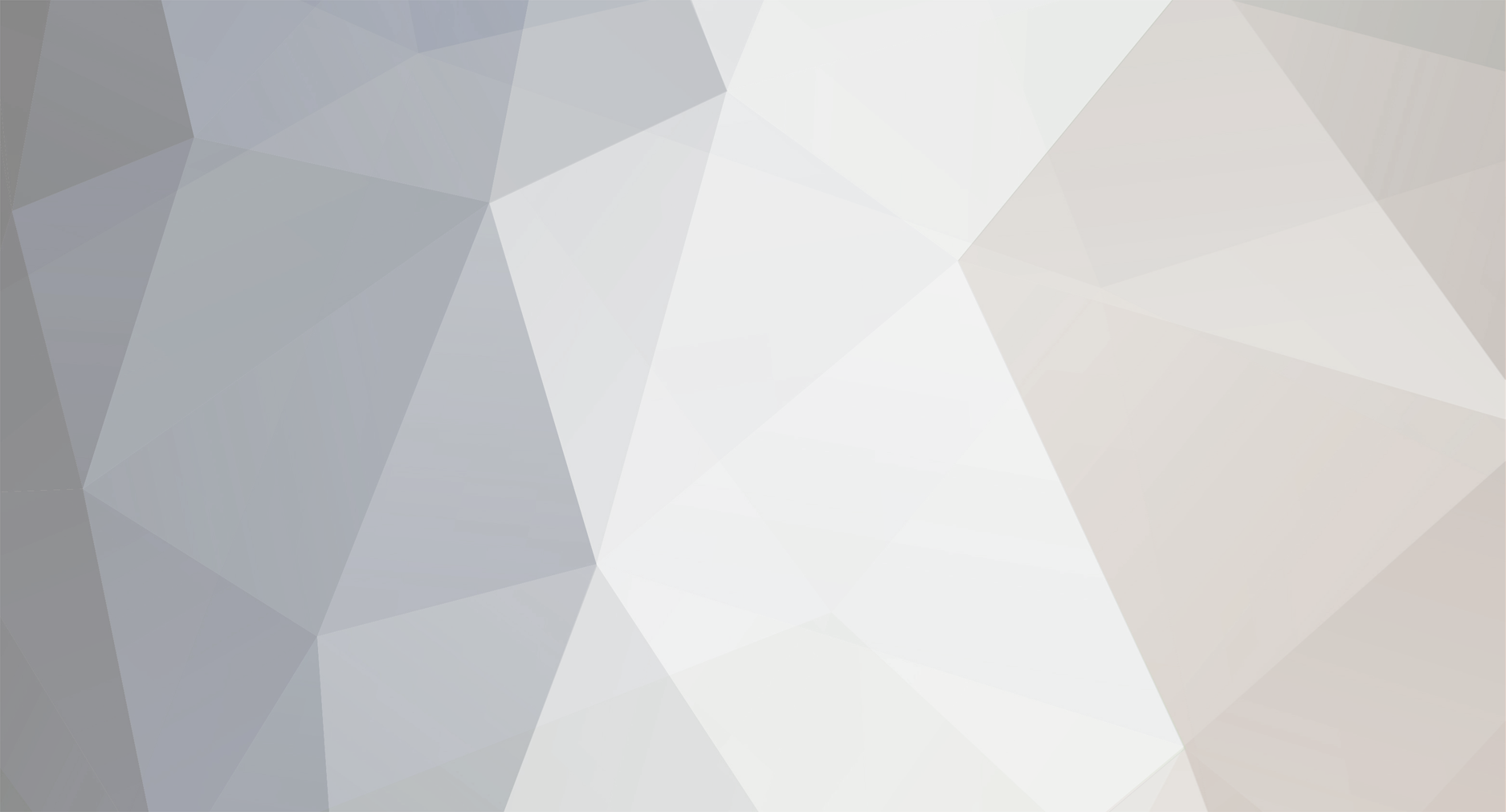
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Create multidimensional array from sql query and display in html table
Psycho replied to mazola's topic in PHP Coding Help
NOt tested, so there may be some syntax issues: <?php //Query the data $query = "SELECT p.paramname, h.year, h.dividenditem FROM `dividendparams` p JOIN `dividendhistorydetails` as h ON p.paramid = h.paramid ORDER BY p.paramname, h.year" $result = mysql_query($query); //Create arrays for the values $params = array(); $years = array(); //Proces the results while($row = mysql_fetch_assoc($result)) { $params[$row['paramname']][$row['year']] = $row['dividenditem']; if(!in_array($row['year'], $years) { $years[] = $row['year']; } } sort($years); //Create header row $output = "<tr>\n"; $output .= "<th>Parameter</th>\n"; foreach($years as $year) { $output .= "<th>{$year}</th>\n"; } $output .= "</tr>\n"; //Create the data rows foreach($params as $paramName => $data) { $output .= "<td>{$paramName}</td>\n"; foreach($years as $year) { $dividendItem = $data[$year]; $output .= "<td>{$dividendItem}</td>\n"; } } ?> <html> <body> <table border="1"> <?php echo $output; ?> </table> </body> </html> -
If you don't compare the value from the database how would you make the determination of whether to show the checkbox as checked or not to indicate the currently saved value? You should use a field type of tinyint
-
You need to define the user_id to be set. I would do something like this $userID = mysql_real_escape_string($_POST['user_id']); $page = mysql_real_escape_string($_POST['page']); $date = mysql_real_escape_string($_POST['date']); $time = mysql_real_escape_string($_POST['time']); $username = mysql_real_escape_string($_POST['username']); $remtAddr = mysql_real_escape_string($_SERVER['REMOTE_ADDR']); $subject = mysql_real_escape_string($_POST['subject']); $comment = mysql_real_escape_string($_POST['comment']); $q = "INSERT INTO `comments` (user_id, page, date, time, username, ip, subject, comment) VALUES ('{$userID}','{$page}', '{$date}', '{$_POST['time']}', '{$username}', '{$remtAddr}', '{$subject}', '{$comment}')";
-
What are the possible values for the 'air_conditioning' field from the DB? It SHOULD be 1/0 for true/false, not Y/N. Anyway, there were some other minor errors. This should work, but change the 'Y' to '1' if you get around to fixing the DB values. <?php $name = htmlentities($row['name']); $checked = ($row['air_conditioning']=='Y') ? 'checked="checked"' : ''; ?> <form id="form1" name="form1" method="post" action=""> <p> <label for="name">name:</label> <input name="name" type="text" class="widebox" id="name" value="<?php echo $name; ?>" /> </p> <p> <label for="air_conditioning">air-conditioning</label> <input name="air_conditioning" value="true" type="checkbox" id="air_conditioning"<?php echo $checked; ?> /> </p> <p> <input type="submit" name="update" value="save changes!" /> <input name="id" type="hidden" value="<?php echo $row['id']; ?>" /> </p> </form>
-
That would work, but you should not call the field "id" as it would imply it is the id for the records in that table. The field needs to be a foreign key (the id from a different table). In fact, I would keep an ID in the table with unique values only to maintain separate records in that table. So, in this case I would have an auto-incrementing "id" field in the table. Don't pass any value for it and let the ISERT statement do it automatically. But, to reference the comments back to the author, I would use a name such as "user_id" and populate that with the id of the author from the users table.
-
If it is creating a new id, then that would imply you set the field up as an auto-incrementing field - abnd very likely a unique field. I would assume that if the comment is to be associated with a user, that there can be multiple comments associated with a user. If that is the case, then you need to change the field to not be auto-incrementing and not a field that must be unique. Then you simply need to provide the ID to pupulate the field in your query. I don't see anything in the query now that provides for a user ID.
-
I think this is what you are after. It will round to the nearest value according to the $roundTo value passed function roundToPartial($value, $roundTo) { return round($value / $roundTo) * $roundTo; } echo roundToPartial(1.70, .5); //1.5 echo roundToPartial(1.80, .5); //2.0
-
I agree with what scampbell stated, always store data in it's raw format, then manipulate the data based upon how you plan to display it. That does NOT mean you should run database queries with unescaped (i.e. unclean data). You should always use mysql_real_escape_string() on all user submitted data (POST, GET, COOKIE, etc.). Then, when you retieve the values determine how the values should be used for display. For example, if you are going to display the values on the HTML page, then you may want to use htmlentities() to prevent the data from wreaking havoc on the display of the page. However, if you are populating a textarea for the user to edit the content you would want to display the data in (mostly) it's raw format and use htmlspecialchars(). Those are only suggestions. You would have to determine what is right for your specific situation.
-
Chained Select Option to number of select boxes
Psycho replied to jmr3460's topic in Javascript Help
Just create all 10 select list and hide/show the appropriate number based upon user's selection. <html> <head> <script type="text/javascript"> function showSizeOptions() { var qty = document.getElementById('quantity').value; var shirtIdx = 1; while(document.getElementById('shirt_'+shirtIdx)) { var divObj = document.getElementById('shirt_'+shirtIdx); var selObj = document.getElementById('aform').elements['size[]'][shirtIdx-1]; divObj.style.visibility = (shirtIdx<=qty) ? 'visible' : 'hidden'; if (shirtIdx>qty) { selObj.selectedIndex = 0; } shirtIdx++; } return; } </script> </head> <body> <form id="aform"> Select a number: <select name="quantity" id="quantity" onchange="showSizeOptions();"> <option value="nothing" selected="selected">Select a site</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> </select> <div id="shirt_1" style="visibility:hidden";> T-Shirt 1: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_2" style="visibility:hidden";> T-Shirt 2: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_3" style="visibility:hidden";> T-Shirt 3: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_4" style="visibility:hidden";> T-Shirt 4: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_5" style="visibility:hidden";> T-Shirt 5: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_6" style="visibility:hidden";> T-Shirt 6: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_7" style="visibility:hidden";> T-Shirt 7: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_8" style="visibility:hidden";> T-Shirt 8: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> <div id="shirt_9" style="visibility:hidden";> T-Shirt 9: <select name="size[]"> <option value="S">Small</option> <option value="M">Medium</option> <option value="L">Large</option> <option value="XL">X Large</option> <option value="XXL">XX Large</option> <option value="XXXL">XXX Large</option> </select> </div> </form> </html> -
Sorry, I misread this line if(FIELD.value.length < 4) { It was late and I misread it because of the way the original logic was built. If you are using regex for character validation, then you can incorporate a length validation in that as well. In any event, it seems you only need to validate a minimum length (because, as I stated previously, you can enforce a maximum length through the maxlength field property). So, assuming you weren't doing a regex check as well, this is all you would have needed: function valUserName(fieldObj) { fieldObj.className = (fieldObj.value.length<4) ? 'bad' : 'good'; }
-
I don't understand? can you elaborate. You state you want to validate that the value is less than 4, correct? Well, if the user enters letters it is interpreted by javascript as being greaer than 4. The isNaN() validation I provided will cause validation to fail if the uesr enters anything other than a numeric value. I made an assumption that that was appropriate in your situation, but that is for you to decide.
-
What is an "offclick"? You do realize that for an onchange event to take place you have to exit the field by clicking outside the field or pressing tab, right? I *think* what you are wanting is onkeyup or onkeydown. No need to add the check for length over 30 characters since you can enforce that on the textbox maxlength property. however, it look slike you are expecting a numeric value, so you could add validation for that: <html> <head> <style> .bad { background-color: 990000; } .good { background-color: 009900; } </style> <script type="text/javascript"> function valUserName(fieldObj) { fieldObj.className = (fieldObj.value<4 || isNaN(fieldObj.value)) ? 'bad' : 'good'; } </script> </head> <body> <input type="text" name="val" id="val" onkeyup="valUserName(this);" maxlength="30" /> </body> </html>
-
Hmm... that's not how it's usually done. If you have two people tied for first, then the next person is considered to be in third place. In your example above, the person you show in 2nd place is really in third place. Also, it would have been helpful if you had provided your current code. Anyway, here is how I would do it for the way you asked: $last_points = 0; $position = 0; while($row = mysql_fetch_assoc($reslt)) { if($last_points != $row['points']) { $position++; $last_points = $row['points']; } echo "{$position} . . . {$row['player']} . . . {$row['points']}\n"; } Or, to do it like this: Pos___Player__Points 1 ............ A ...... 200 1 ............ B ...... 200 3 ............ C ...... 180 4 ............ D ...... 150 4 ............ E ...... 150 $last_points = 0; $position = 0; $place = 0; while($row = mysql_fetch_assoc($reslt)) { $place++; if($last_points != $row['points']) { $position = $place; $last_points = $row['points']; } echo "{$position} . . . {$row['player']} . . . {$row['points']}\n"; }
-
$final_report.="The username and/or password are incorrect. <a href=\"URL\">Recover Password</a>";
-
There is one error on this line echo "It Worked" But, the OP hasn't provided the error he/she is getting, so that might not be the one referenced in the post.
-
Shuld be fixed now. Can't test since I don't have your db
-
Use javascript to set a cookie and then use PHP to see if the cookie exists.
-
And what error are you getting? (although I think I see it already)
-
I would advise against doing that. Industry standard is to simply state that the username and/or password are incorrect. This does not allow a malicious user to knwo if the username is correct or not. Otherwise, if a malicious user can try random usernames until he gets the error that the password is incorrect. Then he can start trying to hack that user's password. There's several toehr things in that code that are problematic as well from a security standpoint. Are you really storing your passwords without hashing them? Take a look at this. session_start(); include_once"config.php"; if(isset($_POST['login'])) { $username = mysql_real_escape_string(trim($_POST['username'])); $password = mysql_real_escape_string(trim($_POST['password'])); if($username == NULL OR $password == NULL) { $final_report.="Please complete both fields"; } else { $query = "SELECT * FROM `members` WHERE `username` = '$username' AND `password` = '$password'"; $check_user_data = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($check_user_data) == 0) { $final_report.="The username and/or password are incorrect"; } else { $start_idsess = $username = $get_user_data['username']; $start_passsess = $password = $get_user_data['password']; $final_report.= "<meta http-equiv='Refresh' content='0; URL=members.php'/>"; } } }
-
Can't really say without seeing the input data, but I would guess that some "records" do not have the 'textbox5' key (you know you should enclose the string key name in quotes, right?). Also, you don't need to use $i, just use []. Try this: foreach($xml as $v1) { foreach($v1 as $v2) { if(isset($v2['textbox5'])) { $name = explode(" ", $v2['textbox5']); $days = round(strtotime($v2['textbox13']) - strtotime($v2['textbox10'])) / (60 * 60 * 24); $reviewname[] = array("first_name" => $name[0], "last_name" => $name[1], "nights_stayed" => $days ); } } }
-
Random colours only coming up with shades of green, brown, orange and red
Psycho replied to Eejut's topic in PHP Coding Help
bocasz's solution seems to work much better, but has one flaw. If the number for any color space is less than 16 the resulting $hex value is only a single digit. This is probably overkill, but this seems to give expected results: function getRandHexValue() { $red = str_pad(dechex(rand(0,255)), 2, '0', STR_PAD_LEFT); $green = str_pad(dechex(rand(0,255)), 2, '0', STR_PAD_LEFT); $blue = str_pad(dechex(rand(0,255)), 2, '0', STR_PAD_LEFT); return $red.$green.$blue; } $colour = getRandHexValue(); echo "<body bgcolor='#$colour'>"; -
Random colours only coming up with shades of green, brown, orange and red
Psycho replied to Eejut's topic in PHP Coding Help
@ken, Did you ever get any results with blue? If I run the code in a loop I get some interesting results. Here is your code run in a loop 20 times: for($i=0; $i<20; $i++) { $colour = rand(0,16777215); $hex_color = dechex($colour); echo "$colour : $hex_color<br />\n"; } As you can see in the results none of the results have any "blue" value, i.e. all the hex numbers end in 00. I'm guessing this has something to do with the rand() function?? 15226880 : e85800 1960448 : 1dea00 6886400 : 691400 3536384 : 35f600 5124096 : 4e3000 4907520 : 4ae200 2010112 : 1eac00 14134784 : d7ae00 3770368 : 398800 5200384 : 4f5a00 9815040 : 95c400 14476800 : dce600 8544256 : 826000 2314752 : 235200 6708224 : 665c00 16621056 : fd9e00 8435712 : 80b800 4114944 : 3eca00 11039744 : a87400 7460352 : 71d600 -
"For" loop to check for number of DIVs on a page.
Psycho replied to ShootingBlanks's topic in Javascript Help
Sorry, I left out the 'SelectDiv' from the code: var divID = 1; while(document.getElementById('SelectDiv'+divID)){ var divObj = document.getElementById('SelectDiv'+divID); //Do something divID++; } The divObj is not technically necessary, I just assumed that you would be doing something with each div in your loop. If you don't need to do anything with the divs then you can remove it. To explain the code a little bit, the while loop will continue as long as the condition is true. So, when "document.getElementById('SelectDiv'+divID)" is in the condition it will return True if the element exists. So, starting at 1, the loop will continue incrementing divID until there is no div with the ID of "'SelectDiv'+divID" -
"For" loop to check for number of DIVs on a page.
Psycho replied to ShootingBlanks's topic in Javascript Help
A while loop is a better solution IMHO. var divID = 1; while(document.getElementById(divID)) { var divObj = document.getElementById(divID); //Do something divID++; } -
Weird problem with SELECT script. Please help!
Psycho replied to patheticsam's topic in PHP Coding Help
[Delete]