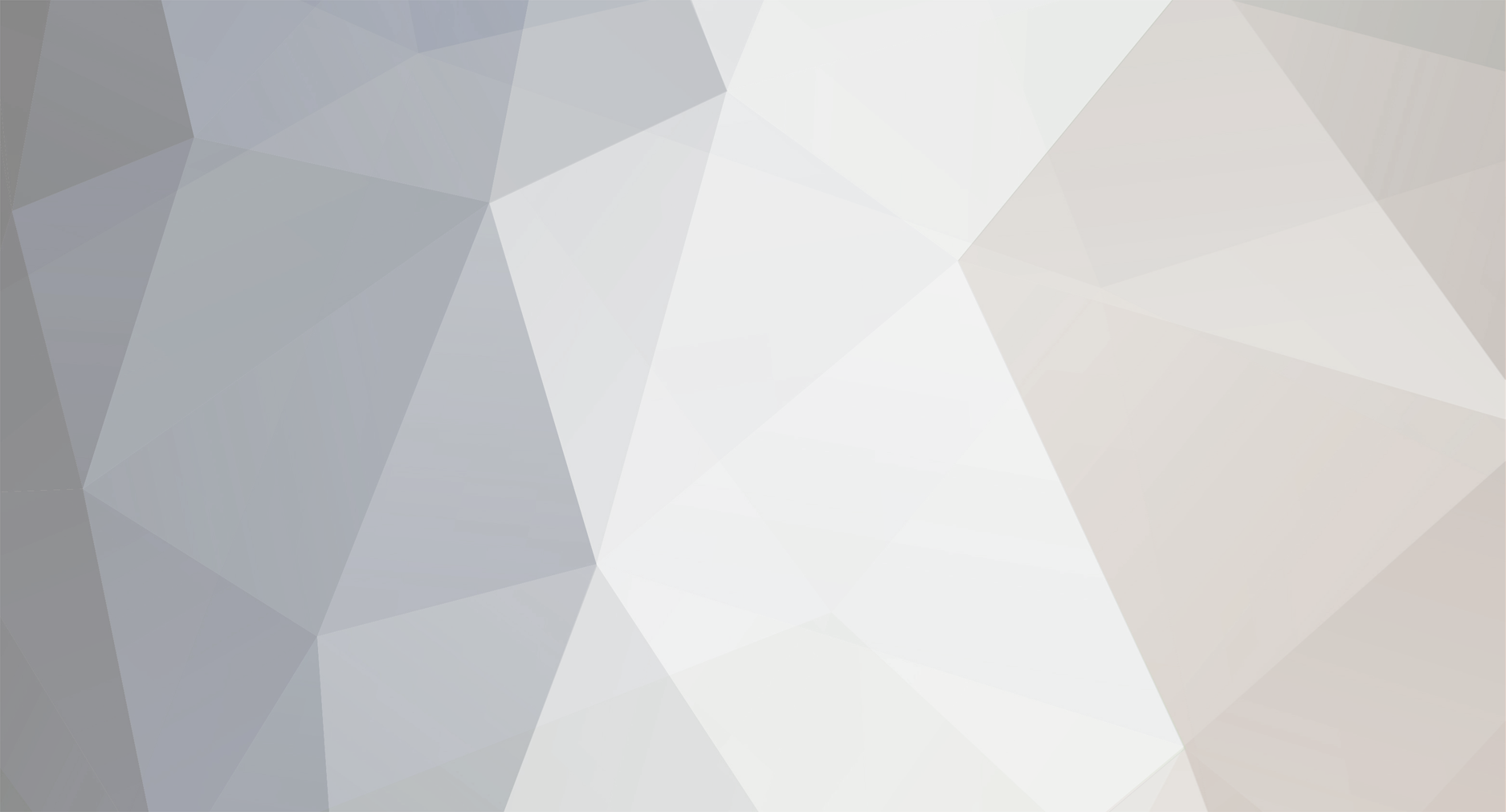
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
ProjectFear's solution is fine, but with respect to the error, you should be using preg_match() instead of ereg().
-
What do you mean it is supposed to give out an error? That query will simply update every record where the conditions exist. If there are no records that match the conditions - then no records are updated. That is not an error. You can either: Do two queries: one to see if the record exists and check the reimburse.check value. Then, if appropriate, do the update query. Or you can do the update query and afterwards check the affected row count. If 0, then you know that either no record exists with that id or the reimburse.check value is already set to 1.
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=307671.0
-
From the manual for ereg_replace(): preg_replace()
-
If you want the last 5 folders by their creation/modified date, this should work. (only tested in windows): //Specify the root directory $root_directory = "./rootfolder"; //Get list of folders in root $foldersAry = glob("{$root_directory}/*", GLOB_ONLYDIR); //Create array of folder with date $foldersByDate = array(); foreach($foldersAry as $folder) { $foldersByDate[basename($folder)] = date('Y-m-d', filemtime($folder)); } //Sort array by date arsort($foldersByDate); //Remove all but the first five folders array_splice($foldersByDate, 5); print_r($foldersByDate);
-
What do you mean by the "modified" date for folders? I know you said this was for Linux, but in Windows at least, there is no modified date for folders - only creation dates. Are you wanting the date the folder was created OR the last time something inside the folder was changed?
-
Read my sig. The only thing that jumps out at me is there needs to be a semi-colon at the end of this line: $filepath = "{$page}.{$ext}"
-
I would never use the file name/path as a GET variable. You are opening yourself up to exploitation. But,this should do what you ask: $pageExtensions = array('htm', 'html', 'php'); if(isset($_GET['page'])) { $page = $_GET['page']; //Gets the (page=) from the URL //Check page against each extension until a match is found foreach($pageExtensions as $ext) { $filepath = "{$page}.{$ext}" if(file_exists($filepath) { $site = $filepath; break; } } } if(!isset($site)) { $site = "home.html"; } include($site);
-
The only errors I see have nothing to do with JS specifically: 1. You commented out the first IF condition so the closing } before the first ELSE IF is out of context and plus you can't have ELSE IF statements without an IF statement. 2. The function is full of variables that are not defined - unless you defined them as gloabl variables somewhere else. The parameters in the function definition are named "click_???" but in the code it is "su_???"
-
Maybe I am not understanding the request - kind of confusing. But, it seems he is just wanting a query with multiple where clauses: SELECT * FROM table WHERE A = subject1 AND B = subject2 AND C = subject3 AND D = subject4 AND E = subject5 AND F = subject6
-
No offense taken. It's jsut that PHP has one of the best online manuals in my opinion and it seems people do not take advantage of it. When using a new function you should always read the manual for that function to understnad the imput parameters and the possiblel output. Additionally, right after the examples for a function is a list of related functions. I can't tell you how many times I was reviewing a function that "kind of" did what I wanted but not exactly and then found exactly what I needed in the related functions section.
-
Order array items moving underscores to front..
Psycho replied to Scooby08's topic in PHP Coding Help
Um, why didn't you SAY you wanted the non-underscore values sorted alphabetically. I stated that I assumed you wanted them sorted how they were originally so they would be in order to their numerical equivalence. If you want both the underscore and non-underscore values sorted alphabetically - that is easy. function sortUnderscoreToFront($a, $b) { if(substr($a, 0, 1)=='_' Xor substr($b, 0, 1)=='_') { //One value starts with an underscore and the other does not return (substr($a, 0, 1)=='_') ? -1 : 1; } //Both start with an underscore or both do not return strcmp(strval($a), strval($b)); } -
Doesn't anyone read the freak'n manual?! No need to create two search strings. Just use stripos() to "Find position of first occurrence of a case-insensitive string" http://us.php.net/manual/en/function.stripos.php
-
Order array items moving underscores to front..
Psycho replied to Scooby08's topic in PHP Coding Help
OK, so if you have two values with underscores, what is the logic to determine which one comes first? Here is a small function that will move values with underscores to the front, but there is no logic for putting them in any order at the front. Plus, without logic to sort the underscore values AND the other values, the current order will likely get messed up. This will put the underscore values at the beginning but will mess up the order of the other values: $testArray = array('one', '_two', 'two', '_three', 'three','_zero'); function sortUnderscoreToFront($a, $b) { if(substr($a, 0, 1)=='_') { return -1; } if(substr($b, 0, 1)=='_') { return 1; } return 1; } usort($testArray, 'sortUnderscoreToFront'); print_r($testArray); Output: Array ( [0] => _zero [1] => _two [2] => _three [3] => one [4] => three [5] => two ) If you need to maintain the order of the non-underscore values OR need the underscore values in a specific order it will require a more complex solution. -
Order array items moving underscores to front..
Psycho replied to Scooby08's topic in PHP Coding Help
You are going to have to write a custom sorter since there is no "logical" manner in which to sort the values. Those are string values, so PHP doesn't know how to interpret those strings back to their numeric values (which is apparently how you want them sorted). Without knowing all the possible values you intend to use I can't provide a full solution. BUt, I will suggest you will want to use usort() or uasort() along with a custom function. If you provide more details on all the possible values and how they should be sorted I might be able to provide some code to get you started. -
Well you have a significant flaw in the logic. You have a loop that runs for each character in the string. Then inside the loop you use preg_replace(). There is absolutely no correlation between the loop and preg_replace(). On the first iteration of the loop preg_replace() will replace the first instance of the search string - regardless if it is at the first character position or not. So, if you have a 200 character string with 5 "x" characters, all the replacements are done after the 5th iteration of the loop - but the code will run the loop 195 more times! Secondly - and more importantly - you are running queries in a loop. This is a HUGE waste of resources. Never, ever run queries in a loop. Query the data you need and process the records in PHP. Besides that loop has no purpose since you are running the same query every time without any where clause. This could be made much more efficient if you separated the string and the ID in the database. But, this shoudl work for what you have //Define search and replacement strings $searchStr = "X" $replaceStr = "replacement_": //Query all possible replacements in table and put into array $query = "SELECT `unique_id`, `unique_id_value` FROM `tableB` WHERE `unique_id` LIKE '{$replaceStr}%'"; $result = mysqli_query($link, $query); $replaceAry = array(); while ($row = mysqli_fetch_array($result)) { $replaceAry[$row['unique_id']] = $row['unique_id_value']; } //Create loop to replace each search string ONLY WHILE the search string exists $idCount = 0; while(strpos($string, $searchStr)!==false) { $idCount++; //Increment the ID $idStr = $replaceStr.$idCount; //If $idStr exists in the replaceAry echo comment to that effect if (isset($replaceAry[$idStr])) { echo "{$idStr} is on TableB and it has a value of {$idStr}<br />\n"; } //Replace the search string with replacement and unique ID $string = preg_replace($searchStr, $idStr, $string, 1); }
-
Can you please be more specific on what you are actually having trouble with? There is no way for anyone to give you code not knowing anything about your application. If you are using AJAX, you will probably need to include variables (using GET or POST) along with the AJAX request to the server. You would then code the receiving page to use those variables to perform whatever queries you need, then return output to the calling JS function to be displayed to the user. There are many tutorials out there. For us to try and teach you in a forum would be a waste of time. Just take one step at a time and if you get stuck on a specific task, then post the code you have and describe the problem you are trying to solve.
-
Just get ALL the TR sections, then throw the first one away. Assuming the input is in the variable $htmlInput, this should work for you //Get all the TR contents preg_match_all("/<tr[^>]*>(.*?)<\/tr>/ms", $htmlInput, $matches); //Set $trMatches to just the matched content $trMatches = $matches[1]; //Remove the first TR content array_shift($trMatches); //$trMatches will be an array of all the TR content except the first.
-
Using shufle() is unnecessary and reset() won't do anything productive since the array is already set to the the first element. Once you have the array of values using file(), just use array_rand() to get a random record from the array $randomRecord = $array[array_rand($array)];
-
This topic has been moved to Application Design. http://www.phpfreaks.com/forums/index.php?topic=307368.0
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=307346.0
-
I would offer two slight modifications that would make the code a little more elegant. Each has different benefits 1. Use the ternary operator. This is straitforward and can be easily implemented in your existing code. Example while ($row = mysql_fetch_array($sql)) { // Print out the contents of each row into a table $bgColor = ($row['sex']=="F") ? '#FF99FF' : '#FFFFFF'; echo "<tr bgcolor=\"{$bgColor}\">\n"; echo "<td>{$row['firstname']}</td>\n"; echo "<td>{$row['lastname']}</td>\n"; echo "<td>{$row['sex']}</td>\n"; echo "<td>{$row['time']}</td>\n"; echo "<td>{$row['place']}</td>\n"; echo "</tr>\n"; } echo "</table>"; 2. Set the colors in an array. This allows you to set the colors at the top of the script, or even in a config file, so you can change the colors without modifying the logic code. Example: $bgColors = array('F'=>'#FF99FF', 'M'=>'#FFFFFF'); while ($row = mysql_fetch_array($sql)) { // Print out the contents of each row into a table echo "<tr bgcolor=\"{$bgColors[$row['sex']]}\">\n"; echo "<td>{$row['firstname']}</td>\n"; echo "<td>{$row['lastname']}</td>\n"; echo "<td>{$row['sex']}</td>\n"; echo "<td>{$row['time']}</td>\n"; echo "<td>{$row['place']}</td>\n"; echo "</tr>\n"; } echo "</table>";
-
Um, yeah. We cannot see into your database or filesystem and know what the values are. One of the previous scripts used basename() to strip off the path information from the values returned by glob() but apparently those values still do not match what is in your database. Do the values in the database include the ".mp3"? Please show what those values look like and we can help modify the glob() results accordingly.
-
First off, you should use glob() to get the mp3 file list. Then do a query to get the ones not in the db. Then Not tested, so there may be some syntax errors <?php $dir = "/uploads/"; $mp3FileList = glob("{$dir}*.mp3"); $query = "SELECT filename FROM dj_pool"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_assoc($result)) { if(in_array($row['path'], $mp3FileList)) { unset($mp3FileList[array_search($row['path'], $mp3FileList)]); } } print_r($mp3FileList); ?>
-
Getting script to insert 40,000 rows without stopping
Psycho replied to RAELWEB's topic in PHP Coding Help
It would really help if you showed the fraking code. Here is an example of how to insert all the records in a single query statement. Of course, none of the field names would work for your exact situation since you have provided no code to work from INSERT INTO privatemessages (senderID, receiverID, message) VALUES (SELECT $adminID as senderID, userID as receiverID, $message as message FROM users WHERE userID <> $adminID)