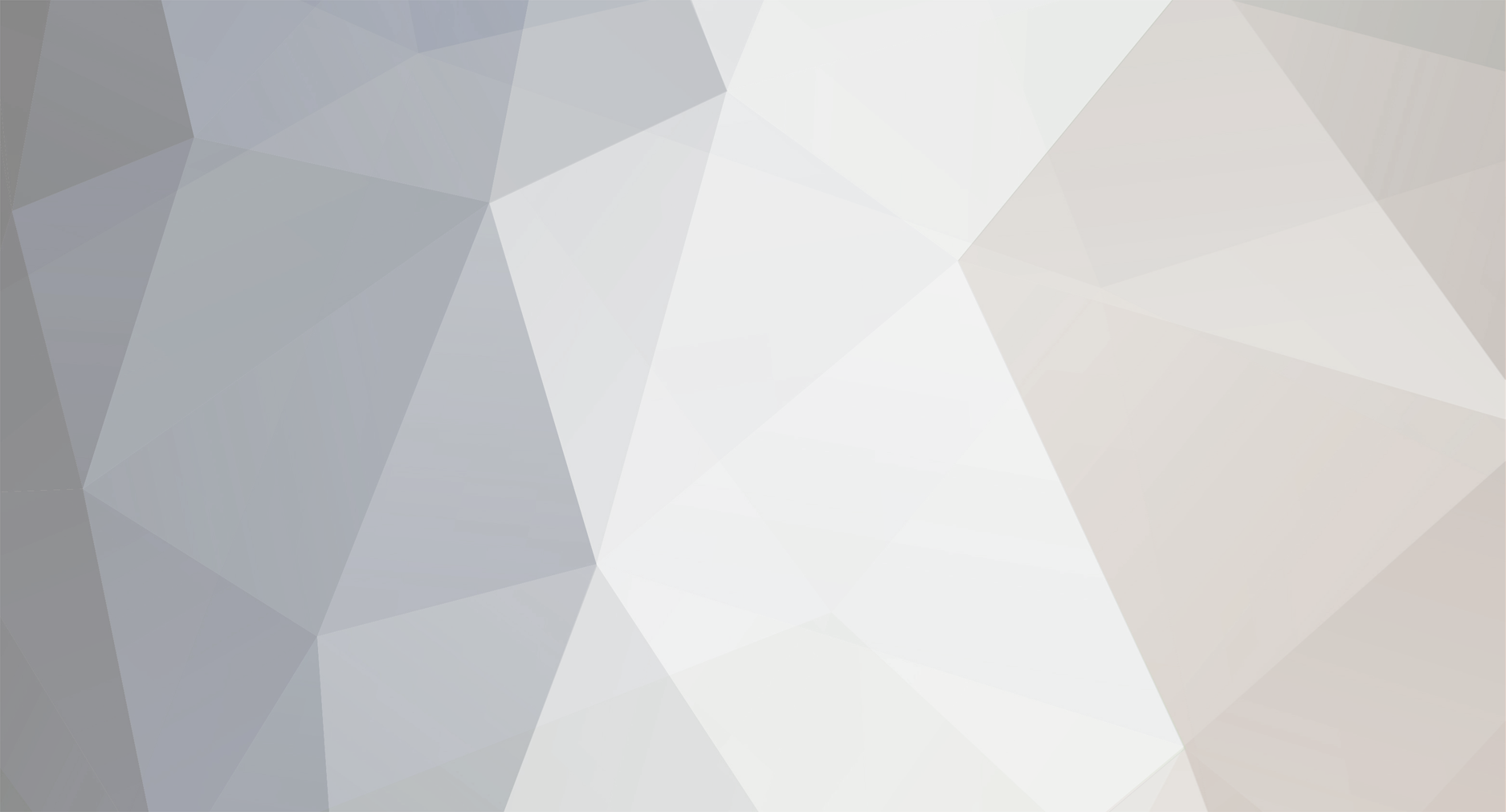
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
The array should look like this: Array ( [1] => 0 [2] => 1 ) Where the 1 & 2 as indexes are the item IDs and the 0 & 1 are the quantities. If you set up your array correctly the code to do what you want could look like this [Note: no need to iterate over every item in the array!]: //remove one quantity from item if (isset ($_GET["remove"])) { //Set the item ID to reduce $itemIdToRemove = intval($_GET["remove"]); if(isset($_SESSION["viks_cart_array"][$itemIdToRemove])) { //Reduce quantity by 1 $_SESSION["viks_cart_array"][$itemIdToRemove]--; //Remove ANY items from cart where quantity is 0 $_SESSION["viks_cart_array"] = array_filter($_SESSION["viks_cart_array"]); } header("location:{$_SERVER['PHP_SELF']}"); exit(); }
-
Well, I'm confused as to what you want since your code doesn't seem to have anything to do with the request. It would be helpful if you stated what is contained in the various variable. The array value $_SESSION["viks_cart_array"][$index] appears to be a sub-array with at least indexes of 'item_id' and 'quantity'. That a poor way of storing that information. It should be in this format: $_SESSION["viks_cart_array"][$item_id] = $quantity Then this becomes a VERY simple process. Creating the item_id and quantity as separate sub-keys is problematic. EDIT: Also the code is inefficient. There is a loop of the array that uses $index & $value - but then those aren't used within the loop as they should be and instead the full array path is listed.
-
I would interpret the "type" of shake as one that has multiple ingredients. So, a pinacolada would have coconut and pineapple. If that is the case, the tables might look more like below. This assumes that each product will have the same sizes available products id | whatever | ---+--------------------- 1 | shake 1 | 2 | shake 2 | ingredients (a list of all possible ingredinets) id | ingredient ---+------------ 1 | banana 2 | chocolate 3 | strawberry 4 | mango 5 | vanilla product_ingredients (which ingredients go with which shake) id | prod_id | ingredient_id ---------------------------- 1 1 1 2 1 2 3 2 3 4 2 4 5 2 5 product_prices (the available sizes for each product and the price) id | prod_id | size | price ---+---------+------+------ 1 | 1 | 500 | 26,50 2 | 2 | 500 | 26,50 3 | 1 | 750 | 28,90 4 | 2 | 750 | 28,90
-
The code you originally posted should have created errors because, as I stated, you had HTML code within the outer PHP tags. That tells me that your page is not being processed by the PHP interpreter. There are a few reasons why this is occurring: 1) Is the page named with a php extension (e.g. mypage.php)? If it is HTML the PHP code will not be processed - unless the web server is specifically set up to do that. 2) Are you opening the page directly through your browser or are you opening it by requesting the page through a web server? The address bar should start with "http://" and not something like "C:\". 3) If still not working when verifying the two items above, it could be that the web server is not set up to support PHP or is mis-configured.
-
Help with if / else with a MySQL bit field?
Psycho replied to dazedconfused's topic in PHP Coding Help
As I stated in my last response, this is not a "bit" field. You really want a boolean - but there is no true boolean in MySQL (that I am aware of). You would instead want to use a Tiny INT field and store a 1 (true) or 0 (false). A bit field is one used to store a bit-wise piece of data - i.e. base 2 notation such as 11010011 -
Help with if / else with a MySQL bit field?
Psycho replied to dazedconfused's topic in PHP Coding Help
FYI: The term you wanted to use for a True/False value is a BOOLEAN - not a "bit field". Also, if you have a condition to check if a value is True/False you don't need to have the comparison operator. You can just do something such as this: if ($row_rsPRODFULL['LEDdimmable']) { //Do this if value is true } else { //Do this is value is false } Also a couple other notes. PHP is a weak type language (as opposed to a strict type language). One of the properties of that is that when comparing values they don't have to be the same "type". For example, the boolean TRUE and the number 1 are both interpreted as true. So, if(TRUE == 1) would result in a true result. This is important because you can't store an actual boolean in the database. You would store a 1 or 0. But, you need to keep this in mind, because sometimes you want to compare to value in a strict sense. For these you would use the string comparison operators. E.g. if(TRUE === 1) - note the THREE equal signs. That comparison would result in a false result. -
Your code is invalid. You start by opening a PHP code block (i.e. <?php) Then you put in HTML code! Then, you have other opening PHP code blocks. You can only have PHP code within PHP code blocks and "actual" HTML must exist outside the PHP code blocks. Of course you can echo HTML within PHP code blocks - but that is still PHP. Also, it is a better process to execute all of your PHP logic before you even start the HTML output. Just create variables with the variable content in the PHP logic and output within the HTML Also, looking at your logic for that field value - it is completely unnecessary! This is what you are doing: if($DateEntered != "") {echo $DateEntered;} So, if the value is not empty echo it - else do nothing. So, why not just echo it unconditionally - the result would be the same. If $DateEntered is empty then it will output an empty string. Edit: <?php //Put all PHP 'logic' at the top of the page - or even in another file if($_SERVER['REQUEST_METHOD'] == 'GET') { $DateEntered = ""; } $txtDateEnteredValue = (isset($DateEntered)) ? $DateEntered : ''; ?> <html> <head> </head> <body> <form action="" method="post"> <table> <tr> <td><b>Date:</b></td> <td align="middle"><input type="text" id="txtDateEntered" name="txtDateEntered" value="<?php echo $txtDateEnteredValue; ?>" /></td> </tr> </table> </form> </html>
-
How do I print a list of transaction with some of them in sub categories
Psycho replied to MockY's topic in PHP Coding Help
Right - and the core problem is that the transaction table is not sufficient to track itemized details. A transaction records should store a single transaction. But, you are wanting to store different details about parts of the transaction. One possible solution without modifying that table would be to create a new table to ONLY records itemized data when needed. This will probably be the same amount of work as restructuring in the proper way and modifying your existing logic. Plus, doing it this way would result in more complicated logic - creating maintainability problems. But, since you asked: Create a new table similar to before for itemized data. But, only use it when the entire transaction does not apply to a single category. This will require you to ensure the 'total' in the transaction table equals the sum of parts in the items table - something you should never have to do. This tale will need an ID column, a foreign key to the transactions table, as well as Category, SubCategory and amount fields. Once you do this, you will need to develop the ability to itemize a transaction (i.e. add/edit/delete). Then almost any functionality that relates with transactions would need to be updated as well. Anything that displays transactions, individually or a list, will likely need to display information about the itemization. Plus, you would have to update any delete functions to prevent orphaned records. The more I think about it, I feel even stronger that the work involved to do it without modifying the transaction table will be the same as not modifying that table. But, it is your project to do what you would like. -
How do I print a list of transaction with some of them in sub categories
Psycho replied to MockY's topic in PHP Coding Help
Yep, you will need two tables for your transactions (well, four if you count the categories and the vendors). Here's an example of how the structure would work Table: transactions trans_id | trans_date | vendor_id ---------------------------------- 1 2013-11-18 11 2 2013-11-18 12 3 2013-11-17 13 Table: vendors vendor_id | vendor_name ------------------------- 11 Chipotle 12 Nugget 13 Amazon Table: items item_id | trans_id | item_amount | category_id ----------------------------------------------------------------- 1 1 17.60 16 2 2 22.12 17 3 2 4.54 18 4 3 81.07 19 Table: categories cat_id | cat_name ------------------------- 16 Meals Out 17 Groceries 18 Misc. Food/Drink 19 Gifts You can see that there is one transaction for "nugget" on the 18th. But, the transaction doesn't have any other details. Those are stored in the 'items' table. That transaction for 'nugget' has two separate 'items'. One in the amount of $22.12 with the category groceries and one for $4.54 for Misc. Food/Drink. Now, I made the assumption that you would group like categories into one itemization. But, if you wish, you could list every single item on your receipt in the items table. -
There is actually an easy solution. The first step is to put these files outside of the web directory. For example, if the root of your site is at C:\webroot\mysite\, then put your files in a folder such as C:\webroot\files\. Now, that folder and the files in it cannot be accessed from the web browser directly through a URL. You can then create a page that will serve the files to the user. You can create that page to use whatever parameters you pass to do whatever you need. Once you verify that the user is authorized you will 'send' them the file //Insert code to determine the file to use and whether the user should access it // . . . // . . . //$file - variable assigned value of path to file // . . . header('Content-Type: application/octet-stream'); header('Content-Disposition: attachment; filename="'.basename($file).'"'); header('Content-Length: ' . filesize($file)); readfile($file);
-
OK, let's take a simple example. You obviously know enough to put a page together. So, as you should know, certain characters or groups of characters can have special meaning and will be 'processed'. For example, here is a simple piece of HTML This is <b>bold</b> text I assume you know that the result of that would be "This is bold text". Ok, so let's take some HTML with dynamic content in it: Name: <?php echo $name; ?> That would result in a custom output based upon the value of name. If name is "Psycho" the output would be "Name: Psycho". But, let's say the value of $name is "<b>Psycho</b>". That would still output"Name: Psycho". But, by allowing that 'code' to exist in the user's name they can modify the output of the page. That's pretty benign. But, users could add JavaScript to their username (or other data) leading to vulnerabilities to your site. You can still allow users to add those characters to their name, but you should escape them so the browser doesn't interpret the content as code. In this case you want to escape the data for output in HTML, so you could use htmlspecialchars(). E.g. Name: <?php echo htmlspecialchars($name); ?>. That will convert the carats (i.e. <>) to < & >. The browser will then interpret those as the <> characters and print them to the page rather than interpreting them as code. Just about every way you can use data (HTML, JavaScript, PHP, file writing.reading, etc.) has ways to escape characters so they do not perform some special function that they have. Now, specific to your problem. URLs have many special characters such as ?, &, +, etc. Go to google and do a search for "PHP Freaks". You will see that the URL contains search?q=php+freaks. Since you can't have spaces in a URL, a plus sign is used to concatenate all the values of the query. But, what if you wanted to search for something with a plus sign in it? You will need to escape the value so it will be interpreted as the literal plus symbol and not the special concatenation symbol. In PHP you would do that with urlencode(). When using that, plus symbols are converted to "%2B" - which will be treated as a literal plus symbol character. But, as mac_gyver already stated you should not be passing string names on the URL to do a look-up. Use the ID from the database.
-
"Echoed"? You didn't have any code that was echoing the $policyandregion value. You only showed that it was being used in a query. You obviously didn't run it - because adding the two values is exactly what it should do.
-
Try: case 1: $policyandregion = "Single{$region}"; break; case 2: $policyandregion = "Duo{$region}"; break; case 3: $policyandregion = "(Duo{$region} + Single{$region})"; break;
-
The problem is probably that you are using $item as a parameter within a URL here: echo "<tr><td width = '50%' > <a href = 'update_sales.php?item=$item&qty=$qty&bc=$barcode&price=$price'>$item</a></td> <td width = '5%' align = center>$qty</td> <td align = center>$barcode</td><td align = center>Php $price</td></tr>"; Some characters have special meaning when used within the URL - unless you properly escape them using urlencode(). Always think about where you are using data and how it may need to be escaped. You aren't even escaping the user submitted data before using them in a query! That's just asking for someone to mess with your database with SQL Injection.
-
I've used file_get_contents() many times on pages with javascript and never seen that issue. So, I don't believe that is the problem. Looking at the code provided I see no way that would happen - but anything is possible. Are you absolutely sure that the page you are loading is the same one you are editing? I've done it before where I copy a page/folder and edit the wrong page wondering why I am not seeing any changes when loading the page. I'm thinking you may have had the page initially output the contents to the page to verify you were getting the right results before changing the code to write to a file. Or, perhaps, that is what you did and the page is showing the cache instead of getting new content. Try Ctrl-F5 Of, it you are certain that is not the problem, provide the URL of the page you are hitting so we can see the problem for ourselves.
-
When you get zero results from that query, what ID are you using for $racun? If you run the other query I provided in reply #23 (my god has it been that many) the results will show you exactly how many records that person has in the clanovi_njihovi_parovi table total and for the current day. based upon your logic, the person must have at least three records for the current day - else you get that error message. So, on the day that you ran that query you should get that error message for ALL IDs except for ID 106 - because that one had at least three record for the current day.
-
We are really working in the blind here. If something doesn't work please try to provide relevant information to help us help you. The screenshot you just linked to provides absolutely no useful information. It only tells us that there are records in the clanovi_njihovi_racuni table. It doesn't tell us if there are at least three records in the clanovi_njihovi_parovi table associated with the record in the clanovi_njihovi_racuni table that you are using in your script. Not to be rude, but you do realize that the query will only count posts for the current day, right? Even if the user has had 1,000 posts in the past, the count is only for the posts that they made today. That was your logic, so I had left it in. Run tthis query and show us the results - not the message stating the number of results: SELECT cnr.id, cnr.klikovi, COUNT(cnr.id) AS total_count, SUM(cnp.datum=curdate()) AS today_count FROM clanovi_njihovi_racuni AS cnr LEFT JOIN clanovi_njihovi_parovi AS cnp ON cnr.id = cnp.racun GROUP BY cnr.id Only the records for id's where the result for today_count is 3 or greater will make the condition if($klikovi<3) false.
-
Unrelated, but pro tip. You can convert all those elseif()s into a switch() statement to clean up the code a bit. The case statements within a switch need to equal the value of the switch(). So, it is counter-intuitive to have a comparison for the case statement. But, this does work switch(true) { case ($lvl < 10): //Do something break; case ($lvl == 1 && $xp >= 200): //Do something break; case (lvl == 2 && $xp >= 500): //Do something break; //Etc. }
-
It looks as if there is PHP code getting into the query. The only way that I see that happening is with the variable $racun, which is defined as $_SESSION['liga_user_id']. Change the query execution to this: $result = mysql_query($query) or die("Query: {$query}<br>Error: " . mysql_error()); Then run the page and post the results.
-
OK, here's my take. Assuming you do send the email to the same email address and it is received some times and not others, it doesn't appear to be a problem with email filtering, spam, etc. That's not 100%, but I would start by looking at the code. So, the first thing I would do is add some logging around the process. How do you know that the email was even generated and that there were no errors? Looking at the methods in your FGMembersite class, you are not capturing errors in most of the ones that send emails. Also, the class is not taking advantage of the reason for creating a class. All of the methods that send an email have code to initiate the mailer class. You should instead create a method to initiate the PHP mailer class and call that from within all the methods that send an email. Never write the same code multiple times when you can write it once. Also, at the end of those methods you have logic to send the email. In at least one you record the error (not sure what happens to it). if(!$mailer->Send()) { $this->HandleError("Failed sending user welcome email."); return false; } return true; But in most, you don't record the error at all if(!$mailer->Send()) { return false; } return true; Pro Tip: No need for that if() condition. If the Send() method returns true/false you could have just done this: return $mailer->Send(); But, that's not what you want to do in order to see what is happening. As I stated before you should create your own method in your class to send the emails. That method would call the Send() method. But, you can then add additional error handling within your method. Have the method record (in DB or Log File) every instance of an attempt to send an email and whether it succeeded or not. It would probably make sense to record all the information about the email: from/to addresses, body, etc. You can create switches to determine how much information to log. So when everything it working well just log the bare necessities. When there are problems, set the logging level higher to help debug the problem.
-
When you state . . . the time it did work were you sending it to the same email address as you were when it was not received? There are many reasons why email may not get to its intended recipient. If it always fails to specific domains vs. inconsistent results to specific domains the root cause will be very different.
-
Why do you assume you have enough clicks? When you encounter a problem such as this a simple TEST should tell you the problem. I made a lot of changes, so I'm not guaranteeing that there were no errors. But, this should be very simple to debug. I'm not sure which condition is the check that you think is working incorrectly. I don't speak Bosnian and when I use a translator none of the message refer to 'clicks'. But, for whichever one you think is working incorrectly, just look at the condition check. Then test the values that are used for that condition check. If they don't look right, then go back to where they are defined to determine why they are not correct.
-
Trying to CONCAT two columns in a Select query...
Psycho replied to Jim R's topic in PHP Coding Help
No, it did not. It would have produced much more than that. var_dump() will tell you they type of variable it is and, based on the type, additional details such as number of characters. Because, as I stated earlier, the variable could have non-printable characters included in it. Which is exactly what I think is happening here. So, when you do a simple echo of the variable it doesn't print those characters to the page - such as a NULL value. That is exactly why I suggested you use var_dump() because it will tell you exactly what the variable contains. If there are non-printable characters it can translate those into a human readable form in many cases. So, please post EXACTLY the output from var_dump(). If there is CSS that is modifying it then get the output from the HTML source code. Programming is an EXACT science, we need to know exactly what is happening to fix it. but, regardless of what is in $slug, the value is getting there from the function single_tag_title() which you have not provided any code for. -
There is nothing wrong with the code. The problem has to be with the variable. what is the output of var_dump($js_inc["id"]); ?
-
Trying to CONCAT two columns in a Select query...
Psycho replied to Jim R's topic in PHP Coding Help
No, you were here asking why you were getting the error which was due to a bad query. Then you were asking why two values were not comparing. Now, you are asking why there is the text 'NULL' at the end of a value. This is all the result of sloppy coding. Do one thing and verify it works. Then move on to the next thing. You don't even state which value has the 'NULL' at the end: $slug or $nameFull. If it is $slug, then the value is getting put there from the function that you use to define $slug $slug = single_tag_title(); My crystal ball is at the shop right now getting its divinator replaced so I am unable to tell you what problem exists in that function. If the NULL is at the end of $fullName, then that value is getting put into the database. I have no what processes you are running to put data into the database to tell you why that may be happening