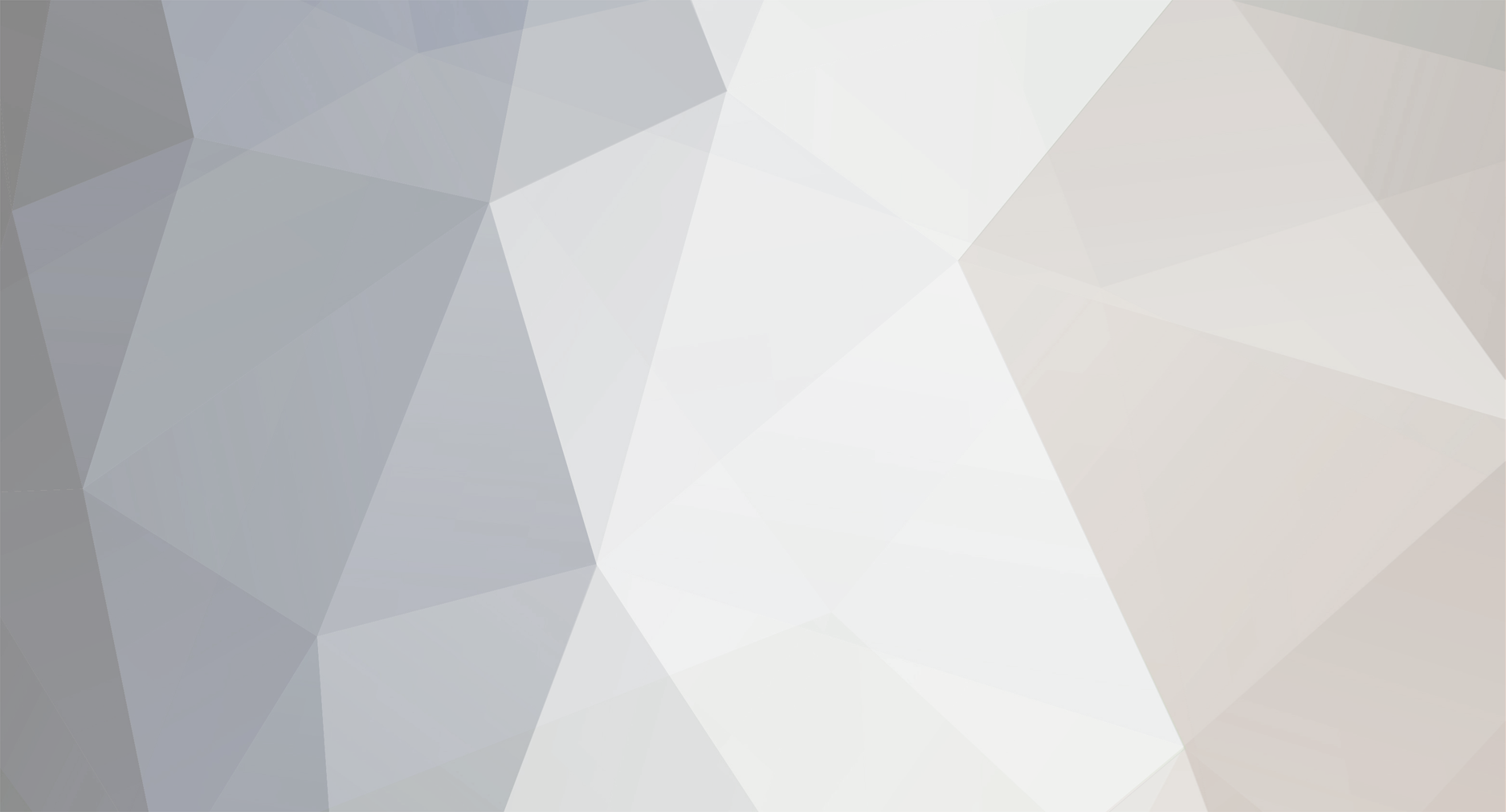
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Well, I'm not quite sure why you are getting "those" results. I get different errors, but I know why - daylight savings time. If that is your problem you can fix this by setting the time to the middle of the day. And you can get the correct results with one function using a more "natural" conversion of "-2 day". echo Date("Y-m-d", strtotime("2010-03-30 12:00:00PM -2 day"));
-
I made some modifications to that code to help in readability and to make more efficient. You shouold really avoid GLOBAL if at all possible. And this is not one where it is needed - at all <?php function makegrid($rows, $cols) { $grid = array(); for($row=0; $row<$rows; $row++) { for($col=0; $col<$cols; $col++) { $grid[$row][$col] = rand(0,1); } } return $grid; } function gridtable($grid) { $tableHTML = "<table border=\"0\">\n"; foreach($grid as $row) { $tableHTML .= "<tr>\n"; foreach($row as $col) { $tableHTML .= " <td width=\"10\">{$col}</td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "</table>\n"; return $tableHTML; } $gridArray = makegrid(10, 10); $puzzle = gridtable($gridArray); ?> <html> <body> <h1>Make a grid</h1> <?php echo $puzzle; ?> </body> </html> Although, unless you need to use the grid array for other purposes, I would suggest combining the funtions into one. function makegrid($rows, $cols) { $tableHTML = "<table border=\"0\">\n"; for($row=0; $row<$rows; $row++) { $tableHTML .= "<tr>\n"; for($col=0; $col<$cols; $col++) { $cellContent = rand(0,1); $tableHTML .= " <td width=\"10\">{$cellContent}</td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "</table>\n"; return $tableHTML; }
-
If you are not using ALL of the columns for the table, then specify the columns you will be using in the SELECT statement instead of using *. Using * is inefficient and is bad practice in many cases. $query = "SELECT * FROM `phdp_en` WHERE `title` LIKE '%$kw%' OR `body` LIKE '%$kw%'"; $result = mysql_query($query); if (mysql_num_rows($result) > 0) { //There were results echo "<ul dir=\"ltr\">\n"; while ($row = mysql_fetch_array($result)) { //view lines go here } echo "</ul>\n"; }
-
I don't think the problem is your variable - I think it is just the regex expression in general. But, I would suggest: 1) Testing the regex with a hard coded value first to ensure it is working properly, THEN introduce the variable 2) creating the expression as a string variable outside of the actual preg_match. then you can echo the expression to the page for debugging purposes. Without seeing what you are using for the $type there's no way to really know what the problem is. Although I do see some potential problems. 1. You use this right after the opening A tag "(.*)". That is a 'greedy' match, so it will likely match more characters than you want since the parser doesn't know when to end. you should probably use the question mark as you do in the previous usage 2. You then follow that by a space. Don't know if that is intentional or not. 2.
-
You can't add a value to HTML content after you have written that content to the page. You just need to add a GROUP BY clause to your query as well as specifically set the values to obtain - don't rely on *. Also, there is no need to get the number of rows and performa a while loop on that. And you should never, never do queries within loops. I don't even see what you are using the $pay result for. $query = "SELECT invoice_id, date, SUM(total) as invoiceTotal FROM si_invoices LEFT JOIN si_invoice_items ON si_invoices.id = si_invoice_items.invoice_id WHERE si_invoices.customer_id='$_SESSION[user_id]' GROUP BY invoice_id ORDER BY invoice_id" $result = mysql_query($query); $row=0; while ($record = mysql_fetch_assoc($result)) { $class = 'd' . ($row % 2); $inv_date=substr($record['date'], 0, -9); echo "<tr class=\"{$class}\">"; echo "<td valign='top'>{$inv_date}</td>"; echo "<td valign='top'>{$record['invoice_id']}</td>"; echo "<td valign='top'>${$record['invoiceTotal']}</td>"; echo "<td><a href='invoices/Invoice{$record['invoice_id']}.pdf' target='_blank'>"; echo "<img src='../images/pdf_icon.gif' width='17' height='17' alt='PDF File' border='0' /></a></td>"; echo "</tr>"; $row++; }
-
I agreee with teamtonic that you should simply strip out the other characters and validate that you have 7 (?) numbers. Although, a regex expression would strip out ALL characters that aren't numbers. If validation fails, populate the original value in the textbox. if validation passes store just the numbers in your database. $phone = preg_replace('/[^\d]/', '', $_POST['phone']); if(strlen($phone)!=7) { //validation failed } else { //validation passed } However, you should always be very careful about manipulating user input when the user is not aware. Also, this does resrict users from entering letters. It is commonly acceptable for people to use letters in their phone numbers to represent the numbers on the keypad.
-
This has been asked and answered many times on this forum. Here's a modification of your code that is cleaned up and more secure <select name="leadprodcategory"> <?php $userID = mysql_real_escape_string($_SESSION['userid']); $query = "SELECT catname FROM category where userid = '{$userID}' ORDER BY catname"; $result = mysql_query($selectLeadProductCatQuery) or die(mysql_error()); while($record = mysql_fetch_assoc($result)) { $selected = ($record['catname'] == $_SESSION['leadProductCategory']) ? ' selected="selected"': ''; echo "<option value=\"{$record['catname']}\"{$selectred}>{$record['catname']}</option>\n"; } ?> </select>
-
Personally I would do this with two regex expressions just to make it easy to work with, but it can be done in one. I'll give you both solutions. These will validate that the password: - meets the minimum and maximum lengths - only contains the allowable characters - has at least one character from each "class" (upper, lower, num&special) Example One: function pwCheck($password, $min, $max) { $validChars = "/^(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9!@#$%^&*()_.-])[A-Za-z0-9!@#$%^&*()_.-]{{$min},{$max}}$/"; return (preg_match($validChars, $password)); } Example Two: function pwCheck($password, $min, $max) { $validChars = "/^[A-Za-z0-9!@#$%^&*()_.-]{{$min},{$max}}$/"; $classCheck = '/(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9!@#$%^&*()_.-])/'; return (preg_match($validChars, $password) && preg_match($classCheck, $password)); } The key of the "class" check is a non-consuming regular expression: (?=.*[a-z]) This means match any lower case letter that follows any other character. By using one of these for each class you get what you are after.
-
Check all and Uncheck all - What am I doing wrong?
Psycho replied to TeddyKiller's topic in Javascript Help
Are you even making an attempt at implementing those changes? Your response was made only seven minutes after my post. This is a "help" forum you know. <html> <head> <script type="text/javascript"> function checkAll(formName, fieldName, state) { var checkObjs = document.forms[formName].elements[fieldName]; if (!checkObjs.length) { //There is only one checkbox checkObjs.checked = state; } else { //There are multiple checkboxes for(var i=0; i<checkObjs.length; i++) { checkObjs[i].checked = state; } } disableCheckLinks((state)?'checkAll':'checkNone'); return; } function disableCheckLinks(optionID) { document.getElementById('checkAll').style.display = (optionID=='checkAll') ? 'none' : ''; document.getElementById('checkNone').style.display = (optionID=='checkNone') ? 'none' : ''; return; } function checkState(formObj, fieldName) { var checkObjs = formObj.elements[fieldName]; for(var i=0; i<checkObjs.length; i++) { if (!checkObjs[i].checked) { //At least one checkbox is unchecked disableCheckLinks('checkNone'); return; } } //All checkboxes are checked disableCheckLinks('checkAll'); return; } function initCheckBox() { checkState(document.forms['myform'], 'list[]'); } window.onload = initCheckBox; </script> </head> <body> <form name="myform"> <input type="checkbox" name="list[]" value="1" onclick="checkState(this.form, 'list[]');" /> One<br /> <input type="checkbox" name="list[]" value="2" onclick="checkState(this.form, 'list[]');" /> Two<br /> <input type="checkbox" name="list[]" value="3" onclick="checkState(this.form, 'list[]');" /> Three<br /> <input type="checkbox" name="list[]" value="4" onclick="checkState(this.form, 'list[]');" /> Four<br /> <br /> <a href="#" id="checkAll" onclick="checkAll('myform', 'list[]', true);" style="display:none;">Check All</a> <a href="#" id="checkNone" onclick="checkAll('myform', 'list[]', false);" style="display:none;">Uncheck All</a> </form> </body> </html> -
Check all and Uncheck all - What am I doing wrong?
Psycho replied to TeddyKiller's topic in Javascript Help
Yeah, I was cleaning up some things when I finished up testing that code. The intent was for the checkbox fields to be named "name[]" so they could be picked up as an array by the procfessing page. If you leave them as "name" you will only get the value of the last checked value in the receiving page. To address your second request, I don't think that makes much sense. Assume the user clicks the "Check All" link. All the checkboxes get checked and the link changes to "Uncheck All". So far, so good. But, what if the use then manually unchecks only one of the options? Shouldn't there be a "Check All" option at that point? If not, what happens when the user then manually unchecks each individual field one-by-one and the "Uncheck All" option is displayed and they are all already unchecked? You would have to monitor every single individual checkbox selection to detemine what to show. Doesn't seem worthwhile, or logical, to me. But, since you ask and I am bored: <html> <head> <script type="text/javascript"> function checkAll(formName, fieldName, state) { var checkObjs = document.forms[formName].elements[fieldName]; if (!checkObjs.length) { //There is only one checkbox checkObjs.checked = state; } else { //There are multiple checkboxes for(var i=0; i<checkObjs.length; i++) { checkObjs[i].checked = state; } } disableCheckLinks((state)?'checkAll':'checkNone'); return; } function disableCheckLinks(optionID) { document.getElementById('checkAll').style.visibility = (optionID=='checkAll') ? 'hidden' : ''; document.getElementById('checkNone').style.visibility = (optionID=='checkNone') ? 'hidden' : ''; return; } function checkState(formObj, fieldName) { var checkObjs = formObj.elements[fieldName]; var firstState = checkObjs[0].checked; for(var i=0; i<checkObjs.length; i++) { if (checkObjs[i].checked != firstState) { //All checkboxes are not the same, enable both links disableCheckLinks(); return; } } //All checkboxes are the same, enable one link disableCheckLinks((firstState)?'checkAll':'checkNone'); return; } </script> </head> <body> <form name="myform"> <input type="checkbox" name="list[]" value="1" onclick="checkState(this.form, 'list[]');" /> One<br /> <input type="checkbox" name="list[]" value="2" onclick="checkState(this.form, 'list[]');" /> Two<br /> <input type="checkbox" name="list[]" value="3" onclick="checkState(this.form, 'list[]');" /> Three<br /> <input type="checkbox" name="list[]" value="4" onclick="checkState(this.form, 'list[]');" /> Four<br /> <br /> <a href="#" id="checkAll" onclick="checkAll('myform', 'list[]', true);">Check All</a> <a href="#" id="checkNone" onclick="checkAll('myform', 'list[]', false);">Uncheck All</a> </form> </body> </html> -
Check all and Uncheck all - What am I doing wrong?
Psycho replied to TeddyKiller's topic in Javascript Help
OK, I'm not sure what your problem is. As long as all the fields are named "list" (and not "list[]") then it should work. FYI: When appending [] to a field name (e.g. "name[]") the field is handled as an array in PHP as "name" (without the brackets). but in JS, the array element is literally "name[]" (with the brackets). One other possible problem is that code would not work if there was only one checkbox. Since I expect these are created dynamically there is a possibility that there may be a single checkbox in some situations. I would also not create two functions to check/uncheck. Just create a single function and pass a parameter. Here is some working code for you to work with: <html> <head> <script type="text/javascript"> function checkAll(formName, fieldName, state) { var checkObjs = document.forms[formName].elements[fieldName]; //In case there is only one checkbox if (!checkObjs.length) { checkObjs.checked = state; return; } //When there are multiple checkboxes for(var i=0; i<checkObjs.length; i++) { checkObjs[i].checked = state; } return; } </script> </head> <body> <form name="myform"> <input type="checkbox" name="list" value="1" /> One<br /> <input type="checkbox" name="list" value="2" /> Two<br /> <input type="checkbox" name="list" value="3" /> Three<br /> <input type="checkbox" name="list" value="4" /> Four<br /> <br /> <a href="#" onclick="checkAll('myform', 'list[]', true);">Check All</a> <a href="#" onclick="checkAll('myform', 'list[]', false);">Uncheck All</a> </form> </body> </html> -
Check all and Uncheck all - What am I doing wrong?
Psycho replied to TeddyKiller's topic in Javascript Help
What do your checkboxes look like? -
Hmm... are you talking about abbreviating a person's name? If so, you aren't going to be able to do this with RegEx. You would need to build a library/table of names and their applicable abbreviations. The abbreviations for many/most names cannot be determined programatically: Michael = Mike Robert = Bob Anthony = Tony etc.
-
Well, why are you using LIKE? If the value of $kodsekolah is something like just the letter 'a', then that query would update the password for ALL records containing the letter 'a' in that field. I have no idea what that value is supposed to represent. You also don't explain how that session value is set. But, that is most likely where the problem lies. By the way, what is this line for? $_SESSION['kodsekolah']; It won't do anything.
-
Well, that sucks. Was there supposed to be a question in there, or perhaps some code for us to look at? Let's see the the block of code that does the update.
-
Not interested in wading through all of that code, especially when I have a two line function that will validate an email format: function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]*[a-z\d])?(\.[a-z\d]([a-z\d-]*[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } Here are the details of the exact validation properties: // NOTES: // // Format test // - Username: // - Can contain the following characters: // - Uppercase and lowercase English letters (a-z, A-Z) // - Digits 0 to 9 // - Characters _ ! # $ % & ' * + - / = ? ^ ` { | } ~ // - May contain '.' (periods), but cannot begin or end with a period // and they may not appear in succession (i.e. 2 or more in a row) // - Must be between 1 and 64 characters // - Domain: // - Can contain the following characters: 'a-z', 'A-Z', '0-9', and '-' (hyphen). // - There may be subdomains, separated by a period (.), but the combined domain may not begin with a period // and they not appear in succession (i.e. 2 or more in a row) // - Hostname parts may not begin or end with a hyphen // - TLD accepts: 'a-z' & 'A-Z' // // Note: the domain and tld parts must be between 4 and 256 characters total // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character
-
OK, I'm not going to "weed" through all of your code, but I'll give you a direction to follow. Yoo already have a "template" of sorts with the HTML code. So, the rest is fairly simple. All you need to do is run the query at the top of the page. Then, if you need to "modify" any of the returned results (e.g. If a field is empty and you want it to state something like "Pending") then do that as well. Then simply echo the values int eh HTML. Here is a simple example: <?php $user_id = (int) $_GET['user_id']; $query = "SELECT name, phone, addr1, addr2, city, state, zip FROM users where user_id = '{$user_id}'"; $result = mysql_query($query); $record = mysql_fetch_assoc($result); $name = htmlentities($record['name']); $phone = htmlentities($record['phone']); //Create the address output $addressAry = array(); if (!empty($record['addr1']) { $addressAry[] = htmlentities($record['addr1']); } if (!empty($record['addr2']) { $addressAry[] = htmlentities($record['addr2']); } //Create & format the City, ST ZIP line if (!empty($record['city'] || !empty($record['state'] || !empty($record['zip']) { $cityStZip = ''; $cityStZip .= htmlentities($record['city']); if (!empty($record['city'] && !empty($record['state']) { $cityStZip .= ", "; } $cityStZip .= htmlentities($record['state']); if (!empty($cityStZip) && !empty($record['zip']) { $cityStZip .= " "; } $cityStZip .= htmlentities($record['zip']); $addressAry[] = $cityStZip; } $addressStr = implode("<br />\n", $addressAry); ?> <html> <body> Name: <?php echo $name; ?><br /> Phone: <?php echo $phone; ?><br /> Address:<br /> <?php echo $addressStr; ?> </body> </html>
-
<html> <head> <script type="text/javascript"> var rotatingTextElement; var rotatingText = new Array(); var ctr = 0; var rotateTime = 5000; var rotateTextProcess; function initRotateText() { rotatingTextElement = document.getElementById("textToChange"); rotatingText[0] = rotatingTextElement.innerHTML; // store the content that's already on the page rotatingText[1] = "Ten Reason to Buy Gumballs"; rotatingText[2] = "White House bla bla"; startRotateText(); } function startRotateText() { rotateTextProcess = setInterval(rotateText, rotateTime); } function pauseRotateText() { clearInterval(rotateTextProcess); } function rotateText() { rotatingTextElement.innerHTML = rotatingText[++ctr%rotatingText.length]; } window.onload = initRotateText; </script> </head> <body> <span id="textToChange" onmouseover="pauseRotateText()" onmouseout="startRotateText()">New Release... Leopard</span> </body> </html>
-
Need help with this short piece of my script... It is breaking my page!
Psycho replied to physaux's topic in PHP Coding Help
Well, I would expect there to be "some" error. In this case I would expect a PHP and/or MySQL error followed by an error about not being able to send headers after output has been sent to the browser. Here is one place that should definitely produce an error: if(mysql_num_rows() == 1){ That command has a required parameter of the resource link. It should be: if(mysql_num_rows($results) == 1){ I see some other problems as well. In the ELSE condition you are trying to do an insert of $chosenlink, but you define that in the IF condition. Also, this line is missing a semi-colon: $rowoutput = mysql_fetch_array($results) Also, why are you deleting the records. If you have a timestamp, then formulate the queries in your application to ignore records older than x days. -
[Moved topic to JavaScript forum] This requires client-side code (i.e. JavaScript). For changing the color you would just need a simple image-swap function. Just google for it and I'm sure you will find plenty. For the text you will need to utilize CSS layers along with the JavaScript. That site has quite a bit of complexity - especially with regard to the font handling. I'd suggest just sticking to basic text for now.
-
That is exactly the problems I was referring to. You can make that much more efficient by not running a query for each file individually.
-
'IF' you can help on this, that would be great
Psycho replied to turn_left_at_mars's topic in PHP Coding Help
I think you need some more opening and closing PHP tags! Is that really how you write your code or is that being generated from DreamWeaver or some other app? It's just difficult to try and "read" that code to understand what is taking place. Anyway, I see that you have hard-coded the "2" in several places. Are you sure you have changed the "2" to a "3" in ALL the places needed? You would be better served by creatng a variable to hold the column count. Then you can change the columns by changing that one variable instead of trying to hunt down all the modifications needed. Ok, after reading the code a little bit, I think I see the problem. In all of the conditions to check which row you are on, the value of $i is being increased: Line 6: <?php if ($i++%2==0): ?> Line :32 <?php if ($i++%2==0): ?> Line : 40 <?php if ($i++%2==0): ?> The first two of those are in the foreach loop. That means $i is getting incremented twice for each record. Instead of incrementing $i in the conditinoal statements, just add a $i++ on the first line aftyer the foreach loops and change your conditionals to just check $i (without incrementing it). Also, define a variable at the top of the script for $max_columns. Then in your conditionals you could use <?php if ($i++%$max_columns==0): ?> Now you can change the number of columns used by chnaging that variable. -
Ok, if I understand you correctly you are trying to identify all the files which are no longer associated with existing forum posts. I am guessing that you are doing a db query against each file individually to see if the file is still listed in the db. If so, runiing many individual queries is very inefficient. Are you also generating an array of all the physical files each time? There is definitely a more efficient method. Here is what comes to mind: 1. Get an array of ALL the physical files on the server using glob() 2. Run a single query to get ALL the file names that are in the database 3. Use the db results to generate an array of valid files 4. Use array_diff() to generate an array of ALL the files that do not exist in the database 5. Run whatever process you need to against the values in the list of invalid file. If this list is too long to complete in a single run, then add the array of invalid files to a temporary table where you can process x number of records at a time. There is no need to manually check each file individually. Example code: <?php //Get array of ALL files on the server $filesOnServer = glob("path/to/files/*.*"); //Create array of ALL files in the DB $filesInDB = array(); $query = "SELECT filename FROM post_files"; $result = mysql_query($query) or die(mysql_error()); while($record = mysql_fetch_assoc($result)) { $filesInDB[] = $record['filename']; } //Create array of ALL files that exist on server //but do not exist in DB $filesNotInDB = array_diff($filesOnServer, $filesInDB); ?>
-
Yes. I was able to read several thousand folders and add tem to the queue. If you are processing against an array, I suppose you could iterate through the array using something like this: foreach($filesList as $idx => $value) { $_SESSION['idx'] = $idx; //Do something with the value } But, that is terribly inefficienet since you have to rebuild the array each time the page loads. Plus, it is problematic if any folders change between page loads. You could probably avoid that by storing the folder path in the session and doing an array_search to find the last record processed. I have a feeling you may be making this more complicated than it needs to be. Without nowing exactly what "manipulations" you are doing I can't say for sure, but it's possible you could run a single query using the array of filenames.
-
ignace's solution should allow the script to run until completion. But, if this is on a hosted serer your host may have other means in place to limit execution time and/or the host may not take kindly to you running memory intensitive scripts for long periods. If that solution does not work for you, here is another option which I have used in processing a lot of files (e.g. scanning my mp3 collection to read the metadata of every file). I created a two part process. The first step was to create a script to read all the folders and subfolders in the root of the music directory and put them into an array. At the end I would run a single query to create a scan queue. The second step was a script which would get the next folder in the queue and read/process the files. I used AJAX on the "processing" page to keep calling the script until the process was complete This also allowed me to create a progress bar. If you have a large number of files in a single directory you could still do something similar to have x number of files processed on each call to the script.