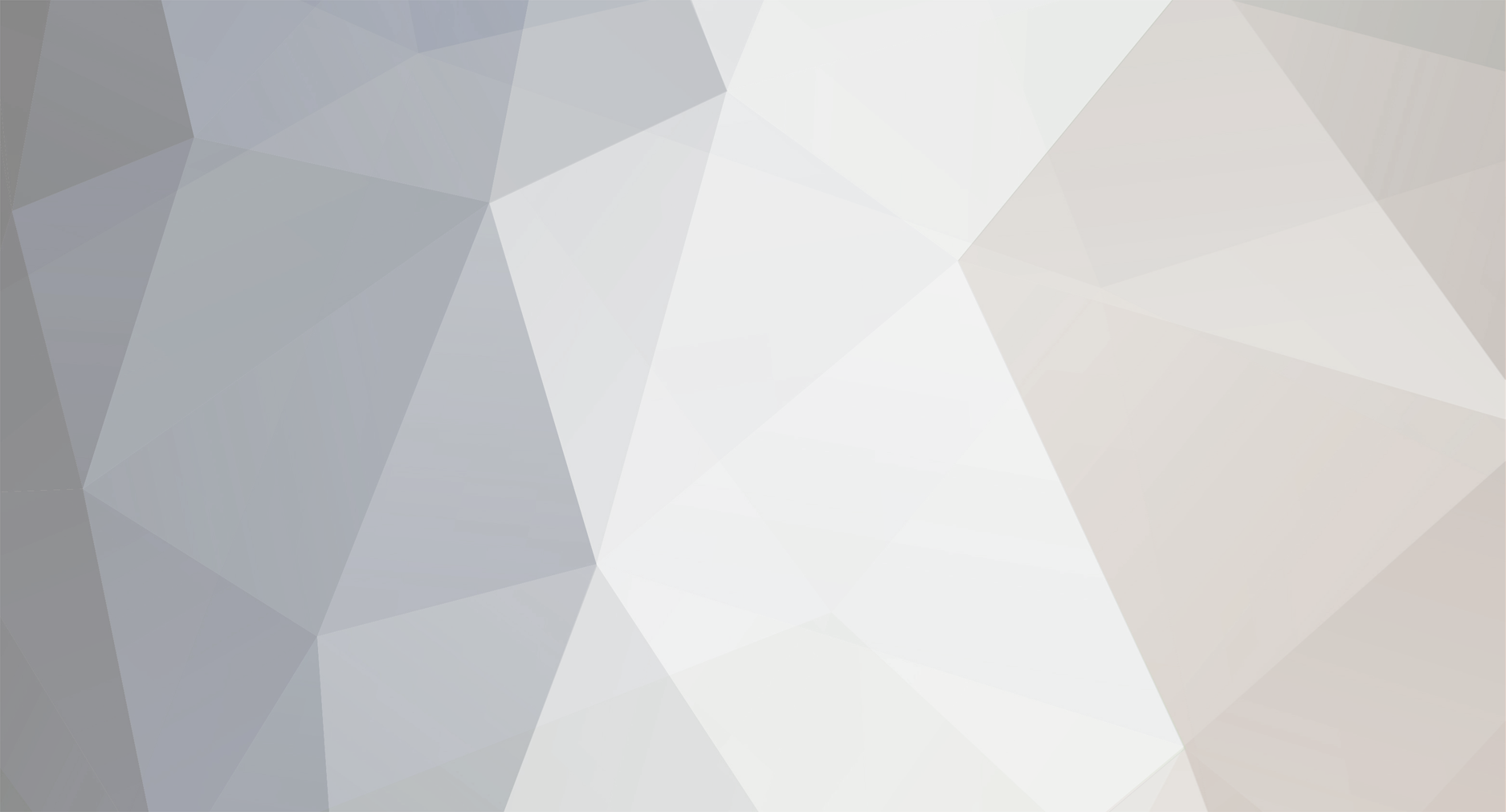
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
What is the format of the date? That would determine how you compare the dates, but assuming the dates are in the format yyyymmdd, then you could do this: function sortByreleaseDate($a, $b) { if ($a['release_date'] = $b['release_date']) { return 0; } return ($a['release_date'] < $b['release_date']) ? -1 : 1; } uasort($feed, 'sortByReleaseDate');
-
Well an UPDATE query won't return any results. For a SELECT query you would do a test of mysql_num_rows(). Example: //Prepare the query $query = "SELECT field1, field2, filed3 FROM tableName WHERE field1 = 5"; //$run the query, assigning to a variable $result = mysql_query($query); //Validate that query ran if(!$result) { //Query failed, add error handling } else { //Check that there were results if (mysql_num_rows($result)==0) { //There were no results } else { //There were results, process the result set } }
-
Well, if by logout you mean a specific button/link on the page that the user will click then, yes, you can do that. But, users will not "log out" of a web application. They will simply close the browser window. And, there is no way for PHP or the server to know if the user has closed their browser window. Instead of a table where you are constantly adding/deleting records, simply have a table with a record for every user (you can use the user table or another one). Each record will record the "last activity" of each user. On each page load you will update the value. Then when getting a list of "active" users, only get the ones whose last active timestamp is within a certain amount of time.
-
I think the problem is that the page must load three times to go through a cycle. The first page load will do nothing except display the form since $_POST['new'] is not set. The second page load, after submitting the form, will add the record. Then the third page load, after again submitting the form, will display the error condition.
-
Nothing stnds out as to why the field names wouldn't work past 9, but there are other issues. 1. That code will not work in browsers other than IE. You will need to create the fields using DOM (not just innerHTML). I don't have any code handy at the moment, but a little google searching should find a solution. 2. You shoudl just create all the field names as arrays and don't worry about adding an index number.Of course if you need to assign an id to the fields, then you do need an index for those, but not the field names. I.e.: name="fieldName[]"
-
Seeing the code you have would help.
-
mysql_affected_rows() tells you exactly that - if any rows were affected/updated. But, if the value is 0 that does not necessarily mean the query failed. The update query could have parameters such that the results are zero. For example, you may have a function to soft delete records that are x days old. If you ran it once and then try and run it again, the results of affected rows will be zero, but it did run successfully. So, use mysql_affected_rows() if you need to know if any records were uodated, but to see if the query ran succesfully you just need to test the query. Either this if (mysql_query($updateQuery)) { //success } else { //failure } Or this: $result = mysql_query($updateQuery; if ($result) { //success } else { //failure }
-
Personally, I would use similar logic to what you first described - execept that the initial processing page will first validate the submitted data. If validation fails I will redisplay the form with the values the user entered already populated/selected. If validation passes I will detemine the correct process to perform and include() the appropriate processing file. That way all of the GET/POST values are still available to the processing script.
-
The OP asked Unless this page is merely for learning purposes and there is no reason for the user to actually submit the page I don't see how JavaScript is an answer. Even if you wanted to have the JavaScript determine some value before submission it still couldn't be used for the only solution since the same logic would need to be copied on the server-side to take care of users without JavaScript enabled.
-
Put the options into an array (or pull from a database): $options = array( '0' => 'Option 1', '1' => 'Option 2', '2' => 'Option 3', '3' => 'Option 4', ); foreach($options as $value => $label) { $checked = ($option == $_POST['group_name'])? ' checked="checked"' : ''; echo "<input type=\"radio\" name=\"group_name\" value=\"{$value}\"{$checked} /> $value<br /> "; }
-
Well, no matter what you do you also need to validate on the server page that processes the input. You can handle this in different ways. Personally I don't like scripts that validate on every keystroke. Instead I will validate onblur or on submission of the form. But, to each his own. The following function should work fine. <html> <head> <script language="JavaScript" type="text/javascript"> function hasPeriods(value) { return value.match(/\./); } function checkDot(fieldObj) { if (hasPeriods(fieldObj.value)) { alert('Periods are not allowed.'); fieldObj.value = fieldObj.value.replace('.', ''); } return; } </script> </head> <body> <input type="text" name="test_field" onkeyup="checkDot(this);" /> </body> </html>
-
Worked fine for me in FF. I suspect there may be some other code on the page that is interfering, but that's just a guess
-
Write your code in a more meaningful, structured way and it will be easier to spot the errors. But, to be honest the script makes no sense. It appears that you are querying the database and after the first record is checked it will either determine the value already exists OR it will add it. But, it only checks the first record, not the others. You should be using criteria in your query. I don't see where $dbusername is defined, so I will it is assigned a value before this section of code <?php if ($submit) { if($groupname) { $connect = mysql_connect("localhost","damgears_blaster","blaster1"); mysql_select_db("damgears_blaster");$groupname $query = "SELECT * FROM groups WHERE groupname = '$groupname' AND user = '$dbusername' "; $result = mysql_query($query); if (mysql_num_rows($result) === 0) { $query = "INSERT INTO groups (groupname, user) VALUES ('$groupname','$dbusername')" mysql_query($query); $response = "Added Group $groupname! $dbusername"; } else { $response = "Group $groupname Already Exists"; } } else { $response = "Please fill in all field"; } } else { $response = "You are not logged in!!! Please login to continue"; } ?> <html> <head></head> <body> <?php echo $response; ?> </body> </html>
-
You should probably make a new post. You now have a database query result with all the records you need and your problem is now procedural in trying to ensure all the users in the result set get sent an email
-
<html> <head> <script language="JavaScript" type="text/javascript"> function enterCheck(e) { var keyCode = (e.keyCode) ? e.keyCode : e.charCode ? e.charCode : e.which; if (keyCode == 13) { alert('User pressed enter key'); } } </script> </head> <body> <input type="text" name="test_field" onkeypress="enterCheck(event);" /> </body> </html>
-
A forum isn't exactly an appropriate medium for explaining all of this. That is why there are tutorials. Have you looked at any tutorials for this? If you have problems understanding something in those tutorials then post a question for clarification. This should get you started: http://www.sitepoint.com/article/generate-pdfs-php/ Note: I have not read/followed that tutorial so I cannot comment on its quality, but the general descriptions sounds like it is just what you need. However, I'm not sure if you can edit an already created PDF document with the PDF library.
-
Ok. I had tried the code you posted and it worked for me with no problems. Don't know what keys you are having problems with, but I had not problems. By the way, the code you have would only work in IE as other browsers don't recognize the "event" object.
-
What you are doing doesn't make sense. You are trying to replace text based upon the result of the function which requires for the replacement to be made first. You need to break out the logic. <?php function create_image($imgid) { return $imgid.",".(int)$imgid; } $text = "[img=16]http://"; $id = preg_replace("/[img=(.+?)]/", '$1', $text); echo create_image($id); //Output: 16,16 ?>
-
Your request isn't clear. It appears you are trying to implement JavaScript functionality to automatically submit a form when the user presses enter. A properly constructed form will do that automatically as long as you have a submit button or elementon the page. I am thinking that the enter key is working right away because of the default form behavior and not your script. You probably need to put an onsubmit() trigger on your form if you don't want it submitted automatically on the enter key. Honestly, I have no clue what you are trying to achieve. So, I can't lend any advice.
-
You are correct. This whole subject is pointless in my opinion. It adds absolutely no value to the user and trying to implement a hack could only serve to create a security risk.
-
I have to ask, why did you find it necessary to change the code I gave you? Do you realize that the change you made is flawed? Did you even read my response in reply #7? Go ahead and create enough groups so you have at least one two-digit group code (i.e. '10'), then use that query for group code '1'. And guess what, the query will return records that are associated with group 10 as well as group 1.
-
You can set the font of the field itself like this <input type="password" style="font-family:wingdings"> I did see a page when I was looking for this previously that changed the masked character to a vertical line.
-
I can't comment on your results. I tested the code I provided and it worked fine for me. The expression I provided is only used to get the results from the database, what you do with those results is out of my hands. Did you at least try running the query and just display the results to see if they are what you expect? Trying to run a query, performing additional functinality on top of those results, and then determining if the query was sufficient by analyzing the output is not legitimate as the post processign could be where the error is. To answer your question the the regex expression does this: 1. Find the value (period)(groupID)(period): \.{groupID}\. OR | 2. Find the value (period)(groupID) at then end of the value: \.{groupID}$
-
Assuming you don't need to reuse all those variables: $calls['stamp']="2009-07-26 12:02:53";; echo date('n-j-Y g:i:s', strtotime($calls['stamp'])); // Output:7-26-2009 12:02:53
-
Well, whoever created a DB design to use a single field to store multiple associations doesn't understand database normalization. Since you are stuck with a jacked up design, I guess I'll show you a jacked up way to work with it. $grpID = mysql_real_escape_string(trim($_POST['sendto'])); //Select any row where the group ID exists $query = "SELECT * FROM ".DB_USERS." WHERE user_newsletter = 1 AND user_groups REGEXP '\.{$grpID}\.|\.{$grpID}$'";