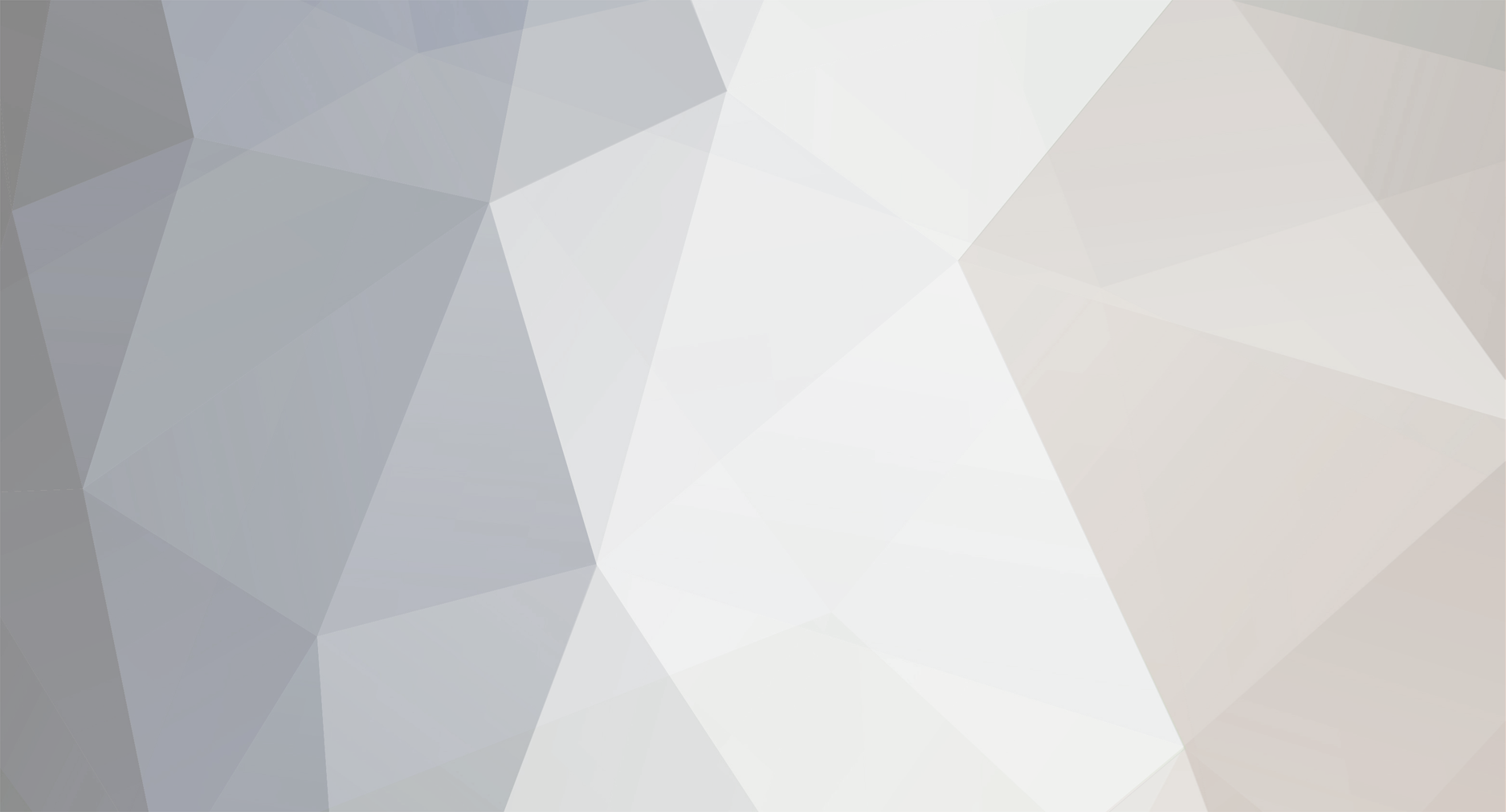
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Duh. Yeah. It has nothing to do with being a "PHP Guru", it's called paying attention to detail. Did you not read the VERY FIRST sentence in my reply? You run that line in the controller file. For just about any application you need a single file to set configuration data and/or make database connections etc. For many of my sites my "pages" do nothing more than set a few variables and then load the index.php file at the root of the site. That page uses those variables to then determine what logic pages to load (which actually reside outside the public folder so none of my files with any core logic are publicly accessible). By placing that line of code in the root of the web accessible file it sets the root directory one level up. So, I can then define the paths to the logic files outside the web root. By using the method above I can easily change the folder structure of the site and then simply change one line and all the pages that reference those paths will work without having to search and replace throughout all the files.
-
@renfley, You were trying to use a foreach loop on the reference ID for a database result set. You need to create a loop (typically a while() loop) to extract each record one at a time and then display/process the data however you want. QuickOldCar gave you a solution in Reply #2. I *think* your reply, in Reply #5, was to prevent having to have multiple echo statemetns - one for each record rather than having empty values displayed. Unless, of course, you have empty values in your database. @QuickOldCa; Why define a variable $title just to use it one time? $title = $row['title']; echo "<div>".$title."</div>"; This would be easier $title = $row['title']; echo "<div>{$row['title']}</div>"; Anyway, here is what I would do (based off of QuickOldCar's script) //Connect to Database $con = mysql_connect("localhost","root",""); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("database", $con); //Create and run query $query = "SELECT title FROM table1"; $result = mysql_query($query) or die("Query:<br>{$query}<br>Error:<br>" . mysql_error()); //Output results while($row = mysql_fetch_assoc($result)) { echo "<div>{$row['title']}</div>\n"; }
-
[Moved to JavaScript forum] You will need to accomplish this using JavaScript. How many strings do you have? If you only have a relatively small number then just create the text strings as JavaScript values (in an array). If it is an excessively large number then you would use AJAX (JavaScript + PHP, or some other server-side technology) to get them from the server.
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=342245.0
-
I would assume you are dynamically creating the links from the results of a database query. Then you should be passing the ID in the URL - not the name.
-
Ah. I missed that. Try this: $query = "SELECT SUM(test_results.hts) FROM test_teams, test_results WHERE test_teams.team = '$team' AND test_teams.teamid = test_results.hometeam"; $result = mysql_query($hometotal) or die(mysql_error()); $hometotal = mysql_result($result, 0); $query = "SELECT SUM(test_results.ats) FROM test_teams, test_results WHERE test_teams.team = '$team' AND test_teams.teamid = test_results.awayteam"; $result = mysql_query($awaytotal) or die(mysql_error()); $awaytotal = mysql_result($result, 0); $total = $hometotal + $awaytotal; Although, I'm curious why you are not using the team ID to begin with.
-
If you don't need the two separate totals and just the combined total, you should just need one query SELECT SUM(test_results.hts) FROM test_teams, test_results WHERE test_teams.team = '$team' AND (test_teams.teamid = test_results.hometeam OR test_teams.teamid = test_results.awaytea)
-
Foreach-ing through mysql query returning null :s
Psycho replied to Zephni's topic in PHP Coding Help
You are absolutely right! I already stated that running loops is bad practice. Learn to do JOINs and use them properly! That is the whole point of having a relational database, so you can "relate" data across tables. You only needed to change the SELECT clause to pull the item name instead of the id. //Run ONE query to get the sum of prices for each item by NAME $query = "SELECT item_types.name, SUM(costs.price) as sum FROM costs JOIN item_types ON costs.item_type = item_types.id GROUP BY costs.item_type"; $result = mysql_query($query) or die(mysql_error()); //Create array of each item name as key and sum as value $item_totals = array(); while($row = mysql_fetch_assoc($result)) { $item_totals[$row['name']] = $row['sum']; } //Create variable with the sum of all totals $item_summery = array_sum($item_totals); -
Foreach-ing through mysql query returning null :s
Psycho replied to Zephni's topic in PHP Coding Help
This will get you the sum by item type in a single query and get the sum of all those records using array_sum() $query = "SELECT costs.item_type, SUM(costs.price) as sum FROM costs JOIN item_types ON costs.item_type = item_type.name GROUP BY costs.item_type"; $result = mysql_query($query) or die(mysql_error()); //Dump results into array so individual sums can be used while($row = mysql_fetch_assoc($result)) { $item_totals[$row['item_type']] = $row['sum']; } $item_summery = array_sum($item_totals); However, if you only need the total and not the total by each type, then it is even easier $query = "SELECT SUM(costs.price) as sum FROM costs JOIN item_types ON costs.item_type = item_type.name"; $result = mysql_query($query) or die(mysql_error()); $item_summery = mysql_result($result, 0); -
Foreach-ing through mysql query returning null :s
Psycho replied to Zephni's topic in PHP Coding Help
Ideas? Yeah, you are doing it wrong. Don't know why $item_summery is null. Are you treating it as an array (which it would be) or as a number/string value? Second, you aren't even checking that the queries are succeeding. If they fail, the while() loops never run. Lastly, if all you want is a total then you can get that with ONE single query instead of all those loops that will put unneeded stress on the server. EDIT: You shouldn't be using the item "name" to associate the records in "costs" to the item types. Use a unique id as the foreign key reference. And, I'm not sure why you even need the first query. Are there records in the "costs" table that don't have an associated item type? And, I see now that your second query is malformed and would be failing. Which is the specific reason for your problem. -
Is it possible to hold MySQL commit over dialogs?
Psycho replied to jayteepics's topic in PHP Coding Help
EDIT:WebStyles solution is similar, but I would use the current table and just add a status column: Create the order with a temporary status (e.g. pending payment verification), then proceed to the payment process. If payment succeeds update the order status to paid. If not, delete the order. -
Well, if it was content run through htmlentities() then it wouldn't be rendered as HTML, but maybe that is the case. I agree that (or something like it) is the problem. @OP: It would be helpful to show a sample of the content before and after running through strip_tags().
-
You don't need a database to process data, a database is primarily for storing the data. Even if you wanted to use a database, you would have to process it first anyway. But, if you have to compare values from one file to other files then a DB might make sense since it will be more efficient. To make a determination as to whether a DB would be more efficient or not will be dependent on how many files you need to compare and what "comparisons" you need to make.
-
First off, let me add some suggestions on coding style: You create an array using explode(); and then loop through the results using for($i=0;$i < sizeof($entries);$i++){ You should use a foreach() loop for arrays. In the loop above the function sizeof() is being executed on each iteration of the loop - very inefficient. If you do need to perform a loop on a static value - define that value beforehand. The only reason to have a loop like that is if the array $entries would change length during the loop. Second, instead of using file_get_contents() and exploding the contents using "\n" use file() instead which reads the file as an array to start with. Anyway, give this a try: //Read file into an array of lines $file = file("33.txt"); $tableHTML = =''; foreach($file as $line) { $tableHTML .= "<tr>\n"; $numbers = explode(',', $line); foreach($numbers as $num) { $tableHTML .= "<td align=\"center\" background=\"test/33_{$num}.gif\" width=\"65\" height=\"65\"> </td>\n"; } $tableHTML .= "</tr>\n"; } echo "Entries <br /><hr />"; echo "<table>\n"; echo $table; echo "</table>\n";
-
If you have a "core" / "controller" file that is run for every page then there is a trick you can use. $ROOT_PATH = dirname(dirname(__FILE__)) . DIRECTORY_SEPARATOR; This will set $ROOT_PATH to the location of the file that runs that line of code. Then, you can create variables to different folders used by the application that you can use throughout the application: $_DS_ = DIRECTORY_SEPARATOR; $_PATHS['modules'] = $_PATHS['root'] . 'modules' . $_DS_; $_PATHS['classes'] = $_PATHS['root'] . 'classes' . $_DS_; $_PATHS['templates'] = $_PATHS['root'] . 'templates' . $_DS_; Although I agree with Adam that the location should be configurable.
-
Not if you want to know if the value is an integer. Yes, I was incorrect in suggesting to use is_int() against a string that is supposed to represent an int value. But, that doesn't mean is_numeric() is the correct function to check for a string that should be an integer. is_numeric() will return true for "1.2345" or "-123", which are not "valid" if you are looking for a positive integer. Typically, the validation is to ensure the value is a positive (non-zero) integer. So, one solution is to use int_val() to get the integer value or use "(int) $value" to convert the value to an integer. Then test that the value is greater than 0. Or, there is also ctype_digit() which wil validate that every character in the string is a digit (does not allow neg sign or decimal).
-
This has already been answered in your previous post. Either: 1. Build the logic into MySQL to calculate the distance and create a dynamic value to use the MySQL sorting functions on or 2. Get all the results from MySQL then process the data into a multi-dimensional array (while also creating the distance value for each record). Then you can sort the results using one of the PHP sorting functions. However, as I stated in your earlier post, this will not allow you to use the queries for pagination. Since you need to use LIMIT to get the correct results for the page AFTER they are sorted. So, if you go this route, you will need to extract the correct "page" of results from the array of all records.
-
I'm not totally understanding that second sentence. For a SELECT input I always validate against the predefined values. In many cases a select list is generated from a list defined in the database. So, I would test the received value against the database (but first 'cleaning' the value based upon it's type: string, int, date, etc.). Although, if the value is 'hard-coded' then I usually have it stored in an array. Then I just check the submitted value against the array.
-
Been to every forum on the internet, no one has a clue. Please help!
Psycho replied to ValkR's topic in PHP Coding Help
Awesome? Not really. This is the exact type of scenario where a database is needed. Replying upon file copy/move procedures to run your site is going to lead to problems. It's understandable if you are perhaps intimidated by databases because you are not familiar or don't have a lot of experience. But, that IS the solution you need. -
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=342157.0
-
No, that is running strip_slashes() regardless of whether magic quotes are enabled or not.You should only run it is magic quotes are enabled. Otherwise you would remove slashes that the user entered. The manual has a function that you can run on page load that will run strip_slashes() on all user input if magic quotes is enabled. I use something similar and put it in one of the config files that I load for every page. http://php.net/manual/en/security.magicquotes.disabling.php (see example #2)
-
Check the manual for strpos(). Then scroll down to the section for "See Also". http://php.net/manual/en/function.strpos.php
-
You are testing if the result of strpos() is the literal TRUE (by using three equal signs). strpos() returns the numerical index of where the string is found 0 - n or FALSE if the string is not found. Since 0 can be interpreted as FALSE you cannot just use a "normal" comparison (two equal signs). So you should check that the value is not a literal FALSE> if ($texassearch !== false) {
-
Well, just to clarify my position. I wouldn't say that client-side validation is "unreliable" to prevent malicious input - I would say it is completely worthless and should never be relied upon. Clint-side validation is a good way to give the user immediate feedback and a more interactive experience. It should never be used as a replacement for server-side validation to prevent malicious input.
-
Where did client-side validation come into this discussion? But since you bring it up. There's nothing "wrong" with client-side validation. In fact, I like implementing client-side validation - it gives the user immediate feedback without having to submit the form. It's just that you should never "rely" upon client-side validation. You always have to validate server-side. You say you are not "looking for anything fancy like float values", but that is the validation you are doing now. By your explanation you want an integer value. So, use the correct validation: is_int().