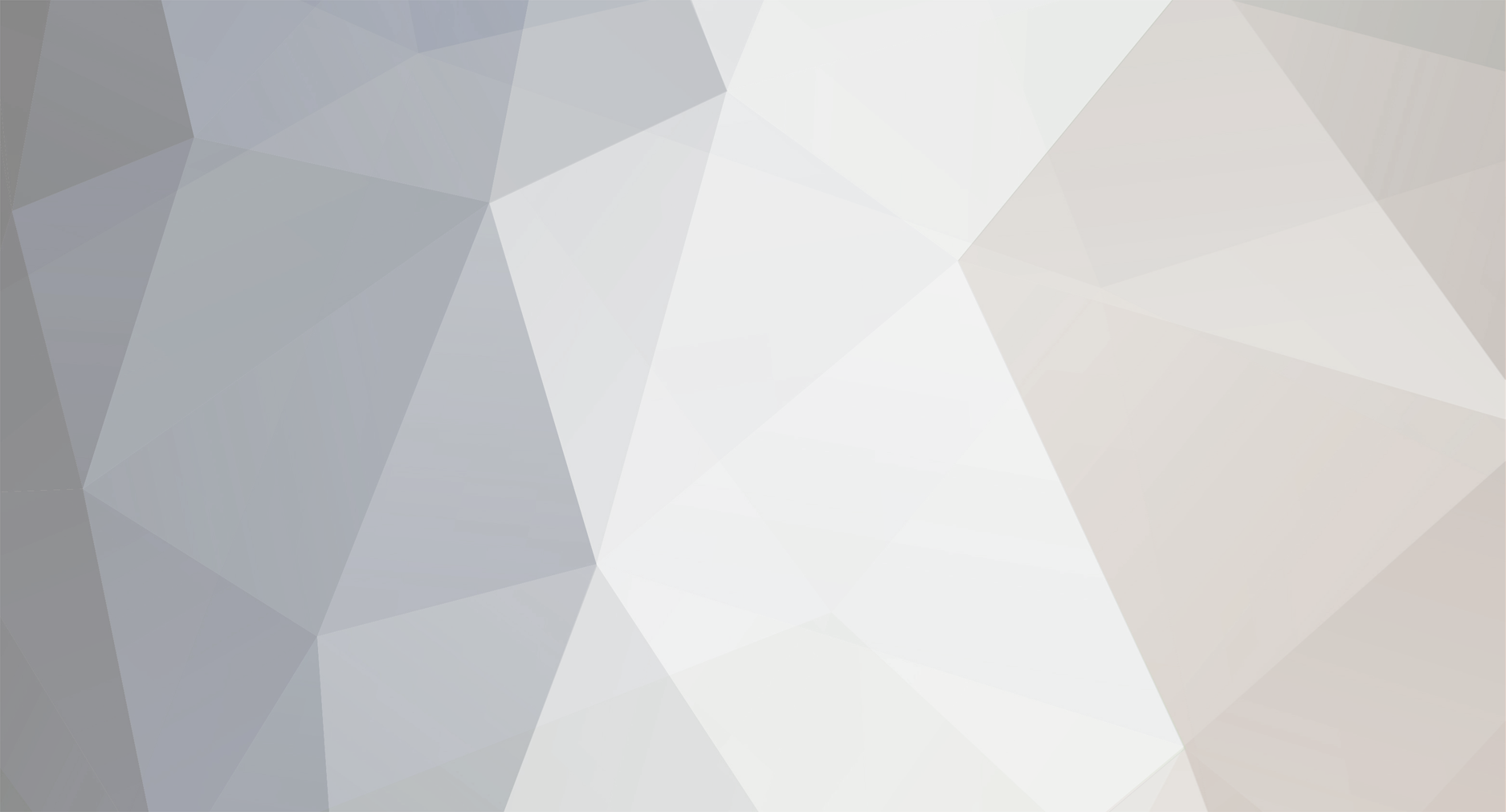
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
filling a form with database information
Psycho replied to andyonline2011's topic in PHP Coding Help
//get default shipping and payment address $mysql = "SELECT cu_id, od_shipping_first_name, od_shipping_last_name, od_shipping_address1, od_shipping_address2, od_shipping_phone, od_shipping_city, od_shipping_state, od_shipping_postal_code FROM tbl_customer WHERE cu_id = $cu_id"; $result = mysqli_query($mysql, $mysqli); $address = mysql_fetch_assoc($result); //populate form with values ? $txtShippingFirstName = $address['od_shipping_first_name']; $txtShippingLastName = $address['od_shipping_last_name']; $txtShippingAddress1 = $address['od_shipping_address1']; $txtShippingAddress2 = $address['od_shipping_address2']; $txtShippingPhone = $address['od_shipping_phone']; $txtShippingCity = $address['od_shipping_city']; $txtShippingState = $address['od_shipping_state']; $txtShippingPostalCode = $address['od_shipping_postal_code']; You should add some validation/error handling logic to that code. For example, what if the value of $_SESSION['cu_id'] does not exist in the database? -
My confusion comes from the fact that your original code appeared to be attempting to associate every member with the blog entry being created in the INSERT query. Since you state that you've resolved this I am making the post as solved.
-
The logic in that doesn't seem right. Those three blocks of code are independant of one another. So, if the user is logged in for 'rest' and not 'chef' then both the first and third sections are executed. But the third section seems to be for users that aren't logged in. The first two blocks are identical except for the URL for the user panel. As for the javascript, you really should create a javascript function instead of trying to stuff all that code into an onclick event parameter. Also, since you are using the exact same format for all the links, I would create a single function to generate the links to prevent errors in display from typos. Plus, you can easily modify the format of the links by changing the function and not many lines of code. Here is what I would do: <?php //Functin to create links in consistent manner function createLinkTD($title, $href, $clickEvent=false) { $onclick = ($clickEvent) ? " onclick=\"{$clickEvent};\"" : ''; $linkHTML = "<td valign=\"middle\" class=\"topnav\">"; $linkHTML .= "<div align=\"center\">"; $linkHTML .= "<a href=\"{$href}\"{$onclick}>{$title}</a>"; $linkHTML .= "</div>"; $linkHTML .= "</td>\n"; return $linkHTML; } //Determine the links to be created $links = createLinkTD('Home', 'index.php'); if(isset($_SESSION['rest']) || isset($_SESSION['chef'])) { //Determine User Panel HREF based on which session value is set $userPanelHREF = (isset($_SESSION['rest'])) ? 'restpanel.php' : 'chefpanel.php'; $links .= createLinkTD('User Panel', $userPanelHREF); $links .= createLinkTD('Logout', 'logout.php'); } else { $links .= createLinkTD('Log In', 'javascript:void(0)', 'logIn()'); $links .= createLinkTD('Sign Up', 'register.php'); $links .= createLinkTD('Contact Us', 'contact.php'); } //Create the HTML output ?> <script type="text/javascript"> function logIn() { document.getElementById('light').style.display='block'; document.getElementById('fade').style.display='block'; } </script> <table width=\"300\" border=\"0\" cellpadding=\"0\" cellspacing=\"0\"> <tr> <?php echo $links; ?> </tr> </table>
-
OK, I don't know what I was thinking. You CAN do this with a single query. Not tested, but it should go something like this: $title = mysql_real_escape_string($_POST['title']); $entry = mysql_real_escape_string($_POST['entry']); $query = "INSERT INTO blogs (member_id, title, entry, blog_date) SELECT member_id, {$title}, {$entry}, NOW() FROM members";
-
Well, the problem is that you aren't running a sub query. $query = "INSERT INTO blogs ( member_id, title, entry, blog_date) VALUES ( 'SELECT member_id FROM members', '{$_POST['title']}', '{$_POST['entry']}', NOW())"; That query is attempty to put the literal text string 'SELECT member_id FROM members' into the "member_id" field. Since that field only accepts integers it won't accept the text and uses 0 instead. I really don't understand what you are trying to accomplish here, but you can't do what you are trying to do. The problem is that you must have separate, "complete" records to input. It seems you are trying to one record for each user into theblogs table using the same values for the title, entry and blog_date. I guess that is what is confusing me. It doesn't make sense that you would create duplicate blog entries for all users. Anyway, if that is what you really, really want to do I think the only way is with two queries. One to get the list of users, then use that data to create a second query to insert the records into the blog table: //Query the members table for the IDs $query = "SELECT member_id FROM members"; $result = mysql_query($query); //Create array to hold the blog records to be inserted $insertValues = array(); $title = mysql_real_escape_string($_POST['title']); $entry = mysql_real_escape_string($_POST['entry']); //Loop through members resutls to populate the values array while($member = mysql_fetch_assoc($result)) { $insertValues[] = "('{$member['member_id']}', '{$title}', '{$entry}', NOW())"; } //Create and run insert query with all values $query = "INSERT INTO blogs ( member_id, title, entry, blog_date)" . implode(', ', $insertValues); $result = mysql_query($query); Note, you could also set up the blog_date field so the default value will be the current timestamp, that way you don't need to include that in the INSERT query.
-
I'm assuming that "accountsettings" is the name of a form and that "country" is the name of a select field. Although the way you are referencing the field usually works, it is not the preferred method. I would suggest referencing the select field by ID (option 1) or refrence explicitly (option 2) Option 1 var selectObj = document.getElementById('country'); var country_text = selectObj.options[selectObj.selectedIndex].text; Option 2 var selectObj = document.forms['accountsettings'].elements['country']; var country_text = selectObj.options[selectObj.selectedIndex].text;
-
Cannot send session cookie - eaders already sent
Psycho replied to mat3000000's topic in PHP Coding Help
So, that is the file login.php? Is there ANYTHING before the first opening "<?php" tag - including spaces, line breaks, etc? EDIT Wait, what application are you using to edit your files? You must save as a plain text file. There are plenty of free text editors available. If all else fails use notepad. -
No to mention you should have stated what errors you were getting. If you want free help at least make the effort to provide the relevant information. If the only problem is that you have a column called replace, then you just need to put backquotes around the field name in your query (which isn't a bad idea just as common practice): $query = "INSERT INTO maintdata (`apt`, `name`, `datereceived`, `time`, `item`, `repair`, `replace`, `action` ,`compday`, `compmoyr`, `cost`, `charge`, `ordno`) VALUES ('$apt', '$name', '$datereceived', '$time', '$item', '$repair', '$replace', '$action', '$compday', '$compmoyr', '$cost', '$charge', '$ordno')";
-
Why is my numbering suddenly skipping in my php, mysql application
Psycho replied to JUSTMELAT's topic in PHP Coding Help
You are correct, but addslashes() is not what you should be using. Instead use mysql_real_escape_string(). Per the manual for addslashes() it states: -
Why is my numbering suddenly skipping in my php, mysql application
Psycho replied to JUSTMELAT's topic in PHP Coding Help
Here is the code you attached: //Add the answers to the Answer table while (list($key,$val) = each($_REQUEST)) { if (preg_match("/^Q/",$key)) { if (is_array($_REQUEST[$key])) { for ($i=0;$i<count($_REQUEST[$key]);$i++) { if (!empty($_REQUEST[$key][$i])) { $sql = "INSERT INTO ANSWER (Q_ID,TEXT,R_NUMBER) VALUES"; $sql .= "('" . $key . "[]','" . addslashes($_REQUEST[$key][$i]) . "','" . $requestNumber . "')"; mysql_query($sql,$db) or die("ERROR: " . mysql_error()); } } } else { if (!empty($_REQUEST[$key])) { $sql = "INSERT INTO ANSWER (Q_ID,TEXT,R_NUMBER) VALUES"; $sql .= "('" . $key . "','" . addslashes($_REQUEST[$key]) . "','" . $requestNumber . "')"; mysql_query($sql,$db) or die("ERROR: " . mysql_error()); } } } Looking at that there are quite a few condition statements that must pass in order for one of the two INSERT queries to be run. Assuming that code is always called after a record is inserted into the REQUEST table I would assume that one fo the conditions is failing. The possible causes I see are as follows: 1. The WHILE loop is not running because $_REQUEST has no values. 2. The preg_match("/^Q/",$key) condition test fails 3. When $_REQUEST[$key] is an array the !empty($_REQUEST[$key][$i]) condition is failing 3. When $_REQUEST[$key] is not an array the !empty($_REQUEST[$key]) condition is failing. I think you should first start looking at #1. Using $_REQUEST is not recommended (google to find out why). That global variable "typically" holds the contents of $_GET, $_POST and $_COOKIE. But, according to the manual So, if that server configuration changed, your code may not functiona correctly. It is really bad practice to use all $_REQUEST values for running db queries. That code will run against all those variables - NOT just the form input. All it would take is to add some JavaScript code to add a cookie for what would seem to be a benign feature and that code would do some very odd things. You should probably change that code to use $_POST or $_GET depending upon the method used by the form. Whichever variable you are using you can test it by printing its contents to the page to validate the values. You could also do the same for the condition statements by echoing to the page whenthe conditions pass/fail to understand what is going on. I have modified your code below to add a simple debugging feature that you can turn on/off to help find the cause. Note: I would typically make this turn on/off based upon adding a parameter to the URL so you can test it in a production environment without affecting the users, but since you are using the $_REQUEST object that is out of the question. <?php //Change to false to suppress debug messages $debug = true; $debugTxt = '' //Add the answers to the Answer table $debugTxt .= "Contents of REQUEST:\n" . print_r($_REQUEST) . "\n\n"; while (list($key,$val) = each($_REQUEST)) { $debugTxt .= "<b>Processing key {$key}:</b>\n"; if (preg_match("/^Q/",$key)) { $debugTxt .= " - preg_match(/^Q/) passed\n"; if (is_array($_REQUEST[$key])) { $debugTxt .= " - Is an array\n"; for ($i=0; $i<count($_REQUEST[$key]); $i++) { if (!empty($_REQUEST[$key][$i])) { $debugTxt .= " - Is NOT empty\n"; $sql = "INSERT INTO ANSWER (Q_ID,TEXT,R_NUMBER) VALUES"; $sql .= "('" . $key . "[]','" . addslashes($_REQUEST[$key][$i]) . "','" . $requestNumber . "')"; mysql_query($sql,$db) or die("ERROR: " . mysql_error()); $debugTxt .= " - Query: {$sql}\n"; } else { $debugTxt .= " - Is empty\n" } } } else { $debugTxt .= " - Is NOT an array\n"; if (!empty($_REQUEST[$key])) { $debugTxt .= " - Is NOT empty\n"; $sql = "INSERT INTO ANSWER (Q_ID,TEXT,R_NUMBER) VALUES"; $sql .= "('" . $key . "','" . addslashes($_REQUEST[$key]) . "','" . $requestNumber . "')"; $debugTxt .= " - Query: {$sql}\n"; mysql_query($sql,$db) or die("ERROR: " . mysql_error()); } else { $debugTxt .= " - Is empty\n" } } } else { $debugTxt .= "preg_match(/^Q/) failed\n"; } } echo "<pre>$debugTxt</pre>"; ?> Also, I was going to make a change for the branch of code for processing arrays. You should not be running queries in loops. Instead, get allt he records to add and run a single query. But, I dodn't want to change the core logic of your code until you have a change to find the cause. -
I can select one keyword, what about multiple?
Psycho replied to brown2005's topic in PHP Coding Help
Oh, hell I totally screwed up my understanding of what you were asking for. My code will not do what you want. You probably should use FULL_TEXT searching. BUt, if not, you don't want to be joining the table on itself an indefinite number of times. Instead, dynamically create multiple AND conditions for the WHERE clause if($keyword !== "") { //Explode the keywords on spaces $keywordsAry = explode(' ', $keyword); //Remove empty array items array_filter($keywordsAry); //Format AND conditions for each keyword foreach($keywordsAry as $key => $value) { $keywordsAry[$key] = "keywords_keyword = '{$value}'"; } //Create combined keyword AND conditions from keywords array $keywordANDs = implode("\n AND ", $keywordsAry); $query = "SELECT * FROM domains, domains_keywords, keywords WHERE domains_keywords_domain = domains_id AND domains_keywords_keyword = keywords_id' AND {$keywordANDs}"; $get = mysql_query($query); } In your example above the query would look something like SELECT * FROM domains, domains_keywords, keywords WHERE domains_keywords_domain = domains_id AND domains_keywords_keyword = keywords_id' AND keywords_keyword = 'cabbage' AND keywords_keyword = 'brussel' -
Yes, but that line would produce the warning "Notice: Undefined index: id ". That is why I set values using the ternary operator with an isset condition a sshown above. Ah, right you are. But, as stated by the OP himself the problem was encountered when the GET value isn't set. So, the test should logically be done on whether the GET value is set. That is why I would set the local variable using isset() as either the passed value or as boolean false. Then test the logical value of the variable.
-
Not to offend, but there is a problem with that code. It wipes out the user input when you change the selection. I would hate to be a user who selects "24", enter all my values, and then decide I only need 22 only to have all my inputs wiped out. Since the maximum allowed is 24, I would generate ALL of the inputs when the page loads and use CSS to display/hide the options. The other benefit with this approach is that it will still work even if the user has JS disabled - they will just have all the fields available to them. BUt your processing logic should be smart enough not to process empty values. Also, instead of giving all the fields different names such as "thumbnail1", "thumbnail2", etc. you can give them all the same name that will be interpreted as an array by the receiving page "thumbnail[]". Additionally, you don't need to include the MAX_FILE_SIZE field multiple times. I would also only include the description about the Size and format once since it applies to all images. And the size in the file input fields is for the physical size of the field on the page - not the file size. Here is the approach I would take <script type="text/javascript"> <!-- function displayFields(selectVal) { var inputCount = parseInt(selectVal); var fieldIdx = 1; while(document.getElementById('thm_'+fieldIdx)) { document.getElementById('thm_'+fieldIdx).style.display = (fieldIdx<=inputCount) ? 'inline' : 'none' ; fieldIdx++; } } window.onload = init; function init() { displayFields(2); } //--> </script> <label> Number of Thumbnails </label> <select name="numberofthumbnails" onChange="displayFields(this.options[this.selectedIndex].value);"> <option value="2">2</option> <option value="4">4</option> <option value="6">6</option> <option value="8">8</option> <option value="10">10</option> <option value="12">12</option> <option value="14"">14</option> <option value="16">16</option> <option value="18">18</option> <option value="20">20</option> <option value="22">22</option> <option value="24">24</option> </select> <br><br> <span id="formInputs"> <input type="hidden" name="MAX_FILE_SIZE" value="10000000" /> <b>Allowed thumbnail specs: Size 200px x 430px, Format: PNG</b><br> <span id="thm_1"><label>Thumbnail 1</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_2"><label>Thumbnail 2</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_3"><label>Thumbnail 3</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_4"><label>Thumbnail 4</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_5"><label>Thumbnail 5</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_6"><label>Thumbnail 6</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_7"><label>Thumbnail 7</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_8"><label>Thumbnail 8</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_9"><label>Thumbnail 9</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_10"><label>Thumbnail 10</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_11"><label>Thumbnail 11</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_12"><label>Thumbnail 12</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_13"><label>Thumbnail 13</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_14"><label>Thumbnail 14</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_15"><label>Thumbnail 15</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_16"><label>Thumbnail 16</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_17"><label>Thumbnail 17</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_18"><label>Thumbnail 18</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_19"><label>Thumbnail 19</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_20"><label>Thumbnail 20</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_21"><label>Thumbnail 21</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_22"><label>Thumbnail 22</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_23"><label>Thumbnail 23</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> <span id="thm_24"><label>Thumbnail 24</label> <input type="file" name="thumbnail[]" accept="image/x-png" class="File"><br></span> </span>
-
Don't use $var!="", or empty. Instead you should be using isset(). While the other two methods will work, they are not technically correct. If you were to turn error reporting on to display all errors and warning you would see that using $var!="" or empty($var) will both generate errors because you are trying to compare a variable to something and the variable doesn't exist. I typically do something such as this: $id = isset($_GET['id']) ? trim($_GET['id']) : false; Then if($id!==false) { //Id was set do something with it } else { //Id was not set, do something else } Note the use of !== with the double equal signs. If I just used ! and one of the possible IDs is '0' that would incorrectly resolve for false for that condition.
-
I can select one keyword, what about multiple?
Psycho replied to brown2005's topic in PHP Coding Help
There's no need for an else statemetn, change the condition logic. Then, if you don't need FULL_TEXT searching, then just parse the input into words and use the IN comparison in your function. if($keyword !== "") { //Explode the keywords on spaces $keywordsAry = explode(' ', $keyword); //Remove empty array items array_filter($keywordsAry); //Create keyword string with single quotes around values $keywordsStr = "'" . implode("', '", $keywordsAry) . "'"; $query = "SELECT * FROM domains, domains_keywords, keywords WHERE domains_keywords_domain = domains_id AND domains_keywords_keyword = keywords_id' AND keywords_keyword IN ({$keywordsStr})"; $get = mysql_query($query); } -
The manual offers some sample code you can add which will use strip_slashes on all your user submitted data ONLY if the server has magic quotes turned on. In the interest of portability you should use that instead. Otherwise, if you move your code to another server or the settings are changed on your current server the strip_slashes will remove content that it shouldn't. Here is the page withthe code to programatically remove magic quotes if used: http://www.php.net/manual/en/security.magicquotes.disabling.php Implement that in any page that takes user submitted data. If you have a page that is included in all pages (which I always do) include the code in there. Then your code just needs to look like this: mysql_connect("localhost", "xxxxxxxx", "xxxxxxxxxxxx") or die(mysql_error()); mysql_select_db("xxxxxxxxxxxxx") or die(mysql_error()); $title = mysql_real_escape_string(trim($_POST['title'])); $content = mysql_real_escape_string(trim($_POST['content'])); $what_id = (int) $_POST['what_id']; mysql_query("UPDATE homepage SET title='$title', content='$content' WHERE id = '1'") or die(mysql_error()); include 'updatedhyperlink1.php'; Also, be sure to validate/cleanse ALL user input. I assumed that "what_id" would be an integer, so I used (int) to force it to be an int even if the user somehow submitted anything else.
-
Take a cloase look at your code! You are first defining $title and $content suing strip_slashes() and trim() on the POST values. Then you are redefining those variables using mysql_real_escape_string() again on the POST values. So you just lost anything you had with trim() and strip_slashes().
-
Could you provide more information? I go to the trouble of writing code for you and you simply state you couldn't get it to work. You don't mention if there were errors, if you validated the data retrieved from the query or offer anything that would help me to help you. I stated it was pseudo code - not meant to be actual working code for you to just copy/paste. It was meant to guide you on the logic you could implement to get the result you want.
-
You have a typo. You named the array $categories, but in the in_array() function you are using $categories. But, if you plan to skip those values anyway, why add them to the array to begin with. Add your logic to the foreach loop so it doesn't add them to $categories to begin with.
-
First of all, why are you posting the entire page and why did you not enclose it in CODE tags [Thanks Maq]? Second, what debugging have you done to narrow down the problem and/or rule out certaint hings and what were the results? I have no problem helping people, but it is nice to see that they have at least put forth some effort. There are several include files in that script so how do you know none of them are creating the duplicate or that you don't have a recursiv loop some where? The first thing I would do is change that UPDATE query to mysql_query("UPDATE articles SET `article_views` = `article_views`+ 0 WHERE idarticles='$id'"); Then if the counter goes up by 1 then you kow the problem is some other code is incrementing the counter. If it doesn't then you know the UPDATE query is being run twice. SInce it doesn't appear to be in a loop I would guess the page is being called twice. I do notice that you seem to be calling the exact same SELECT query on the page: $query = "SELECT * FROM articles WHERE idarticles= {$_GET['id']}"; There should be no reason for that. I woudn't think that has anything to do with this specific problem, but may indicate that the logic is to blame.
-
One reason is because parens have special significance within regular expressions and that code is not interpreting those parens as parens. You will need to escape them. $words = preg_replace("/\([0-9]+\)/", "", $words);
-
As stated before, I cannot really offer any suggestions that I think are appropriate for your situation wiothout doing the analysis work. And, to be honest, I think I have invested way more time into this post than I should. To answer your question simply, I would put all materials into a single materials table. If som material requires additional parameters, I would proabably put those parameters into a separate table (material_parameters?). That table would list the parameters along with the appropriate values. Plus, each parameter may have different types of inputs (select list, radio group, etc.). So, in effect, you would need to build dynamic form logic. Plus, when saving a quote you would want to store the selected parameters in a separate table as well.
-
Think about the security risks of what you are asking if it were that simple to allow someone to edit files on a remote server. A couple of options are you could create a process on the external server to accept requests to update that file or you could programatically download the file via FTP, modify it, then re-upload it via FTP again.
-
Instead of building an inefficient while() loop, just use the function that PHP already provides! array_rand() takes a second, optional parameter for the number of random values to get. $links = array_rand($cds, 4); $links will then old the random keys that you can use to reference the values of the links