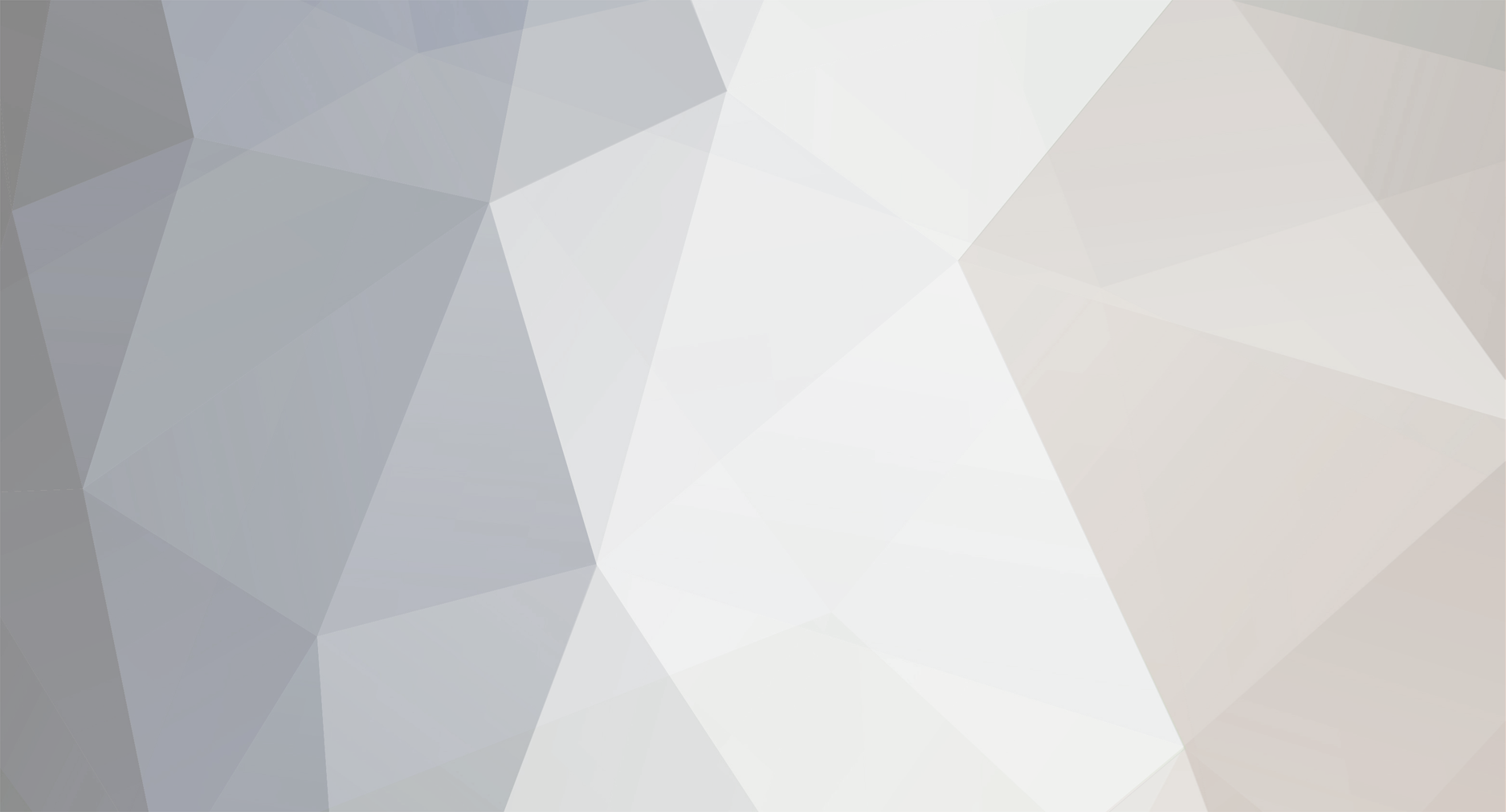
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Your operator is wrong. It would delete records which are less than 24 hours old. Plus, you need to convert the time field to seconds as well. DELETE FROM `logs` WHERE UNIX_TIMESTAMP(`time`) + 86400 < UNIX_TIMESTAMP()
-
At the head or logic of your page (assumes $currentPage is set): <?php $totalPages = (ceil($total/$entriesPerPage)); $pageList = ''; for($page=1; $page<=$totalPages; $page++) { if ($currentPage==$page) { $pageList .= " <b>[{$page}]</b> "; } else { $pageList .= " <a href="?page={$page}">{$page}</a> "; } } ?> In the body of your HTML <P align="right"><?php echo $pageList; ?></p>
-
You need to build the table with the results. Are you wanting the table to always be 14 x 4 no matter how many results there are? If so, that is easily done. <?php $rows = 4; $cols = 14; mysql_connect("localhost", "Master", "password); mysql_select_db("Ustack"); $maxCount = $rows * $cols; $query = "SELECT * FROM Stacks Where username='Admin' LIMIT 0, {$maxCount}"; $result = mysql_query($query) or die (mysql_error()); $idList = array(); while($row = mysql_fetch_assoc($result)) { $idList[] = $row['id']; } $tableOutput = ''; for($row=0; $row<$rows; $row++) { $tableOutput .= " <tr>\n"; for($col=0; $col<$cols; $col++) { $idIndex = ($row * $cols) + $col; $tableOutput .= "<td>"; $tableOutput .= (isset($idList[$idIndex]) ? $idList[$idIndex] : ' '); $tableOutput .= "</td>\n"; } $tableOutput .= " </tr>\n"; } ?> <table border="0"> <?php echo $tableOutput; ?> </table>
-
Not true. You can't pass a date to the Date() object that it does not understand. But, it can parse a string into a date object. But, it only parses dates in the format the humans use, not what machines use yyyy-mm-dd. Here are some acceptable methods of creating a date object from a string (note that some of these may depend on the user's locale setting since many countries use dd-mm-yyyy, whereas the US uses mm-dd-yyyy) var dateObj = new Date('Oct 31, 1968 12:11:32'); var dateObj = new Date('October 31, 1968 12:11:32'); var dateObj = new Date('10/31/1968'); var dateObj = new Date('10/31/68');
-
Good point. I guess it depends on what the purpose is. If I was running some start and end times of a process to gather metrics on performance I would probably include the microtime call int he function itself. Since each start and stop would have to call the function, the time to start the function should be "equivalent" if not for the single instance it should be over the long term.
-
It is a constraint with PHP. From the manual date():
-
function microDateTime() { list($microSec, $timeStamp) = explode(" ", microtime()); return date('F jS, Y, H:i:', $timeStamp) . (date('s', $timeStamp) + $microSec); } echo microDateTime(); //Output: November 3rd, 2009, 15:38:38.671753 Modify format as needed
-
It's not clear from your post if you want to have two lines of the text file appear in one column (each in a separate cell) or if you want to split each line into two cells of the table. If you need each line split we need to know what the rule is for splitting them. If it is the former you want, try this (not tested): <?php $maxColumns = 2; $currentCol = 1; $lines = file ('/path/to/your/file'); foreach ($lines as $line) { if ($currentCol==1) { $tableHTML .= " <tr>\n"; } $tableHTML .= " <td valign=\"top\">{$line}</td>\n"; $currentCol++; if ($currentCol>$maxColumns) { $tableHTML .= " </tr>\n"; $currentCol==1 } } if($currentCol<=$maxColumns) { for ($currentCol; $col<=$maxColumns; $currentCol++) { $tableHTML .= " <td valign=\"top\"> </td>\n"; } $tableHTML .= " </tr>\n"; } ?> <table width="100%"> <?php echo $tableHTML; ?> </table>
-
I pretty much concur with the above. I would split out the functions into separate files based upon which ones are used together. For example, if you have a set of functions that are only used for pages that include a form, for validation and such, then I would only load those functions on pages which include forms. Not knowing what functions are in those files or how they are used it's difficult to say if that is optimal. But, I do like using smaller files with specific usage - it makes debugging and modifications much easier. For example, if you are needing to modify a function that is used to display a set of records, it is much safer if that file does not also include functions that insert/delete records. Otherwise you could accidentally instroduce a typo into other functions in that file and not know right away because you are only testing the display output. Anyway, that's my two cents.
-
NightZ, you will find people much more responsive when you format your posts appropriately, e.g. use [ CODE ] or [ PHP ] tags. From the Forum Guidelines Note: spaces added to prevent forum from parsing the tags.
-
This is a separate problem. Your first problem has apparently been fixed. You should mark this thread as solved and open a new topic for the numbering issue.
-
if (!(isset($pagenum))); { $pagenum = 1; } $pagenum is never defined before this code is run, therefore it will always get set to 1. Replace the above with this: $pagenum = (isset($_GET['pagenum'])) ? (int) $_GET['pagenum'] : 1;
-
Do you have the PECL extension installed? http://www.php.net/manual/en/ssh2.installation.php
-
Well, first of all, I would highly discourage using "1" and "2" as field names (assuming that wasn't just for illustrative purposes). Use a meaningful name. Anyway, you are creating the field names in a way that they will be processed by PHP as unindexed arrays. Therefore, PHP will give them numeric indexes starting at zero. Assuming you have exactlythe same number of field 1's and field 2's and they are in the same order as they are to be paired, you should be able to make the association that $field1[0] is paired with $field2[0], and $field1[1] is paired with $field2[1], and so on. However, I would prefer to be more explicit and to give my fields explicit indexes to ensure they are paired correctly <select name="field1[0]"> <option>1</option> <option value="2">2</option> </select> <input type="text" name="field2[0]" /> <select name="field1[1]"> <option>1</option> <option value="2">2</option> </select> <input type="text" name="field2[1]" /> ...etc.
-
Not sure I follow you. new path will contain the complete path from the root of a drive, so using % at the beginning doesn't make sense to me. Using % at the end will only find other entries that are paths to sub-folders, or childs, of the new path (which I do want tio find). I also need to find paths that are parent folders of new path. Here is an example.But, The new path to be added is: C:\folderB\folder1\apple\ If the following values are from the current entries, I would need to find the ones indicated with a [P] or [C] for a parent or child directory: C:\folderA\ [P] C:\folderB\ C:\folderA\folder1\ [P] C:\folderB\folder1\ C:\folderB\folder2\ [C] C:\folderB\folder1\apple\sub1 [C] C:\folderB\folder1\apple\sub2 C:\folderB\folder1\banana\ C:\folderB\folder1\pear\ Using the first query I posted I can find the child directories. I can use the second query I posted to get the parent directories, but it just didn't seem an efficient manner.
-
Seriously? It would have taken you less time to test it than it would for you to have posted here. Just put an on Die trigger to echo out the mysql_error() that will be produced
-
There must be some conflict then. You should always work from rendered HTML when trying to debug javascript code (i.e. not the pre-rendered PHP code). There was a lot of JavaScript and other code that I didn't have the time to try and figure out. So, when I removed all the code except for the necessary code to run the insert() function, it worked as I would expect. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" > <head> <title>nc-cms | HTML Editor</title> <meta http-equiv="content-type" content="text/html; charset=UTF-8"/> <meta name="robots" content="noindex" /> <meta name="robots" content="nofollow" /> <link rel="stylesheet" type="text/css" media="screen" href="system/css/editor.css"/> <link rel="stylesheet" type="text/css" media="screen" href="system/css/editor_html.css"/> <!--[if lt IE 7]><link rel="stylesheet" type="text/css" media="screen" href="system/css/ie.css"/><![endif]--> <script type="text/javascript"> function insert(){ var newtext = "test"; alert("test"); document.getElementById("editordata").value = "test"; //document.editorform.editordata.value = "test"; } </script> </head> <body> <div id="wrapper"> <div id="editor"> <h1 title="Powered by nc-cms"><?php echo NC_WEBSITE_NAME; ?> </h1> <form name="editorform" id="editorform" method="post" action="index.php?action=save&ref=<?php echo $_SERVER['HTTP_REFERER']; ?>" /> <br /> <textarea cols="102" rows="20" name="editordata" id="editordata" class="textfield">Initial Text</textarea> <br /> <button onclick="insert();">Insert Text</button> </form> </div> </div> </body> </html>
-
Here's a tip, when using PHP to generate JavaScript, always check the rendered HTML to ensure the JavaScript looks like what you think it should. In this istance your PHP is not writign anything to the page, so the Javascript is setting the value to nothing no matter what the value of number is. Also, although having separate IF statements would work when they are all testing for different values of the same variable, I doubt many would consider that proper design. Try this: <script type="text/javascript"> function insert_text(var number) { var newtext = (number==2) ? <?php echo $two; ?> : <?php echo $one; ?>; document.editorform.editordata.value = newtext; } </script>
-
$ignoreList = array('admins'); $query = "SHOW TABLE STATUS"; $result = mysql_query($query); while($record = mysql_fetch_assoc($result) { if(!in_array($record['Name'], $ignoreList)) { echo "Table: {$record['Comment']}<br />\n"; } }
-
I guess you are going to make me relpeat myself: [quiote]Without seeing the function getsub() there's no way to know what should be submitted There's nothing wrong that I can see with the code you have and, yes, using "this" as the parameter will pass an object reference of the object makign the call - in this instance the form object from which the onsubmit was called.
-
need to view javascript output to copy resulting source code
Psycho replied to detox's topic in Javascript Help
Without knowing how the javascript is outputting the HTML there is no way to help you. The JS is probably writing the HTML to the page using a document.write, changing the value of an objects innerHTML parameter or soemthign else. If you need to get the HTML output you are going to need to build a custom solution to do so. -
Without seeing the function getsub() there's no way to know what should be submitted. But, you are making it more difficult than it needs to be. You don't need to use getElementById() in your function call - just use 'this' which will reference the object making the call <form action="gosub(this);">
-
Although, if there is the possibility that it may not always be the first character that you need to get rid of, you can remove all characters that are not 0-9 or a decimal using the following: $beforediscountedPrice = preg_replace('/[^\d.]/','',$beforediscountedPrice);
-
[SOLVED] rand (1000, 1025)(2000,2025)(3000,3025)
Psycho replied to markvaughn2006's topic in PHP Coding Help
I'll throw in another solution: $values = array_merge(range(1000, 1025), range(2000, 2025), range(3000, 3025)); $rand_value = $values[array_rand($values)]; EDIT: MrAdam went with a similar solution, but mine picks a single random value out of the entire list instead of first selecting one of three lists and then selecting a value out of that. -
[SOLVED] looping throgh arrays with arrays inside them
Psycho replied to nadeemshafi9's topic in PHP Coding Help
If this solves your problem, please mark topic as solved.