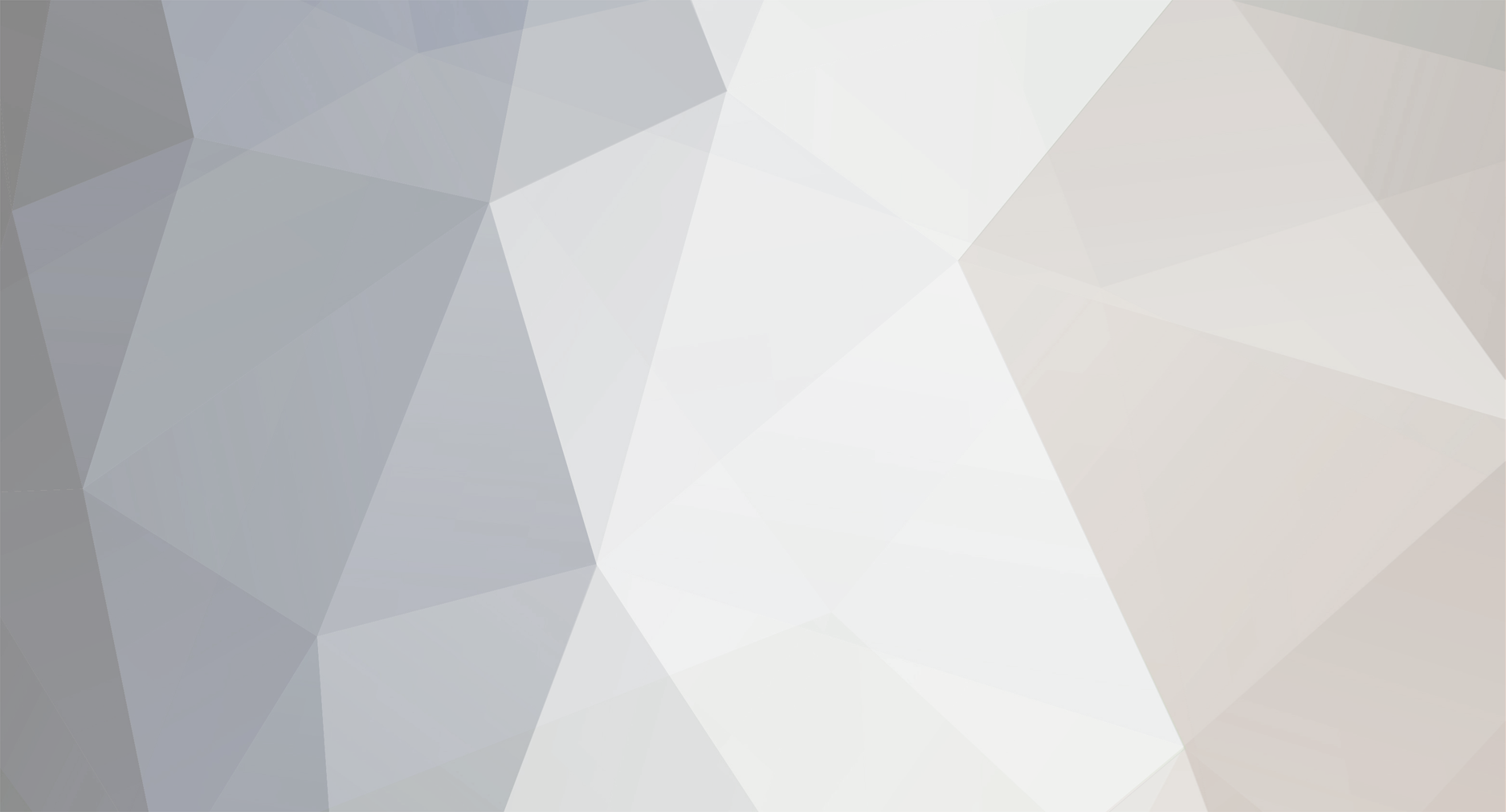
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
[SOLVED] How do I pass two variables from php to java script?
Psycho replied to Hardwarez's topic in Javascript Help
I'm assuming this is AJAX? I typically just concatenate the values together with a delimiter and then send them to javascript as a single string. Then the javascript parses the string based on the delimeter. Just be sure to pick a delimeter that would not be included in the return values. PHP <?php $var1 = "foo"; $var2 = "bar"; $returnVal = $var1 . '|' . $var2; echo $returnVal; ?> JavaScript xmlHttp.onreadystatechange=function() { if(xmlHttp.readyState==4) { var varArray = xmlHttp.responseText.split('|'); document.myForm.var1.value=varArray[0]; document.myForm.var2.value=varArray[1]; } } -
Try this if ($id != (int)$id) {
-
Well, after you run the update you will need to run the SELECT if you want to display the data. You will also want to create content to display for the error conditions (ID not an int, ID not in database, etc.) <?php $id=mysql_real_escope_string($_GET['id']); if ($id !== (int)$id) { echo "Invalid ID"; } else { $sql = "UPDATE interviews SET views=views+1 WHERE id='$id'"; mysql_query($sql) or die (mysql_error()); $sql="SELECT * FROM interviews WHERE id='$id'"; $result = mysql_query($sql) or die (mysql_error()); $rows=mysql_fetch_array($result) } ?>
-
Just add some debugging code to see what is going on: <?php $id=$_GET['id']; echo "ID passed on query string [".$id."]<br>"; if ($id == (int)$id) { echo "ID is an int<br>"; $sql = "UPDATE interviews SET views=views+1 WHERE id='$id'"; echo "Query: $sql<br>"; mysql_query($sql) or die (mysql_error()); } ?>
-
Here is a solution for both: <?php $today = time(); $dow = date("w", $today); echo "Week from Sunday to Saturday<br>"; $sunday = $today - ($dow * 86400); $saturday = $today + ((6-$dow) * 86400); echo "Today is: ".date('F j, Y', $today)."<br>"; echo "First DOW (Sunday) is: ".date('F j, Y', $sunday)."<br>"; echo "Last DOW (Saturday) is: ".date('F j, Y', $saturday)."<br>"; echo "<br><br>"; echo "Week from Monday to Sunday<br>"; $monday = ($dow==0)?$today - (6 * 86400):$today - (($dow-1) * 86400); $sunday = ($dow==0)?$today:$today + ((7-$dow) * 86400); echo "Today is: ".date('F j, Y', $today)."<br>"; echo "First DOW (Monday) is: ".date('F j, Y', $monday)."<br>"; echo "Last DOW (Sunday) is: ".date('F j, Y', $sunday)."<br>"; ?>
-
Hmm, are you considering Sunday as the last day of the week (Mon - Sun)? Becauser in actuality Sunday is the first day of the week and Monday is the 2nd day of the week. Saturday is the last day. (Sun - Sat) The former will be slightly more complex to do, but definitely doable.
-
http://www.url.domain/blah.php?id=3&blah.htm - HOW?
Psycho replied to Perry|'s topic in PHP Coding Help
How do you create it? You just did, didn't you. I think your question needs to be more specific. But, I will provide some info that might be what you are looking for. First off you must have a valid "base" path to a page such as http://www.mydomain.com Then you can add as many name/value pairs as you need. You start by adding a question mark after the base URL and then separate name value pairs with an ampersand. And, name/value pairs are indicated with an equal sign. Here is an example with spaces added for clarity http://www.mydomain.com ? id=14 & page=display & method=list You can then access those values in the php code using the $_GET array like so: echo "The page value is " . $_GET['page'] -
You really shuldn't be using FONT tags any more, but here you go: <?php $result = mysql_query( "SELECT * FROM merc_users" ) or die("SELECT Error: ".mysql_error()); print "There are " . mysql_num_rows($result) . " records.<P>"; print "<table width=\"1000\" height=\"400\" border=\"1\">\n"; $showHeaders = true; while ($get_info = mysql_fetch_row($result)){ //Show headers on 1st pass if ($showHeaders) { print "<tr>\n"; foreach ($get_info as $header => $field) print "\t<th><font face=\"arial\" size=\"2\">$header</font></th>\n"; print "</tr>\n"; $showHeaders = false; } //Display the record data print "<tr>\n"; foreach ($get_info as $field) print "\t<td><font face=\"arial\" size=\"2\">$field</font></td>\n"; print "</tr>\n"; } print "</table>\n"; ?>
-
The part about part of the text "disappearing" is very odd. I don't see how that could possibly happen with the code you have. When I run into these types of problems I will sometimes remove all the code from the effected area and then add a line (or a few lines) back in and then test. If those linse work correctly I will ad a few more back and so on. Once it "breaks" I know the line(s) of code that are causing the problem.
-
You should have proper error handling. Try this: <?php $pagename1=mysql_real_escape_string($_GET['member']); $query = "UPDATE members SET count = count + 1 WHERE pagename='$pagename1'"; mysql_query ($query) or die ("Error:<br>".mysql_error()."<br>Query:<br>$query"); //For testing purposes echo "Query:<br>$query<br>Rows Affected: ".mysql_affected_rows(); ?>
-
Take a look at the HTML in the processed page. I can see at least a ouple of problems which may, or may not, be related to your specific problem. For example, you are printing strngs using single quotes and you have varialbes inside the string. Variable are only interprested inside strings created with double quotes. Also, you have a HTML break tag at the end of the TR closing tag for the form. It shouldn't screw up the form, but it is not valid HTML to have "content" inside a table that is not inside TD tags. There's no telling how each brower will interpret that.
-
Can you please post a "test" page with all the necessary code to test this?
-
I don't have the time to test that out, but this should be the problem: The "div" name in the function call does not match the one you are testing against in the function: var cheese = open_url("page.php", "returnin"); --------------------------------- else if (div == "returnit") {return link.responseText;}
-
Showoff! RegEx is geekier.
-
I don't understand the scripts you have above as they don't correspond to what yousay you want to do. The following function will insert an HTML break tag at every 50 characters: <?php function formatME($input) { return preg_replace('/(.{50})/', '$1<br />', $input); } ?>
-
What do you mean? That query format will return exactly what you just stated. Just modify the query for the item name and it will return a list of all the items and the number of times each item exists in the table.
-
function showInMap(coords,imageID){ var tag = document.getElementById('showTag'); tag.style.position = 'absolute'; tag.style.border = 'solid 2px #9a0000'; var left = document.getElementById(imageID).offsetLeft; left = parseInt(left); var top = document.getElementById(imageID).offsetTop; var nums = coords.split(','); alert(parseInt(top) + parseInt(nums[1])); tag.style.left = left+'px' }
-
This appears to work too if (!@fopen($file, 'r')) EDIT: Just did some searching and it appears the manual for file_exists() includes several scripts in the user comments for external filess: http://us.php.net/manual/en/function.file-exists.php
-
Here's a quick and dirty function that will work using file_get_contents. Although I remember reading information about this previously that there are some specific issues when doing this. It worked n some simple tests that I ran though. function httpFileExists($url) { if (!@file_get_contents($url)) { return false; } return true; }
-
populate textarea with checkboxes and radiobuttons
Psycho replied to technotool's topic in Javascript Help
<html> <head> <script language="Javascript" type="text/javascript"> function populateTextArea(){ var output = new Array(); var fieldID = 1; while (fieldObj = document.form_pe['pe_gen'+fieldID]) { if (fieldObj.length) { for (var j=0; j<fieldObj.length; j++) { if (fieldObj[j].checked) { output[output.length] = fieldObj[j].value; } } } else { if (fieldObj.checked) { output[output.length] = fieldObj.value; } } fieldID++; } document.getElementById('targetarea').value = output.join(', '); } </script> </head> <body> <textarea id="targetarea" cols="100" rows="5"></textarea> <br><b>Physical Exam:</b><br> <form name="form_pe" method="" action=""> <a href="#" onClick="populateTextArea();">Populate</a> General<br> <form name="pe_exam"> <input type="checkbox" name="pe_gen1" id="pe_item2" value="alert and oriented x 3">A & O x3 <br> <input type="radio" name="pe_gen2" id="pe_item4" value="No apparent distress">NAD <input type="radio" name="pe_gen2" id="pe_item6" value="Obvious distress">Obvious distress<br> <input type="radio" name="pe_gen3" id="pe_item8" value="normal respiation">Normal Respiration <input type="radio" name="pe_gen3" id="pe_item10" value="Labored Respiration">Labored Respiration <br> <input type="radio" name="pe_gen4" id="pe_item12" value="normal mood">Normal mood <input type="radio" name="pe_gen4" id="pe_item14" value="Depressed Mood">Depressed Mood<br> </form> </body> </html> -
1. This is not a JavaScript questin it is a CSS question. 2. You do not need to specify properties in the "a:hover" option if they are the same in the standard "a" class. You just need to use named classes to achieve this Example: <html> <head> <style> a { font-family: Arial, Helvetica, sans-serif; font-size: 14px; text-decoration: none; font-weight: bold } a:hover { text-decoration: underline; } .red { color: #990000; } .blue { color: #000099; } </style> </head> <body> <a href="#" class="red">Link 1</a><br> <a href="#" class="blue">Link 2</a><br> </body> </html>
-
Well, there is a lot of unneccessary code. For example: foreach($item_val as $value){ $i++; } You could get the same thing by $i = count($item_val). But even that is unnecessary. <?php //Remove the first index w/ the value of 1 (if it exists) if (array_search(1, $item_val)!==false) { unset($item_val[array_search(1, $item_val)]); } //Combine remaining array elements into a string $write_val = implode('', $item_val); ?> The IF statment may not even be necessary, but I didn't take the time to test it.
-
make the checkboxes an array and make the values the file names. Then when the page is submitted iterrate through the array and delete the files in the array. Sample code Form: file1.jpg <input type="checkbox" name="fileDelete[]" value="file1.jpg"><br> file2.jpg <input type="checkbox" name="fileDelete[]" value="file2.jpg"><br> file3.jpg <input type="checkbox" name="fileDelete[]" value="file3.jpg"><br> Processing Page: <?php if (count($_POST['fileDelete'])) { foreach ($_POST['fileDelete'] as $file) { //Delete the file } } ?> Of course you are going to want to implement some good validation else someone might maliciously delete any files on the server by submitting custom forms.
-
This was the problem: <?php $query = "SELECT * FROM benchracers WHERE race_id = '$race_id'"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $rid = $row['racer_ID']; $query = "SELECT placed FROM race_Racer WHERE race_id = '$race_id' AND racer_id = '$rid'"; $result = mysql_query($query) or die(mysql_error()); } ?> You should NEVER do looping queries like that. It is extremely taxing on the server. What really doesn't make sense is you didn't do anything with the results. In any event, you can do the same thing with a single query: SELECT * FROM benchracers br JOIN race_Racer rr ON br.race_id = rr.race_id AND br.racer_id = rr.racer_id WHERE br.race_id = '$race_id'
-
[SOLVED] Using $_POST[''] Variable in a SQL Query
Psycho replied to jjacquay712's topic in PHP Coding Help
Better yet, create your query as a string so you can echo it to the page when there is an error: <?php $query = "CREATE TABLE " . $_POST['name'] . " (" . $_POST['email'] . " varchar)"; mysql_query($query) or die("Could not Create Table<br>Query: $query<br>Error: ".mysql_error()); ?>