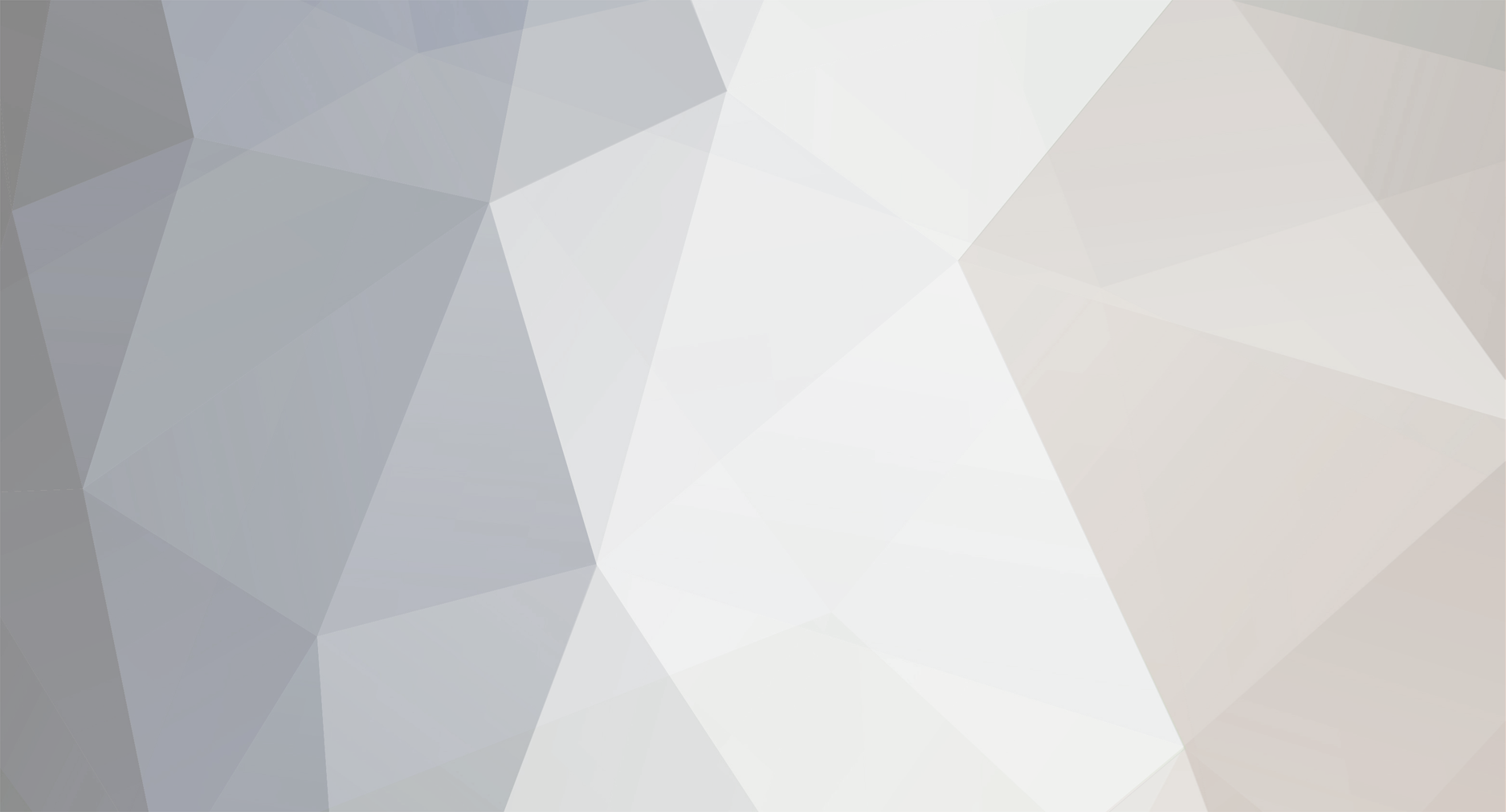
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Unless I am misunderstanding what you are trying to accomplish, you do not need the SELECT or INSERT queries at all! It appears to me that your code is simply trying to update the number of times a record is viewed. If the record does not exist why do you need to add one? You just need to validate that the value passed is a integer and run a single query. If the int doesn't exist in the database the statement will do nothing <?php $id=$_GET['id']; if ($id == (int)$id) { $sql = "UPDATE interviews SET views=views+1 WHERE id='$id'"; mysql_query($sql); } ?>/code]
-
problem with onClick event in drop down menu using safari
Psycho replied to bluehope's topic in Javascript Help
We don't have a crystal ball. Post some code and explain (in detail) what the problem is. -
Validate, validate, validate! Never assume that data coming from the user is valid or safe. It doesn't matter if it is POST or GET data. If '14' is not a valid value for the ID then don't process it.
-
Well, first off using 'yes' and 'no' is terrible. You should be using 0 (false) and 1 (true). Anyway, it can be done much simpler. This should be able to be done with a single query, but I couldn't get it to work so, I made it into two; <?php //Get the IDs of the minimums for each sku $query = "SELECT dataID, MIN(dataP) FROM admin GROUP BY sku"; $result = mysql_query($query) or die ("Query: $query<br>".mysql_error()); //Create array of the min IDs while($min_record = mysql_fetch_array($result)) { $mins[] = $min_record[dataID]; } //Update instock value for all records that are not the mins $query = "UPDATE admin SET inStock='no' WHERE dataID NOT IN (" . implode(',', $mins) . ")"; $result = mysql_query($query) or die ("Query: $query<br>".mysql_error()); ?>
-
.text is the option Label and .value is the option value. Use whichever it is you are trying to accomplish. I tested that code before I posted, so I know it works. As long as the $id value is inthe array you are searching (text or value) it will work.
-
This line already takes care of that if(count($_POST['delete'])) { This check goes a step further than just isset because it also validates that the value is an array.
-
It works for me. I have included a slightly modified version of what you had with my code and it works fine. There must be a syntax error somewhere. Or, I uspect, your checkboxes don't have the same array name like I specified. That would explain the implode error. Because I changed the checkboxes to be returned as an array I used if(count($_POST['delete'])) to determine if any checkboxes had been checked. You could probably also just use if($_POST['delete']). Because the checked values are returned as an array I used the implode function to combine the id's comma separated. You can use the "IN" statement to find any records matchng one of the values. The resulting query should look something like this: DELETE FROM news WHERE id IN (2,3,4) My test script: <?php if(count($_POST['delete'])) { $dels = "DELETE FROM news WHERE id IN (" . implode(',', $_POST['delete']) . ")"; echo "Query: $dels<br>"; //$delres = mysql_query($dels) OR die(mysql_error()); } ?> <form action="" method="post"> <?php //while($edit = mysql_fetch_array($nres)) { for ($i=1; $i<6; $i++) { //$del = $edit['id']; $del = $i; echo "<div id='edit'>"; echo $del . ". "; echo "<input type='checkbox' id='$del' name='delete[]' value='$del' style='border: 0' />"; echo "[user$i] "; echo "<a href='index.php?page=Account&do=editnews&id=$del'>"; echo "Subject$i</a>"; echo "</div>"; } ?> <button type="submit">Submit</button> </form>
-
In my opinion, this should be handled server-side when generating the select list. If you really want to do it with JS, then either add the option index to the passed values so you canuse selectedIndex. Otherwise you need ot iterrate through every option to find the one that matches the value then set the selectedIndex that way. <script language="javascript" type="text/javascript"> function load() { selObj = document.getElementById('lstGroup'); for (i=0; i<selObj.options.length; i++) { if(selObj.options.text=='<?php echo $id; ?>') { selObj.selectedIndex = i; break; } } } </script>
-
Only checked values are passes from the form. A better method is to create the checkboxes as an array. Then you just need to loop througt that one array element to find the selected checkboxes. Rough example: <?php if(count($_POST['delete'])) { //Create one query to delete all the selected values $query = "DELETE FROM table WHERE id IN (" . implode(',', $_POST['delete']) . ")"; $result = mysql_query($query) or die (mysql_error()); } while($edit = mysql_fetch_array($nres)) { $del = $edit['id']; echo "<div id='edit'>"; echo $del . ". "; echo "<input type='checkbox' id='$del' name='delete[]' value='$del' />"; echo "[" . $edit['user'] . "] "; echo "<a href='index.php?page=Account&do=editnews&id=$del'>"; echo $edit['subject'] . "</a>"; echo "</div>"; } ?>
-
Don't use short tags <ul id="crossfadehomepage"> <?php $img_indexes = range(1,11); //Create an array from 1 to 11 shuffle($img_indexes); //Shuffle (randomize) the array foreach($img_indexes as $index) { print '<li><img src="/crossfade/header/photo'.$index.'.jpg" /></li>'; } ?> </ul>
-
Hopefully simple problem that needs solving
Psycho replied to thesecraftonlymultiply's topic in PHP Coding Help
You are not providing the email for the sprintf() $insertSQL = sprintf("INSERT INTO email (email) VALUES (%s)"; Try this: $insertSQL = sprintf("INSERT INTO email (email) VALUES ('%s')", $email); -
I forgot the double quote after that slash before the style attribute. All you need to do is LOOK at the HTML in the generated page and see where the error is. Use whatever debugging info you have (Firebug, IE, etc) and figure out what wasn't properly formatted and fix it.
-
Well, it's a JavaScript error because the PHP is not formatted correctly (By the way it is Javascript not Java). The problem is onthis line echo "<a href=\"javascript:newWindow('sendemail.php?'+document.location.href,'email',400,300,'') 'style=display: block;'>"; Try this: <?php echo "<td valign=\"center\">"; echo "<div style='width:100%; height:100%; vertical-align: center;'>"; echo "<a href=\"javascript:newWindow('sendemail.php?'+document.location.href,'email',400,300,'')\; style=\"display: block;\">"; echo "<span>"; echo "<img src=\"".$mosConfig_live_site."/components/com_marketplace/images/system/writead.gif\" border=\"0\" align=\"top\" >"; echo "</span>"; echo " "; echo "<span>" . JOO_TELL_FRIEND . "</span>"; echo "</a>"; echo "</div>"; echo "</td>"; ?>
-
Use setTimeout() or setInterval(). window.onload = doSomething; function doSomething ( ) { // (do something here) setTimeout ( "doSomething()", 5000 ); //Repeats every 5 seconds }
-
What, does Google not work for the OP? I can understand that he may not know where to get started, but once he knows the name of the process to use a simple google search will give him a wealth of information.
-
No need to "count" the number of values in the array. array_rand() will return the index of a random value in an array. <?php $modarray = array ( 0 => 'value1', 1 => 'value2', 2 => 'value3', 3 => 'value4' ); for($i = 0; $i < $repeatlength; $i++) { $randommod = $modarray[array_rand($modarray)]; fwrite($fh, $variable1 . "," . $variable2 . "," . $variable3 . "," . $randommod . "," . $variable4 . "," . $variable5 . "\r\n"); } ?>
-
curl http://us2.php.net/curl
-
Nevertheless, I still get errors on different lines than you state above. I get the following errors: Line 33: ajaxDisplay.innerHTML = ajaxRequest.responseText; Line 48: ajaxRequest.send(null); So, I have to assume that the errors you are getting are different because of the external code. That's not to say there is a problem with the external code, just that it is difficult to properly debug without it.
-
After I fixed the problems I already described I did not get any errors on the lines you showed above. I then rec'd an error on the last line of the javascript (i.e. the null). Since I do not have access to the external script being called I can't determine if the error is in this code or what is being returned from the external code.
-
This site has [ code ] tags for the specific purpose of being able to properly "display" code as opposed to having it interpreted. Also, bold tags don't work in code tags.
-
The code would be much easier to debug if it was propery indented to follwo the structure. I did go through it and found several problems which solved some of the problems. 1. The opening and closing script tags are wrong. The opening script tag uses "sc" and the closing uses just "s" 2. There is BBCode bold tags within the script ( & ).
-
Not to be rude, but your use of double negatives makes your statements difficult to follow. This statement makes absolutely no sense. There are seven different choices in that select statement. So, there will always be choices besides "other" that are not selected. If English is not your native tongue please take this as constructive criticism. If not, then take it however you wish. In any case, your last sentence seems to be the most informative as to what you are trying to accomplis - namely that you do not want the user to be able to select the option with the label of "select". Well, one of the problems with your initial code was that none of the options (except the last one) had a value. So, the select list would be pretty useless. The code I provided corrected that error and it ensured: 1. If the user slected "other" that they have entered a value in the "other" field. 2. If the user selected anything except "other" there would be no value in the "other" field 3. The user cannot select the "select" option. So, based upon what you stated I believe the code I provided meets all of your needs. Did you try it? If so, you didn't state if it did or did not meet your needs.
-
In my opinion the best way to handle this is to use the same page for the form and for validation. That way if a piece of validation fails you can show the form with the input the user already submitted. Here's an incomplete script that shuld show the process: <?php if (isset($_POST['submit']) { $errors = array(); //validate form if ($_POST['name']=='') { $errors[] = 'You must enter a name.'; } if ($_POST['phone']=='') { $errors[] = 'You must enter a phone number.'; } //If no errors, insert data if (count($errors)==0) { //Isert data to db and redirect to next page header("Location: confirmation_page.php"); exit(); } } //Show the form ?> <?php if (count($errors)>0) { echo "The following errors occured:<br>"; echo "<ul>"; echo '<li>' . explode('</li><li>', $errors) . '</li>'; echo "</ul>"; } ?> Name: <input name="name" value="<?php echo $_POST['name'] ; ?>"> Phone: <input name="phone" value="<?php echo $_POST['phone'] ; ?>">
-
Not quite sure what you are asking since your script already seems to check if the select value is other. But, here is some additional functionality that I would incorporate int othe page: <html> <head> <style> .readOnly { background-color:#cecece; } .txtInput { background-color:#ffffff; } </style> <script type="text/javascript"> <!-- function validate_form() { var error = false; var selObj = document.forms['sample'].elements['where']; var selValue = selObj.options[selObj.selectedIndex].value; var otherObj = document.getElementById('other'); //alert(selValue+":"+otherVal); if (!selValue) { error = "You must select an option."; } else if (selValue=='other' && otherObj.value=="") { error = "If other, Please specify."; otherObj.focus(); } if (error!=false) { alert(error); return false; } return true; } function setOther(selObj) { otherObj = document.getElementById('other'); if (selObj.options[selObj.selectedIndex].value=='other') { otherObj.readOnly = false; otherObj.className = 'txtInput'; otherObj.focus(); } else { otherObj.readOnly = true; otherObj.className = 'readOnly'; otherObj.value = ''; } return; } //--> </script> </head> <body> <form name="sample" method="post" onsubmit="return validate_form();"> <table> <tr> <td><div align="right">How did you find our website? </div></td> <td><select name="where" onchange="setOther(this);"> <option selected="selected" value="">< - - Select - - ></option> <option value="Camping In Ontario website">Camping In Ontario website</option> <option value="Google search listings">Google search listings</option> <option value="Yahoo search listings">Yahoo search listings</option> <option value="MSN search listings">MSN search listings</option> <option value="Camping in Ontario Directory">Camping in Ontario Directory</option> <option value="other">Other</option> </select></td> </tr> <tr> <td align="right"><label for ="other"> If Other, Please specify </label></td> <td><input name="other" id="other" type="text" maxlength="20" class="readOnly" readonly="readonly" /></td> </tr> <tr> <td colspan="2" align="center"> <input name="submit" type="submit" value="Send" /> <input name="reset" type="reset" /> </td> </tr> </table> </form> </body>
-
The only solutino I can think of would be JavaScript by using the onload() trigger. Either "cover" the page with a layer that covers everythign or make the styles such that things are not visible. Then, one the page loads the onload trigger can change the style properties or remove the coverign div.