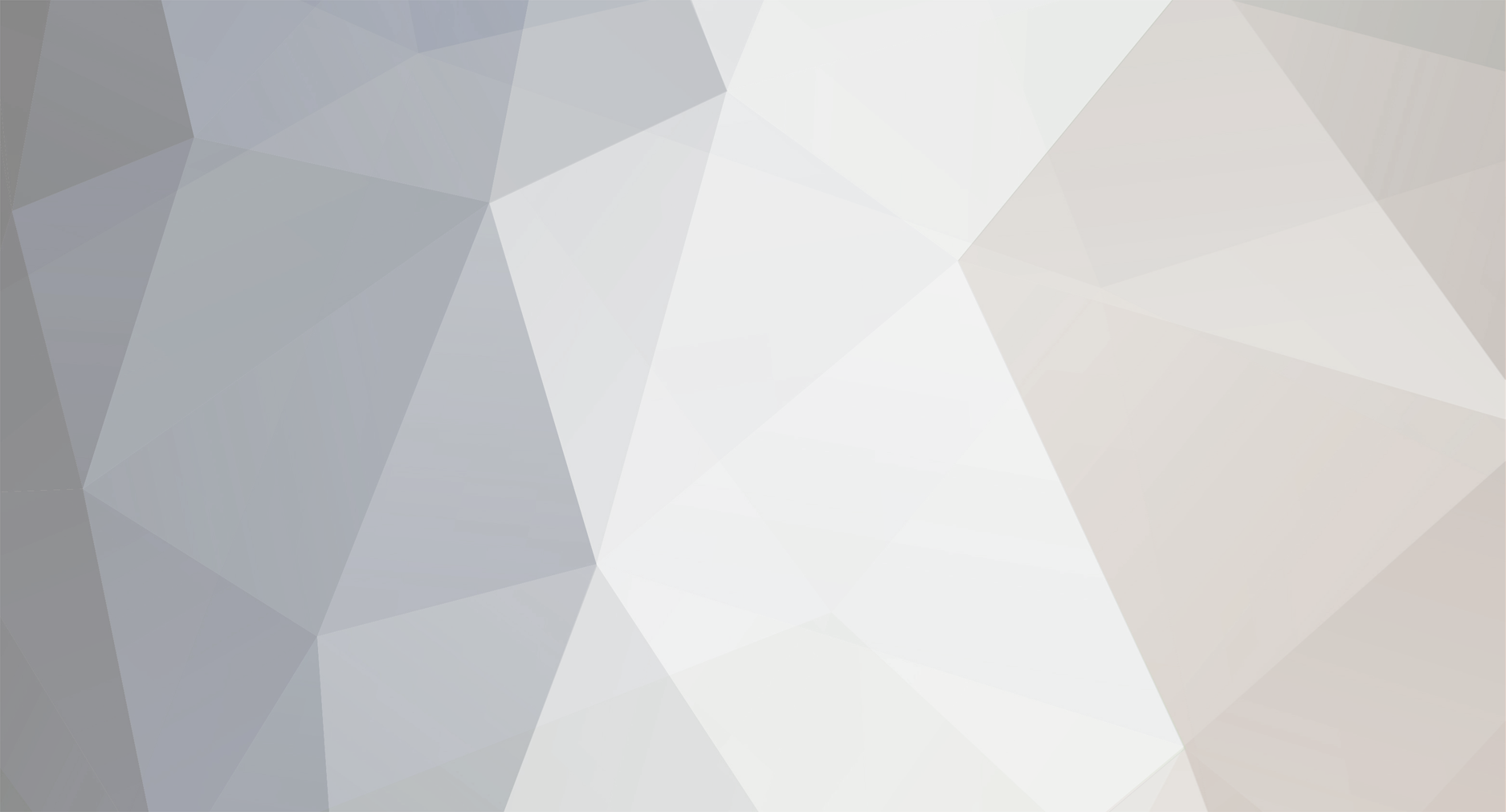
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I already provided an answer to help you solve this yourself. There are plenty of tutorials out there on how to implement cURL to do what you need. I see no reason to provide a piece of code that may not meet your specific needs requiring many back and forth posts to get it exactly right. If you read (and follow) a tutorial on the subject you will understand exactly what the process does and how to implement it for your specific needs. http://coderscult.com/php/php-curl/2008/05/20/php-curl-tutorial-and-example/
-
The problem is in the javascript, but the javascript doesn't have much meaning without the HTML form to put it into context. Try this <html> <head> <script type="text/javascript"> function getIntValue(fieldObj) { if(isNaN(parseInt(fieldObj.value))) { fieldObj.value = ''; return 0; } return parseInt(fieldObj.value); } function reCalcType(typeName) { var qty = getIntValue(document.getElementById('credit_'+typeName+'_num')); var amt = getIntValue(document.getElementById('credit_'+typeName+'_value')); document.getElementById('credit_'+typeName+'_total').value = (amt * qty); reCalcTotal(); return; } function reCalcTotal(fieldObj) { //Calc subtotal var pp_total = getIntValue(document.getElementById('credit_pp_total')); var pr_total = getIntValue(document.getElementById('credit_pr_total')); var jobs_total = getIntValue(document.getElementById('credit_jobs_total')); var event_total = getIntValue(document.getElementById('credit_event_total')); var subTotal = (pp_total + pr_total + jobs_total + event_total); document.getElementById('subtotal').value = subTotal; //Calc total var disc = getIntValue(document.getElementById('discount')); var vat = getIntValue(document.getElementById('discount')); document.getElementById('total').value = (subTotal - (subTotal * (disc/100) * (1+(vat/100)) ) ); return; } function tt(fieldObj) { alert(fieldObj.value*2); } </script> </head> <body> <form action="" method="post"> <input type="hidden" name="creditRecordAdded" value="<?php echo $client_id; ?>"> <table border="1" width="100%"> <tr><td width="25%">Order Date </td><td><input type="text" name="credit_order_date" size="30"></td></tr> <tr><td>Order Number </td><td><input type="text" name="credit_order_num" size="30"></td></tr> <tr><td>Billing Info </td><td><textarea name="credit_bill_info" cols="50" rows="2"></textarea></td></tr> <tr> <td colspan="2"> <table width="98%" align="center" border="1"> <tr><td colspan="4"><b>Package</b></td></tr> <tr> <td colspan="2">Start Date : <input type="text" name="credit_start_date" size="10"></td> <td colspan="2">End Date : <input type="text" name="credit_end_date" size="10"></td> </tr> <tr> <td>PP</td> <td><input type="text" name="credit_pp_num" size="4" onChange="reCalcType('pp')" /></td> <td>@ <input type="text" name="credit_pp_value" size="10" onChange="reCalcType('pp')" /></td> <td> = <input type="text" name="credit_pp_total" size="10" id="credit_pp_total" disabled="disabled" /></td> </tr> <tr> <td>PR</td><td><input type="text" name="credit_pr_num" size="4" onChange="reCalcType('pr')" /></td> <td>@ <input type="text" name="credit_pr_value" size="10" onChange="reCalcType('pr')" /></td> <td> = <input type="text" name="credit_pr_total" size="10" id="credit_pr_total" disabled="disabled" /></td> </tr> <tr> <td>Jobs</td><td><input type="text" name="credit_jobs_num" size="4" onChange="reCalcType('jobs')" /></td> <td>@ <input type="text" name="credit_jobs_value" size="10" onChange="reCalcType('jobs')" /></td> <td> = <input type="text" name="credit_jobs_total" size="10" id="credit_jobs_total" disabled="disabled" /></td> </tr> <tr> <td>Events</td><td><input type="text" name="credit_event_num" size="4" onChange="reCalcType('event')" /></td> <td>@ <input type="text" name="credit_event_value" size="10" onChange="reCalcType('event')" /></td> <td> = <input type="text" name="credit_event_total" size="10" id="credit_event_total" disabled="disabled" /></td> </tr> </table> </td> </tr> <tr> <td>Subtotal</td> <td><input type="text" name="credit_subtotal" id="subtotal" size="30" onChange="reCalcTotal()" disabled="disabled" /></td> </tr> <tr> <td>Discount (%) </td><td><input type="text" name="credit_discount" id="discount" size="30" onChange="reCalcTotal()" /></td></tr> <tr> <td>VAT (%) </td> <td><input type="text" name="credit_vat" id="vat" size="30" value="17.5" onChange="reCalcTotal()" /></td> </tr> <tr> <td>Total </td> <td><input type="text" name="credit_total" id="total" size="30"disabled="disabled" /></td> </tr> <tr> <td>Invoice Date</td> <td><input type="text" name="credit_inv_date" size="30"></td> </tr> <tr> <td>Invoice Number </td> <td><input type="text" name="credit_inv_no" size="30"></td> </tr> <tr> <td>Paid Date</td> <td><input type="text" name="credit_paid_date" size="30"></td> </tr> <tr> <td>Contact </td> <td><input type="text" name="credit_contact" size="30"></td> </tr> <tr> <td>Contact Number</td> <td><input type="text" name="credit_contact_num" size="30"></td> </tr> <tr> <td>Contacted On</td> <td><input type="text" name="credit_contacted" size="30"></td> </tr> <tr> <td>Notes </td> <td><textarea name="credit_notes" cols="40" rows="5"></textarea></td></tr> <tr> <td colspan="2" align="center"><input type="submit" value="Add a new credit record"></td></tr> </table> </form> </body> </html>
-
Your code assumes the fields have a value. You will want to typecast the value as an integer - and if NOT a number return an appropriate value. Most likely you are getting the error NaN and the code doesn't know what to do. Since you haven't provided the HTML that invokes the script I can't tell exactly how it is invoked and I'm not going to spend time trying to backward engineer your code to figure it out. (if you posted the form I would be a simple process of copying and pasting into an HTML document).
-
Then, the function can be simplified to this function getClosestValue($array, $searchKey) { //Check if there is an exact match if(array_key_exists($searchKey, $array)) { return $array[$searchKey]; } //No exact match, find next lowest key value $nextLowest = false; //Determine next lowest keys foreach($array as $key => $value) { if($key < $searchKey) { $nextLowest = $key; } else { break; } } //Return next lowest value, if exists return ($nextLowest!==false) ? array[$nextLowest] : false; }
-
Huh? Let me give you an example. Let's say your array contains the following: $array_odds = array( "1.10" => "1/10", "1.11" => "1/9", "1.14" => "1/7" ); What value would you want returned for 1.13? Do you want "1/9", which is the closest lower value? Or, do you want "1/7" which is the closest absolute value?
-
You still didn't answer my question. You said you wanted the "closest" value, but your example returns the closest LOWER value. So, are you always wanting the lower value even if the higher value is closer? HArd to tell since your example has an upper and lower value that are the same difference from the search value. The following function will return the value of the matching key. If no matching key it will return the closest value. but, if the upper and lower keys are the same difference from the search key, it reverts to the lower key [Note: this assumes the array key values are in logical, numerical order] function getClosestValue($array, $searchKey) { //Check if there is an exact match if(array_key_exists($searchKey, $array)) { return $array[$searchKey]; } //No exact match, find nearest lowest/highest key values $nextLowest = false; $nextHighest = false; //Determine next lowest and next highest keys foreach($array as $key => $value) { if($key < $searchKey) { $nextLowest = $key; } else { $nextHighest = $key; break; } } //Determine nearest value if($nextHighest!==false && $nextLowest!==false) { //Neither value is false, return closes key value $closestDiff = min(($searchKey-$nextLowest), ($nextHighest-$searchKey)); return $array[(($closestDiff==($searchKey-$nextLowest))?$nextLowest:$nextHighest)]; } if($nextLowest===false XOR $nextLowest===false) { //One value is false return ($nextLowest!==false) ? array[$nextLowest] : array[$nextHighest]; } //Both values are false return false; }
-
The only solution I can think of is building a function that would have to iterrate through the values in the array. BUt, you state in your request "it gets me the closest fraction". Are you wanting the closest value (those are decimals not fractions) or are you wanting the closest lower value? For exampl, what if 1.12 didn't exist, but 1.14 did. Would you want the 1.11 value or the 1.14 value?
-
So, what was the problem?
-
The $i variable is unnecessay in that code. Try this: $query = "SELECT field_item_id_value FROM content_type_ads"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)) { $my_array[] = $row['field_item_id_value']; } echo "<pre>"; print_r($my_array); echo "</pre>";
-
I don't see any array_diff() in that code. $row should be an array (although it is an array with just one element based on your query), but $row['field_item_id_value'] would not. Are you sure the query is executing without errors?
-
No, the connection ends when the script finishes. You should connect to the database once, then run all needed queries. Edit: PFMaBiSmAd beat me to that response. Rather than a function, you could create a db class. Then just have a method in the class to run a function. When that method is called the class will check if a db conneciton is already open. If so, it runs the query. If not, it opens the connection before running the query. That way a db connection is only opened if needed and you only need to open it once per page. You could do the same thing with a function, but that would require using a global variable for the db connection. But, there are many db classes freely available - no need to reinvent the wheel.
-
Look into cURL.
-
Ok, I made the mistake in the code I posted to you, but you still should have caught the problem. As my signature states, I don't typically test the code I provide and I usually just write the code "on-the-fly". Anyway, textareas don't have a value parameter: <textarea name="opmerkingen" rows="5" cols="29" value="<?php echo $opmerkingen; ?>"></textarea> The value goes between the tags: <textarea name="opmerkingen" rows="5" cols="29"><?php echo $opmerkingen; ?></textarea> Note: you will need to "clean" the inputs (all of them) to protect against values that may screw up the rendered page. For example, if the user entered '<' or ", etc.
-
If the password is already encrypted (but most likely hashed) you need to know what method was used to encrypt/hash the password. The idea is that you do not store passwords in the database in plain text - otherwise someone who gains access to the database will have access to the passwords. So, you hash the password when you store it and then when the user attempts to authenticate you first hash it then compare that hashed value to the db value.
-
I've given you plenty of code to use as a base that you can then modify from. Which step? There are 10 lines of code there. It is a loop that builds the HTML output to be displayed on the page. Each loop creates a new table row. I wrote it to output the data similar to what you provided, but if you don't like then just change it to what you need. However, if you want to use list items, I'd suggest just using CSS to create columns. Take a look at this tutorial: http://www.communitymx.com/content/article.cfm?cid=27f87
-
If user ahs a foreign key to country and country has a foreign key to continent then you don't need (or want) to link the user and continent directly. Correct, makes queries much more readable.
-
Don't use "*" in your query - specify the fields you want. You need to enclose conditions in quotes. The way you have it written will bring up all records where description matches and date greater than now OR date is null What you want is description matches AND (date greater than now or date is null) SELECT * FROM products WHERE productDescription LIKE '%testProd% AND (productExpiry >= NOW() OR productExpiry IS NULL)
-
I'm betting the problem is in the file app.php. Try commenting out that include. What is in that file?
-
OK, maybe I am not understanding you then. I made the changes I specified above and the page then works for dates in the format mm-dd-yyyy. Are you wanting users to enter dates in BOTH formats? If so, what logic do you use to determine which value is the day and which is the month? For a date where the day is >12 it can be done easliy enough, but what if the date is entered as: 2-8-2010? Is that February 8th or August 2nd? I also have code that allows the user to use about any character as a delimiter (i.e. 2-15-2010 or 2.15.2010 or 2/15/2020). But, if you are going to have a problem with the user entering the month and day in different orders then I would suggest you find a javascript popup calendar to incorporate.
-
Would have been nice to see the relevant fields from the user table. I "assume" that the user record only has a foreign key back to the country table. SELECT u.field1, u.field2, u.field3, ctry.country_name cont.continent_name FROM user as u LEFT JOIN location_country as ctry ON u.country_id = ctry.country_id LEFT JOIN location_continent as cont ON ctry.continent_id = cont.continent_id
-
Change if(isset($_POST)) To if(isset($_POST['naam']))
-
I hope you are not setting the date in the query using PHP. Just use NOW() in your query. Anyway, to include records with NULL values you could do this: SELECT * FROM `products` WHERE products.productExpiry >= NOW() OR products.productExpiry IS NULL Although you may need to normalize NOW() to be midnight of the current date.
-
Did you remove the condition to check if the form was submitted? if(isset($_POST)) { //User submitted data - perform validation That IF condition only performs validation if the user has submitted the form. So, the errors should not display on first accessing the page. Yes, of course, hence the comment. I only added a few sample validations. You didn't provide any criteria on what or how you wanted validation to occur.
-
Well, you did a piss poor job of explaining what you are trying to accomplish. You stated that you wanted "the user" to select a directory. ftp_get() downloads a file from an FTP file onto the web server. If "the user" is selecting a folder to save a file using ftp_get() then you are apparently wanting the user to select a folder on the web server. I am assuming then, that "the user" is operating on the server. This is NOT the common configuration for a web application - the user is always assumed to be on a remote computer. You should have stated that the user was operating on the server and/or you wanted a remote user to select a folder on the server. Perhaps instead of criticizing people for what you perceived to be a lack of ability, you should have taken a closer look at the responses and your requests. Then you might have identified that the problem was your inability to properly explain yourself. I have an application for browsing/playing my mp3 collection and the management functionality has a feature that allows me to select the source folders for the mp3's on the server. Here is an old copy of that code, so it probably has some cleanup needed (and I have only used it on Windows, so not sure if it works on Linux). <?php session_start(); $drives_list = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'; $_DS_ = DIRECTORY_SEPARATOR; //Set the default drive $drive='C'; if(isset($_POST['drive']) && strpos($drives_list, $_POST['drive'])!==false) { $drive = substr($_POST['drive'], 0, 1); //Reset path $_SESSION['path'] = ''; } else if(isset($_SESSION['drive']) && strpos($drives_list, $_SESSION['drive'])!==false) { $drive = substr($_SESSION['drive'], 0, 1); } //Set the saved path $savedPath = (isset($_SESSION['path'])) ? $_SESSION['path'] : ''; if(isset($_GET['updirectory']) && strpos($savedPath, $_DS_)!==false) { $savedPath = substr($savedPath, 0, strrpos($savedPath, $_DS_, -2)+1); } //Set the newFolder directory $newFolder = (isset($_GET['folder'])) ? $_GET['folder'].$_DS_ : ''; //Determin the current path $path = ''; if(is_dir("{$drive}:{$_DS_}{$savedPath}{$newFolder}")) { $path = $savedPath.$newFolder; } else if (is_dir("{$drive}:{$_DS_}{$savedPath}")) { $path = $savedPath; } //Save current drive and path to session $_SESSION['drive'] = $drive; $_SESSION['path'] = $path; //Create select options of valid drives $drive_options = ''; for ($i=0; $i<strlen($drives_list); $i++) { $drive_letter = $drives_list[$i]; if (is_dir("{$drive_letter}:{$_DS_}")) { $selected = ($drive_letter==$drive)?' selected="selected"':''; $drive_options .= "<option value=\"{$drive_letter}\"{$selected}>{$drive_letter}:</option>\n"; } } //Get the current folder path $full_path = "{$drive}:{$_DS_}{$path}"; //Get the list of subfolders for the current folder $folder_list = ''; $upDirectory = "<img style=\"border:0px;\" src=\"{$_PATHS['images']}up_directory.gif\" alt=\"Up One Directory\">Up One Directory"; if($path!='') { $folder_list .= "<a href=\"{$_PATHS['current_web']}?action=browseFolder&updirectory=true\">{$upDirectory}</a><br />\n"; } else { $folder_list .= "<span style=\"font-style:italic;\">{$upDirectory}</span><br />\n"; } $folders = glob($full_path."*", GLOB_ONLYDIR); foreach($folders as $folderPath) { $folder = substr($folderPath, strrpos($folderPath, $_DS_)+1); $folderURL = rawurlencode($folder); $folder_list .= "<a href=\"{$_PATHS['current_web']}?action=browseFolder&folder={$folderURL}\">$folder</a><br />\n"; } ?> <html> <body> <div style="width:300px;"> <form name="drive_select" action="<?php echo $_PATHS['current_web'].'?action=browseFolder'; ?>" method="post"> Drive:<br> <select name="drive" style="width:300px;align:right;" onchange="this.form.submit();"> <?php echo $drive_options; ?> </select> </form> <form name="folder_select" action="<?php echo $_PATHS['current_web'].'?action=addFolder'; ?>" method="post"> Current folder: <br /> <textarea name="currentFolder" style="width:300;height:60px;background-color:#cecece;"><?php echo $full_path; ?></textarea> <br /> Select a subfolder: <br /> <div style="width:300px;height:200px;border:1px solid black;overflow:auto;padding:2px;"> <?php echo $folder_list; ?> </div> <button type="submit" name="action" style="float:right;">Cancel</button> <button type="submit" name="action" style="float:right;">Add Current Folder</button> </form> </div> </body> </html>
-
You need to stop obsessing over needing an array - at least not in the manner you are thinking. Just because there is an existing piece of code that does something similar to what you want doesn't necessarily mean you have to do it exactly how that code was written. By dumping the results into an array and then processing the array you are making the server do twice as much work as needed. Instead you just need to modify the processing script for the db results - which is very easy using the existing code you have as a model. The example below does use an array - but not how you have it above - it is only a temporary container. By the way, that code you just posted has a flaw if you wanted to use it with a multidimensional array since it wouldn't take into account the headers. I even added logic to add the alternating background colors as shown in the image you first posted. <?php //Run the query $query = 'SELECT u.name FROM users as u JOIN groups as g ON u.Group_ID = g.id JOIN simulationgroups as sg ON sg.Group_ID = g.id WHERE sg.Simulation_ID = 5 AND g.kind_of_user NOT IN (1,2) ORDER BY g.id'; $result = mysql_query($query); //Process the results into table cells $bgColors = array('#00FFFF', '#FF8040', '#FFFF00', '#FF00FF'); $groupCount = 0; $current_groupID = false; $cells = array(); while($row = mysql_fetch_assoc($result)) { if($current_groupID != $row['Group_ID']) { $current_groupID = $row['Group_ID']; $bgColor = $bgColors[$groupCount%count($bgColors)]; $groupCount++; $cells[] = " <th style=\"background-color:{$bgColor};\">Group {$current_groupID}</th>\n"; } $cells[] =" <td style=\"background-color:{$bgColor};\">{$row['name']}</td>\n"; } //Ouput into multi-column table $columns = 4; //Set the number of columns to use $tableOutput = ''; $cellsPerCol = ceil(count($cells)/$columns); for($row=0; $row<$cellsPerCol; $row++) { $tableOutput .= " <tr>\n"; for($col=0; $col<$columns; $col++) { $index = ($col*$cellsPerCol+$row); $tableOutput .= (isset($cells[$index])) ? $cells[$index] : ''; } $tableOutput .= " <tr>\n"; } ?> <html> <body> <table border="1"> <?php echo $tableOutput; ?> </table> </body> </html>