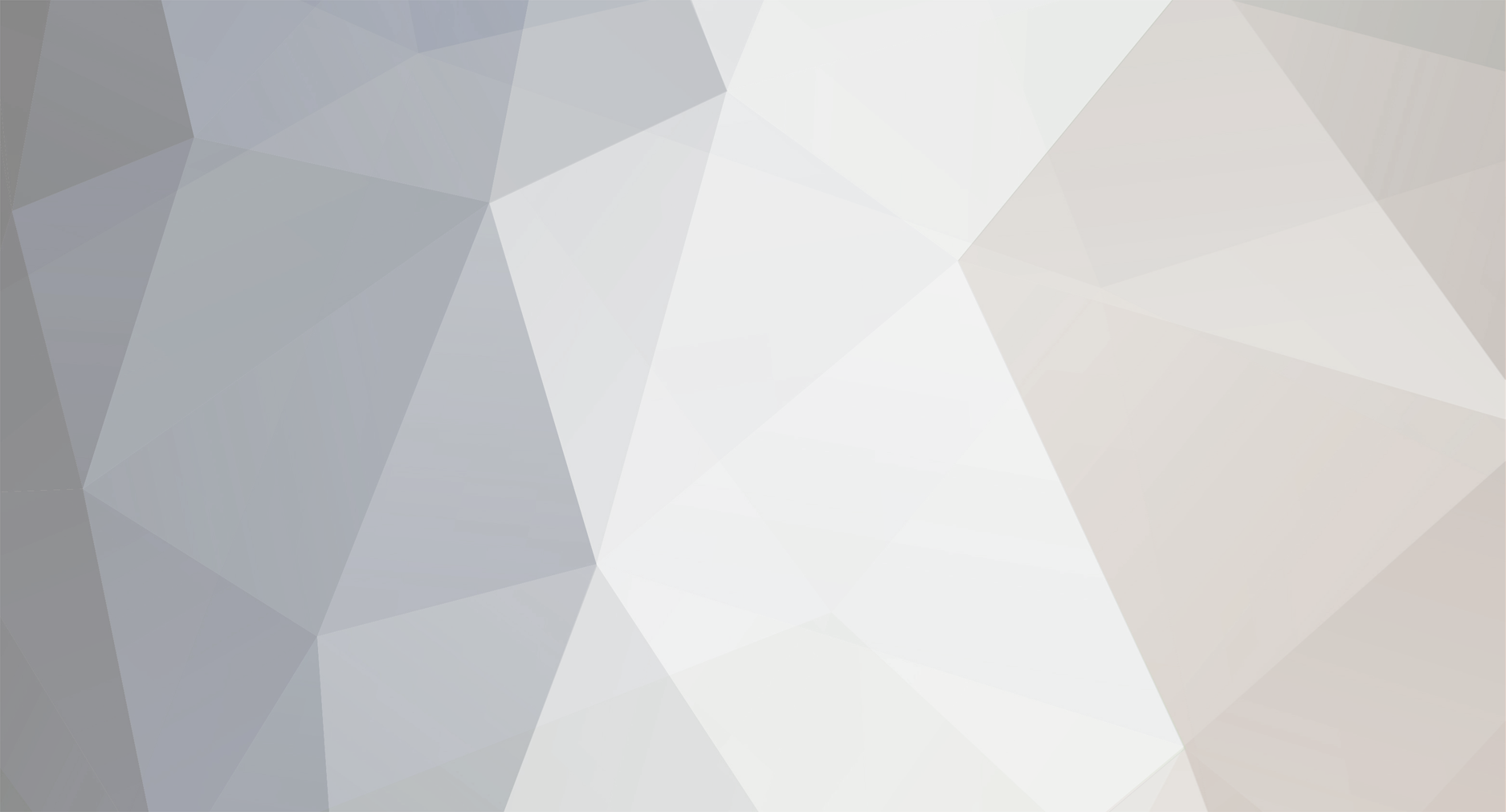
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Can someone translate this PHP code for me?!
Psycho replied to oliverj777's topic in PHP Coding Help
nope - that would be isset($_GET['user']) What do you think (!$_GET['user']) can be used for? First off being set (as you first proposed) and having a value are two entirely different things. isset() is - obviously - the function to test if a variable is set. If you want to check if the string variable has a value you would use empty(). (!$_GET['user']) checks to see if the value resolves to the boolean false. That is a terrible method of testing if a variable is set and/or has a value. If the user entered the value '0' that would return false even though the variable is set and has a non-empty value. -
There is another solution. As I understand it, when the page loads it is making a determination of whether or not the data is 180 seconds old. If not, it reads the current XML file. But, if the file is older than 180 seconds it queries the db and writes a new file - then you attempt to read that new file (which is where you are experiencing the error.) If you just queried the database and wrote the new file - why do you need to read the file? You already have the content. By trying to read the file (of data you just wrote) the script is being inefficient. Here is a "rough" example of a possible solution. This is not tested, and I don't know if this would work as is - but the logic is valid if((time()-filemtime($data)) < 180) { //Read the current file xml_parse($xml_parser, $data, feof($fp)); } else { //Get new data $query = "SELECT * FROM table WHERE foo='bar'"; $result = mysql+query($query); //Create loop to generate XML content into variable while($record = mysql_fetch_assoc($result)) { $xml_data = //Process to create new XML content } //Write the data to xml file $fh = fopen($data, 'w') or die("can't open file"); fwrite($fh, $xml_data); //Parse the xml variable - not the xml file just created xml_parse($xml_parser, $xml_data); }
-
Did you even try the solution? json_encod returns the values in exactly the same format as you just stated: "{key1:value1, key2:value2, key3:value3, ...}". Although you would want to "add them up" within a loop that extracts the records. Your query is only selecting fields "A" and "B", but you state you need data for name and email. If those fields really are called "A" & "B" then just change the names of the columns returned. Off topis: I wonder why I see so many examples of people using a numeric counter to iterrate through database results instead of just using a while loop. Sample code: $query = "SELECT `A` as `name', `B` as 'email' FROM USS"; $result = mysql_query($query); $jsVarsAry = array; while($user = mysql_fetch_assoc($result)) { $jsVarsAry = json_encode($user); } $jsVarsStr = implode(",\n", $jsVarsAry); <script type="text/javascript"> var contacts = '[<?php echo $jsVarsStr; ?>]'; </script> Not tested, so there may be some minor tweaking needed.
-
I rewrote it as a funtion that takes two parameter: the text to be modified and the offset. The offset represents how many letters forward or backward that the text should be shifted. So you can shift it 1, 2, 3 or -1, -2, -3 or whatever number of characters. function shiftLetters($text, $offset) { for($i=0, $n=strlen($text); $i<$n; ++$i) { $val = ord($text[$i]); if(($val>=65 && $val<=90) || ($val>=97 && $val<=122)) { $base = ($offset>0) ? (($val<=90)?65:97) : (($val<=90)?90:122); $text[$i] = chr($base + ($val-$base+$offset)%26); } } return $text; } echo shiftLetters('abcxyz', 2); //Ouput: cdezab echo shiftLetters('abcxyz', -2); //Ouput: yzavwx
-
I can't answer your question. I don't know how all the code relates to the HTML page. The onchange event would be tied to the cities field. To be honest I'm not going to dig throught he code and write the solution for you. This forum is for people who want help with code they have written. if you need someone to write the code for you I'm sure there's someone willing to do this for you (for a fee) in the freelance forum.
-
Forms not sending numbers, and not sure how to check
Psycho replied to richrock's topic in Javascript Help
Your code is pretty comprehensive in the erro handling. The problem is that an empty field does not have a NULL value - it has an empty string value. There is a fine distinction between the two. I took the liberty of making some other modifications function addTotal() { var boyObj = document.getElementById('boyval'); var girlObj = document.getElementById('girlval'); var memtot = document.getElementById('memtot'); if(boyObj.value=='' || isNaN(boyObj.value)) { boyObj.value = '0'; } if(girlObj.value=='' || isNaN(girlObj.value)) { girlObj.value = '0'; } memtot.value = parseInt(boyObj.value) + parseInt(girlObj.value); } -
Assuming the last field will also be a select list, just use the html pages for each city as the value of the options. Then use an onchange event such as this onchange="window.location=this.options[this.selectedIndex].value;"
-
EDIT #2: After some testng I found that my original solution does not work, but the modification I posted to AlexWD's code does.
-
Did you check the manual for str_replace()? If so, I would think the answer would jump out at you. Although, it may have just not occured to you, so don't take this as an insult. Anyway $search = array('a','b','c','d','e','f','g','h','i','j','k','l','m', 'n','o','p','q','r','s','t','u','v','w','x','y','z'); $replace = array('b','c','d','e','f','g','h','i','j','k','l','m','n', 'o','p','q','r','s','t','u','v','w','x','y','z','a'); $string = str_replace($search, $replace, $text); Of course, that only works for lower case letters. If you need uppercase as well, you would need to do a 2nd replacement using arrays of uppercase or use regular expression. EDIT: @AlexWD: That solution doesn't work for 'z' as requested by OP. Here is a modification of that code: for($i=0, $n=strlen($text); $i<$n; ++$i) { $val = ord($text[$i]); if(($val>=65 && $val<=90) || ($val>=97 && $val<=122)) { $base = ($val<=90) ? 65 : 97; $text[$i] = chr($base + ($val-$base+1)%26); } }
-
There are a lot of pieces to that puzzle - none of which are difficult, but trying to explain them - in detail - here would be laborious. Here is an outline of what you need to do: 1. Create a form in the page where you have the select list The db records really should have a unique ID field to use as the value instead of the name. You can use the name but then you need to add additionally handing to prevent duplicates. 2. Create a prage to receive the form post. That page will take the selected item and query for the record's data. Then create a form inputting the current data. The user can then make change and submit the changes. 3. Create another page to handle the submission of the change. You can of course create one big page to handle all the activities, but I find creating separate pages based upon functionality is better - although I may use one page that all the pages submit to and just include the necessary pages based upon the actions taking place.
-
You should really be more explicit in your usage of database results. <?php $dbuser="*******"; $dbpass="*******"; $dbname="virtuda_db"; //the name of the database $chandle = mysql_connect("localhost", $dbuser, $dbpass) or die("Connection Failure to Database"); mysql_select_db($dbname, $chandle) or die ($dbname . " Database not found. " . $dbuser); $mainsection="license"; $query="SELECT name FROM license"; $result = mysql_db_query($dbname, $query) or die("Failed Query of " . $query1); //do the query $optionList = ''; while($row=mysql_fetch_assoc($result)) { $optionList .= "<option value=\"{$row['name']}\">{$row['name']}</option>\n"; } echo "<select name=\"somename\">\n{$optionList}\n</select>"; ?>
-
Your query is failing. I suspect it is because you have a field with the name "from" and MySQL is confusing it with the "FROM" for selecting the table. Enclose your field/table names with back ticks. Try this $query = "SELECT `messid`, `message`, `from`, `read`, `date` FROM `messages` WHERE id = '$id'"; $result2 = mysql_query($query) or die("Query:<br />$query<br /><br />Error:<br />".mysql_error());
-
It would have been VERY helpful if you had stated that you were using JavaScript/JQuery for this. In any event I still see no reasong for JavaScript to "create" the columns. (In fact it doesn't even work in IE browsers). You can create columns using just CSS and it makes your site more usable. However, in order to dynamically determine if a column is too long and should be spanned across multiple columns is something that will most likely need to be handled by JavaScript. PHP can't "know" how tall a div will be displayed in the browser because it will be different based upon the users resolution and browser settings. (Note: even attempting to do what you are asking could potentially "break" the output for some users. You will want to test on different browsers, resolutions, font-size, and even hand-helpd devices) You can determine a div's hieght in JS and then dynamically increase the width of the column as needed. Here is a very rough example. It still needs some work with regard to the container div CSS properties. I'm sure there is a way to let the width of the container be dynamic AND forcing the columns not to break without using JS, but there is enough for you to work with. The page will load with the inital maximum column height set to 500px. Chnage it to 400 and click the button to see the results. <html> <head> <style> #columnContainer { border: 1px solid red; width: 2200px; } .column { border: 1px solid black; width: 400px; float: left; padding-right: 20px; font-family: arial; font-size: 10pt; } </style> <script type="text/javascript"> function resetColumns() { var totalWidth = 0; var baseWidth = 400; var maxHeight = document.getElementById('maxHeight').value; var colIdx = 1; while(document.getElementById('column'+colIdx)) { var divObj = document.getElementById('column'+colIdx); widthMult = 1; divObj.style.width = baseWidth + 'px'; while(divObj.offsetHeight > maxHeight) { widthMult++; newWidth = baseWidth * widthMult; divObj.style.width = newWidth + 'px'; } totalWidth += parseInt(divObj.style.width) + 20; colIdx++; } document.getElementById('columnContainer').style.width = totalWidth + 'px'; return; } </script> </head> <body> Max Col Height: <input type="text" id="maxHeight" value="500" /> <button onclick="resetColumns();">Reset Columns</button> <div id="columnContainer"> <div id="column1" class="column"> <h4>Post Title Example One</h4> Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Suspendisse aliquet arcu a ipsum. Nunc arcu est, pulvinar vitae, consectetuer ac, pellentesque interdum, augue. Fusce iaculis nulla et sapien. Aliquam erat volutpat. Etiam iaculis turpis nec libero. Nam adipiscing ullamcorper quam. Pellentesque erat. Sed hendrerit. Duis id nisl. Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan.Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan. <br /> </div> <div id="column2" class="column"> <h4>Post Title Example Two</h4> Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Suspendisse aliquet arcu a ipsum. Nunc arcu est, pulvinar vitae, consectetuer ac, pellentesque interdum, augue. Fusce iaculis nulla et sapien. Aliquam erat volutpat. Etiam iaculis turpis nec libero. Nam adipiscing ullamcorper quam. Pellentesque erat. Sed hendrerit. Duis id nisl. Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan.Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan. <br /><br /> Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Suspendisse aliquet arcu a ipsum. Nunc arcu est, pulvinar vitae, consectetuer ac, pellentesque interdum, augue. Fusce iaculis nulla et sapien. Aliquam erat volutpat. Etiam iaculis turpis nec libero. Nam adipiscing ullamcorper quam. Pellentesque erat. Sed hendrerit. Duis id nisl. Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan. </div> <div id="column3" class="column"> <h4>Post Title Example Three</h4> Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Suspendisse aliquet arcu a ipsum. Nunc arcu est, pulvinar vitae, consectetuer ac, pellentesque interdum, augue. Fusce iaculis nulla et sapien. Aliquam erat volutpat. Etiam iaculis turpis nec libero. Nam adipiscing ullamcorper quam. Pellentesque erat. Sed hendrerit. Duis id nisl. Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan.Cras arcu mauris, mollis vel, convallis non, elementum a, tortor. Donec ac est eget elit consequat sollicitudin. In id odio quis tortor volutpat mollis. Nulla iaculis lobortis est. Pellentesque in elit vitae nulla tempus euismod. Nam non erat in lacus rutrum malesuada. Donec commodo purus sed quam posuere eleifend. Nam accumsan. </div> </div> </body> </html>
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=308022.0
-
You are going to have to provied some examples (image mockups perhaps or a link to the current page) because based upon what you are saying I still don't see the difficulty. This is not a PHP issue, but an HTML/CSS one. Moving to the CSS forum.
-
Just change the width of the div/container that the text is in and let the browser determien where the word wraps will be.
-
That scenario still works for the structure I provided and the structure I provided is better from a database perspective. If I am followign what you are saying each "person" will have one account number - so just make the unique ID on the "customers" table the account number. You still want unique IDs in the accounts table to keep everything organized, but you don't need to expose that number to the user. Plus, I have never been with a finincial institution that does nt have different acct number for each account type. But, oh well.
-
Yeah, some of the most difficult things to do with programming are ones that are "obvious" to a human. The trick is to walk throuh the problem and break it down into specific steps - before you write the first line of code. Please mark the topic as solved.
-
Select Date fields in the future but remove those that have past
Psycho replied to topshelfbleu's topic in PHP Coding Help
Well if your field is a true date field, then this should work $query = "SELECT * FROM fix10 WHERE date >= CURDATE() ORDER BY Date"; -
Hmm.. you are using a class to create the actual HTML and I made some assumptions about what the class code is doing. It's kind of hard for me to really provide a solution without knowing what those functions do - I just made a guess. Since it is still only showing 5 I will make another *guess* that the $tpl->parse() method is hard coded to only process 5 records. If that is the case, this *might* work: <?php function map_showCA() { global $db, $tpl; // ShowLocalResources $sSQL = "SELECT id, state, statename, statename_html FROM states_canada ORDER BY state;"; $db->query($sSQL); $recordIdx = 0; $records_per_column = 5; $column_names = array('first', 'second', 'threeth', 'fourth', 'fifth'); while ($db->next_record()) { $state = $db->f("state"); $statename = $db->f("statename"); $statename_html = (!USE_SEO_LINKS) ? $statename_html = $db->f("id") : $db->f("statename_html"); //Determine the column and column name $colIdx = $recordIdx%count($column_names); $col_name = $column_names[$colIdx]; $parameters = "http://{$statename_html}ilocalize.com"; // $column_link = generate_vendor_seo_url($parameters); $tpl->set_var("{$col_name}_column_link", $parameters); $tpl->set_var("{$col_name}_column", tohtml($statename)); $recordIdx++; //After 5 records create row if($recordIdx%$records_per_column==0) { $tpl->parse("ShowLocalResourcesCA", true); } } //If any remainginrecords create last row if($recordIdx%$records_per_column>0) { $tpl->parse("ShowLocalResourcesCA", true); } } ?> if it doesn't work I'd need to know what that class is doing
-
Define the maximum columns you want at the top <?php $max_columns = 5; //validate item $query = "SELECT p.thumb, p.title, p.name FROM product AS p LEFT JOIN picks AS s on s.item_id = p.id WHERE s.date = CURDATE()"; $get_item_sql = mysql_query($query) or die(mysql_error()); if (mysql_num_rows($get_item_sql) < 1) { //invalid item $display_block .= "<p><em>Invalid item selection.</em></p>"; } else { //Start the table and row $display_block = "<table>\n"; //valid item, get info $item_count = 0; while ($item = mysql_fetch_array($get_item_sql)) { //Create opening TR if needed if($item_count%$max_columns==0) { $display_block .= "<tr>\n"; } $item_count++; $display_block .= "<td >"; $display_block .= "<img src=\"image_files/{$item['thumb']}\" alt=\"{$item['name']}\" />"; $display_block .= "<br />{$item['title']} " $display_block .= "</td>\n"; //Close TR if max reached if($item_count%$max_columns==0) { $display_block .= "</tr>\n"; } } //Close TR if any are left if($item_count%$max_columns>0) { $display_block .= "</tr>\n"; } //Close the row and table $display_block .= "</table>"; } ?>
-
I wonder if my wife would buy that excuse if I cheated. Probably not worth finding out.
-
I don't follow you.
-
And you were there for...? I was searching for the site in question using the email address used in the code, but capsonewire.com doesn't resolve to any website. When I googled that domain it hit on the post I linked to. I am faithfull to PHPFreaks (at least as far as you know I am).
-
To add to Nightslyr's response, HTML tags are just that - HTML tage which tell the browser how to render the page. The browser has nothing to do with PHP code and doesn't know how to process it. The idea behind PH (and other server-side code) is that the server will process the server-side code and output a complete HTML page to send to the browser. Here is an example php page (date.php): <html> <body> The date today is <?php echo date('F d, Y'); ?> </body> </html> When a user requests the page date.php the page will first be processed by the server (html files normally go strait to the user). The PHP processor will look for any PHP codeblocks and process the code. In this instance, the processor will echo (i.e. print) the current date where indicated. The completed page (consisting of only HTML content) is then sent to the user. The final HTML code would look like this: <html> <body> The date today is August 20, 2010 </body> </html> The user who views the page would only see that content if they were to "view source" on the displayed page.