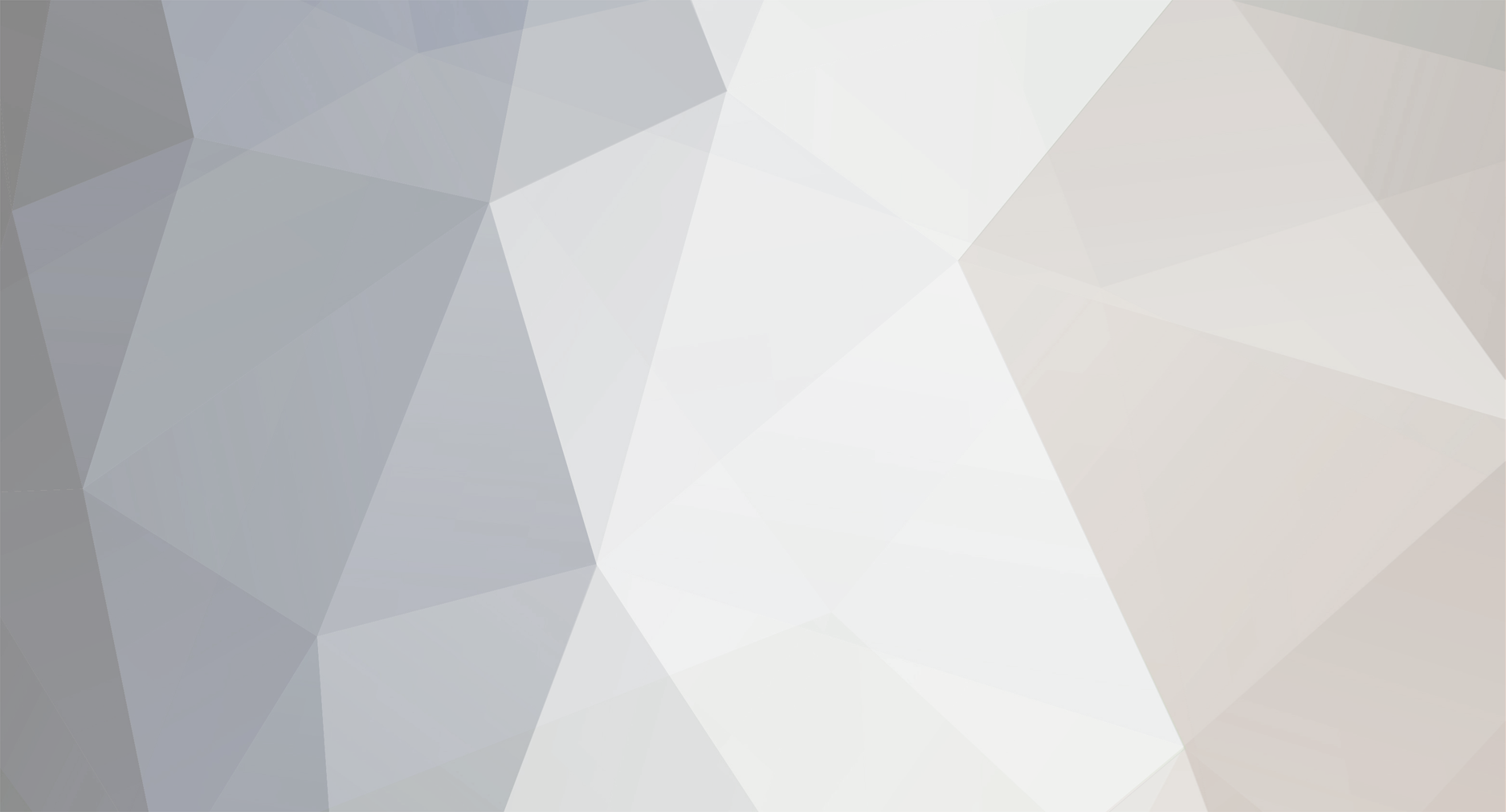
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Really? Did you read what I posted.
-
There may be free alternatives, I just didn't bother looking. Wouldn't be a bad idea to spend some time googling to see.
-
1. The offset is incorrect. The +1 at the end would cause the records to be shifted forward one record. So, page one would start at the 2nd record and every page would be shifted by one as well. $offset = ($wavesPerPage * ($currentPage - 1)) + 1; 2. You are hard-coding the current page, so you would never be able to get to any other page. I.e. you next/prev and individual page links would all show page 1 $currentPage = 1; 3. You can't do math in HTML <a href="http://www.cysticlife.org/Airwave_build.php?page=$currentPage - 1"> <a href="http://www.cysticlife.org/Airwave_build.php?page=$currentPage + 1">Next</a> That will create URLs that would look like this <a href="http://www.cysticlife.org/Airwave_build.php?page=2 - 1"> <a href="http://www.cysticlife.org/Airwave_build.php?page=2+ 1">Next</a> You need to do the math in PHP for the prev/next page and then use that in the URL. Also, referring to #2 above these parameters for the page number on the URL would have no effect since you are hard-coding the page to display. #4. Again, HTML does not do math <a href="http://www.cysticlife.org/Airwave_build.php?page=$i" class="$class">Page $i</a> Try this <a href="http://www.cysticlife.org/Airwave_build.php?page=$i" class="$class">Page <?php echo $i; ?></a> I will say that the logic in your code isn't terrible, but it is far from ideal (even when the defects are fixed). Your statement about shows, to me at least, that you are not really interested in learning.
-
So, you are saying that the correct image is shown when there are records? The fact that it shows a "missing image" means that the image tag is getting generated. Since you are hard coding the missing image URL that tells me that the URL is incorrect. Check the HTML source and check the path and name of the image. Are you running this from a page where there is no "images" folder in relation to that page? I always get this backwards, but if the url is NOT proceeded by a "/" then the path is relative from the current page. If you include a "/" at the beginning of the URL then the path is relative from the root of the site. So, if the images directory is located at www.mydomain.com/images and you are running this in the page www.mydomain.com/somefolder/index.php, then that code will make the page look for the image in www.mydomain.com/somefolder/images/
-
Since the "page" is just HTML code, there is no "screenshot" you could take. Could you do something with PHP? Absolutely! But, you would have to build your own HTML parsing engine in PHP to convert the HTML code into an image file. Basically you would have to build a browser within PHP. That would be a herculean task for what you are wanting. There are 3rd party solutions though that you could use to generate "screenshots" of web pages using PHP. Though, any good ones are going to cost you. Also, it will likely require you to install software on the web server (unless the company provides a web based service). Here is one possibility http://www.acasystems.com/en/web-thumb-activex/ $130 for a personal developer license.
-
I always recommend creating your queries as string variables and, if there is an error, echoing the query to the page so you can verify that the query is formed how you think it should be. It should be understood that you would only do this in a development environment. You would never want to display queries on the page in a production environment.
-
All that does is set a value. What you need to do is send a request to the URL (same as if a user typed it into their browser). Others may have a better solution, but one easy way to do this is with file_get_contents(). That function will call a page (as defined by the url you provide) and return the results. I would think that their service would return something that would be of value such as a success or failure code. So, if there is a failure you could re-queue the request for later or recheck your data for errors. $key = '3adfa886-415f-4e80-8b53-bddedf59c0ec'; $clickID = 'some value'; $ip = '123.123.123.123'; //Only an example $result = file_get_contents("http://bidsystem.adknowledge.com/conversion_sts.php?key={$key}&click_id={$clickID}&ip={$ip}");
-
I think you are missing the point of requinix's post. That query (and others) is trying to reference an array value - but you are not referencing the array correctly. The array name doesn't have a dollar sign in front of it to indicate it as a variable. It should look something like this "WHERE teamID=$teamAID[$i]"
-
That line would not produce that error. That error would come from trying to reference a value in the array using a key that does not exist in the array. I haven't touched this code in a long time and I must have edited it at some point without testing it. I suspect the error is from this line: $userLanguage = (!in_array($defaultLang, $languageList)) ? $languageList[0] : $defaultLang; Looking at the format of the array of languages, there wouldn't be a value with the index of 0. You can just hard-code the default language there or fix the function. As for how you get the text for the specifid language from the database, that is up to you. I would suggest a table with a column for keyname (a placeholder value to use for replacements) and a separate column for each language (using the same value as returned from the getLanguage function. Then you simply do a query of that table pulling the columns for the keyname and the selected language. However, depending on the size of your site, that could return a LOT of records - most of which you wouldn't even need. You only need the text for the current page being displayed. So, you could also have an optional column to specify the page/area that the text is for. That way you can limit the results to those that would be applicable to the page being displayed.
-
I do see your problem though. The form has an action set as action="update3.php"> So, when the form is POSTed there is no GET value for the id, therefore the query you have at the end (to get the current values to populate the form) has no $id with which to use for the query. Here is a modification of your code that should work. If the ID was sent in the POST data it will use that, otherwise it will use the GET value (if available), if neither are set it will display an error. <?php $hostname = "local"; $username = "lash"; $password = "lash"; //Connect to database $db = mysql_connect($hostname, $username, $password) or die ("not able to connected to mysql"); mysql_select_db("lash")or die("Connection Failed"); //Check if form was POSTed if(isset($_POST['id'])) { //Update record if POST data submitted $id = (int) $_POST['id']; $content = mysql_real_escape_string($_POST['content']); $sql="UPDATE page SET dtl='$content' WHERE id = $id"; mysql_query($sql) or die(mysql_error()); if(mysql_affected_rows()>0) { $message = "<span style=\"\">The data was sucessfully saved</span>"; } else { $message = "<span style=\"color:red;\">There was a problem saving the data.</span>"; } } elseif(isset($_GET['id'])) { //Get ID from query string $id = (int) $_GET['id']; } else { $message = "<span style=\"color:red;\">No record ID available.</span>"; } if(isset($id)) { //Get current value for selected record $sql = "SELECT `id`, `dtl` FROM `page` WHERE `id` = $id" $result = mysql_query($sql) or die(mysql_error()); $row = mysql_fetch_assoc($result); } mysql_close($db); ?> <html> <head></head> <body> <?php echo $message; ?></span> <form name="change_content" method="POST" action="update3.php"> <input type="hidden" name="id" value="<?php echo $row["id"]; ?>"> <textarea name="content"><?php echo $row["dtl"]; ?></textarea> <input type="submit" value="change"> </form> </body> </html>
-
Well, I get the following error when submitting the form (i.e. not a blank page)
-
Since the string appears to always contain values for each time period (even if it is 0) you could also you a simpler regex expression: preg_match_all("#\d+#", $string, $foo); list($days, $hours, $minutes, $seconds) = $foo[0]; echo "{$days} Days, {$hours} Hours, {$minutes} Minutes, {$seconds} seconds."; Not sure if that is more efficient or not, just providing an alternative solution as it helps to think differently when trying to solve problems.
-
The second argument is an additional modification to the password (i.e. SALT) that is to be done BEFORE creating the hash. But, you must use the SAME salt each time you create/compare passwords. The reason for this is that people have created what are called Rainbow Tables which are large collections of values and their corresponding hashes (with no salt). So, lets say a malicious user has gained access to our database. There is a column with all the users' hashed passwords. If there was no salt on those hashes then the malicious user could run those hashed values against the rainbow table and, potentially, determine the users' passwords. That is why it is so important for user to use passwords that are not just basic dictionary words. It would be impossible to generate a database with all possible passwords and their corresponding hashes due to CPU and memory requirements. But, these tables do include most if not all possible passwords that contain simple "words". By applying a SALT to the value before hashing it a malicious user would have to 1) know how the salt is created and 2) generate a unique rainbow table just to attempt to backward engineer your hashes. That would be an extravagant amount of work and may return little if any results. So, when you add a salt to a value and store the hashed result you will need to apply the same salt when comparing the user entered value when they attempt to log in. So, you must always use the same salt, but it can be unique to each user. For example, you could append the timestamp of when their account was created. But, the SALT can be any manipulation of the password such as reversing the text, appending other text, etc.
-
You know, I was looking at that linked page again and trying to figure out why the example function was unsetting the array elements. Then looking at the first user submitted comment I remembered why: magic quotes are applied to the keys as well as the values. So, you need to run strip slashes against both the keys and the values, but I don't think you can change a key value - you need to unset the current one and recreate a new one with the modified value?
-
Here is what you need to do. 1. Have a default language to use when no language is set. Alternatively, you could redirect the user to a page to select a language if they have not set one before. 2. When the user selects a language you need to store that value. Then when the user selects another page you would first check if they selected a language (i.e. POST value) or if there is a stored selection. You can choose to store the selected language in a SESSION or COOKIE or both. Here is a function I built previously. You would just call getLanguage() on each page load and it will return the language to use for that page. There are plenty of comments so you should be able to modify if needed. The code below is a complete working example <?php // Function: getLanguage($languageList [, $selectedLang] [, $defaultLang]) // // Parameters: // - $languageList: (Required) An array of all available languages for the user // - $selectedLang: (Optional) The language the user has selected (GET or POST) // - $defaultLang: (Optional) the default language to use if unable to determine // user's language from seleted or saved values. function getLanguage($languageList, $selectedLang=null, $defaultLang=null) { //Set the default value (or first option in $languageList) $userLanguage = (!in_array($defaultLang, $languageList)) ? $languageList[0] : $defaultLang; //Detemine selected/saved user language if (!is_null($selectedLang) && in_array($selectedLang, $languageList)) { //Request was made to change the language $userLanguage = $selectedLang; setcookie('language', $userLanguage, time()+3600*24*365); //Expires in 1 year $_SESSION['language'] = $userLanguage; } else if (isset($_SESSION['language']) && in_array($_SESSION['language'], $languageList)) { //There is a saved language value in the SESSION data $userLanguage = $_SESSION['language']; } else if (isset($_COOKIE['language']) && in_array($_COOKIE['language'], $languageList)) { //There is a saved language value in the COOKIE data $userLanguage = $_COOKIE['language']; $_SESSION['language'] = $userLanguage; } //return the user's language return $userLanguage; } //Example usage // //Create list of available languages $languages = array ('Spanish'=>'sp', 'French'=>'fr', 'English'=>'en'); //Get the language to show the user $currentLanguage = getLanguage($languages, $_GET['lang'], 'en'); //Create vars for illustrative purposes $sessionLang = (isset($_SESSION['language'])) ? $_SESSION['language'] : 'Not set' ; $cookieLang = (isset($_COOKIE['language'])) ? $_COOKIE['language'] : 'Not set' ; ?> <html> <body> Current language: <?php echo array_search($currentLanguage, $languages) . " ($currentLanguage)"; ?> <br /><br /> $_GET['lang']: <?php echo $_GET['lang']; ?><br /> Session language: <?php echo $sessionLang; ?><br /> Cookie language: <?php echo $cookieLang; ?><br /><br /> Change Language <?php foreach ($languages as $name => $value) { echo "<a href=\"?lang={$value}\">{$name}</a> "; } ?> </body> </html>
-
The manual does include a process to properly handle cleansing input that may be affected by magic quotes - including sub-arrays: http://www.php.net/manual/en/security.magicquotes.disabling.php See example #2
-
1. Do not run queries in loops. You don't need to run the query to get the list of names multiple times - it will be the same data. just query it once before you run that loop and then use the data. 2. You don't show how you are creating the select field. I provided some code below that shows a simple method of creating the select options using the single query of name values. 3. In this type of scenario I would a) name the checkboxes as you have them - an unindexed array with the uniqu ID as the value. b) name the corresponding fields as arrays BUT give them a named index using the unique ID. Then when you process the POST results, just check the list of IDs passed in the checkbox array. Then for each ID passed in the checkbox array reference the corresponding fields. Sample replacement code //Function to create select options function createOptions($values, $selected) { foreach($values as $value) { $selected = ($value==$selected) ? ' selected="selected"' : ''; echo "<option value='{$value}'{$selected}>{$value}</option>\n"; } } //Run query to get names ONE TIME $queryNames = "SELECT name FROM users"; $resultNames = mysql_query($queryNames) or die(mysql_error()); $names = array(); while($row = mysql_fetch_assoc($resultName)) { $names[] = $row['name']; } while($row = mysql_fetch_array($result)) { $app_id = $row['app_id']; echo "<tr class='alt' align='center'>"; echo "<td><input type='checkbox' name='periods[]' value='{$app_id}'/></td>"; echo "<td>{$app_id}</td>"; echo "<select name=\"UploadedBy[{$app_id}]\">"; echo createOptions($names, $row['UploadedBy']); echo "</select>"; }
-
As long as you check the GET value and explicitly set the values that you are checking to determine mobile or PC, yes it will work.
-
Never do queries in loop or how you are doing it there. All you need is ONE QUERY: SELECT * FROM `$show` ORDER BY `season` ASC, `episode` ASC You will than have all the records first ordered by season, then ordered by episode. Then process the records using PHP
-
Simple. If you are using User Agent as the means to determine when to show "mobile" content. Then just check for the value on the query string you are using to "trick" the system and hard code the user agent value if(isset($_GET['view']) && $_GET['view']=='myothersite.com') { $_SERVER['HTTP_USER_AGENT'] = 'some_mobile_device_value'; }
-
Well, in my opinion, and I would say the vast majority of people knowledgeable in web development, you shouldn't be using IFrames at all - even if the site is meant only for typical web browsers on a computer. One method of having a site display differently based upon the type of device is the CSS media property. You create the content in a generic fashion and determine the display of the output using CSS style sheets. Within the style sheets you can specify different style properties based on the media (screen, handheld, print, etc.). But, if you wrap content inside an IFrame, the CSS can't "fix" that for a handheld device.
-
Instead of doing a print_r() on $games, do a print_r() on $_POST to validate that the data is getting passed correctly
-
Looking For Advice, Select 2 or 3 Numbers for 20 columns
Psycho replied to Failing_Solutions's topic in PHP Coding Help
Building upon silkfire's suggestion, the following query should give you the number of times that a "set" of numbers has appeared in any drawing. To be more specific it is the number of drawings that contained all three of the target numbers. The pattern of the query should be obvious so you can modify it for any quantity of target numbers. SELECT count(`GameId`) FROM june122011 WHERE $num1 IN (`A`,`B`,`C`,`D`,`E`,`F`,`G`,`H`,`I`,`J`,`K`,`L`,`M`,`N`,`O`,`P`,`Q`,`R`,`S`,`T`) AND $num2 IN (`A`,`B`,`C`,`D`,`E`,`F`,`G`,`H`,`I`,`J`,`K`,`L`,`M`,`N`,`O`,`P`,`Q`,`R`,`S`,`T`) AND $num3 IN (`A`,`B`,`C`,`D`,`E`,`F`,`G`,`H`,`I`,`J`,`K`,`L`,`M`,`N`,`O`,`P`,`Q`,`R`,`S`,`T`) Although I used the table name you described above, "june122011", you should not use different tables to separate records by date. have one table and add a column for the date. -
I already explained that. By putting the function call in the while() loop you are calling the grab_ip() function on each iteration of the loop. So, instead of iterating through the results of ONE call to the grab_ip() function you are only processing the first record, getting a new set of records, processing the first record, getting a new set of records, etc., etc.
-
Well, you only have one loop in that code while($row = mysql_fetch_array($result->grab_ip(), MYSQL_BOTH)){ On each iteration of the loop you are calling$result->grab_ip(). So it is rerunning the query on each iteration of the loop - so it never ends. You need to call grab_ip() ONE TIME and THEN run the loop on that result. Also, why have the overhead of using mysql_fetch_array() to return both associative and numerically indexed values when you are only usign the associative values? $ipResult = $result->grab_ip(); while($row = mysql_fetch_assoc($ipResult)){