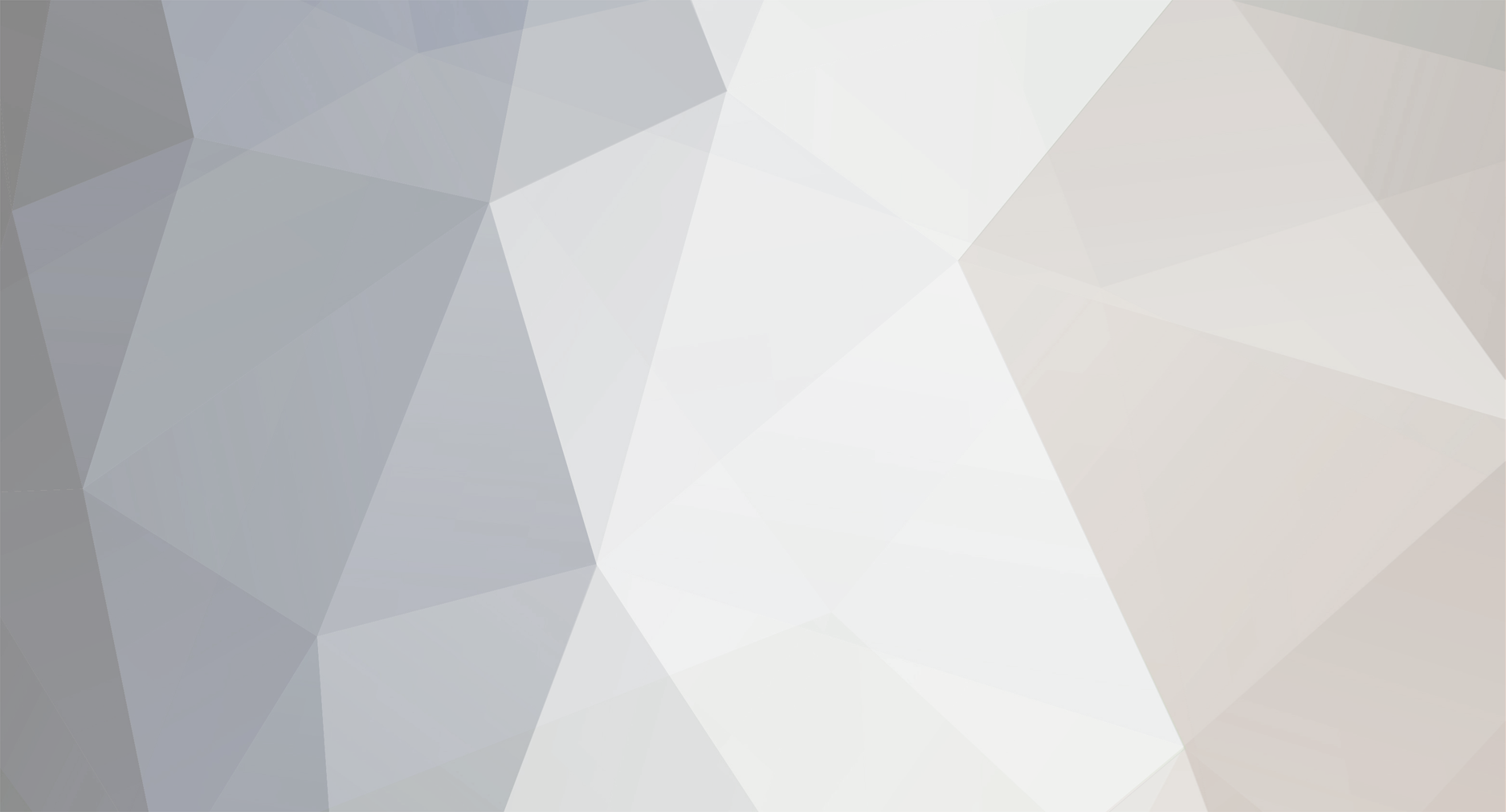
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Just be aware that it is possible for a user to view the source page of the form and send any data they like - even for hidden fields. So, always validate the sent data before using it. In this case since you are sending an email address I would suggest saving the email addresses to a database or creating an include file with an array defined with the email addresses. In the form page put the id of the email address. Then in the processing page use the ID to get the actual email address. You should never include an email address in a web page (visible or not) unless you want that page to be picked up by spam bots.
-
You don't want to "write" your own comma separated string. If there is any chance that the values would include commas or quote marks the output would not be valid. Take a look at the function fputscsv(). And, if you don't want to create a physical file (which that function requires) then look at the first user submitted comment on that function in which the author provides a solution.
-
OK, going through your code, I see some inefficiencies. The code is does a lot if looping of the records to see if there is a match with the query. The problem is that even if there is a match it continues to loop through the rest of the queries. The code below should solve that. I also added code at the beginning of the loop which should filter out non CAD entries if the CAD filter is on. I'm not guranteeing it will work since I can't test it against your data. But, it should point you in the right direction. Note: You should always add plenty of comments to your code to assist others reviewing the code and for yourself. function displayArch(query) { showList(); var html = ""; html += "<form id='archsearchform' name='archsearchform'>\n"; html += "Search Here:\n"; html += "<input type='text' id='archsearchquery' value='' style='width: 20%'></input>\n"; html += "<input type='submit' value='search' onclick='displayArch(document.getElementById(\"archsearchquery\").value);return false;'></input>"; html += "<input type='submit' value='refresh list' onclick='displayArch(\" \");return false;'></input>"; html += "<br />\n"; html += "<input type='checkbox' id='checkbox_CAD' name='CAD'>CAD Available\n"; html += "<input type='checkbox' id='checkbox_Hardcopy' name='Hardcopy'>Hardcopy Available\n"; html += "<input type='submit' value='Filter' onclick=''></input>"; html += "<br />\n"; html += "</form>\n"; html += "<br />\n"; html += "<form id='archform' name='archform'>\n"; html += "<table style='border-collapse:collapse;'>\n"; html += "<tr>\n"; html += "<td class='table_name' colspan='14'>Architectural Project Listings</td>\n"; html += "</tr>\n"; html += "<tr>\n"; html += " <td class='table_header'><input type='button' value='Add' onclick='viewAddArch()'></input></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"archive_num\";displayArch();'>Archive Number</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"project_title\";displayArch();'>Project Title</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"project_description\";displayArch();'>Project Description</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"project_num\";displayArch();'>Project Number</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"cip_num\";displayArch();'>CIP Number</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"drawing_date\";displayArch();'>Drawing Date</a></td>\n"; html += " <td class='table_header'><a href='#' onclick='sort_type=\"architectural_firm\";displayArch();'>Architectural Firm</a></td>\n"; html += "</tr>\n"; if (json.errorstr) { alert(json.errorstr); return; } //jsonArch.archives.sort(sortArch); var row; var cnt=0; var inSearch; car CAD_Filter = (document.getElementById('checkbox_CAD').value == 'CAD'); for (var c=0; c<jsonArch.archives.length; c++) { //Filter records is CAD_Filter is true if(!CAD_Filter || jsonArch.archives[c].sheets.cad == 'y') { inSearch = false; if ((query!=null) && (query!="")) { if ( (jsonArch.archives[c].archive_num.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].project_title.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].project_num.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].drawing_date.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].architectural_firm.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].project_description.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].cip_num.toLowerCase().indexOf(query)>-1) ) { inSearch = true; } if(!inSearch) { for (var v=0; v<jsonArch.archives[c].boxes.length; v++) { if ( (jsonArch.archives[c].boxes[v].box_num.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].boxes[v].box_contents.toLowerCase().indexOf(query)>-1) ) { inSearch = true; break; } } } if(!inSearch) { for (var x=0; x<jsonArch.archives[c].cds.length; x++) { if ( (jsonArch.archives[c].cds[x].book_num.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].cds[x].cd_contents.toLowerCase().indexOf(query)>-1) || (jsonArch.archives[c].cds[x].cd_num.toLowerCase().indexOf(query)>-1) ) { inSearch = true; break; } } } } if (query==null || query=="" || inSearch) { row = (c%2==0)?"row_even":"row_odd"; html += "<tr>\n"; // this is the view button (it will be in a loop) html += "<td class='" + row + "'><input type='button' value='View' onclick='loadSheets(\"" + jsonArch.archives[c].archive_num + "\")'></input></td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].archive_num + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].project_title + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].project_description + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].project_num + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].cip_num + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].drawing_date + "</td>\n"; html += "<td class='" + row + "'>" + jsonArch.archives[c].architectural_firm + "</td>\n"; html += "</tr>\n"; cnt++; } }//End If statement to filter by CAD } html += "</table>\n"; html += "</form>\n"; document.getElementById('divlist').innerHTML = html; }
-
Your query is failing. I've never seen that method of checking for a db error. Plus, even if there is one, the code continues on to the next step that would produce the error. Try this: function get_fund($ISIN) { $ISIN = mysql_real_escape_string($ISIN); $query = "SELECT * FROM fund WHERE ISIN='{$ISIN}'"; $result= mysql_query($query); if (!$result) { echo "Error:<br />" . mysql_error() . "<br />Query: {$query}"; return false; } if ( mysql_num_rows($result) == 0 ) { return NULL; } return mysql_fetch_object($result); }
-
It appears you are getting the results from an AJAX query. You need to pass the filtering criteria along with the AJAX call and do the filtering on the server-side page that retrieves the data.
-
Just create the products folder in a folder that is accessible to both sites: /products/ /www/site1.com /www/site2.com But, yes, you can have the upload copy the content to folders for both sites.
-
$clean = preg_replace("#^(\\\|/)*(.*?)(\\\|/)*$#", '${2}', $not_clean);
-
One limitation of the above code snippet is that it doesn't break the line on a full word. This is the typical behavior when getting an excerpt of a line. Otherwise you could end up with something very unintended if a word gets broken in the wrong place. Here is a function I keep in my personal library: function truncateString($string, $length, $ellipse='...') { if (strlen($string) <= $length) { return $string; } return array_shift(explode("\n", wordwrap($string, $length))) . $ellipse; } Just pass the string, the maximum length, and the value to use for the ellipse (optional, default is '...')
-
As my signature states, I do not always test the code I provide. Code I provide is to be considered a "guide" in completing your code, not somethign you can simply drop in. I also expect people to be able to debug simple errors such as this. In this case, just remove the ")" at the end of that line.
-
Really? That seems like a very poor method of doing something such as this. If the browser on the server crashes (or starts consuming gobs of memory due to a memory leak) you will have problems. If you were to provide a little more detail as to how the counter operates we can provide a much more elegant solution.
-
Did you even try the sample code I provided? It used explode() as well as list() to populate the individual values into discrete variables. You can then output in any manner you wish such as the example I provided.
-
Hmm...then I'm not sure how to interpret this statement How would you increment a hit counter without relying on visitors to the site? IF the OP is only asking how to reset the counter without relying on visitors to the site it might make sense. Even so, it would be helpful to know how he is storing the hit counter. Rather than running a cron job I would suggest storing the date along with the counter. Then the counter could be reset each day the first time it is incremented.
-
Well, unless you want to provide more specifics such as examples of the input data and the location of where the parameter will go I don't think you need regular expression. if(strpos($value, '&rel=0')===false) { $value .= '&rel=0'; }
-
Not sure exactly how you plan to use this counter. But, you could also have the counter determined dynamically in the code. For example, if you wanted the counter to start at 0 and increase by 1 every 15 minutes you could do something like this: $counter = floor((date('G')*60 + date('i')) / 15);
-
You have not given enough information for anyone to help you. I have no idea by what you mean by filtering the results. Apparently all you need to do is have a condition to do something different in a specific instance: if(jsonArch.archives.sheets.cad == 'y') { //Do what you normally do } else { //Do something different }
-
I think a better question is what EXACTLY are you trying to accomplish. All of the functions you listeed above and the filter functions all have different purposes. If you are saving data to a MySQL database, then use the function built for that: mysql_real_escape_string(). That function will "escape" the data so that it is safe for a DB query - it doesn't change the result of the input. The two html functions do the same thing. They convert/escape specific characters so they will display as intended. For example, if a user entered their name as "<b>Myname</b>" and you do not want user entered HTML code to be parsed you could use one of the two html functions to convert the greaterthan/lessthan characters to specific codes so the name will display as "<b>Myname</b>" instead of Myname The filter functions allow for many different filters. They can remove unwanted values or they may just return false if the value does not pass the filter. See this page to get an idea of the filters available: http://us3.php.net/manual/en/filter.filters.php In other words, all of the functions/filters have a specific purpose. You should pick the appropriate method for each situation. There is not one method that is superior to the others for all situations.
-
You really should change the db structure to save those address parameters in separate fields, but here is a quick fix: list($street, $city, $state, $zip) = explode(',' $addressFromDB); echo "$street<br />\n"; echo "$city, $state $zip";
-
Having an expiration date column in the table may be useful if there is ever a chance that the expiration dates could ever be something different than exactly 1 year from the registration date. For example, if a customer calls to complain about a problem perhaps a service rep would be allowed to extend their subscription or what if customers are allowed to make a two year subscription. So, I would definitely look into that as a possible solution. But, if the expiration date will always be 1 year from the registration date, you can easily display the date 1 year in the future by using strtotime() Just modify the first parameter of the date function to customize the output to your liking $registrationDate = "2010-09-05 09:58:12"; $expirationDate = date('M j, Y', strtotime("$registrationDate +1 year")); echo "Your subscription will expire on $expirationDate"; //Output: Your subscription will expire on Sep 5, 2011
-
I need a way to puch selected data to the beginning of an array.
Psycho replied to gibigbig's topic in PHP Coding Help
Well, that's helpful. Do you care to tell me "how" it didn't work such as any errors you received or how the results were not what you expected? Or am I supposed to debug the problem with my clarivoiant abilities? -
WTF?! That's such a simple and perfect solution. I just had one of those "Why didn't I think of that" moments.
-
$username = 'Psycho'; $musername = '<font color="#ff0000"><u>Psycho</u></font>'; preg_match("/(.*)$username(.*)/", $musername, $matches); $pre = $matches[1]; // <font color="#ff0000"><u> $post = $matches[2]; // </u></font>
-
I'm not entirely understanding your question, but do a return false if you don't want the return key to submit the form. Example page: <html> <head> <script type="text/javascript"> function keyDownFunction() { if(window.event.keyCode=='13') { return false; } } </script> </head> <body> <form action="here.php" method="POST"> <input type="text" name="dfsf" onkeydown="return keyDownFunction();" /> <button type="submit">Submit</button> </body> </html>
-
The page I linked to showed a method to Re-post the data without using cURL. Here is an example of what I proposed (I left a LOT of necessary code out, but this should provide an illustration): Form Page (Server #1) <form action="processing.php" method="POST"> // Form fields go here </form> Processing Page: processing.php (Server 1) if(isset($_POST)) { //Validate the POST data to ensure it is complete if(!$valid) { //Redirect back to form with error message } else { //Resend POST data to Server #2 processing page using function linked to above $result = do_post_request('http://server2/finalprocessing.php', $_POST); if($result!==true) { echo "The following errors occured: $result"; } else { echo "The record has been added"; } } } The page 'finalprocessing.php' would exist on Server #2 and would need to provide a response based on the POST data if(isset($_POST) { //Validate and process the post data. If all goes well echo "true" //else echo an error message. // //The content echo'd will be returned to the processing page on server #1 }
-
I find database operations more expensive than PHP operations. But, no matter. In the code you just posted, where are you getting the table "all_numbers_available" from? If the OP is dealing with a fixed set of number that will never change I guess you could pre-populate the table. But, if the range of numbers is constantly changing you would have to create a process that exists somewhere to conastantly update the "all_numbers_available" table. Personally, I would like to know the purpose and frequency for this process as that would detemine how much effort should be made to make this as efficient as possible.
-
Um, sure, use fsockopen() if you want. I'm not sure how you would use it to send the POST data to the processing page on Server 2. I'm sure it can be done, I've just never seen it done in that way. I think you *may* have to build a more complex service on Server 2 to make it work though. I think the solution I provided is much more elegant as you can just repurpose the POST data and send it to Server 2 directly from Server 1 instead of having it come from the user. So the user is not connecting to Server 2 at all.