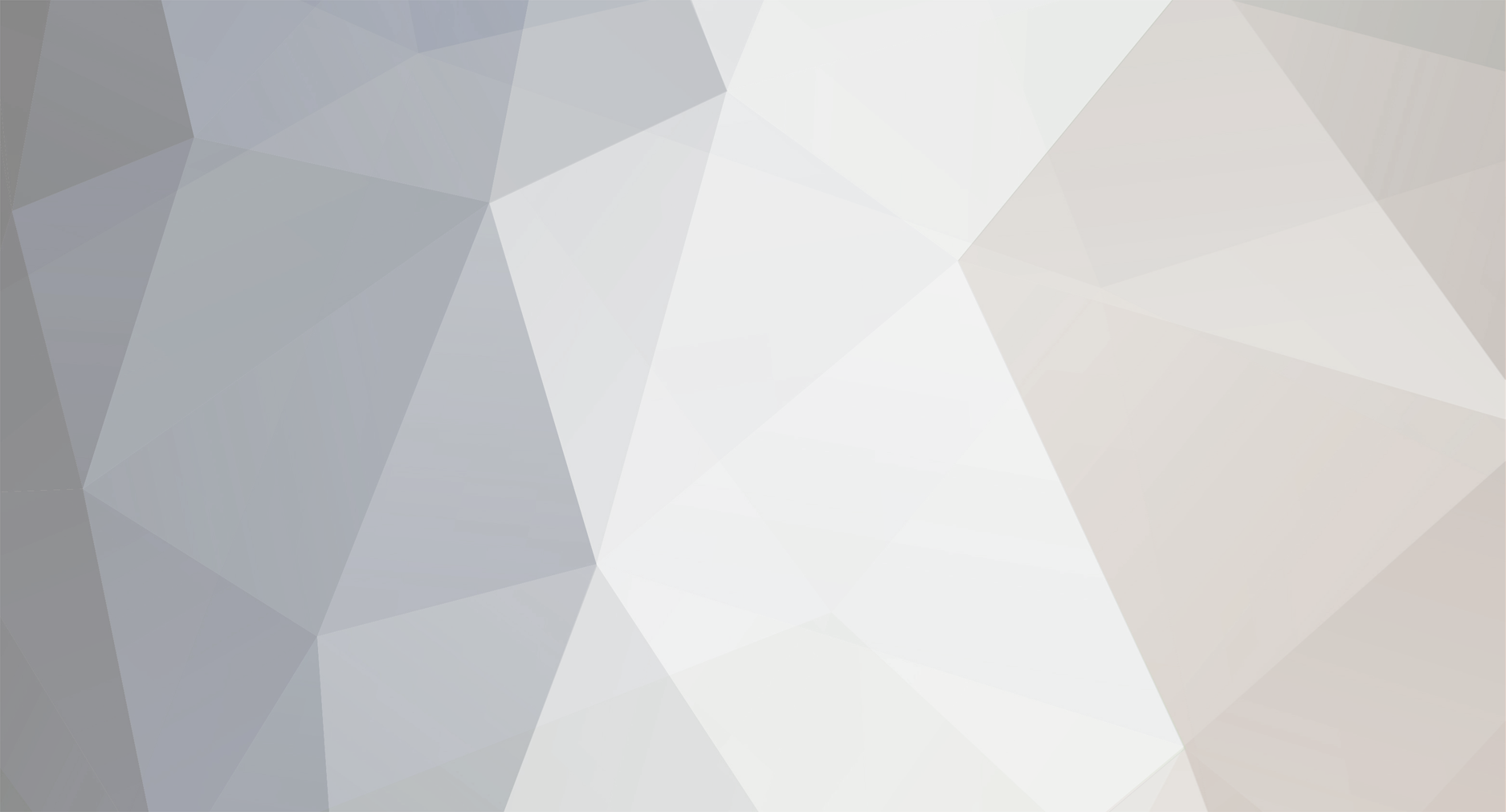
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
xyph posted a good solution, but I have an alternative that may be much easier. I assume that these records are getting created through some manner that reads the file structure, so it should be fairly simple to determine the full path for each folder from the parent. If so, then just modify your table to include the full path for each folder as follows: +----+----------------+-------------------------------+ | Id | ParentFolderId | Name | FullPath +----+----------------+-------------------------------+ | 1 | 0 | 2011 | 2011 | 2 | 1 | Flowers | 2011/Flowers | 3 | 1 | Misc | 2011/Misc | 4 | 5 | Computers | 2010/Computers | 5 | 0 | 2010 | 2010 | 6 | 5 | Fishing | 2010/Fishing | 7 | 6 | Europe | 2010/Fishing/Europe +----+----------------+--------------------------------+ Then you can get the results in the proper order using SELECT * FROM table ORDER BY FullPath And creating the options as you requested is a trivial task. $selectOptions = ''; while($row = mysql_fetch_assoc($result)) { $label = str_replace("/", " : ", $row['FullPath']); $selectOptions .= "<option value=\"{$row['Id']}\">{$label}</option>\n"; )
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=337922.0
-
I understand what you are saying, but performance issues aside, running the queries in a loop is really no more "quick and easy" to code than what I provided. Instead of running individual queries in the loop I added the values to the array in the loop - then ran the one query after the loop. So, it was one additional line of code. Well, two lines if you count the line where I instantiated the $valuesAry variable.
-
Best way to traverse a single dimensional array to find combinations?
Psycho replied to anupamsaha's topic in PHP Coding Help
One of the reasons you may be experiencing a performance issue is "how" you are checking the words against the text file. Are you reading the text file on each iteration of the loop (which would be a performance problem) or are you reading the file into a variable and checking against that on each iteration/ How big is the text file? Show some more code of what you are doing. Also, where is the array of words coming from. If the words are coming from a database you can do a simple query to create all the combinations by simply joining the table on itself. -
I have to second xyph's advice. Do NOT run queries in loops. It is terribly inefficient. It may not be a problem with only 12 records and with your expected site traffic, but it does not scale. So, if you try running many queries in loops (e.g. reporting pages) or if you have high site traffic you will run in to problems very fast. It is better to follow good programming techniques for the smaller solutions as well as the larger ones. Here is an example of how you can accomplish all the INSERTs with a single query: First off, as xyph suggested, change your field names in the form so they will be interpreted as an array by PHP. The following example is text fields, but you can do the same with multiple select lists, checkboxes, etc. Group 1: <input type="text" name="groups[]" /> Group 2: <input type="text" name="groups[]" /> Group 3: <input type="text" name="groups[]" /> ... Then your PHP processing code would look something like this: //Process user input $userid = mysql_real_escape_string(trim($_POST['userid'])); //Put group selections into arry in MySQL INSERT value format $valuesAry = array(); foreach($_POST['groups'] as $group) { $group = mysql_real_escape_string(trim($group)); if($group != '') { //Only add if not empty $valuesAry[] = "('{$userid}', '{$group}')"; } } //Create ONE INSERT query $query = "INSERT INTO table VALUES " . implode(', ', $valuesAry); The resulting query will look like xyph showed INSERT INTO table (`user_id`, `group`) VALUES ('1', 'Group1Value'), ('1', 'Group2Value'), ('1', 'Group3Value') ('1', 'Group4Value'), ('1', 'Group5Value'), ('1', 'Group6Value') ('1', 'Group7Value'), ('1', 'Group8Value'), ('1', 'Group9Value') ('1', 'Group10Value'), ('1', 'Group11Value'), ('1', 'Group12Value')
-
Well, you can lock the database at the beginning of the script and then unlock it when the page completes. I've never done it so I don't know how to do it, but a Google search would probably find some resources. But, there are better solutions in most cases (which is why I suspect fenway asked to see some examples). In may instances there are ways to handle concurrency problems by "how" you do your queries. As an easy example, let's say I have two users (User a & User B) who both have a list of records on their screens. Then both try to delete the same record. This can happen at the same time or some time apart since once User A deletes the record, it is still being displayed on User B's screen. Anyway, I would handle the potential concurrency issue by running the delete query and THEN checking the affected rows. If it is 0, then you know someone else deleted the record previously. $query = "DELETE FROM table WHERE id = $delete_id"; $result = mysql_query($query); if(!$result) { echo "There was a problem running the query"; } elseif(mysql_affected_rows()==0) { echo "The record was previously delete."; } else { echo "The record was successfully deleted."; }
-
Trying to output Top 10 list from mysql db using php
Psycho replied to gengo's topic in PHP Coding Help
You can echo it wherever you want. -
Trying to output Top 10 list from mysql db using php
Psycho replied to gengo's topic in PHP Coding Help
I was writing this before Pikachu2000 posted, but I'll go ahead and post anyway since the code above to echo the results has an error (the value $i is getting redefined on each iteration of the loop as 1, so the number would not increase) $query = "SELECT `title`, AVG(`score`) AS average FROM `table_name` GROUP BY `title` ORDER BY average DESC LIMIT 10"; $result = mysql_query($query) or die(mysql_error()); $place = 0; while($row = mysql_fetch_assoc($result)) { $place++; echo "#{$place} {$row['title']}: {$row['average']}<br>\n"; } -
That is because at the time that you define $N, the $i variable is either not defined or an empty string. When you define a string using double quotes, the string is defined using the values of the variables at the time you define it. The PHP parser has no clue that you plan to use a different value later on. Try this: $N = "<td class='num'>[i_VAR]</td>"; for ($i=1; $i<=8;$i++) { echo "<tr>" . str_replace("[i_VAR]", $i, $N) . "</tr>"; }
-
Well, nothing happens at the exact same time and a CPU does not do everything at once. It does things one at a time (per core and/or thread). If 10 users are accessing pages which run queries they will "hit" the server in a specific order and be processed in that order (although that order my be in milliseconds). However, if there are multiple queries on a page you can't be sure that all the queries on one user's page will complete before the queries on another user's page are started. This can lead to concurrency problems. Is that what your concern is? So what potential problem are you trying to solve.
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=337849.0
-
Here is a solution if you want the id component to restart each day. It still uses three fields: ticket, id and date, but the difference is that the id field is not an auto-increment field and the ticket field is the primary index for the table. //Get the last ticket entered for today $query = "SELECT `id` FROM `tableName` WHERE DATE(`date`) = DATE(NOW()) ORDER BY `id` DESC LIMIT 1"; $result = mysql_query($query) or die(mysql_error()); //Detemine the new ticket id component if(mysql_num_rows($result)==0) { $ticketID = 1; } else { $row = mysql_fetch_assoc($result); $ticketID = $row['id'] + 1; } $ticketDate = date("Y-m-d"); $newTicket = "{$ticketDate}-{$ticketID}"; //Create INSERT query $query = "INSERT INTO `tableName` (`ticket`, `date`, `id`) VALUES ('$newTicket', '$ticketDate', $ticketID)"; $result = mysql_query($query) or die(mysql_error());
-
It's not clear from your post, but do you want the incrementing number to reset each day or does it continue with the next number the following day? For example, this: 2011-07-04-00001 2011-07-04-00002 2011-07-05-00001 Or this: 2011-07-04-00001 2011-07-04-00002 2011-07-05-00003 The second option is actually easier to do. Just have an auto-increment field in the table (I assume you are using a database) AND a date field. The "ticket" number would be the concatenation of those two values.
-
I would suggest doing a search for a PHP/MySQL tutorial to just get the basics. Then find something that YOU would find useful, but don't make it too elaborate. I have always found it easier to learn something when the project is something that I want. If I stumble across some PHP functions that I have never used, I'll try to think of a problem or feature that would best be solved by that function. As an example of how to find a project: My son has to learn his multiplication tables before school starts up in the fall. I'm too lazy to sit with him and and do it, so I build a little web app to do it for me. I made it so I can determine the number of questions he will be asked, for each question it randomly chooses two numbers between 2 and 10 and then provides four possible answers. Each question is timed to see how long it takes to answer. When the test is complete all the details of the test are saved to a database. Right now, I only use the data to view just summary information for each "test" that he performs: percent correct, min/max/avg times, etc.. My next step is to make the application analyze the data so that the "random" numbers will be weighted towards the combinations that he has the most trouble with. All told, it took me about three hours to complete this over a couple of days.
-
Checking text for a string to run though function
Psycho replied to jobbe's topic in PHP Coding Help
make the function recursive where it searches for text matching the pattern, replaces it and then calls the function again until all matches have been found/replaced. I made a lot of changes to your function to remove inefficiencies. function replaceText($str) { //Search the string for a {} match if(preg_match("#{-[^-]+-}#", $str, $matchAry)==0) { //If no matches found return the string return $str; } //Parse the match found $matchStr = $matchAry[0]; $data = array(); foreach(explode('|', substr($matchStr, 2, -2)) as $pair) { list($name, $value) = explode(':', $pair); $data[$name] = $value; } //Determine replacement string for match found switch($data['type']) { case 'button': $replaceStr = "<input type=\"button\" class=\"button\" href=\"popup.php?r={$data['info']}\" value=\"{$data['text']}\" />"; break; case 'link': $http = ($data['secure']=='yes') ? 'https://' : 'http://'; $replaceStr = "<a class=\"button\" href=\"{$http}{$data['link']}\" target=\"{$data['target']}\">{$data['text']}</a>"; break; default: //Need a default to prevent infinite loops $replaceStr = ''; } //Repalce the matched {} string witht he replacement defined $str = str_replace($matchStr, $replaceStr, $str); //Call function recursively until all {} matches are replaces return replaceText($str); } $str_1 = '{-type:button|info:item|text:Read more about item-}'; $str_2 = '{-type:link|target:blank|link:www.google.com|secure:no|text:Read more about item-}'; $str = $str_1.$str_2; echo replaceText($str); Output: <input type="button" class="button" href="popup.php?r=item" value="Read more about item" /> <a class="button" href="http://www.google.com" target="blank">Read more about item</a> -
Parse error: syntax error, unexpected T_FUNCTION
Psycho replied to techrahul87's topic in PHP Coding Help
You need to follow each line of code with a semi-colon. I would suggest never putting a line of code on the same line as the opening PHP tag for multi-line blocks of code - it impairs readability. The exception would be when echo'ing a value within the HTML content. Also, that line won't work because the include file needs to be a string: <?php include('db.inc'); if(isset($_REQUEST['submit'])) EDIT: one last note. Since you are using a double-quoted string to define your query, you don't need to exit the string to include variables. Within double quoted strings variables will be interpreted as their assigned values - it makes the strings much more readable, IMHO. Also, when doing so, it is a good idea to enclose the variables within curly braces (i.e. {}) - that helps to prevent possible parsing errors. $sql="SELECT COUNT(1) AS c FROM userdata WHERE username='{$user}' AND password='{$pass}' AND status=1 AND NOW() < expireon"; -
Well, there you go. The name of your ID column is member_id, right? There is a lot wrong with your code that can cause failures and malicious attacks. I hope this is an assignment or just a tutorial you are working on and not a live site.
-
You have no error handling on your query call. I suspect there is an error. Change that line to this: mysql_query($query) or die("Query: $query<br>Error: " . mysql_error()); By the way, why are you allowing the member_id to be changed? I suspect that is the primary id for that table and it would be used as a foreign key in other tables. It shouldn't be changed.
-
Why are you using HTML comments to comment your PHP code? That means those comments will be sent to the user's browser. Bad idea. Anyway, I modified the structure of your code. I didn't like the flow. I always do error conditinos first and the success condition at the end. <?php require 'config.inc.php'; //Verify if user exists for login if(!isset($_GET['useridint']) || !isset($_GET['interest'])) { echo 'Error! Please fill all fileds!'; } else { //Parse user input $user_id = mysql_real_escape_string(urldecode($_GET['useridint'])); $interest = mysql_real_escape_string(urldecode($_GET['interest'])); //Get current count of interests for user $query = "SELECT COUNT(*) FROM user_interests WHERE user_id = '{$user_id}'"; $result = mysql_query($query) or die(mysql_error()); if(mysql_result($result, 0)>2) { //There are at least three interests echo 'Error! Only three interests are allowed!'; } else { //Perform the insert $query = "INSERT INTO user_interests (user_id, interest) VALUES('{$user_id}' , '{$interest}')"; mysql_query($query) or die(mysql_error()); echo $interest; } } ?>
-
Looping through a mySQL IN() query.. and stuck
Psycho replied to monkeytooth's topic in PHP Coding Help
You're not showing enough of your code, but it would go something like this: $query = "SELECT email FROM memb_loginInfo WHERE email IN ('{$email}') ORDER BY email ASC"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo "{$row['email']}<br>\n"; } -
Multiple Arrays and shifting data between them.
Psycho replied to monkeytooth's topic in PHP Coding Help
Your "question" is really just a generalized question since you haven't provided any code. There is an infinite number of ways to accomplish the same thing and I can't provide any relevant solutions without seeing some code. -
Multiple Arrays and shifting data between them.
Psycho replied to monkeytooth's topic in PHP Coding Help
You should reconstruct your first array so the username is the key and the email is the value. You can then use one of the array_dif() functions on the results of a query for matching emails. I think you are making this more complicated than it needs to be. It would be helpful to see some of the relevant code you have. -
Depending on what fields you want to allow for update: UPDATE newUsers2 SET firstname = $newFirtsname, lastname = $newLastname WHERE id = $idToBEUpdated
-
In the Edit link you need to pass the member id as a parameter of the anchor tag. Such as: echo "<span class=\"edit\"> <a href=\"edituser.php?id={$rows[$id]}\">EDIT</a> </span>"; Then on the edituser.php page you can access that ID value using $_GET['id']. On that page you can use that value to 1) run a query to get the current values to populate the form and 2) populate a hidden field in that form with the ID. Then when the user POSTs that page take the ID and the other form field values (escape the values) and run an update query. However, if this page does not require authentication anyone can edit any record by trying different IDs in the URL