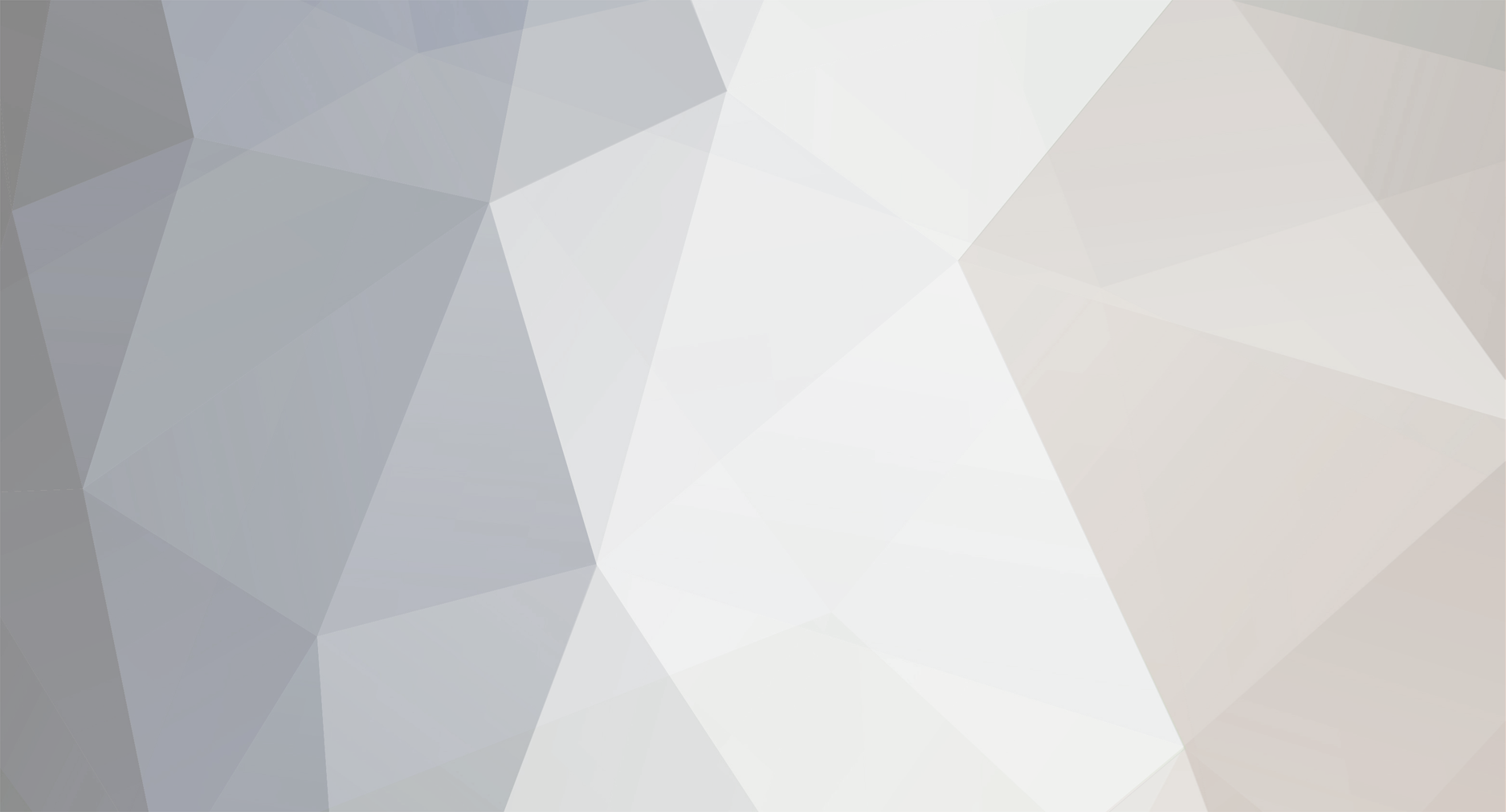
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Displaying mysql records on same div container?
Psycho replied to blacksharkmedia's topic in Javascript Help
No it does not display OK in IE & FF. If you remove the "display:none" from the DIV style properties you still won't see it. Because it is outside the page boundries - at least using the code you provided. The position is relative. So, if you place the code for that DIV where the "inline" position would be at least 475 pixels down from the top, then it will be visible. Are you purposefully setting that value to -475 for a reason or are you setting it through trial and error. If the latter you may need to reevaluate your placement/styles as there seems to be a problem. -
Displaying mysql records on same div container?
Psycho replied to blacksharkmedia's topic in Javascript Help
Seriously? You're giving the DIV a relative position of "top: -475"!!! That is 475 pixels above the viewable area of the browser window. The DIV has a height of 490 pixels. So, IF the DIV had content to completely fill the height the user would only see the bottom 15 pixels of it. The code is working perfectly - you just can't see the div because you placed it outside the viewable area. -
Huh? HTML does not use variables. HTML is just a markup language. Here is a very rudimentary example of option 1 <html> <head> <script type="text/javascript"> //Create global var var colorSelection; function selectColor(selection) { //Set global var value colorSelection = selection; //open the color picker document.getElementById('colorSelector').style.visibility = 'visible'; return; } function changeColor(colorCode) { //Set color of text or background based on global var document.getElementById('input').style[colorSelection] = colorCode; //Hide the color selector document.getElementById('colorSelector').style.visibility = 'hidden'; return; } </script> </head> <body> <span id="input">Here is the text to be modified</span> <br /><br /> <button onclick="selectColor('color');">Change Text Color</button> <button onclick="selectColor('backgroundColor');">Change Background Color</button> <br /><br /> <div id="colorSelector" style="visibility:hidden;"> <table> <tr> <td onclick="changeColor('#ff0000');" style="background-color:#ff0000;">   </td> <td onclick="changeColor('#00ff00');" style="background-color:#00ff00;">   </td> <td onclick="changeColor('#0000ff');" style="background-color:#0000ff;">   </td> </tr> </table> </div> </body> </html>
-
Displaying mysql records on same div container?
Psycho replied to blacksharkmedia's topic in Javascript Help
For each menu item create a DIV for the content to be displayed. Each div should have a name in the format: "content_1", "content_2" etc. In the menu items have an onclick event which calls a function and passes the number for each div. The function can iterate through all of the divs and set the display property for each div based upon the one which was selected. Example: <html> <head> <script type="text/javascript"> function showContent(menuID) { var index = 1; var divObj; while(document.getElementById('content_' + index)) { divObj = document.getElementById('content_'+index); divObj.style.display = (index==menuID) ? 'block' : 'none'; index++; } return; } </script> </head> <body> <div id="menu" style="border:1px solid black;float:left;"> <a onclick="showContent('1');">Menu 1</a><br /> <a onclick="showContent('2');">Menu 2</a><br /> <a onclick="showContent('3');">Menu 3</a><br /> </div> <div id="content_1" style="border:1px solid black;display:none;"> Content for menu item 1<br /> Content for menu item 1<br /> Content for menu item 1<br /> </div> <div id="content_2" style="border:1px solid black;display:none;"> Content for menu item 2<br /> Content for menu item 2<br /> Content for menu item 2<br /> </div> <div id="content_3" style="border:1px solid black;display:none;"> Content for menu item 3<br /> Content for menu item 3<br /> Content for menu item 3<br /> </div> </body> </html> -
I'm not going to read through your code to give specific assistance, but it seems pretty simple to me. You have two button, one to change the text and one to change the background. You want each button to bring up the same colorpicker. But, when the user selects a color you want it to "know" which element to change, correct? Here are two possible solutions off the top of my head: 1. Create a global variable to determine what the color will update. Then when the user chooses the button to change text color or background color, the buttons will call the function to display the color picker AND will pass a value to the function ('text' or 'background'). When the funtion to display the color picker runs it will take that passed value and set the global variable. Then when the user selects a color from the picker it will call another function (e.g. changeColor(colorID)) and pass the colorID to be set. That function will check the global variable and call the appropriate function (changeTextColor(colorID) or changeBackgroundColor(colorID)) 2. The other option is to have two color pickers. I am assuming the color picker is always there and you are just displaying/hiding it as needed. So, you could have two color pickers - one for the text and one for the background. The buttons could call the appropriate color picker and the picker will make the call the the appropriate function to change the text ot background color.
-
Here is a very rough JS only solution. The URLs must be separated by at least one line break. There could be better functionality to extract the URLs but I'm not going to spend the time. Also, there is really no validation of the URLs except to exclude blank lines. Again, this should have some more validation. If this is close to what you want then you need to look at each requirement and tackle them one by one. <html> <head> <style> input {background-color: red; } </style> <script type="text/javascript"> function showImages() { var urlListTxt = document.getElementById('urls').value; var urlListAry = urlListTxt.split('\r\n'); //Process inpur for valid urls var validURLsAry = new Array(); for(var idx=0; idx<urlListAry.length; idx++) { if(urlListAry[idx]!='') //Add condition to test for valid urls { validURLsAry[validURLsAry.length] = urlListAry[idx]; } } //Create the output var imgListOutput = ''; var url; for(var idx=0; idx<validURLsAry.length; idx++) { url = validURLsAry[idx]; imgListOutput += '<a href="'+url+'" target="_blank"><img src="'+url+'" height="50" width="50" /></a><br /><br />'; } document.getElementById('imgListDiv').innerHTML = imgListOutput; } </script> </head> <body> <textarea name="urls" id="urls" style="height:150px;width:600px;"> http://irapuato.files.wordpress.com/2009/11/cancuun.jpg http://irapuato.files.wordpress.com/2009/11/cancun.jpg http://www.mexicotravelnet.com/cancun/Cancun%20Photo3.jpg </textarea> <br /> <button onclick="showImages();">Show Images</button> <br /><br /> <div id="imgListDiv"></div> </body> </html>
-
It doesn't have to be PHP, but as I clearly stated you will need server-side code to create thumbnails. JavaScript can't do that. You could, however, use JavaScript to simply display the images on screen but at a much smaller percentage than the original. But, that can put a load on the user's browser since the entire full-size image is being loaded into memory regardless of what size you choose to display it. You also stated you wanted the user to submit the page. As soon as the page is loaded, JavaScript has no knowledge of what was submitted. That is typically what a server-side language is for. There is a workaround. You could use the GET method in your form and the information the user entered will be passed in the URL. But, it will get complicated trying to extract the information from the URL using JavaScript and to url decode it so the JavaScript on the receiving page can work with it. Also, I don't think JavaScript can validate that a URL is pointing to a valid resource. If you want to do this in JS, sure it can be done, but will be more difficult and will have some issues that cannot be resolved. But, if you want to go that route, you should have a button that initiates the JS function and NOT submit the form. Then use the JS to create the images on the same page.
-
regular expression that matches the time without the am/pm
Psycho replied to kee2ka4's topic in Regex Help
The ":" at the end was just part of my unit test code couput. It has nothing to do with the function. The function will simply return true/false based upon whether the passed value matches the pattern. -
regular expression that matches the time without the am/pm
Psycho replied to kee2ka4's topic in Regex Help
When needing a validation such as this, it is very important to have explicit, definitive rules. You gave a few examples of what should pass, but it is not enough. The easy approach would be to validate that the string starts with one or two numbers, has a colon, and then has two more numbers. But, that means 25:01 or 10:99 would pass. Is that acceptable? I don't know your situation. Also, if 00:00 is valid is 24:00 valid as well? Here is one possible solution: function validTimeStr($timeStr) { return (preg_match("/^((\d|[0-1]\d|2[1-3]):[0-5]\d)|24:00$/", $timeStr)>0); } Output of tests: 00:00: true 1:25: true 01:25: true 001:25: false 11:59: true 2:64: false 23:23: true 24:00: true 24:01: false You should test against anything else you think might be problematic. -
Oh man, that was fun. I've watched it about six times and it's still funny. Make more please.
-
Ok, this is an example where more information would have been better. It wasn't until your third post that what you are trying to achieve made sense: I was going to suggest ON DUPLICATE KEY in your query (as harristweed suggested) until I read that. The solution to this should be very simple. I will assume that the ID field for your tables is also an auto-increment field. So, the easy solution is DON'T INCLUDE THE ID FIELD IN THE INSERT QUERY. In fact, if both tables are in the same database (copy one into a temp table in the other if they aren't) then you only need a single query to combine the records into one table: Not tested, but this should be about right INSERT INTO users (col1, col2) VALUES (SELECT col1, col2 FROM users_temp)
-
It is NOT being used as a compare it is doing an assignment. That if condition will return TRUE if $result_row can be assigned a value from the mysql_fetch_row() function. Each time that function is called the next record will be retrieved and - in this instance - assigned to $result_row. So, once the last record from the result set is used a new value is not assigned to $result_row and the condition is false. Same answer as above - as each record is extracted from the result set, the pointer moves to the next record in the result set. You should take a few minutes and read the manual. Huh?! It makes prefect sense. Continue assigning the next record to $result_row until there are no more records left. Again, read the manual. What doesn't make sense in that code is the use of $i. The way it is used it will show the first field from the first record, the second field from the second record, etc.
-
Your request isn't making sense. You say you want to JOIN tables but then you are asking if you can INSERT. Based upon your examples you are trying to run the same insert statement twice. If the ID is non existent you won't get a duplicate error. I *think* you are trying to run an insert of multiple records where some of those records may be duplicates. But your question and example aren't clear. Please explain again.
-
strpos() (and its alternatives such as stristr()) may not be appropriate for this usage since they do not know when "bad" is a whole word in the string ("bad dog") vs. part of another word, ("good badger"). That function would incorrectly filter out "good badger" because of the "bad" in the word "badger". In this case you will want to use a regular expression. This is a little more flexible. The function takes the input array, an array of words to use for filtering, and an optional third parameter to determine whether you want the return value to be the array of values that are not filtered(default) or the array fo the filtered values. function filterArrayByWords($inputAry, $excludeWordsAry, $returnInclude=true) { $excludeAry = array(); $includeAry = $inputAry; foreach($includeAry as $keyInt => $valueStr) { foreach($excludeWordsAry as $excludeWordStr) { //Check for exclude word using word boundrys if(preg_match("/\b{$excludeWordStr}\b/i", $valueStr)!=0) { $excludeAry[$keyInt] = $valueStr; unset($includeAry[$keyInt]); break; } } } return ($returnInclude) ? $includeAry : $excludeAry; } $animals = array("Bad Dog", "Bad Cat", "Bad Bear", "Nice Bear", "Nice Dog", "Good Badger"); $angry_animals = array('bad', 'ugly', 'mad'); print_r(filterArrayByWords($animals, $angry_animals)); //Output: Array ( [3] => Nice Bear [4] => Nice Dog [5] => Good Badger ) print_r(filterArrayByWords($animals, $angry_animals, false)); //Output: Array ( [0] => Bad Dog [1] => Bad Cat [2] => Bad Bear )
-
Sure, but there is no one place to start and it will require more than just javascript. If you want thumbnails to be created then you will need some back-end functionality (e.g. PHP) to create the thumbnails. You need to break down all the pieces of functionality and then tackle each one separately. Here is a quick list as I see it. 1. Create form for user input 2. Processing page needs to parse the URLs into separate strings 3. Next, use PHP to attempt to "get" the images. Error handling in case the URL doesn't exist or is not an image 4. Use PHP to create a thumb of the image. 5. Using the list of URLs and thumbs create the HTML output page.
-
No worries. I did notice that I copied the error. I did fix a lot of minor HTML formatting errors in the original code and provided a more logical flow. So, I hope my time wasn't wasted. But, no matter, the OP will do what he will.
-
I really don't care if you use my code or not, but I did include code to handle when there are no results which your code does not.
-
I just gave you the solution. Do not pass a single value to the function. Instead have the function create the query string as needed. Or just create a function called submitForm() which then calls the showCD(). function submitForm() { var formData; formData += 'name=' + document.getElementById('name').value; formData += '&email=' + document.getElementById('email').value; formData += '&address=' + document.getElementById('address').value; formData += '&phone=' + document.getElementById('phone').value; showCD(formData); } Really, that tutorial is just that - a tutorial. You really should rewrite the function(s) as needed for your situation.
-
Well "Problems..." isn't very helpful is it? Why don't you actually display the error (at least during the developement phase) so you can know what to fix? You query is failing because it is misformatted. You should echo the query and the error to the page. Here is a more logic format for the page - plus it will show you the errors needed to correct. <?php include("dbinfo.inc.php"); $HTMLoutput = ''; $search = trim($_POST['search']); if (empty($search)) { //No search value was entered $HTMLoutput .= "Search string is empty.<br />Go back and type a string to search."; } else { //Perform the database query mysql_connect(localhost,$username,$password) or die ("Problem connecting to Database"); $srch="%{$search}%"; $query = "SELECT first, last, phone, mobile, fax, email, web, comment FROM contacts WHERE first LIKE '$srch' OR last LIKE '$srch' OR phone LIKE '$srch' OR mobile LIKE '$srch' OR fax LIKE '$srch OR email LIKE '$srch' OR web LIKE '$srch'"; $result = mysql_db_query("contacts", $query); if (!$result) { //There was an error in running the query $HTMLoutput .= "problems....<br /><br />"; //The following line is for debugging only and //can be commented out once in production. $HTMLoutput .= "<b>Query:</b><br />{$query}<br /><b>Error:</b><br />" . mysql_error(); } elseif(mysql_num_rows($result)<1) { //No results from query $HTMLoutput .= "There were no matches."; } else { //Query ran successfully and had results $HTMLoutput .= "Here are the results:<br><br>"; $HTMLoutput .= "<table width=\"90%\" align=\"center\" border=\"1\">\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= "<th>First Name</th>\n"; $HTMLoutput .= "<th>Last Name</th>\n"; $HTMLoutput .= "<th>Phone Number</th>\n"; $HTMLoutput .= "<th>Mobile Number</th>\n"; $HTMLoutput .= "<th>fax</th>\n"; $HTMLoutput .= "<th>Email</th>\n"; $HTMLoutput .= "<th>Web</th>\n"; $HTMLoutput .= "</tr>\n"; while ($r = mysql_fetch_array($result)) { $HTMLoutput .= "<tr>\n"; $HTMLoutput .= "<td>{$r['first']}</td>\n"; $HTMLoutput .= "<td>{$r['last']}</td>\n"; $HTMLoutput .= "<td>{$r['phone']}</td>\n"; $HTMLoutput .= "<td>{$r['mobile']}</td>\n"; $HTMLoutput .= "<td>{$r['fax']}</td>\n"; $HTMLoutput .= "<td>{$r['email']}</td>\n"; $HTMLoutput .= "<td>{$r['web']}</td>\n"; $HTMLoutput .= "</tr>\n"; $HTMLoutput .= "<tr><td colspan=\"7\" bgcolor=\"#ffffa0\">{$r['comment']}</td></tr>\n"; } $HTMLoutput .= "</table>\n"; } } ?> <html> <head> <style> th { text-align: center; background-color: #00FFFF; } </style> </head> <body> <?php echo $HTMLoutput; ?> </body> </html>
-
if ($search) // perform search only if a string was entered. $search is not defined. The value would be referenced as $_POST['search'] Add this right before the IF condition $search = trim($_POST['search']);
-
You would just change the javascript to get all the fields and create a single string for the data being sent. Here is only part of the code you need. I'm not going to write the whole thing //Create string of all the fields and values var formData; formData += 'name=' + document.getElementById('name').value; formData += '&email=' + document.getElementById('email').value; formData += '&address=' + document.getElementById('address').value; formData += '&phone=' + document.getElementById('phone').value; //... //Send the data in the ajax call xmlhttp.open("GET", "somepage.php?"+formData, true); xmlhttp.send(); However, if you are sending a whole form, is there really a need to do this in AJAX? Just submit the form normally.
-
You cannot give multiple elements the same ID. It is against the standards and has no purpose since you cannot reference all of them by the same ID. Only the last element will be assigned the ID. If you fix that problem you make the solution to this much, much easier. When creating the input fields use an incrementing counter to give all the fields unique ids in the format "num_0", "num_1", etc. Name A <input name="num[1]" type="text" id="num_0" value="12" /><br /> Name B <input name="num[3]" type="text" id="num_1" value="8" /><br /> Name C <input name="num[6]" type="text" id="num_2" value="15" /><br /> Name D <input name="num[8]" type="text" id="num_3" value="5" /><br /> Then you can use this more efficient function to calculate the total: function getTotal() { var total = 0; var idx = 0; while(fieldObj = document.getElementById('num_'+ idx++)) { total += parseInt(fieldObj.value); } return total; } Working example: <html> <head> <script type="text/javascript"> function getTotal() { var total = 0; var idx = 0; while(fieldObj = document.getElementById('num_'+ idx++)) { total += parseInt(fieldObj.value); } return total; } </script> </head> <body> Name A <input name="num[1]" type="text" id="num_0" value="12" /><br /> Name B <input name="num[3]" type="text" id="num_1" value="8" /><br /> Name C <input name="num[6]" type="text" id="num_2" value="15" /><br /> Name D <input name="num[8]" type="text" id="num_3" value="5" /><br /> <button onclick="alert(getTotal());">Get Total</button> </body> </html>
-
Server-side validation = must have Client-side validation = nice to have
-
Sure, however, the solution will be slightly different based upon where the total options come from. They should either be coming from a database or a hard-coded array. Here is one possible solution $colors = array('Red', 'Blue', 'Green', 'Yellow', 'Black', 'White'); $colorOptions = ''; foreach($colors as $color) { $selected = ($row['color']==$color) ? ' selected="selected"': ''; $colorOptions .= "<option value=\"{$color}\"{$selected}>{$color}</option>\n"; } echo "<select name=\"\">\n"; echo $colorOptions; echo "</select>\n";
-
Thanks. This serialize method for caching makes me headache . I understand how to write serialized data, but dont realize how to return data in strings. For example, I serialize category, ID, name, parent, child. And dont understand how to pull this data into strings $catID, $catName, $catDescription etc...but thats another topic. I'm not sure where you are going there. I've been slammed today and have not had time to work on this. This is really more of a MySQL problem and not a PHP problem. Here is the MySQL reference section that is at the heart of the issue: http://dev.mysql.com/doc/refman/5.0/en/example-maximum-column-group-row.html For example, you can either select only the parent categories and join the table on itself to get the assciated sub categories or you could just do a query for all the categories and subcategories creating a custom value to use for ordering the records. But the real difficulty is selecting the most recent topic along with each category in a single query. That is what the link above is about. Here is a query that will get you the parent categories, subcategories and the most recent topic post for each. Because I don't have your DB to test against I can't say there are no errors. But, hopefully it will work. Category/subcategory records should be ordered like this: Category 1 Sub Category 1-A Sub Category 1-B Sub Category 1-C Category 2 Sub Category 2-A Sub Category 2-B $query = "SELECT c.cat_id, c.cat_name, c.cat_description, c.cat_icon, c.cat_parent, c.cat_child, IF(c.cat_parent=0, c.cat_id, c.cat_parent) as firstOrderBy, t.topic_id, t.topic_subject, t.topic_last_poster, t.topic_last_post_date, COUNT(t.topic_id) AS topic_count FROM {$table_prefix}.categories c LEFT JOIN {$table_prefix}.topics t ON t.topic_id = (SELECT topic_id FROM {$table_prefix}.topics WHERE t.topic_cat = c.cat_id ORDER BY topic_last_post_date DESC LIMIT 0,1) GROUP BY c.cat_id, c.cat_name, c.cat_description ORDER BY firstOrderBy ASC, c.cat_parent ASC";