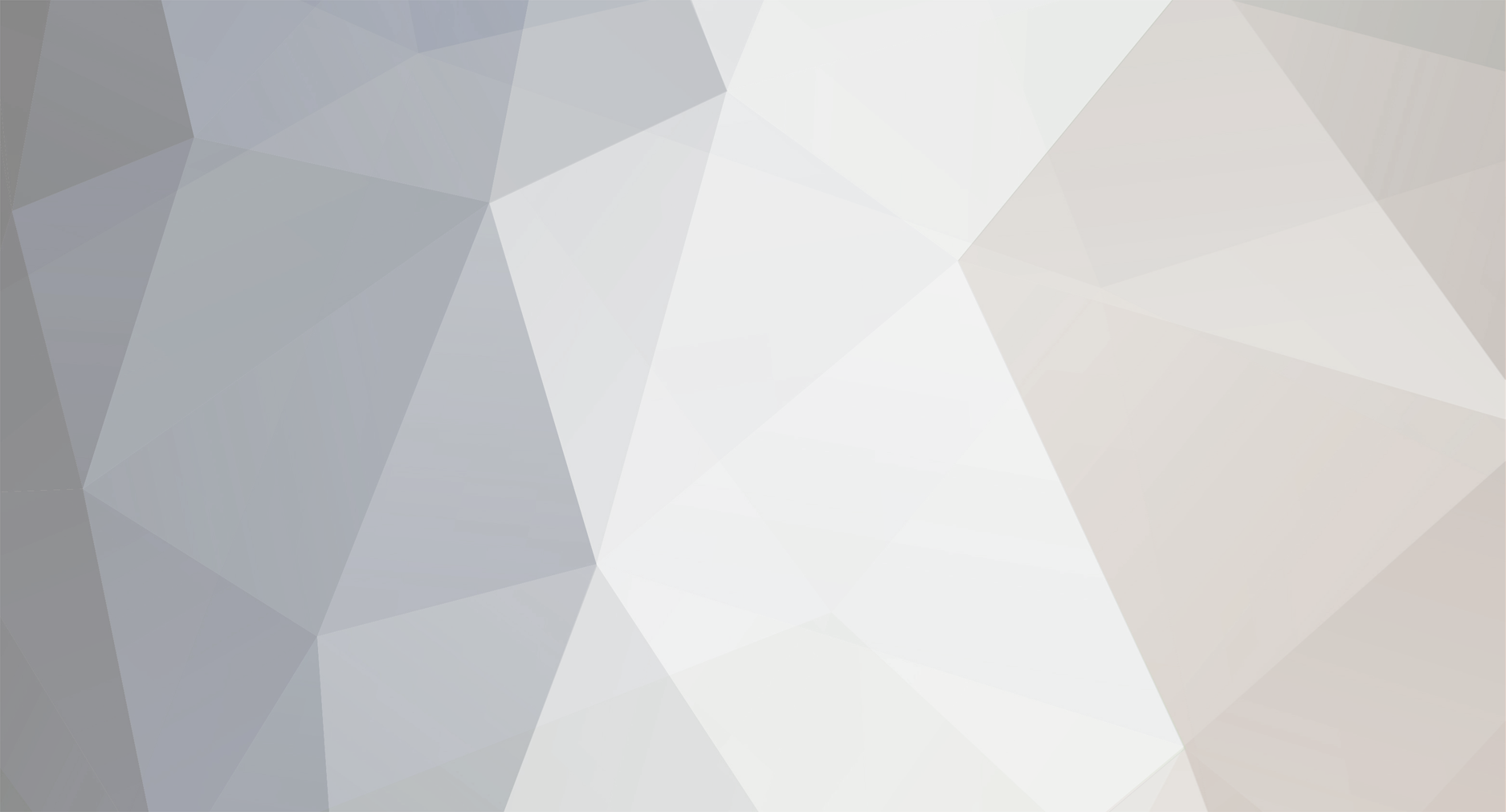
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
question about user input security on a search box
Psycho replied to php beginner's topic in PHP Coding Help
You are making it way harder than it needs to be. Allow the user to input anything they want and just use mysql_real_escape_string() on the input. If the user enters "/php ? ` DROP TABLE" it will be safe to run and they just won't get any matching results EDIT: The only thing you might think about doing is splitting the words into separate pieces so if the user types in "soccer tournament" you could preset results that match "soccer" or "tournament" -
Passing the value in clear text on each page transition is less risky than storing the value in a session value on the server? It would then only pass in clear-text one time (when sent from the client to the server) as opposed to many times back and forth. "Don't use sessions as a way to make variables 'global' between pages.". That is the whole point of session values. You can set them and have them persist for the entire session. As to the "issues" you are referring to where you want to set the similar values for different pages - you just set different session values. It's not complicated at all. For instance, I have built applications where there are several different screens where data can be paginated and I give the user the option to change the number of records to be displayed per page. Some settings should apply to "similar" records while others should not. So, I simply create a different session array for each: $_SESSION['client']['records_per_page'] would be used for any pages displaying clients, $_SESSION['staff']['records_per_page'] would be used for any pages displaying staff, etc.
-
You still haven't followed the advice given in the previous post to display the contents of the array(s). I can suggest some changes, but since I can't see the contents of the arrays I would only be guessing. Here is a rewrite of that code in a more logical flow. If it doesn't work, uncomment the debug line and post the debug code and state what is not right about the output. I didn't test this since I don't have your data, so there may be syntax errors. <?php while ($row = $result->fetch()) { if(!isset($items[$row['itemid']])) { $items[$row['itemid']] = $row; } $items[$row['itemid']]['sizes'][] = $row['size']; } ##DEBUG LINE //echo "<pre>".print_r($items, true)."</pre>\n"; foreach($items AS $subitem) { list($prodid, $item ,$size, $description, $price) = $subitem; //Create field for item name based upon record count if(count($subitem['sizes']) > 1) { $item_name_field = "<li><select name=\"my-item-name\" id=\"foo\">\n"; foreach($subitem['sizes'] as $size) { $item_name_field .= "<option value=\"{$size}\">{$size}</option>\n"; } $item_name_field .= "</select></li>\n"; } else { $item_name_field = "<input type=\"hidden\" name=\"my-item-name\" value=\"{$subitem['sizes'][0]}\" />"; } //Creat the form if ($count % NUMCOLS == 0) { echo "<tr>"; } //new row echo "<td>\n"; echo "<form method=\"post\" action=\"\" class=\"jcart\">\n"; echo " <fieldset>\n"; echo " <input type=\"hidden\" name=\"jcartToken\" value=\"{$_SESSION['jcartToken']}\" />\n"; echo " <input type=\"hidden\" name=\"my-item-id\" value=\"{$prodid}\" />\n"; echo " <input type=\"hidden\" name=\"my-item-price\" value=\"{$price}\" />\n"; echo " <input type=\"hidden\" name=\"my-item-url\" value=\"http://yahoo.com\" />\n"; echo " {$item_name_field}\n"; echo " <ul>\n"; echo " <li>Price: $<span class=\"price\">{$subitem['price']}</span></li>\n"; echo " <li><label>Qty: <input type=\"text\" name=\"my-item-qty\" value=\"1\" size=\"3\" /></label></li>\n"; echo " </ul>\n"; echo " <input type=\"submit\" name=\"my-add-button\" value=\"add to cart\" class=\"button\" />\n"; echo " </fieldset>\n"; echo "</form>\n"; echo "</td>\n";; $count++; if ($count % NUMCOLS == 0) { echo "</tr>\n"; } # end row //$counter++; //Doesn't appear this is used } ?>
-
Hmm, I don't know how you can say you are getting the correct number of forms (which is part of the data) but you now say you can't display the data. Anyway, to be blunt, your code is very disorganized. For example, at one point you define some variables using the first record of a sub array. list($prodid,$item,$size,$description,$price) = $subitem[0]; But, you never use them and instead "try" to reference the value like this: <input type="hidden" name="my-item-id" value="'.$subitem['prodid'].'" /> But, isn't $subitem a multi-dimensional array (with the first index numerically based)? Therefore, $subitem['prodid'] doesn't even exist, right? Also, your logic to create the different rows will create invalid HTML code.
-
Validate the data, then resend it to the final URL via POST. Here is an article/tutorial/. I have not read it so I can't guarantee anything http://wezfurlong.org/blog/2006/nov/http-post-from-php-without-curl/ EDIT: You might also want to check out this page in the PHP manual: http://www.php.net/manual/en/context.http.php
-
Um, yeah . . . a whole pagination script. The only thing different between what you want and what typically constitutes a pagination script is the number of records per page. Whether you want 100 or 1 records on a page, it is still pagination. Your "trick" is invalid it simply shows the record which has an ID that is 1 greater than the last. There are several problems with that: 1) Such a record may not exist, 2) Your original requirement was to show records that match a specific criteria (i.e the URL value). How do you know the record with the next highest id matches that criteria? Yes, you could do a simpler solution, but why? There is already logic for pagination that is easily implemented. If you wanted to do a different solution, then you need to build it from scratch. Off the top of my head you could always retrieve 2 records - the current record and the next record. You the first result to display on the page and the second to create the next link. But, what if you need a back link. Well, you could pass the current id into the next page to use for a previous, but then you need to account for the first page that won't have a previous link.
-
I agree that session variables would be better. But, I think the problem is here on page 4: $weekNo[]=$_GET['weekNo']; You are assigning the passed value into an array. But, then you try to reference it as a string echo"$weekNo"; Unless there is some reason to be using an array, you should be using $weekNo=$_GET['weekNo'];
-
How is this different from your last post? Do not double post. Several questions were asked of you in the original post and you did not answer any of them, except to post more of your code. Did you validate that the array has values? Do as wildteen88 suggested.
-
Dynamic drop down field works but when chosen, nothing happens.
Psycho replied to snoreboy01's topic in PHP Coding Help
OK, I've gone through your code and there were a lot of problems. I rewrote the logic and added some rudimentary error handling. I wasn't able to test this since I don't have your database, so there may be some syntax errors. NOTE: I turned on full error reporting on the first line. If any errors such as db results missing were to occur, you would get a lot of PHP warning messages because the variables for the table data would not be defined and the code would try to echo them. Ideally, you should be conditionally creating that table only if results were obtained to fill it. <?php //Turn all full error reporting error_reporting(E_ALL); session_start(); require "db_connect.php"; //Define error message var for error handling $errorMsg = false; //Defined the selected fighter is sent in POST data $selectedFighter = isset($_POST['fighter']) ? trim($_POST['fighter']) : false; //Query to get list of fighters for select list $query = "SELECT fighterName FROM fighters ORDER BY fighterName ASC"; $result = mysql_query($query); if(!$result) { $errorMsg = "Unable to get list of fighters."; } elseif(mysql_num_rows($result)<1) { $errorMsg = "There are no fighters to select from."; } else { //Create select list options $fighterOptions = ''; while($row = mysql_fetch_assoc($result)) { $selected = ({$row['fighterName'] == $selectedFighter) ? ' selected="selected"' : ''; $fighterOptions .= "<option value=\"{$row['fighterName']}\"{$selected}>{{$row['fighterName']}}</option>\n" } } //Query to get details of selected fighter if set if($selectedFighter!==false && $errorMsg!==false) { $selectedFighter = mysql_real_escape_string($selectedFighter); $query = "SELECT * FROM fighters WHERE fighterName='{$selectedFighter}'" $result = mysql_query($query); if(!$result) { $errorMsg = "Error retrieving information for selected fighter."; } elseif(mysql_num_rows($result)<1) { $errorMsg = "The selected fighter does not exist."; } else { //Get data $fighter = mysql_fetch_assoc($result); $NickName = htmlentities($fighter["fighterName"]); $RealName = htmlentities($fighter["fullName"]); $gender = htmlentities($fighter["gender"]); $Tech = htmlentities($fighter["tech"]); $Type = htmlentities($fighter["charType"]); $Height = htmlentities($fighter["height"]); $Weight = htmlentities($fighter["weight"]); $Age = htmlentities($fighter["age"]); $Rank = htmlentities($fighter["rank"]); $Concept = htmlentities($fighter["concept"]); $Signature = htmlentities($fighter["signature"]); $STR = htmlentities($fighter["Fstr"]); $DEX = htmlentities($fighter["Fdex"]); $STA = htmlentities($fighter["Fsta"]); $CHA = htmlentities($fighter["Fcha"]); $MAN = htmlentities($fighter["Fman"]); $APP = htmlentities($fighter["Fapp"]); $PER = htmlentities($fighter["Fper"]); $INT = htmlentities($fighter["Fint"]); $WIT = htmlentities($fighter["Fwit"]); $imageLocation = "/Fighters/chars/"; $imageName = "{$NickName}.jpg"; $imageSrc = "{$imageLocation}{$imageName}"; } } //Format the error message if($errorMsg!==false) { $errorMsg = "<span style=\"color:#ff0000;\">{$errorMsg}<br><br>\n"; } else { $errorMsg = ''; } ?> <html> <head> <title>Fighter Details</title> </head> <body> <br> <a href="/Fighters/index.php">Home!</a> <a href="createFighter.php">Create Fighter!</a> <br><br> <?php echo $errorMsg; ?> <form method="post" action="viewFighter.php"> Choose a Fighter! <select name="fighter"> <?php echo $fighterOptions; ?> </select> <input type="submit" value="Choose!"> </form> <br> <h4><?php echo $NickName; ?></h4> <br><br> <table border="1"> <tr> <td>Nick Name:</td> <td width="20%"><?php echo $NickName; ?></td> <td>Technique:</td> <td><?php echo $Tech; ?></td> <td rowspan="7" colspan="2" width="25%"><img src="<?php echo $imageSrc; ?>" height="200"/></td> </tr> <tr> <td>Real Name:</td> <td><?php echo $RealName?></td> <td width="20%">Gender:</td> <td><?php echo $gender ?></td> </tr> <tr> <td>Height:</td> <td><?php echo $Height; ?> ft</td> <td>Weight:</td> <td><?php echo $Weight; ?> lbs</td> </tr> <tr> <td>Type:</td> <td><?php echo $Type; ?></td> <td>Rank:</td> <td><?php echo $Ran;k ?></td> </tr> <tr> <td>Age:</td> <td colspan="3"><?php echo $Age; ?></td> </tr> <tr> <td>Concept:</td> <td colspan="3"><?php echo $Concept; ?></td> </tr> <tr> <td>Signature:</td> <td colspan="3"><?php echo $Signature; ?></td> </tr> <tr> <td>Strength:</td> <td><?php echo $STR; ?></td> <td>Charisma:</td> <td><?php echo $CHA; ?></td> <td>Perceptiom:</td> <td><?php echo $PER; ?></td> </tr> <tr> <td>Dexterity:</td> <td><?php echo $DEX; ?></td> <td>Manipulation:</td> <td><?php echo $MAN; ?></td> <td>Intelligence:</td> <td><?php echo $INT; ?></td> </tr> <tr> <td>Stamina:</td> <td><?php echo $STA; ?></td> <td>Appearance:</td> <td><?php echo $APP; ?></td> <td>Wits:</td> <td><?php echo $WIT; ?></td> </tr> <tr> <td>Alertness:</td> <td width="20%"><?php echo $Alert; ?></td> <td>Blind Fighting:</td> <td><?php echo $Blind; ?></td> <td>Academics:</td> <td><?php echo $Academics; ?></td> </tr> </table> </body> </html> -
Dynamic drop down field works but when chosen, nothing happens.
Psycho replied to snoreboy01's topic in PHP Coding Help
You say But, that is not totally clear. You currently have a submit button so that tells me youwould want to submit the form and refresh the page. When you state "stays on the same page" that could imply using AJAX to update the page without needing to submit/refresh the page. But you can, and should, do both. So, first get the page working by submitting the page normally, then once you get that working youcan work on adding AJAX handling if you want. So, looking at your current code, some things to check: The action attribute in the FORM tag is "viewFighter.php" is that the same page of the script? If so, you can jsut remove the value in the action attribute so if you ever change the name of the file it will still work. But, if it is a different page, then it obviously won't work. OK, I think this is your problem. You have several lines where you are defining variables, such as $NickName = $a_row["fighterName"]; $RealName = $a_row["fullName"]; $gender = $a_row["gender"]; ... However, I don't see where $a_row is ever defined! So, nothing would be displayed in the page for those values. You would have found this if you had turned on full error reporting. EDIT: I also see that your SUBMIT button is INSIDE your selection list immediately following the options, but before the closing "</select>" tag -
You have a space between "*" and the ".tablenames". Why did you deviate from what I supplied?
-
OK, I FAIL. I didn't catch that part about the fiscal year end in July (and I work for an accounting software company, LOL).
-
Quit showing off, LOL. At least give the guy some comments I'm with Permiso, I'm surprised we have someone actually admitting they have a homework problem and they actually attempted it before posting.
-
You have a field where you put the string value of "true"? If the only value is true or false you should be using a tinyint type field and storing a 0 or 1. SELECT COUNT(*) FROM `news` WHERE `show` = 'true' AND YEAR(`date`) = YEAR(NOW())
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=339292.0
-
Ok, but where are you getting those URLs and how are you going to associate them with the correct tag? Here's some code to output the tags as links, but it just uses a hard-coded URL because I have no idea where you are getting them from. //Explode the DB result into an array using the commas $keywordsArray = explode(',', $blog_query_results['keywords']); //Iterate through each keyword foreach($keywordsArray as $keywordString) { //Parse the tag value. Don't know the purposes of stripslashes and strip_tags, but I left them in // - I would think htmlenteties woud be a better option $label = stripslashes(strip_tags(trim($keywordString))); //Get URL for the tag. You didn't provide any details, so this is just hard-coded $url = "http://www.yahoo.com"; //Output the final link echo "<a href=\"{$url}\">{$label}</a><br>\n"; }
-
What code do you have so far? Can you run a query to get the value from the database. Show an example of the output you want to create. Give us something to go on.
-
Hmm, I question if you have the right database design. Instead of having separate tables for current school,, first school (and I'm assuming other previous schools) it would mmke more sense to have a single table with an identifier to determine first/current school. No matter, I *think* this query will get you what you need. If not, please state what - EXACTLY - is wrong with the results. SELECT *.view_teachers_info, *.view_teachers_first_school FROM view_teachers_current_school JOIN view_teachers_info USING nic JOIN view_teachers_first_school USING nic WHERE view_teachers_current_school.nic = '$this->schoolId'
-
A couple other points/questions 1. No need to dump the DB results into an array if you are only then going to loop through the array one time. Just process the data as you extract the results from the database and save time and memory 2. Where is the constant "NUMCOLS" defined and have you validated that it does have a value? 3. The logic for starting/closing a table row will cause invalid HTML 4. I also see a problem with this logic while ($row = $result->fetchrow()) { $superitem[$row['itemid']][] = $row; } foreach($superitem AS $subitem) { list($prodid, $item, $description, $price) = $subitem[0]; Based upon what I see the foreach loop will iterate over each record from the DB results and $subitem will be an array of the fields in the record. You are then trying to use list() on "$subitem[0]". but, that will only be the first value of a record - not an array. Please show the query you are using and the fields you are using from the database if you use "*" in your SELECT statement. EDIT: Scratch that last statement, I now see that you are creating a multi-dimensional array.
-
If you want help you need to take a moment and try to explain what you are trying to achieve in a meaningful manner. Give an explanation of the data stored in each table and what you are trying to get out of the database. I can make some assumptions based on the table names, but I'm not going to invest any time in trying to provide a query when its more likely than not that I have made the wrong assumption. Looking at the first two queries you provided, I can see the relationship between the two tables and the "view_teachers_current_school" table. But, without knowing how those two other tables relate to each other I can't really know what you need. Here are my assumptions/question, please either confirm or correct and provide additional information if needed: - I assume that "nic" is a unique ID for each teacher. - Is there only ONE record per teacher (or nic) in the "view_teachers_info" table or can there be multiple? - Is there only ONE record per teacher (or nic) in the "view_teachers_first_school" table or can there be multiple? - Is it possible for a record to exist in one of those tables associated with a "nic" but no record(s) in the other table for the same "nic"? If so, is there one table that will have at least one associated record for each "nic" or is there one table that should be considered the "primary"? That means if there is a record in the primary, but no associated record in the secondary, it should be included in the results. But, if an associated record exists in the secondary, but not the primary, it will not display in the results.
-
As I stated, you use the PHP code to build up the HTML in a variable.Then you only echo the variable in the html code. Here is a very basic example below. You can then separate your logic (PHP) from the output (HTML) into different files. That gives you a log of flexibility - then you can reuse the logic and repurpose it for different displays/output for example <?php //Create and run query $query = "SELECT id, first, last, phone, email FROM users ORDER BY last, first"; $result = mysql_query($query); //Check results and create output if(!$result) { //A DB error occured $tableOutput = "<tr><td colspan=\"5\">There was a problem fetching the records</td></tr>\n" } elseif(mysql_num_rows($result)==0) { //No results returned $tableOutput = "<tr><td colspan=\"5\"No users exist</td></tr>\n" } else { //Process results into HTML $tableOutput = ''; while($row = mysql_fetch_assoc($result)) { //Created vars for full name, edit url and delete url $name = "{$row['last']}, {$row['first']}"; $editURL = "editRecord.php?id={{$row['id']}}"; $deleteURL = "deleteRecord.php?id={{$row['id']}}"; //Create the HTML forthe table record $tableOutput .= "<tr>\n"; $tableOutput .= "<td>{$name}</td>\n"; $tableOutput .= "<td>{$row['phone']}</td>\n"; $tableOutput .= "<td>{$row['email']}</td>\n"; $tableOutput .= "<td><a href=\"{$editURL}\"><Edit</a></td>\n"; $tableOutput .= "<td><a href=\"{$deleteURL}\"><Edit</a></td>\n"; $tableOutput .= "</tr>\n"; } } ?> <html> <body> <h1>User List</h1> <table> <tr> <th>Name</th> <th>Phone</th> <th>Email</th> <th>Edit</th> <th>Delete</th> </tr> <?php echo $tableHTML; ?> </table> </body> </html>
-
Making the Senders Name appear in the from area of an e-mail.
Psycho replied to iconicCreator's topic in PHP Coding Help
No we are not. Manually setting the values defeats the purpose of verifying that the values are getting properly set! All you need to do is add an echo $headers; after you define that variable. I went ahead and implemented a debug mode for the script above. But, there were other issues, so I went ahead and addressed those as well. Also, some of you validations are problematic. For example, for the name fields you only allow "a-z". But what the user has a name such as "De la Hoya" or "D'Angelo"? I didn't change those, but you need to reconsider what you are doing. But, the way you are using preg_match(0 is incorrect as well. You are testing if it is returning false (or 0). But, the pattern is only checking to see if ANY letter is in the string. So event the value "#%^&%^$-A-^&^&*#$" would pass the validation. I did fix that by changing the pattern to look for any character that is NOT a-z. I made a lot of changes, so there may be some syntax errors - I didn't test. Set the $debug variable to false to have the code work normally - i.e. send the email. Or, set it to true and it will print debug info to the page <?php $debug = true; session_start(); $_SESSION = array(); function cleanPost($value) { if (get_magic_quotes_gpc()) { $value = stripslashes($value); } return(trim($value)); } $errors = array(); if (isset($_POST["submitBtn"])) { //Parse POST values $firstName = cleanPost($_POST['firstName']); $lastName = cleanPost($_POST['lastName']); $email = cleanPost($_POST['email']); $phoneNumber = cleanPost($_POST['phoneNumber']); $comment = cleanPost($_POST['comment']); $subscribe = cleanPost($_POST['subscribe']); //VALIDATE FIRST NAME if (empty($firstName)) { $errors[] = "First name is required"; } elseif (preg_match('#[^a-zA-Z]#', $firstName)>0) { $errors[] = "First name is invalid"; } //VALIDATE LAST NAME if (empty($lastName)) { $errors[] = "Last name is required"; } elseif (preg_match('#[^a-zA-Z]#', $lastName)>0) { $errors[] = "Last name is invalid"; } //VALIDATE EMAIL if (empty($email)) { $errors[] = "Email is required"; } elseif (!preg_match("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$^", $email)) { $errors[] = "Email is invalid"; } //VALIDATE PHONE NUMBER if (empty($phoneNumber)) { $errors[] = "Phone Number is required"; } else { $digits = preg_replace('/[^\d]/', '', $phoneNumber); if(strlen($digits)!=10) { $errors[] = "Phone number is invalid"; } else { $phoneNumber = substr($digits, 0, 3).'-'.substr($digits, 3, 3).'-'.substr($digits, 6); } } //VALIDATE MESSAGE OR COMMENTS if (empty($comment)) { $errors[] = "Comment is required"; } if (count($errors)==0) { //No errors, prepare and send the email $address = "example@example.com"; $subject = "Example"; $message = "You have recieved new information via your website form. The details entered are as follows:<br><br>"; $message .= "<b>First Name:</b> ".$post['firstName']."<br>"; $message .= "<b>Last Name:</b> ".$post['lastName']."<br>"; $message .= "<b>Email:</b> ".$post['email']."<br>"; $message .= "<b>Phone Number:</b> ".$post['phoneNumber']."<br><br>"; $message .= "<b>Comments/Message:</b> ".$post['comment']."<br><br>"; $message .= "<b>Subcribed:</b> ".$post['subscribe']."<br><br>"; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers .= 'From: '.$post['firstName']." ".$post['lastName'].'<example@example.com>' . "\r\n"; if($debug != true) { //Send the email $sent = mail($address, $subject, $message, $headers); if(!$sent) { echo "There was a problem sending the email"; } else { $_SESSION['firstName'] = $firstName; header('Location: message-confirm.php'); exit; } } else { //Display the variable values echo "<b>Debug Info:<b><br>\n"; echo "Address: " . htmlentities($address) . "\n\n"; echo "Subject: " . htmlentities($subject) . "\n\n"; echo "Message: " . htmlentities($message) . "\n\n"; echo "Headers: " . htmlentities($headers) . "\n\n"; } } else { //Display the errors echo "The following errors occured:<br>\n"; echo "<ul>\n"; foreach($errors as $error) { "<li>{$error}</li>\n"; } echo "/<ul>\n"; } } ?> -
Although this is possible, you should really consider keeping your logic (PHP code) and presentation (HTML code) separate. Run all your PHP code to build variables that contain the output, then just echo those variables within the HTML code. It will make your life so much easier in the long run. Interspersing PHP code within HTML code makes the code more difficult to read/understand and is harder to maintain.
-
Making the Senders Name appear in the from area of an e-mail.
Psycho replied to iconicCreator's topic in PHP Coding Help
So, you are saying you didn't take the 30 seconds to perform the one debugging step I proposed? Did you echo "$headers" to the page to validate it has the information you expect? -
Making the Senders Name appear in the from area of an e-mail.
Psycho replied to iconicCreator's topic in PHP Coding Help
Did you validate the values you are passing into the mail() function? Specifically the $headers vaeriable? Echo it to the page to ensure it contains the values for first .name and last name that you expect. Fior some reason you decided to create an array called $post to store your $_POST values. This is confusin since it really isn't POST values. But, anyway you first define all the keys (including first and last name) as empty values and then populate it with values from $_POST. But, since we can't see the contents of $_POST we can't know if there are values under the indexes of "firstName" and "lastName". Could be that you have a mismatch between the key names and you are workign with empty strings. So, echo the different variables used in the mail() function to the page to validate their values. This is debugging 101. The one thing that *may* be a problem is you don't have a space between the last name and the "<". Don't know if that is acceptable or not.