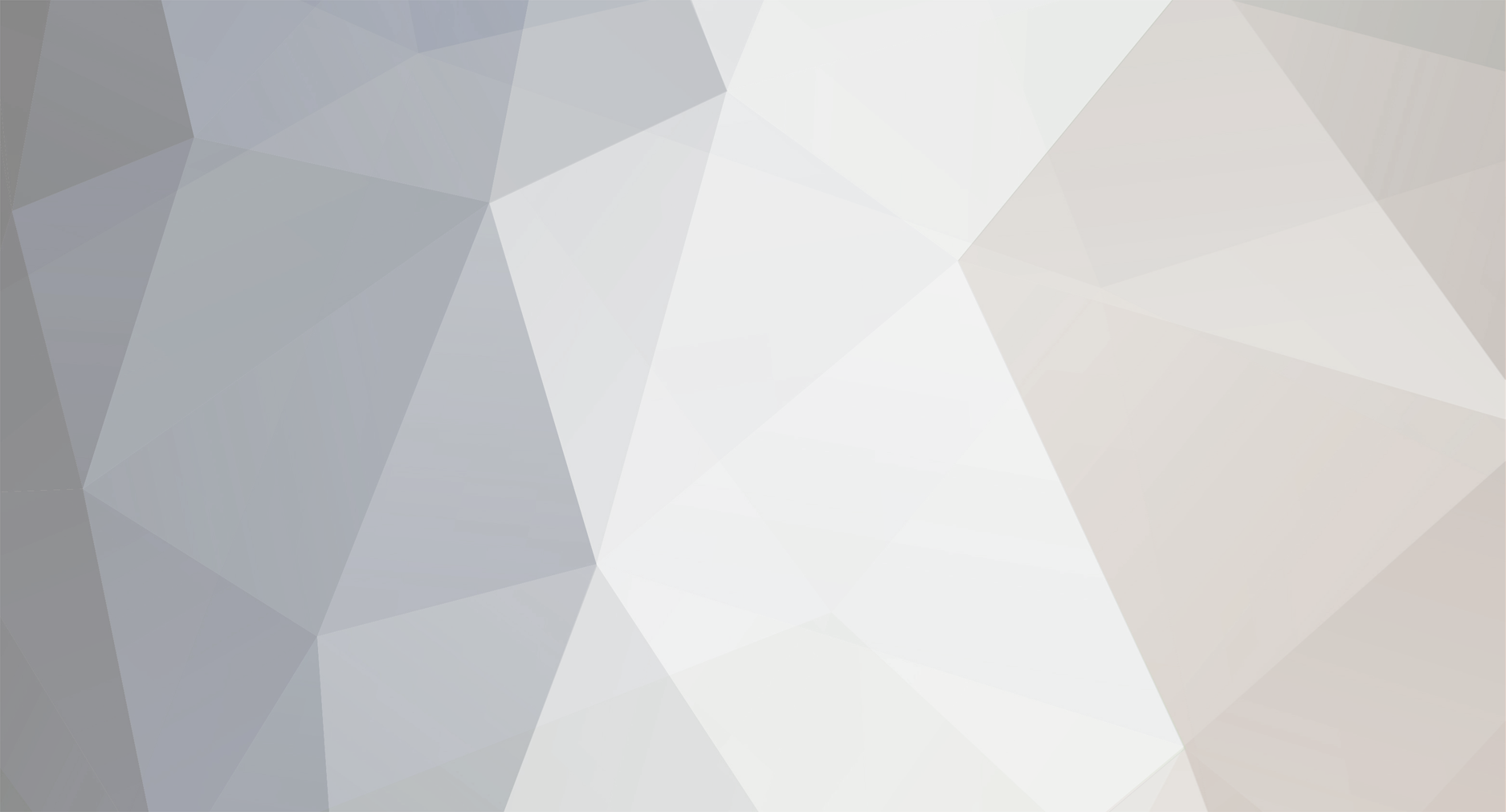
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Yeah, "writing" JavaScript from within PHP code can be problematic. There are several solutions: 1. In your original code you are defining the string using single quote marks. So, if you need to include single quote marks in the string you need to escape them using a back-slash: $tblString = '<table> <td height="19">Message date</td> <td valign="top"> <input type="text" name="schedule_SomeFunc" class="Textbox"> <a href="javascript:NewCal(\'date_schedule\', \'ddmmmyyyy\',false,24)"> <img src="cal.gif" width="16" height="16" border="0" alt="Pick a date"> </td>'; Or you could "exit" out of PHP code as the last post suggests, but I think that makes the code confusing. Or you could define the string with double quotes, but you would then need to escape any double quotes that need to be in the string: $tblString = "<table>\n"; $tblString .= " <tr>\n"; $tblString .= " <td height=\"19\">Message date</td>\n"; $tblString .= "<td valign=\"top\">"; $tblString .= "<input type=\"text\" name=\"schedule_SomeFunc\" class=\"Textbox\">"; $tblString .= "<a href=\"javascript:NewCal('date_schedule', 'ddmmmyyyy',false,24)\">"; $tblString .= "<img src=\"cal.gif\" width=\"16\" height=\"16\" border=\"0\" alt=\"Pick a date\">"; $tblString .= "</td>\n"; And, then there is always the hredoc method of defining a string $tblString = <<<TBL_HTML <table> <tr> <td height="19">Message date</td> <td valign="top"> <input type="text" name="schedule_SomeFunc" class="Textbox"> <a href="javascript:NewCal('date_schedule', 'ddmmmyyyy',false,24)"> <img src="cal.gif" width="16" height="16" border="0" alt="Pick a date"> </td> TBL_HTML;
-
As mikesta707 already showed, you can achieve this with the modulus operator. here's a bit more elaborate example: //Define max number of groups $groups = 3; //Determine user's group based upon id $user_group = ($groups%$id!=0) ? $groups%$id : $groups; mysql_query("UPDATE $tab['user'] SET group=$user_group WHERE id='$id';");
-
How to change format from (800)555-1212 to 8005551212
Psycho replied to ghurty's topic in PHP Coding Help
Well, that assumes this IS simple. True, if ALL the values are exactly in the same format, str_replace() would be faster. But, we are talking about hundredths if not thousandts of seconds. Nothing that would affect overall performance in the least. Plus, a regex expression will have more flexibility as you can create a single regex expression that could process many different types of formats - or the formats chould change and the regex would continue to work. With a str_replace() you would need to have specific logic to handle multiple formants and you would need to change it if the inputs were to ever change. Again, as long as the format will ALWAYS be the same, str_replace() will suffice. But, only if that is the case. -
Your use of default makes it so the error message is also used for the first time load of the page. Take a look at the code below. I'd suggest having a separate page to handle the success scenario. <?php $error = ''; $msg = ''; if(isset($_GET[fname])) { //User has submitted the form $name = trim($_GET[fname]); if(empty($name)) { //name was empty $error = "Please input a name"; } else { //Name was entered $msg = "Welcome Home {$name}"; include('welcome_page.php'); exit(); } } ?> <html> <head></head> <body> <?php echo $error; ?><br /> <form action="test.php" method="get"> Name: <input type="text" name="fname" /> Age: <input type="text" name="age" /> <input type="submit" /> </form> </body> </html>
-
The only testing that you would do in a production environment would be performance/load testing. If you are not confident in the testing you are doing in the test environment, why have a test environment? The progression in environments should be Dev -> Test -> Stage -> Production. There need to be specific quality gates and processes in place at each progression. Also there can be multiple Development and Test environments based upon the size of the application and business needs. With Development/Test environments you may go though several minor iterative changes. So, the purpose of Stage is to do a full update of all the changes since the last deployment to Production to ensure all the changes are made correctly "en masse".
-
Why would testing DROP TABLE bring you "to your knees"? You should be testing in a development environment, right? Create your test database (if not already in place), back up the data, run your tests, restore the backup. Basic testing methodology. You should never do general testing against a production environment - especially for anything which could be expected to corrupt the data.
-
Just set the three variables in the function: The year, the shift that will do training on the first week, and the number of total shifts <?php function getShiftForWeek($weekNo) { $output = array(); $initialYear = 2010; $firstShift = 5; $totalShifts = 6; $firstMonday = strtotime("first monday jan $initialYear"); $output['week'] = $weekNo; $output['timestamp'] = $firstMonday + (($weekNo-1) * 604800); $output['monthDay'] = date('M d', $output['timestamp']); $output['shift'] = (($weekNo + $firstShift - 1) % $totalShifts); if ($output['shift']==0) { $output['shift'] = $totalShifts; } return $output; } for ($week=1; $week<15; $week++) { $data = getShiftForWeek($week); echo "Week {$data['week']} - Week of {$data['monthDay']} = Shift {$data['shift']}<br />\n"; } ?> Output: Week 1 - Week of Jan 04 = Shift 5 Week 2 - Week of Jan 11 = Shift 6 Week 3 - Week of Jan 18 = Shift 1 Week 4 - Week of Jan 25 = Shift 2 Week 5 - Week of Feb 01 = Shift 3 Week 6 - Week of Feb 08 = Shift 4 Week 7 - Week of Feb 15 = Shift 5 Week 8 - Week of Feb 22 = Shift 6 Week 9 - Week of Mar 01 = Shift 1 Week 10 - Week of Mar 08 = Shift 2 Week 11 - Week of Mar 15 = Shift 3 Week 12 - Week of Mar 22 = Shift 4 Week 13 - Week of Mar 29 = Shift 5 Week 14 - Week of Apr 05 = Shift 6
-
Well, that output shows this [error] => 2 A simple search opf php file upload error codes seems to suggest the error is: Reference: http://www.php.net/manual/en/features.file-upload.errors.php The file size you set in the configuration is only 1MB, what was the actual size of the file you uploaded?
-
I'm glad you got it working. That's the whole point of the forum. I don't hold up my code as a correct standard for others to follow. But, when I do see someone who has code that is obviously following no standards (because they are learning) I will provide at least advice on my standards as a place to start. Good luck to you.
-
THAT is what you should have asked. I'm more than happy to provide an explanation. And, trying to change code because you don't understand it is not a good way to learn. The best way to learn is to walk through unfamiliar code and figure out what it is doing. If you don't understand a command/funciton, look it up. First off, you should always try to separate your logic (the PHP code that builds the content) from the presentation (the actual HTML code that is output to the browser. The only PHP code I typically have in my code after the HTML tag is just PHP echo statements of the content I created before that. In fact, I typically have the logic and the presentation as two separate files. there are many reasons why this is the preferred approach which is too much to go into here. <?php require_once('Connections/hairstation.php'); mysql_select_db($database_hairstation, $hairstation); //Create a query to join the tables and ONLY retrieve //the fields you need - no need to use * //This query creates aliases for the table names of 'p' and 'c' //So fields can be referenced like "p.category" instead of //"products.category" //Although you can "join" tables using the WHERE clause, the preferred //method is to use proper JOINS that give you MUCH more flexibility $query = "SELECT c.category, p.product, p.price FROM products p JOIN categories c ON p.categoryID = c.categoryID ORDER BY c.category, p.product"; $results = mysql_query($query, $hairstation) or die(mysql_error()); //Create variables to use as a flag for tracking //new categories and to store the output $currentCategory = ''; $outputHTML = ''; //Get one record at a time from the query results //until there are no more while($product = mysql_fetch_assoc($results)) { //Test if the current record's category is a NEW category if($currentCategory!=$product['category']) { //It is a new category, set the flag and output the category //This block is only run for records where the current record's //category is different from the last record. $currentCategory = $product['category']; $outputHTML .= "{$currentCategory}<br />\n"; } //Output the product name and price //This line is executed for every record $outputHTML .= "{$product['product']} - {$product['price']}<br />\n"; } mysql_free_result($prices); //The LOGIC is complete, now generate the actual page ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Untitled Document</title> </head> <body> <?php echo $outputHTML; ?> </body> </html>
-
Um, what? Care to elaborate? I've taken the time to provide three different solutions and you can't even explain why it doesn't meet your needs. Then you post that crap code aboveand ask how to not make it a do/while loop Why on earth would you go in and out of PHP code so many times when you could simply do this: <?php do { echo "{$row_prices['category']}<br />\n"; echo "{$row_prices['product']} - {$row_prices['price']}<br />\n"; echo "<br />\n"; } while ($row_prices = mysql_fetch_assoc($prices)); ?> So, what exactly is the problem with the do/while loop? Do you want to change it to a while loop, a foreach loop, what??? I really have no idea what you are wanting.
-
^^ Exactly! I think you meant the IF condition to test if the query ran successfully, but that is not what it is doing. Give this logic a try: <?php $sql = "Select pf.student_id, pf.attendance, pfl.lab_attendance FOM ProgrammingFoundations pf INNER JOIN ProgrammingFoundationsLab pfl ON pf.student_id = pfl.student_id"; $result = mysql_query($sql) or die(mysql_error());; if (!$result) { //Query failed echo 'not successful'; } else if (mysql_num_rows($result)===0) { //Query ran, but no results returned echo 'no results returned'; } else { ($row = mysql_fetch_array($result)) { echo " lecture attendance : {$row['attendance']}"; echo " lab attendance : {$row['lab_attendance']}"; } } ?>
-
OK, I got you. The SERVER needs to read the image and output it to the browser since the user (you) are not on the same network as the camera. I tried reading the image directly from another server and outputting to the browser, but couldn't get it to work (not to say it is n't possible). One solution that did work was to copy the image to the server. <?php //Create URL to image $cameraIP_1 = '192.168.1.201'; $imageURL = "http://{$cameraIP_1}/nphMotionJpeg?Resolution=320x240&Quality=Standard"; //Read the image file $content = file_get_contents($imageURL); //Write image file to server $fp = fopen("{$cameraIP_1}.jpg", 'w'); fwrite($fp, $content); fclose($fp); ?> Then in the page you can diaplay the image like so <img src="<?php echo $cameraIP_1; ?>.jpg" />
-
How to display 3 rows, 8 columns, 24 total orded by time correctly?
Psycho replied to slyte33's topic in PHP Coding Help
Ok, I double failed. Forgot to make the change. Doh! That line should be echo'd like this: echo outputRecord($record[$i+($col*$rows)]); -
How to display 3 rows, 8 columns, 24 total orded by time correctly?
Psycho replied to slyte33's topic in PHP Coding Help
Oh hell! It's the little things that screws you up. Change that line to this outputRecord($record[$i+($col*$rows)]); And fix the last line in that function. I misspelled the variable name -
How to display 3 rows, 8 columns, 24 total orded by time correctly?
Psycho replied to slyte33's topic in PHP Coding Help
Not tested, so may be some synta errors: <?php //Config variables $max_rows = 8; $max_cols = 3; //End config variables function outputRecord($record) { if (!is_array($record)) { return false; } $color = ($record['sign']=="+") ? "green" : "red"; $date = date('G:i', $record['time']); $tdOutput = "<td><b>{$date}</b> | ${$record['newprice']} | "; $tdOutput .= "<span style=\"color:{$color};\">{$record['sign']}${$record['pchange']}</span><br>"; $tdOutput .= "</td>\n"; return $tdOuptu; } if ($_GET['chart']) { $total_records = $max_rows * $max_cols; $chart = mysql_real_escape_string($_GET['chart']); $query = "SELECT * FOM drugs_pricechange WHERE name='{$chart}' ORDER BY time DESC LIMIT {$total_records}"; $drugprices=$db->execute($query); //Dump restults into temporary array $records = array(); while($dp=$drugprices->fetchrow()) { $records[] = $dp; } //Ouput the results into columns $tableData = ''; $rows = floor(count($records)/3); for ($row=0; $row<$rows; $row++) { $tableData .= "<tr>\n"; for ($col=0; $col<$max_cols; $col++) { $tableData .= outputRecord($record[$i+($col*$rows)]); } $tableData .= "<tr>\n"; } } ?> <table width=\"540\" bgcolor=\"666666\" cellspacing=\"1\"> <tr> <th class=\"dark\" colspan=\"3\">Drug price changes for <?php echo $_GET['chart']; ?></th> </tr> <?php echo $tableData; ?> </table> -
It all depends on what, exactly the camera is returning and what you are wanting to do with it. I don't know what the mode of "rb" is supposed to be doing. "r" is for read, but there's nothing about "b" in the manual. If that "link" is the correct format for the camera to display an image, you probably don't need to add all the additional ligic. Have you tried just a simple IMG tag: <img src="http://192.168.1.112/nphMotionJpeg?Resolution=320x240&Quality=Standard" /> By the way, what camera are you using?
-
I don't use JQuery, but here is a quick example in plain JS. You can repurpose for JQuery as needed: <html> <head> <style> #submit { visibility: hidden;} </style> <script type="text/javascript"> var defaultText = "write message here!"; function textFocus() { var textareaObj = document.getElementById('message'); var submitObj = document.getElementById('submit'); if (textareaObj.value==defaultText) { textareaObj.value = ''; } submitObj.style.visibility = 'visible'; } function textBlur() { var textareaObj = document.getElementById('message'); var submitObj = document.getElementById('submit'); if (textareaObj.value=='') { textareaObj.value = defaultText; } if (textareaObj.value==defaultText) { submitObj.style.visibility = 'hidden'; } } </script> </head> <body> <form> <label>Message:</label> <textarea name="message" id="message" rows="5" cols="30" onfocus="textFocus()" onblur="textBlur()">write message here!</textarea> <br /> <input type="button" name="submit" id="submit" value="submit" /> </form> </body> </html>
-
Or in PHP: $date = '2010-03-24 14:15:00'; echo date('g:i a', strtotime($date)); //Output: 2:15 pm Not sure what is more efficient - extracting from MySQL in the desired format or converting in PHP.
-
Well, HE is not on the same network, but that doesn't mean the SERVER isn't. Of course, that does rule out a JS solution. @bcamp1973: Where are you hosting this page? If you are hosting on another machine at home, then you won't have to open the cameras to the internet. Here is a quick script on what I would do. note, the form uses POST but that is because the FORM is not sending the data to the camera. The form is POSTing to the same script which then sends the GET data to the camera. The script simply uses file_get_contents() to send the URL to the camera. But, you can use another method if that doesn't work <?php $cameras = array( 'Camera 1' => '192.168.1.121', 'Camera 2' => '192.168.1.122', 'Camera 3' => '192.168.1.123' ); $actions = array( 'Turn On' => 'power=on', 'Turn Off' => 'power=off', 'Pan Up' => 'direction=panUp', 'Pan Left' => 'direction=panLeft', 'Pan Right' => 'direction=panRight', 'Pan Down' => 'direction=panDown' ); if (in_array($_POST['action'], array_keys($actions)) && in_array($_POST['camera'], $cameras)) { $urlRequest = "http://{$_POST['camera']}/cameraCGI?{$actions[$_POST['action']]}"; file_get_contents($urlRequest); $cameraName = array_search($_POST['camera'], $cameras); $response = "Request sent to {$cameraName} at IP {$_POST['camera']} to {$_POST['action']}\n"; } //Create camera options $cameraOptions = ''; foreach($cameras as $name => $ip) { $cameraOptions .= "<option value=\"{$ip}\">{$name}</option>\n"; } ?> <html> <head></head> <body> <pre> <?php echo $response; ?> </pre> <form method="POST"> <div style="text-align:center;"> Camera: <select name="camera" id="camera"> <?php echo $cameraOptions; ?> </select> <br /><br /> <input type="submit" name="action" value="Turn On" /> <input type="submit" name="action" value="Turn Off" /> <br /><br /> <input type="submit" name="action" value="Pan Up" /> <br /> <input type="submit" name="action" value="Pan Left" /> <input type="submit" name="action" value="Pan Right" /> <br /> <input type="submit" name="action" value="Pan Down" /> </div> </form> </body> </html>
-
As long as the web server is running on the same network as the cameras, there is no need to expose them to the internet. He only needs to forward port 80 to the machine running the web server. That machine then can send requests to the cameras on the same network.
-
No, you do not need to open up the cameras to the internet. I'm assuming you want these actions to be user initiated, so a form and/or links would make the most sense. Personally, I would create a form with a drop-down to select the camera and have different buttons for each action I want to perform. Then, instead of actually submitting the form, I would use Javascript on the button clicks to submit the request to the appropriate camera.
-
I'm pretty sure the problem is with the first conditional in the getBetsFor() function. if ($this->bets === null) { $ret = queryf('SELECT * FROM bets WHERE username = %s ORDER BY date DESC LIMIT %d', $this->username, MAX_DISPLAY_BETS); If I am reading this right, you are only running the query if $this->bets has not been set. So, on the second call you make to that function $this->bets (which has scope in the class) has been set so the query doesn't run. Then, the only code that does run in that function is this $this->bets = $bets; return $this->bets; $bets has scope only in the function, so on the second run it is not getting set from the query because of the initial conditional so it is a null value. So, the end of the query replaces the previous value of $this->bets with a null value.
-
You want the textarea to change how? The code has nothing in it that would change the textarea. The code is trying to set options for a textarea? There is not such thing. Are you trying to populate the textarea? Honestly, I can't figure out what it is supposed to do. Please explain.