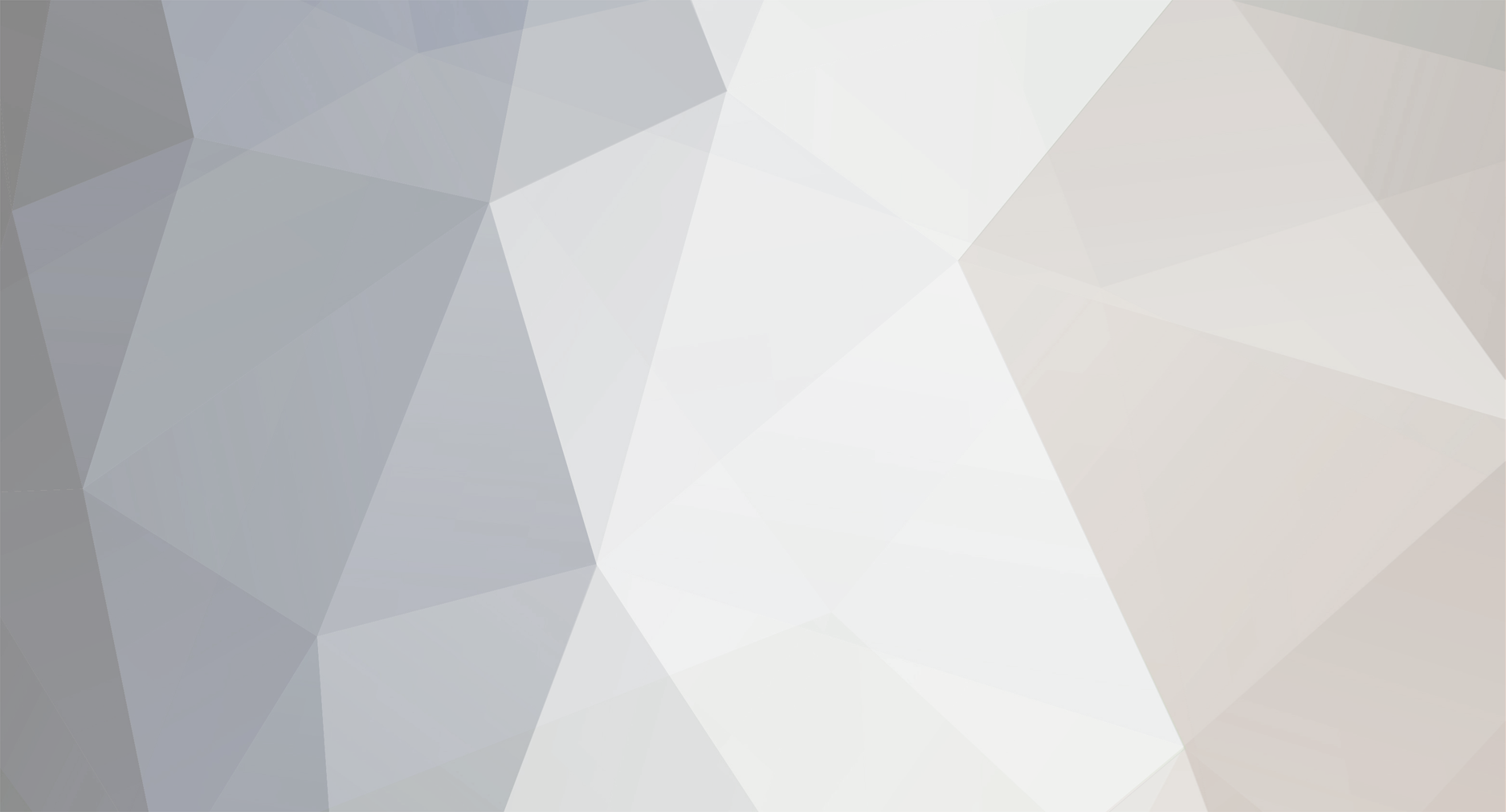
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
<input type="text" name="referral" disabled="disabled" value="<?php echo $_GET['referral']; ?>" />
-
OK, you decided to respond to me through IM for some reason, but I'll respond here. You have not provided any code, so I can't give specifics, but I'll give you the basics. You apparently already have some code that determines whether the user has submitted a search query and filters the results for the pagination accordingly. When you take that data to run your query you want to also save the data to session variables. But, you will also need to clear any existng variables when the user changes the query. Here is an example: session_start(); $whereClauseAry = array(); $recordsPerPage = 10; $page = 1; if (isset($_POST)) { //User has submitted new criteria //Reset the saved criteria fields $_SESSION['restaurant_query'] = array(); $name = trim($_POST['name']); $zip = trim($_POST['zip']); if(!empty($name)) { $whereClauseAry[] = "`name` = '" . mysql_real_escape_string($name) . "'"; $_SESSION['restaurant_query']['name'] = $name; } if(!empty($zip)) { $whereClauseAry[] = "`zip` = '" . mysql_real_escape_string($zip) . "'"; $_SESSION['restaurant_query']['zip'] = $zip; } } elseif (isset($_GET['page'])) { //User has selected a new page for the current criteria //Get the saved criteria values if (isset($_SESSION['name'])) { $whereClauseAry[] = "`name` = '" . mysql_real_escape_string($_SESSION['name']) . "'"; } if (isset($_SESSION['zip'])) { $whereClauseAry[] = "`zip` = '" . mysql_real_escape_string($_SESSION['zip']) . "'"; } $page = (int) $_GET['page']; } $whereClauseStr = ''; if (count($whereClauseAry)>0) { $whereClauseStr = "WHERE " . implode(' AND ', $whereClauseAry); } //Run the query for the current page of the current criteria $limitStart = ($page-1) * $recordsPerPage; $query = "SELECT * FROM restaurants {$whereClauseStr} LIMIT {$limitStart}, {$recordsPerPage}";
-
$previous = 0; while ($row = mysql_fetch_array($show)) { print "Total: {$row['score']}<br />\n"; print "Previous: {$previous}<br /><br />\n"; $previous = $row['score']; }
-
Here is a quick and dirty example. Take a look what the page looks like in a browser and then look at the print preview (or actually print it if you wish). The PHP created random data for 10 accounts and created the page using classes and styles so you get one HTML page with all the records, but get separate pages for each account when printing. <?php $totalAccts = 10; $date = date('m-d-Y'); $content = "<div id=\"allAcctHeader\"><h2>Details for all accounts on {$date}</h2><br /><br /></div>\n"; for ($acct=1; $acct<=$totalAccts; $acct++) { $recordsForAcct = rand(1, 6); $acctTotal = 0; $content .= "<table border=\"1\">\n"; $content .= "<tr><th colspan=\"2\">Output for account #{$acct}<span class=\"printView\">, Printed on: {$date}</span></th></tr>\n"; for ($rec=1; $rec<=$recordsForAcct; $rec++) { $amt = rand(1, 200); $content .= "<tr><td>Record {$rec}</td><td>{$amt}</td></tr>\n"; $acctTotal += $amt; } $content .= "<tr><th>Total</th><th>{$acctTotal}</th></tr>\n"; $content .= "</table>\n"; if ($acct<$totalAccts) { $content .= "<div class=\"pageBreak\"><br /></div>\n"; } } ?> <html> <head> <style> /* default styles */ #header { width:100%;border:1px solid #000000; } #menu { width:150px;border:1px solid #000000;line-height:30px;float:left; } #content { border:1px solid #000000;margin-left:150px; } .printHead { display: none; } .printView { display: none; } /* print specific styles */ @media print { #header { display: none; } #menu { display: none; } #content { margin-left:0px; border:0px; } #allAcctHeader { display: none; } .printHead { display: inline; } .printView { display: inline; } .pageBreak { page-break-after: always; } } </style> </head> <body> <div id="header"> <h1>Site Header</h1> </div> <div id="menu"> <br /> Menu Item 1<br /> Menu Item 2<br /> Menu Item 3<br /> Menu Item 4<br /> <br /><br /><br /><br /> </div> <div id="content"> <?php echo $content; ?> </div> </body> </html>
-
Yes, this is very possible and not that difficult. It just requires the use of some specialized CSS. The CSS framework includes the ability to define different styles based upon the output media. For example, you can have a page display differently on a mobile phone than it does in a browser. One of the supported media formats is the "print" media. Basically, the styles defined in the print media format are rendered when you print the page. For example, when I render some results on a page it will typically have the website header image across the top along with navigational elements on the left and maybe some footer links as well. In those situations I will define the styles for those sections in the "print" media format to not display. So, when the user prints the output they get only the output and not all the other elements of the website. It makes the output much cleaner. Additionally, and more importantly, the CSS framework supports page breaks. I will assume that the printed output for each account will need a header and some other generic data that you do not want displayed when the records are displayed sequentially on a web page. To solve your problem I would create a page that allows the user to pull the results for all of their accounts. Then when building the page include ALL the data you need displayed in the web page and the print output. Then use the media property to hide the multiple headers when displayed in a browser and to display when printed. And, insert page breaks between each account set of records.
-
Yes, there is a much easier method: array_diff_key() Using your example above: //Create an array with idexes of those to be removed $arrayKeysToRemove = array('2' => ''); $newArray = array_diff_key($originalArray, $arrayKeysToRemove);
-
Your IF condition is doing an assignment (which is always returning true) not a comparison. Change it to double equal signs to do a comparison. Although, you are making the code more complext than it needs to be. Just do this: function disable() { var formObj = document.forms['form1'].elements; formObj['t2'].disabled = (formObj['txt_c'].value==formObj['spore'].value); }
-
Is it possible to load a page with jquery that uses javascript?
Psycho replied to samoi's topic in Javascript Help
Yes, you can include your JS in the index page. In fact, if the index page is where you create the HEAD, then that is the best pace to include your JavaScript includes. Heck, you could even include JavaScript code dynamically, but... I know this is your personal site, but any web developer with any experience would tell you that implementing such a site that relies so heavily on JavaScript is a bad idea. Why not just build your site in PHP or someo other server-side language? -
how to check if string is gzdeflate compressed?
Psycho replied to newbtophp's topic in PHP Coding Help
I con't think of any bullet proof method, but assuming you are talkin about strings that are in the english language I guess you could create a function to test teh percentage of characters int eh string that are in a-zA-Z0-9. If less than a certain percentage (say 50%) then you are probably dealing with a deflated string. But, I question why the format of the data being processed is not known. I would think the data in question should either be stored as deflated or not. -
I'm not following what you are trying to achieve with "[[anythinggoeshere.anyextension]]". Are you wanting to replace any text that appears within double brackets that has a period in it? Try providing some real exampes of the search text and what, exactly, you want replaced.
-
Returning to the previous page, in the position of last added product
Psycho replied to monicao's topic in PHP Coding Help
There's no reason you can't simply redirect to the page adding tyhe anchor tag on the end. BUt, if this is the code you are using, it is wrong header("location:domain.php#product'.$name.'") You are defining the location in double quotes and then apparently try to add the $name within single quotes. Try this: header("location:domain.php#product{$name}") -
No problem. I misunderstood what you were doing at first and ended up changing gears half way through, so it is not as efficient as it could be. If I were to rewrite it, I would just add all the records to the temp array and then generate the tables in one go from that array.
-
OK, I decided to test my code and found some errors. I created some test data and it seems to work as you are looking for. It is completely customizable as to how many records (columns) you want for each table by setting the $recordsPerTable variable <?php $recordsPerTable = 2; $recordCount = 0; $aiOutput = ''; function resetDataArray() { $dataArray = array(); $dataArray['name'] = array(); $dataArray['nie'] = array(); $dataArray['address'] = array(); $dataArray['citystzip'] = array(); return $dataArray; } function createTable($dataArray, $maxRecords) { $htmlOutput = "<table width=\"100%\" border=\"1\" cellspacing=\"0\" cellpadding=\"0\" style=\"font-size:8pt;\">\n"; foreach($dataArray as $field => $values) { $htmlOutput .= "<tr>\n"; for ($i=0; $i<$maxRecords; $i++) { $value = (isset($values[$i])) ? $values[$i] : ''; $htmlOutput .= "<td width=\"175\">{$value}</td>\n"; } $htmlOutput .= "</tr>\n"; } $htmlOutput .= "</table>\n"; return $htmlOutput; } $tableRecords = resetDataArray(); foreach($listAI as $ai) { $recordCount++; //Add current record data to temp array $tableRecords['name'][] = $ai->ai_name; $tableRecords['nie'][] = $ai->ai_nie; $tableRecords['address'][] = $ai->ai_address; $tableRecords['citystzip'][] = "{$ai->ai_city} {$ai->ai_state}, {$ai->ai_zip}"; if ($recordCount%$recordsPerTable==0) { //Create output for table and reset array $aiOutput .= createTable($tableRecords, $recordsPerTable); $tableRecords = resetDataArray(); } } //Output last table if there were uneven records if ($recordCount%$recordsPerTable>0) { $aiOutput .= createTable($tableRecords, $recordsPerTable); } echo $aiOutput; ?>
-
Give this a try. Not tested so there may be some syntax or other minor issues: <?php $recordsPerTable = 2; $aiOutput = ''; $tableRecords = array(); function createTable($dataArray, $maxRecords) { $htmlOutput = "<table>\n"; foreach($dataArray as $field => $values) { $htmlOutput .= "<tr>\n"; for ($i=0; $i<$maxRecords; $i++) { $value = (isset($values[$i])) ? $values[$i] : ''; $htmlOutput .= "<td width=\"175\">{$value}</td>\n"; } $htmlOutput .= "</tr>\n"; } $htmlOutput .= "</table>\n"; return $htmlOutput; } foreach($listAI as $ai) { //Add current record data to temp array $tableRecords['name'][] = $ai->ai_name; $tableRecords['nie'][] = $ai->ai_nie; $tableRecords['address'][] = $ai->ai_address; $tableRecords['citystzip'][] = "{$ai->ai_city} {$ai->ai_state}, {$ai->ai_zip}"; if (count($tableRecords)==$recordsPerTable) { //Create output for table and reset array $aiOutput .= createTable($tableRecords, $recordsPerTable); $tableRecords = array(); } } //Output last table if there were uneven records if (count($tableRecords)>0) { $aiOutput .= createTable($tableRecords, $recordsPerTable); } echo $aiOutput; ?>
-
Just populate a hidden field on the form with the ID of the random word you have pulled from the database. If you need that particular word available on later pages, then save it as a session variable.
-
SELECT team_id, c1.first_name as coach1_fname, c1.last_name as coach1_lname, c2.first_name as coach2_fname, c2.last_name as coach2_lname, FROM teams t JOIN coaches c1 ON t.coach1 = c1.coach_id JOIN coaches c2 ON t.coach2 = c2.coach_id
-
I think an even easier solution is to just modify how you are selecting the boxes. Instead of using links, which require you to submit the page or use AJAX, you could just have a checkbox in each div. Then the user can select 10 checkboxes and submit their answer. Using the above method you can simply store the correct answer in a session variable and check the response without needing a database.
-
Why you would respond to me through PM instead of this post I have no clue. I provided an example of how the resulting HTML would need to be formatted. I assumed you could implement that in your existing code. <?php $currentdir = getcwd(); chdir("/home/site/public_html/music"); $filelist = glob("*.mp3"); if(empty($filelist)) { echo "DIR is empty.."; } else { echo "<form action=\"filesafe.php\" method=\"POST\">\n"; echo "Choose your files:<br />\n"; echo "<div style=\"height:100px;width:100px;overflow:auto;border:1px solid #000000;\">\n"; foreach($filelist AS $file) { if(is_file($file)) { echo "<input type=\"checkbox\" name=\"checkfiles[]\" value=\"$file\">$file<br />\n"; } } echo "</div>\n"; echo "<button type=\"submit\">Submit</button>\n"; echo "</form>\n"; } chdir("$currentdir"); ?>
-
Your problem has nothing to do with PHP. It is an HTML/CSS issue: <div style="height:100px; width:100px;overflow:auto;border:1px solid #000000"> One<br /> Two<br /> Three<br /> Four<br /> Five<br /> Six<br /> Seven<br /> Eight<br /> </div>
-
At some point in your script you you are making a decision to set the vlaue to null before you execur, correct? Just do something like this $customer_id_sql = ($a==$b) ? "'{$customer_id}'" : 'NULL'; $query="INSERT INTO tasks (task_id,task,customer_id) VALUES ('', '{$task}', {$customer_id_sql})"; Just replace $a==$b with whatever condition you are using to determine whether you want a null value or not.
-
Just use a counter and compare with the total records returned from the query using mysql_num_rows() as jl5501 suggests above. Another option is to put the results into an array and implode() the array with whatever divider you want. Exmple #1: $totalRows = mysql_num_rows($result); $rowCount = 0; while($row = mysql_fetch_assoc($result)) { $rowCount++; //Echo the results echo "{$row['somedata']}<br />\n"; if ($rowCount < $totalRows) { echo "<hr>\n"; } } Exmple #2: $outputArray = array(); while($row = mysql_fetch_assoc($result)) { //Add data to result array $outputArray[] = "{$row['somedata']}<br />\n"; } //Echo output echo implode("<hr>\n", $outputArray);
-
Please explain what you mean by a "scrolling list". From what I am seeing in the above code it will echo the results vertically on the page. Not sure what you mean by scrolling. If you want the list to dynamcally scroll on the page, then you will need to implement JavaScript. Or, maybe, you just want the restuls in a "container" on the page with scrollbars so it doesn't fill up the page. In that case, put the results in a DIV with the appropriate style properties.
-
Getting table row data based on ticked checkbox
Psycho replied to mrherman's topic in PHP Coding Help
You should just need the ID, since the ID should be associated with all the details for the student. You should create the checkboxes as arrays and se the value as the ID of the record <input type="checkbox" name="students[]" value="<?php echo $row['id']; ?>" />