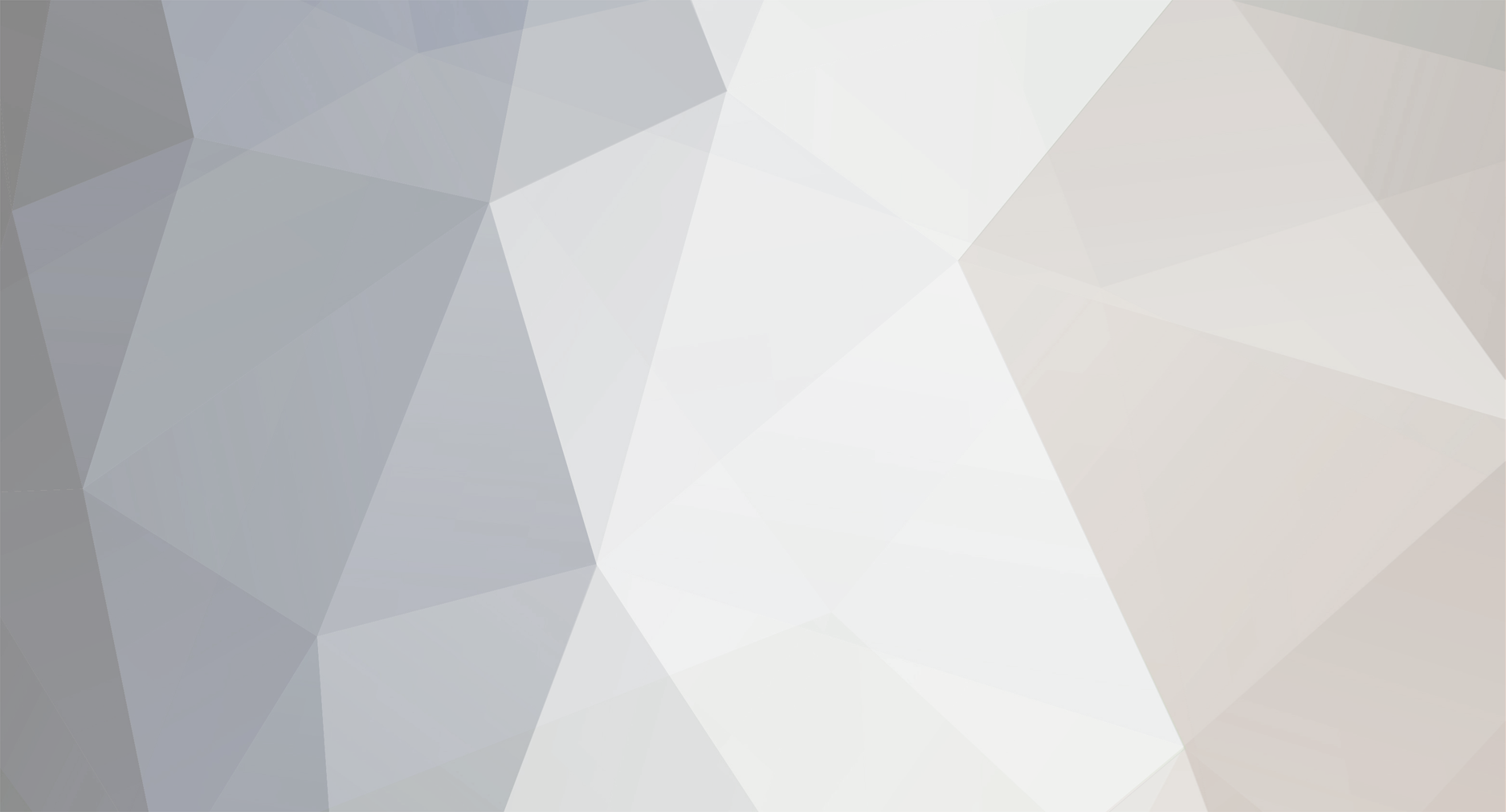
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I reversed the queries since the code I have uses the results of the jloc table first //Function to create select options and pre-select the selected value function selectOptions($optionsAry, $selectedValue) { $optionsHTML = ''; foreach($optionsAry as $value => $label) { $selected = ($value==$selectedValue) ? ' selected="selected"' : ''; $optionsHTML .= "<option value=\"{$value}\"{$selected}>{$label}</option>\n"; } return $optionsHTML; } //Create and run queries $query1 ="SELECT jloc_id, jloc_loc FROM jloc"; //SELECT from a reference table for the select input options list $query2 ="SELECT jitem_thumb, jitem_title, jitem_desc, jitem_price, jitem_loc FROM jitem JOIN jloc ON jloc.jloc_id IN(jitem.jitem_location)"; //SELECT ALL MY jitem items $result1 = mysql_query($query1); $result2 = mysql_query($query2); if(!$result1 || !$result2) { echo "There was a problem retrieving the records."; } { //Dump results of query1 into array $optionsAry = array(); while($row = mysql_fetch_assoc($result1)) { $optionsAry[$row['jloc_id']] = $row['jloc_loc']; } while($row = mysql_fetch_assoc($result2)) { print "<tr>\n"; print "<td align=center valign=top><img src='thumbs/{$row['jitem_thumb']}' alt='{$row['jitem_title']}' /></td>\n"; print "<td align=center valign=top><input size=25 type=text name=jitem_title value='{$row['jitem_title']}'></td>\n"; print "<td align=center valign=top><textarea name=jitem_desc maxlength=1000 cols=35 rows=5>{$row['jitem_desc']}</textarea></td>\n"; print "<td align=center valign=top>\$<input size=5 type=text name=jitem_price value='{$row['jitem_price']}'></td>\n"; print "<td align=center valign=top>\n"; print "<select name=\"jloc_id\">\n"; print selectOptions($optionsAry, $row['jitem_loc']); print "</select></td>\n"; } }
-
Um, no, it has the value that you set in the "value" attribute of the input tag.
-
As part of your configuration script you can have the user provide a username/password and save the values to a configuration file. You can't have your script "magically" connect to a DB without someone providing the connection info. Think about the havoc a script could cause.
-
You are providing the wrong credentials for connecting to the database. You need to get/create the right credentials.
-
What errors are you experiencing?
-
Well, I understand why you are getting the results you are getting, but I can't figure out what you are trying to do. The code makes no sense to me. Here is what I see in the function: 1. You get the first row, as an array, from the query as $s 2. You do a foreach loop on $s using $key and $ss. 3. On the first iteration of the loop $key will be a string var as 'field1' and $ss will be a string var of '5' 4. You then try to return an array of values from the variable $ss. But you are referencing array indices for $ss and it is a string - so you are getting empty values. If you want the array of values from the first row (assuming that is what you want since you say $s has the "correct" values. Then just return $s. There is no need to do a foreach loop.
-
Yes, mixed case, number and special characters DO equate to a strong password. It's not just a matter of a person trying to guess your password, you have to guard against someone trying to brute force your password, which is done using using the most probable combinations first. Even if you assume a site hashes your password, which you shouldn't, you have no way of knowing if they apply a salt and whether the salt is unique to each user. The recent intrusion into the PS Network is a prime example of why people need strong passwords. But, back to the issue at hand, did I answer your question?
-
First off, if you don't like the password requirements here you don't have to come here. There are very good reasons to require a strong password. As to your question, GMDate (aka Greenwich Mean Time, Zulu time, etc. is the "base" time upon which all other timezones are based. Daylight Savings time is a man-made adjustment to shift daylight during the summer months. not every country (or even all regions in a country) observe DST. You should be using the correct timezone for your location and use the appropriate functions to get DST as appropriate. http://php.net/manual/en/function.date-default-timezone-set.php
-
You are not returning a query. You are running UPDATE queries. Since you are using an "or die()" condition on the query calls (instead of properly handling them) you would ONLY EVER return from this function IF BOTH QUERIES successfully ran. So, you don't need to return anything. If there are errors the "or die()" statements will prevent any further execution of the script. So, let the function run, if the function completes, then you know both queries ran.
-
per the manual (emphasis added) I hope you can deduce the answer from that.' Although I see some logical problems with that function. You have the 2nd query wrapped in an IF statement. So, the mysql_query() call for that query will fail if that IF statement is false. You should never, knowingly, try to run an empty/invalid query.
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=337463.0
-
if variables are passed on the query string they cannot be referenced via $_POST. So, yes, that link above wouldn't work.
-
calculate weeks, weekends and days between any 2 dates?
Psycho replied to rich_traff's topic in PHP Coding Help
Ok, you need to go find the person that changed the requirements and hit them upside the head with a blunt object. I can't tell you how many times we have encountered significant rework because what should be simple decisions are not thought out before hand. Anyway, the code below should work. I did some testing, but not extensive. function getDateParts($startDate, $endDate) { //Normalize dates for 12noon to ensure full days calulation $startDate = strtotime(date("d F Y", $startDate) . " 12:00pm"); $endDate = strtotime(date("d F Y", $endDate) . " 12:00pm"); //Initialize array for return variable $return['totalWeeks'] = 0; $return['singeWeekendDays'] = 0; $return['singleWeekdays'] = 0; //Calculate total days in range $totalDays = round(($endDate-$startDate) / (60*60*24)) + 1; //Calculate full weeks, remaining week days, and remaining weekend days for($day=0; $day<$totalDays; $day++) { $checkDate = strtotime(date("d F Y", $startDate) . " +{$day} days"); if(date("N", $checkDate)>5) { //Add weekend day $return['singeWeekendDays']++; } elseif(date("N", $checkDate)==1 && ($totalDays-$day)>6) { //Add full week $return['totalWeeks']++; $day+=6; } else { //Add week day $return['singleWeekdays']++; } } return $return; } -
If your form is set up to send data via POST AND you are properly referencing them (i.e. using $_POST and NOT $_REQUEST) then it is impossible for users to "feeding these variables to the PHP script via the address bar?". Your processing page should be referencing the variables as $_POST and not $_GET - as they would if appended to the query string. Now, as for someone creating a copy of your form and submitting it to your page, yes that is a possibility. But, do realize that just because the data comes from someone else's form and not yours does not make it any more or less secure. But, you can prevnt it by using $_SERVER['REQUEST_URI'] or you could populate the form with a hidden variable that you check on the processing page. It could be a session variable or it could be a random value that you populate into a DB for a one-time use. It all depends on wha you are really trying to achieve and how elaborate you want to get.
-
mysql inserting multiple tables and autoincrement
Psycho replied to garry27's topic in PHP Coding Help
No, you cannot INSERT multiple records into separate tables with a single query. However, you can look into using stored procedures, which is beyond the ability to cover in a forum post. Also, you should never rely upon the number of records in a table to get the last insert ID. There is a built in function for this: mysql_insert_id(). Which will return the ID of the last record that was inserted. -
Hmm, I don't think this is going to be an easy query because of the way you created your tables. It looks like the `user_equipped_items` contains three separate columns that can contain an item_id. If instead you has a separate record in the `user_equipped_items` for each weapon type (i.e. column for user id, item_id and "type" id to determine whether primary, secondary, melee) then this would be fairly simple. o3d's solution is one possibility, but it only takes into consideration the primary weapon and not the secondary or melee. Give this a try: SELECT * FROM `items` LEFT JOIN `user_items` USING(`item_id`) LEFT JOIN `user_equipped_items` USING (`userid`) WHERE `user_items`.`userid` = '$userid' AND `user_items`.`item_id` <> `user_equipped_items`.`primary_id` AND `user_items`.`item_id` <> `user_equipped_items`.`secondary_id` AND `user_items`.`item_id` <> `user_equipped_items`.`melee_id`
-
How do i add a checkbox to delete all games that are tiicked ?.
Psycho replied to Davie33's topic in PHP Coding Help
You haven't stated what problems you are having. I'm not going to try and read through all of your code and try to guess what your problem is. You need to: state what is happening, what you expect to happen, and provide the relevant code that is producing the unwanted code. You do that, by debugging the code. Put some echo statements in at key points to determine what "branches" the logic is going through: e.g. a different echo within the IF and ELSE blocks of code. If there are key variables being interpreted, you should be echoing those at key points in the logic as well. -
How do i add a checkbox to delete all games that are tiicked ?.
Psycho replied to Davie33's topic in PHP Coding Help
Well, I added plenty of comments to explain what was happening at each step. What do you need clarification on? -
I don't think you understand the logic I provided. Using that solution it ensures that answer 1 matches question 1 and answer 2 matches question 2. You could do one query to validate answer 1 then do another query to validate answer 2. But that is inefficient. Just do one query to validate that both answers match the appropriate questions.
-
Ok, but in your code you don't do any validation that the answer matches the question - only that the answer matches one of the possible answers. Plus, it it not obvious what question1 and question2 contain. Are these the "text" values of the questions? If so, you should not be doing that. You should be passing in the POST data the IDs of the manager_users_secretAnswers records and the answers provided by the user. In fact you could do both within the inputs for the answers. Something like this: Answer 1: <input type="text" name="answer[3]" /> Answer 2: <input type="text" name="answer[8]" /> The 3 and the 8 represent the IDs for the associated questions that the user is supposed to enter (I would assume you would echo the question text next to those fields). Then the implementation of checking the input is fairly easy. Just do ONE query with specific criteria for both questionID/answer values and check that the number of records returned = 2. $userID = mysqli_real_escape_string($dbc, $_POST['userID']); $whereParts = array(); foreach($_POST['answer'] as $id => $answer) { $id = intval($id); $answer = mysqli_real_escape_string($dbc, $answer); $whereParts[] = "id='$id' AND answer = '$answer'" } $query = "SELECT * FROM manager_users_secretAnswers WHERE userID = '{$userID}' AND ( ({$whereParts[0]}) OR ({$whereParts[1]}) )"; $result = mysqli_query($dbc, $query); if(mysql_num_rows($result)!==2) { //Both answer did not match } else { //Both answers matched }
-
To be honest, I really don't care about what this is all used for. You do not seem to understand that your logic is flawed. I would have been able to provide a much more specific solution for you but you never answered several of the questions I posed previously. Specifically, are there multiple records for each user. Although you never answered that, I assume by your last explanation and your original code that you have a separate record for each question/answer pair? But, what really doesn't make sense is that you are only comparing to see fi the user submitted answers match one of the answers for the user. You are not verifying that the answer is the correct answer for any specific question. If that is what you are doing, then you should be passing an identifier with the POST data to explicitly determine which question each answer belongs to. I would love to help you further, but it would take a complete rewrite of your code and your requirements are still vague.
-
I previously explained the error in your original logic. Not sure where you came up with that last code. If you are looking for one record that matches then you need to STOP the loop as soon as you have found a match (or alternatively set the code up to assume a false match and only set to true if a match is found). The problem with your logic is that even if you find a match you continue processing more records from the query. So, you would only have the results of the LAST record. Example $searchValue = "foo"; //Set a variable to detemine if a match was found - assume false $matchFound = false; //Loop through the Db results to look for a match while($row = mysql_fetch_assoc($result)) { if($searchValue = $row['fieldName']) { //A match was found - set flag to true $matchFound = true; //Exit the loop no need to check more records break; } }
-
How do i add a checkbox to delete all games that are tiicked ?.
Psycho replied to Davie33's topic in PHP Coding Help
Not necessarily. Although it looks like you are doing more than deleting the DB records - you are also deleting some files. Below is some "expanded" sample code. By the way, giving your checkboxes the name of "checkbox[]" is just dumb, give your objects meaningful names. I'll let you replace with your custom DB functions //Check if ANY of the delete checkboxes were checked // If any were checked then $_POST['deleteIDs'] will be in the post data if(!isset($_POST['checkbox']) { //No records were selected for deletion echo 'No games selected for deletion.<br />'; echo '<a href="index.php?act=managegames">Click here to go back</a>'; } else { //Create a string of the IDs which is comma separated $idList = implode(', ', $_POST['checkbox']); //Create/run a query to select all the records seelcted for deletion $query = "SELECT file, thumbnail FROM games WHERE id IN ({$idList})"; $result = mysql_query($query); //Delete files associated with each record in DB result while($record = mysql_fetch_assoc($result)) { @unlink('../' . $row['file']); @unlink('../' . $row['thumbnail']); } //Create/run a query that will delete all the records within the comma separated list $query = "DELETE FROM games WHERE id IN ({$idList})"; $result = mysql_query($query); echo '<p style="text-align:center;">Game(s) successfully deleted.<br />'; echo '<a href="index.php?act=managegames">Click here to proceed</a></p>'; } -
How do i add a checkbox to delete all games that are tiicked ?.
Psycho replied to Davie33's topic in PHP Coding Help
I'm not going to read through all of that code to try and figure out the exact changes to make. Not to be rude, but it is very disorganized. Think about separating the "logic" of the page (the PHP code) from the presentation (the HTML). Anyway, the solution is very straight-forward. Just create your checkboxes named as an array variable with the values as the IDs of the records to be deleted. Example: <input type="checkbox" name="deleteIDs[]" value="1"> Record 1 <input type="checkbox" name="deleteIDs[]" value="2"> Record 2 <input type="checkbox" name="deleteIDs[]" value="3"> Record 3 Then in the processing page, simply use the array values in a query to delete all the recorsd if(isset($_POST['deleteIDs']) { $idList = implode(', ', $_POST['deleteIDs']); $query = "DELETE FROM table WHERE id IN ({$idList})"; $result = mysql_query($query); } -
Okay so I'm looping through the results that contains two questionIDs and two answers and I'm trying to match the two answers with the two answers from the form submission. What you just stated doesn't seem to match your code. You are comparing ONE value from the database, $answer, against TWO values submitted by the user: $answer1Post & $answer2Post Also, can there be multiple records returned from the query? If so, your code will fail at least half the time. Your IF condition would constitute a match, but all you have is an empty code block. So, if you find a match you do nothing. Then you check the next record. So, if the LAST record returned from the query fails you will get a failure even if there were previous records that matched.