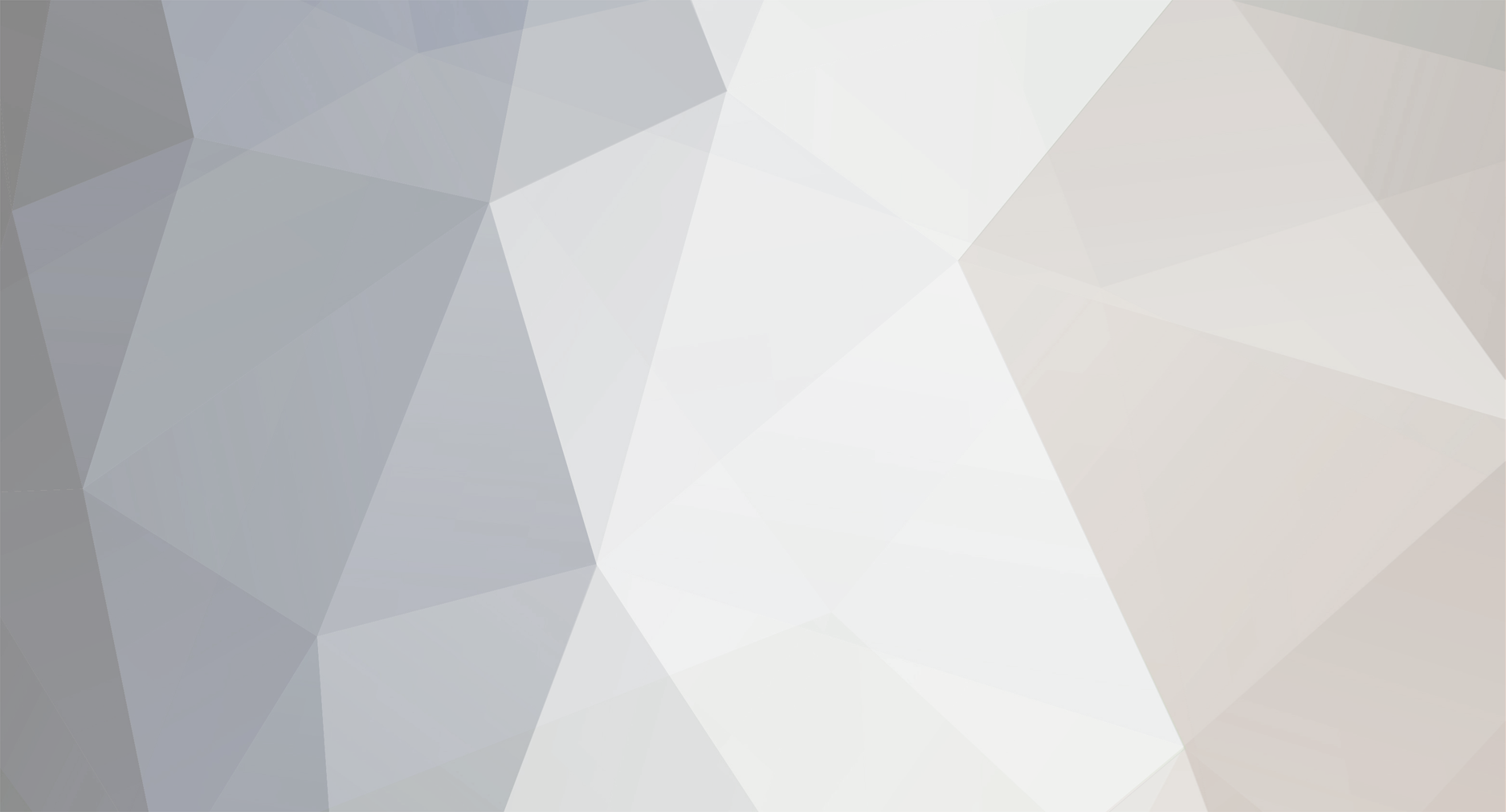
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Of course, you could have found this information yourself by simply reading the manual regarding arrays. http://php.net/manual/en/language.types.array.php Not only will it take a few milliseconds longer to process, the code could stop working entirely in future versions of PHP if they decide to fix that "bug".
-
There's a lot of ivalid HTML markup in that code. For example, once you open a table, any "non table" markup should only be inside TD or TH tags until the table is closed. Anyway, add this trigger to your select tag: onchange="displayText(this);" Then create a DIV for the text to be displayed in and give it an ID parameter, such as "description" Then add this to the head of the document: <script type="text/javascript"> function displayText(selObj) { var selVal = selObj.options[selObj.selectedIndex].value; var outputObj = document.getElementById('description'); switch(selVal) { case 'Apple': outputObj.innerHTML = 'A green fruit'; break; case 'Lime': outputObj.innerHTML = 'Another green fruit'; break; case 'Banana': outputObj.innerHTML = 'A yellow fruit' break; default: outputObj.innerHTML = '' } } </script>
-
Comparing values within two different arrays and pulling my teeth out
Psycho replied to soma56's topic in PHP Coding Help
What?! You asked about finding like values in two different arrays. Now you are posting code that is doing database queries. Try explaining what you are trying to achieve instead of only giving half the info because you *think* you know what you are doing. Besides, you should NEVER be running queries in a loop like that. -
There is no one/right answer to your question - it all depends on exactly how the code is to be used. But, for illustrative purposes, let's say that in this example the "Hello" will be displayed at the top of the page, but you won't know whether it should be displayed until after you have processed all of the code for the rest of the page. Here is ONE example <?php $showHello = false; function getHelloText($showHelloBool) { return ($showHelloBool) ? 'Hello' : ''; } if($thisUser=='NewUser') { $content = "Welcome."; $showHello = true; } else { $content = "Welcome back."; } $helloText = getHelloText($showHello); ?><html> <body> <?php echo $helloText; ?> <?php echo $content; ?> </body> </html>
-
Correct. Unless you are using SSL (i.e. https) the data submitted by the user is transmitted in plain text. McAfee has no knowledge of what hashing (SHA is hashing not encryption) you are using on the server - so I don't know what it is reporting on. You *could* use some javascript to obfuscate the input before it sends the data. But, to be honest, you then open yourself up to a whole host of potential errors and wouldn't be worth it IMHO.
-
Comparing values within two different arrays and pulling my teeth out
Psycho replied to soma56's topic in PHP Coding Help
You're making this much more difficult on yourself than you need to. Take a look in the manual and you will find many existing functions for what you want. $both = array_intersect($array1, $array2); $diff1 = array_diff ($array1, $array2); $diff2 = array_diff ($array2, $array1); echo "<pre>"; echo "These values exist in both array1 and array2:\n"; print_r($both); echo "\n\nThese values exist in array1 but not in array2:\n"; print_r($diff1); echo "\n\nThese values exist in array2 but not in array1:\n"; print_r($diff2); -
You don't need to do all this: foreach (mysql_fetch_array($query) as $row){ $event_data[] = array( "event" => $row['event'], "event_id" => $row['event_id'], "aid" => $row['aid'], "creator" => $row['creator'], "creatorname" => $row['creatorname'], "email" => $row['email'], "longitude" => $row['longitude'], "latitude" => $row['latitude'], "category" => $row['category'], "rating" => $row['rating'] ); } Just do this: foreach (mysql_fetch_array($query) as $row) { $event_data[] = $row; }
-
Question about breaking up MySQL results
Psycho replied to PartisanEntity's topic in PHP Coding Help
Um, where do you want it? Assuming you want it after each category, this should work for you. //Query for the data $query = "SELECT `title`, `body`, `image` FROM `posts` ORDER BY `category`, `date` DESC"; $content = mysql_query($query) or die(mysql_error()); $current_category = false; while($row = mysql_fetch_array($content)) { //Check if new categories if($current_category != $row['category']) { //If not first category add horizontal rule if($current_category!==false) { echo "<hr />\n"; } //Set and display current category header $current_category = $row['category']; echo "<p class=\"cat\">category: {$current_category}</p>\n"; } //Display record echo "<h2 class=\"post_title\">{$row['title']}</h2>\n"; echo "<p class=\"post_body\">{$row['body']}</p>\n"; echo "<p class=\"image\">image: {$row['image']}</p>\n"; } -
Well, I'm not guaranteeing that there are no errors in the code I provided. Did you get any errors? glob() is the solution you need. Try the following to see if it is at least getting the files you want: echo "<pre>"; print_r(glob('thumbs/*_th.jpg')); echo "</pre>";
-
All your images are in the "thumbs" directory - even the non-thumbs? A simple fix would be to have the in different folders. But, there is still an easy fix. Instead of using opendir() and then reading all the files, just use glob(). I cleaned up the logic as well. It looks like you are determining the selected image according to the order in which the images are displayed. You really should be using a unique ID for that. I would also suggest passing $pic to the function instead of referencing it through the global name space <?php // CHANGE PARAMETERS HERE BEGIN $senderName = " Holidays From Home "; // Eg.: John's Postcards $senderEmail = "holidaysfromhome@voluntary.awardspace.co.uk"; // Eg.: john@postcard.com // Change only if you have problems with urls $postcardURL = "http://".$_SERVER["HTTP_HOST"].$_SERVER["SCRIPT_NAME"]; // CHANGE PARAMETERS HERE END $result = 0; $msg = ""; $msg1 = ""; $pic = ""; function displayPhotos() //edit photos and change image sizes { global $pic; $columns = 5; $count = 0; //Get all thumb files $thumbs = glob('thumbs/*_th.jpg'); // Read all file from the actual directory foreach($thumbs as $file) { $count++; //Open row if first record for row if($count%$columns==1) { echo "<tr>"; } $checked = (isset($pic[1]) && $pic[1]==$count) ? ' checked="checked"' : ''; //displays the images echo "<td align=\"center\">"; echo "<img src=\"thumbs/{$file}\" alt=\"postcard\" border=\"1\" /><br/>"; echo "<input type=\"radio\" name=\"selimg\" value=\"{$file},{$act1}\"{$checked}/>"; echo "</td>"; $act++; $act1++; //Close row if columns count reached if($count%$columns==0) { echo "</tr>"; } } //Close last row if($count%$columns!=0) { echo "</tr>"; } } ?>
-
It's called a JOIN. Go read some tutorials on all the wonderful things you can do with them: SELECT m.id, m.name, m.email, pv.time FROM profile_views as pv JOIN members as m ON pv.viewerid = m.id ORDER BY m.id
-
Question about breaking up MySQL results
Psycho replied to PartisanEntity's topic in PHP Coding Help
Please follow AbraCadaver's example. @litebearer, never do queries within loops such as that. It puts a huge load on the server and will eventually cause timeouts and other problems. Just get all the records in one query and process the results accordingly such as AbraCadaver showed. -
Well, what you have is overcomplicated and has some problems in the logic. To get it "perfect" would require a complete rewrite. But, I can get you closer to what you want with the following changes (I have added comments to the lines with changes) var intervalw = Math.round((mainw * mainh) / (180 * thumbh)); var intervalh = Math.round(mainh/(mainw/intervalw)); //Define to be proportional to intervalw if(main.offsetLeft+20 < center) main.style.left = main.offsetLeft+5+'px'; else l=true; if(main.offsetWidth < mainw) main.style.width = main.offsetWidth+intervalw+'px'; else { main.style.width = mainw+'px'; w=true; } if(main.offsetHeight < mainh) main.style.height = main.offsetHeight+intervalh+'px'; //Use the proportional value The reason it will still be slightly off is because the intervals are rounded. So, one dimension will always complete before the other (unless the dimenaions work out to be perfectly similar). A better approach would have been to predetermine the number of steps you want the process to take. Then on each step you could cacluate the new dimensions accordingly. Then both the width and height would finish at exactly the same time. To do that would require a complete rewrite of the logic.
-
No need to get complicated here. Your current code simply uses this validation to check if the GET value was not set or was a null string if($FirstName=="") {echo $error1; exit;} So, just change the way that you set the value. Change this $FirstName = $_GET['FirstNameTextBox']; To this $FirstName = (isset($_GET['FirstNameTextBox'])) ? $_GET['FirstNameTextBox' : ''; Then all your existing code will work as designed. Do the same for all the other values you set from the GET values.
-
The link you provided only shows random characters that appear to be hashed values or something.
-
I started to work on this, but without a working example (i.e. the necessary HTML code) it is very difficult to understand what that code is doing. Making matters worse there are no comments and varaibles such as "l", "w", and "h" are not descriptive enough to know what they are for. I think there is quite a bit of inefficiency in that code (especially since the sme variables ahve to be calculated on each iteration of the function), but can't really say without better understanding.
-
PHP, HTML and Javascript problem with Select drop down
Psycho replied to etymole's topic in Javascript Help
You want to use AJAX. There are countless tutorials available and I couldn't explain it adequately in a forum to do it justice. Basically, you will use JS to trigger when a chnage is made to the select list and then "submit" that value using JS to a PHP page. That PHP page can then use the value(s) to run a query, or whatever you want to do, then return results back to the JS calling function. The JS can then do something such as update a table of records based on the currently selected country. All of this happens in real time without having to submit/refresh the page. -
Ah yes, forgot about that. Although I don't like the fact that readonly fields display as normal fields whereas disabled fields "look" disabled. By making them readonly they can appear as editable to the user - so I'll usually use some style properties to make the readonly fields appear "disabled" to the user.
-
Heaven forbid you actually spend a few precious moments of your time to find out WHY I used it. You might actually learn something. I don't typically use it either. But, if you were to read the manual for ip2long() you would know that there is a valid reason to use sprintf() with the %u formatter! From the manual (emphasis added)
-
OK, first, I made a mistake by applying the discount as the vat: var vat = getIntValue(document.getElementById('discount')); Second, I used the function getIntValue for all the fields, which was another mistake since all the values won't be ints (only the quantity fields). So we need another function to get float values. Third, the math wasn't right in my code. It was difficult to interpret the match from what you have. I have rewritten the formula to be simpler: total = (subTotal * (1-disc/100) * (1+vat/100)) Yes it is. You should NOT be relying upon javascript calculated values anyway. A malicious user can hijack your form and make the total whatever they want it to be. You need to be recacluating on the server-side. If you do want the user to be able to edit those fields, then by all means make the fields editable. But, I don't think that is what you are wanting. If you don't want the user to be able to edit those values but you think you can safely rely upon JS calculated values that the user could potentially modify, then you could always create a second set of fields for those values and make them hidden fields. It is still a security risk though. I just realized none of your fields actually have IDs!!! IE will interpret the field name as an ID if no ID exists. That is one problem with IE is that it will try and "correct" errors and make it seem like the code is correct when it isn't. This has all the corrections and will work in FF. <html> <head> <script type="text/javascript"> function getIntValue(fieldObj) { if(isNaN(parseInt(fieldObj.value))) { fieldObj.value = ''; return 0; } return parseInt(fieldObj.value); } function getFloatValue(fieldObj) { if(isNaN(parseFloat(fieldObj.value))) { fieldObj.value = ''; return 0; } return parseFloat(fieldObj.value); } function reCalcType(typeName) { var qty = getIntValue(document.getElementById('credit_'+typeName+'_num')); var amt = getFloatValue(document.getElementById('credit_'+typeName+'_value')); document.getElementById('credit_'+typeName+'_total').value = (amt * qty); reCalcTotal(); return; } function reCalcTotal(fieldObj) { //Calc subtotal var pp_total = getFloatValue(document.getElementById('credit_pp_total')); var pr_total = getFloatValue(document.getElementById('credit_pr_total')); var jobs_total = getFloatValue(document.getElementById('credit_jobs_total')); var event_total = getFloatValue(document.getElementById('credit_event_total')); var subTotal = (pp_total + pr_total + jobs_total + event_total); document.getElementById('subtotal').value = subTotal; //Calc total var disc = getFloatValue(document.getElementById('discount')); var vat = getFloatValue(document.getElementById('vat')); var total = (subTotal * (1-disc/100) * (1+vat/100)); document.getElementById('total').value = total; return; } function tt(fieldObj) { alert(fieldObj.value*2); } </script> </head> <body> <form action="" method="post"> <input type="hidden" name="creditRecordAdded" value="<?php echo $client_id; ?>"> <table border="1" width="100%"> <tr><td width="25%">Order Date </td><td><input type="text" name="credit_order_date" size="30"></td></tr> <tr><td>Order Number </td><td><input type="text" name="credit_order_num" size="30"></td></tr> <tr><td>Billing Info </td><td><textarea name="credit_bill_info" cols="50" rows="2"></textarea></td></tr> <tr> <td colspan="2"> <table width="98%" align="center" border="1"> <tr><td colspan="4"><b>Package</b></td></tr> <tr> <td colspan="2">Start Date : <input type="text" name="credit_start_date" size="10"></td> <td colspan="2">End Date : <input type="text" name="credit_end_date" size="10"></td> </tr> <tr> <td>PP</td> <td><input type="text" name="credit_pp_num" id="credit_pp_num" size="4" onChange="reCalcType('pp')" /></td> <td>@ <input type="text" name="credit_pp_value" id="credit_pp_value" size="10" onChange="reCalcType('pp')" /></td> <td> = <input type="text" name="credit_pp_total" id="credit_pp_total" size="10" id="credit_pp_total" disabled="disabled" /></td> </tr> <tr> <td>PR</td><td><input type="text" name="credit_pr_num" id="credit_pr_num" size="4" onChange="reCalcType('pr')" /></td> <td>@ <input type="text" name="credit_pr_value" id="credit_pr_value" size="10" onChange="reCalcType('pr')" /></td> <td> = <input type="text" name="credit_pr_total" id="credit_pr_total" size="10" id="credit_pr_total" disabled="disabled" /></td> </tr> <tr> <td>Jobs</td><td><input type="text" name="credit_jobs_num" id="credit_jobs_num" size="4" onChange="reCalcType('jobs')" /></td> <td>@ <input type="text" name="credit_jobs_value" id="credit_jobs_value" size="10" onChange="reCalcType('jobs')" /></td> <td> = <input type="text" name="credit_jobs_total" id="credit_jobs_total" size="10" id="credit_jobs_total" disabled="disabled" /></td> </tr> <tr> <td>Events</td><td><input type="text" name="credit_event_num" id="credit_event_num" size="4" onChange="reCalcType('event')" /></td> <td>@ <input type="text" name="credit_event_value" id="credit_event_value" size="10" onChange="reCalcType('event')" /></td> <td> = <input type="text" name="credit_event_total" id="credit_event_total" size="10" id="credit_event_total" disabled="disabled" /></td> </tr> </table> </td> </tr> <tr> <td>Subtotal</td> <td><input type="text" name="credit_subtotal" id="subtotal" size="30" onChange="reCalcTotal()" disabled="disabled" /></td> </tr> <tr> <td>Discount (%) </td><td><input type="text" name="credit_discount" id="discount" size="30" onChange="reCalcTotal()" /></td></tr> <tr> <td>VAT (%) </td> <td><input type="text" name="credit_vat" id="vat" size="30" value="17.5" onChange="reCalcTotal()" /></td> </tr> <tr> <td>Total </td> <td><input type="text" name="credit_total" id="total" size="30"disabled="disabled" /></td> </tr> <tr> <td>Invoice Date</td> <td><input type="text" name="credit_inv_date" size="30"></td> </tr> <tr> <td>Invoice Number </td> <td><input type="text" name="credit_inv_no" size="30"></td> </tr> <tr> <td>Paid Date</td> <td><input type="text" name="credit_paid_date" size="30"></td> </tr> <tr> <td>Contact </td> <td><input type="text" name="credit_contact" size="30"></td> </tr> <tr> <td>Contact Number</td> <td><input type="text" name="credit_contact_num" size="30"></td> </tr> <tr> <td>Contacted On</td> <td><input type="text" name="credit_contacted" size="30"></td> </tr> <tr> <td>Notes </td> <td><textarea name="credit_notes" cols="40" rows="5"></textarea></td></tr> <tr> <td colspan="2" align="center"><input type="submit" value="Add a new credit record"></td></tr> </table> </form> </body> </html>
-
I guess reading the other responses was too much work for you? I already provided a working solution that was more efficient than what you posted later.
-
I don't use JQuery, but all you need to do is give the element an ID, then change the value accordingly <div id="pp"> <form action="url" method="post"> <input type="hidden" name="amount" id="amount" value="THIS VALUE"> </form> </div> Then have some javascript code that is triggered through some event to change the value document.getElementById('amount').value = "NEW VALUE";
-
For some reason this creates an infinite loop when I try to increment $decimal using $decimal++, so I just incremented it long hand. $startIP = '192.168.10.1'; $endIP = '192.168.11.10'; $startDecimal = sprintf("%u\n", ip2long($startIP)); $endDecimal = sprintf("%u\n", ip2long($endIP)); for($decimal=$startDecimal; $decimal<=$endDecimal; $decimal=$decimal+1) { echo long2ip($decimal) . "<br />\n"; }
-
What the?! Did you even LOOK at the links he posted? One was on creating forms in PHP and the other was for modifying files. Isn't that what you want to do? I had already completed this before I read that response of yours which suggests you can't even take the time to look at the information provided to you. So, I'll post this anyway, but I do so with hesitation <?php $fileName = "temp.txt"; $lineToEdit = 10; if(isset($_POST['editLine'])) { $lines = file($fileName, FILE_IGNORE_NEW_LINES); $editLine = $_POST['editLine']; $lines[$lineToEdit-1] = $editLine; $fh = fopen($fileName, 'w') or die("can't open file"); fwrite($fh, implode("\n", $lines)); fclose($fh); } //Get 10th line from if(!isset($line10)) { $lines = file($fileName, FILE_IGNORE_NEW_LINES); $editLine = (isset($lines[$lineToEdit-1])) ? $lines[$lineToEdit-1] : ''; } ?> <html> <body> <form method="POST"> Value of line <?php echo $lineToEdit; ?>:<br /> <input type="text" style="width:300px;" name="editLine" value="<?php echo $editLine; ?>" /><br /><br /> <button type="submit">Change Value</button> </form> <?php //This block of code for debugging purposes only echo "<pre>\n"; print_r($lines); echo "</pre>\n"; ?> </body> </html>
-
Perhaps if you explained your problem better and showed the code you have we could help you. I have no idea what you mean by "I want to update a txt files 10. row with a form...". Give an example of the input and the output.