limitations. I don't think you need regular expression at all. IN fact, you are using deprecated function. Try this $firstSentence = substr($text, 0, strpos($text, '. '))
-
You state that you are getting the slash on the returned data. I think your problem is that you are probably using mysql_real_escape_string() (or possibly addslashes()). But the problem is that your server probably has magic quotes enabled. So, when you send data through POST/GET/COOKIE the server is automatically adding slashes. So, when you then run the input through mysql_real_escape_string() it is escaping the slashes to make them part of the data. Magic quotes are deprecated and should be disabled. If you cannot disable them through the ini file, then you should add code to dynamically remove them at run time. http://php.net/manual/en/security.magicquotes.disabling.php
-
Well, you shouldn't need TWO queries to begin with. You can almost certainly get the data you need with ONE query. But, looking at the data you have above the results are correct. Not only do the values do not match - but you are using in_array() on two string values - not arrays.
-
You can't do a 'numeric' comparison on a formatted date (i.e. 2011-10-10). Once you create a formatted date, it is a string as far as PHP is concerned. So, when you try a comparison such as <=, PHP converts the values to a format that would make sense with such a comparison (i.e. numeric). You might as well be asking if("car">="boat") Create the dates as timestamps and then compare those.
-
Um, yeah. The fact that the PHP script is being initiated via an AJAX call is irrelevant as to what you can do in PHP. At least I can't think of anything that you can't do from an AJAX call that runs a PHP script than one that is run via a call from the browser's location bar.
-
Not sure what you mean by sending the cookie through AJAX. You can read and write cookies through both JavaScript and PHP. So, there is no need to "send it through AJAX".
-
$file = "I went back home to see my family as I was studying in China. By the time I arrived home I was so hungry and the weather was cloudy."; $words = array("see", "reader", "stud", "China", "cloudy", "hungry", "answer", "el", "prefer"); $pattern = '/\b(' . implode("|", array_map("preg_quote", $words)) . ')\b/i'; preg_match_all($pattern, $file, $matches); //Remove duplicates and convert to lower case for matching $found_words = array_map('strtolower', array_unique($matches[0])); //Show all words, indicating matches foreach ($words as $word) { $found = (in_array(strtolower($word), $found_words)) ? 1 : 0; echo "$word : $found</br>"; }
-
From what I understand, it's supposed to add a backslash in front of each quote so as to prevent injection. My problem is, why doesn't it? It DOES. The slashes tell the MySQL engine to treat those characters after the slash as literal characters and to treat it as part of the text value and not as a delimiter (i.e. quotes marks used to delineate a string). But, it does NOT insert the slash. That would just be stupid. If mysql_real_escape_string() was not working, your query would be failing due to the single quotes.
-
Ok, aside from your stated problem there are some things in that code that don't make sense. For example, why do you have these lines if ( $row[2] != $members_id ) continue; Don't query querying all records when you only want the records where the credit_member_id matches that value. Use a WHERE clause to only return the records you want. You are already using the DB variable @rows - so no need to get ALL records Second, you are grouping by credit_members_id, but calculating view on id - that doesn't seem right. Third, your logic to add the ordinal suffixes ("st', 'nd' and 'rd') has a problem. The values 11, 12, and 13 will be given the wrong value. There are some simple functions you can use if you just google. Lastly, use the "names' of your values from the query instead of referring to them by their index. It makes your code easier to read and less error prone (i.e. adding/removing fields from the SELECT statement could break your code). Give this a try function ordinal_suffix($n) { $n_last = $n % 100; if (($n_last > 10 && $n_last < 14)){ return "{$n}th"; } switch(substr($n, -1)) { case '1': return "{$n}st"; case '2': return "{$n}nd"; case '3': return "{$n}rd"; default: return "{$n}th"; } } mysql_query("SET @rank = 0;"); $query = "SELECT rank, views FROM (SELECT @rank:=@rank+1 AS rank, COUNT(credit_members_id) AS views FROM vtp_tracking GROUP BY credit_members_id ORDER BY views DESC) WHERE credit_members_id = '$members_id'" $result = mysql_query($query); if(!$result) { echo "No result found."; } else { $row = mysql_fetch_assoc($result); $rank = ordinal_suffix($row['rank']); echo " You are in {$rank} place with {$row['views']} views."; }
-
You are asking a lot of questions. Some have easy glutinous and some have more difficult ones (if any). First though, I think you need to ask your self if you need to create hard-coded reports. For example, if these reports are generated from data in a database then you can probably create a reporting feature to pull reports for any previous week dynamically. Then there is no need to create these reports on a weekly basis in some sort of flat-file format that you need to maintain. But, assuming you have to create the flat-file reports, there are many options. I think the easiest is as MarPlo suggested - create HTML reports. I wouldn't even go the PDF route. HTML documents have the following benefits: utilizes a format that you should be familiar with if you do web development, you can format the content very easily (bold, color, etc.) and it has "pretty good" handling of tabular data (i.e. data in tables) so that the data will try to fit within a page horizontally without being cut off (assuming you don't hard code the width to be too wide). It does have some disadvantages. If you want images yu will need to store them as separate files. HTML is not very "smart" on creating page breaks. You can try to programatically insert page breaks, but it can be a pain. Note, however, if you use HTML as an intermediary to create another file type (e.g. PDF) you will still have this problems. IF you just want PDF, there are libraries within PHP as well as many classes available to allow you to pretty easily create PDF documents. But, depending on how complex the pages need to be this can be easy or difficult. You can also create Excel documents. However, 'true' Excel documents are rather complex and you would have to find/buy a class to do that for you. But, it is rather simple to create either CSV documents (no formatting) or HTML documents (i.e. have formatting) and save them as an xls document. They will then open in Excel, but the user might get a warning when doing so that the format is not an Excel document.
-
I really have no idea what you are talking about now. What EXACTLY are you trying to accomplish? What is supposed to mean? Are you wanting the replacement to happen when you output the values to the page, are you wanting to change the values in the database, or what? You are not making any sense. If you are wanting to make the replacements as you output to the page, then str_replace() is a viable option. But, as I stated above, if the values from the DB are only the links, you don't need any special handling, just append the session value as you output the URLs. If you are trying to update the values IN the database there are a couple of simple solutions - but again, I would need to know if the "values" consist of only the URLs or if the URLs are embedded within more text of the DB value. In other words, would http://mysite.com/index.php?page=offers&blah=blah&sid= be the entire DB value for the field, or would it be something like Text before the link http://mysite.com/index.php?page=offers&blah=blah&sid= text after the link?
-
If the "links" are the complete value from the database, you don't need a replace function - just append the session value to the end of the links. But, if the links are embedded in the values from the database, then you would use a replace function. There is a very simple function you could use called str_replace()
-
No, you would have to build your own function to do something like that
-
Form fields, checkboxes, and radio button lose data on refresh
Psycho replied to Scott B's topic in PHP Coding Help
Where is your code? -
Need Help Removing Certain Elements From Array
Psycho replied to savagenoob's topic in PHP Coding Help
It would be more efficient to change your processing code to simply skip the fax elements. But, yes, you can remove the fax elements first. It would be helpful to see the relevant code you have now to see what would be the best way to implement though. -
Form fields, checkboxes, and radio button lose data on refresh
Psycho replied to Scott B's topic in PHP Coding Help
Yes. When the page is submitted, and there is a validation error, you need to repopulate the fields based upon what the user submitted. How you would do this would be based on the current logic of your page(s). -
Look for this: <a href="/server_info/76.73.3.42:1716/top_players/?searchipp=50&searchpge=2#search"> > </a> That is the > button for the next page. The searchpage value will be variable. But, if that link exists, that means there is another page. When there are no more pages the > on the page is not a link <span> > </span> So, as long as that link exists you know there is another page. So, just create a while() loop to continue getting the next page as long as that link exists.
-
Just be aware that to do the pagination requires that you read ALL the data from all the files on every page load - that would be the only way to properly paginate. You *could* implement some "workarounds" to alleviate that based on your specific needs. For example, if you will be updating the news feeds on manual basis, you could could create a script that would read all the data and programmatically create the "pages" as individual files. Then there would be no performance issues when the users are accessing the pages. It's really going to be determined on how much data you need to work with and the various functions you want to support.
-
The error reporting wouldn't make it work. Most likely you happened to change something else in the process and fixed the actual error at the same time.
-
Did you implement the line of code I provided above so you could see the actual database error? The PHP errors are not going to help you. You need to see the actual query that was submitted to the DB server and the DB error. It could be something as simple as your $trimmed value is not what you expect. Regarding your last query - I would expect it to fail. Since you changed the field names the ORDER BY clause is referencing a field that does not exist. You would need to alias the field for each sub-query to be the same. But, that wasn't even needed - you could have left the field names alone. That was obvious according to the example from the manual I posted above. Anyway, I just created three tables (freelistings, basicpackage, and premiumuser) each with a field called postcode. I then ran the query I provided to you previously and it worked perfectly with the results I was expecting and in the proper order. Like I said, you need to add error handling for the query. If the query fails echo the "constructed" query and the DB error.
-
Help incorporating email validator with field check
Psycho replied to sphinx's topic in PHP Coding Help
Your test is trying to call the function is_valid_email(), but you used the function is_email(). -
Neither do i, because as I said it will cause a circular reference and cannot be done. Take the following scenario: Alan is friends with Bob, Bob is friends with Chris, and Chris is friends with Alan. To Alan's score you have to calculate the points based upon his friendship with Bob (which will be based upon Bob's score). But, to determine Bob's score you first have to calculate the points he will get based upon his friendship with Chris - which required you to calculate Chris's score. To determine Chris's score you have to determine how many points he gets for his friendship to Alan. And THAT requires you to calculate Alan's score which is what you started out trying to determine. The model you are proposing is not possible. You can have a variable scoring model, but you need to come up with something that can be done. As I said, you can do this IF you only calculate the "friendship" score only at the time the friendship is made. But, if the friendship score is to be variable in real-time it just won't work.
-
Error reporting will only show you PHP errors - not Database errors. Echo out the generated query and the mysql error message. $result = mysql_query($query) or die("<b>Query:</b> $query<br><b>Error</b>: " . mysql_error()); OK, looking at the query you built though - it doesn't look right. Here is the 2nd example from the MySQL Manual for UNION (SELECT a FROM t1 WHERE a=10 AND B=1) UNION (SELECT a FROM t2 WHERE a=11 AND B=2) ORDER BY a LIMIT 10; You see how they enclosed the sub-queries in parens. I don't think that is necessarily required in all situations. But, you have the added problem that you have a field with the exact same name in all the tables. So, it would be best to make sure you "separate" those sub-queries as much as you can. Lastly, you don't want the sub-queries ordered individually - you want them ordered together, correct? So, I would follow the example above and use $query = "(SELECT postcode FROM freelistings WHERE postcode like '%$trimmed%') UNION (SELECT postcode FROM basicpackage WHERE postcode like '%$trimmed%') UNION (SELECT postcode FROM premiumuser WHERE postcode like '%$trimmed%') ORDER BY postcode";