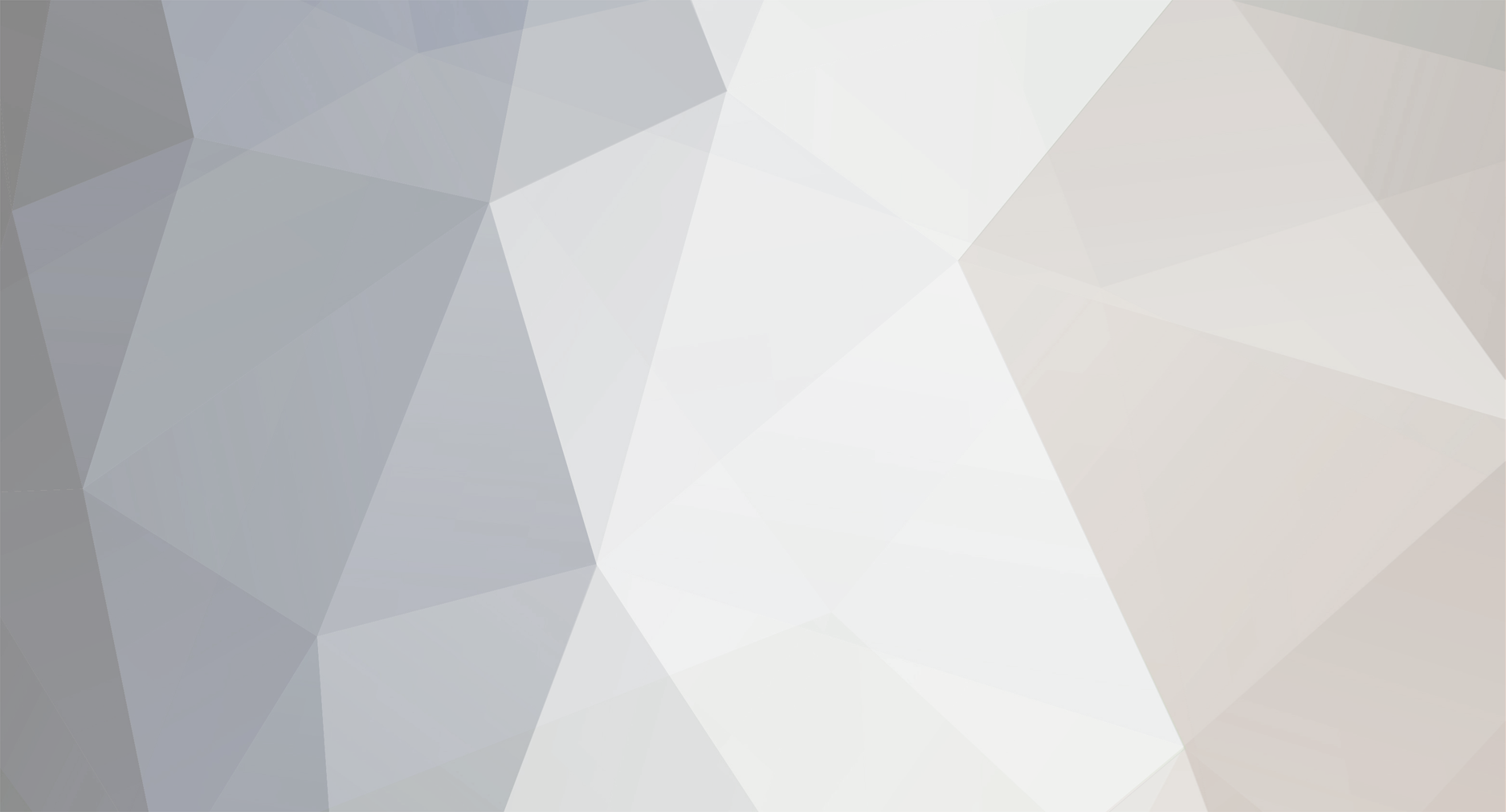
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Any, why won't string_pad() work? It works fine for me: <?php $text = "My Text"; echo str_pad($text, 10, "|"); //Output: My Text||| echo str_pad($text, 10, "|", STR_PAD_LEFT); //Output: |||My Text echo str_pad($text, 10, "|", STR_PAD_BOTH); //Output: |My Text|| ?> Of course, you can also use printf()/sprintf()
-
OK, I believe this should produce the same result as your original script with much, much less overhead. <?php if (isset($_POST['key1'])) { $key1 = $_POST['key1']; $key2 = "C 0."; $key3 = "F "; $key4 = "F 0."; //Load file into an array $fileLines = file("input.txt"); //Open same file and use "w" to clear file $fileObj = fopen("output.txt","w"); //loop through array using foreach foreach($fileLines as $line) { //Keep lines which contain $key1 & $key3, but do not contain $key2 or $key4 if (strpos($line, $key1) && !strpos($line, $key2) && strpos($line, $key3) && !strpos($line, $key4)) { fputs($fileObj, $line); //place $line back in file } } //Close the file handle fclose($fileObj); } ?> <html> <head></head> <body> <form name="keyInput" action="" method="POST"> Enter Key: <input type="text" name="key1"> <br /><br /> <button type="submit">Submit key</button> </form> </body> </html>
-
After further review, that code is really inefficient. There is no reason to loop over every line multiple times. Let me see what I can do and I'll post back.
-
Changes made include the IF statement at the top, assignment of $key right after that, and an inclusion of a form at the borrom of the script. <?php if (isset($_POST['key'])) { $key = $_POST['key']; // <--------- [b]to prompt to ask for this[/b] //load file into $fc array $fc=file("input.txt"); //open same file and use "w" to clear file $f=fopen("output.txt","w"); //loop through array using foreach foreach($fc as $line) { if (strstr($line,$key)) //look for $key in each line -adding ! if (!strstr($line,$key)) does the opposite it will delete everything else than A fputs($f,$line); //place $line back in file } fclose($f); $key2 = "C 0."; //load file into $fc array $fe=file("output.txt"); //open same file and use "w" to clear file $fi=fopen("final.txt","w"); //loop through array using foreach foreach($fe as $line2) { if (!strstr($line2,$key2)) //look for $key in each line -adding ! if (!strstr($line,$key)) does the opposite it will delete everything else than A fputs($fi,$line2); //place $line back in file } fclose($fi); $key3 = "F "; //filters just this text at the begining of a line //load file into $fc array $fc3=file("input.txt"); //open same file and use "w" to clear file $f3=fopen("output2.txt","w"); //loop through array using foreach foreach($fc3 as $line3) { if (strstr($line3,$key3)) //look for $key in each line -adding ! if (!strstr($line,$key)) does the opposite it will delete everything else than A fputs($f3,$line3); //place $line back in file } fclose($f3); $key4 = "F 0."; //load file into $fc array $fc4=file("output2.txt"); //open same file and use "w" to clear file $f4=fopen("final2.txt","w"); //loop through array using foreach foreach($fc4 as $line4) { if (!strstr($line4,$key4)) //look for $key in each line -adding ! if (!strstr($line,$key)) does the opposite it will delete everything else than A fputs($f4,$line4); //place $line back in file } fclose($f4); } ?> <html> <head></head> <body> <form name="keyInput" action="" method="POST"> Enter Key: <input type="text" name="key"> <br /><br /> <button type="submit">Submit key</button> </form> </body> </html>
-
Yes, please explain a little better what you are trying to do. If you are just wanting to check if any element in array1 exists in array2, then you don't need to iterate over each element, just use array_interset(). Or, depending upon your need you could use array_diff (or any of the variants of those two functions): if (count(array_intersect($array1, $array2))>0) { echo "At least 1 item in array 1 exists in array 2.<br>"; echo "They are: " . print_r(array_intersect($array1, $array2)); } if (count(array_diff($array1, $array2))==0) { echo "All the items in array 1 exists in array 2."; }
-
Yeah, you could do a query to add up all the subtotals, but since you are already getting all the individual records a better approach would be to calculate the subtotals as you process each record.
-
I'm out of ideas then. I had already tried creating a solid color on top of the red circle and then setting it to transparent before placing the blue circle. That didn't work for me - it appears the transparency only applies to the specific color after the image is complete. I also tried using imagesetthickness() and imageellipse() to just create a red outer ring. Looking at the descriptions and examples in the manual it shoudl work, but the output was always just a 1 pixel wide ring. Seems to be a bug as the manual states: The only other option I can think of would be to create the red/blue circle as above (with a solid white circle in the middle) as a separate object and then merging that object on top of whatever other elements you need. Not really sure and don't intend to invest the time. Good luck to you.
-
Hmm, I tried using imagecolortransparent() but with no positive results. BUt, I think I found a solution. When creating the blue circle we want it to have the set color with bleed-through for the green square but not the red circle. So, using the same code as above, I created a completely opaque white circle so the blue circle would have the right color. But, since you want to have the green from the square show through - I created a 90 degree green arc on the white circle before placing the blue circle. <?php $img = imageCreateTrueColor( 600, 600); $white = imagecolorallocatealpha($img, 255, 255, 255, 0); $green = imagecolorallocate($img, 0, 255, 0); $red = imagecolorallocatealpha($img, 255, 0, 0, 50); $blue = imagecolorallocatealpha($img, 0, 0, 255, 50); imagefill ($img, 0, 0, $white); //Draw the background shape..a rectangle imagefilledrectangle($img, 0,0,300,300, $green); //Now draw the objects imagefilledellipse ($img, 300, 300, 400, 400, $red); imagefilledellipse ($img, 300, 300, 350, 350, $white); imagefilledarc($img, 300, 300, 350, 350, 180, 270, $green, IMG_ARC_PIE); imagefilledellipse ($img, 300, 300, 350, 350, $blue); header( 'Content-Type: image/png' ); imagePNG( $img );imagedestroy($img); ?>
-
Well, your original code was doing what it was supposed to do then - the red image was showing through the blue image. Although, I think I understand, what you are trying to accomplish. The only solution I can see is to do as one poster suggested above and modify the blue color (with the red showing through) to achieve the color you want. EDIT: After a little trial and error I think you can get close to what you want by changing the Alpha value for the $white color. Try setting it to 50 and see what you think. EDIT #2: Or you could look into using imagefilledarc() to create the red outer circle around the blue circle so it isn't underneath the blue circle.
-
The problem has been addressed previously. I found the solution on a different forum post here: http://www.webdeveloper.com/forum/showthread.php?t=195186 The brackets are needed because \r and \n are interpreted as newline/linbreak characters. So, you have to use the brackets and extra slashes to make the regular expression interpreter know you are wanting the actual characters and not the newline characters. I'm actually surprised that three backslashes are required - I would have expected two were needed.
-
Hold on, there's some conflicting information here. In your original post you showed this code $body = preg_replace("'\r?\n'","<br>", $row[body]); But, now you are saying you don't want to replace the line breaks with BR tags?! Also, it appears you are saying the "original" content contains this: <p align=\"center\"><strong>TITLE HERE</strong></p>\r\n <p>Vancouver,\r\nBC:& and the output looks like this: TITLE HERE \r\n Vancouver,\r\nBC Is that correct? How, exactly, is the original content defined? It looks like you are saving the ACTUAL characters \r and \n and NOT the escape codes for line breaks. You would never "see" the \r\n codes. Something is fishy here. If you are seeing the actual characters \r\n, then this should remove them $body = preg_replace("/[\\\][r][\\\][n]/", '', $row['body']); But, a bigger question is why those characters are in your data at all. There seems to be a problem with how the data is defined/saved.
-
That statement doesn't make sense. PHP reads the "value" of the variable. So, the value you are getting from the database must not contain the characters you think it does. I would suggest running a preg_replace to just remove any \r's and then use nl2br() on the result. See what that does.
-
I just tested it and the blue came out perfectly (although my image defaulted to a black background which messes up the red. I suspect you are setting the initial background to white?). This code gives me the EXACT image you showed you were after above: <?php $img = imageCreateTrueColor( 600, 600); $red = imagecolorallocatealpha($img, 255, 0, 0, 50); $white = imagecolorallocatealpha($img, 255, 255, 255, 0); $blue = imagecolorallocatealpha($img, 0, 0, 255, 50); imagefill ($img, 0, 0, $white); imagefilledellipse ($img, 300, 300, 400, 400, $red); imagefilledellipse ($img, 300, 300, 350, 350, $white); imagefilledellipse ($img, 300, 300, 350, 350, $blue); header( 'Content-Type: image/png' ); imagePNG( $img );imagedestroy($img); ?>
-
The function takes parameters for separate RGB values. So, this $blue = imagecolorallocatealpha($img, 0, 0, 255,50); Red = 0, Green = 0, Blue = 255, could be expected to be the same as the HTML equivalent of #0000ff, which is close to the color in the test image. But, that function also takes a fourth parameter (the alpha channel) for opacity! You are laying the blue image on top of the red image and the opacity is set at 50, which is somewhat transparent. So, some of the red from the first circle is bleeding through the blue. Since I am assuming you want the blue to have the slightly subdued appearance as opposed to being completely opaque, my suggestioin would be that after you create the red circle and before you create the blue circle, you should create a completely opaque white circle (alpha set to 0) at the same size and position as you will be placing the blue circle. The blue circle will then be placed on top of a white background. If you get a funny looking edge around the blue circle try decreasing the size of the white circle slightly.
-
You are welcome. I have updated the script with expanded comments. I suspect the most difficult part to understand is within the for() loop at the end. <?php //Create an array of all the "commands" //These should be in the order in which you want the columns displayed $commands = array('SEDESC', 'PSEQ', 'IOPT', 'PLANE', 'FOV', 'SLTHICK', 'SPC', 'MATRIXX', 'MATRIXY', 'TR', 'TE', 'FLIPANG'); //Run a single query to get ALL the records for ALL commands $dataAry = array(); $query = "SELECT command, value FROM test WHERE type='SET' AND command IN ('" . implode("', '", $commands) . "')" $result = mysql_query($query); //Reads the results of the query and put into a multi-dimensionals array //With the command as the primary key and the values as subarray elements while($record = mysql_fetch_assoc($result)) { $dataAry[$record['command']][] = $record['value']; } //Determine the length of the longest "command" list in the array //and set the varaible $maxLength to that $maxLength = 0; foreach($commands as $command) { if(count($dataAry[$command])>$maxLength) { $maxLength = count($dataAry[$command]); } } //create the table html $tableHTML = "<table>\n"; //Create headers by iterating through the command list above $tableHTML .= "<tr>\n"; foreach($commands as $command) { $tableHTML .= "<th>{$command}</th>\n"; } $tableHTML .= "</tr>\n"; //Create data output //Run a loop to iterate through all the indexes for the //values using the $maxLength value defined above to ensure //all values are displayed (some "commands" may have //less values. First time through loop will get the value //at index 0 for each command, second pass will get the //values at index 1, etc. for($idx=0; $idx<$maxLength; $idx++) { //Open the table row $tableHTML .= "<tr>\n"; //Iterate through each command foreach($commands as $command) { //This is a ternary operator (i.e. If/Else shorthand) //$value is set to the current index of the current command IF //that value is set Otherwise, $value is set to an empty string $value = (isset($dataAry[$command][$idx])) ? $dataAry[$command][$idx] : ''; //Create the table cell for the current command/index value $tableHTML .= "<td>{$value}</td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "<table>\n"; ?>
-
It's not the same code. Look at the second parameter in this for loop for (var i=0; i->checkboxLen; i++) { That should be i<checkboxLen Also, posting in an old thread, especially a solved one, is not a good idea. Start a new thread and link back tothe original if it is pertinent EDIT: This line is also incorrect: if (form1['platform[]'].checked) { Take a look at the originally provided code to see the problem.
-
Which should not logically be done within the body of the script. The logic and the output should be separate.
-
Something is missing from that code. You are using the variable $series as a database result, but it is not set any where. Give this a try. I think it is a little more organized and flexible (not tested as I don't have your database) <?php $commands = array('SEDESC', 'PSEQ', 'IOPT', 'PLANE', 'FOV', 'SLTHICK', 'SPC', 'MATRIXX', 'MATRIXY', 'TR', 'TE', 'FLIPANG'); //Query ALL the records $dataAry = array(); $query = "SELECT command, value FROM test WHERE type='SET' AND command IN ('" . implode("', '", $commands) . "')" $result = mysql_query($query); while($record = mysql_fetch_assoc($result)) { $dataAry[$record['command']][] = $record['value']; } //Determine the length of the longest list $maxLength = 0; foreach($commands as $command) { if(count($dataAry[$command])>$maxLength) { $maxLength = count($dataAry[$command]); } } //create the table html $tableHTML = "<table>\n"; //Create headers $tableHTML .= "<tr>\n"; foreach($commands as $command) { $tableHTML .= "<th>{$command}</th>\n"; } $tableHTML .= "</tr>\n"; //Create data output for($idx=0; $idx<$maxLength; $idx++) { $tableHTML .= "<tr>\n"; foreach($commands as $command) { $value = (isset($dataAry[$command][$idx])) ? $dataAry[$command][$idx] : ''; $tableHTML .= "<td>{$value}</td>\n"; } $tableHTML .= "</tr>\n"; } $tableHTML .= "<table>\n"; ?>
-
It worked for me. Can you output the text from the database to a text file and attach here?
-
Try this: $body = preg_replace("/\r?\n/","<br>", $row[body]);
-
Assuming the last example was a mistake as gamblor01 suggests, why would array_diff() not work for you? $array1 = array('Mike', 'Mike', 'Kim', 'Kim', 'John'); $array2 = array('Mike', 'Kim'); $result = array_diff($array1, $array2); print_r($result); Ouput: Array ( [4] => John ) Is that not what you want? No need to loop through the values.
-
Use uksort() and uasort() which allows you to define a custom function for sorting an array. I'll make an attempt at the function for you, but what you stated doesn't make sense to me. You state you want to initially sort by the length of the first key, but that doesn't seem correct based upon your example where the last item "folder2" has a shorter key than the item before it - a subfolder under "folder1". I assume you mean to sort the keys alphanumerically. This will take two different sort functions, one for the keys and one for the values of the subarrays. <?php //Sort by file path in primary key function sort_by_path($a, $b) { return strcasecmp(trim($a, '\\'), trim($b, '\\')); } //Sort by name sub-element in each path element function sort_by_filename($a, $b) { return strcasecmp($a['name'], $b['name']); } uksort($filelist, 'sort_by_path'); foreach($filelist as &$path) { uasort($path, 'sort_by_filename'); } ?>
-
You will need a combination of JavaScript and PHP. Here are some scripts to look into: http://www.hotscripts.com/blog/javascript-image-cropping-scripts/
-
I made an assumption that you are wanting to remove one item from array1 for each single items in array2. So, if array 1 has three "Kim" and array 2 has two "Kim" the result would give you one "Kim". If that is correct, this should work for you (array keys are preserved): <?php function array_diff_unique($array1, $array2) { foreach($array2 as $search) { unset($array1[array_search($search, $array1)]); } return $array1; } $array1 = array('Mike', 'Mike', 'Kim', 'Kim'); $array2 = array('Mike', 'Kim'); $result = array_diff_unique($array1, $array2); print_r($result); ?> Ouput: Array ( [1] => Mike [3] => Kim )
-
What result are you wanting? Since there are duplicates I'm not sure as there are different ways this could be interpreted. For exmple, you might be wanting to exlude one item from the second array for each item in the first array (which would result in one Mike and one Kim) or you might be wanting to exclude every matching item from the second array for every item in the first array (which would result in nothing). Plus, when there are results, do you need to preserve the indexes or not? You need to explain what you want better - examples would help.