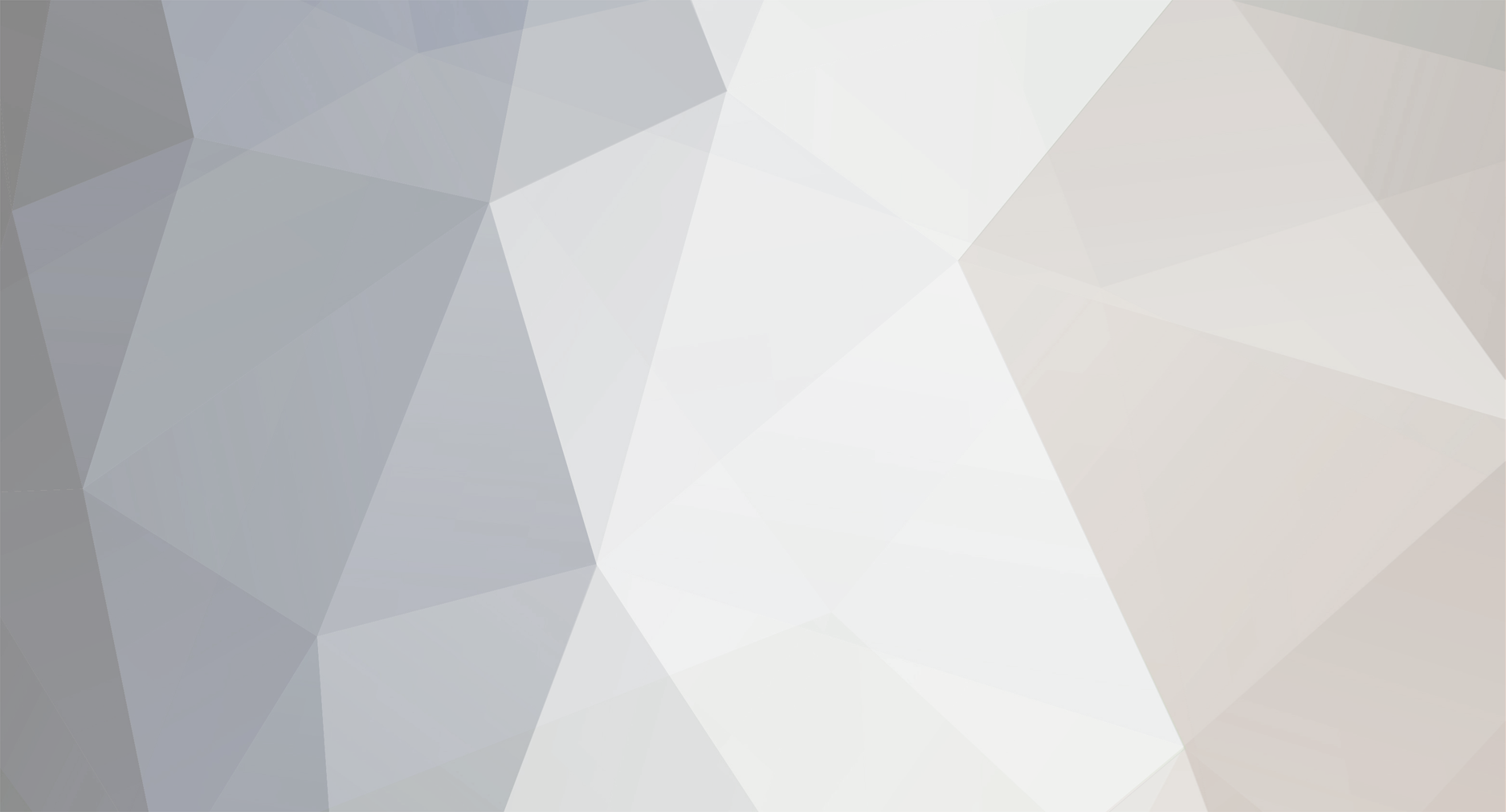
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
I'm pretty sure the problem is with the first conditional in the getBetsFor() function. if ($this->bets === null) { $ret = queryf('SELECT * FROM bets WHERE username = %s ORDER BY date DESC LIMIT %d', $this->username, MAX_DISPLAY_BETS); If I am reading this right, you are only running the query if $this->bets has not been set. So, on the second call you make to that function $this->bets (which has scope in the class) has been set so the query doesn't run. Then, the only code that does run in that function is this $this->bets = $bets; return $this->bets; $bets has scope only in the function, so on the second run it is not getting set from the query because of the initial conditional so it is a null value. So, the end of the query replaces the previous value of $this->bets with a null value.
-
You want the textarea to change how? The code has nothing in it that would change the textarea. The code is trying to set options for a textarea? There is not such thing. Are you trying to populate the textarea? Honestly, I can't figure out what it is supposed to do. Please explain.
-
Here is a rough example of how you could build the Form page and the Processing page in one. This allows you to redisplay the form (with the submitted values) when there is a validation error. <?php $name = trim($_POST['name']); $email = trim($_POST['email']); $business = trim($_POST['business']); $number = trim($_POST['number']); $message = trim($_POST['message']); $error = ''; if (isset($_POST['name']) && (empty($name) || empty($email) || empty($number) || empty($message))) { $error .= "<p>You appear to not have filled in the necessary fields.</p>"; } else { //validation passed, create and send the email $headers .= "MIME-Version: 1.0rn"; $headers .= "Content-Type: text/html; charset=ISO-8859-1rn"; mail($to, $subject, $message, $headers); //redirect to success page header('Location: http://www.example.com/message_sent.php') exit(); } ?> <html> <body> <?php echo $error; ?> <form action=""> *Name: <input type="text" name="name" value="<?php echo $name; ?>" /><br> *Email: <input type="text" name="email" value="<?php echo $email; ?>" /><br> Business: <input type="text" name="business" value="<?php echo $business; ?>" /><br> *Number: <input type="text" name="number" value="<?php echo $number; ?>" /><br> *Message:<br /><textarea name="message"><?php echo $message; ?></textarea><br> <button type="submit">Send Email</button> </form> </body> </html>
-
Tags to display my PHP & Also exporting to a PDF
Psycho replied to Gilly79's topic in PHP Coding Help
NOt sure what you mean exactly. echo and print are the common functions for outputting content to the browser. You are free to putput the content in any manner you want - in talbes or not. Just determine how you want your output and build your pages accordingly. As for PDF, you can use PHP to build PDF pages. -
Why would you do that? One query is ALWAYS better than running multiple queries. Here is a quick example of how you could produce a multi-company report from a single query <?php $query = "SELECT * FROM orders WHERE company IN ('company1', 'company2', 'company3') ORDER BY company, order_date"; $result = mysql_query($query); $currentCompany = ''; $grandTotal = 0; while($order = mysql_fetch_assoc($result)) { //Detemine if this is a new company if ($order['company'] != $$currentCompany) { //Display last company sub total if ($currentCompany!='') { echo "<b>Subtotal for {$currentCompany}: $companySubTotal</b><br /><br />\n"; } //Reset sub total vars $companySubTotal = 0; //Display company header echo "<h1>Subtotal for {$currentCompany}: $companySubTotal</h1><\n"; } $companySubTotal += $order['order_total']; $grandTotal += $order['order_total']; echo "{$order['order_date']} : {$order['product_name']} : {$order['order_total']}<br />\n"; } //Display last company subtotal echo "<b>Subtotal for {$currentCompany}: $companySubTotal</b><br /><br />\n"; //Display grad total for all companies echo "<b>Grand total: $grandTotal</b>\n"; ?>
-
Just check whatever variables you are using in the process of the invoice and reset all of them on each foreach loop of clients.
-
The configuration in the php.ini file need to point to a mailserver on which you can send email. By default, a XAMPP installation expects that you are running a mailserver on your local machine, thus the localhost entry. The XAMPP package also includes the Mercury mailserver application. You can run that along with the Apache server or you can change the php.ini setting to point to another mailserver.
-
Well, I doubt you are going to find a more efficinet method than the functions that already exist - which you have already used. There may be shortcuts you could implement if you are only using some of the data from the arrays, but it would be specific to your needs.
-
Well, I was only modifying the code to output data to the screen so I could see some of the internal variables to see what was going on. When it started to work I undid my changes, and it continued to work. So, the problem was not "fixed" by anything I did. That is why I assume it has something to do with the session variables.
-
Upon initial inspection it did seem to present the same problem, but then after playing around with it for a while it seemed to work correctly. I have to assume that it has something to do with the SESSION variables. I haven't had time to dive into it more. Personally, it wouldn't be worth my time. I could probably rewrite a better solution in less time than it would take to fix that code. But, I just don't have the time right now.
-
Is there some reason you can't store the data in an associated table insted of serializing it?
-
If the arrays have absolutely NO relation to one another (i.e. don't have common values) and you will NEVER need to query based upon those values the go ahead and serialize them. But, if that is not the case, then you should definitely store them in a separate, associated table. I wouldn't think you would need multiple tables (unless you are talking about multiple fields with different arrays). Why is that? It will be more efficient to have a separate table with the associated data and to get it in a single query than to have to query serialized values and then to unserialize it on-the-fly. Not to mention you lose the integrity of your data. Are you not comfortable using JOINs?
-
Impossible to say what the problem may be. If it is not reaching the end, then it is likely there is an error encountered in what is taking place in the loop. You didn't provide any details, but I have to assume the loop is processing data. Could be there is a problem with some of the input data that the script is not handling correctly. You should implement some logging to help pinpoint the problem. At the very least you could log the value of $i (or whetever your real counter is) so you will know what the last record processed is. Or you could go further and log the actual data being processed - if that is feasible. However, it would be interesting to know exactly what your processing consists of. If any script is taking more than 5 hours to finish, then even the smallest performance improvements can reap huge benefits.
-
There have been numerous posts on this issue in the past. Just search if you are interested in reading them. THe bottom line is that MD5() is perfectly fine for password hashing - just use a salt, just like you would for any other algorithym. Those who state that MD5() has been "cracked" do so based upon the existence of rainbow (i.e. lookup/dictionary) tables. Anyone can create a table of hashed values using any algorithym for known values. That is why it is important for users to use "strong" passwords. The more complex a password becomes, the probability that the value is NOT in a lookup table raises exponentially.
-
So, check the actual error and/or check the query. I always advise against building the query directly inside the mysql_query() command. Build the query as a string variable so you can echo it to the page if needed: $query = "INSERT into art_glass (name, price, thumb_url, big_url) VALUES ('$name', '$price', '$thumb_url', '$big_url')" mysql_query ($query, $connectID) or die ("Query:<br />$query<br />Error:<br />".mysql_error());
-
If you properly indent the code to show structure you probably would have noticed that the function doesn't having a closing curly bracket. function check() { if(document.getElementById('id1').checked == true) { alert("number 1 checked"); } else { alert("number 1 not checked"); } By the way, there's no reason to use "== true" int he coparison. When doing an IF condition, the whole point is to determine if the conditions equal true. So just testing "document.getElementById('id1').checked" would be more efficient.
-
if($tags = @get_meta_tags($url)) { echo "worked"; } else { echo "failed"; }
-
OK, I'll let it slide . . . this time!
-
Remove created hidden input when delete is clicked
Psycho replied to hcdarkmage's topic in Javascript Help
Well, there is a much simpler solution than creating hidden fields. Change the select list to a multiple select field. Then on submission of the form use JavaScript to select all of the options. Then you don't have to muck around with all these hidden fields. -
Remove created hidden input when delete is clicked
Psycho replied to hcdarkmage's topic in Javascript Help
Well, what you are asking is pretty easy, but there are some problems with that code already. Plus, when posting JS problems it is much more helpful to post the "processed" code. When including the pre-processed server-side code it is much more difficult to work with. Some of the problems I see are: 1. Select OPTIONS don't have names, only the SELECT tag has a name. 2. You are using the same id for the options "options[]". You can't use the same ID for more than one element on a page. You don't need an id for the optinos, so take it out as well. 3. There is a bug. If you don't change the value in the input field and press teh add button multiple times, it creates multiple hidden fields, but doesn't add an additional vlaue to the select list. I modified the function to clear the field after an add. Not entirelysure what you are using all the hidden fields for. But, I think a better approach would be to use a single hidden field and store all the select options as a comma separated list whenever the select list changes. Anyway, here are changes to your current approach <tr id="tbrowOption" style="display:none"> <td valign="top"><? echo $lang['contactform']['fieldoptions'] ?></td> <td> <table border=0 cellpadding=0 cellspacing=0 width=98%> <tr> <td> <input name="optionvalue" id="optionvalue" style="width:160px"> <input type="button" value="<? echo $lang['contactform']['add'] ?>" onclick="addOption();"> <input type=button value="<? echo $lang['contactform']['del'] ?>" onclick="deleteOption()"> </td> </tr> <tr> <td><select size=5 name="options1" id="options1" style="width:230px"> <?php if (($pageaction=="edit" || $pageaction=="update") && $field_id !=""){ foreach($options as $value){ echo "<option value=\"{$value}\">{$value}</option>"; } } ?> </select> <?php if (($pageaction=="edit" || $pageaction=="update") && $field_id !=""){ foreach($options as $value){ echo "<input type=\"hidden\" name=\"options[]\" id=\"{$value}\" value=\"{$value}\" />"; } } ?></td> </tr> </table> </td> </tr> function trimString(input) { var output = input; while (output.substring(0, 1) == ' ') { output = output.substring(1, output.length); } while (output.substring(output.length - 1, output.length) == ' ') { output = output.substring(0, output.length - 1); } return output; } function addOption() { var textbox = document.getElementById('optionvalue'); var listbox = document.getElementById('options1'); var inList = false; textbox.value = trimString(textbox.value); var myOption; if(textbox.value == '') { return false; } for(var i=0; i<listbox.options.length; i++) { if(textbox.value.toLowerCase() == listbox.options[i].text.toLowerCase()) { textbox.value = ''; return false; } } myOption = new Option(textbox.value, textbox.value); listbox.options[listbox.options.length] = myOption; createhidden(textbox.value); textbox.value = ''; return true; } function createhidden(vValue) { var input = document.createElement("input"); if (vValue != '') { input.setAttribute("type", "text"); input.setAttribute("name" , "options" + "[]"); input.setAttribute("value", vValue); input.setAttribute("id", vValue); document.getElementById("thisform").appendChild(input); } } function deleteOption() { var listbox = document.getElementById('options1'); var listLen = listbox.length; for(var i=0; i<listbox.options.length; i++) { if(listbox.options[i].selected) { deletehidden(listbox.options[i].value); listbox.options[i] = null; } } } function deletehidden(vValue) { if(document.getElementById(vValue)) { var deleteObj = document.getElementById(vValue); deleteObj.parentNode.removeChild(deleteObj); } return; } -
OK, good thing you got it. I was about to write a post calling you an idiot for not reading my explanation.
-
Could it be because of this: $comment->photographid = (int)$photoid; //<==== $comment->created = strftime("%Y-%m-%d %H:%M:%S", time()); $comment->author = $author; $comment->body = $body; You are defining a variable $comment->photographid, and it may be looking for $comment->photo_id. Not sure how you are building the array of values, but if it is pairing up index names with variable names it seems there is a mismatch. Also, FYI: if the id field is an auto-increment, there is no need to include it in your INSERT query EDIT: Yes, I think that is the problem, look at this protected function attributes(){ $attributes = array(); foreach(static::$db_fields as $field){ $attributes[$field] = $this->$field; } return $attributes; } The $db_fields includes array('id', 'photo_id', 'created', 'author', 'body'); But, your defined fields don't include a $this->photo_id Change the references for "photographid" to "photo_id"
-
Also, that code could use some work. glob() would be a much better solution than reading all the files in that directory. Plus, using explode() to determine the extension is a poor choice. Give this a try <?php $current_dir = "includes/videos/"; // Location to read files from. $flvFiles = glob($current_dir."*.flv"); $fileList = ''; foreach($flvFiles as $file) { if (is_file($file)) { $fileName = basename($file); $fileList .= " <tr>\n"; $fileList .= " <td><a href=video_player.php?video=" . rawurlencode($fileName) . "> {$fileName} </a><br /></td>\n"; $fileList .= " <td><a href=delete_video.php?video=" . rawurlencode($fileName) . "> Delete </a><br /></td>\n" $fileList .= " </tr>"; } } if ($fileList == '') { $fileList = "<tr><td>No tutorial files in current directory</td></tr>\n"; } ?> <html> <body> <p><h1>Video Tutorials:</h1></p> <hr><br /> <table> <?php echo $fileList; ?> </table> <hr><br /> </body> </html> <?php //delete_video.php $current_dir = "includes/videos/"; // Location to read files from. $file = rawurldecode($_GET['video']); unlink($current_dir.$file); header("location:view_videos(admin).php"); ?>
-
Also, the second error is due to the fact that the first error is outputting content to the page. You can't do a header() after any content is output to the page. Fixing the first error should resolve the second as well. As Jagual pointed out, you are not setting the path to the file. In the first script you use $current_dir = "includes/videos/"; // Location to read files from. $dir = opendir($current_dir); // Opens the directory. But in the delete script there is no path specified. It doesn't "know" to look for the file in the "includes/videos/" directory.
-
Yes, but it depends what you are trying to achieve. If you are wanting to allow the user to not select a value, then set that option to an empty value. However, if you want to force the user to select one of the values, then still set that option to an empty value and then do a validation on the server to ensure the value of that field !-''