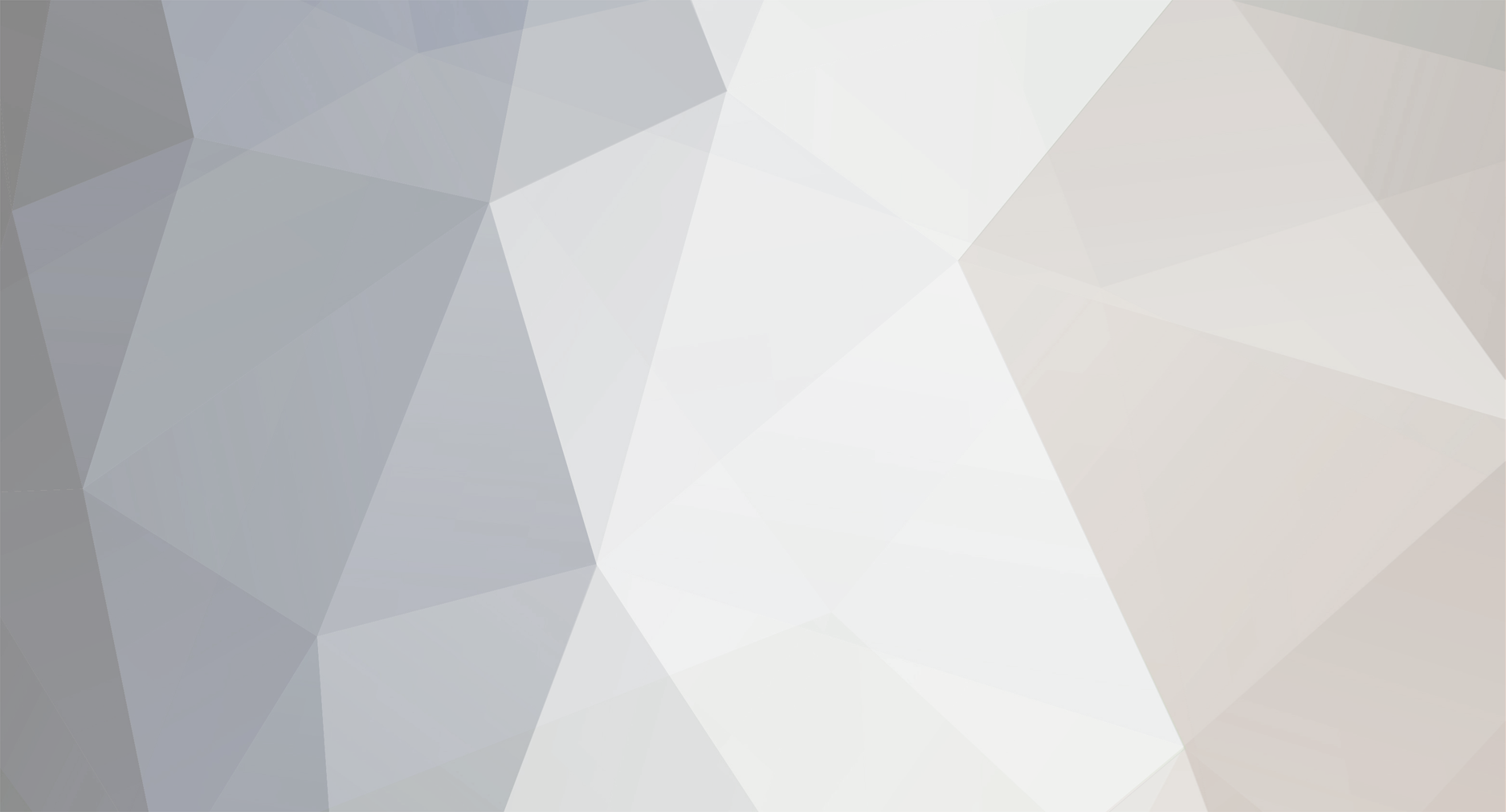
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
No, no ,no. You do NOT need to update the Teams table with the results from the Matches table. The whole point of using a relational database is that you can access records across tables in a single query. So, if you have a page to display a team's profile you access ALL the data dynamically in real time. You need to look at how to JOIN tables in your queries. You could do a JOIN in this instance, but I would just use two subqueries. Run this query and you will get all the profile data for a team as well as their win/loss record in a single query. $team_id = 5; // $query = "SELECT *, (SELECT COUNT(*) FROM test WHERE (team_id_1=$team_id OR team_id_2=$team_id) AND winner=$team_id) as wins, (SELECT COUNT(*) FROM test WHERE (team_id_1=$team_id OR team_id_2=$team_id) AND winner<>$team_id) as losses FROM `teams` WHERE teams.team_id = $team_id";
-
[SOLVED] Inserting information into an SQL DB
Psycho replied to ciaran1987's topic in PHP Coding Help
The last echo statement will fail as well <?php $category = mysql_real_escape_string($_POST['category']); $problem = mysql_real_escape_string($_POST['problem']); $solution = mysql_real_escape_string($_POST['solution']); mysql_connect ("localhost", "root","password") or die (mysql_error()); mysql_select_db ("heskdb"); $query="INSERT INTO `hesk_std_replies` (`category`, `problem`, `solution`) values ('{$category}', '{$problem}', '{$solution}')"; mysql_query($query) or die ('error updating database'); echo "Database updated with: {$category}, {$problem}, {$solution}"; ?> -
Well, there is apparently some details you are leaving out, which makes this more difficult to debug. Based upon the code above I would have to assume there is a gloabl array variable for "questionno". Also the function you have never increases the variable "number" (see note below). Lastly, I don't se anything that actually adds the field to the page! Once I made those changes, it works fine for me. form page <html> <head> <script type="text/javascript"> //Gloabl variables var questionno = new Array(); var number = 0; function addquestion() { //Create the form element questionno[number] = document.createElement("input"); questionno[number].id = "questionno" + number; questionno[number].name = "questionno" + number; questionno[number].size = 2; questionno[number].type = "text"; questionno[number].value = number; //Add form element to page document.getElementById("questionForm").appendChild(questionno[number]); number++; return; } </script> </head> <body> <form name="question" id="questionForm" method="post" action="thanks.php"> <input type="button" id="create" value="add" onClick="addquestion()"> <input type="submit" id="savecreate" name="savecreate" value="save"> </form> </body> </html> thanks.php page <?php echo "<pre>"; print_r($_POST); echo "</pre>"; ?> If I add three fields to the form page the results I get on the thanks.php page are as follows: Array ( [savecreate] => save [questionno0] => 0 [questionno1] => 1 [questionno2] => 2 ) however, it is completely unnecessary to add an index number to every field - and not as efficient. Simply create the fields with a name such that the resulting POST data will be returned as an array variable. (you would need to use an index for the ID values for the fields if you must use that). questionno[number].name = "questionno[]"; Then on the processing page you can access all of the values entered into that group of fields using the variable $_POS['questionno'] which will be an array of all the values.
-
[SOLVED] simple time and date conversion help please!
Psycho replied to icklechurch's topic in PHP Coding Help
That code fixes the issue where the month and day were transposed, but there is still another problem. By specifying a "Day" of 0 (zero) you will get incorrect results (i.e. the previous month) since it interprets that as the last day of the previous month. Need to set the day to 1. <?php echo date('F Y', mktime(0, 0, 0, $_GET['m'], 1, $_GET['y'])); ?> However, after realizing that problem I found that you could also use negative numbers for the day. So, -1 would the the second to last day of the previous month. That's an interesting feature. Not sure how it may be used, but using zero to specifiy the last day of the month will certainly come in handy. -
Perhaps it's because you are creating the field names as "questionno"+number and you are trying to access with the name of $_POST['question0'] Assuming "number" is 0, you should be using $_POST['questionno0']
-
[SOLVED] Can't figure out what's wrong with my code...
Psycho replied to LostinSchool's topic in Javascript Help
Please mark topic as solved -
[SOLVED] Can't figure out what's wrong with my code...
Psycho replied to LostinSchool's topic in Javascript Help
If you check the errors produced, you shoudl have enough info to determine the problems: First error: Line: 37 Error: Syntax Error There is a closing bracket after the else, but no opening bracket. Just remove the closign bracket on line 38/ 36 } 37 else 38 } 39 return true; Second error: Line: 46 Error: Syntax Error There's a closing bracket on line 46 with no corresponding opening bracket. Remove it. 41 function confirmReset() { 42 var resetForm = window.confirm("Are you sure you want to reset the form?"); 43 if (resetForm == true) 44 return true; 45 return false; 46 } 47 } That will get your validation working (didn't test it all). As to the problem with your FormProcessor.html page, that page would need to have server-side scripting in order to access the POST data. As far as I know you can't access that data directly with HTML/JavaScript code. Also, here's a tip - don't create unneeded variables. No need to assign a value to a variable to use it just once. This function function checkForNumber(fieldValue) { var numberCheck = isNaN(fieldValue); if (numberCheck == true) { window.alert("You must enter a numeric value!"); return false; } } Could have been written simply as this function checkForNumber(fieldValue) { if (isNaN(fieldValue)) { window.alert("You must enter a numeric value!"); } return (!isNaN(fieldValue)); } Then if you need to reference something many times, then you should create a variable. I would have written this function confirmSubmit() { if (document.forms[0].name.value == "" || document.forms[0].address.value == "" || document.forms[0].city.value == "" || document.forms[0].state.value == "" || document.forms[0].zip.value == "" || document.forms[0].email.value == "" || document.forms[0].telephone.value == "") { window.alert("You must enter your personal information."); return false; } else { return true; } } Like this function confirmSubmit() { var df = document.forms[0]; if (!df.name.value || !df.address.value || !df.city.value || !df.state.value || !df.zip.value || !df.email.value || !df.telephone.value) { window.alert("You must enter your personal information."); return false; } return true; } -
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
Perhaps it would. have you tried it? -
Yes, I understand the difficulty in trying to reverse engineer someone elses poorly documented code. And, I think building your own solution is a good idea. I would just limit your request to a single piece of functionality instead of a blanket statement for a lot of functionality. For example, if you wanted a piece of code that would "scan" a folder for all images you would probably get several solutions. But, I know I as well as others like to at least see a person make an attempt instead of just handing them code. I wish you all the luck.
-
You are asking for a lot. There are many photo galleries out there that have these functions. You can go review those and try to reengineer the code to fit you or you can break down the functionality you want into specific requirements: 1) Allow user to browse to and select a folder. 2) Allow user to specify which photos to include in "album" 3) Create thumbs of the photos. 4) Process allt he selected images to a) Create thumbs and b) save to database Etc., Etc. Then just tackle one task at a time. In some instances you will probably be able to find some existing code or you can proably get some help here for specific pieces of functionality. But, I think the only placve you are going to find all of that type of functionality already existing together is in applications that already exist.
-
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
Sorry, if I was a bit harsh, but when someone asks "will this work" it appears they only want someone to provide a solution to them and are unwilling to be part of the solution by participating in the process. I am only here to help people that want to help themselves. Not saying that is your attitude, but that is how I perceive that type of statement. In any event, this is no longer a javascript issue. The JavaScript is fine. This is a CSS issue. You need to find a way to construct the page such that you can change the CSS properties to display/hide the partial content that is cross-browser compliant. -
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
Why don't you just try it? Edit: this is a browser compatability issue. Works fine in IE, but not in FF. You'll need to experiment with the format to make it work in both. -
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
That was what I was referring to, but as I said it won't look consistent on every browser. <HTML> <head> <script> function showHide(idx) { var textDiv = document.getElementById('text_'+idx); var linkObj = document.getElementById('display_'+idx); textDiv.style.overflow = (textDiv.style.overflow != 'visible') ? 'visible' : 'hidden'; linkObj.innerHTML = (textDiv.style.overflow != 'visible') ? 'Show' : 'Hide'; return; } </script> </head> <body> <a href="#" onclick="showHide(1);" id="display_1">Show</a><br> <div id="text_1" style="width:250px;height:50px;overflow:hidden;"> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vestibulum suscipit congue diam. Vivamus nisi. Curabitur augue libero, hendrerit vel, porttitor id, bibendum ut, massa. Maecenas porta lectus vitae erat. Praesent quam. Nunc lacus augue, sollicitudin ac, mattis ut, consectetur eget, urna. Mauris tortor dolor, hendrerit id, sollicitudin ut, egestas vitae, lacus. Nulla sed sem. Pellentesque scelerisque erat id nisl. Morbi sed purus in velit volutpat tempor. Mauris a metus. Suspendisse eleifend malesuada enim.</div> </body> </html> -
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
Um, that is a CSS effect. CSS is not programatical; you can't use it to logically display a certain number of characters/words. You could use javascript to change the "height" of the container div so it only takes up so much room. But, the problem there is that you can't control exactly how each browser will display the page. So, you might only have 2 lines of text show in one browser, whereas in a different browser you woudl get two lines and the top half of the third line. That would be the simplest approach though. To keep the HTML formatting and use the method I provided can be done, but would involve more code to handle the conversion. -
If you try to echo it in an HTML page it will "appear" on one line, but the line breaks are there. When a browser renders HTML code it does not interpret actual line breaks (only BRs). The exceptions would be when you use a PRE tag or populate a textarea, etc. If you look at the actual HTML code that is genmerated you will see that the line breaks are there. It will work fine as long as you use the value (without the nl2br() ) in a container that will interpret them - as I would expect your process of populating a word document would do. When I used the code above like this: //Format the record data $myData = getMyData($row); //Output the data NOT for HTML output echo $myData; The "rendered" HTML in the browseer looks like this: Alpha1 Alpha1a Alpha2 Alpha2a Alpha3 Alpha3a However, if you look at the actual HTML code, it looks like this Alpha1 Alpha1a Alpha2 Alpha2a Alpha3 Alpha3a That is how you should want your data - keep it in it's most basic format and the transform the data based upon the format of the output. (such as using nl2br() when outputin onto an HTML page)
-
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
You could have also used something other than a "link", such as a div with an onclick event or a button. -
I'll deal with your second question firt. You should compile your data using logical line breaks. Then when you want to display it in HTML use nl2br() to add BR tags where there are line breaks. As to the first problem, just use appropriate IF statements to build the output appropriately. I would use a function to do this since it appears you may need to run this in a loop. function getMyData($dataAry) { $myData[] = array(); if ($dataAry['A1']!='' || $dataAry['A1a']!='') { $myData[] = trim($dataAry['A1'] . ' ' . $dataAry['A1a']); } if ($dataAry['A2']!='' || $dataAry['A2a']!='') { $myData[] = trim($dataAry['A2'] . ' ' . $dataAry['A2a']); } if ($dataAry['A3']!='' || $dataAry['A3a']!='') { $myData[] = trim($dataAry['A3'] . ' ' . $dataAry['A3a']); } $myDataStr = implode("\n", $myData); return $myDataStr; } while ($row = mysql_fetch_assoc($result)) { //Format the record data $myData = myData($row); //Output the data for HTML output echo nl2br($myData) . '<br>'; //To output the data to a word doc, //just use $myData without the nl2br }
-
<html> <head> <script type="text/javascript"> function updateText() { var field1Value = document.getElementById('field1').value; var field2Value = document.getElementById('field2').value; var field3Value = document.getElementById('field3').value; var newText = field1Value + '\n' + field2Value + '\n' + field3Value document.getElementById('output').value = newText; return; } </script> </head> <body> Field 1 <select name="field1" id="field1" onchange="updateText();"> <option value="">--SELECT--</option> <option value="line1-A">line1-A</option> <option value="line1-B">line1-B</option> <option value="line1-C">line1-C</option> </select><br> Field 2 <select name="field2" id="field2" onchange="updateText();"> <option value="">--SELECT--</option> <option value="line2-A">line2-A</option> <option value="line2-B">line2-B</option> <option value="line2-C">line2-C</option> </select><br> Field 3 <select name="field3" id="field3" onchange="updateText();"> <option value="">--SELECT--</option> <option value="line3-A">line3-A</option> <option value="line3-B">line3-B</option> <option value="line3-C">line3-C</option> </select><br><br> Textarea:<br> <textarea name="output" id="output" rows="5"></textarea> </body> </html>
-
If I'm not mistaken that code will only destroy the "session" cookie. You need to destroy all the cookies with that user. But, here's the thing - the whole point of a cookie is that your data can be saved on the client side. Why store any data in cookies if you want to destroy it between sessions - use session data. But, let's assume you want the cookie data to be remembered IF the user logs in with the same credentials as the previous visit. After authentication save a cookie value for the username/userID/or some unique value. Then when user logs in check if there is a cookie for that value and if it matches the value of the user that just logged in. If they are the same - do nothing - the user will retain their "cookie" cart from the previous visit. If they are not the same, then destroy all the previous cookie values. foreach ($_COOKIE as $name => $value) { setcookie($name, '', time()-1); }
-
All of your echo statements are within IF conditions. All of them must have resulted in false. When you use an include() it is basically the same as if you had copied and pasted the code from the included file into the original file at the location of the include. It's not a difficult concept. file1.php <?php echo "Hello"; include('file2.php'); echo "World"; ?> file2.php <?php echo " Foo Bar "; ?> The output from accessing page1.php would be Hello Foo Bar World
-
[SOLVED] Limit text in an area, and click button to view all?
Psycho replied to Jason28's topic in Javascript Help
I think this is a two step process. The first step would be to process the text server-side (assuming PHP). Using PHP determine what part of the text should be displayed in partial vs. full mode. Then split the text accordingly. The strait-forward approach would be to use number of characters since determining the number of lines would be difficult, if not impossible. Then echo both pieces of text to the page such that they can be controlled via javascript. Assuming you have mutiple pieces of text that would be displayed I would suggest adding an index number to each piece. Here is an example of how the PHP code could look $index = 1; $maxLength = 50; //max characters to show for the preview while ($record = mysql_fetch_assoc($result)) { $text = $record['text']; //Determin if preview is needed $preview = (strlen($text)>$maxLength); if ($preview) { echo "<a href=\"#\" onclick=\"showHide({$index});\" id=\"display_{$index}\">Show</a><br>\n"; } echo "<div id=\"text_{$index}\">\n"; if ($preview) { //Preview needed, break out the text on the space before $maxLength //Determine the break point $tempString = substr($text, 0, $maxLength+1); $breakPoint = strrpos($tempString, ' '); //Display the text parts echo "<span>" . substr($text, 0, $breakPoint) . "</span>"; echo "<span id=\"ellipse_{$index}\">...</span>"; echo "<span id=\"full_{$index}\" style=\"display:none;\">" . substr($text, $breakPoint) . "</span>"; } else { //No preview needed, display entire text string echo $text; } echo "</div><br><br>\n"; $index++; } Then use a javascript function to show/hide the full text or the ellipse (plus chang ethe link text) function showHide(idx) { var fullTextObj = document.getElementById('full_'+idx); var ellipseObj = document.getElementById('ellipse_'+idx); var linkObj = document.getElementById('display_'+idx); if (fullTextObj.style.display != 'inline') { fullTextObj.style.display = 'inline'; ellipseObj.style.display = 'none'; linkObj.innerHTML = 'Hide'; } else { fullTextObj.style.display = 'none'; ellipseObj.style.display = 'inline'; linkObj.innerHTML = 'Show'; } return; } Here is a fully working page made from the PHP and JavaScript code above <html> <head> <script type="text/javascript"> function showHide(idx) { var fullTextObj = document.getElementById('full_'+idx); var ellipseObj = document.getElementById('ellipse_'+idx); var linkObj = document.getElementById('display_'+idx); if (fullTextObj.style.display != 'inline') { fullTextObj.style.display = 'inline'; ellipseObj.style.display = 'none'; linkObj.innerHTML = 'Hide'; } else { fullTextObj.style.display = 'none'; ellipseObj.style.display = 'inline'; linkObj.innerHTML = 'Show'; } return; } </script> </head> <body> <a href="#" onclick="showHide(1);" id="display_1">Show All</a><br> <div id="text_1"> <span>FORT MYERS, Fla. – A passenger landed a</span><span id="ellipse_1">...</span><span id="full_1" style="display:none;"> twin-engine plane in Florida after the pilot died in flight with a total of six people on board. Federal Aviation Administration officials say the pilot died after takeoff from an airport in Naples on Sunday. It was on autopilot and climbing toward 10,000 feet when the pilot died.</span></div><br><br> <a href="#" onclick="showHide(2);" id="display_2">Show All</a><br> <div id="text_2"> <span>SPRINGDALE, Ark. - Josh and Anna Duggar are</span><span id="ellipse_2">...</span><span id="full_2" style="display:none;"> following in the family tradition. Josh, the eldest of Michelle and Jim Bob Duggar's 18 kids, announced Monday that he and new bride Anna were about to start their own brood. The couple, who's been married since September, said Anna Duggar is due Oct. 18</span></div><br><br> <a href="#" onclick="showHide(3);" id="display_3">Show All</a><br> <div id="text_3"> <span>BANGKOK - Thousands of troops fired warning shots</span><span id="ellipse_3">...</span><span id="full_3" style="display:none;"> and tear gas to turn back rampaging anti-government protesters Monday night, forcing retreating demonstrators into one neighborhood where a clash with residents left two people dead</span></div><br><br> </body> </html> -
I can't tell from your code if you expect the select list to also remove rows or only to add rows. If it needs to delete rows when changing the value, it is much more difficult because of how different browsers count childNodes, but this will work to add rows (tested in IE & FF) Note, change the field names to name[] so the values will be treated as an array by the processing page. Also, you should remove the IDs from the input fields since you can't have multiple items on a page with the same ID. Also, I made the initial row hidden. Otherwise when you copy the row youalso copy any values the user has already entered. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <script type="text/javascript"> function changeFormLength(newRows) { //Create references to table bode and reference row var formObj = document.getElementById('form2').getElementsByTagName("TBODY")[0]; var defRowObj = document.getElementById('defaultRow'); for (var row = 1; row<=newRows; row++) { //Create new row object var newRow = document.createElement("TR"); //Create the cells for th enew row for(i=0; i<defRowObj.childNodes.length; i++) { if (defRowObj.childNodes[i].tagName=='TD') { var newTDObj = document.createElement("TD"); newTDObj.innerHTML = defRowObj.childNodes[i].innerHTML; newRow.appendChild(newTDObj); } } //Append the new row to the table formObj.appendChild(newRow); } return; } </script> </head> <body> Add how many rows?<br /> <select name="rows" id="rows" onchange="changeFormLength(this[this.selectedIndex].value);"> <option value="1" selected="selected">1</option> <option value="5">5</option> <option value="10">10</option> <option value="15">15</option> <option value="20">20</option> <option value="25">25</option> <option value="30">30</option> <option value="35">35</option> <option value="40">40</option> <option value="45">45</option> <option value="50">50</option> <option value="55">55</option> <option value="60">60</option> </select> <form id="form2" name="form2" method="post" action=""> <label></label> <label></label> <label></label> <table width="78%" border="1" cellpadding="2"> <tr> <td width="17%">First Name</td> <td width="17%">Last Name</td> <td width="14%">Card Number</td> <td width="16%">Card Type</td> <td width="23%">Card Expiration</td> <td width="5%">CVV</td> <td width="8%">Ammount</td> </tr> <tr id="defaultRow" style="display:none;"> <td><input type="text" name="firstname[]" id="firstname" /></td> <td><input type="text" name="lastname[]" id="textfield2" /></td> <td><input name="textfield3[]" type="text" id="textfield3" size="20" maxlength="16" /></td> <td><label> <select name="cc_type[]" id="select"> <option value="visa" selected="selected">Visa</option> <option value="mastercard">MasterCard</option> <option value="discover">Discover</option> <option value="amex">American Express</option> </select> </label></td> <td><label> <select name="cc_exp_m[]" id="cc_exp_m"> <option value="01">1 January</option> <option value="02">2 Feburary</option> <option value="03">3 March</option> <option value="04">4 April</option> <option value="05">5 May</option> <option value="06">6 June</option> <option value="07">7 July</option> <option value="08">8 August</option> <option value="09">9 September</option> <option value="10">10 October</option> <option value="11">11 November</option> <option value="12">12 December</option> </select> / <select name="cc_exp_y[]" id="cc_exp_y"> <option value="2009">2009</option> <option value="2010">2010</option> <option value="2011">2011</option> <option value="2012">2012</option> <option value="2013">2013</option> <option value="2014">2014</option> <option value="2015">2015</option> <option value="2016">2016</option> <option value="2017">2017</option> <option value="2018">2018</option> <option value="2019">2019</option> <option value="2020">2020</option> </select> </label></td> <td><input type="text" name="cc_cvv[]" id="cc_cvv" size="5" maxlength="4" /></td> <td><input type="text" name="amt[]" id="amt" size="10" maxlength="7" /></td> </tr> <div id="rowSpace"> </div> </table> </form> </body> </html>
-
Just set the dsplay value to an empty string and the browser will display it as it you hadn't set any display value to begin with. Also, that function could be written much more simply function show_hide_uploadfields(num) { var idx = 1; while(document.getElementById('file'+idx)) { var displayValue = (idx<=num)?'':'none'; document.getElementById('file'+idx).style.display = displayValue; idx++; } }
-
Hmm... you really didn't provide enough informatin as to what you are trying to accomplish. I will rephrase what I think you are trying to accomplish and ask some questions to validate: You are starting with a select list with a single empty item (value of 1, text is empty) - or does that select list have multiple values and you are only showing part of the code. You then want to change the "value" of the select list. The question is, do you want to change the value of the currently selected option or do you want to add an option with the new value and then select that value (leaving the original option(s) in place)? Try explaining what you want to happen from a user perspective.
-
Um, query the database for the values. Run them through the function to generate the new values, and put them back in the DB.