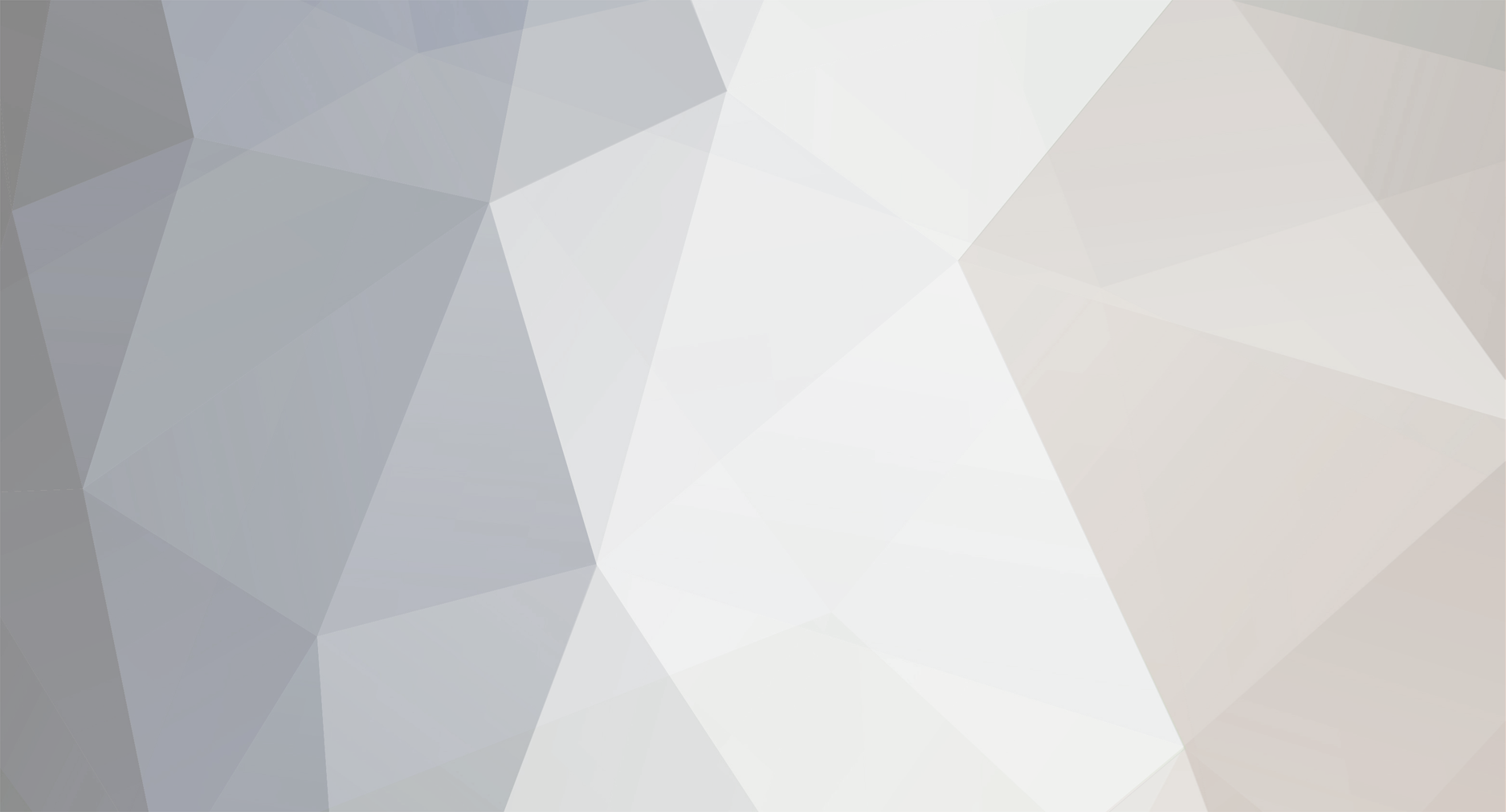
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
[This has nothing to do with Regular Expressions. Moving to PHP forum]
-
Yeah, what he said. The code I provided was only to use for illustrative purposes to see the data being returned from the query to ensure it is what you are wanting/expecting. I leave it up to you to output the results how you need them.
-
Also, stop putting "IN =". WHERE f.user_id IN = '$user->id' Use "=" when wanting to test against a single value, use "IN" when wanting to test against multiple values. You should just be using "=" here. Here is the last query you posted (with corrections) SELECT u.username, u.avatar, us.* FROM `users` AS u JOIN `user_status` AS us ON u.id = us.user_id JOIN `friends` AS f ON us.user_id = f.friend_id WHERE f.user_id = '$user->id' ORDER BY us.posted DESC Do you really need ALL the fields from the user_status table? I would suggest you try running that code to see what results you have. I notice you have no error handling on your query command. How do you expect to find and fix any errors? Although you should implement full-featured error handling that can exist in a prodduction environment, this will suffice for now: $query = "SELECT u.username, u.avatar, us.* FROM `users` AS u JOIN `user_status` AS us ON u.id = us.user_id JOIN `friends` AS f ON us.user_id = f.friend_id WHERE f.user_id = '$user->id' ORDER BY us.posted DESC"; $result = mysql_query($query) or DIE("Query:<br />$query<br />Error:<br />".mysql_error()); $first_record = true; echo "<table>\n"; while($row = mysql_fetch_assoc($result)) { if($first_record) { echo "<tr>\n"; foreach($row as $header=>$value) { echo "<th>{$header}</th>\n"; } echo "</tr>\n"; $first_record = false; } echo "<tr>\n"; foreach($row as $value) { echo "<td>{$value}</td>\n"; } echo "</tr>\n"; } echo "</table>\n";
-
The real lesson is you should NEVER do queries within loops. Doing so is a huge overhead on the sesrver. 99% of the time there is a way to get the same results with a single query. The other 1% you probably set up your database wrong to begin with. The following query should get you the same results as the multiple queries above gave you. I did this on-the-fly so I may have a typo or missed a table relation somewhere. But the logic is sound and should give you a place to start. I expect you will want to order the results in some manner. SELECT u.* FROM `users` u JOIN `user_status` us ON u.id = us.user_id JOIN `friends` f ON us.user_id = f.friend_id WHERE f.user_id IN = '$user->id'
-
Having an All check box with multiple values passed into the array?
Psycho replied to KitCarl's topic in Javascript Help
Building upon Zanus's code, here is an example using your form elements (with some corrections to the HTML) <html> <head> <style> label { font-weight: bold; } </style> <script type="text/javascript"> function checkUncheckAll(groupName, checkState) { var groupObj = document.forms[0].elements[groupName]; var groupLen = groupObj.length; for(var groupIdx=0; groupIdx<groupLen; groupIdx++) { groupObj[groupIdx].checked = checkState; } return; } </script> </head> <body> <form name="test"> <input type="checkbox" name="association[]" value="1" checked id="association_4" /> <label for="association_4">IBGA</label> <input type="checkbox" name="association[]" value="3" id="association_5" /> <label for="association_5">PBGA</label> <input type="checkbox" name="association[]" value="2" id="association_6" /> <label for="association_6">NEHBA</label> <br /><br /> <input type="checkbox" name="checkAll" value="x" id="checkAll" onclick="checkUncheckAll('association[]', this.checked)" /> <label for="checkAll">Check All</label> </form> </body> </html> -
Persoanlly, I think determining if the style has already been seleted previously has no value. Just state that the selected ID has been set. But, in any event, here is a better way to do what you are after function defaultstyle($id) { //Set new style to enabled mysql_query("UPDATE themes SET enabled = '1' WHERE id = '{$id}'"); //Set all others to disabled mysql_query("UPDATE themes SET enabled = '0' WHERE id <> '{$id}'"); //Determine if the new id was already set if(mysql_affected_rows()==0) { echo "<center>This theme is already set to default</center>"; } else { echo "<center>The theme $style[name] is now the default theme for the CMS</center>"; } }
-
By learning how to do it the right way. There is absolutely no need to do a loop. Besides, that's a pretty lame attempt at trying to update all the records since it would be possible for an id number to be skipped if you were ever to delete a record. And, that just might be your problem. To update every record, simply leave off the WHERE clause UPDATE themes SET enabled = '0'
-
You need to remove the extra 'as', plus add DESC to order from high to low SELECT concepto, COUNT(concepto) As conceptocount FROM tbl_ventas GROUP BY concepto ORDER BY conceptocount DESC
-
I'm guessing one of the "images" in the DB query is not an image or has the wrong extension. FYI: A jpg with a gif extension will typically display in most applications but would fail if you tried to read it as a jpg in PHP.
-
You have no closing braket on the IF condition. But, I would clean that code up by doing this: <?php $cpage = $_GET['cpage']; $cpage_list = ('intro', 'directions', 'hours', 'jam', 'pointe', 'about', 'tc', 'privacy'); if (!in_array($cpage, $cpage_list) && $xlspg !='contact_us') { echo "<div id=\"contain2\">" . $this->crumbTrail->Render() . "</div>"; } else { echo "<img class=\"img1\" src=\"css/images/bcreplace.jpg\" width=\"695\" height=\"29\" alt=\"bcreplace\" />"; } ?>
-
Getting Started with a Caching system? How to use it and so on?
Psycho replied to jordz's topic in PHP Coding Help
There are many different caching methods and the correct one would depend on many factors. BUt, for what you are asking I would do the following: Create a secondary table ("top_photos") with columns for "day", "week", "month", etc. As well as a field for "last_updated". When loading a page where you want to display those photos. That table would only ever have one record. When loading a page where you need the top photos you would query that table for the record. Before using the data, however, the code would check the last_updated timestamp. If more than 24 hours old it would qwuery the tables with the actual data to programatically determine the top photos for day, week, month and then update the record in the "top_photos" table. So, you would only need to query the single record in that table to get the top photos - which should be very fast. Only once a day would you run the more intensive query to update that table. -
A couple comments. 1. Have you checked the values in the database to see if the BR tags are there? 2. You are doing this all wrong. You should save the user's original input to the database without modification (except for mysql_real_escape_string()). You would then modify the data when displaying it on screen according to how it is being displayed. If you are displaying in "one the page" then you would want to use nl2br() and possibly something like htmlentities(). But, if you want the user to edit the data, then you would use it to populate a textarea without those modifications. Just a thought, but does the text in the textarea have actual line breaks OR are you considering the word wrapping, due to the width of the teatarea, as line breaks.
-
That would retrieve the first two results. The query Ken2K7 posted first gets you the results you asked for. Are you saying you can't figure out how to display them in the order you want? $query = "SELECT * FROM table ORDER BY id DESC LIMIT 2"; $result = mysql_query($query); $lastTwo = array(); while($record = mysql_fetch_assoc($result)) { $lastTwo[] = $record; } $lastTwo =array_reverse($lastTwo); foreach($lastTwo as $record) { echo $record['fieldName1']; echo $record['fieldName2']; //etc }
-
That code makes no sense. The first query gets ALL names from the DB, but only extractst he first one. The 2nd query serves no purpose. Then you compare the input name with the first name extracted from the first query. You only need one query $name = mysql_real_escape_string($db['name']); $query = "SELECT name FROM ".PREFIX."cup_all_clans WHERE name='$name'"; $result = safe_query($query); if(mysql_num_rows($result)>0) { echo "There is already a team with the name '$name'!"; } else { //not a duplicate, proceed }
-
[Moved to MySQL forum]
-
Still not working . . . how? What is it doing and how does it deviate from what you want it to do?
-
if(strtotime($_POST['Date_of_Birth'])==-1) { //invalid date }
-
You haven't provided a definition of what you consider to be an "invalid" date. Your regular expression ensures the date is in the "format" you want of yyyy-mm-dd. And, I already provided a means to validate that the "date" can be translated into a valid calendar date using strtotime(). So, what else are you needing? The years 0001 and 9999 ARE valid years. But, based on your application they may not be. If you are asking the user to enter the birthdate of biblical people or when future relatives will be born anything is possible. Personally, I think you are going about this all wrong. Let the user enter anything they want as the date and use strtotome() to validate and use date() to format to your specifications.
-
wish to know how to reuse JS for multiple calanders in same page
Psycho replied to jasonc's topic in Javascript Help
I'm not going to read through all of that code above, but the solutioin is pretty simple. You just need an onclick event for each field. The event can be attached to the actual field or to a button. But, the onclick event should call the function to display the calendar AND pass the ID of the field where the data should be populated. You can then use that to populate the correct field. There are many free scripts available if you do some searching that can do this for you. But, if you are interested in doing this yourself here is a very rough script to illustrate the idea to get you started. <html> <head> <script language="JavaScript"> var fieldObj, calendarObj; function setDate(date) { fieldObj.value = date; calendarObj.style.display = 'none'; } function pickDate(fieldID) { fieldObj = document.getElementById(fieldID); calendarObj = document.getElementById('calendar'); calendarObj.style.left = fieldObj.offsetLeft; calendarObj.style.top = fieldObj.offsetTop+25; calendarObj.style.display = ''; } </script> </head> <body> Date 1: <input type="text" name="date[]" id="date_1" onclick="pickDate(this.id)" readonly="readonly" /> Click the field to set the date<br /> Date 2: <input type="text" name="date[]" id="date_2" readonly="readonly" /> <button onclick="pickDate('date_2');">Pick a Date</button> Click the button to set the date<br /> Date 3: <input type="text" name="date[]" id="date_3" onclick="pickDate(this.id)" readonly="readonly" /> <button onclick="pickDate('date_3');">Pick a Date</button> Click button or field to set the date<br /> <div id="calendar" style="position:absolute;z-index:1;top:0;left:0;background-color:yellow;display:none;"> <a href="#" onclick="setDate(this.innerHTML)" >1</a> <a href="#" onclick="setDate(this.innerHTML)" >2</a> <a href="#" onclick="setDate(this.innerHTML)" >3</a> <a href="#" onclick="setDate(this.innerHTML)" >4</a> <a href="#" onclick="setDate(this.innerHTML)" >5</a> <a href="#" onclick="setDate(this.innerHTML)" >6</a> <a href="#" onclick="setDate(this.innerHTML)" >7</a><br /> <a href="#" onclick="setDate(this.innerHTML)" >8</a> <a href="#" onclick="setDate(this.innerHTML)" >9</a> <a href="#" onclick="setDate(this.innerHTML)" >10</a> <a href="#" onclick="setDate(this.innerHTML)" >11</a> <a href="#" onclick="setDate(this.innerHTML)" >12</a> <a href="#" onclick="setDate(this.innerHTML)" >13</a> <a href="#" onclick="setDate(this.innerHTML)" >14</a> </div> </body> </html> -
Just use LIMIT starting at $second_count+2 with an arbitrary large number for the second parameter. $com=mysql_query("select * from comments where msg_id_fk='130' and com_id > '4' order by com_id limit $second_count+2, 999999"); EDIT: I *think* you can add values in a limit clause. If not you will just need to do the addition before you create the query.
-
This seems to be a solution, but you will need to install lame on the web server: http://snippets.dzone.com/posts/show/5126 Note: I have not tired it and tale no responsibility for it.
-
how do i echo out the field names from the database?
Psycho replied to silverglade's topic in PHP Coding Help
Well, that will get you the field name from the database - which is not what you want. You want user friendly labels. I hope you noticed I changed mysql_fetch_array() to mysql_fetch_assoc() and am using the field names when referencing the record values. This is much more intuitive in my opinion. -
how do i echo out the field names from the database?
Psycho replied to silverglade's topic in PHP Coding Help
// 4. Use returned data while ($row = mysql_fetch_assoc($result)) { echo "Widget Corp: {$row['About_Widget_corp']}<br />\n"; echo "Products: {$row['Products']}<br />\n"; echo "Services: {$row['Services']}<br />\n"; } -
You want to use ORDER BY and LIMIT. SELECT field FROM table ORDER BY field DESC LIMIT 1
-
Writing a print function that draws from an array
Psycho replied to alecsloman's topic in PHP Coding Help
Put this in menus.php file function printmenu($array) { foreach($array as $value) { echo "<li>$value</li>"; } } In index.php you would do this include('menus.php'); printmenu($menu[main]);