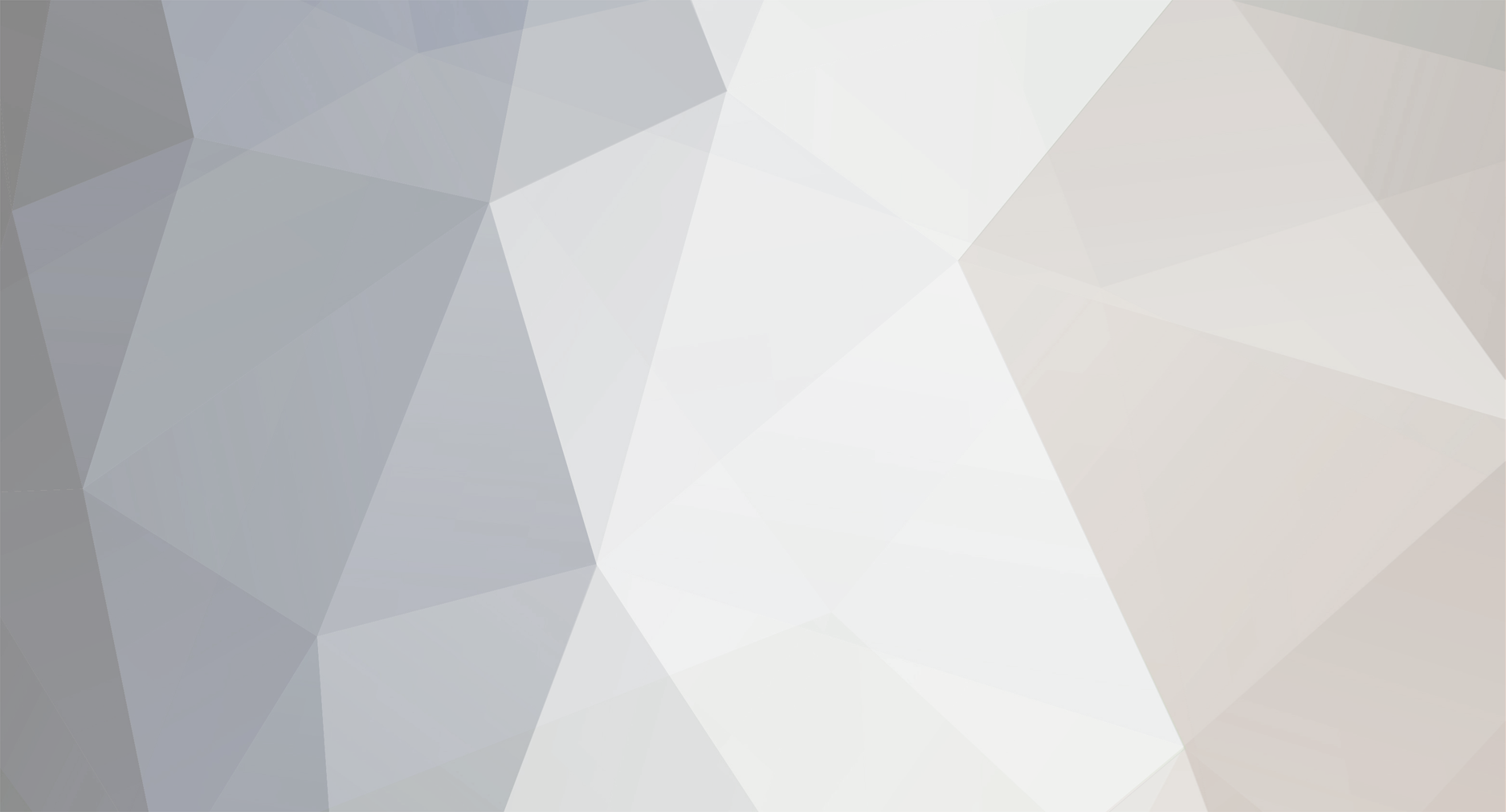
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=320749.0
-
How is this a PHP question? Moving to MySQL forumn. You should always create your queries as a string variable so you can echo the entire query to the page for debugging purposes. But, from the little information you have provided I can see that there is no opening quotation mark for the state value. Change your code to $query = "INSERT INTO user (username, email, last, address1, address2, City, State, Postcode, Country, Phone) VALUES ('$frist', '$mail', '$lastname', '$add1', '$add2', '$city', '$state', '$post', '$country',' $number')"; mysql_query()or die("Query:<br />{$query}<br />Error:<br />".mysql_error()); EDIT: Also, if id is an auto-increment value, there is no need to include it in the query at all. EDIT #2: That code should work assuming there are no other errors, but I don't think you meant to name the variable for the first name as $frist. You used the same name when you set it and when you use it, so it would work, but I'm guessing it was a typo that you copied/pasted
-
I don't see where $status is being updated. If $status is never updated the loop would run indefinitely. I would probably add some sort of counter to halt execution at some outer boundry; $page = 1; while($status != null) { curl_setopt($ch, CURLOPT_HTTPHEADER, array("Accept: application/xml", "Authorization: xxxxxxxxxxxxx:xxxxxxxxxxxxxxxx", "Page: {$page}")); $page++; //Stop at 1000 pages to prevent infinite loop if($page>1000) { break; } }
-
Just noticed there was an error in the last variable of the last query. It should look like this $query = "UPDATE records SET order = $newPosition WHERE id = $recordID" Also, I would add some validation logic to the code to ensure the new position is valid (an integer, not less than 1, and not greater than the number of current records).
-
So, there is an explicit ordering of the records. That means when the order of one record is updated, you will need to update the order of one or more other records as. Therefore, when you get the new order index for a record you will need to first query for the current order index and then update multiple records. Example 1. Record A 2. Record B 3. Record C 4. Record D (Change to position 2) 5. Record E In the above scenario you would query the current position of Record D (4), update that record's position AND update the position for all records from the new postion to record D's original position. You will need to figure out the logic for Of course the logic is different based upon whether the record is moving up or down Psuedo code $recordID = mysql_real_escape_string($_GET['recordID']); $newPosition = mysql_real_escape_string($_GET['position']); //Get records current position $query = "SELECT position FROM records where id = {$recordID}"; $result = mysql_query($query); $oldPosition = mysql_result($result, 0); if($newPosition<$oldPosition) { $movement = 1; $movementStart = $newPosition; $movementend = $oldPosition; } else { $movement = -1; $movementStart = $newPosition; $movementend = $oldPosition; } //Move all the affected records $query = "UPDATE records SET order = order+$movement WHERE order >= $movementStart AND order <= $movementend" $result = mysql_query($query); //Move the specific record to its new position $query = "UPDATE records SET order = $newPosition WHERE id = $oldPosition" $result = mysql_query($query);
-
I can only say that I have had problems receiving emails to certail addresses from certain domains and the only parameter I could not rule out was that the accounts experiencing the problem were redirects. I assumed - possibly incorrectly - was that the sneding server was doing some type of check.
-
I'll also add that for my personal domain I use a "redirect" address. For example, any email sent to me@mydomain.com will be redirected to me@myispdomain.com. So, trying to "validate" my email will fail. I agree with Pikachu2000. Simply validating that they have supplied a valid email address tells you nothing.
-
Very simple example: $from_domain = strtolower(strrchr($email_from, '@')); if($from_domain!='@xyz.com' && $from_domain!='@xxx.com') { echo "That domain is not allowed."; exit(); }
-
I'm still not sure I fully understand what you are saying. I woud assume that the category(ies) are determined at the time the suer creates the initial post. Either as a selection by the user or selected automatically based upon the contect of where the user chooses to initiate the post creation (use a hidden field). So, I would expect you are asking how to associate new comments with the appropriate post. I would assume that on the page where a user views the post there is a link or a button to add a comment. If it is a link, then I would add the post ID as a parameter. If a button, then it should be a form with a hidden field for the post ID. Then, on the page where the comment is actually added, use the post ID (passed via GET or POST) and populate a hidden field so it will be available with the form submission for the comment. Also, you would use mysql_real_escape_string() on the data received from the user. There is no benefit on running it against data the code puts into a form and could actually cause the script to fail for reasons I don't want to take the time to explain right now. I meant to add aditional information about that. So, as stated previously, the links for the categories would look something like this: <a href="http://www.mydomain.com/blog/category/?id={$rcategory['id']}">[Category Name]</a>[code=php:0] The page that is loaded from that link would have a query to find all posts associated with that categoryID. Based upon how the tables are constructed the query might look like this: [code=php:0]$categoryID = mysql_real_escape_string($_GET['id']); $query = "SELECT p.post_id, p.post_text, etc. FROM posts p JOIN post_catergories as pc WHERE pc.category_id=$categoryID"; //Run query and use results to generate output of posts for selected category
-
Can articles belong to multiple categories? If so, then you should use two tables for categories. One table will contain the list of available categories (category_id and category_name). And a second table would be used to associate posts to categories (article_id, category_id). If articles cannot belon to multiple categories, then I would still use the first table to list the available categories and then add a column to the articles table to specify the category_id. I'm not following you here. It seems you are mixing up different things in the same question. First, as to whether you should use a form (which can use GET or POST) or to simply have links with the ID appended to them, there is no right or wrong. Each method has its benefits and drawbacks. For the purpose you have explained I would simply append the ID to the URL as you showed above. But, you should always think about validating the data. If any posts are restricted you need to validate that the user has access to the post id being passed in the URL. Once you have the post/article id everything else is fairly straitforward. In many instances you would use JOINs, but for what you have explained I would do three different queries: one for the post, one for the comments, and one for the categories. For the categories, when listing them I would create them as links in the form <a href="http://www.mydomain.com/blog/category/?id={$rcategory['id']}">[Category Name] That page would then use the category ID to query all the relevant posts</a> The one thing I am not sure of is if yuo want only the comments applicable to the currently displayed post to be diaplyed or all the posts. I have assumed the former in the code below. So, the queries on the posts page would go something like this: $postID = mysql_real_escape_string($_GET['id']); $query = "SELECT field1, field2, etc. FROM articles WHERE id=$postID"; //Run query and use results to generate output of the post $query = "SELECT field1, field2, etc. FROM comments WHERE article_id=$postID"; //Run query and use results to generate output for the comments $query = "SELECT c.category_id, c.category_name, etc. FROM categories c JOIN post_catergories as pc --table to assoc posts to categories WHERE pc.article_id=$postID"; //Run query and use results to generate categories ouput
-
I see multiple problems. 1. This is not valid HTML code <action="http://www.myserver.com/myscript.php" method="post"> action is a parameter for a FORM tag - there is no ACTION tag. 2. When you submit values via a form you will need to access them using $_POST['fieldName'] (assuming you are using the method POST) So your form should look something like this: <form action="" method="post"> Email: <input type="hidden" name="email" id="email" value="example_username"><br /> Password: <input type="password" name="password" id="password" size="15"><br /> <button type="submit">Submit</button> And your processing code should look something like this $subject = trim($_POST['email']); $message = trim($_POST['password']); mail ("email@myserver.com", "Hello", $subject, $message);
-
I would also add that having two statuses with the values of "read" and "unread" is not ideal. Unless you plan to add additional statuses I would suggest the database column be called "read" and you should store a boolean value (i.e. true/false). Anyway, building upon dropkick_pdx's suggestioin here is what I would propose. 1. Add CSS code to your page or to an external style sheet to create two classes "read" and "unread". 2. Name the images "read.jpg" and "unread.jpg" 3. Add the following logic to the top of your script (ionclude the db query of course): $messagesHTML = ''; while ($row = mysql_fetch_assoc($result)) { $messagesHTML .= " <tr class=\"{$row['message_status']}\">\n"; $messagesHTML .= " <td><img src=\"assets/{$row['message_status']}.jpg\" /></td>\n"; $messagesHTML .= " <td>{$row['message_status']}</td>\n"; $messagesHTML .= " <td>{$row['date_sent']}</td>\n"; $messagesHTML .= " <td>{$row['contact_name']}</td>\n"; $messagesHTML .= " <td>{$row['subject']}</td>\n"; $messagesHTML .= " <tr>\n"; } 4. Then in the body of the HTML code do this <table border="1"> <tr width="200"> <th>View</th> </tr> <?php echo $messagesHTML; ?> </table>
-
Um, no. How is the server going to know that? You would have to submit the file time when you upload the file. There is no automated way to do that which I can think of. You could use a java (not javascript) applet. You would either have to find one or build one yourself, but that would create a security risk for the user. I would never install such an applet for any site. So, you would need to include a field for the user to enter the date in when they upload the file.
-
if I dont use strip_tags, how can I prevent users to send textareas or other html codes in postings,since I just want to allow br tags and not any other things Sorry, I mistook that for strip_slashes, but my reply still holds true. Unless there is a business need to remove those tags - let the user submit them and store the data in the database exactly as the user submitted it (escaping for SQL Injection of course). Then modify the data when you display it. In this instance I wouldn't use strip_tags(), instead I would use htmlentities() when I display the text to convert any HTML code so it is displayed on the page and not interpreted.. That way if a user inputs "<b>my name</b>", the output - as displayed on screen would be "<b>my name</b>" not "my name". There may be a reason why someone would enter html code as a value and using strip_tags would remove that data.
-
filemtime(): This function returns the datetime the file was last modified. http://www.php.net/manual/en/function.filemtime.php
-
Not quite. If you turn off magic_quotes there is no need to strip_tags on user input and you don't want to add BR tags to the data you are storing. You add the BR tags when displayng the information Storing the data: $dbSafeInput = mysql_real_escape_string($_POST['userInput']); Displaying the input (if it may contain line breaks echo nl2br($inputFromDB); But, if you were displaying that same data in a textarea to be edited then you wouldn't use nl2br <textarea><?php echo $inputFromDB; ?></textarea>
-
If you are on a server where magic_quotes is turned on the slashes are automatically added to user input. In those instances you should turn off magic_quotes if you have access to do so. If you don't have access to turn off magic_quotes, then you should use strip_slashes() on user input THEN run mysql_real_escape_string() before you insert int he database. The rule I always try to follow is to store data in its native/raw format. Then modify the data based on how I need it. For example, by using nl2br() when you output to the screen you maintain the original line breaks. That way you can still use the native content stored int he database to populate a textarea for editing purposes.
-
You can also set the field to auto-populate the timestamp whenever the record is created/updated.
-
Changing the date format from epoch to human readable
Psycho replied to toocreepy's topic in PHP Coding Help
I really hate trying to code in the blind, but I will make some assumptions and give you a possible solution: Add the second line below where specified // View column for publishdate field $this->dataset['publishdate'] = date('l, F j, Y H:i:s A', $this->dataset['publishdate']); $column = new TextViewColumn('publishdate', 'Publishdate', $this->dataset); $column->SetOrderable(true); If that doesn't work do a data dump of $this->dataset so we can determine what really needs to be modified. -
Changing the date format from epoch to human readable
Psycho replied to toocreepy's topic in PHP Coding Help
Line 536 is not outputting anything it is calling a class. And the class is not included in that file. Which is amazing since that file has nearly 2,000 lines of code (should really look into modularizing your code). Anyway, it is impossible for us to know what that class is really doing. I was going to suggest modifying the date before you call the class to output the publish date, but the date is apparently in the object $this->dataset. However, you didn't state how the value is stored in that object and I'm not going to reverse engineer all of that code to figure it out. It could be simply $this->dataset['publishdate'] or $this->dataset['PublishDate'] (I see both used) or it could be in a subarray of that object. I can tell from the values passed to the class $column = new TextViewColumn('publishdate', 'Publishdate', $this->dataset); That the class must have some specific code for each value since the output actually has "Publish Date". So, I would edit the class so the code to output the publish date will expect a timestamp but will output the date in the format you want. -
What are you using to convert the page to a PDF? If that process uses the "print" output of the page to generate the PDF then there is a simple solution. CSS allows you to specify different styles for elements based upon the "media". I have used this may times in the past to have elements such as the navigation section not display in the printed output (the navigation links have no purpose in a printed output). For any elements that you do not want in the printed output, give them a display property of none for the "print" media. Here is an old post where I provided some example code: http://www.phpfreaks.com/forums/javascript-help/print-function!/msg1093659/#msg1093659
-
Not tested <?php //Specify the number of columns $max_columns = 4; if (is_array($order->products)) { $context = array( 'revision' => 'formatted', 'type' => 'order_product', 'subject' => array('order' => $order,), ); //Start output table $output = "<table border=\"1\">\n"; $recordCount = 0; foreach ($order->products as $product) { $price_info = array( 'price' => $product->price, 'qty' => $product->qty, ); $context['subject']['order_product'] = $product; $context['subject']['node'] = node_load($product->nid); //generate the output $recordCount++; if($recordCount % $max_columns == 1) { //Open table row if first record of row $output .= "<tr>\n"; } $output .= "<td>" . $product->model . "</td>\n"; if($recordCount % $max_columns == 0) { //Close table row if last record of row $output .= "</tr>\n"; } } //Close out last row if needed if($recordCount % $max_columns != 0) { while($recordCount % $max_columns != 0) { $output .= "<td></td>\n"; } $output .= "</tr>\n"; } //Close output table $output .= "</table>\n"; echo $output; } ?>
-
Working with times stored with one separate date.
Psycho replied to arrayGen's topic in PHP Coding Help
You did post this in the PHP forum, right? So it is only logical that you were looking for a PHP solution. If you wanted a database or other type of solution you should post in the appropriate forum. -
Working with times stored with one separate date.
Psycho replied to arrayGen's topic in PHP Coding Help
Why do you see the need to resort to name calling? What was not clear in the previous posts was that the end time may - intentionally - be less than the start time. If that is the case, then I would assume that the end time is for the next day. So the solution is simple. If the end time is before the start time, then increment the end date to +1 days. $start_time = "11:00:00"; $end_time = "00:00:00"; $date = "2011-01-01"; $start_timestamp = strtotime("{$date} {$start_time}"); $end_timestamp = strtotime("{$date} {$end_time}"); //Check if end time is before start time if($end_timestamp < $start_timestamp) { //Adjust end date to next day $end_timestamp = strtotime("{$date} {$end_time} +1 days"); } echo "Start date/time: " . date('m-d-Y H:i:s', $start_timestamp); echo "<br />End date/time: " . date('m-d-Y H:i:s', $end_timestamp); //Output //Start date/time: 01-01-2011 11:00:00 //End date/time: 01-02-2011 01:00:00 -
Working with times stored with one separate date.
Psycho replied to arrayGen's topic in PHP Coding Help
I don't think you should be throwing derogatory names around like that. Because as arrayGen just alluded to, it seems YOU are the only one that doesn't see the obvious solution: Combine the date with each time - individually - to come up with two timestamps! Although your example is in error since the end time is before the start time. 00:00:00 is the beginning of a day not the end of a day. So, in reality your two timestamps should be Start timestamp: 2011-01-01 11:00:00 End timestamp: 2011-02-01 00:00:00