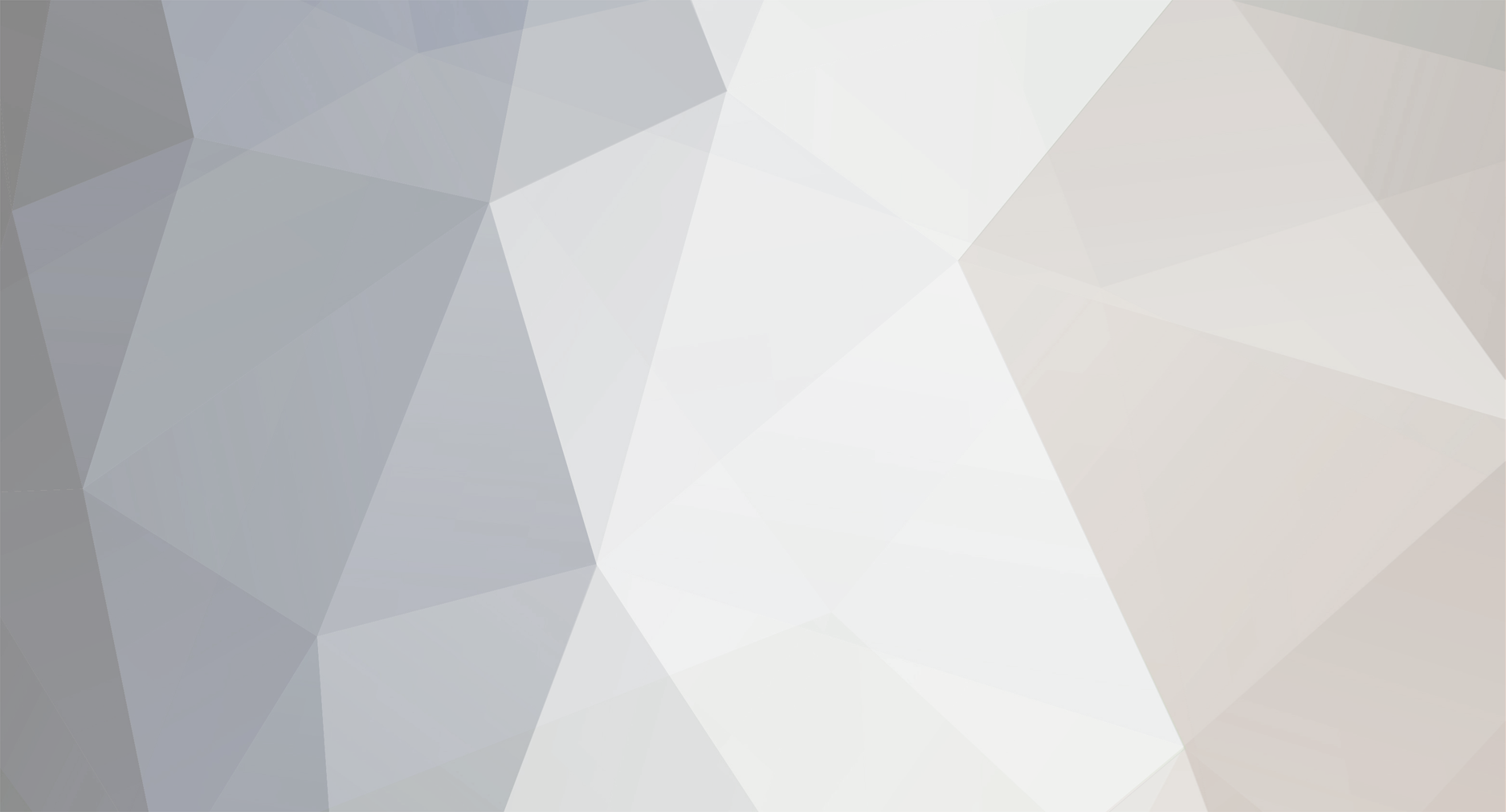
Psycho
Moderators-
Posts
12,160 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
How much usage are you expecting this page to get? Are you expecting a lot of users to be accessing the frame many times a day or will this page get limited usage? If you are just using this for personal use and it will not get a lot of usage, I wouldn't bother with creating a scheduled process at all. Instead, I would create a process when access the page to check if there are any newly uploaded images and, if so, get the most recent image and rename it. So, let's say this is the page that you would display in the iframe: <html> <body> <img src="webcam.jpg" /> </body> </html> Just put some PHP code at the top to detect if there were any new uploads. If so, find the most recent and rename to the name/location you want and then delete the others. <?php //Define locations for uploads and final file $path_to_uploads = "uploads/"; $final_image = "images/webcam.jpg"; //Path to save the most recent //Get the files in the upload directory $uploaded_files = glob("{$path_to_uploads}*"); //Create var to detect recent files $recent_date = 0; //Iterate through all uploads foreach($uploaded_files as $file) { $file_date = filectime($file); //If file is newer, rename it else delete it if($recent_date < $file_date) { rename($recent_file, $final_image); $recent_date = $file_date; } else { unlink($file); } } ?> <html> <body> <img src="<?php echo $final_image; ?>" /> </body> </html>
-
Your query is failing. And since you decided to group all the functions together you have no way to check for errors - not that you have added any error handling at all. But,the bigger issue is that you should NOT be running queries in loops - especially for what you have. You only need ONE query with a GROUP BY clause. .josh is right about mysql_ functions being deprecated and you should change them, but here is a solution with what you currently have - which only uses a single query <?php $query = "SELECT province, COUNT(province) as total FROM property WHERE country = '{$country}' GROUP BY province ORDER BY province ASC"; $result = mysql_query($query, $link_id); if(!$result) { die("Query: {$query}<br>Error: " . mysql_error()); } while ($row = mysql_fetch_assoc($result)) { //Assign variables $province = $row['province']; $total = $row['total']; $title = "{$province} {$country}"; //Create output echo "<li><a href=\"houses.php?province={$province}&country={$country}\" title='{$title}'>{$province} ({$total})</a></li>\n"; } ?>
-
@jazzman1, I think he wants the most recent stage value, not the minimum stage value. Maybe it is just me, but something about this seems to hint at a bad schema design. For one, I'm not understanding why you have two separate tables. Both seem to be a mirror image of each other except one has a total column and the other has a stage column. Is there a reason you should not have one table that just includes those two columns? At the very least you shouldn't need to duplicate the id & member_id values and should use the primary key from one table as a foreign key in the other. But, I don't know enough about the data to be able to give an intelligent response on that. Second, you apparently want the 'last' or most recent stage value, but you have no accurate way to get that info. The only thing you have to rely on is the numerical value of the ID column. The value of the ID field should not be used for that type of purpose. You should instead have a column for date_added or something similar to use for that purpose. You could even create the field to be auto-populated when the record is created and/or updated. Anyway, with the current structure you have (which may be the real issue), here is my solution SELECT sp.member_id, sp.total, st.stage FROM ( SELECT MAX(id) as id, member_id, SUM(total) as total FROM student_points sp WHERE class = 'math' GROUP BY member_id ) as sp JOIN student_times as st USING(id)
-
Validating max number of characters in input field
Psycho replied to eldan88's topic in PHP Coding Help
Because I always start a class with a construct method - even if it is empty. I don't know if I was going to need it or not. In fact, I think I may have had some code in it while I was throwing that example together and may have ended up removing it. It's just one of the practices I follow when coding. -
You're mixing up properties. echo '<p style="color:red; font-family:verdana; font-size:20px;"> Heading </p>';
-
Secure way of displaying PDF's that is HTML5 friendly.
Psycho replied to drewdan's topic in PHP Coding Help
Can't be done. Anything the user is viewing in their browser has been downloaded to their machine/device. You can put a lot if time and effort into obfuscating and disabling features (like right-click menu) but at the end of the day the content is sitting their in the user's cache. And, for the user that doesn't know where the cache is they can simply take a screenshot of any content they want to share. The only thing that makes sense is to prevent users from passing off content as their own through watermarking. But, if this is a PDF and the "text" is the content you want to protect, well that's pointless. Sure, you can make it so the user can't easily copy the text off the PDF by how you create it, but there's nothing to prevent someone from having the content re-keyed. -
You can't honestly say you made a real effort to find the solution yourself. If you Google any number of things you would have found the answer in about 20 seconds: "php format number" "php show number as currency" "php number trailing zeros", etc.
-
Validating max number of characters in input field
Psycho replied to eldan88's topic in PHP Coding Help
Too much to try and go into. But, I'll give a few details. It assumes every field is required. That may be the case today, what do you do later when you want to add a non-required field? Well, you would have to hard-code something into the class to specifically skip the require check for the particular field and that completely ruins the portability of the class. Here is a VERY quick and dirty example (not tested). I'm sure this could be greatly improved upon, but should show what I am talking about class validator { $errors = array(); $currentValue = false; public function __contruct() { } public function validateField($fieldName, $fieldValue, $validations) { $this->currentValue = $fieldValue; //Verify all validation needs for the field foreach($validations as $validate => $args) { switch($validate) { case 'require': if(!$this->required()) { $this->errors[] = "The field {$fieldName} is required."; return false; //No need to do any other validations } break; case 'length': if(!$this->length($args)) { $this->errors[] = "The field {$fieldName} must be between {$args['min']} and {$args['max']}."; return false; //No need to do any other validations } break; case 'email': if(!$this->email()) { $this->errors[] = "The field {$fieldName} contain a valid email."; return false; //No need to do any other validations } break; } } //Return true or false based upon whether there were errors return (count($error) == 0); } public function getErrors() { return $this->errors; } private function required() { return (strlen($currentValue) > 0); } private function length($args) { $valueLen = strlen($currentValue); return ($valueLen >= $args['min'] && $valueLen <= $args['max']); } private function required() { //Add code to validate value is in proper email format } } //Usage $validator = new validator(); $validator->validateField('Name', $_POST['name'], array('required'=>null, 'length'=>array('min'=>5,'max'=>20)); $validator->validateField('Email', $_POST['email'], array('required'=>null, 'email'=>null); $validationErrors = $validator->getErrors(); if(count($validationErrors)) { //display errors } else { //Continue } -
Hmm, going back to the link you posted, did you read through the article and write out the code to ensure you understood every line of code or did you just copy/paste the code? I think that is why you are getting negative reactions. It appears you are taking working code and then trying to add additional logic without really understanding the current logic. You are trying to run before you know how to walk. That is why my earlier reply was so terse. The question you asked would be extremely basic for someone who had already created code to complete a registration process. Sort of like someone asking "Hey I just built this house and want to put a bay window into the living room wall". Most people would be "WTF, you built a house and you can't figure out how to put in a window <scratches head>". And, the way you are expressing things shows a great lack of understanding of how all the processes work - as I stated previously about the form not needing to have a member field. I'm not sure I can really help you at this point except to add the following: 1. If users will only ever belong to one group, then add a group field to the user table and set the default value to 'members' - instead of using a separate table. That will solve your immediate need. You don't need a form field or even change the queries for the registration process as the value will be automatically set with the default value when new records are created. You can then create an administrative page to allow you to change the membership of users later.
-
Validating max number of characters in input field
Psycho replied to eldan88's topic in PHP Coding Help
You could, for example, include it in the field name as an array index key like so <inpyt type="text" name="cc[15-16]"> But you absolutely shouldn't. Never trust ANY data coming from the user. Why even validate the input if you are going to pass the logic on how to validate to the user? I don't like the look of that class (maybe there is more I am not seeing). I would create the class to have methods for each type of validation that may be done to a field. Then - in the code - I would call each method for the POST values as appropriate. You could create a method that allows you to run multiple validations on a single field so you don't have to call each individually as well. -
So, after you insert the user record, get the user ID and insert another record into the user_group table as I showed above. Sorry, but I have to ask, did you write that code or did you simply copy/paste that from a tutorial?
-
UNION queries have specific requirements such as each query must return the same number of fields and each field must be the same 'type". You need to make sure to create them to meet that requirement. Yes, you can merge the 'common' data for those four tables. You could leave those four tables exactly as they are, but remove the service_code value. Then use a single the Serv table as I showed above. That way you have one table for all the common data which you can use for JOINing on the ServErog table and can additionally JOIN out to those individual table for the additional info. I can't really know what the best way is to set these up without understanding the entire project (which I don't want to do), but am only saying that what you just stated doesn't preclude you from doing it that way.
-
Why didn't you post this in response to the solution I provided in your other thread? It's poor form to take someones code like that and then simply turn around and ask for help with it. The solution I provided worked perfectly with the (sparse) information you provided. I even included the explicit disclaimer in that post The data you provided was in the same format and there was nothing to show otherwise. So, now we know differently. As I also stated in that thread you are relying on several things that are completely out of your control. Namely, "what" is getting copied/pasted into the input field. Based upon the browser and OS of the user it could be very different for one user to another. So, no matter what solution you come up with, it will likely have flaws (if not now, later). So, having said all that, you can probably get very close to perfection. You state only the Duty and Arr fields will have variable content format, but all the other fields will adhere to a specific format. If that is the case, then I would go another route entirely: RegEx. Which I considered previously but, based on the information you provided, it didn't seem it was needed. I assume that Duty will always have a value though. I am also assuming that Dep will be exactly a three letter value. $output = ''; $lines = explode("\n", $_POST['input']); foreach($lines as $line) { if (preg_match("#([^,]+), \w{3}[\s]+(.+?)[\s]+(\w{3})[\s]+(\d\d:\d\d) Z[\s]+(\d\d:\d\d) Z[\s]+(.*)#", $line, $match)) { $date = date('m/d/Y', strtotime($match[1])); $duty = $match[2]; $dep = $match[3]; $begin = $match[4]; $end = $match[5]; $arr = $match[6]; $output .= "<tr><td>{$date}</td><td>{$duty}</td><td>{$dep}</td><td>{$begin}</td><td>{$end}</td><td>{$arr}</td></tr>\n"; } } echo "<table border='1'>\n"; echo "<tr><th>Date</th><th>Duty</th><th>Dep</th><th>Begin</th><th>End</th><th>Arr</th></tr>\n"; echo $output; echo "</table>"; This includes some additional logic to hopefully capture any differences in copy/paste values based on system. Namely when copy/pasting the table data the values between columns can be separated by one (or more) white-space characters: space, tab, etc.
-
Why do you have separate tables for ServA, ServB, etc.? You include a column in each with is defining the 'type' already. All these records belong in ONE table such as this (let's just call it "Serv"): +------+---------------+------+ | idS | service_code | type | +------+---------------+------+ | 1 | codice blaA | 1 | | 2 | codice eccA | 1 | | 3 | bla blaA | 1 | | 4 | codice blaB | 2 | | 5 | codice eccB | 2 | | 6 | bla blaB | 2 | | 7 | codice blaC | 3 | | 8 | codice eccC | 3 | | 9 | bla blaC | 3 | | 10 | codice blaD | 4 | | 11 | codice eccD | 4 | | 12 | bla blaD | 4 | +------+---------------+------+ Then your original query will get all the associated records in a much simpler way SELECT ServErog.idSE, ServErog.servtype, ServErog.typeid, Serv.idS, Serv.type FROM ServErog LEFT JOIN Serv On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS ORDER BY ServErog.idSE, Serv.type You can determine in the processing which types are included and which ones are not. Then to get all the ones not associated with a record in ServErog you would run something like this SELECT Serv.idS, Serv.service_code, Serv.type FROM Serv LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS WHERE ServErog.idSE IS NULL Or, if you wanted the ones not associated with a specific ServErog record SELECT Serv.idS, Serv.service_code, Serv.type FROM Serv LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS AND ServErog.idSE = $id WHERE ServErog.idSE IS NULL With what you have, to get all the records from four tables in a single result set would take a more complicated UNION type query. Maybe something like: SELECT ServA.idS, ServA.service_code, ServA.type FROM ServA LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS WHERE ServErog.idSE IS NULL UNION SELECT ServA.idS, ServA.service_code, ServA.type FROM ServA LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS WHERE ServErog.idSE IS NULL UNION SELECT ServA.idS, ServA.service_code, ServA.type FROM ServA LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS WHERE ServErog.idSE IS NULL UNION SELECT ServA.idS, ServA.service_code, ServA.type FROM ServA LEFT JOIN ServErog On ServErog.servtype = Serv.type AND ServErog.typeid = Serv.idS WHERE ServErog.idSE IS NULL When you have to create such complicated queries to get what should be something simple, it is an indication that the DB structure is not right. Put all the Serv records into a single table.
-
Yeah, can't use a SELECT for only some of the values. As Ch0cu3r showed, just use a SELECT for all the values of the record and just use hard coded values in the field list for those that you want to specifically set.
-
I don't see how we can help you with the information provided. You've stated you are using a database, but the only code you are showing is HTML - which has nothing to do with the problem as I understand it. You've stated you have a "registration process". I have to assume that this process creates a record in the database associated with the user. You stated the problem as you want that new users to be automatically put in the "user_group database as 'member' by default". You further stated "I'm having to edit the database each time a new user signs up because it's not in-putted via the form". This is a business rule you want to implement. The "form" has nothing to do with this. As I stated previously, you (by 'you' I mean YOU) need to implement some additional logic in the code that creates the user record to also add a record in the user_group table. You've shown zero code related to the user creation/registration process nor given any details on the table structures. Therefore, the help that was provided was generic in nature. But, I am feeling generous so I will provide the following mock example. This assumes the user table has an auto-insert incrementing ID //Run query to insert user record $query = "INSERT INTO users (name, email, birthdate) VALUES ('$name', '$email', '$bdate')"; $result = mysqli_query($link, $query); $user_id = mysqli_insert_id($link); //Get id of last inserted record //Run query to add record to user_group table for new user $query = "INSERT INTO user_group (user_id, group) VALUES ($user_id, 'member')"; $result = mysqli_query($link, $query);
-
Using isset() in this scenario is probably fine, but it can cause problems when using it in some scenarios to see if a specific array key exists. The manual for array_key_exists() even includes a specific statement about the two I actually got tripped up by this before. Although isset() would work in this specific scenario, in the interest of good programming practices, I think array_key_exists() would be appropriate. Building upon Kicken's solution, you can build a function to do this: function updateArray(&$sourceArray, $date, $country, $value) { //Check if date exists in source array if(!array_key_exists($date, $sourceArray)) { //Date not found return false; } //Update value $sourceArray[$date][$country] = $value; return true; } //Usage updateArray($array, '10/3/14', 'US', 100);
-
String Variable Changing value and hidden value not working
Psycho replied to OutOfInk's topic in PHP Coding Help
Hmm, the font tag has officially been deprecated for a full 15 years - since the release of HTML 4.01 in 1999. So, even if you were coding 5 years ago it had been deprecated for at least 10 years before that! But, it's all good, the internet is strewn with tutorials and examples that still use it. Which explains why it we still see it so much here. -
String Variable Changing value and hidden value not working
Psycho replied to OutOfInk's topic in PHP Coding Help
OK, looking at your original code it is impossible to tell if you are running any of the same queries. But, if you are duplicating any of those queries you should not write them twice in the code - instead structure your logic so you only need to run them once. Below is some "mock" code based upon what you posted with some assumptions that the first queries were duplicated. I also made the assumption that $checkBids is a Boolean value (true/false). Some other things: Don't use the FONT tag it has been deprecated for over a decade! And, don't put presentation logic in the code. Again, this was based on a lot of assumptions <?php if (!$checkBids) { //Run processes for when $checkBids is false mysql_query("UPDATE"); $responseClass = 'redclass'; if ($arrayTeam == 'Draw') { mysql_query("UPDATE"); $response = "You have updated your bid that <b>$fdraw[0]</b> and <b>$fdraw[1]</b> will draw round <b>$arrayRound</b>, placing <b>$arrayBet</b> PPC as your bet!"; } else { mysql_query("UPDATE"); $response = "You have updated your bid for <b>$arrayTeam</b> to win on round <b>$arrayRound</b>, placing <b>$arrayBet</b> PPC!"; } } else { //Run processes for when $checkBids is true mysql_query("UPDATE"); $responseClass = 'greenclass'; if($arrayTeam == 'Draw') { mysql_query("INSERT INTO"); $response = "You placed a bet that <b>$fdraw[0]</b> and <b>$fdraw[1]</b> will draw round <b>$arrayRound</b>, placing <b>$arrayBet</b> PPC as your bet!"; } else { mysql_query("INSERT INTO"); $response = "You placed a bet on <b>$arrayTeam</b> to win for round <b>$arrayRound</b>, placing <b>$arrayBet</b> PPC as your bet!"; } } ?> <table cellpadding='2' cellspacing='0' border='0' class='innnertable' width='620'> <tr class='innertable'> <td align='center' class="<?php echo $responseClass; ?>"><?php echo $response; ?></td> </tr> </table> -
String Variable Changing value and hidden value not working
Psycho replied to OutOfInk's topic in PHP Coding Help
You don't show enough in the hacked code to really show a good example, but give me a while to put something together. -
You have a process around creating the user. That process may entail a confirmation process or something as well. Just determine at what point in that process that you want to add them to the group and, well, add them by running a query. You could do it right after creating the user int he database if you want. This is not something you would handle through the form/HTML.
-
I have no clue, since you were supplied a solution for what you asked. You keep changing the problem, just look at the sample data you posted in your first post, third post and fifth post. In this last go around you state you expect the TACS result for the records with a TOE of a to be '9' when you do a GROUP BY. Well, your last example data had: TICS TACS TOE --------------------------------------- 6 9 a 4 9 a So, both records have a 9. So, there is no way for us to have any clue as to what logic to provide you. What if they were different? Do you want the highest value, the lowest, an average, or what? If is very frustrating to provide free help to people to provide a solution only to have them change the requirements. So, have some consideration and clearly explain what it is you are trying to achieve. If this TIC TAC TOE nonsense is just that, then explain what you are really doing as it may add some context to help us understand better.
-
String Variable Changing value and hidden value not working
Psycho replied to OutOfInk's topic in PHP Coding Help
Because you are giving the hidden fields the same name. So, if you replicate the hidden fields and they are all submitted with the form, then the value of the last one is what will get passed. But, to tell you the truth, the format of your code is atrocious. You need to take a more structured approach to your code. All those ifelse() statements should be simplified and you should only include logic in those conditions - then create the output later. -
String Variable Changing value and hidden value not working
Psycho replied to OutOfInk's topic in PHP Coding Help
Your problem is on the very first line if (($checkBids > 0) && ($arrayTeam = 'Draw')){ . . . or more precisely $arrayTeam = 'Draw' That is not comparing the variable $arrayTeam tot he string 'Draw', it is assigning the string 'Draw' to the variable $arrayTeam To compare you use two equal signs if (($checkBids > 0) && ($arrayTeam == 'Draw')){ -
Well, let's step back a second. By copying and pasting the table you are talking about the output of the table and not the source code, right? Since the output is being copied onto the user's clipboard, I can't guarantee that the "copied" output would always be exactly the same based upon browser or, especially, operating system. A MAC may copy the content much differently than a PC. However, based upon the example you have posted, the output was simply adding a space between each cell. So, the problem here is you need to differentiate between spaces that exist within the data field and the spaces that delineate the different pieces of data. If the data will always be in the same format then you can do it be creating the rules on processing the data. Based on what I see, every line will have the same number of spaces (i.e. there are no fields where some values have more or less spaces than other values in the same column). So, the easiest solution, IMO, is to simply explode the lines and then use the parts you want. Here is some sample code with absolutely no verification that this will work for all possibilities $lines = explode("\n", $_POST['input']); foreach($lines as $line) { if(substr($line, 0, 4)=='Date') { //Skip header line continue; } $parts = explode(' ', $line); $date = $parts[1] . ' ' . $parts[0] . ', 20' . trim($parts[2], ','); $duty = $parts[4]; $dep = $parts[5]; $begin = $parts[6]; $end = $parts[8]; $arr = $parts[10]; echo "Date: {$date}<br>\n"; echo "Duty: {$duty}<br>\n"; echo "Dep: {$dep}<br>\n"; echo "Begin: {$begin}<br>\n"; echo "End: {$end}<br>\n"; echo "Arr: {$arr}<br><br>\n"; } Part of the sample output Date: Apr 1, 2014 Duty: 7743 Dep: HME Begin: 04:40 End: 06:20 Arr: AWY Date: Apr 1, 2014 Duty: 6473 Dep: AWY Begin: 06:45 End: 08:55 Arr: FRG Date: Apr 1, 2014 Duty: 1231 Dep: FRG Begin: 09:20 End: 11:25 Arr: AWY . . .
- 2 replies
-
- php
- html table
-
(and 1 more)
Tagged with: