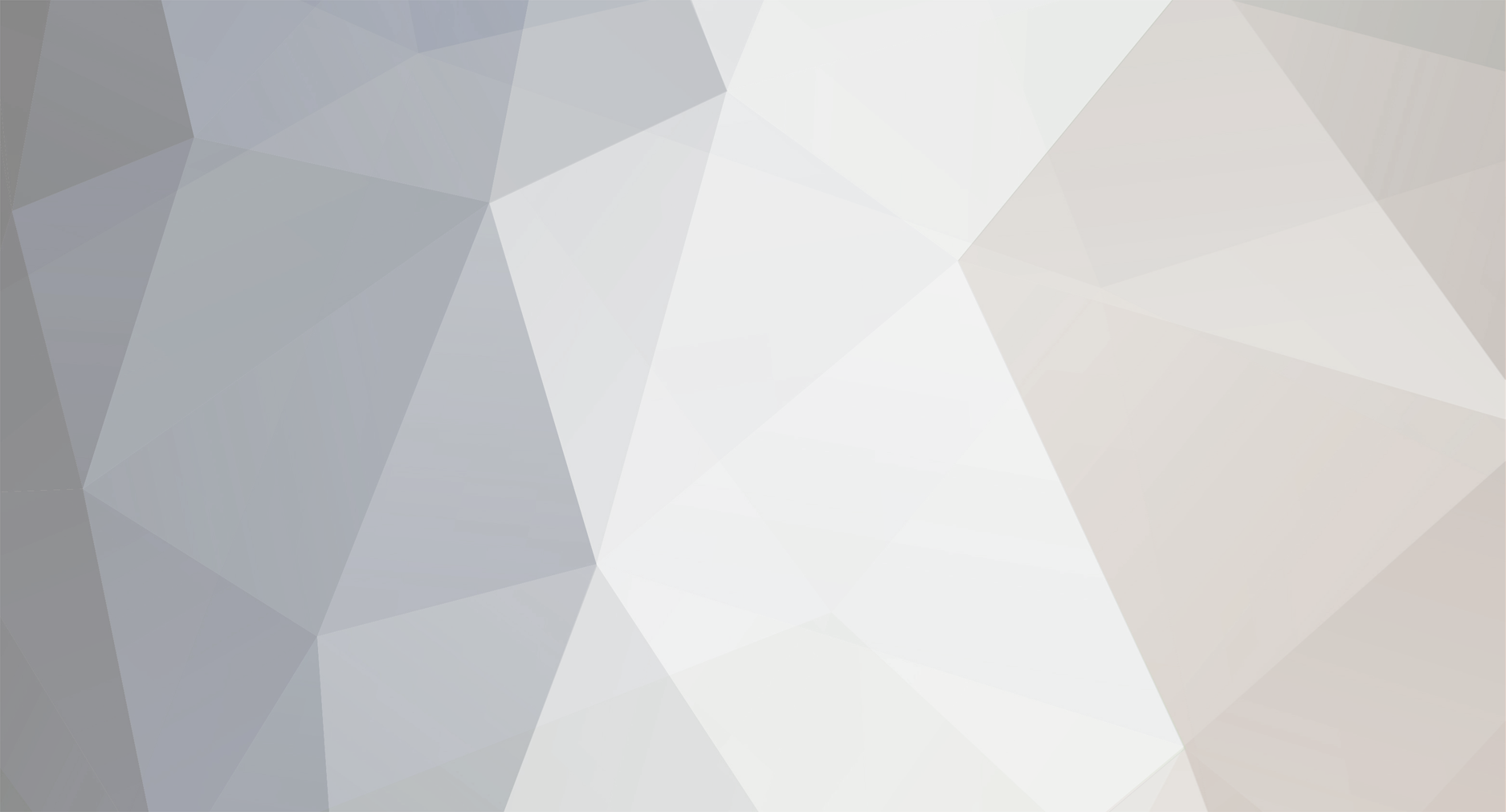
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
First, don't run queries in a loop. You should be joining the $forum_info_query on the $parent_info_query as well. When joining tables, if a joined table record can be null (and you want the first table record anyway) you should be using a LEFT JOIN or something similar. Also, instead of referencing the table name (along with the DB Prefix) for all the fields in the SELECT statement, it would be easier and more readable to create an alias for the table names and use that in the SELECT portion of the query. Here is how I would rewrite the query as you previously have it $query = "SELECT f.forum_id, f.forum_name, f.forum_description, f.forum_topics, f.forum_posts, f.forum_last_poster, f.forum_last_post_time, f.forum_last_post, m.user_id, m.user_username, m.user_group, t.topic_id, t.topic_name FROM ".DB_PREFIX."forums as f LEFT JOIN ".DB_PREFIX."members as m ON f.forum_last_poster = m.user_id LEFT JOIN ".DB_PREFIX."topics as t ON f.forum_id = t.forum_id WHERE f.parent_id = $parent_id"; And here is how I would create ONE single query with all the necessary info. $query = "SELECT f.forum_id, f.forum_name, f.forum_description, f.forum_topics, f.forum_posts, f.forum_last_poster, f.forum_last_post_time, f.forum_last_post, p.parent_id, p.parent_name m.user_id, m.user_username, m.user_group, t.topic_id, t.topic_name FROM ".DB_PREFIX."forums as f JOIN ".DB_PREFIX."parents as p ON f.parent_id = p.parent_id LEFT JOIN ".DB_PREFIX."members as m ON f.forum_last_poster = m.user_id LEFT JOIN ".DB_PREFIX."topics as t ON f.forum_id = t.forum_id ORDER BY p.parent_id"; That query should give you all the information you need without having to run queries in a loop.
-
I think the problem is with the usage of the group by. Can you show the data in question and the WHERE paramaters that you are using?
-
Well, this is a JavaScript question, so you should be posting the HTML output and not the PHP code. Trying to decypher what the HTML will look like from PHP creates more work on people reviewing your code. However, I will say that there are issues with your PHP logic that should be corrected. First off, you are running a query for membergroup within the loop and it is the EXACT same query each time. Running queries within a loop is very inefficinet - and in this instance it is totally unnecessary since it is the same query. For the query to get the topic_id, you should do a JOIN on the main query instead of doing this query in a loop. I'm also curious why you are referencing the last post for a forum using the topic name instead of the ID. Besides, there is absolutely no reason to identify the last post in the forum record AT ALL. Use the database to get that information dynamically. You are working much harder than you have to. Second, give your variables descriptive names. $sql2 means nothing to someone reviewing the code. You will save yourself and others a lot of time by following good programming standards. I was going to rewrite all of this to be more efficient, but it would require DB changes and other things which I'm not interested in tackling at the moment. Here is a sample script that you can implement. <html> <head> <script type="text/javascript"> function expandCollapseTable(tableObj) { var rowCount = tableObj.rows.length; for(var row=1; row<rowCount; row++) { rowObj = tableObj.rows[row]; rowObj.style.display = (rowObj.style.display=='none') ? '' : 'none'; } return; } </script> </head> <body> Click on the table to expand/collapse the data rows<br /><br /> <table border="1" id="myTable" onclick="expandCollapseTable(this)"> <tr id="tr1"> <th>Header 1</th> <th>Header 2</th> <th>Header 3</th> </tr> <tr class="gradient"> <td>Cell 1-1</td> <td>Cell 2-1</td> <td>Cell 3-1</td> </tr> <tr class="gradient"> <td>Cell 1-2</td> <td>Cell 2-2</td> <td>Cell 3-2</td> </tr> <tr class="gradient"> <td>Cell 1-3</td> <td>Cell 2-3</td> <td>Cell 3-3</td> </tr> </table> </body> </html> To implement you will need to define a trigger (e.g. an onclick event) that will fire the function above and the event will need to pass an object reference to the table.
-
this.id is exactly what you need. If it is coming back as undefined, then I suspect that $row['id'] doesn't have a value. have you looked at the generated HTML code to validate that the fields have values for the ID paramater? I'd bet money thatthe HTML looks like this: <select class="attendance-presence" id="" onchange="send_attendance(this.value);">
-
If you have MS Office, just turn on the Office clip board which allows up to 24 copied items. Otherwise, download any of the many freeware program available. Here is one: http://download.cnet.com/Copy-Paste-Clipboard-Extender/3000-2384_4-10316888.html
-
http://php.net/manual/en/function.implode.php echo $values to the page to see what the result is.
-
You could set up your array to take a single parameter of an array of the values. function example($argArray) { extract($argArray); //Function continues from here //... } example(array('arg1'=>'red', 'arg2'=>'horse', 'arg5'=>'texas'));
-
http://www.php.net/manual/en/reserved.variables.server.php
-
Seriously, you waited a whole 12 minutes before bumping your thread. Perhaps the lack of responses is due to the lack of clarity in your request. I *assume* you are wanting to find records where the value does not exist in the values of the array. The query would use the operator "NOT IN" followed by a list of values. $values = "'" . implode("','", $browser_hide) . "'"; $query = "SELECT * FROM hitlist_hits WHERE month='$month' AND browser NOT IN ($values) ORDER by id DESC";
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=310924.0
-
Um, there is a CSS forum. [Moving post]
-
Although your problem is not related to this, I always enclose the code blocks associated with a condition within brackets to make it easier to read/debug. The first echo statement above spans multiple lines which should be easily misinterpreted which could add additional time when debugging. I'd format the code like this: if( $mlsInfo['PhotoURL'] != "") { echo "<a href='emailListing.php?type=rs&mlsn=".$propInfo['MLSNumber']."' target='_blank' onMouseOver='doTooltip(event, 0)' onMouseOut='hideTip()' ><img src='images/buttons/fb_eListing.gif' width='106' height='20' border='0'></a> <a href='emailPostcard.php?type=rs&mlsn=".$propInfo['MLSNumber']."' target='_blank' onMouseOver='doTooltip(event, 2)' onMouseOut='hideTip()' ><img src='images/buttons/fb_ePostcards.gif' width='106' height='20' border='0'></a> <a href='emailFlyer.php?type=rs&mlsn=".$propInfo['MLSNumber']."' target='_blank' onMouseOver='doTooltip(event, 3)' onMouseOut='hideTip()' ><img src='images/buttons/fb_Flyer.gif' width='110' height='20' border='0'></a> <a href='../print_rs.php?type=rs&mlsn=".$propInfo['MLSNumber']."' target='_blank' onMouseOver='doTooltip(event, 5)' onMouseOut='hideTip()' ><img src='images/buttons/fb_printFlyer.gif' width='106' height='20' border='0'></a> <a href='fb_propertyRS_print.php?type=rs&mlsn=".$propInfo['MLSNumber']."' target='_blank' onMouseOver='doTooltip(event, 4)' onMouseOut='hideTip()' ><img src='images/buttons/fb_printListing.gif' width='106' height='20' border='0'></a> "; } else { echo "You Dont have any photos please add some"; }
-
Not exactly. An empty string IS a value. There are many variations of what the lay person would consider an empty value. But, programatically speaking there can be significant differences based upon the context. Here are some examples: Undefined variable (variable has not been created) Null variable (variable is created but no value is assigned) Empty string (A variable which holds an empty string value) False value (a variable with a boolean false value) The value zero (0) (a variable with the value 0 - can be interpreted as false in some conditions) So, as I stated it is testing if the variable $id is NOT an empty string. If the variable was set to Null, false, or was undefined that test would return true just as if the value was "foo"
-
It is a conditional check to test if the variable $id does not equal an empty string.
-
<?php $var = "http://www.google.com" ; ?> <iframe id="I1" name="I1" src="<?php echo $var; ?>" style="width: 1186px; height: 1346px; float: middle"> Your browser does not support inline frames or is currently configured not to display inline frames. </iframe></div>
-
You can't put double quotes within a string defined with double quotes - at least not without escaping them: <?php echo" <table> <TD onMouseOver=\"this.bgColor='#00CC00'\" onMouseOut=\"this.bgColor='#009900'\" bgColor=\"#009900\"><tr> "; ?>
-
Yes, it is possible. I don't have any code for this. I would guess there is some available on the web, but could not find any with some initial searches. I could probably build a solution, but that is way more time than I am willing to invest. Trying to do it in an existing WYSIWYG editor makes the implementation more difficult since you would need to create the controls within the editor itself, but you would also need hidden fields to save the user set sizes. The user would only be resizing the displayed size, not the actual size of the image. So, along with the upload of the image you would have to pass the "displayed" sizes that the user set so you can use server-side code to actuall resize the physical image. I'd suggest looking for a different editor.
-
You mean you want [2] => string(0) "" The poblem is that the plus sign (+) will match 1 or more instances. So, a non-comma has to exist between commas. Instead you want to match none or more. Try this: preg_match_all("/\"[^\"]*\"|[^,]*/", $input, $output);
-
Are you trying to piss me off? I say that jokingly, but it is kind of frustrating when you provide a solution to someone and then they change the requirements (not once, but twice!). Just tell me up front what you want. If I can't provide it all then I will provide what I can. <html> <head> <script type="text/javascript"> window.onload = function() { countDown('my_div1', '<a href="cdtl.html">Hello 1</a>', 3); countDown('my_div2', '<a href="cdtl.html">Hello 2</a>', 5); countDown('my_div3', '<a href="cdtl.html">Hello 3</a>', 10); } function countDown(elID, output, seconds) { document.getElementById(elID).innerHTML = (seconds==0) ? output : 'Time until link appears: ' + seconds; if(seconds==0) { return; } setTimeout("countDown('"+elID+"', '"+output+"', "+(seconds-1)+")", 1000); } </script> </head> <body> <div id="my_div1"></div> <div id="my_div2"></div> <div id="my_div3"></div> </body> </html>
-
document.getElementById(elID).innerHTML = (seconds==0) ? output : 'Time until link appears: ' + seconds;
-
Works fine for me. Must be a problem somewhere else in your code. Here is the code I tested with Parent <html> <body> <button onclick="window.open('child.htm')">Open Child</button> <div id="resultDIV">Doesn't work</div> </body> </html> Child <html> <head> <script type="text/javascript"> function update_parent() { window.opener.document.getElementById('resultDIV').innerHTML = 'works'; window.close(); } </script> </head> <body> <button onclick="update_parent()">Update Parent</button> </body> </html>
-
<html> <head> <script type="text/javascript"> window.onload = function() { countDown('my_div', '<a href="cdtl.html">Hello</a>', 3); } function countDown(elID, output, seconds) { document.getElementById(elID).innerHTML = (seconds==0) ? output : seconds; if(seconds==0) { return; } setTimeout("countDown('"+elID+"', '"+output+"', "+(seconds-1)+")", 1000); } </script> </head> <body> <div id="my_div"></div> </body> </html>
-
To be honest, I have lost interest in this post. I only provided generic answers because your code is a little disorganized and would take me longer to rework than I am willing to invest. Plus, you haven't provided any information as to any debugging you have done to find the errors yourself. Not saying you haven't done any, but it would be helpful if you provided information such as Also, as a side note, I would highly suggest giving your variables descriptive names pertaining to what they really are. I *think* the $splits variable has something to do with calculating the total number of pages, but I shouldn't have to read through the code to figure that out. For the first block of code, I would use the following (assuimg the $WHERE_CLAUSE has already been defined): //Define the max records on a page $records_per_page = 3; //Run query to get total record count (for current filter) $query = "SELECT COUNT(*) FROM `behan` {$WHERE_CLAUSE} GROUP BY `na`"; $getcount = mysql_query($query) or die(mysql_error()); $total_records = mysql_result($getcount,0); //Calculate number of pages (for current filter) $num_pages = ceil($total_records/$records_per_page); //Determine the page to display $current_page = (isset($_GET['pg'])) ? (int) $_GET['pg'] : 1; if($current_page<1 || $current_page>$num_pages) { $current_page = 1; } //Define the limit start position for the current page of records (for current filter) $limitstart = (($current_page-1)*$records_per_page); //Create query for the page of records to be displayed $query = "SELECT * FROM `behan` {$WHERE_CLAUSE} GROUP BY na ORDER BY total_value DESC LIMIT $limitstart, $records_per_page"; Then in the code to disply the page links: if($num_pages>1) { $thisroot = $_SERVER['PHP_SELF']; echo "<strong>Sider:</strong> "; //Create link to navigate to last page if($current_page > 1) { echo "<a href=\"{$thisroot}?pg=" . ($current_page-1) . "\"><< Forrige</a> \n"; } //Create individual page links for($page=1; $page<=$num_pages; $page++) { echo ($page!= $current_page) ? "<a href=\"{$thisroot}?pg={$page}\">$page</a>" : $page; echo " "; } //Create link to next page if($current_page < $num_pages) { echo "<a href=\"{$thisroot}?pg=" . ($current_page+1) . "\">Næste >></a>"; } }
-
I'm sure there are other efficiencies to be had, but the one that jumps out at me is that you are running queries in a loop. Doing that is horribly inefficient. There are two possible solutions I see: 1. Use an INSERT statement with ON DUPLICATE KEY UPDATE instead of just an UPDATE statement. You would then gather the data for the query within the loop and after the loop completes run a single query. Example: In the loop change this line $sql_edit = "Update sppindex Set rem3 = '$piccount' Where ID = '$ID' "; To this $values[] = "('$ID', '$piccount')"; Then after the loop has completed, create a single query like this $sql_edit = "INSERT INTO sppindex (ID, rem3) VALUES " . implode(', ', $values) . ON DUPLICATE KEY UPDATE rem3=VALUES(rem3)"; 2. The other option is similar. But, instead of using ON DUPLICATE KEY UPDATE you create a single UPDATE statement that will do the same thing. Example: In the loop change this line $sql_edit = "Update sppindex Set rem3 = '$piccount' Where ID = '$ID' "; To this $cases[] = "WHEN '$ID' THEN '$piccount'"; Then after the loop has completed, create a single query like this $sql_edit = "UPDATE `sppindex` SET `rem3` = CASE `ID` " . implode("\n", $cases) . "END"; I'm sure one method is more efficient than the other. You could do some testing to find out which is better.
-
Unless you are leaving out code that file_get_contents() serves no purpose. If you are using that content somewhere is there some reason you can't store the contents in the database to begin with?