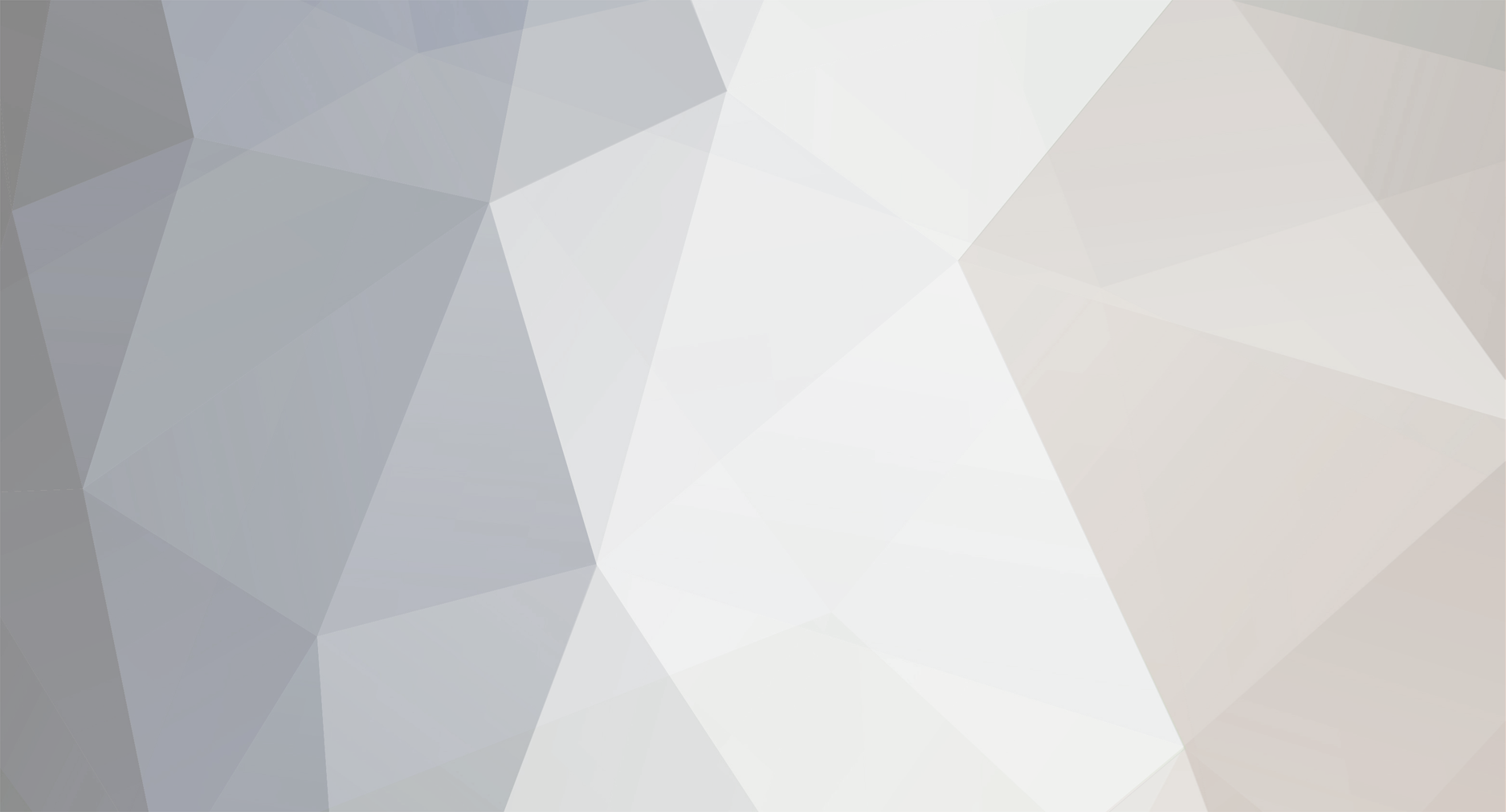
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Your form is posting to a page called "bestellen.php". Is that the form page? If not, you want the form page to post to itself. You can do this by simplyleaving the action attribute blank. Then, just add logic at the top of the page to perform the validation. If validation fails, generate the error messages and redisplay the form. If validation passes include the processing page (order.php). Here is a rewrite of the form page which will do the validation as well. I only included validation for the first three fields. Note: I used an include to add the page to process the data after validation passes, using header() would remove all the data. But, using header after processing the data (for example taking the user to a thank you page) is a great idea. That way if a user refreshes the page it won't process the data a second time. <?php //Values for select lists $productList = array( 'proefmonster' => 'Proefmonster (Gratis!)', '1kartalin' => '1x Kartalin - €30,00', '2kartalin' => '2x Kartalin - €58,00', '3kartalin' => '3x Kartalin - €80,00' ); $verzendingList = array( 'proefmonster' => 'N.v.t. bij proefmonster', 'dhl' => 'DHL (binnen 2 á 3 werkdagen) - €4,95', 'tnt' => 'TNT-Post (volgende werkdag) - €6,50' ); function createSelectOptions($optionList, $selectedValue=false) { foreach($optionList as $value=>$text) { $selected = ($value===$selectedValue) ? ' selected="selected"' : ''; echo "<option value=\"{$value}\"{$selected}>{$text}</option>\n"; } } function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } //Create initial variables $naam = (isset($_POST['naam'])) ? trim($_POST['naam']) : ''; $achternaam = (isset($_POST['achternaam'])) ? trim($_POST['achternaam']) : ''; $email = (isset($_POST['email'])) ? trim($_POST['email']) : ''; $straat = (isset($_POST['straat'])) ? trim($_POST['straat']) : ''; $huisnummer = (isset($_POST['huisnummer'])) ? trim($_POST['huisnummer']) : ''; $postcode = (isset($_POST['postcode'])) ? trim($_POST['postcode']) : ''; $plaats = (isset($_POST['plaats'])) ? trim($_POST['plaats']) : ''; $telefoon = (isset($_POST['telefoon'])) ? trim($_POST['telefoon']) : ''; $product = (isset($_POST['product'])) ? trim($_POST['product']) : ''; $verzending = (isset($_POST['verzending'])) ? trim($_POST['verzending']) : ''; $opmerkingen = (isset($_POST['opmerkingen'])) ? trim($_POST['opmerkingen']) : ''; $errorText = ''; if(isset($_POST)) { //User submitted data - perform validation $errors = array(); if(empty($naam)) { $errors[] = "Name is required."; } if(empty($achternaam)) { $errors[] = "Achternaam is required."; } if(empty($email)) { $errors[] = "Email is required."; } else if(!is_email($email)) { $errors[] = "Email is not valid."; } if(count($errors)>0) { $errorText .= "<span style=\"color:#ff0000;\">"; $errorText .= "The following errors occured:<ul>\n"; foreach($errors as $errorMsg) { $errorText .= "<li>$errorMsg</li>"; } $errorText .= "</ul><span style=\"color:#ff0000;\">"; } else { //Form validation passed include page to process the data include('order.php'); exit(); } } ?> <html> </head></head> <body> <?php echo $errorText; ?> <form action="" method="post"> <table border="0"> <tr> <td align="right">Naam:</td> <td><input name="naam" size="25" type="text" value="<?php echo $naam; ?>" /></td> </tr> <tr> <td align="right">Achternaam:</td> <td><input name="achternaam" size="25" type="text" value="<?php echo $achternaam; ?>" /></td> </tr> <tr> <td align="right">Email:</td> <td><input name="email" size="25" type="text" value="<?php echo $email; ?>" /></td> </tr> <tr> <td align="right">Straat & huisnummer:</td> <td> <input name="straat" size="16" type="text" value="<?php echo $straat; ?>" /> <input name="huisnummer" size="4" type="text" value="<?php echo $huisnummer; ?>" /> </td> </tr> <tr> <td align="right">Postcode:</td> <td><input name="postcode" size="7" type="text" value="<?php echo $postcode; ?>" /></td> </tr> <tr> <td align="right">Plaats:</td> <td><input name="plaats" size="25" type="text" value="<?php echo $plaats; ?>" /></td> </tr> <tr> <td align="right">Telefoon:</td> <td><input name="telefoon" size="12" type="text" value="<?php echo $telefoon; ?>" /></td> </tr> <tr> <td align="right">Product:</td> <td><select name="product" size="4"> <?php createSelectOptions($productList, $product); ?> </select> </td> </tr> <tr> <td align="right">Verzenden:</td> <td><select name="verzending" size="1"> <?php createSelectOptions($verzendingList, $verzending); ?> </select> </td> </tr> <tr> <td align="right">Opmerkingen:</td> <td><textarea name="opmerkingen" rows="5" cols="29" value="<?php echo $opmerkingen; ?>"></textarea></td> </tr> <tr> <td></td> <td><input name="verzenden" value="Verzenden" type="submit"> <input type="reset" value="Opnieuw"></td> </tr> </table> </form> </body> </html>
-
Seems like a lot of work when you could simply set the session timeout period to whatever value you wanted. Then just ckeck if there is an active session on each page load.
-
Once you have all the data, why would you need to get them into an array? Just process the db results into the output you want. Here is some sample code. I made some assumptions on a copuple db fields $query = 'SELECT u.name FROM users as u JOIN groups as g ON u.Group_ID = g.id JOIN simulationgroups as sg ON sg.Group_ID = g.id WHERE sg.Simulation_ID = 5 AND g.kind_of_user NOT IN (1,2) ORDER BY g.id'; $result = mysql_query($query); $current_groupID = false; while($row = mysql_fetch_assoc($result)) { if($current_groupID != $row['Group_ID']) { $current_groupID = $row['Group_ID']; echo "<h2>{$current_groupID}</h2>\n"; } echo "{$row['name']}<br />\n"; }
-
Seriously? I just rewrote that code (which originally worked for mm-dd-yyy) to work for dd-mm-yyyy as you asked. I don't have the time to review and update the code again, but I think all you need to do is change day = matchArray[1]; month = matchArray[3]; year = matchArray[5]; To month = matchArray[1]; day = matchArray[3]; year = matchArray[5]; And var dateObj = new Date(matchArray[4], matchArray[3]-1, matchArray[1], 12, 0, 0); To var dateObj = new Date(matchArray[4], matchArray[1]-1, matchArray[3], 12, 0, 0);
-
md5() is more than adequate for hashing a password. Although you should use a salt just as you should for any hashing algorithm. The "flaw" in the md5() algorithm is around the ability to knowingly create a collision. FOr the purposes of a password (which is made up of a limited range of characters and from a specific list of characters) a collission would be nearly impossible. Even if it was possible the "hacker" would have to know the md5() hash which they were trying to duplicate. The purpose of hashing a password is in protecting the password. Creating a collision does not expose the password. Plus, to create a collision, the hacker would need access to the hashes (i.e. the database). But, then there is no purpose to tryng to create a collision since the hacker already has access to all the data anyway (except the passwords which are hashed). The threat is a user who gets a copy of your database and then tries to obtain users's actual passwords. That is why you use a salt so the user cannot simply use a rainbow table. That is also why you should never use a simple word as your password since malicious users will typically start with a dictionary attack.
-
You should only be using ONE query with a JOIN statement between the two tables. Then you just need to order by group and the records will be automatically "grouped" by the group value.
-
PHP is as secure as YOU make it. The answers to your questions could (nad have) fill entire books. Trying to answer them in a forum post cannot be done in an adequate manner. There are plenty of articles out there on different aspects of security (file locaitons, database, encryption, etc.). You will need to do research on each type of security to decide how best to approach it. however, if you have questions about a specific "piece" then by all means aska question here to get answers/opinions.
-
Well, the code you have is flawed to begin with. For example: var years = Math.floor( diff / secPerYr ) That assumes that there are the same number of seconds per year. But, that is not the case with leap years and you would get inaccurate results. Here is a rewrite of what you had plus some code of mine modified for a date in the format you specified. I did some testing but not comprehensive <html><head><title>Datediff</title><script type='text/javascript'>//****************************************************************//// FUNCTION: isDate (dateStr) //// //// This function takes a string variable and verifies if it is a //// valid date or not. Dates must be in the format of dd-mm-yyyy //// or dd/mm/yyyy. It checks to make sure the month has the proper //// number of days, based on the month. The function returns true //// if a valid date, false if not. //// //// Day/Month must be 1 or 2 digits, Year must be 2 or 4 digits. ////****************************************************************//function isDate(dateStr){ var datePattern = /^(\d{1,2})(\/|-)(\d{1,2})\2(\d{2}|\d{4})$/ var matchArray = dateStr.match(datePattern); //Check valid format if (matchArray == null) { return false; } day = matchArray[1]; month = matchArray[3]; year = matchArray[5]; // check month range if (month < 1 || month > 12) { return false; } //Check day range if (day < 1 || day > 31) { return false; } //Check months with 30 days if ((month==4 || month==6 || month==9 || month==11) && day>30) { return false; } //Check Feb days if (month == 2) { var leapYr = (year%4 == 0 && (year%100 != 0 || year%400 == 0)); if (day > 29 || (day==29 && !leapYr)) { return false; } } return true;}function getDateObj(dateStr){ var datePattern = /^(\d{1,2})(\/|-)(\d{1,2})\2(\d{2}|\d{4})$/ var matchArray = dateStr.match(datePattern); var dateObj = new Date(matchArray[4], matchArray[3]-1, matchArray[1], 12, 0, 0); return dateObj;}//***************************************************************//// FUNCTION: dateDiff(Date1Obj, Date2Obj, [units], [precision]) //// //// Returns the difference between two date objects in the units //// specified (optional, default is days). The optional precision //// parameter determines the number of decimal places the result //// will be rounded to. Note: When the 'days' units is used and //// precision is 0, then output will be in calendar days. //// //// The units parameter includes the following: d=days (default), //// h = hours, m = minutes, s = seconds, ms = milliseconds ////***************************************************************//function dateDiff(date1Obj, date2Obj, units, precision){ //set the default untis var units = (units)?units:'d'; var calcPrecision = (precision)?Math.pow(10, precision) : 1; //Calculate the units divisor switch (units) { case 'ms': //Milliseconds var units = 1; break; case 's': //Seconds var units = 1000; break; case 'm': //Minutes var units = 1000 * 60; break; case 'h': //hours var units = 1000 * 60 * 60; break; case 'd': //Calendar Days default: var units = 1000 * 60 * 60 * 24; //Normalize time to 12:00am to count calendar days if precision = 0 if (precision==0) { date1Obj.setHours(0); date2Obj.setHours(0); } break; } //Convert dates to milliseconds var date1ms = date1Obj.getTime(); var date2ms = date2Obj.getTime(); //Calculate the difference in selected units var difference = (date2ms - date1ms) / units; //Convert to precision parameter difference = (Math.round(difference*calcPrecision))/calcPrecision; return difference;}function CalculateDiff(date1ID, date2ID){ var date1Val = getVal(date1ID); var date2Val = getVal(date2ID); if(!isDate(date1Val) || !isDate(date2Val)) { return false; } setVal('days', dateDiff(getDateObj(date1Val), getDateObj(date2Val))); return;}function field(id){ var ele = document.getElementById( id ); if ( !ele ) { alert( 'Element not found. id="' + id + '"' ); } return ele;}function setVal(id, val){ var ele = field(id); if (ele) { ele.innerHTML = val; }}function getVal(id){ var ele = field(id); var result = null if (ele) { result = ele.value; } return result;}</script></head><body><form name='' action=''> <br>Date 1 <input type='text' value='11/01/2006' id='date1'/> <br>Date 2 <input type='text' value='12/29/2007' id='date2'/><br> <input type='button' value='Calculate difference' onclick='CalculateDiff("date1","date2");'><br> Calculated Difference <table border='1'> <tr> <th>Days </th><td><textarea name='days' rows='1' cols='8' id='days'> </textarea></td> </tr> </table></form></body></html>
-
You are taking the wrong approach. If a product and/or sub-product can belong to multiple categories then you should use an associative table to associate a product to one or many categories. If you duplicate products (for the same product) you are going to run into serious problems at some point. For example, if you need to manage inventory it would be very difficult if you are duplicating products. Here is a quick example of the DB structure (with only the relevant fields for identifying categories) Table: products - prod_id Table: categories - cat_id - cat_description Table: Prod_cat - prod_id - cat_id
-
Unset local arrays with global scope: not working as expected
Psycho replied to ExpertAlmost's topic in PHP Coding Help
OK, I know that is only mock code, but there are some issues with it. 1. Declare the $my_array as gloabl before you start the loop. Having it in the loop causes unnecessary processing. 2. Do you actually use $my_array in second_function() at all? In the code above it serves no purpose to even use $my_array in that function. -
Your answer is in the manual. In other words, if you are using a PHP version prior to v5 then it is only using the first character. Looking at your logic though, are you really wanting to use strpos()? Or are you wanting to see if the $cat_id value is equal to $allcat?
-
That will work fine as long as you want the comparisons to be case sensitive. i.e. "wood" != "Wood". If you need the comparison to be case insensitive you can do something similar to what you had before using strcasecmp() if(strcasecmp($q, 'the')==0 || strcasecmp($q, 'wood')==0 || strcasecmp($q, 'host')==0)
-
Unset local arrays with global scope: not working as expected
Psycho replied to ExpertAlmost's topic in PHP Coding Help
I don't know that you can use global for a variable while at the same time setting a valule for it. It may work, not sure. If not just do it this way: global $my_array; $my_array = array[]; -
Unset local arrays with global scope: not working as expected
Psycho replied to ExpertAlmost's topic in PHP Coding Help
To get the functions working as you apparently want them to, you can simply define $my_array as global in second_function(). -
Unset local arrays with global scope: not working as expected
Psycho replied to ExpertAlmost's topic in PHP Coding Help
Your results make perfect sense. When you create "$my_array" in second_function() it is local to that function. Using global $my_array; in third_function() does not give you access to $my_array; from second_function(). In order to access a global variable the variable must first exist in the global scope, i.e. it has to be defined outside any functions. Example: $globalA = "A"; function createLocalVar() { $localB = "B"; } function outputVarsFromLocal() { global $globalA, $localB; echo "GlobalA: {$globalA}<br />\n"; echo "LocalB: {$localB}<br />\n"; $localB = "C"; } createLocalVar(); outputVarsFromLocal(); echo "LocalB (second time): {$localB}<br />\n"; Output GlobalA: A LocalB (first time): LocalB (second time): C The variable $localB was defined in a local scope so it cannot be accessed from the global scope (first time). However, by defining the variable as Global in the function, that variable is now also accessible in the global scope. So, we can modify the value in the function and access it while in the gloable scope (i.e. outside any functions). -
Try rephrasing your question, I don't understand what you are asking. If you don't want there to be line breaks in an element then just use the CSS property "white-space" with a value of "nowrap"
-
I knew there had to be a function for doing that but couldn't find it.
-
I stated previously that the regex code for a word boundry was "\b", but that you would need to add a second backslash to escape the first backslash. So.... If you need to escape another character (such as the ":" or ")") then the same logic would apply - wouldn't it? searchWord[0] = "\\:\\)"; I'm pretty sure you only need to escape the paren and not the colon, but it doesn't hurt to escape them both.
-
Might I suggest an alternative solution that "may" fit your needs better? The values you specified in your example may just be "mock" data that is not representative of the values you are really using. But, at first I thought that the first list of values were "labels" and the second list were "values", but I'm not sure. At the very least you are creating a one-to-one relationship. In that case, a better approach may be to create a single dimension array where one list is used as the keys and the other list is used as the values: $final = array(); for($i=0, $count=min(count($path), count($location)); $i<$count; ++$i) { $final[$path[$i]] = $location[$i]; } Content of $final Array ( [base] => india [category] => USA [subcategory] => UK [item] => RUSSIA )
-
Just to second what BlueSkyIS stated, you state you want to update the second select list dynamically. You should know that once a page is delivered to the browser PHP has no way of interacting with the page by itself. You either need to utilize JavaScript (which can initiate a PHP call) or you can have the user make a selection from the primary drop-down and manually submit the page. AJAX IS the solution you are looking for - you just don't know it.
-
Not quite! That query would match ALL records in the table because it is using OR. Change the OR's to AND's to get the results you are looking for.
-
Sorry for your loss, but I have to agree with CV. I'm also curious as to why you did have time to post a reply and ask an additional question about something that apparently wasn't important to you at the time. I really don't care about an answer, just thinking out loud. In any event, you have your solution and you've at least said "thank you" (which is more than many people do these days). So, I have all I need from this thread.
-
I don't see the original code, but I see two uses of unset in your example unset($positions[$index++]); and unset ($var_1++); The second usage makes no sense. Why would you want to unset a variable while at the same time incrementing it. However, the first example does make sense. In that example you are unsetting the array variable $positions[n] where n is the value of $index. Then $index is incremented by 1. It is not trying to unset $index - only the array value with an index equal to the $index variable.
-
Constructing SQL search strings from multiple html form elements?
Psycho replied to DCM's topic in PHP Coding Help
That is the ternary operator. Basically it is the same as the following: if (count($whereParts)>0) { $WHERE = 'WHERE ' . implode(' AND ', $whereParts); } else { $WHERE =''; } So, if there were any where parts it creates a string in the format: 'name` = 'enteredName' AND `job_title` = 'enteredTitle' Otherwise the sting is empty