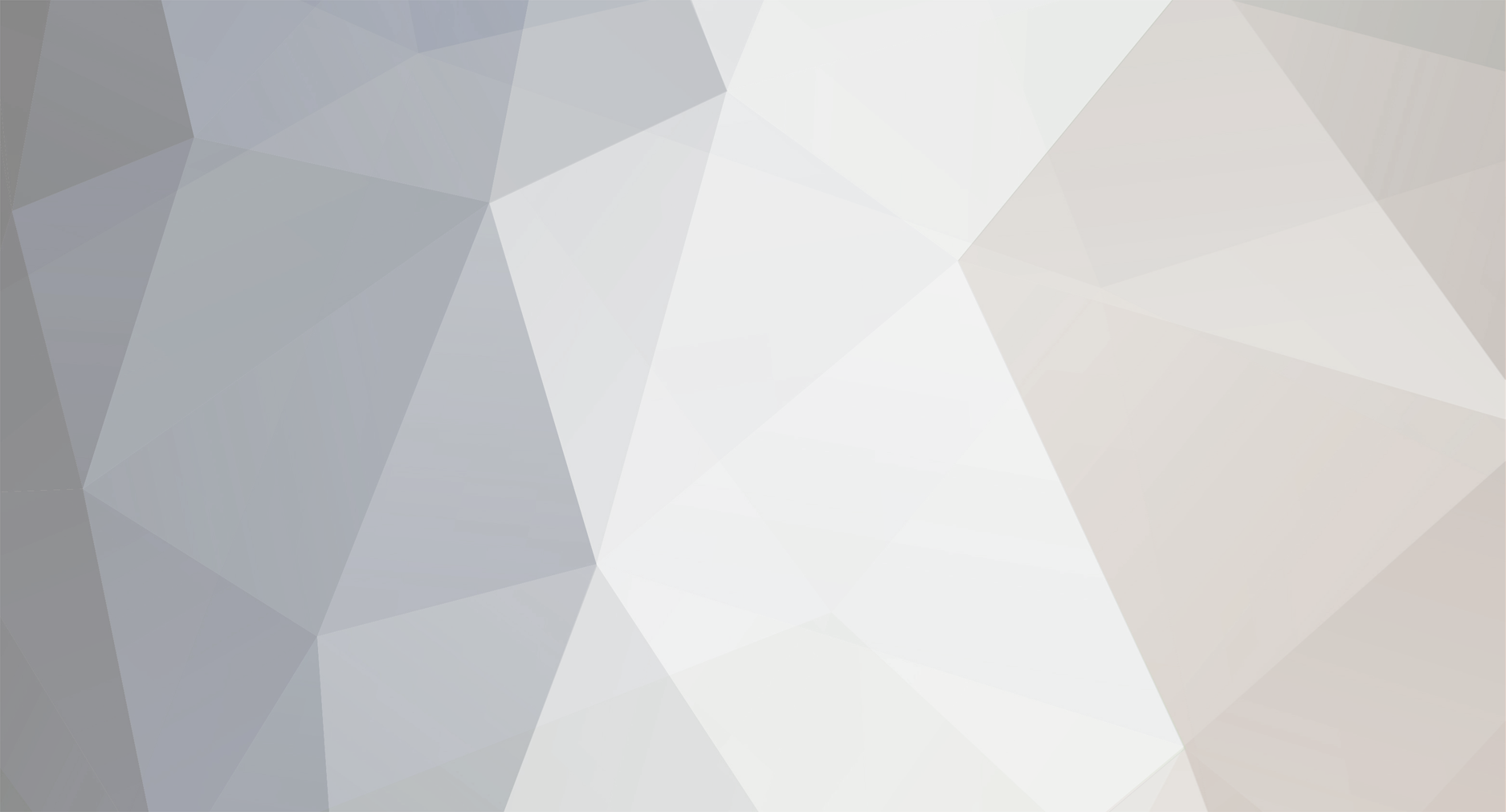
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
You don't need to include it in a call to the method. Since it is previously defined you can call it IN the method using $this->toDownloadFileName Example: The Call [ echo $testar_classe->uncompressZipFile('GeoLiteCity_20101201.zip', './'); The method public function uncompressZipFile ($fileNameToUncompress, $extractTo) { // Criar a instancia $zip = new ZipArchive; // Abrir $open_file = $this->toDownloadFileName . $zip->open($fileNameToUncompress); // Extrair para $extract_file_to = $zip->extractTo($extractTo);
-
Var = while loop?--Need help with best method
Psycho replied to RopeADope's topic in PHP Coding Help
Well, although I like having elegant code that can work as you change the parameters, you will lose the ability to perform the appropriate validation logic based upon the field types: String, int, date, etc. There are ways you could implement coe that requires little setup but still allows you to validate efficiently. For instance you could set up a list for each form. The list would include each field name and type and you could use that list to populate each form as well as validate and perform the inserts. But, taking the problem as you have just stated it, this is the approach you could take. First, name your fields as an array - BUT - use the database field name as the index. Example Form: <input type="hidden" name="table" value="someTable" /> Activity: <input type="text" name="fields['activity']" /> Hours: <input type="text" name="fields['hours']" /> Description: <input type="text" name="fields['descr']" /> Then in the processing code, do something similar to this: //Retrieve table name $table_name = mysql_real_escape_string(trim($_POST['table'])); //Parse all "fields" for db insert $fields_ary = array(); $values_ary = array(); foreach($_POST['fields'] as $field => $value) { $fields_ary[] = "`" . mysql_real_escape_string(trim($field)) . "`"; $values_ary[] = "'" . mysql_real_escape_string(trim($value)) . "'"; } //Create the query $fields_str = implode(',', $fields_ary); $values_str = implode(',', $values_ary); $query = "INSERT INTO `{$table_name}` ({$fields_str}) VALUES ({$values_str})"; -
Var = while loop?--Need help with best method
Psycho replied to RopeADope's topic in PHP Coding Help
You don't want to give all the inputs the name "values[]" based upon the form you posted. The original PHP code you posted appeared to suggest you were submitting multiple values of the same logical entity "type". (e.g. submitting a list of book titles). However, the form you show has mutiple unique data types. You should keep the fields with their descriptive names and change your PHP processing code to actually use those name. The whole point of allowing you to put a name for values is so that you can reference them in a logical coherant way. That is why you shouldn't just do a foreach() loop on the POST data. So, change your PHP code to something like this: $date = (isset($_POST['date'])) ? trim($_POST['date']) : ''; $activity = (isset($_POST['activity'])) ? trim($_POST['date']) : ''; $hours = (isset($_POST['hours'])) ? trim($_POST['date']) : ''; $descr = (isset($_POST['ddescription'])) ? trim($_POST['date']) : ''; //Perform any needed validation or data conversions (e.g. mysql_real_escape_string()) //If validation passes import into db $query = "INSERT INTO tableName ('date', 'activity', 'hours', 'description') VALUES ('$date', '$activity', '$hours', '$descr')"; mysql_query($query); -
Definitely agree with that approach, but... You have a typo. The field names are "checkbox[]", but the PHP code is referencing them as $_POST['checkboxes']. Besides, naming a field "checkbox" (or "checkboxes") is poor implementation. Always give elements/variables descriptive names. Also, I am hyper-sensitive to preventing errors. The code above uses 'mysql_real_escape_string', but the values passed "should" be integers. If a non-integer is passed the query would fail. You could either do more intensive validating of the values, or just use intval() to ensure the values are interpreted as integers. Definitely several options you could go with here. Updated/corrected: <input type="checkbox" name="delete_ids[]" value="1"/> <input type="checkbox" name="delete_ids[]" value="2"/> <input type="checkbox" name="delete_ids[]" value="3"/> $values = implode(',', array_map('intval', $_POST['delete_ids'])); $query = "DELETE FROM table_name WHERE id IN ($values)";
-
Var = while loop?--Need help with best method
Psycho replied to RopeADope's topic in PHP Coding Help
By adding the [] to the variable names, the values are passed to the PHP code as an array. As I was trying to say, using a loop over ALL post fields is just a bad idea. This way, have one field for the table name and then use multiple fields (with the same name) as the values. Variables in double quoted stings are interpreted by the PHP parser. The {} helps to avoid problems in the interpretation if there are other characters next to the variable that could cause problems. Especially useful when referencing arrays within a string. I just make it a habit. http://www.php.net/manual/en/language.types.string.php#language.types.string.parsing -
Var = while loop?--Need help with best method
Psycho replied to RopeADope's topic in PHP Coding Help
First, I would recommend referencing your POST variables by name. If you ever need another field int he POST data that should not be part of your query you will have a difficult time implementing it with what you have. You can give all the fields used for your query the same name as an array to begin with: Table: <input type="text" name="table" /> Value 1: <input type="text" name="values[]" /> Value 2: <input type="text" name="values[]" /> etc... Then you can reference all the fields needed for your query using a foreach on $_POST['values'] Anyway, no matter how you get your values in an array, creating a single query is fairly straitforward. $sql_values = array(); foreach($_POST['values'] as $value) { $sql_values[] = "('" . mysql_real_escape_string($value) . "')"; } //Create complete insert query $table = mysql_real_escape_string($_POST['table']); $query = "INSERT INTO {$table} VALUES " . implode(', ', $sql_values); -
I would also suggest that you should not be using htmlentities on your data before storing it in the database. IMO, you should always maintain data in it's original state when storing it in the database (except for using something such as mysql_real_escape_string() to prevent injection attacks). If you ever need to allow the user to modify the input you then need to try and revert the code back to it's original format which is not easy to do. I always try to store the original data and then determine what modifications, if any, need to be used during runtime based upon the context of where the data is being used.
-
How to Valid Phone Numbers when NO Spaces between Digits
Psycho replied to n1concepts's topic in PHP Coding Help
You are making it harder than it needs to be. Simply use regex to remove all non-numeric characters and then determine if there are exactly 10 digits. You can then format the phone number as you wish in a consistent format. function validPhone($phone) { $phone = preg_replace("#[^\d]#", '', $phone); if(strlen($phone) != 10) { return false; } return '('.substr($phone, 0, 3).') '.substr($phone, 3, 3).'-'.substr($phone, 6); } validPhone('1234567890') //Output: (123) 456-7890 validPhone('123-456-7890') //Output: (123) 456-7890 validPhone('123.456.7890') //Output: (123) 456-7890 validPhone('(123) 456-7890') //Output: (123) 456-7890 validPhone('123456789') //Output: false validPhone('123-4567') //Output: false -
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=318036.0
-
I *think* this is what you really want to do if(isset($_GET['intro']) || isset($_GET['port']) || isset($_GET['about']) || isset($_GET['contact'])) { echo "<img src='graphics/left-a.png'>"; } else { echo "<img src='graphics/leftb.png'>"; }
-
Since I don't have your DB to test against I can't run the code to debug it. The thing is that code is NOT complicated. In fact it is more logical than what you had previously provided. It will also make it easier for you to debug. THe comment "//Generate the search results" was just a comment to explain the following code - which you should always be doing in your code. Did you even look at the code that follows the comment? At least look at the code I provided to see if you can understand what it is doing. Anyway, I do see a couple of errors without having to run the code. Replace the function to create the options with this //Create the options for select lists function createOptions($optionsQuery) { $result = mysql_query(optionsQuery); $options = '<option value="select" selected="" >-SHOW ALL-</option>'; while($row=mysql_fetch_assoc($result)) { $options .= "<option value=\"{$row['id']}\">{$row['value']}</option>\n"; } return $options; }
-
I decided not to try and decypher your logic and instead rewote the entire script with a more logical flow. I suggest always trying to put the logic (that generates dynamic content) at the top of your page and just use PHP within the HTML to output the results of the logic. I rewrote all of this on-the-fly without any testing, so there might be some syntax errors <?php include("includes/dbconnect120-gem.php"); include("includes/db_auth_bits.php"); include("includes/db_stp.php"); $search_results = ''; if(isset($_POST)) { //Generate the WHERE clause $WHERE_PARTS = array(); if(isset($_POST['name']) && $_POST['name']!='') { $search_name = mysql_real_escape_string(trim($_POST['name'])); $WHERE_PARTS[] = "r.pname_id = '$search_name'"; } if(isset($_POST['comp']) && $_POST['comp']!='') { $search_comp = mysql_real_escape_string(trim($_POST['comp'])); $WHERE_PARTS[] = "r.comp_id = '$search_comp'"; } if(isset($_POST['year']) && $_POST['year']!='') { $search_year = mysql_real_escape_string(trim($_POST['year'])); $WHERE_PARTS[] = "r.year_id = '$search_year'"; } $WHERE_CLAUSE = (count($WHERE_PARTS)>0) ? 'WHERE ' . implode(' AND ', $WHERE_PARTS) : ''; //Generate the search results $sql = "SELECT p.pname, i.comp, m.place_name, yr.year_full FROM intcomp_result r LEFT JOIN playername p ON r.pname_id=p.name_id LEFT JOIN intcomp i ON i.comp_id = r.comp_id LEFT JOIN place m ON m.place_id = r.place_id LEFT JOIN yearname yr ON r.year_id = yr.year_id {$WHERE_CLAUSE} ORDER BY r.year_id desc, r.comp_id, r.place_id"; $search_result = mysql_query($sql); while($row = mysql_fetch_assoc($search_result)) { $search_results .= "{$row['pname']} - {$row['comp']} - {$row['year_full']} - {$row['place_name']}<br />\n"; } } //Create the options for select lists function createOptions($optionsQuery) { $result = mysql_query(optionsQuery); $options = '<option value="select" selected="" >-SHOW ALL-</option>'; while($row=mysql_fetch_assoc($result)) { $options .= "<option value=\"{$row['id']}">{$row['value']}</option>\n"; } } $names_query = "SELECT distinct r.pname_id as id, p.pname as value FROM intcomp_result r LEFT JOIN playername p ON r.pname_id=p.name_id ORDER BY p.pname"; $names_options = createOptions($names_query); $comps_query = "SELECT comp_id as id, comp as value FROM intcomp ORDER BY comp_id ASC"; $comps_options = createOptions($comps_query); $years_query = "SELECT distinct r.year_id as id, y.year_full as value FROM intcomp_result r LEFT JOIN yearname y ON r.year_id = y.year_id ORDER BY y.year_id $years_options = createOptions($years_query); ?> <html> <head></head> <body> <form method="post" action="<?php echo $PHP_SELF;?>"> Name: <select name="name"> <?php echo $name_options; ?> </select><br /> Competition: <select name="comp"> <?php echo $comps_options; ?> </select><br /> Year:<select name="year"> <?php echo $comps_options; ?> </select><br /> <input name="Submit" type="submit" class="button" tabindex="14" value="Submit" /> </form> <?php echo $search_results; ?> </body> </html> Also, I think there might be some inneficiency in your queries but didn't take the time to really review those.
-
Can you be more specific please? How does it work or not work?
-
Well, the values will need to be EXACTLY the same for in_array() to return true. Other than that the logic looks correct. However, the HTML is not formatted correctly. For one, there is no space after the closing quote of the value and to correctly check a field you should use checked="checked". BUt, that doesn't seem to be the cuase of the issue you are describing. This code is a little more efficient. If you still have problems with this code post the contents of the arrays for further help foreach($DB_Seconday_muscles as $value) { $checked = (in_array($value, $user_body_group)) ? ' checked="checked"' : ''; echo "<input name=\"colors[]\" type=\"checkbox\" value=\"{$value}\"{$checked}> {$value} \n"; }
-
What I mean is that if you are returning the results to an AJAX query, then the data will need to be in the format that you want to use in the jquery code to display on the page. For example, you could have the PHP code format the error message to display in red echo "<span style="color:#ff0000;">Error message here</span>"; Or you might send the data to return multiple parameters that are deliniated in some fashion. For example, you might want to return a true/false along with an appropriate message. Then use the javascript code to pull apart the individual parameters and handle accordingly in the javascript, such as enabling/diabling buttons, or whatever echo "1|Query executed succesfully"; echo "0|Query failed due to db error"; etc.
-
I can help with the server-side code, but since I don't know what format to return the error, I'll leave it to you to coordinate the logic with your AJAX response. Anyway, here is a rough example: $characterIDs = explode(',', $_POST['characterIDList']); $defaultcharid = (int) $characterIDs[0]; $query = "SELECT FROM `handlers` WHERE default_character_id = '$defaultcharid'"; $result = mysql_query($query); if(!$result) { echo "Error running query (1)."; } elseif(mysql_num_rows($result)!==0) { echo "That default character already exists."; } else { $query = "INSERT INTO `handlers` (username, password, firstname, lastname, email, status_id, isadmin, default_character_id, creator_id, datecreated) VALUES ('$username','$password','$firstname','$lastname','$email', '$status','$admin', '$defaultcharid', 1, NOW())"; $result = mysql_query($query); if(!$result) { echo "Error running query (2)."; } else { echo "New default character ID saved."; } }
-
Firebug only works client side, so it isn't going to provide much help in determining a server-side issue. You should echo the server-side variables you are having problems with. First, your post processing appears to have some unneccessary code. There is a foreach loop that is continually redefining the variable $id. That loop has no purpose since you aren't even using the value. However, you are trying to use the first value in the array and convertning it to an int. But, you need to be sure that there is a legitimate value that can be converted to an int. I also see that you "seem" to have the admin and default_character_id fields/values switched between the field list and the values list in the query. That is probably the problem. That is why I always write my code with appropriate line breaks and spacing to enhance readability of the code. Give this a try: $characterIDs = explode(',', $_POST['characterIDList']); $defaultcharid = (int) $characterIDs[0]; $query = "INSERT INTO `handlers` (username, password, firstname, lastname, email, status_id, isadmin, default_character_id, creator_id, datecreated) VALUES ('$username','$password','$firstname','$lastname','$email', '$status','$admin', '$defaultcharid', 1, NOW())"; echo "<b>Debugging info:</b><br />\n"; echo "POST['characterIDList']: {$_POST['characterIDList']}<br />\n" echo "defaultcharid: {$defaultcharid}<br />\n" echo "Query: {$query}<br />\n";
-
You have not provided enough information. How are you determining how many points to subtract/ There may be a way to do it with a single query. For example, you shouldn't even be using a loop to calculate the total $fq = "SELECT SUM(points) as total FROM `files` WHERE owner='$username' AND active=1 GROUP BY owner"; $fw = $sql->query($fq); $reward = 0; $fr = $fw->fetch_assoc(); $total = $fr['total'];
-
list mysql results in an array for easy call back
Psycho replied to Sleeper's topic in PHP Coding Help
I assume the code you posted is probably just example code, but it is flawed while($row = mysql_fetch_array($users)) { $username[$i] = $row[username]; $fontcolor[$i] = $row[fontcolor]; } One, $i is not defined or incremented Two, you are not enclosing the indexes (i.e. db field names) in quotes. Yes, it will wor, but it is not efficient. I will also add that, most likely, you should not need (or want) to do what you are asking. Unless you need to process the database results multiple times, dumping the data into an array only increases the overhead. Some new programmers have difficulty in efficiently working with database results and fall back to using arrays. But, if you really need to use an array, here is how I would handle it while($row = mysql_fetch_array($users)) { $result[] = $row; } You could then reference the third fontcolor using $results[2]['fontcolor'] -
That's because you don't use JavaScript in PHP. Nothing you are asking for requires JavaScript. In any event, this forum is for people who want "help" with code they have written. I think you should be posting in the freelance forum.
-
You really haven't clarified the EXACT problem. What specific problem are you having. Are you getting errors? What?
-
Your query is failing - uncomment the "or die()" code to find out what the db error is.
-
Not sure what you want. Do you want the variable to be interpreted? If so, use $y="\\{$GLOBALARRAY} = array( \n"; If not, use single quotes to define the string
-
Why would you do that? I suggest always keeping your data in it's "raw" state. If you ever need to use that data as HTML code you will have a harder time converting that code back. A better solution, IMHO, would be to simply use htmlspecialchars() or htmlentities() to convert the text on-the-fly to replace the necessary characters.
-
I really don't understand what the problem is based upon your description. But, looking at the code I think I know what the problem is. After you run the query and define the array $list, you then run a foreach loop on $list to output the records. But, inside that loop you are echoing $year and $month. Those variables were assinged values on each iterration of the while loop. So, when it comes time to run the foreach loop $month and $year will always display the last values defined in the previous while loop. You could solve this by modifying your foreach loops like this: foreach($list as $year => $years) foreach($months as $month => $item) But a better solution would be to revise your query and only process the records once $query = "SELECT title, id, dateColumn FROM theTable ORDER BY dateColumn ASC"; $result = mysql_query($query); $current_year = false; $current_month = false; while($row = mysql_fetch_assoc($result) ) { $year = date("Y", $row['dateColumn']); $month = date("m", $row['dateColumn']); if($current_year != $year) { echo "$year : "; } if($current_month != $month || $current_year != $year) { echo "$month : "; } $current_year = $year; $current_month = $month; echo "<a href=\"site address by {$row['id']}\">{$row['title']}</a>"; }