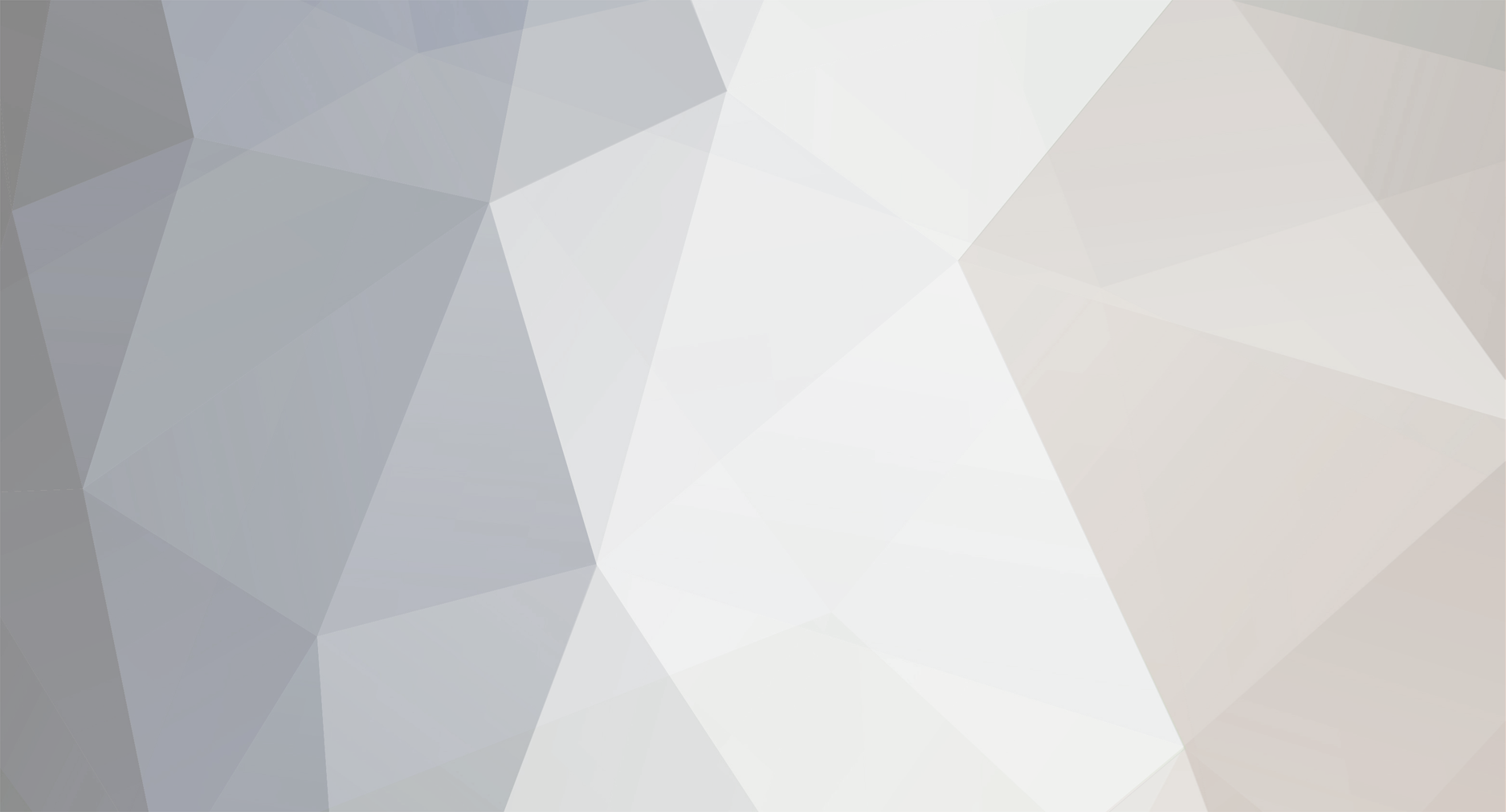
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
You could use strtotime() which will return -1 on failure. http://php.net/manual/en/function.strtotime.php
-
setting up and printing out associative array values
Psycho replied to webguync's topic in PHP Coding Help
Not sure what you are trying to do. But, according to your table structure... This will echo the question echo "{$record['Question']}<br />\n"; This will echo the section echo "{$record['Section']}<br />\n"; Assuming, of course, you have done a successful DB query and assigned the extracted results to $record. -
I'd also add that you do not need two IF statements to validate the same field. There should be an else if so if the first condition fails you do not run the second condition. If you have a validation of birth date to ensure it is not empty, then you do not need to run the validation to check the format. Example: // check to see if the user supplied a valid date of birth if (empty($_POST['Date_of_Birth'])) { $error['Date_of_Birth'] = 'Please enter your date of birth in the format of yyyy/mm/dd.'; } else if (!preg_match('/^[0-9]{4}-(0[1-9]|1[0-2])-(0[1-9]|[1-2][0-9]|3[0-1])$/', $_POST['Date_of_Birth'])) { $error['DOB_Invalid'] = 'Your date of birth is invalid. Please enter a valid date of birth in the format of yyyy/mm/dd.'; } By the way, that regular expression will allow ANY four digit year, e.g. 9999 or 0000.
-
setting up and printing out associative array values
Psycho replied to webguync's topic in PHP Coding Help
Well, first off, you are trying to echo $result1 and $result2 which would be resource identifiers from the query NOT the results. There is, however, an error in the second query. You are trying to use 'q' as a pointer for the 'Questions' table, but you didn't define it. But, since you are only querying one table you don't need it anyway. Add, some debuggingto validate the data at each step. <?php //Query for the user's worng responses $query ="SELECT `incorrect_resp` FROM `results_April2010` WHERE `user_id` = '$user_id'"; $result = mysql_query($query) or trigger_error('Query failed: ' .mysql_error()); $record = mysql_fetch_assoc($result1); //For debugging echo "Query: $query<br />\n"; echo "Records returned: " . mysql_num_rows($result) . "<br />\n"; echo "Incorrect_resp: $record['incorrect_resp']<br />\n"; //Query for the questions of wrong responses $query = "SELECT * FROM `Questions` WHERE `Q_ID` in ({$record['incorrect_resp']})"; $result = mysql_query($query) or trigger_error('Query failed: ' .mysql_error()); //For debugging echo "Query: $query<br />\n"; echo "Records returned: " . mysql_num_rows($result) . "<br />\n"; echo "Questions: <br />\n"; while($record = mysql_fetch_assoc($result)) { echo "{$record['Question']}<br />\n"; } ?> -
setting up and printing out associative array values
Psycho replied to webguync's topic in PHP Coding Help
Youreally shouldn't be storing comma se;arated values - that is what a tagble is for. Are the number in "incorrect_resp" the same as "Q_ID"? If so, here is what you could do (the LONG way). $query = "SELECT `incorrect_resp` FROM `results_April2010` WHERE `user_id` = '$user_id'"; $result = mysql_query($query); $record = mysql_fetch_assoc($result); $query = "SELECT * FROM `Questions` WHERE q.Q_ID in ({$record['incorrect_resp']})"; If you had the incorrect responses in an associative table, you could get all the information you need in a single query. -
Your example has a list of variables to be replaced and the values to use. I will ask again, how are you determining what values to use for the replacements. Are you expecting this to be automated? If you "know" the values to be replaced, then you can do something like this: $replacements = array( 'c' => 60, 'yb' => 30, 'xb' => 100, 'ya1' => 50, 'D' => 25 ); function formatReplacements($val) { return "#\b{$val}\b#"; } $find = array_map('formatReplacements', array_keys($replacements)); $input = 'c = (l1 ^ 2 - l2 ^ 2 + xb ^ 2 + yb ^ 2) / 2 ya1 = ((2 * c * yb) + SQR(D)) / (2 * (xb ^ 2 + yb ^ 2))'; echo preg_replace($find, $replacements, $input); Output: 60 = (l1 ^ 2 - l2 ^ 2 + 100 ^ 2 + 30 ^ 2) / 2 50 = ((2 * 60 * 30) + SQR(25)) / (2 * (100 ^ 2 + 30 ^ 2))
-
record is deleted whether or not condition is met
Psycho replied to webguync's topic in PHP Coding Help
I had a typo on this line (surprised it didn't create an error). $output .= ' : Records = ' . mysql_num_rows($reslt); Try fixing the variable name on that line -
You still haven't stated what "number" or numbers are being used for the replacements. Also, I don't think the logic of "...to replace all alphanumeric ( [a-zA-Z0-9] ) between math symbols or parentheses ( "+-/*^()" ) ..." will work. In your example above SQR IS between parenthesis: So, what rule would you use to differentiate "SQR" from "yb". It could be made to not replace is the value preceeds an opening paren, but if this is user entered code you are going to be really struggling for a solution. Do, you have a known list of ALL the math functions that should not be replaced? It might be easier to do this through mutliple steps instead of one preg_replace(). For example, 1) replace all the functions with a temporary value, 2) then replace all the letters and numbers, 3) lastly, replace the temp values back to the functions.
-
You need to be more explicit on "special characters". You can either specify specific characters NOT to allow or specify the characters that ARE allowed.
-
record is deleted whether or not condition is met
Psycho replied to webguync's topic in PHP Coding Help
Looking through that code there is a lot of in and out of PHP code that makes the whole page difficult to follow. Plus, it seems that page is doing an authentication. You should have a separate authenticatiuon script that is called on the pages that require it. Lastly, you are doing your DB queries two different ways, using the procedural $result = mysql_query($query) or die(mysql_error()); And using the object oriented approach $report = $db->query($sql); That makes no sense to me. Basically, the code is somewhat disorganized which is probably a contributing factor. Anyway, here is another debugging approach. Create a function to "test" for the exisitance of the record that would be deleted. Then call that function at different intervals during the script. Then you can identify where in the process the delete is taking place. I just threw this together, so it may need some work. Add this to the top of your page: function testRecords($lineNo) { $output = "{$lineNo}: "; if(!isset($_SESSION['user_id'])) { $output .= '$_SESSION[\'user_id\'] does not exist'; } else { $query = "SELECT user_id FROM test_log_April2010 USING test_log_April2010 INNER JOIN test_roster_April2010 WHERE test_log_April2010.user_id = test_roster_April2010.user_id AND test_roster_April2010.user_id = '{$_SESSION['user_id']}'"; $result = mysql_query($query); $output .= '$_SESSION[\'user_id\'] = ' . $_SESSION['user_id']; $output .= ' : Records = ' . mysql_num_rows($reslt); } return "$output<br />\n"; } Then at different point in the script add the following line: $debugOutput .= testRecords(__LINE__); Finally at the end of the script, echo the results of the debugging: echo $debugOutput; -
What is the location of the javascript files in relation to the script calling them?
-
You can't send an email with JavaScript. You can use JavaScript to fire off a PHP page to send the email (i.e. AJAX). There are many AJAX tutorials available.
-
record is deleted whether or not condition is met
Psycho replied to webguync's topic in PHP Coding Help
Again, if the ELSE condition is running you would be seeing one of those two echo conditions. If not, then that ELSE condition is not running and the DELETE is taking place somewhere else. I've been in this type of situation before and the answer always turns out to be something logical that I overlooked. If you are not seeing one of those two echo statements, post the entire page (attach to the post if it is very long). -
record is deleted whether or not condition is met
Psycho replied to webguync's topic in PHP Coding Help
If that DELETE query is running (inside the ELSE condition) then you should also be seeing one of the two echo statments if ($result_delete) { echo "Delete Successful"; }// end if else { echo "No record of taking exam"; } //end else Which one is being displayed? -
Well, I'm still lost. Can you provide an example of what you want the output of that quote to be? Do you have specific values to use as replacement for the individual patterns? Your request also assumes that you want to replace the numbers with numbers as well . Due to the lack of specifics, this regex would do what you ask: echo preg_replace("/[a-z0-9]+/", '1', $text); It replaces all alphanumeric characters with the number 1 1 = (1 ^ 1 - 1 ^ 1 + 1 ^ 1 + 1 ^ 1) / 1 1 = ((1 * 1 * 1) + SQR(D)) / (1 * (1 ^ 1 + 1 ^ 1)) However, I made it only replace lowercase letters since you want to keep the functions like SQR() which you have in caps. I can't believe that is what you want, but again, you are not giving enough information.
-
Not sure if anyone is willing to be at your beck and call whenever you have a question. But, this forum should suffice. Walk through the code you have and when you run into an issue, post here with the specifics of the problem you are having and the code in question. Be sure to identify what you expect the code to do and what it is or is not doing. I'm sure someone will provide an answer.
-
record is deleted whether or not condition is met
Psycho replied to webguync's topic in PHP Coding Help
Validate that the values used in your IF condition are what you expect them to be. I have yet to ever see an IF condition make the wrong decision - it is always a failure of the values or the condition specified: Try adding this before the IF condition for debugging purposes echo '$pcnt[$i] = ' . $pcnt[$i] . '<br />'; echo '(($pcnt[$i]*100) > 79) = ' . ((($pcnt[$i]*100) > 79) ? 'True' : 'False'); You want to take that back? -
What, exactly, are you wanting? I don't see a question being asked. This forum is for people asking for help with specific PHP code. Are you wanting someone to teach you PHP or are you wanting someone to write the code for a specific project? If the latter, then I'll move this post to the freelancing section. If the former, well, that's quite a tall order. The more specific your request, the more likely you are to get a good response.
-
Confusing mysql_fetch_row output problem
Psycho replied to OriginalSixRules's topic in PHP Coding Help
Personally, I always use mysql_fetch_assoc(), but as an alternative there is also mysql_fetch_array() which can retrieve the results as an enumerated array, an associative array or both (default). -
Why are you exploding() $list2 when it is already an array? You don't even use $tmp2 anyway. Why are you using a for() loop to look for matches? You should use array_intersect() What EXACTLY does $row['ingredients'] look like. Are there spaces after the commas? if so, you will need to trim the values after exploding. <?php //This line for testing only $row['ingredients'] = "Acetone,formaldehyde,paraben"; $toxics = array( "2-butoxyethanol","acetone","ammonia","aerosol","bleach","butylcellosolve","d-limonene", "DEA","diethyleneglyco","diethanolamine","ethoxylatednonylphenol","formaldehyde","fragrance", "methylenechloride","monoethanolamine","morpholine","NPE","NTA","naphthalene","nonylphenolethoxylate", "paraben","paradichlorobenzene","perchloroethylene","petroleum","phosphate","phosphoricacid","silica", "sodiumdichloroisocyanuratedihydrate","sodiumhypochlorite","sodiumlaurylsulfatetoluene", "trisodiumnitrilotriacetate","turpentine","xylene"); $ingredients = explode(',', $row['ingredients']); //ingredients array_map("trim", $ingredients); //Trim the values //Find matches of $ingredients in $toxics $toxic_matches = array_intersect($ingredients, $toxics); //Output the matches foreach($toxic_matches as $match) { echo "<span style=\"color:red;\">Toxics exist: {$match}</span><br />"; } ?> NOTE: this does not handle cases where there is a different in the "case" of the letters. In the above "Acetone" will not be found as a match. If you need that functionality please state so.
-
setting up and printing out associative array values
Psycho replied to webguync's topic in PHP Coding Help
Yes, all of that is possible. I only provided possible solutions. Without knowing the final schema of the database this is all just conjecture. Here is a more complete example of what the DB could look like: Table: questions Description: this table copntains one entry for each question. It is not for storing results Fields: id, question, slide_id (foreign key) Table: answers id, answer, question_id (foreign key), correct (boolean true/false) Table: Sections: Fields: id, section Table: Slides: Fields: id, slide, section_id (foreign key) Table: results Fields: user_id, question_id, answer_id Assuming the results table has all the correct and incorrect responses, here is a query that would get the question text, slide and section for all the questions the user got incorrect SELECT s.section, sl.slide, q.question FROM results r JOIN questions q on q.id = r.question_id JOIN answers a ON a.question_id = q.id AND a.correct = 1 JOIN slides sl ON sl.id = q.slide_id JOIN sections s ON s.id = sl.section_id WHERE r.answer_id <> a.id Note, none of this is tested. I may have missed something in the query, but it is possible. -
1. Create a function(s) to do the validations. In addition to including appropriate alert() messages for validation failures, the function should return a true or false based on whether validation passes. 2. In the FORM tag add an onsubmit trigger such as <form action="" name="languageform" method="post" onsubmit="return validateFunction();">
-
setting up and printing out associative array values
Psycho replied to webguync's topic in PHP Coding Help
So, why not add the section information to the database. It would be very easy to do so. 1. Create a table for the section descriptions: Table: Sections: Fields: section_id, section_name 2. Create a table for the slide descriptions: Table: Slides: Fields: slide_id, slide_name, section_id (foreign key) 3. In the questions table add a new field (foreign key) to associate each question to a slide -
What I understand of your request is that you are displaying an image that belongs to a specific category and when the user selects next you want the next image - in that category - to be displayed. I'm not going to wade through your code to try and understand it, but you would be better off having an explicit field for the sequence of the images instead of relying upon the image IDs. It would make this functionality much easier and the database format would be better off as well. Anyway, here are a couple of solutions: 1. Using your current design you could do two additional queries when displaying an image: one to get the prev photo id and one to get the next photo id. Example: $cid = mysql_real_escape_string($cid); $pid = mysql_real_escape_string($pid); //Query to get the next photo $query = "SELECT id FROM art_photos WHERE photo_category='{$cid}' AND id > '{$pid}' LIMIT 1"; //Query to get the prevphoto $query = "SELECT id FROM art_photos WHERE photo_category='{$cid}' AND id < '{$pid}' LIMIT 1"; You could then append the appropriate image IDs to the prev/next links 2. Alternatively, the next/prev link could simply pass two variables a) the current photo ID and b) either 'next' or 'prev'. Using those two variables you could first determine the category of the image that was viewed and then query for the next or prev image n that category. This would be a more efficient solution. Since you would not need to run the two queries identified in #1 each time an image is displayed. However, if you would not be able to determine if the prev/next links should be disabled in this process if the image being viewed is the first/last.
-
If these records have any importance I can't see how creating an automated process to print and then delete the records is, in any way, a good idea. The printer can't commnicate back to your script to verify if the record was actually printed or readable. Would it be feasible to create a script to create a printable versioin of all the currently unprinted records so a user can print those records - then verify that all the records were printed?